package com.coresun.powerbank.ui.main.viewpager;
import android.annotation.SuppressLint;
import android.content.Context;
import android.database.DataSetObserver;
import android.graphics.Canvas;
import android.graphics.Rect;
import android.graphics.drawable.Drawable;
import android.os.Parcel;
import android.os.Parcelable;
import android.os.SystemClock;
import android.support.v4.os.ParcelableCompat;
import android.support.v4.os.ParcelableCompatCreatorCallbacks;
import android.support.v4.view.MotionEventCompat;
import android.support.v4.view.PagerAdapter;
import android.support.v4.view.VelocityTrackerCompat;
import android.support.v4.view.ViewCompat;
import android.support.v4.view.ViewConfigurationCompat;
import android.support.v4.widget.EdgeEffectCompat;
import android.util.AttributeSet;
import android.util.Log;
import android.view.FocusFinder;
import android.view.KeyEvent;
import android.view.MotionEvent;
import android.view.SoundEffectConstants;
import android.view.VelocityTracker;
import android.view.View;
import android.view.ViewConfiguration;
import android.view.ViewGroup;
import android.view.ViewParent;
import android.view.accessibility.AccessibilityEvent;
import android.view.animation.Interpolator;
import android.widget.Scroller;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
/**
* Layout manager that allows the user to flip left and right
* through pages of data. You supply an implementation of a
* {@link android.support.v4.view.PagerAdapter} to generate the pages that the view shows.
*
* <p>Note this class is currently under early design and
* development. The API will likely change in later updates of
* the compatibility library, requiring changes to the source code
* of apps when they are compiled against the newer version.</p>
*/
public class NoPreloadViewPager extends ViewGroup {
private static final String TAG = "<span style=\"font-family:Arial, Helvetica, sans-serif;\">NoPreLoadViewPager</span>";
private static final boolean DEBUG = false;
private static final boolean USE_CACHE = false;
private static final int DEFAULT_OFFSCREEN_PAGES = 0;//默认是1
private static final int MAX_SETTLE_DURATION = 600; // ms
static class ItemInfo {
Object object;
int position;
boolean scrolling;
}
private static final Comparator<ItemInfo> COMPARATOR = new Comparator<ItemInfo>(){
@Override
public int compare(ItemInfo lhs, ItemInfo rhs) {
return lhs.position - rhs.position;
}};
private static final Interpolator sInterpolator = new Interpolator() {
public float getInterpolation(float t) {
// _o(t) = t * t * ((tension + 1) * t + tension)
// o(t) = _o(t - 1) + 1
t -= 1.0f;
return t * t * t + 1.0f;
}
};
private final ArrayList<ItemInfo> mItems = new ArrayList<ItemInfo>();
private PagerAdapter mAdapter;
private int mCurItem; // Index of currently displayed page.
private int mRestoredCurItem = -1;
private Parcelable mRestoredAdapterState = null;
private ClassLoader mRestoredClassLoader = null;
private Scroller mScroller;
private PagerObserver mObserver;
private int mPageMargin;
private Drawable mMarginDrawable;
private int mChildWidthMeasureSpec;
private int mChildHeightMeasureSpec;
private boolean mInLayout;
private boolean mScrollingCacheEnabled;
private boolean mPopulatePending;
private boolean mScrolling;
private int mOffscreenPageLimit = DEFAULT_OFFSCREEN_PAGES;
private boolean mIsBeingDragged;
private boolean mIsUnableToDrag;
private int mTouchSlop;
private float mInitialMotionX;
/**
* Position of the last motion event.
*/
private float mLastMotionX;
private float mLastMotionY;
/**
* ID of the active pointer. This is used to retain consistency during
* drags/flings if multiple pointers are used.
*/
private int mActivePointerId = INVALID_POINTER;
/**
* Sentinel value for no current active pointer.
* Used by {@link #mActivePointerId}.
