//
// MMProgressHUD.h
// MMProgressHUD
//
// Created by Lars Anderson on 10/7/11.
// Copyright 2011 Mutual Mobile. All rights reserved.
//
#import <UIKit/UIKit.h>
#import "MMHud.h"
extern NSString * const MMProgressHUDDefaultConfirmationMessage;
extern NSString * const MMProgressHUDAnimationShow;
extern NSString * const MMProgressHUDAnimationDismiss;
extern NSString * const MMProgressHUDAnimationWindowFadeOut;
extern NSString * const MMProgressHUDAnimationKeyShowAnimation;
extern NSString * const MMProgressHUDAnimationKeyDismissAnimation;
extern CGFloat const MMProgressHUDStandardDismissDelay;
@class MMProgressHUDWindow;
@class MMProgressHUDOverlayView;
typedef NS_ENUM(NSInteger, MMProgressHUDPresentationStyle){
MMProgressHUDPresentationStyleDrop = 0, //default
MMProgressHUDPresentationStyleExpand,
MMProgressHUDPresentationStyleShrink,
MMProgressHUDPresentationStyleSwingLeft,
MMProgressHUDPresentationStyleSwingRight,
MMProgressHUDPresentationStyleBalloon,
MMProgressHUDPresentationStyleFade,
MMProgressHUDPresentationStyleNone
};
typedef NS_ENUM(NSInteger, MMProgressHUDWindowOverlayMode){
MMProgressHUDWindowOverlayModeNone = -1,
MMProgressHUDWindowOverlayModeGradient = 0,
MMProgressHUDWindowOverlayModeLinear,
/*MMProgressHUDWindowOverlayModeBlur*/ //iOS 7 only
};
//iOS 7 only
//typedef NS_ENUM(NSInteger, MMProgressHUDOptions) {
// MMProgressHUDOptionGravityEnabled = 1 << 0,
// MMProgressHUDOptionGyroEnabled = 1 << 1,
//};
@interface MMProgressHUD : UIView
/** An enum to specify the style in which to display progress.
The default style is indeterminate progress.
@warning Deprecated: To use determinate progress, set a progressViewClass and call showDeterminateProgressWithTitle:status and friends. All other show methods default to indeterminate progress.
*/
@property (nonatomic, assign) MMProgressHUDProgressStyle progressStyle DEPRECATED_ATTRIBUTE;
/** The determinate progress state.
The progress ranges from 0-1.
*/
@property (nonatomic, assign) CGFloat progress;
/** A Boolean value that indicates whether or not the HUD is visible. */
@property(nonatomic, readonly, getter = isVisible) BOOL visible;
/** A boolean value that indicates whether or not the HUD has been cancelled manually. */
@property (nonatomic, assign, getter = isCancelled) BOOL cancelled;
/** The presentation style for the HUD.
Persistent across show calls.
*/
@property(nonatomic, assign) MMProgressHUDPresentationStyle presentationStyle;
/** The glow color for the HUD.
The glow color is used during confirmation of a user-cancelled action.
*/
@property(nonatomic, assign) CGColorRef glowColor;
/** The default confirmation message for user-confirmed cancellation.
This message will be presented to the user when a cancelBlock is present after the user taps on the screen while the HUD is presented.
*/
@property(nonatomic, copy) NSString *confirmationMessage;
/** The void block executed when the user confirms their intent to cancel an operation. */
@property(nonatomic, copy) void(^cancelBlock)(void);
/** The image to be used for the error state. Persistent across show calls.
The image size is currently fixed to 100x100. The image will scale to fit if the image is larger than 100x100, otherwise it will remain centered.
*/
@property (nonatomic, strong) UIImage *errorImage;
/** The image to be used for the success state. Persistent across show calls.
The image size is currently fixed to 100x100. The image will scale to fit if the image is larger than 100x100, otherwise it will remain centered.
