This directory contains data needed by Bison.
# Directory content
## Skeletons
Bison skeletons: the general shapes of the different parser kinds, that are
specialized for specific grammars by the bison program.
Currently, the supported skeletons are:
- yacc.c
It used to be named bison.simple: it corresponds to C Yacc
compatible LALR(1) parsers.
- lalr1.cc
Produces a C++ parser class.
- lalr1.java
Produces a Java parser class.
- glr.c
A Generalized LR C parser based on Bison's LALR(1) tables.
- glr.cc
A Generalized LR C++ parser. Actually a C++ wrapper around glr.c.
These skeletons are the only ones supported by the Bison team. Because the
interface between skeletons and the bison program is not finished, *we are
not bound to it*. In particular, Bison is not mature enough for us to
consider that "foreign skeletons" are supported.
## m4sugar
This directory contains M4sugar, sort of an extended library for M4, which
is used by Bison to instantiate the skeletons.
## xslt
This directory contains XSLT programs that transform Bison's XML output into
various formats.
- bison.xsl
A library of routines used by the other XSLT programs.
- xml2dot.xsl
Conversion into GraphViz's dot format.
- xml2text.xsl
Conversion into text.
- xml2xhtml.xsl
Conversion into XHTML.
# Implementation note about the skeletons
"Skeleton" in Bison parlance means "backend": a skeleton is fed by the bison
executable with LR tables, facts about the symbols, etc. and they generate
the output (say parser.cc, parser.hh, location.hh, etc.). They are only in
charge of generating the parser and its auxiliary files, they do not
generate the XML output, the parser.output reports, nor the graphical
rendering.
The bits of information passing from bison to the backend is named
"muscles". Muscles are passed to M4 via its standard input: it's a set of
m4 definitions. To see them, use `--trace=muscles`.
Except for muscles, whose names are generated by bison, the skeletons have
no constraint at all on the macro names: there is no technical/theoretical
limitation, as long as you generate the output, you can do what you want.
However, of course, that would be a bad idea if, say, the C and C++
skeletons used different approaches and had completely different
implementations. That would be a maintenance nightmare.
Below, we document some of the macros that we use in several of the
skeletons. If you are to write a new skeleton, please, implement them for
your language. Overall, be sure to follow the same patterns as the existing
skeletons.
## Symbols
### `b4_symbol(NUM, FIELD)`
In order to unify the handling of the various aspects of symbols (tag, type
name, whether terminal, etc.), bison.exe defines one macro per (token,
field), where field can `has_id`, `id`, etc.: see
`prepare_symbols_definitions()` in `src/output.c`.
The macro `b4_symbol(NUM, FIELD)` gives access to the following FIELDS:
- `has_id`: 0 or 1
Whether the symbol has an id.
- `id`: string
If has_id, the id (prefixed by api.token.prefix if defined), otherwise
defined as empty. Guaranteed to be usable as a C identifier. This is
used to define the token kind (i.e., the enum used by the return value of
yylex).
- `tag`: string
A human representation of the symbol. Can be 'foo', 'foo.id', '"foo"' etc.
- `user_number`: integer
The code associated to the `id`.
The external number as used by yylex. Can be ASCII code when a character,
some number chosen by bison, or some user number in the case of
%token FOO <NUM>. Corresponds to yychar in yacc.c.
- `is_token`: 0 or 1
Whether this is a terminal symbol.
- `kind`: string
The symbol kind, i.e., the enumerator of this symbol (token or nonterminal)
which is mapping to its `number`.
- `number`: integer
The code associated to the `kind`.
The internal number (computed from the external number by yytranslate).
Corresponds to yytoken in yacc.c. This is the same number that serves as
key in b4_symbol(NUM, FIELD).
In bison, symbols are first assigned increasing numbers in order of
appearance (but tokens first, then nterms). After grammar reduction,
unused nterms are then renumbered to appear last (i.e., first tokens, then
used nterms and finally unused nterms). This final number NUM is the one
contained in this field, and it is the one used as key in `b4_symbol(NUM,
FIELD)`.
The code of the rule actions, however, is emitted before we know what
symbols are unused, so they use the original numbers. To avoid confusion,
they actually use "orig NUM" instead of just "NUM". bison also emits
definitions for `b4_symbol(orig NUM, number)` that map from original
numbers to the new ones. `b4_symbol` actually resolves `orig NUM` in the
other case, i.e., `b4_symbol(orig 42, tag)` would return the tag of the
symbols whose original number was 42.