*/
private static final int INVALID_POINTER = -1;
/**
* Determines speed during touch scrolling
*/
private VelocityTracker mVelocityTracker;
private int mMinimumVelocity;
private int mMaximumVelocity;
private float mBaseLineFlingVelocity;
private float mFlingVelocityInfluence;
private boolean mFakeDragging;
private long mFakeDragBeginTime;
private EdgeEffectCompat mLeftEdge;
private EdgeEffectCompat mRightEdge;
private boolean mFirstLayout = true;
private OnPageChangeListener mOnPageChangeListener;
/**
* Indicates that the pager is in an idle, settled state. The current page
* is fully in view and no animation is in progress.
*/
public static final int SCROLL_STATE_IDLE = 0;
/**
* Indicates that the pager is currently being dragged by the user.
*/
public static final int SCROLL_STATE_DRAGGING = 1;
/**
* Indicates that the pager is in the process of settling to a final position.
*/
public static final int SCROLL_STATE_SETTLING = 2;
private int mScrollState = SCROLL_STATE_IDLE;
/**
* Callback interface for responding to changing state of the selected page.
*/
public interface OnPageChangeListener {
/**
* This method will be invoked when the current page is scrolled, either as part
* of a programmatically initiated smooth scroll or a user initiated touch scroll.
*
* @param position Position index of the first page currently being displayed.
* Page position+1 will be visible if positionOffset is nonzero.
