#include<iostream>
#include<string>
#include<fstream>
using namespace std;
#define NULL 0
int n=0;
class student
{private:
long xuehao;
string mingzhi;
char xingbie;
int shuoxue;
int yingyu;
int chengxu;
int PE;
int suzhi;
int qidaishi;
int sum;
float ave;
student *next;
public:
long getxuehao();
string getmingzhi();
char getxingbie();
int getshuoxue();
int getyingyu();
int getchengxu();
int getPE();
int getsuzhi();
int getqidaishi();
int getsum();
float getave();
void save(student *);
void Output(student*);
void welcome();
void exchange(student *p1,student *p2);
student *Creat();
student *dele(student *head);
student *Lookup(student *head);
student *total_ave(student *head);
student *paixu(student *head);
student *Modify(student *head);
student *load(void);
student *chakan(student *head);
student *ave_search(student *head);
student *sum_search(student *head);
student *chakanyouxiu(student *p);
};
long student::getxuehao()
{return xuehao;}
string student::getmingzhi()
{return mingzhi;}
char student::getxingbie()
{return xingbie;}
int student::getshuoxue()
{return shuoxue;}
int student::getyingyu()
{return yingyu;}
int student::getchengxu()
{return chengxu;}
int student::getPE()
{return PE;}
int student::getshuozhi()
{return shuozhi;}
int student::getqidaishi()
{return qidaishi;}
int student::getsum()
{return sum;}
float student::getave()
{return ave;}
void student::save(student *p1)
{
ofstream outfile("信息文件.dat",ios::out);
if(!outfile)
{
char a;
cerr<<"打开文件失败!"<<endl;
cin>>a;
exit(1);
}
while(p1!=0)
{
outfile<<p1->getxuehao()<<" ";
outfile<<p1->getmingzhi()<<" ";
