1. MySQL Connector/J
_______________________________________________________
MySQL provides connectivity for client applications developed in
the Java programming language via a JDBC driver, which is called
MySQL Connector/J.
MySQL Connector/J is a JDBC-3.0 "Type 4" driver, which means that
is pure Java, implements version 3.0 of the JDBC specification,
and communicates directly with the MySQL server using the MySQL
protocol.
This document is arranged for a beginning JDBC developer. If you
are already experienced with using JDBC, you might consider
starting with the Section A.2, "Installing Connector/J."
Although JDBC is useful by itself, we would hope that if you are
not familiar with JDBC that after reading the first few sections
of this manual, that you would avoid using "naked" JDBC for all
but the most trivial problems and consider using one of the
popular persistence frameworks such as Hibernate
(http://www.hibernate.org/), Spring's JDBC templates
(http://www.springframework.org/) or Ibatis SQL Maps
(http://ibatis.apache.org/) to do the majority of repetitive work
and heavier lifting that is sometimes required with JDBC.
This section is not designed to be a complete JDBC tutorial. If
you need more information about using JDBC you might be interested
in the following online tutorials that are more in-depth than the
information presented here:
* JDBC Basics
(http://java.sun.com/docs/books/tutorial/jdbc/basics/index.htm
l) --- A tutorial from Sun covering beginner topics in JDBC
* JDBC Short Course
(http://java.sun.com/developer/onlineTraining/Database/JDBCSho
rtCourse/index.html) --- A more in-depth tutorial from Sun and
JGuru
1.1. Basic JDBC concepts
This section provides some general JDBC background.
1.1.1. Connecting to MySQL using the DriverManager Interface
When you are using JDBC outside of an application server, the
DriverManager class manages the establishment of Connections.
The DriverManager needs to be told which JDBC drivers it should
try to make Connections with. The easiest way to do this is to use
Class.forName() on the class that implements the java.sql.Driver
interface. With MySQL Connector/J, the name of this class is
com.mysql.jdbc.Driver. With this method, you could use an external
configuration file to supply the driver class name and driver
parameters to use when connecting to a database.
The following section of Java code shows how you might register
MySQL Connector/J from the main() method of your application:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
// Notice, do not import com.mysql.jdbc.*
// or you will have problems!
public class LoadDriver {
public static void main(String[] args) {
try {
// The newInstance() call is a work around for some
// broken Java implementations
Class.forName("com.mysql.jdbc.Driver").newInstance();
} catch (Exception ex) {
// handle the error
}
}
After the driver has been registered with the DriverManager, you
can obtain a Connection instance that is connected to a particular
database by calling DriverManager.getConnection():
Example 1. Obtaining a Connection From the DriverManager
This example shows how you can obtain a Connection instance from
the DriverManager. There are a few different signatures for the
getConnection() method. You should see the API documentation that
comes with your JDK for more specific information on how to use
them.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
... try {
Connection conn = DriverManager.getConnection("jdbc:mysql
://localhost/test?user=monty&password=greatsqldb");
// Do something with the Connection
....
} catch (SQLException ex) {
// handle any errors
System.out.println("SQLException: " + ex.getMessage());
System.out.println("SQLState: " + ex.getSQLState());
System.out.println("VendorError: " + ex.getErrorCode());
}
Once a Connection is established, it can be used to create
Statement and PreparedStatement objects, as well as retrieve
metadata about the database. This is explained in the following
sections.
1.1.2. Using Statements to Execute SQL
Statement objects allow you to execute basic SQL queries and
retrieve the results through the ResultSet class which is
described later.
To create a Statement instance, you call the createStatement()
method on the Connection object you have retrieved via one of the
DriverManager.getConnection() or DataSource.getConnection()
methods described earlier.
Once you have a Statement instance, you can execute a SELECT query
by calling the executeQuery(String) method with the SQL you want
to use.
To update data in the database, use the executeUpdate(String SQL)
method. This method returns the number of rows affected by the
update statement.
If you don't know ahead of time whether the SQL statement will be
a SELECT or an UPDATE/INSERT, then you can use the execute(String
SQL) method. This method will return true if the SQL query was a
SELECT, or false if it was an UPDATE, INSERT, or DELETE statement.
If the statement was a SELECT query, you can retrieve the results
by calling the getResultSet() method. If the statement was an
UPDATE, INSERT, or DELETE statement, you can retrieve the affected
rows count by calling getUpdateCount() on the Statement instance.