*/
@property (nonatomic, strong) UIImage *successImage;
/** A block to be executed when the progress fed to the HUD reaches 100%.
This block will also fire when the progress exceeds 100%. This block was designed to be used by setting up your completion call before calling show: on the HUD, then simply feed progress updates to the HUD via the updateProgress: methods. When progress reaches 100%, this block is automatically fired. This block is automatically released after firing and is guaranteed to only fire once.
@warning If the HUD is not visible and any of the updateProgress methods are called with a progress of at least 100% (1.f), the progressCompletion block is not guaranteed to fire. For example, if you were to call updateProgress: with 100% progress without having previously presented the HUD, the HUD will be forced to manually display itself, which will not fire the progressCompletion block.
*/
@property (nonatomic, copy) void(^progressCompletion)(void);
/** A block to be executed as soon as the dismiss animation has completed and the HUD is offscreen.
This block will be automatically released and nil'd after firing and is guaranteed to fire only once.
*/
@property (nonatomic, copy) void(^dismissAnimationCompletion)(void);
/** A block to be executed as soon as the show animation has completed and the HUD is fully on screen.
This block will be automatically released and nil'd after firing and is guaranteed to fire only once.
*/
@property (nonatomic, copy) void(^showAnimationCompletion)(void);
/** The HUD that is displaying all information. */
@property (nonatomic, strong) MMHud *hud;
/** The overlay type for the view behind the HUD */
@property (nonatomic, assign) MMProgressHUDWindowOverlayMode overlayMode;
/** The overlay view that is placed just behind the HUD.
*/
@property (nonatomic, strong, readonly) MMProgressHUDOverlayView *overlayView;
/** The class to use for the progress view. Instances of this class must confrom to the MMProgressView protocol. When setting a custom value this value must be set before setting the indeterminate property to YES.
Defaults to MMRadialProgressView
*/
@property (nonatomic, assign) Class progressViewClass;
#pragma mark - Instance Methods
- (void)showWithTitle:(NSString *)title
status:(NSString *)status
confirmationMessage:(NSString *)confirmationMessage
cancelBlock:(void(^)(void))cancelBlock
images:(NSArray *)images;
- (void)showDeterminateProgressWithTitle:(NSString *)title
status:(NSString *)status
confirmationMessage:(NSString *)confirmation
cancelBlock:(void (^)(void))cancelBlock
images:(NSArray *)images;
- (void)updateProgress:(CGFloat)progress
withStatus:(NSString *)status
title:(NSString *)title;
#pragma mark - Class Methods
/** Gives access to the shared instance of the HUD.
@return Shared MMProgressHUD instance.
*/
+ (instancetype)sharedHUD;
@end
@interface MMProgressHUD (Class)
//-----------------------------------------------
/** @name Presentation */
//-----------------------------------------------
/** Shows indeterminate HUD.
@warning All show methods are mutually exclusive of one another. Use the updateStatus: method to update the HUD's status while maintaining all previously set presentation attributes such as image, images, cancelBlock, title, or confirmationMessage. For example: calling showWithTitle:status: after calling showWithTitle:status:image: will wipe out the image specified in the latter call.
*/
+ (void)show;
/** Shows indeterminate HUD with specified title.
@warning All show methods are mutually exclusive of one another. Use the updateStatus: method to update the HUD's status while maintaining all previously set presentation attributes such as image, images, cancelBlock, title, or confirmationMessage. For example: calling showWithTitle:status: after calling showWithTitle:status:image: will wipe out the image specified in the latter call.
@param title Title to display.
*/
+ (void)showWithTitle:(NSString *)title;
/** Shows user-blocking HUD with only a status message.
Since the title of this HUD is nil, the status message font will become bold by default.