outfile<<p1->getxingbie()<<" ";
outfile<<p1->getshuoxue()<<" ";
outfile<<p1->getyingyu()<<" ";
outfile<<p1->getchengxu()<<" ";
outfile<<p1->getPE()<<" ";
outfile<<p1->getsuzhi()<<" ";
outfile<<p1->getqidaishi()<<" ";
p1=p1->next;
}
outfile.close();
cout<<"已保存!"<<endl;
cout<<"回主页单请按0 "<<endl;
}
void student::Output(student *head)
{
student *p;
cout<<"学生信息为:"<<endl;
cout<<"学号 "<<"姓名 "<<"性别 "<<"高数 "<<"英语 "
<<"程序 "<<"大学体育 "<<"数字逻辑 "<<"近代史 "<<"总分 "<<"平均分 "<<endl;
p=head;
if(head!=NULL)
do
{cout<<p->getxuehao()<<" "<<p->getmingzhi()<<" "<<p->getxingbie()<<" "<<p->getshuoxue()
<<" "<<p->getyingyu()<<" "<<p->getchengxu()<<" "<<p->getPE()
<<" "<<p->getsuzhi()<<" "<<p->getqidaishi()<<" "<<p->getsum()<<" "<<p->getave()<<endl;
p=p->next;
}
while(p!=NULL);
}
void student::welcome()
{
cout<<endl<<endl;
cout<<endl<<endl;
cout<<" "<<endl;
cout<<" "<<endl;
cout<<" 我的成绩管理系统 "<<endl;
cout<<" "<<endl;
cout<<" 1 写入 2 显示 3 清除 4 查询 5 排序 "<<endl;
cout<<" "<<endl;
cout<<" 6 修改 7 存盘 8 查看 9 输出 "<<endl;
cout<<" "<<endl;
cout<<" "<<endl;
cout<<" 请选项:";
cout<<" "<<endl;
}
student *student::Creat(void)
{
student *head=NULL;
student *p1,*p2;
char t='y';
p1=p2=new student;
cout<<"欢迎进入学生信息建立系统!"<<endl;
while(t=='y')
{
cout<<"学号"<<" "<<"姓名"<<" "<<"性别"<<" "<<"高数"
<<" "<<"英语"<<" "<<"程序"<<" "<<"大学体育"<<" "<<"数字逻辑"<<" "<<"近代史"<<endl;
cin>>p1->getxuehao()>>p1->getmingzhi()>>p1->getxingbie()>>p1->getshuoxue()>>p1->getyingyu()>>p1->getchengxu()>>p1->getPE()>>p1->getsuzhi()>>p1->getqidaishi();
n=n+1;
if(n==1)head=p1;
p2->next=p1;
p2=p1;
cout<<"请选择是否继续输入学生信息(y/n)?";
cin>>t;
if(t=='y')
p1=new student;
continue;
else if(t=='n')break;