Example 2. Using java.sql.Statement to Execute a SELECT Query
// assume that conn is an already created JDBC connection
Statement stmt = null;
ResultSet rs = null;
try {
stmt = conn.createStatement();
rs = stmt.executeQuery("SELECT foo FROM bar");
// or alternatively, if you don't know ahead of time that
// the query will be a SELECT...
if (stmt.execute("SELECT foo FROM bar")) {
rs = stmt.getResultSet();
}
// Now do something with the ResultSet ....
} finally {
// it is a good idea to release
// resources in a finally{} block
// in reverse-order of their creation
// if they are no-longer needed
if (rs != null) {
try {
rs.close();
} catch (SQLException sqlEx) { // ignore }
rs = null;
}
if (stmt != null) {
try {
stmt.close();
} catch (SQLException sqlEx) { // ignore }
stmt = null;
}
}
1.1.3. Using CallableStatements to Execute Stored Procedures
Starting with MySQL server version 5.0 when used with Connector/J
3.1.1 or newer, the java.sql.CallableStatement interface is fully
implemented with the exception of the getParameterMetaData()
method.
MySQL's stored procedure syntax is documented in the "Stored
Procedures and Functions
(http://www.mysql.com/doc/en/stored-procedures.html)" section of
the MySQL Reference Manual.
Connector/J exposes stored procedure functionality through JDBC's
CallableStatement interface.