@warning All show methods are mutu
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【资源说明】 1、该资源内项目代码都是经过测试运行成功,功能正常的情况下才上传的,请放心下载使用。 2、适用人群:主要针对计算机相关专业(如计科、信息安全、数据科学与大数据技术、人工智能、通信、物联网、数学、电子信息等)的同学或企业员工下载使用,具有较高的学习借鉴价值。 3、不仅适合小白学习实战练习,也可作为大作业、课程设计、毕设项目、初期项目立项演示等,欢迎下载,互相学习,共同进步!
资源推荐
资源详情
资源评论
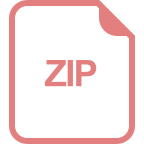
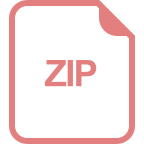
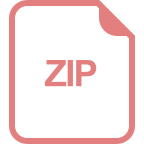
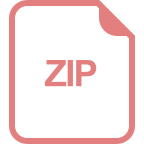
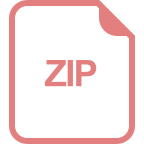
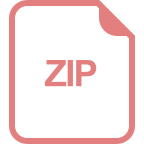
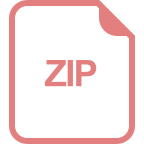
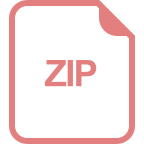
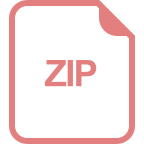
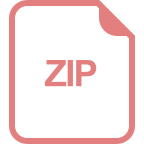
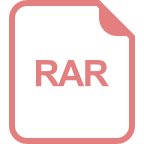
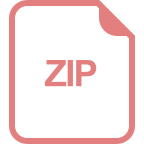
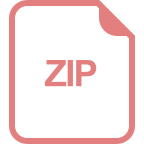
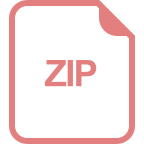
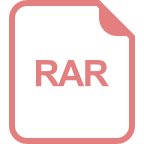
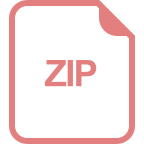
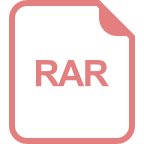
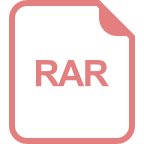