* @param positionOffset Value from [0, 1) indicating the offset from the page at position.
* @param positionOffsetPixels Value in pixels indicating the offset from position.
*/
public void onPageScrolled(int position, float positionOffset, int positionOffsetPixels);
/**
* This method will be invoked when a new page becomes selected. Animation is not
* necessarily complete.
*
* @param position Position index of the new selected page.
*/
public void onPageSelected(int position);
/**
* Called when the scroll state changes. Useful for discovering when the user
* begins dragging, when the pager is automatically settling to the current page,
* or when it is fully stopped/idle.
*
* @param state The new scroll state.
* @see android.support.v4.view.ViewPager#SCROLL_STATE_IDLE
* @see android.support.v4.view.ViewPager#SCROLL_STATE_DRAGGING
* @see android.support.v4.view.ViewPager#SCROLL_STATE_SETTLING
*/
public void onPageScrollStateChanged(int state);
}
/**
* Simple implementation of theinterface with stub
* implementations of each method. Extend this if you do not intend to override
* every method of
*/
public static class SimpleOnPageChangeListener implements OnPageChangeListener {
@Override
public void onPageScrolled(int position, float positionOffset, int positionOffsetPixels) {
// This space for rent
}
@Override
public void onPageSelected(int position) {
// This space for rent