The following example shows a stored procedure that returns the
value of inOutParam incremented by 1, and the string passed in via
inputParam as a ResultSet:
Example 3. Stored Procedure Example
CREATE PROCEDURE demoSp(IN inputParam VARCHAR(255), INOUT inOutParam
INT)
BEGIN
DECLARE z INT;
SET z = inOutParam + 1;
SET inOutParam = z;
SELECT inputParam;
SELECT CONCAT('zyxw', inputParam);
END
To use the demoSp procedure with Connector/J, follow these steps:
1. Prepare the callable statement by
没有合适的资源?快使用搜索试试~ 我知道了~
资源详情
资源评论
资源推荐
收起资源包目录

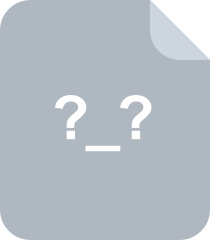
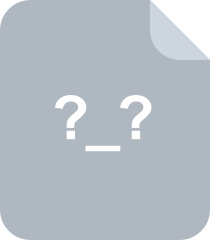
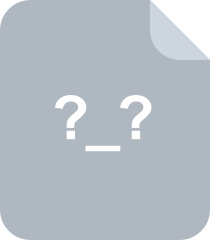
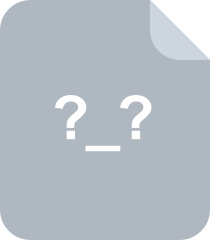
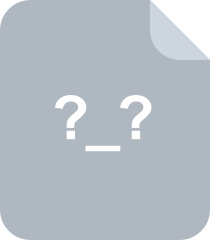
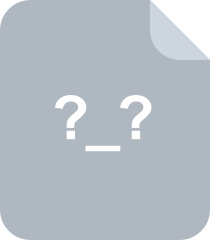
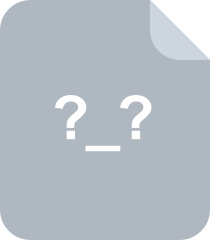
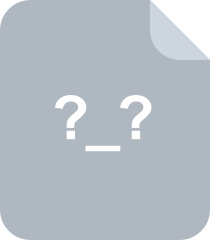
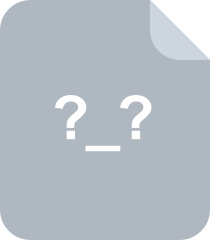
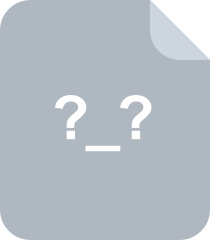
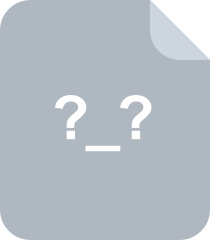
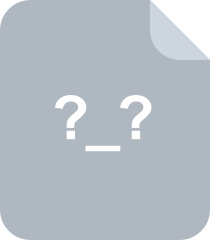
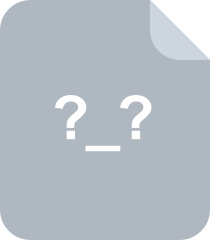
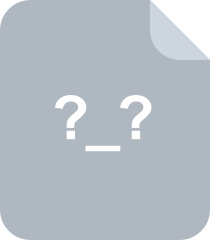
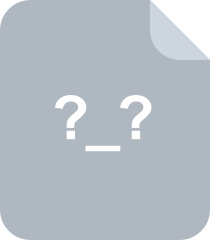
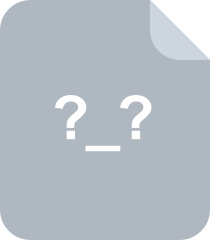
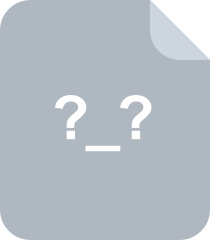
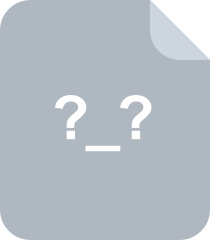
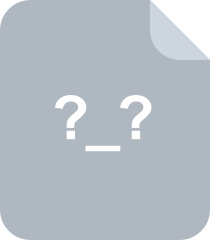
