package com.zghzbckj.project.utils;
import com.ourway.base.CommonConstants;
import com.ourway.base.utils.JackSonJsonUtils;
import com.ourway.base.utils.TextUtils;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.util.Base64;
import java.util.zip.GZIPInputStream;
import java.util.zip.GZIPOutputStream;
public class StringUtils {
final static Base64.Encoder encoder = Base64.getEncoder();
final static Base64.Decoder decoder = Base64.getDecoder();
/**
*<p>方法:返回指定長度的字符串 </p>
*<ul>
*<li> @param s TODO</li>
*<li> @param len TODO</li>
*<li>@return java.lang.String </li>
*<li>@author JackZhou </li>
*<li>@date 2019/4/13 18:18 </li>
*</ul>
*/
public static String getSubStr(String s, int len) {
if (TextUtils.isEmpty(s)) {
return "";
}
if (s.length() > len) {
return s.substring(0, len);
}
if (s.length() <= len) {
return s;
}
return "";
}
/**
*<p>方法:compress 压缩字符串</p>
*<ul>
*<li> @param str TODO</li>
*<li>@return java.lang.String </li>
*<li>@author D.chen.g </li>
*<li>@date 2019/12/5 14:18 </li>
*</ul>
*/
public static String compress(String str) throws IOException {
if (null == str || str.length() <= 0) {
return str;
}
// 创建一个新的输出流
ByteArrayOutputStream out = new ByteArrayOutputStream();
// 使用默认缓冲区大小创建新的输出流
GZIPOutputStream gzip = new GZIPOutputStream(out);
// 将字节写入此输出流
gzip.write(str.getBytes(CommonConstants.CHARSET_UTF_8)); // 因为后台默认字符集有可能是GBK字符集,所以此处需指定一个字符集
gzip.close();
// 使用指定的 charsetName,通过解码字节将缓冲区内容转换为字符串
return out.toString(CommonConstants.CHARSET_ISO8859_1);
}
/**
*<p>方法:unCompress 解压字符串 </p>
*<ul>
*<li> @param str TODO</li>
*<li>@return java.lang.String </li>
*<li>@author D.chen.g </li>
*<li>@date 2019/12/5 14:17 </li>
*</ul>
*/
public static String unCompress(String str) throws IOException {
if (null == str || str.length() <= 0) {
return str;
}
// 创建一个新的输出流
ByteArrayOutputStream out = new ByteArrayOutputStream();
// 创建一个 ByteArrayInputStream,使用 buf 作为其缓冲 区数组
ByteArrayInputStream in = new ByteArrayInputStream(str.getBytes(CommonConstants.CHARSET_ISO8859_1));
// 使用默认缓冲区大小创建新的输入流
GZIPInputStream gzip = new GZIPInputStream(in);
byte[] buffer = new byte[256];
int n = 0;
// 将未压缩数据读入字节数组
while ((n = gzip.read(buffer)) >= 0) {
out.write(buffer, 0, n);
}
// 使用指定的 charsetName,通过解码字节将缓冲区内容转换为字符串
return out.toString(CommonConstants.CHARSET_UTF_8);
}
/**
*<p>方法:base64Code base64编码 </p>
*<ul>
*<li> @param text TODO</li>
*<li>@return java.lang.String </li>
*<li>@author D.chen.g </li>
*<li>@date 2019/12/5 14:17 </li>
*</ul>
*/
public static String base64Code(String text) throws Exception {
if (TextUtils.isEmpty(text)) {
text = "";
}
byte[] textByte = text.getBytes(CommonConstants.CHARSET_UTF_8);
final String encodedText = encoder.encodeToString(textByte);
return encodedText;
}
/**
*<p>方法:deBase64Code base64编码后的字符串解码 </p>
*<ul>
*<li> @param text TODO</li>
*<li>@return java.lang.String </li>
*<li>@author D.chen.g </li>
*<li>@date 2019/12/5 14:16 </li>
*</ul>
*/
public static String deBase64Code(String text) {
String encodedText="";
try {
encodedText = new String(decoder.decode(text), CommonConstants.CHARSET_UTF_8);
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
return encodedText;
}
/**
*<p>方法:base64Andcompress base64编码以及压缩 </p>
*<ul>
*<li> @param text TODO</li>
*<li>@return java.lang.String </li>
*<li>@author D.chen.g </li>
*<li>@date 2019/12/5 14:21 </li>
*</ul>
*/
public static String base64Andcompress(String text) {
try {
return compress(base64Code(text));
} catch (Exception e) {
e.printStackTrace();
return "";
}
}
/**
*<p>方法:base64解码以及解压 </p>
*<ul>
*<li> @param text TODO</li>
*<li>@return java.lang.String </li>
*<li>@author D.chen.g </li>
*<li>@date 2019/12/5 14:22 </li>
*</ul>
*/
public static String deBase64AndUncompress(String text) {
try {
return deBase64Code(unCompress(text));
} catch (Exception e) {
e.printStackTrace();
return "";
}
}
/**
*<p>方法:base64AndcompressJson 数据返回bean变成Json字符串后编码压缩 </p>
*<ul>
*<li> @param data TODO</li>
*<li>@return java.lang.String </li>
*<li>@author D.chen.g </li>
*<li>@date 2019/12/6 17:52 </li>
*</ul>
*/
public static String base64AndCompressJson(Object data) {
try {
String text=JackSonJsonUtils.toJson(data);
return base64Andcompress(text);
} catch (Exception e) {
e.printStackTrace();
return "";
}
}
}

benyuanone
- 粉丝: 15
- 资源: 22
最新资源
- 没用333333333333333333333333333333
- 基于Vue和SpringBoot的企业员工管理系统2.0版本设计源码
- 【C++初级程序设计·配套源码】第2期-基本数据类型
- 基于Java和Vue的kopsoftKANBAN车间电子看板设计源码
- 影驰战将PS3111 东芝芯片TT18G23AIN开卡成功分享,图片里面画线的选项很重要
- 【C++初级程序设计·配套源码】第1期-语法基础
- 基于JavaScript、CSS、HTML的简易DOM版飞机游戏设计源码
- 基于Java开发的日程管理FlexTime应用设计源码
- SM2258XT-BGA144-4BGA180-6L-R1019 三星KLUCG4J1CB B0B1颗粒开盘工具 , EC, 3A, 94, 43, A4, CA 七彩虹SL300这个固件有用
- GJB 5236-2004 军用软件质量度量
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


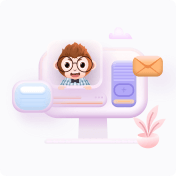