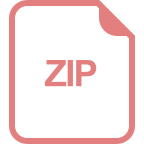
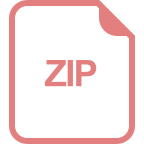
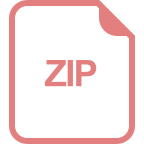
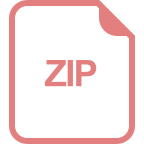
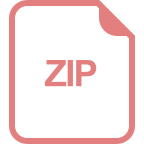
收起资源包目录

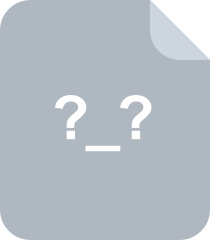
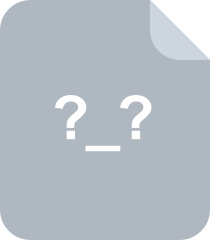
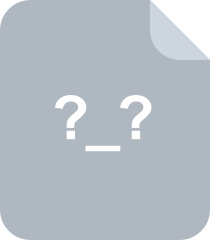
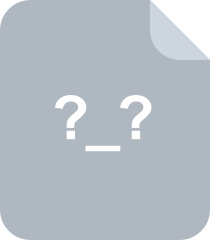
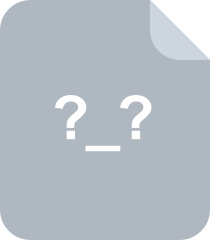
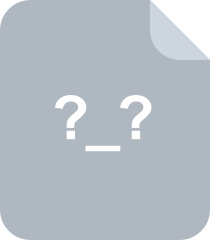
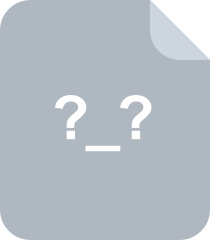
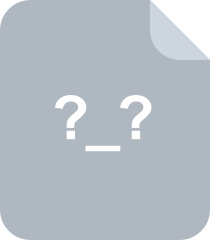
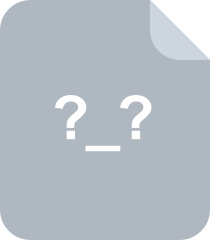
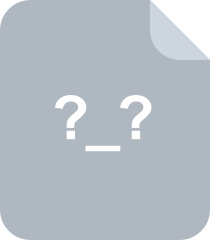
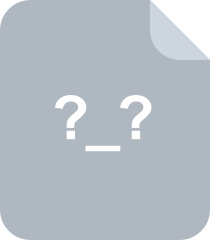
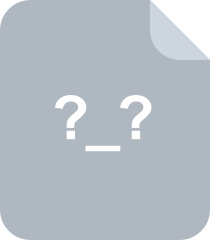
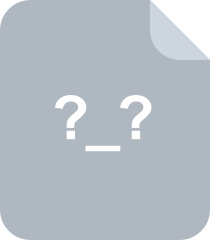
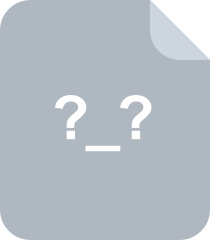
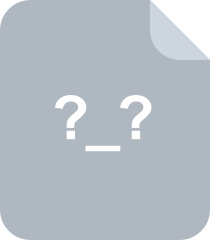
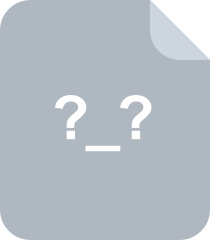
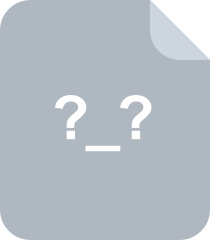
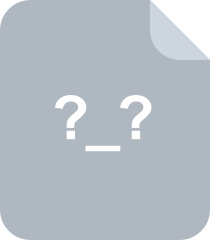
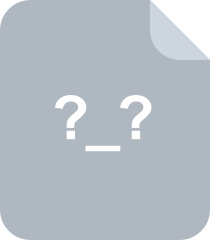
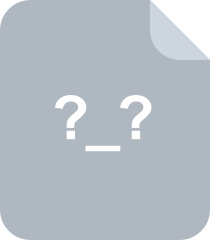