- `has_type`: 0, 1
Whether has a semantic value.
- `type_tag`: string
When api.value.type=union, the generated name for the union member.
yytype_INT etc. for symbols that has_id, otherwise yytype_1 etc.
- `type`
If it has a semantic value, its type tag, or, if variant are used,
its type.
In the case of api.value.type=union, type is the real type (e.g. int).
- `has_printer`: 0, 1
- `printer`: string
- `printer_file`: string
- `printer_line`: integer
- `printer_loc`: location
If the symbol has a printer, everything about it.
- `has_destructor`, `destructor`, `destructor_file`, `destructor_line`, `destructor_loc`
Likewise.
### `b4_symbol_value(VAL, [SYMBOL-NUM], [TYPE-TAG])`
Expansion of $$, $1, $<TYPE-TAG>3, etc.
The semantic value from a given VAL.
- `VAL`: some semantic value storage (typically a union). e.g., `yylval`
- `SYMBOL-NUM`: the symbol number from which we extract the type tag.
- `TYPE-TAG`, the user forced the `<TYPE-TAG>`.
The result can be used safely, it is put in parens to avoid nasty precedence
issues.
### `b4_lhs_value(SYMBOL-NUM, [TYPE])`
Expansion of `$$` or `$<TYPE>$`, for symbol `SYMBOL-NUM`.
### `b4_rhs_data(RULE-LENGTH, POS)`
The data corresponding to the symbol `#POS`, where the current rule has
`RULE-LENGTH` symbols on RHS.