}
p2->next=NULL;
head=total_ave(head);
cout<<"回主页请按0。"<<endl;
return(head);
}
//清除
student *student::dele(student *head)
{
student *p1,*p2;
int xuehao;
char t='y';
cout<<"欢迎进入信息删除系统!"<<endl;
while(t=='y')
{
cout<<"请输入要删除的学生的学号:";
cin>>xuehao;
p1=head;
while(xuehao!=p1->xuehao&&p1->next!=NULL)
{
p2=p1;p1=p1->next;
}
if(xuehao==p1->getxuehao())
{
if(p1==head) head=p1->next;
else p2->next=p1->next;
cout<<"已删除:"<<getxuehao()<<endl;
}
cout<<"是否继续删除其他学生信息(y/n)?:";
cin>>t;
if(t=='y')continue;
else if(t=='n')break;
}
return(head);
cout<<"回主页请按0。"<<endl;
}
// 查询
student *student::Lookup(student *head)
{
long getxuehao();
stu *p1,*p2;
char t='y';
cout<<"欢迎进入查询系统!"<<endl;
while(t=='y')
{
cout<<"请输入要查询的学生的学号:";
cin>>xuehao;
p1=head;
while(getxuehao()!=p1->getxuehao()&&p1->next!=NULL)
{
p2=p1;p1=p1->next;
}
if(getxuehao()==p1->getxuehao())
cout<<"该学生的信息为:"<<endl<<"高数:"<<p1->getshuoxue()<<"\t"
<<"英语:"<<p1->getyinyu()<<"\t"<<"程序:"<<p1->getchengxu()<<"\t"
<<"大学体育:"<<p1->getPE()<<"\t"<<"数字逻辑:"<<p1->getsuzhi()<<"近代史:"<<p1->getqidaishi()<<endl;
cout<<"是否继续查询其他信息(y/n)?:";
cin>>t;
if(t=='y')continue;
else if(t=='n')break;
}
return(head);
cout<<"回主页请按0。"<<endl;
}
// 总成绩 平均成绩
student *student::total_ave(student *head)
{
student *p;
p=head;
if(p->getxuehao()==NULL)
cout<<"这是个空表"<<endl;
while(p->next!=NULL)
{
p->getsum()=p->getshuoxue()+p->getyingyu()+p->getchengxu()+p->getPE()+p->getsuzhi()+p->getqidaishi();
p->getave()=p->getxuehao()/6;
p=p->next;
}
p->getsum()=p->getshuoxue()+p->getyingyu()+p->getchengxu()+p->getPE()+p->getsuzhi()+p->getqidaishi();
p->getave()=p->getxuehao()/6;
return(head);