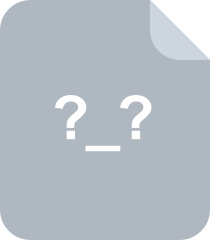
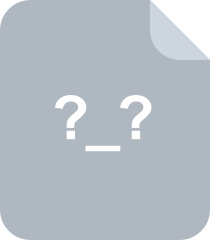
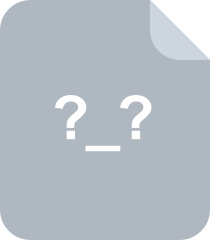
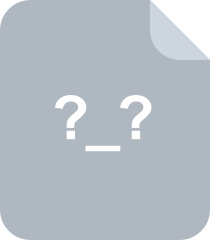
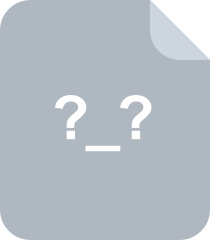
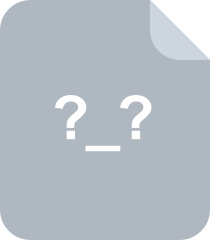
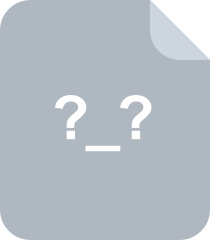
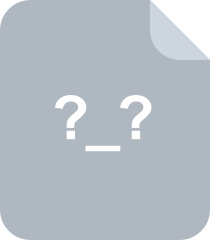
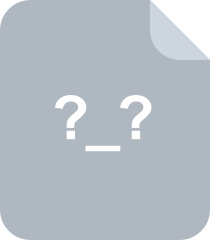
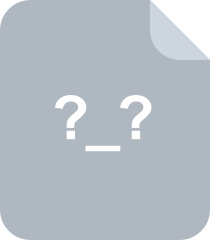
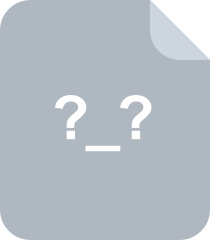
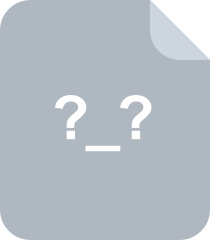
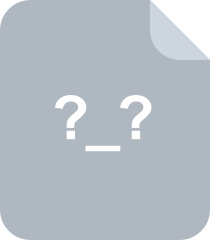
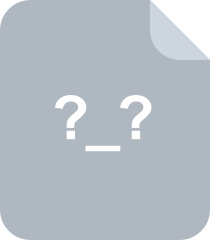
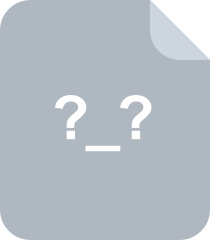
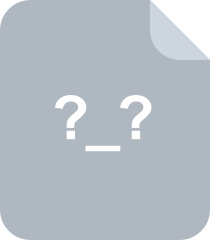
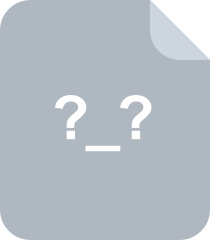
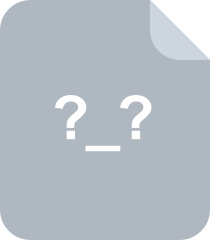
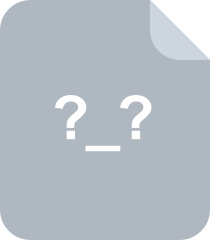
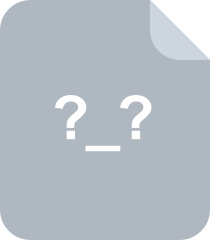
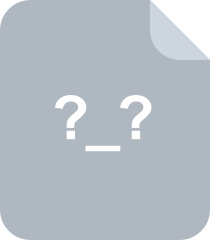
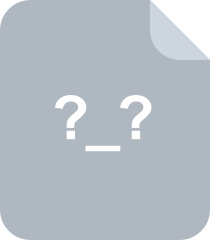
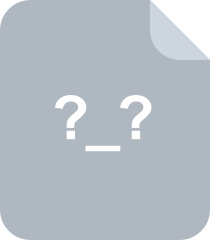
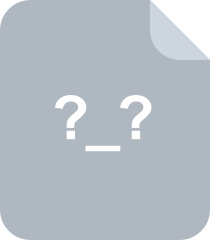
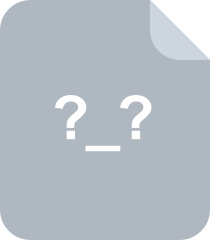