}
@Override
public void onPageScrollStateChanged(int state) {
// This space for rent
}
}
public NoPreloadViewPager(Context context) {
super(context);
initViewPager();
}
public NoPreloadViewPager(Context context, AttributeSet attrs) {
super(context, attrs);
initViewPager();
}
void initViewPager() {
setWillNotDraw(false);
setDescendantFocusability(FOCUS_AFTER_DESCENDAN
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【资源说明】 1、该资源包括项目的全部源码,下载可以直接使用! 2、本项目适合作为计算机、数学、电子信息等专业的课程设计、期末大作业和毕设项目,作为参考资料学习借鉴。 3、本资源作为“参考资料”如果需要实现其他功能,需要能看懂代码,并且热爱钻研,自行调试。 充电宝广告机(项目源码+说明).zip
资源推荐
资源详情
资源评论
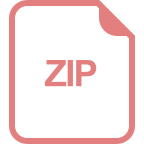
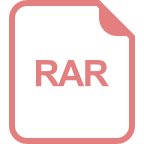
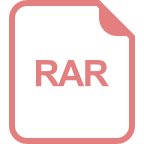
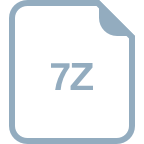
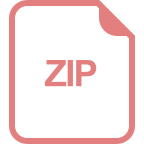
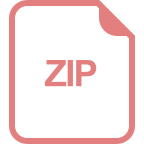
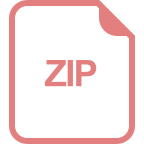
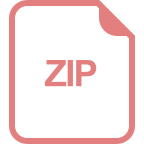
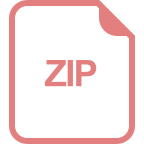
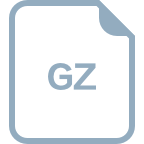
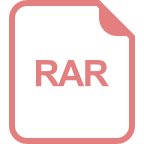
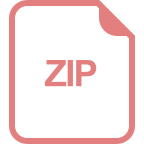
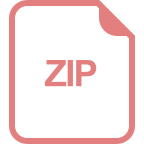
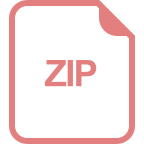
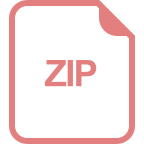
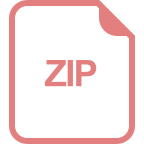
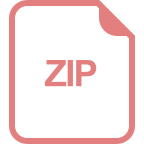
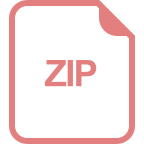
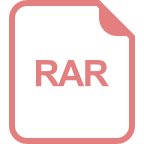
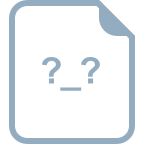