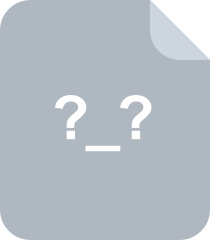
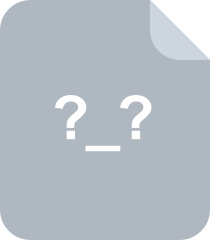
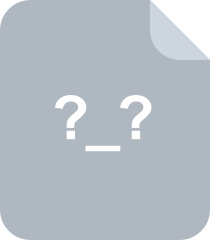
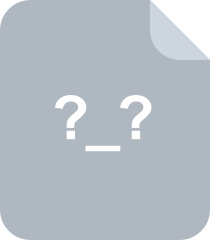
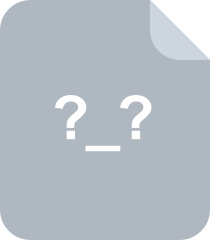
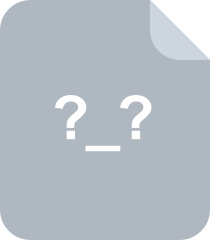
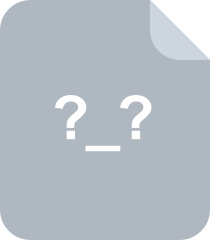
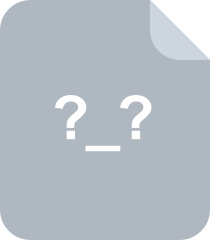
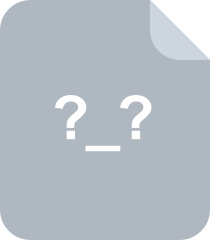
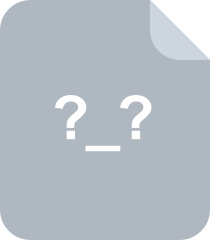
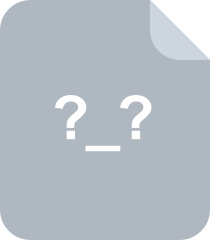
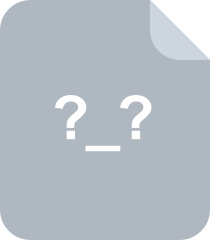
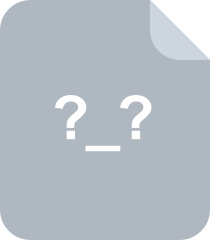
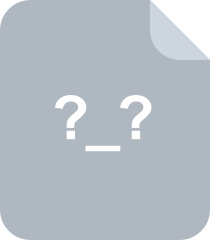
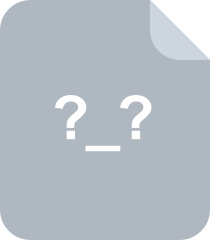
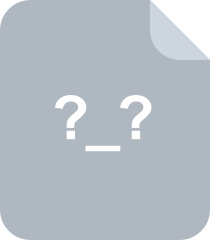
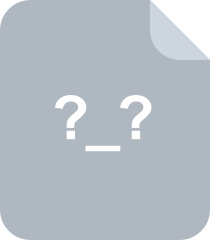
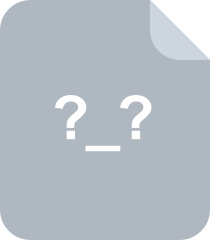
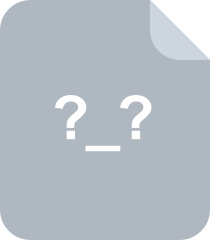
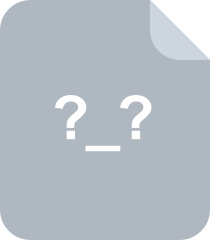
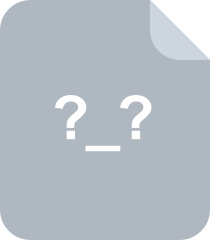
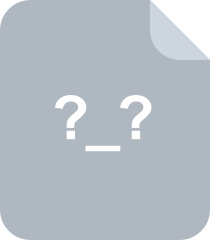
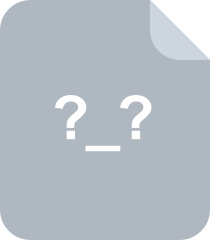
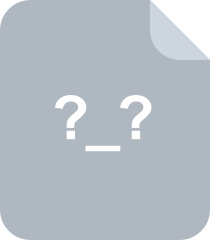
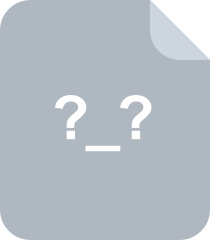
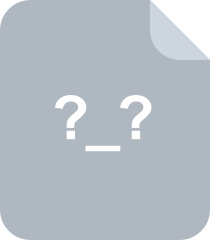
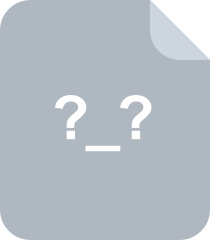
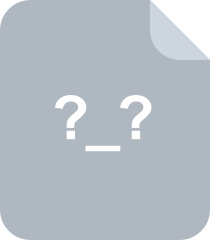
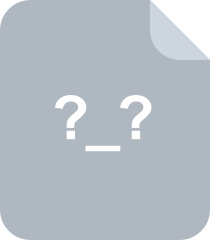
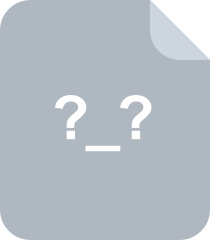
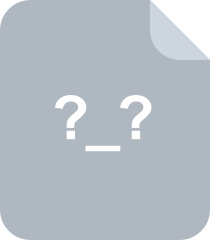
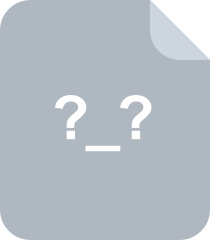