}
// 交换
void student::exchange(student *p1,student *p2)
{
int s;
string h;
s=p1->getshuoxue();p1->getshuoxue()=p2->getshuoxue();p2->getshuoxue()=s;
s=p1->getxuehao();p1->getxuehao()=p2->getxuehao();p2->getxuehao()=s;
h=p1->getmingzhi();p1->getmingzhi()=p2->getmingzhi();p2->getmingzhi()=h;
s=p1->getxingbie();p1->getxingbiee()=p2->getxingbie();p2->getxingbie()=s;
s=p1->getyingyu(); p1->getyingyu()=p2->getyingyu();p2->getyingyu()=s;
s=p1->getchengxu();p1->getchengxu()=p2->getchengxu();p2->getchengxu()=s;
s=p1->getPE();p1->getPE()=p2->getPE();p2->getPE()=s;
s=p1->getsuzhi();p1->getsuzhi()=p2->getsuzhi();p2->getsuzhi()=s;
s=p1->getqidaishi();p1->getqidaishi()=p2->getqidaishi();p2->getqidaishi()=s;
s=p1->getxuehao(); p1->getxuehao()=p2->getxuehao();p2->getxuehao()=s;
s=p1->getave(); p1->getave()=p2->getave(); p2->getave()=s;
}
student *student::paixu(student *head)
{
student *p1,*p2;
p1=head;
p2=p1->next;
while(p1->next!=NULL)
{
while(p2->next!=NULL)
{
if(p1->getxuehao()<p2->getxuehao())
exchange(p1,p2);
p2=p2->next;
}
p1=p1->next;
}
p2=head;
while(p2->next!=NULL)
{
if(p2->getxuehao()<p1->getxuehao())
exchange(p1,p2);
p2=p2->next;
}
cout<<"按总分排列如下:"<<endl;
Output(head);
return(head);
cout<<"回主页请按0。"<<endl;
}
student *student::Modify(studebt *head)
{
long getxuehao();
char t='y';
student *p1,*p2;
cout<<"欢迎进入信息修改系统!"<<endl;
while(t=='y')
{
cout<<"请输出要修改的学生的学号:";
cin>>xuehao;
p1=head;
while(getxuehao()!=p1->getxuehao()&&p1->next!=NULL)
{p2=p1;p1=p1->next;}
if(getxuehao()==p1->getxuehao())
cout<<"该学生的信息为:"<<"高数:"<<p1->getshuoxue()<<"\t"
<<"英语:"<<p1->getyingyu()<<"\t"<<"程序:"<<p1->getchengxu()<<"\t"