### `b4_rhs_value(RULE-LENGTH, POS, SYMBOL-NUM, [TYPE])`
Expansion of `$<TYPE>POS`, where the current rule has `RULE-LENGTH` symbols
on RHS.
-----
Local Variables:
mode: markdown
fill-column: 76
ispell-dictionary: "american"
End:
Copyright (C) 2002, 2008-2015, 2018-2020 Free Software Foundation, Inc.
This file is part of GNU Bison.
This program is free software: you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program. If not, see <http://www.gnu.org/licenses/>.
没有合适的资源?快使用搜索试试~ 我知道了~
bison-3.6.3.tar.gz
需积分: 1 0 下载量 95 浏览量
2024-01-30
00:39:49
上传
评论
收藏 4.42MB GZ 举报
温馨提示
Bison 是一个由 GNU 项目开发的解析器生成器,通常用于将语法描述转换成一个 C 程序。它是 Yacc(Yet Another Compiler Compiler)的一个自由软件替代品,广泛用于编译器和解释器的开发中,尤其是在处理复杂的语法和编程语言设计时。开发者和系统管理员可以下载这个文件,解压并编译源代码,从而在他们的系统上安装或更新 Bison。Bison 主要被用于开发那些需要解析复杂文本数据的程序,如编译器、解释器和配置文件处理器。它提供了一种灵活而强大的方式来描述和处理语法结构,使开发者能够构建出高效且可靠的解析器。
资源推荐
资源详情
资源评论
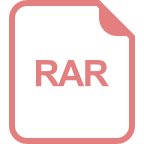
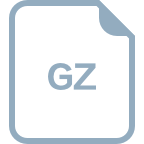
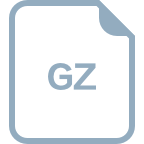
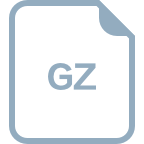
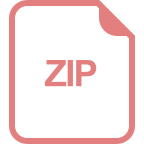
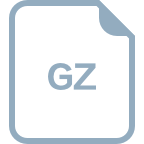
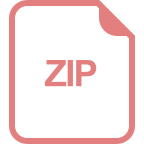
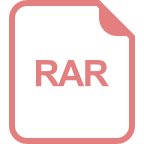
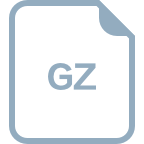
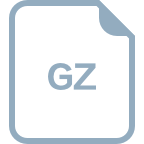
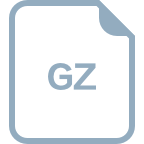