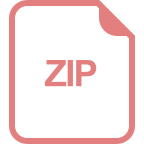
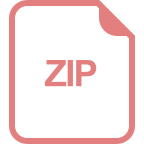
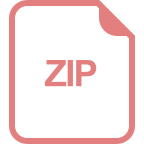
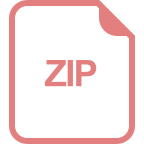
收起资源包目录

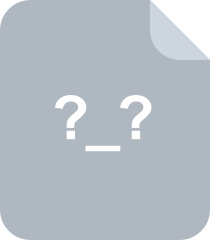
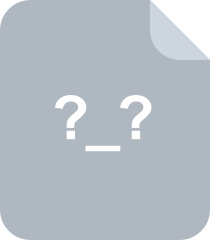
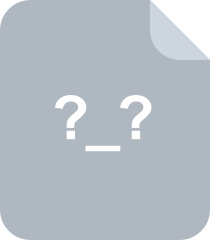
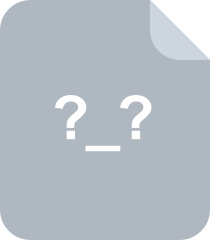
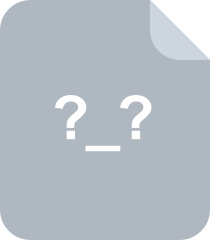
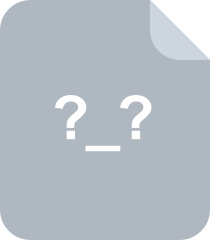
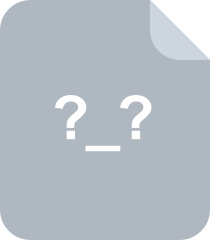
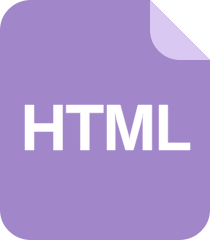
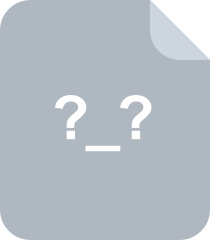
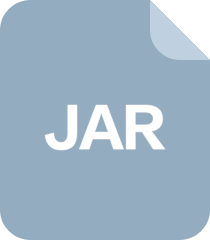
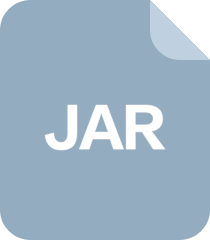
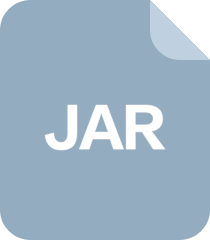
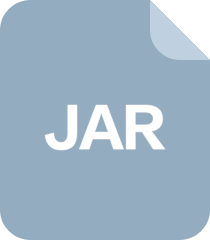
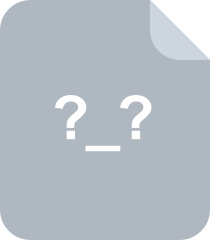
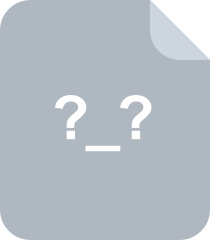
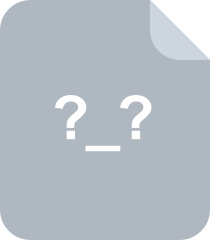
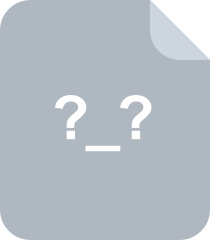
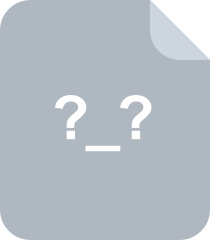
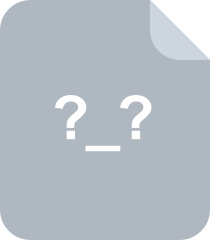
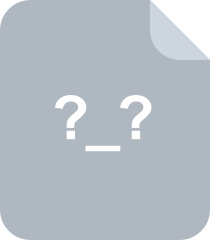
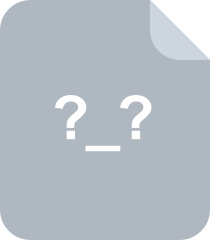
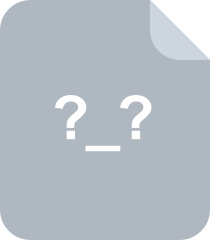
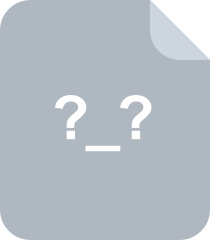
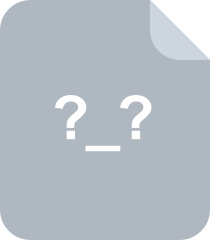
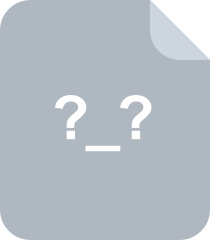
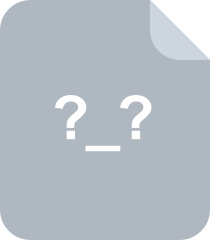
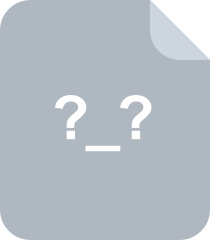
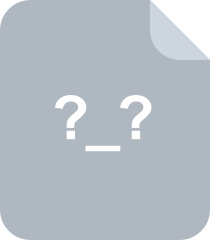
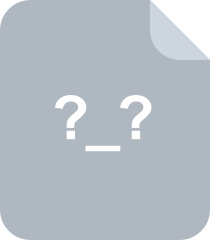
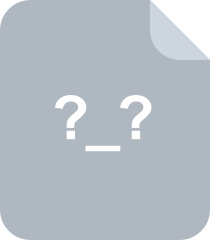