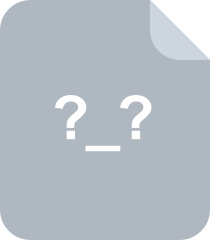
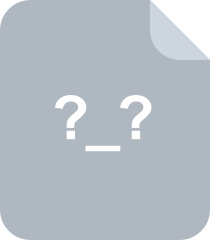
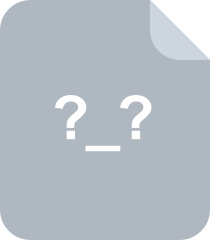
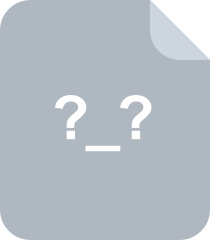
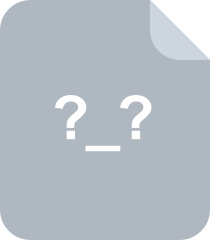
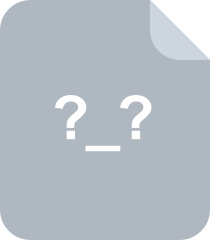
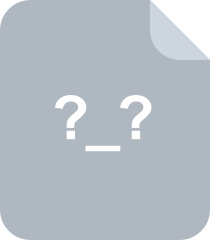
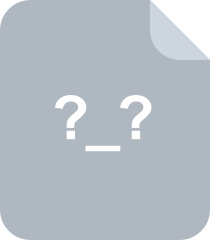
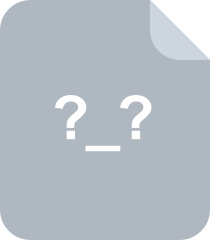
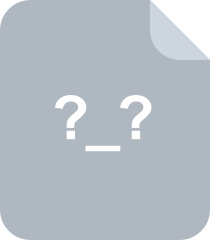
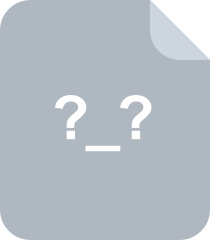
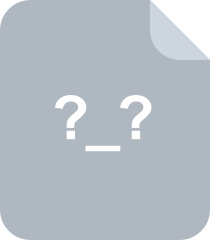
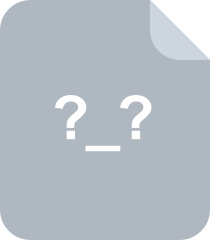
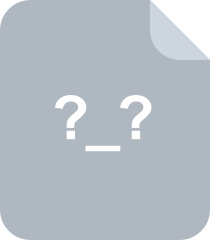
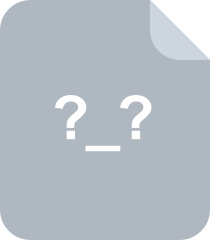
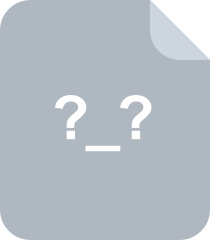
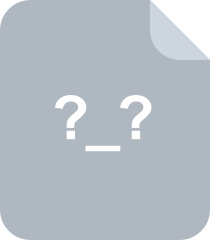
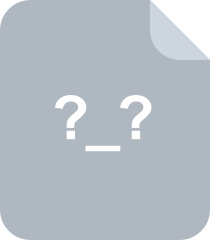
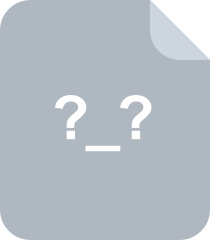
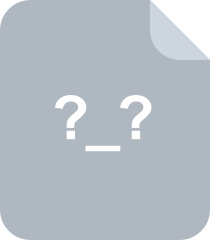
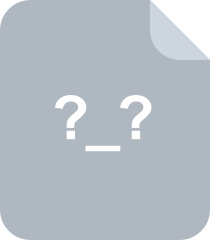
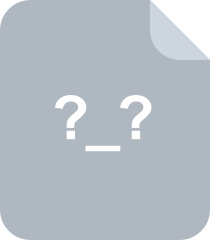
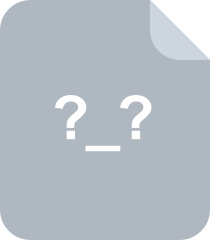
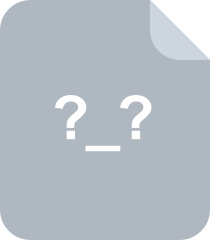
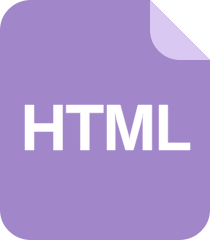
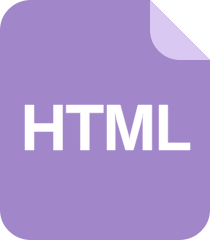
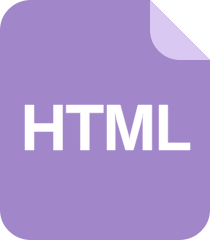
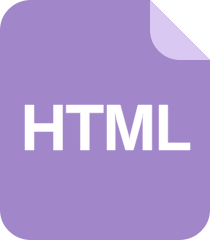
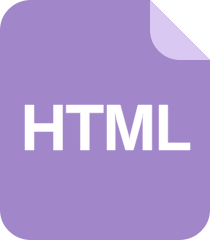
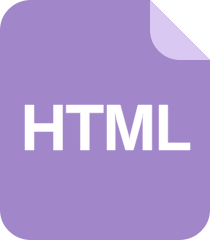
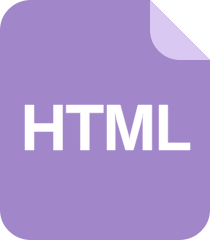
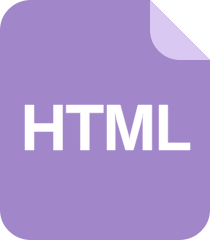
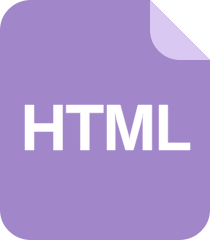