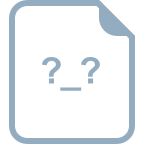
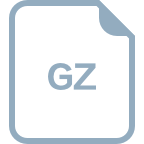
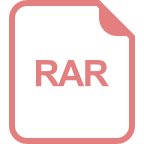
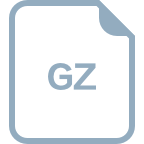
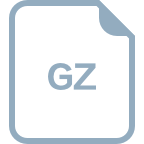
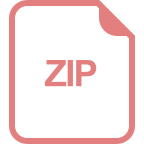
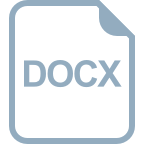
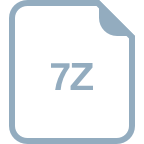
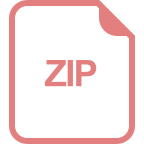
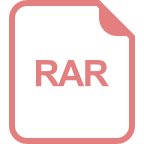
收起资源包目录

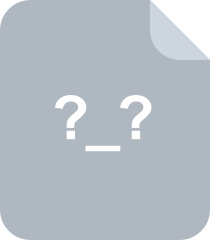
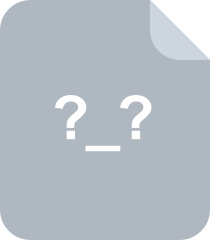
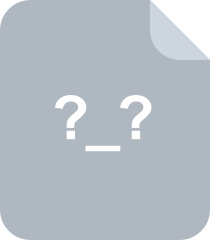
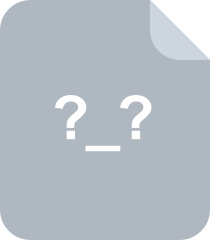
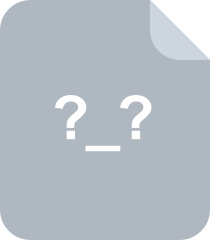
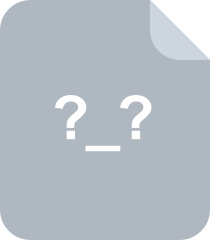
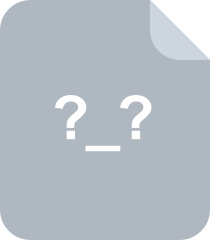
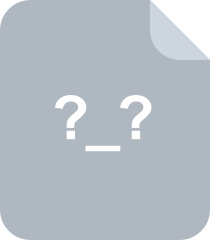
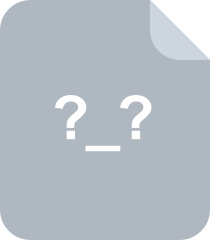
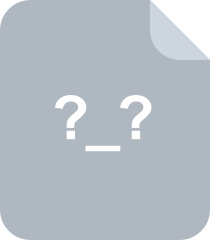
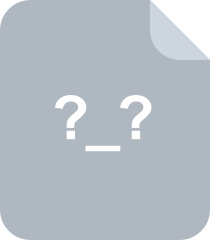
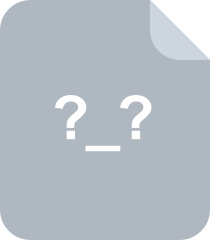
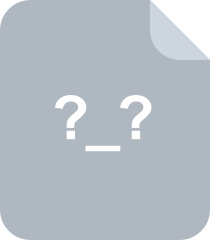
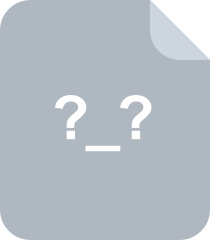
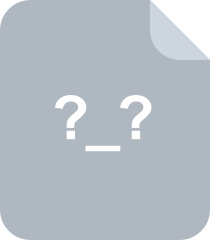
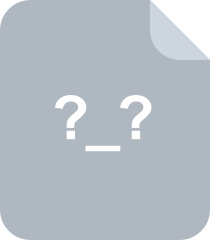
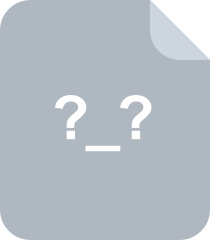
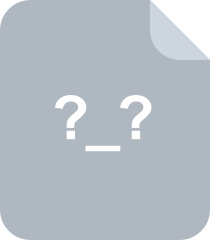
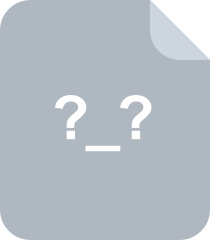
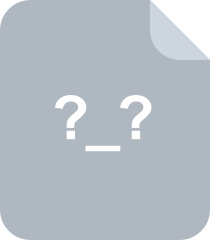
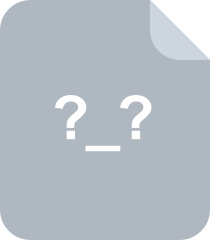
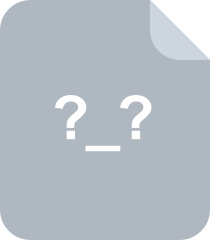
