双向链表的建立,插入,删除,寻找元素等算法

根据给定文件的信息,本文将详细介绍双向链表的创建、插入、删除以及查询元素等相关算法。双向链表是一种常见的线性数据结构,在计算机科学中有着广泛的应用,它不仅支持向前遍历,还支持向后遍历,使得在链表中的操作更加灵活。 ### 双向链表简介 双向链表(Doubly Linked List)是一种更复杂的线性表存储结构,每个节点包含三个域:数据域、前驱指针域和后继指针域。其中前驱指针指向其前一个节点,而后继指针则指向其后一个节点。这种结构使得节点可以在任意方向上进行访问或修改,提供了更多的灵活性。 ### 创建双向链表 首先定义双向链表的基本结构: ```c typedef int elemtype; typedef struct DuLNode { elemtype data; struct DuLNode *prior; struct DuLNode *next; } DuLNode, *DuLinklist; ``` 初始化双向链表涉及到为头结点分配内存空间,并将其前后指针置空: ```c status Initial_DuLinklist(DuLinklist &DL) { printf("Initial the DuLinklist\n"); DL = (DuLinklist)malloc(sizeof(DuLNode)); if (!DL) exit(overflow); DL->next = NULL; return ok; } ``` 接下来是创建一个双向链表的过程,通过读取用户输入的数据来动态构建链表: ```c status Creat_DuLinklist(DuLinklist &DL) { DuLinklist p, q; elemtype e; int i; printf("How many members do you want in the DoLinklist?\n"); scanf("%d", &Link_len); printf("Create the DuLinklist\n"); p = DL; for (i = 0; i < Link_len; i++) { q = (DuLinklist)malloc(sizeof(DuLNode)); p->next = q; scanf("%d", &e); q->data = e; q->prior = p; q->next = NULL; p = q; } fflush(stdin); return ok; } ``` ### 打印双向链表 打印链表中的所有元素,以便于检查链表的状态: ```c status Print_Link(DuLinklist &DL) { printf("Print the DuLinklist\n"); DuLinklist p; for (p = DL->next; p; p = p->next) printf("%3d", p->data); printf("\n"); return ok; } ``` ### 查询链表中的指定位置元素 查询链表中指定位置的元素,这通常涉及到遍历链表直到找到目标位置: ```c status Get_Position(DuLinklist &DL, int P) { printf("Get the element in a position, which position do you want?\n"); scanf("%d", &P); int i = 0; DuLinklist p = DL; while (i < P && p) { i++; p = p->next; } if (i > P || !p) exit(overflow); printf("The element you get is %3d:\n", p->data); return ok; } ``` ### 插入新元素 在链表的特定位置插入新元素,首先需要定位到插入位置的前一个节点: ```c status Insert_Element(DuLinklist &DL, int I_P, elemtype I_E) { DuLinklist q, s; int i; printf("Which position and what number do you want to insert into the link?\n"); scanf("%d", &I_P); scanf("%d", &I_E); if (I_P < 1 || I_P > Link_len) return error; printf("Now insert into the link\n"); q = DL; for (i = 0; i < I_P; i++) q = q->next; // 此处应继续完成插入逻辑 } ``` ### 删除链表中的元素 删除链表中的指定元素涉及找到该元素所在的节点并更新其前后节点的指针: ```c status Delete_Element(DuLinklist &DL, elemtype D_E) { DuLinklist p, q; p = DL->next; while (p != NULL && p->data != D_E) { q = p; p = p->next; } if (p == NULL) return error; // 元素不存在 q->next = p->next; if (p->next != NULL) p->next->prior = q; free(p); return ok; } ``` 以上是基于给定代码片段对双向链表的一些基本操作的实现与解释,包括了创建、插入、删除和查询等功能。这些操作是双向链表中最基础也是最常用的,掌握了这些操作可以帮助更好地理解和使用双向链表。
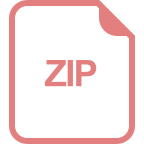
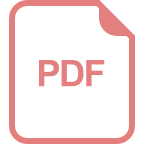
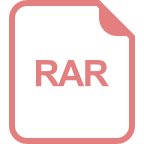
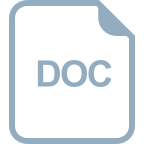
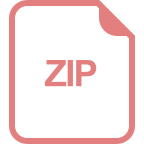
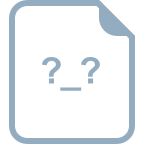
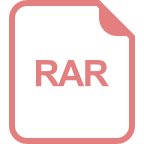
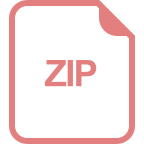
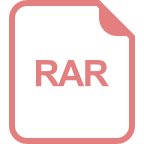
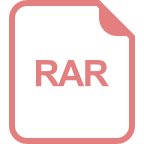
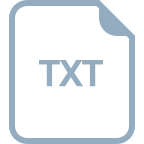
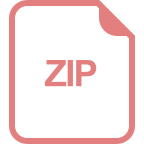
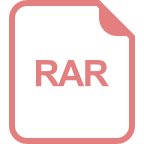
#include<stdlib.h>
#define ok 1
#define error 0
#define overflow 1
typedef int status;
typedef int elemtype;
typedef struct DuLNode{
elemtype data;
struct DuLNode *prior;
struct DuLNode *next;
}DuLNode,*DuLinklist;
//=================全局变量===========================================
DuLinklist DL;
int Link_len,P,D_P;
elemtype I_E,D_E;
//==================Initial_DuLinklist==================================
status Initial_DuLinklist(DuLinklist &DL)
{ printf("Initial the DuLinklist\n");
DL=(DuLinklist)malloc(sizeof(DuLNode));
if(!DL) exit(overflow);
DL->next=NULL;
return ok;
}
//====================Creat_DuLinklist=======================================
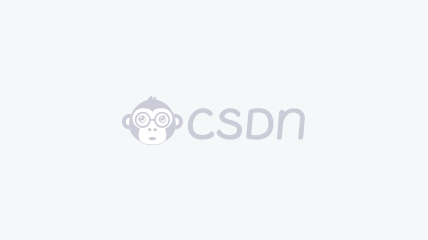
- FATTY19932013-11-20非常感谢 很有用

- 粉丝: 0
- 资源: 2
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

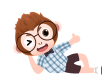
最新资源

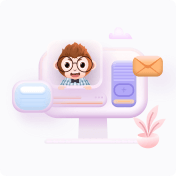