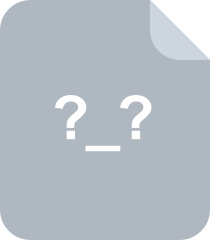
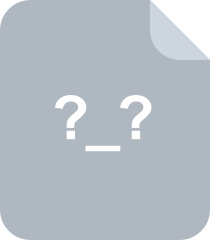
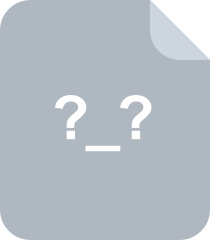
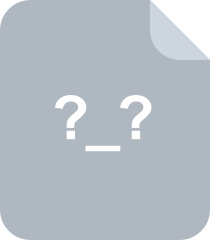
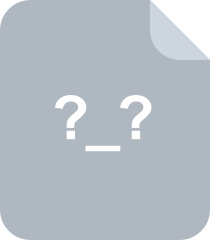
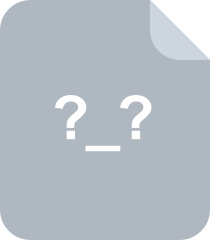
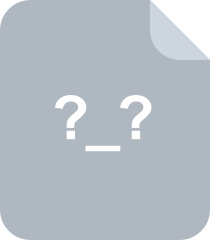
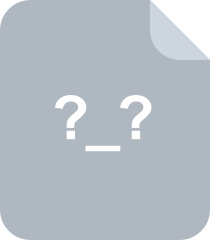
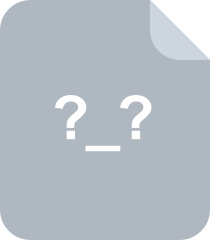
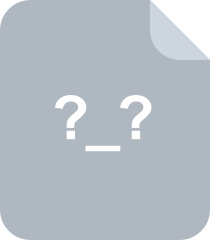
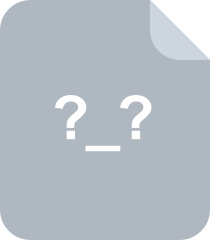
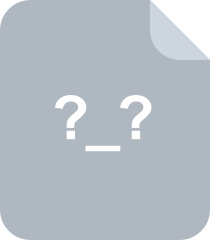
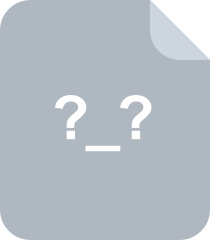
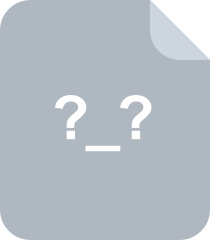
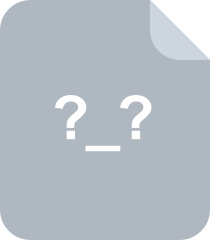
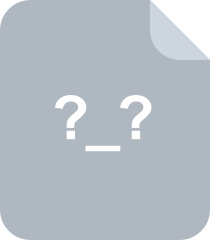
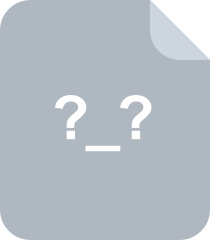
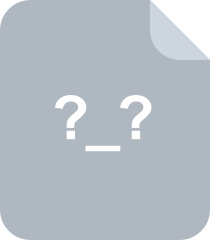
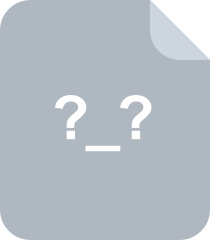
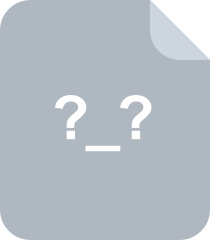
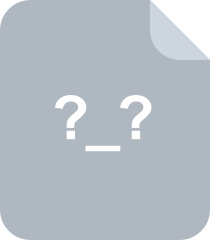
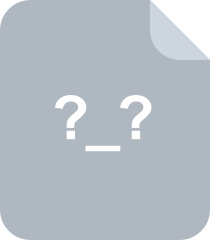
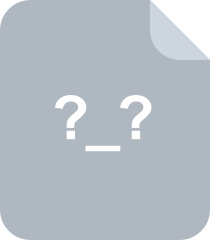
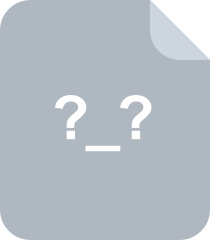
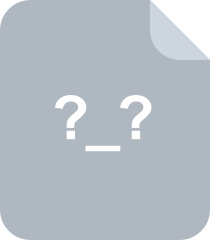