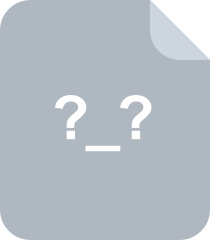
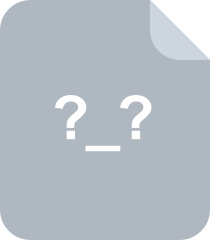
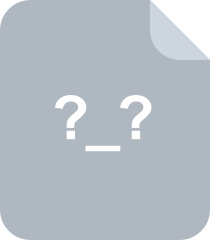
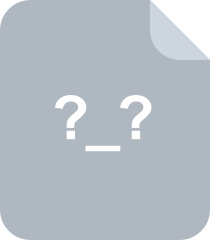
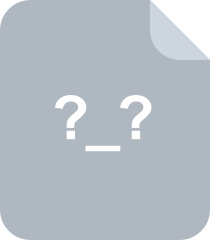
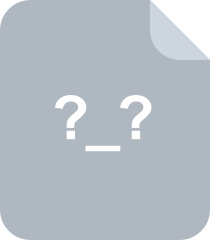
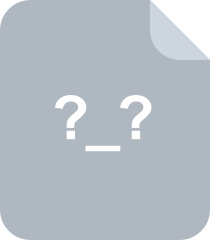
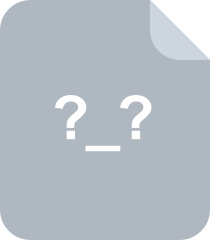
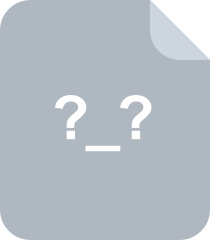
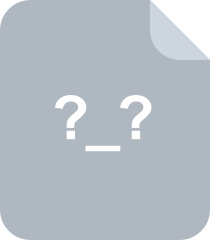
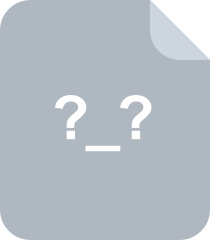
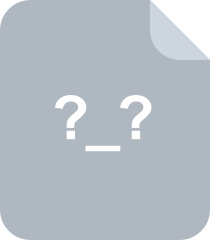
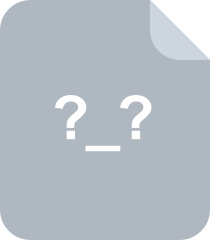
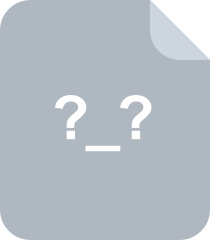
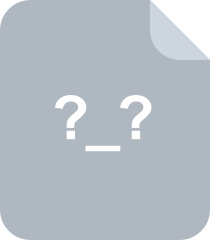
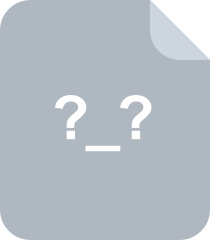
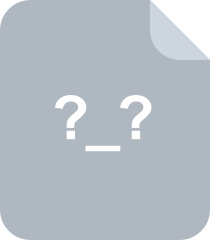
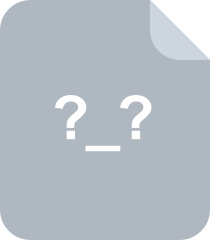
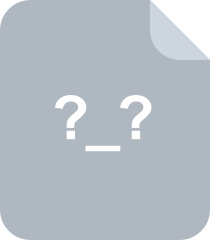
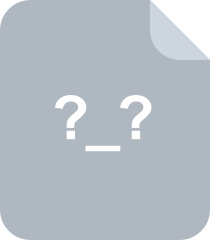
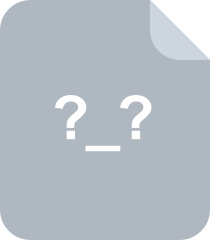
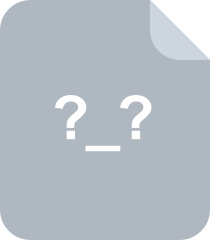
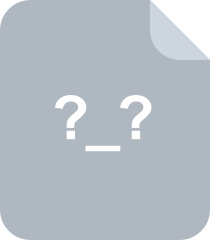
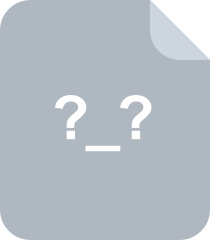
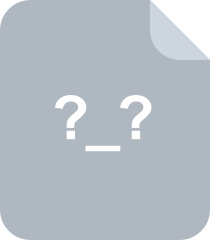
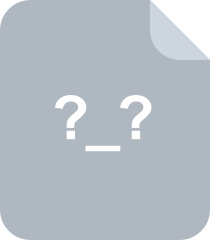
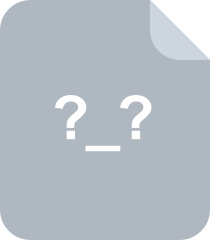
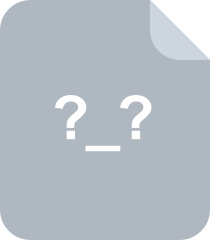
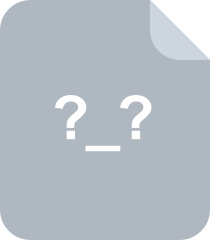
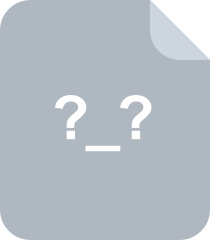
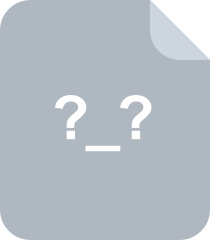