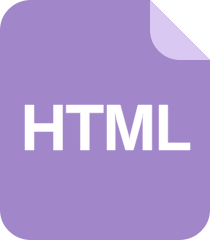
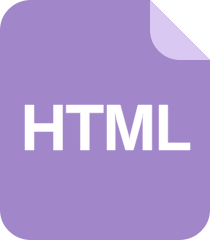
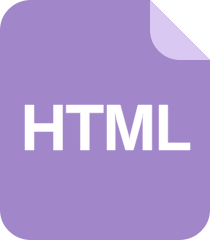
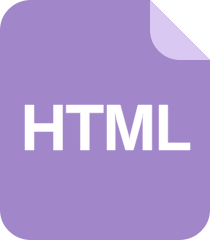
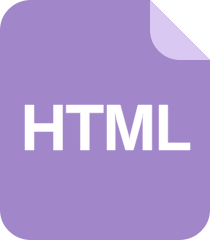
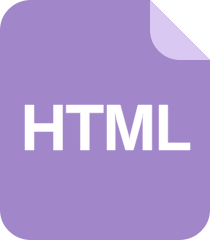
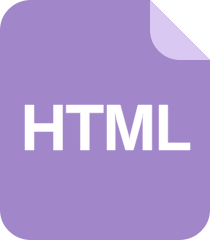
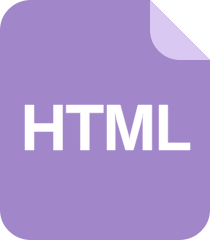
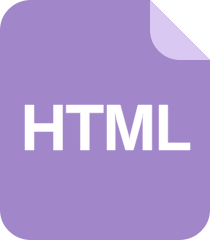
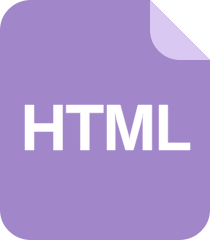
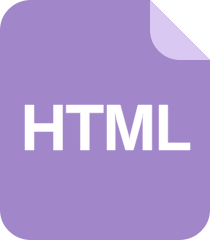
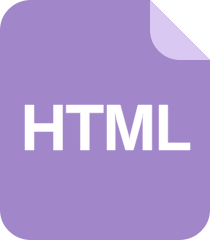
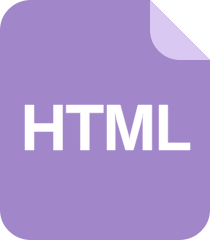
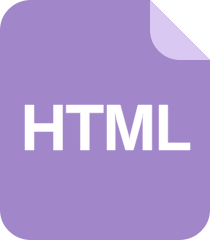
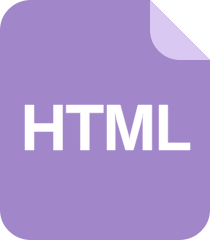
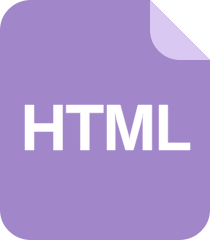
共 3811 条
- 1
- 2
- 3
- 4
- 5
- 6
- 39
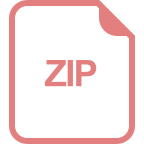
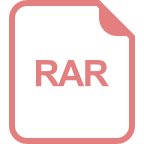
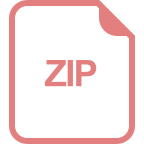
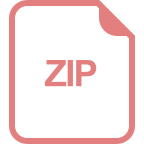
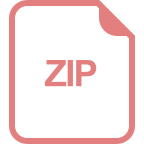
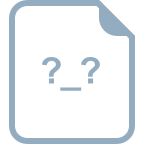
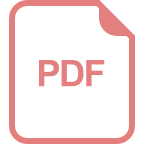
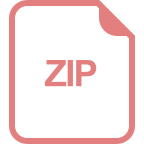
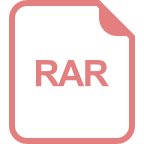
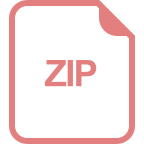
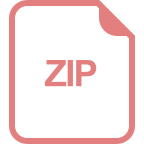

bi_griver
- 粉丝: 0
- 资源: 7
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

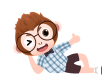
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0