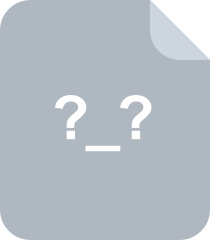
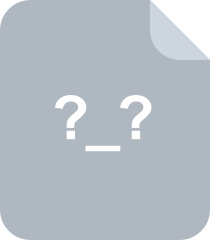
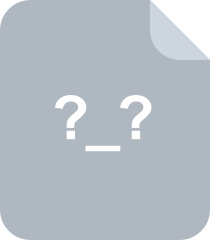
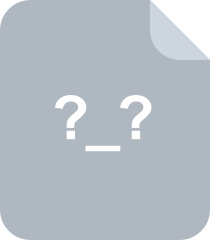
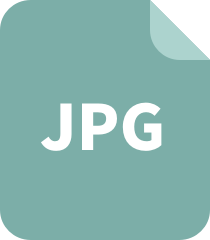
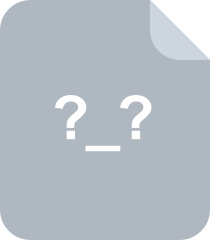
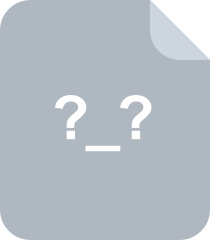
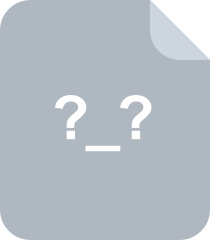
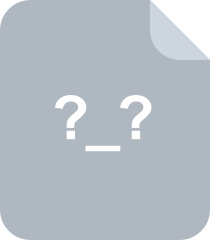
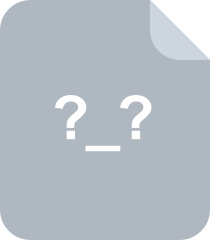
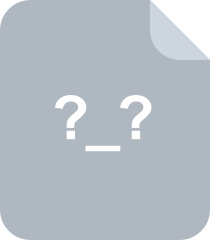
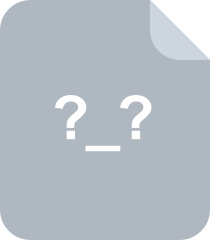
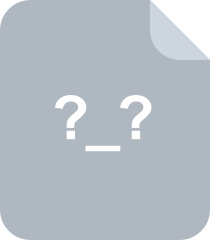
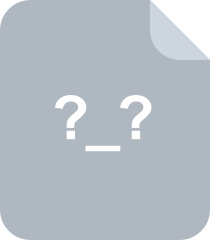
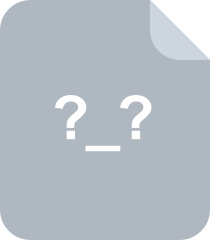
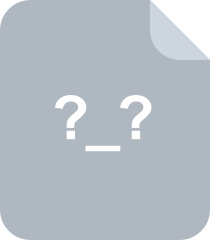
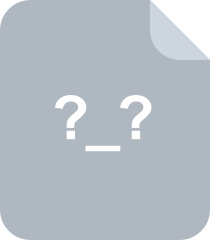
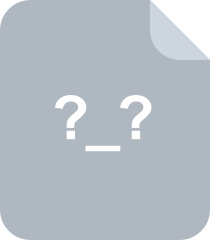
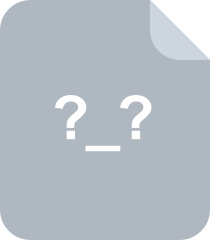
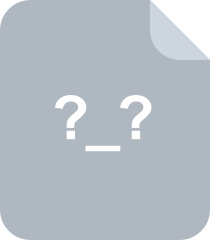
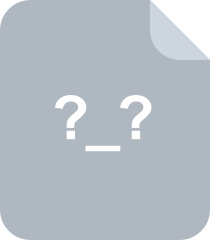
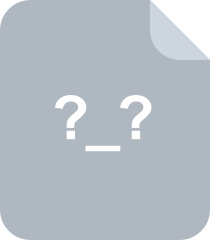
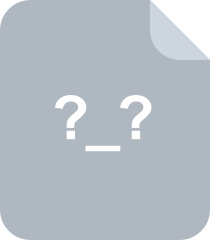
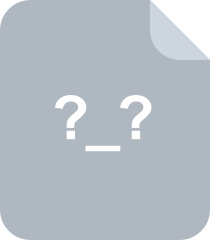
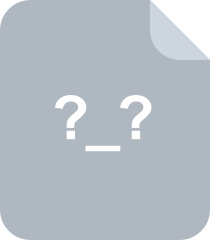