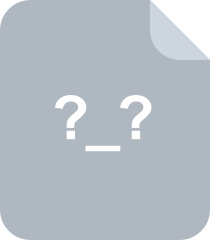
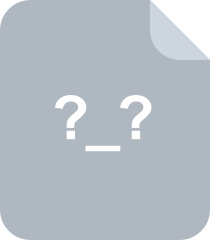
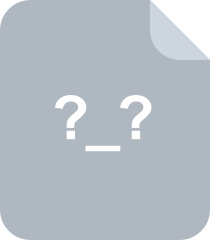
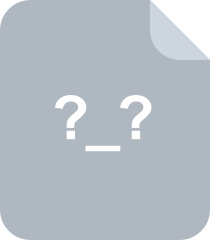
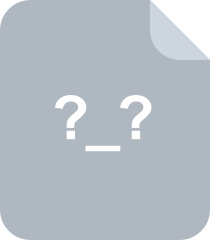
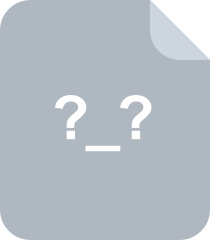
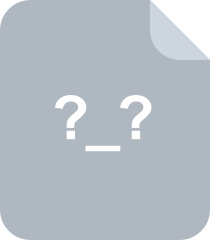
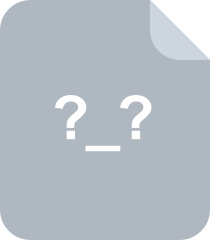
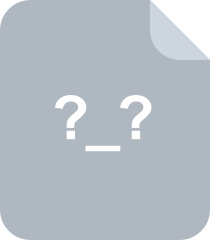
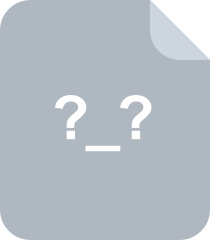
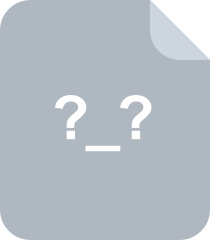
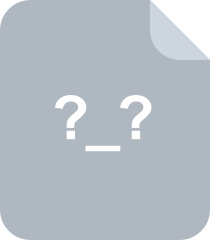
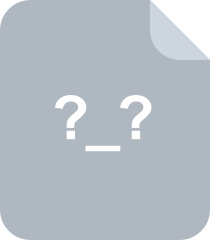
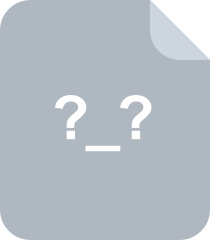
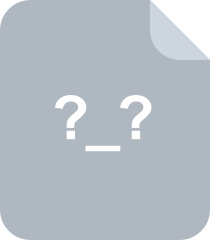
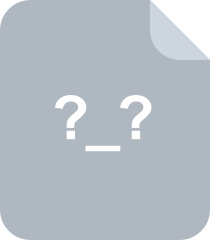
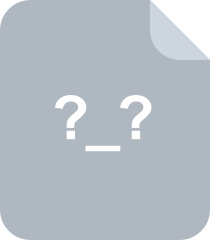
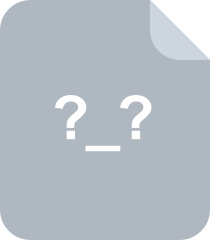
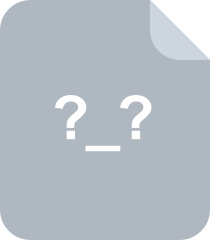
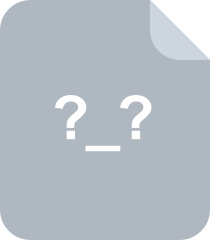
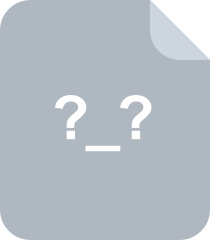
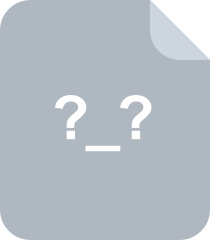
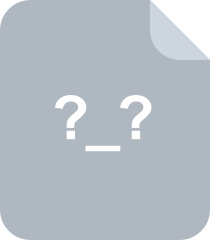
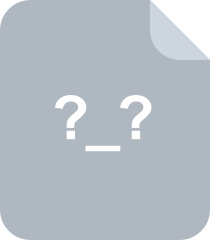
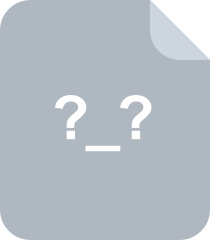
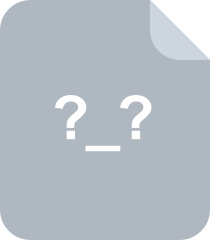
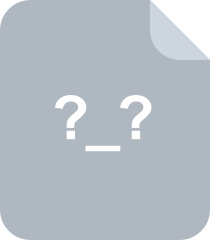
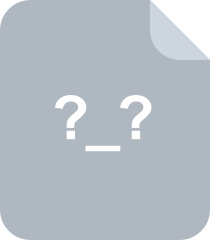
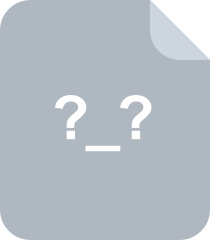
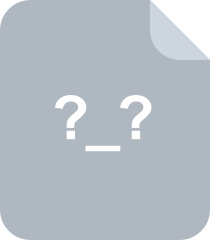
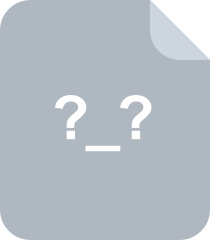
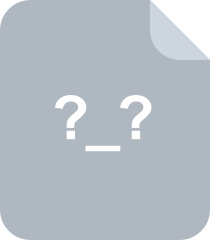