<<"大学体育:"<<p1->getPE()<<"\t"<<"数字逻辑 :"<<p1->getsuzhi()<<"\t"<<"近代史:"<<p1->getqidaishi()<<endl;
cout<<"是否修改(y/n)?";
cin>>t;
cout<<"学号 "<<" "<<"姓名 "<<" "<<"性别 "<<" "
<<"高数 "<<" "<<"英语 "<<" "<<"
没有合适的资源?快使用搜索试试~ 我知道了~
学生成绩管理系统课程设计
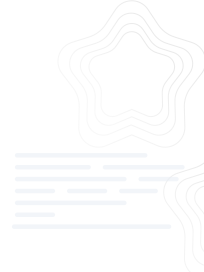
共80个文件
opt:10个
txt:8个
pdb:8个

需积分: 0 25 下载量 24 浏览量
2008-12-04
17:01:49
上传
评论
收藏 4.27MB RAR 举报
温馨提示
有完整的程序和算法,简单易懂,利于上手。
资源详情
资源评论
资源推荐
收起资源包目录



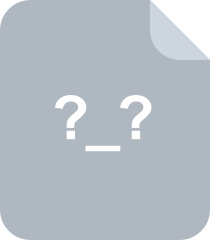
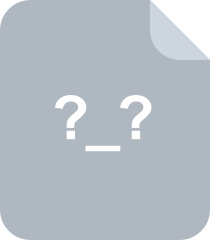

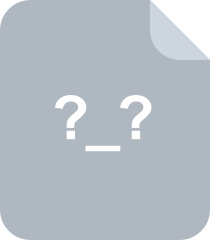
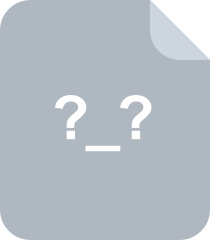
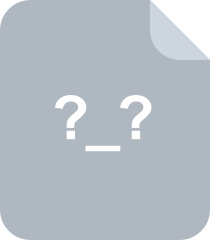
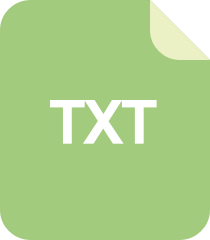
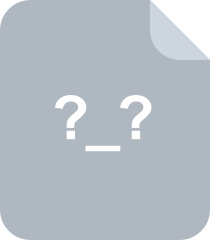
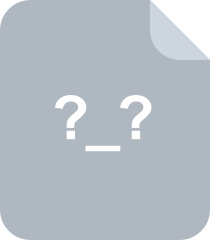
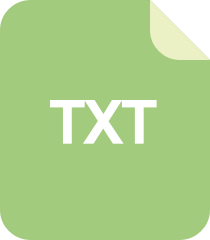
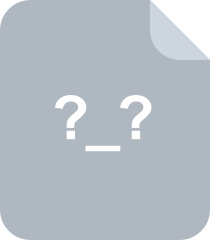
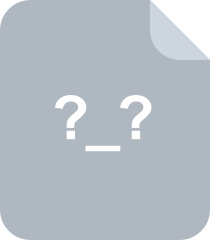

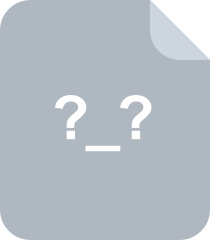
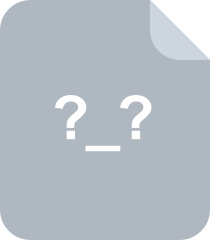
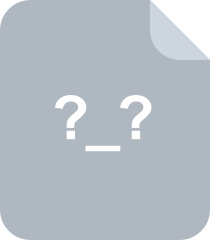
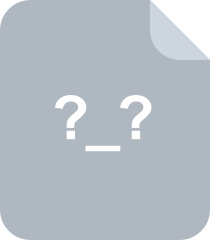
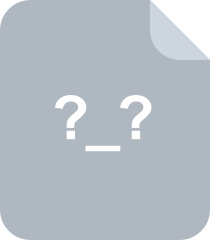
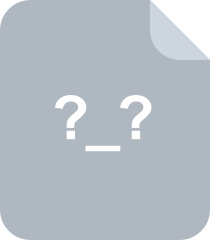
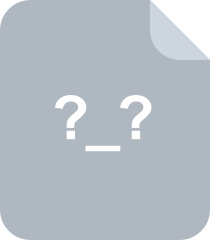
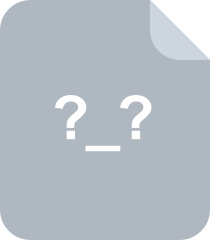
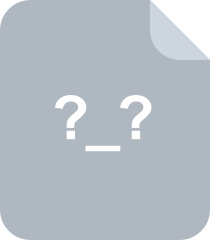
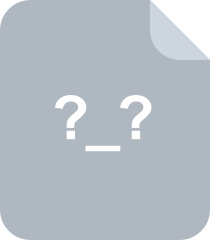
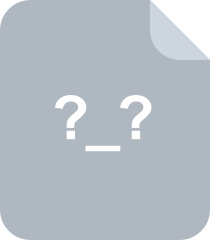
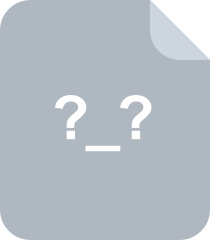
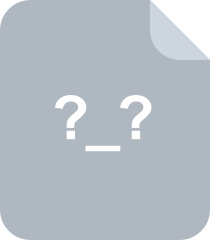
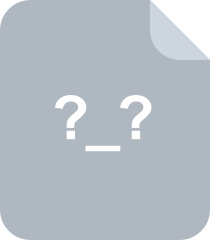
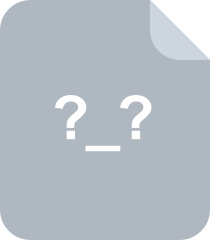
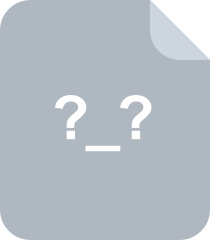
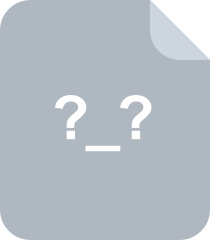