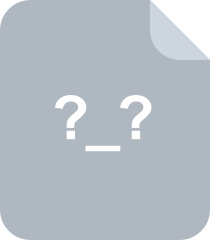
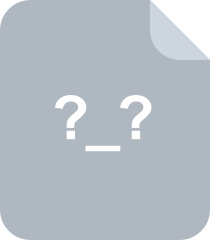
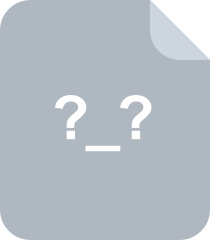
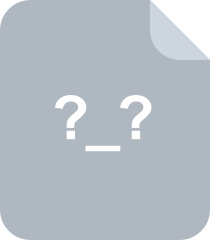
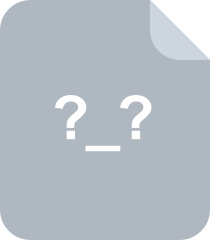
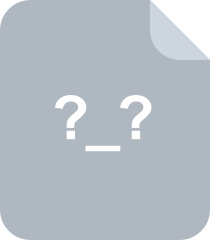
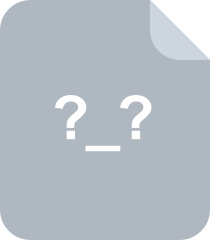
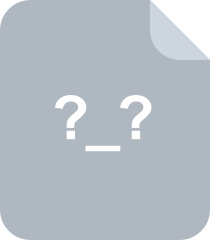
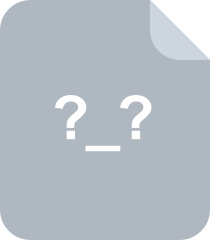
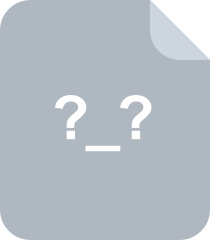
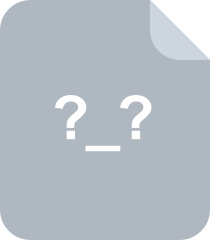
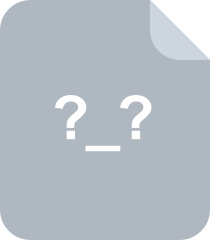
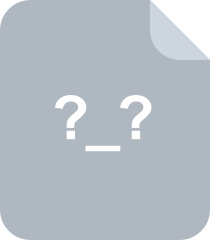
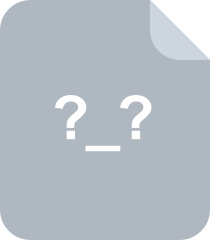
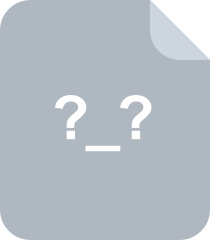
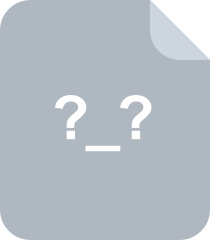
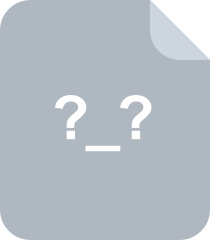
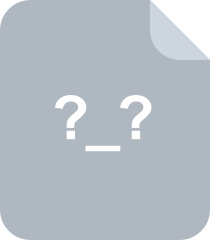
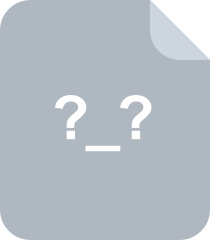
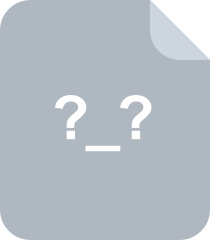
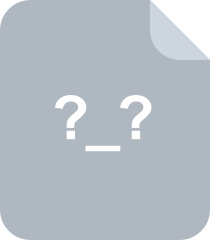
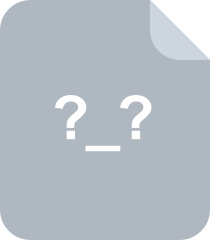
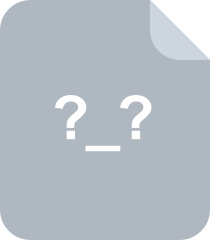
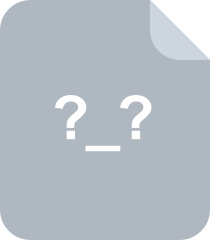
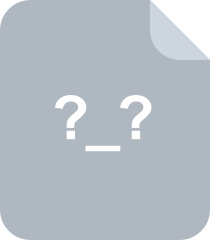
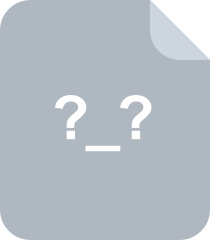
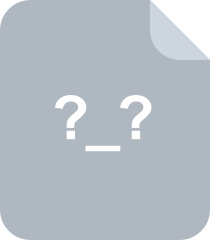
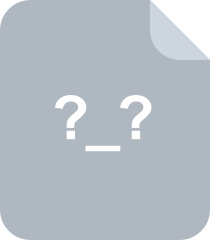
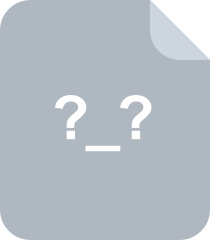
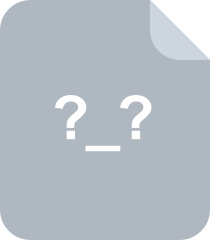
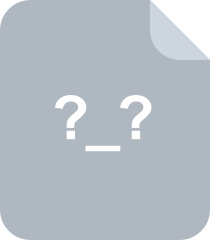
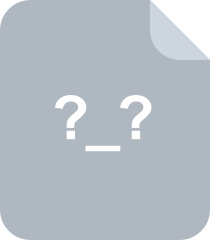
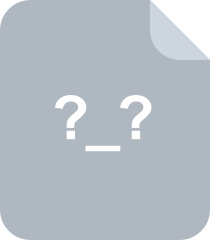
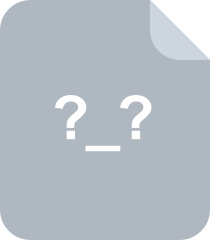