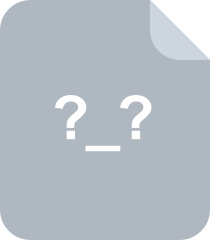
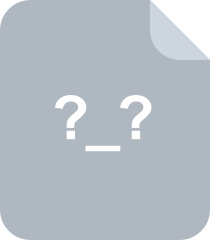
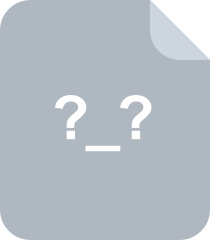
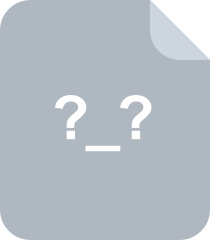
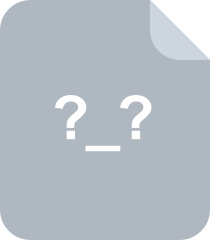
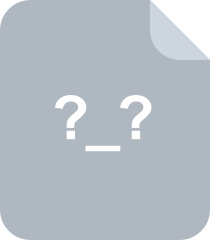
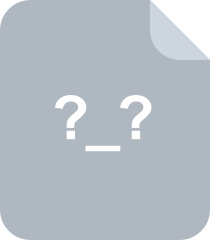
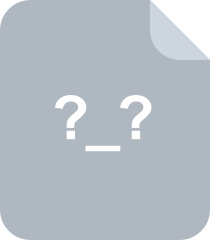
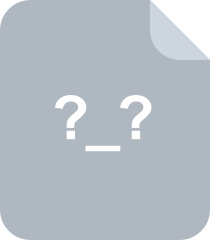
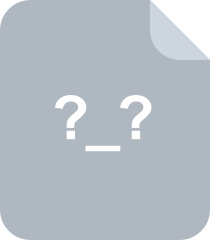
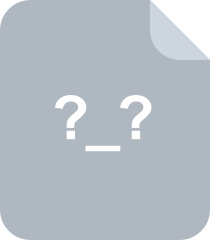
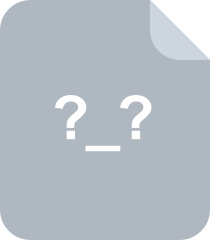
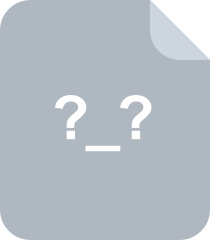
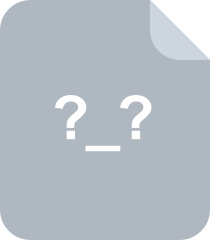
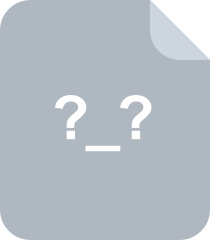
共 1136 条
- 1
- 2
- 3
- 4
- 5
- 6
- 12
资源评论
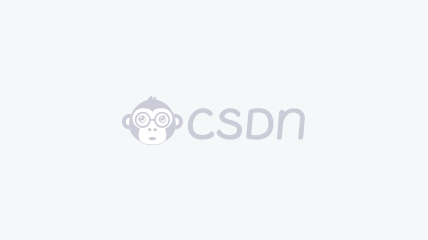

程序员Chino的日记
- 粉丝: 3644
- 资源: 5万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

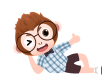
最新资源
- 【Unity尾巴动画插件】Tail Animator 轻松创建和控制角色的尾巴动画
- RabbitMQ 延时执行的功能插件
- Java数组反转技巧:保持元素原始类型与代码实现
- 【Unity纹理生成和材料编辑工具】Surforge
- 【日常办公必须工具】文件管理+批量移动文件+实用工具+软件开发+windows必备
- 基于stm32的六轴机械臂控制+openmv颜色识别-识别不同的物块分放(源码+文档说明)
- 深入浅出Pandas:利用Python进行数据处理与分析 (李庆辉)
- 【Unity烟雾特效插件】VFX Graph - Stylized Smoke - Vol. 1 高质量的烟雾特效
- 2019-灵活就业数据集.dta
- 2017-灵活就业数据集.dta
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


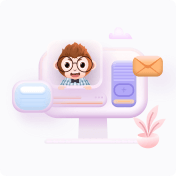
安全验证
文档复制为VIP权益,开通VIP直接复制