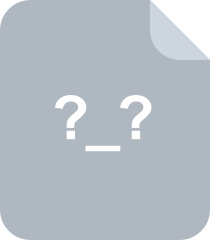
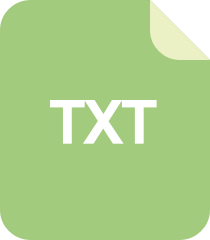
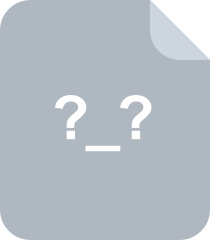
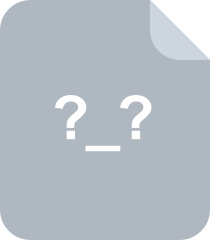
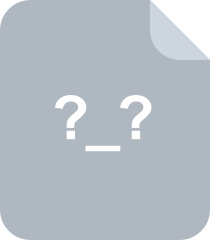
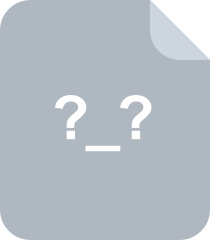
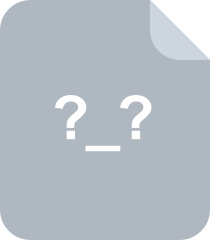
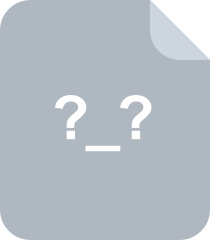

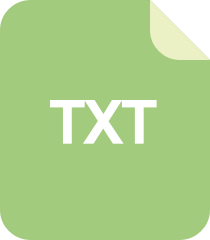
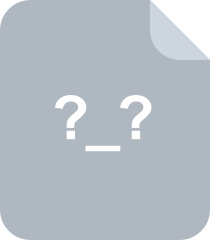
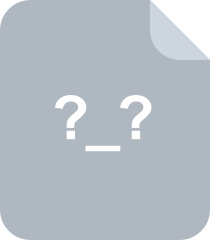
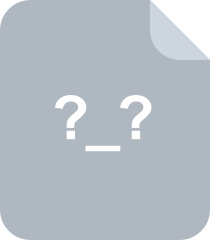


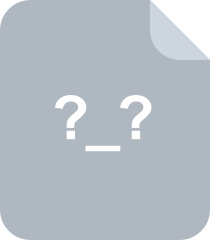
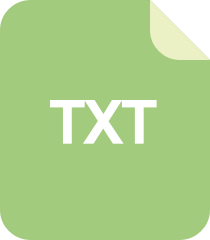
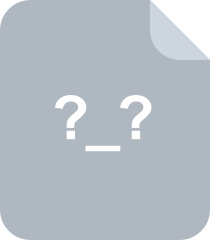

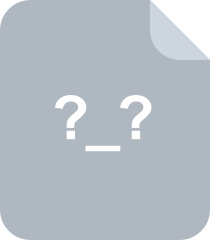
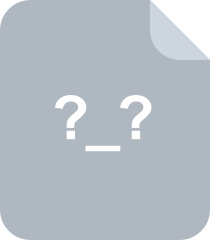
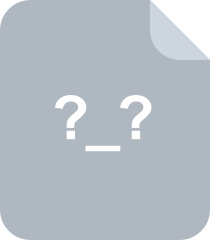
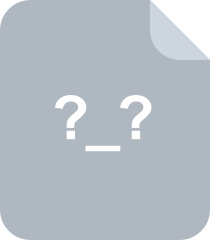

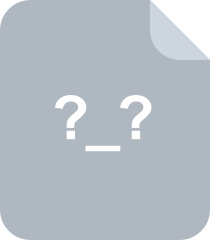
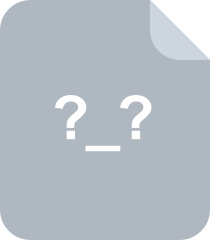
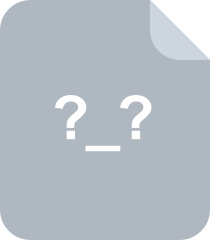
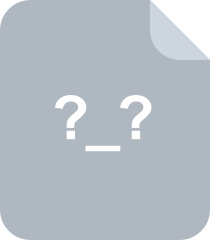
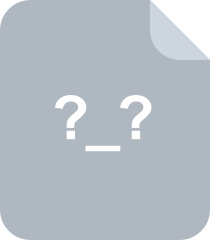
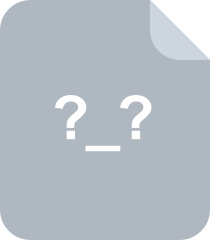
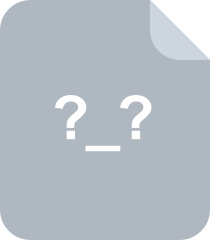
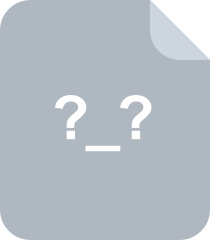
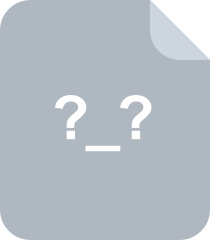
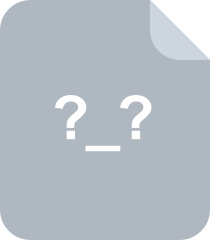
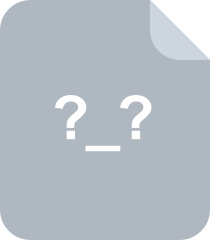
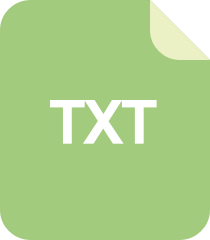
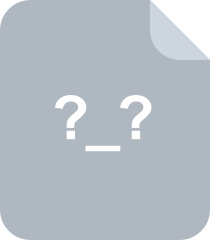
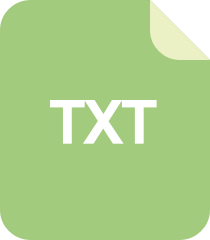
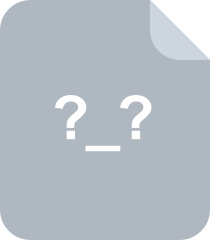
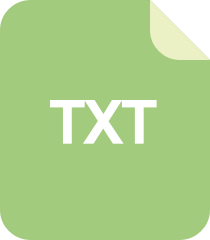
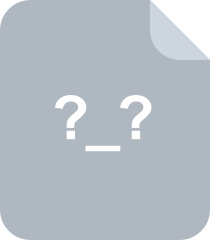
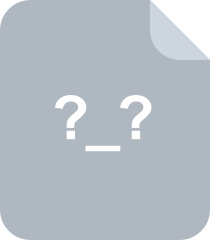

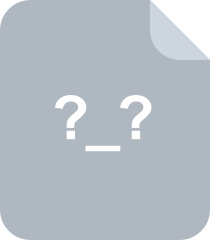
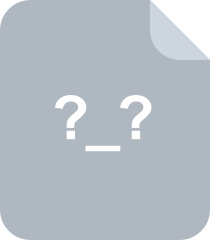