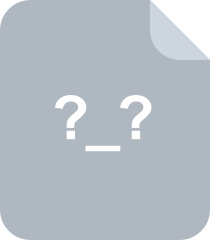
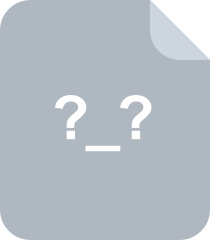
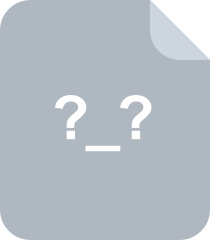
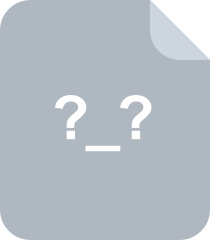
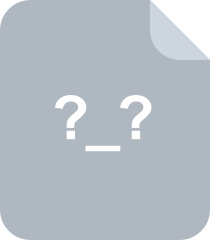
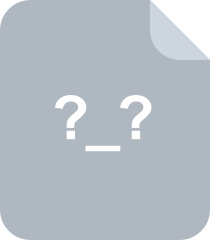
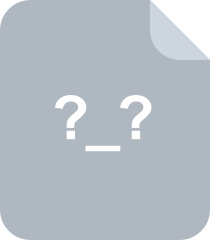
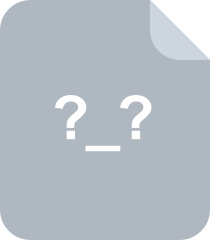
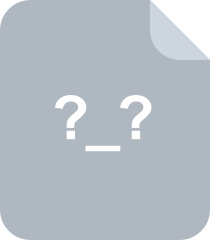
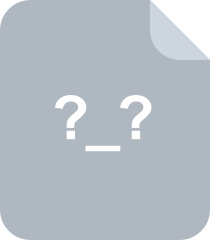
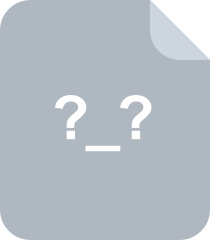
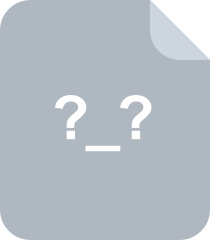
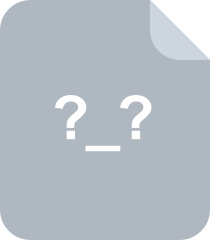
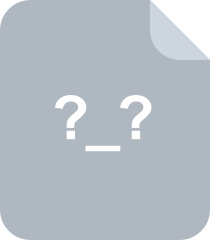
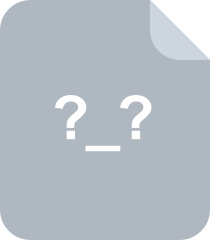
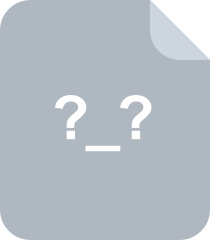
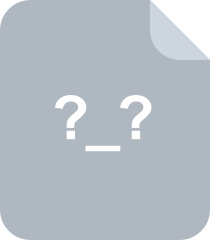
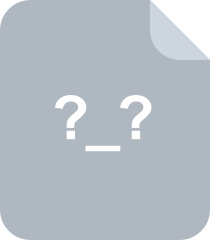
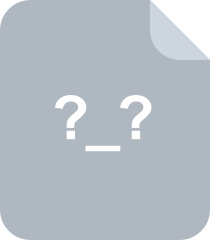
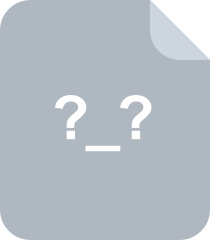
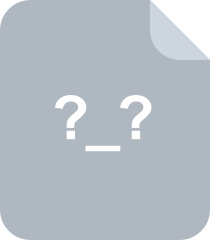
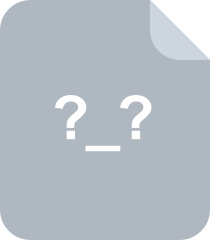
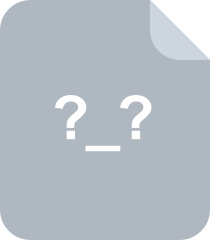
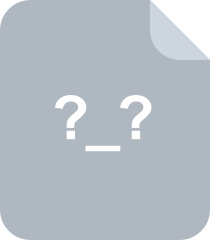
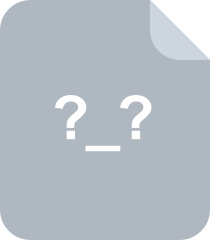
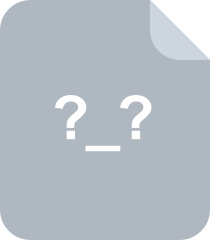
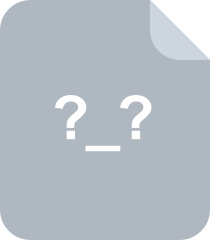
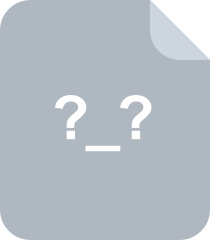
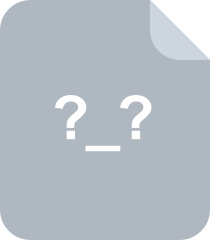
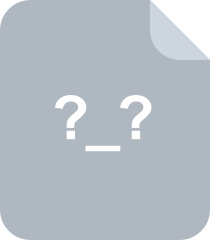
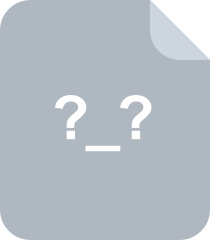
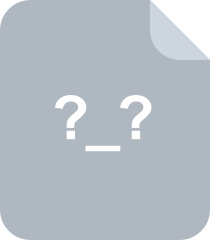
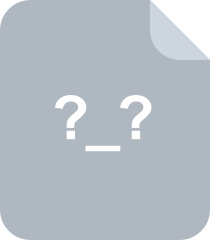
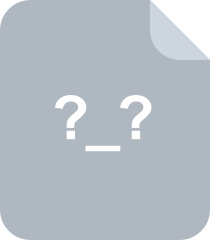
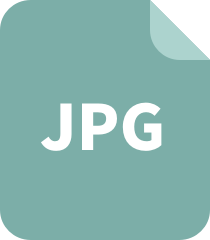