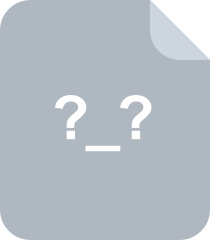
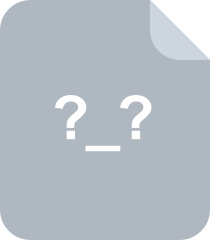
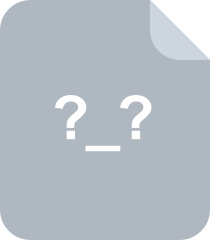
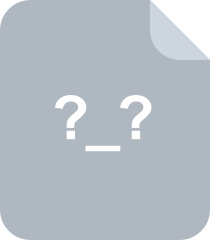
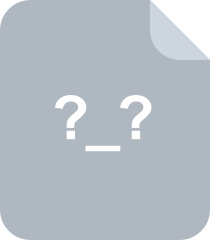
共 345 条
- 1
- 2
- 3
- 4
资源评论
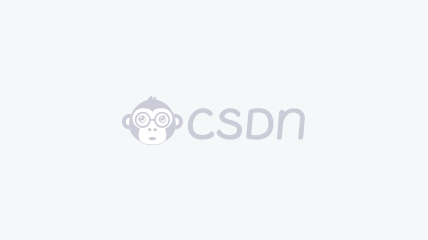

龙年行大运
- 粉丝: 1385
- 资源: 3960
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

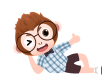
最新资源
- HTML5实现好看的游戏开发上市公司网站模板.zip
- HTML5实现好看的游戏公司官网网站模板.zip
- 国开-大数据技术导论-实验5 大数据可视化.doc
- 国开-大数据技术导论-实验4 大数据去重.doc
- 国开-大数据技术导论-实验3 网页数据获取.doc
- 国开-大数据技术导论-实验1 Linux操作系统部署.doc
- 冒泡排序,插入排序,选择排序
- (21688012)微信商城小程序
- (24517238)17 CDMA2000码分多址通信系统.zip
- (9993602)购物车小程序
- (172604420)STL常用容器1
- (173992034)完整word版-C语言程序设计(郑莉)课后习题答案.doc
- (174151238)EDFA的matlab建模,EDFA的matlab建模,EDFA的matlab建模,EDFA的matlab建模,EDFA的mat
- springboot2.x课程配套课件笔记springboot版PDF
- (174269454)C语言课程设计-考试报名管理系统
- (174517244)大一上学期C语言大作业.7z
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


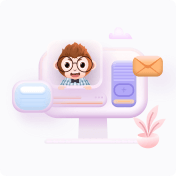
安全验证
文档复制为VIP权益,开通VIP直接复制