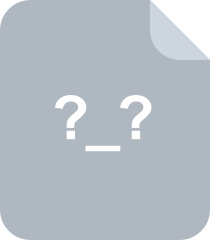
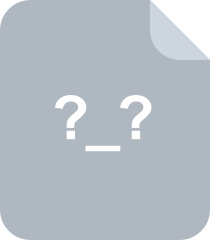

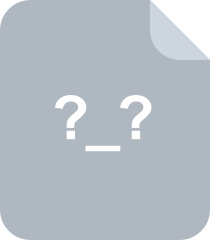
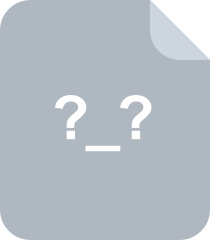
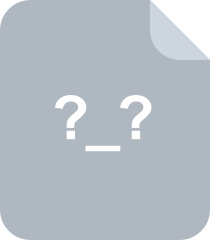
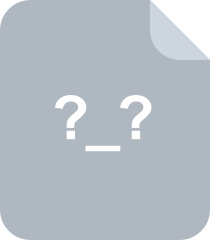
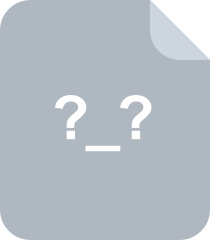
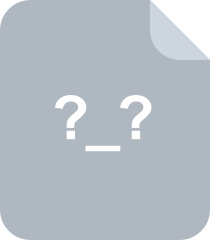
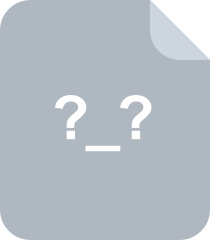
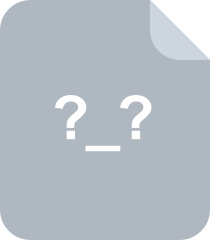
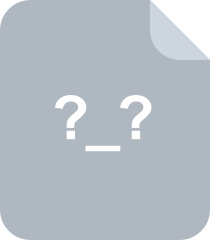
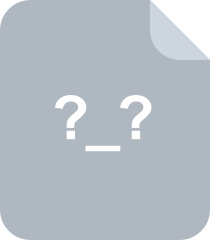
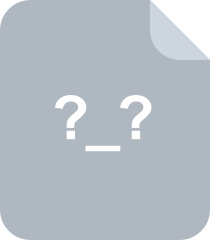
共 80 条
- 1
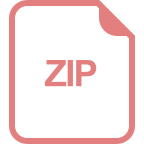
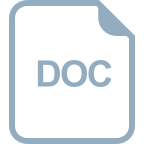
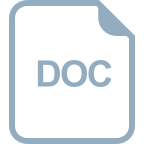
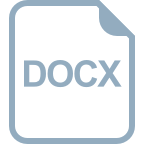
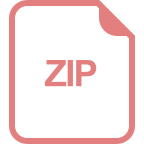
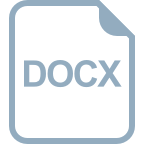
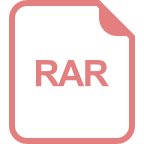
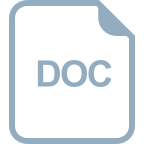
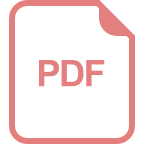
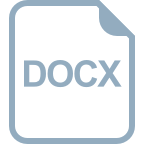
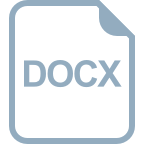
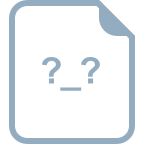
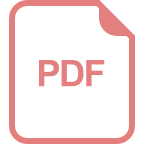
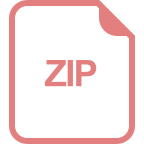
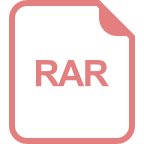
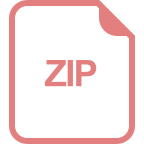
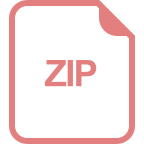
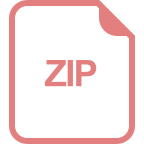
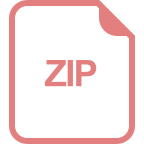
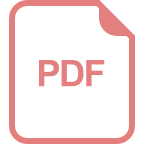
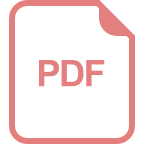
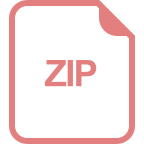

caili_yt
- 粉丝: 0
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

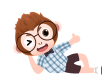
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0