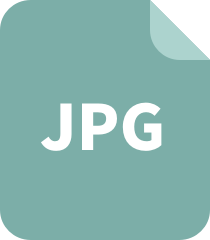
共 1535 条
- 1
- 2
- 3
- 4
- 5
- 6
- 16
资源评论
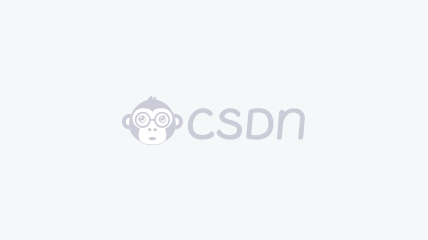
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整

土豆片片
- 粉丝: 1857
- 资源: 5869
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

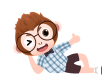
最新资源
- 大学生心理测评与分析-基于springBoot大学生心理测评与分析系统(毕业论文+PPT)
- PHP黄金庄园农村区块链种植游戏源码 【修复版可运营】
- 最简单的基于FFmpeg的推流器(以推送RTMP为例)
- wCMU电芯性能数据采集模块的管理工具开发,做准备工作,查阅CANHUB及CMU用户手册,了解其设备配置、网络管理、程序下载及更新等
- 乌燕鸥算法SAOT优化随机森林做二分类和多分类预测建模 程序内注释详细直接替数据就可以使用 程序语言为matlab 程序直接运行可以出分类预测图,迭代优化图,特征重要性排序图,混淆矩阵图 想要
- 《使用 YOLOv3 算法进行基于深度学习的人脸检测》计算机相关专业毕业设计&大作业 (源码、说明、论文、数据集一站式服务,拿来就能用的绝对好资源)
- 改进的鲸鱼优化算法GSWOA优化随机森林做二分类和多分类预测建模 程序内注释详细直接替数据就可以使用 程序语言为matlab 程序直接运行可以出分类预测图,迭代优化图,特征重要性排序图,混淆矩阵
- 关键词:多时间尺度;模型预测控制;日内滚动优化; 描述:以包含风力场、光伏电站、微型燃气轮机、蓄电池、余热锅炉、热泵、储热罐和电 热负荷的多能源微网系统为研究对象,构建了各微源的数学模型 然后,提出
- 激光熔覆comsol热流耦合模型,温度场分布和三维流场分布 考虑马兰戈尼效应,表面张力 考虑热传导,动网格模拟相界面流体流动 提供模型+参考文献+参数说明+模型指导+操作视频
- matlab和Python互转 人工手动转两种程序语言,不是简单的转软件,可以转复杂的不同求解器的内容
- 娱快编程-Python开源扫雷游戏
- 鸽群优化算法PIO优化BP做多特征输入单输出的二分类及多分类模型 程序内注释详细,直接替数据就可以用 程序语言为matlab 程序可出分类效果图,迭代优化图,混淆矩阵图,ROC曲线图,具体效果如
- 《使用 PyTorch、OpenCV 和排序跟踪的 YOLOv7 对象跟踪》计算机相关专业毕业设计&大作业 (源码、说明、论文、数据集一站式服务,拿来就能用的绝对好资源)
- 正点原子lwIP执行流程图(转)
- 爱尔兰数据分析,jupyter 源文件
- 计算excel两列时间的时间差
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


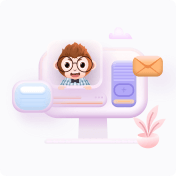
安全验证
文档复制为VIP权益,开通VIP直接复制
