《CAS集成手册(Java版)》是一份详细指导文档,主要针对CAS(Central Authentication Service,中央认证服务)在Java环境下的集成与优化。CAS是一种开放源码的单点登录(SSO)系统,旨在简化用户身份验证过程,提高安全性,并减少用户在多个应用间反复登录的繁琐操作。 SSO(Single Sign-On)是现代企业信息化建设中不可或缺的一环,它允许用户在一个应用系统中登录后,无须再次验证即可访问其他关联的应用系统。CAS作为SSO的一种实现,因其开源、跨平台和易于集成的特点,在业界广泛应用。 在Java环境下集成CAS,主要涉及以下几个核心组件: 1. **CAS客户端**:如`platform-cas-client-0.0.1-SNAPSHOT.jar`,这是用于与CAS服务器通信的库,负责处理用户的认证请求和票证验证。它提供了一系列API,使得开发者可以方便地在Java应用中集成CAS服务。 2. **数据模型**:`platform-datamodel-0.0.1-SNAPSHOT.jar`可能包含了应用与CAS交互所需的数据结构和对象模型,例如用户信息、票证状态等。 3. **缓存机制**:`platform-cache-0.0.1-SNAPSHOT.jar`可能是用于存储和检索认证票证(TGT,Ticket Granting Ticket)和会话票证(ST,Service Ticket)的缓存模块,提高系统性能。 4. **配置管理**:`platform-configuration-0.0.1-SNAPSHOT.jar`可能包含CAS客户端的配置类和配置文件,用于设定CAS服务器地址、端口、安全策略等参数。 5. **工具类库**:`platform-utils-0.0.1-SNAPSHOT.jar`包含了一些通用工具函数,可能用于URL解析、加密解密、HTTP请求等操作。 6. **控制器**:`HelloController.java`是Spring MVC中的控制器,处理来自前端的请求,可能是SSO登录逻辑的一部分。 7. **页面跳转**:`nologin.jsp`可能是未登录用户的默认页面,当用户未通过SSO验证时,会被重定向到这个页面。 8. **部署配置**:`web.xml`是Web应用的部署描述符,其中会包含CAS客户端的过滤器和监听器配置,用于拦截用户请求并进行SSO处理。 通过以上组件的协同工作,CAS能够实现高效的身份验证和授权,提升用户体验,同时确保系统安全。对于Java开发者来说,理解和掌握这些组件的工作原理以及如何配置和使用它们,是成功集成CAS的关键。在实际应用中,还应关注性能优化,如调整缓存策略,减少网络延迟,以达到"提速N倍"的效果。此外,由于CAS支持多种语言集成(如PHP、.NET),因此具备良好的可扩展性,能适应各种复杂的企业架构需求。
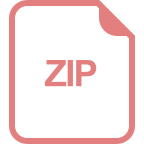
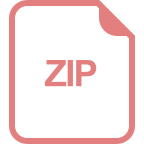
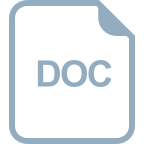
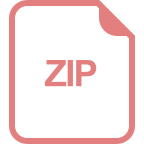
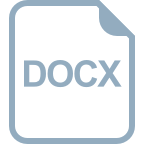
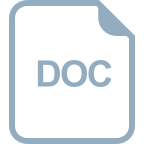
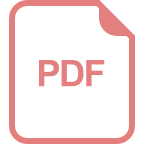
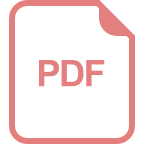
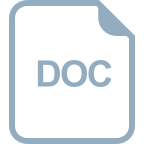
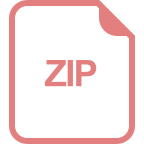
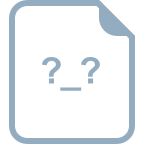
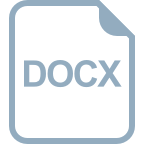
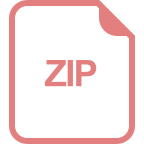
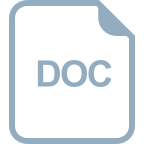
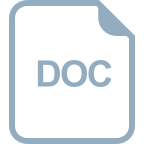
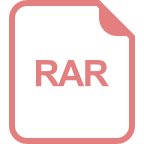
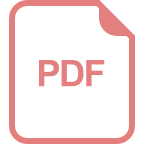
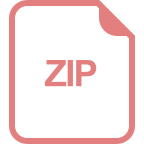
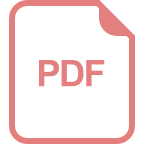
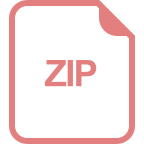

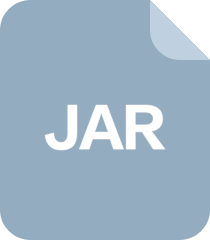
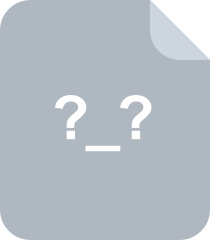
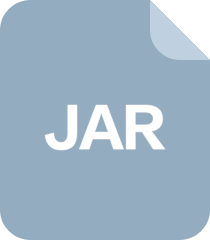
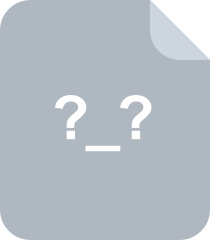
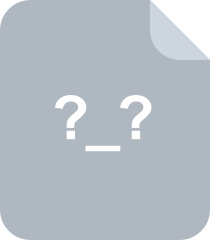
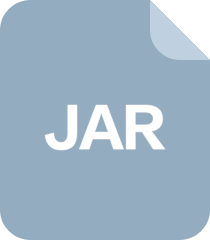
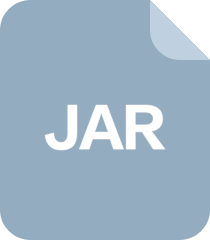
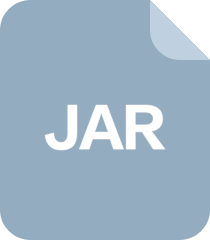
- 1
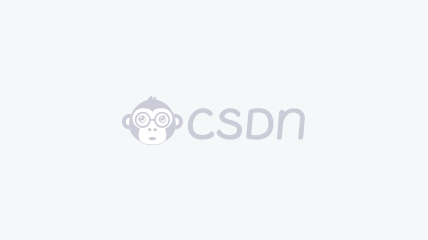

- 粉丝: 37
- 资源: 56
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

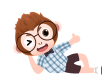
最新资源
- (源码)基于Arduino和Python的实时歌曲信息液晶显示屏展示系统.zip
- (源码)基于C++和C混合模式的操作系统开发项目.zip
- (源码)基于Arduino的全球天气监控系统.zip
- OpenCVForUnity2.6.0.unitypackage
- (源码)基于SimPy和贝叶斯优化的流程仿真系统.zip
- (源码)基于Java Web的个人信息管理系统.zip
- (源码)基于C++和OTL4的PostgreSQL数据库连接系统.zip
- (源码)基于ESP32和AWS IoT Core的室内温湿度监测系统.zip
- (源码)基于Arduino的I2C协议交通灯模拟系统.zip
- coco.names 文件

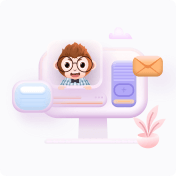
