[](http://travis-ci.org/dankogai/js-base64)
# base64.js
Yet another [Base64] transcoder.
[Base64]: http://en.wikipedia.org/wiki/Base64
## HEADS UP: switch to TypeScript since version 3.3
In version 3.0 `js-base64` switch to ES2015 module. That made it easy to switch to TypeScript(just renaming `base64.mjs` to `base64.ts` was almost enough). Now `base64.mjs` is compiled from `base64.ts` then `base64.js` is generated from `base64.mjs`.
## Install
```shell
$ npm install --save js-base64
```
## Usage
### In Browser
Locally…
```html
<script src="base64.js"></script>
```
… or Directly from CDN. In which case you don't even need to install.
```html
<script src="https://cdn.jsdelivr.net/npm/js-base64@3.4.4/base64.min.js"></script>
```
This good old way loads `Base64` in the global context (`window`). Though `Base64.noConflict()` is made available, you should consider using ES6 Module to avoid tainting `window`.
### As an ES6 Module
locally…
```javascript
import { Base64 } from 'js-base64';
```
```javascript
// or if you prefer no Base64 namespace
import { encode, decode } from 'js-base64';
```
or even remotely.
```html
<script type="module">
// note jsdelivr.net does not automatically minify .mjs
import { Base64 } from 'https://cdn.jsdelivr.net/npm/js-base64@3.4.4/base64.mjs';
</script>
```
```html
<script type="module">
// or if you prefer no Base64 namespace
import { encode, decode } from 'https://cdn.jsdelivr.net/npm/js-base64@3.4.4/base64.mjs';
</script>
```
### node.js (commonjs)
```javascript
const {Base64} = require('js-base64');
```
Unlike the case above, the global context is no longer modified.
You can also use [esm] to `import` instead of `require`.
[esm]: https://github.com/standard-things/esm
```javascript
require=require('esm')(module);
import {Base64} from 'js-base64';
```
## SYNOPSIS
```javascript
let latin = 'dankogai';
let utf8 = '小飼弾'
let u8s = new Uint8Array([100,97,110,107,111,103,97,105]);
Base64.encode(latin); // ZGFua29nYWk=
Base64.btoa(latin); // ZGFua29nYWk=
Base64.btoa(utf8); // raises exception
Base64.fromUint8Array(u8s); // ZGFua29nYWk=
Base64.fromUint8Array(u8s, true); // ZGFua29nYW which is URI safe
Base64.encode(utf8); // 5bCP6aO85by+
Base64.encode(utf8, true) // 5bCP6aO85by-
Base64.encodeURI(utf8); // 5bCP6aO85by-
```
```javascript
Base64.decode( 'ZGFua29nYWk=');// dankogai
Base64.atob( 'ZGFua29nYWk=');// dankogai
Base64.atob( '5bCP6aO85by+');// 'å°é£¼å¼¾' which is nonsense
Base64.toUint8Array('ZGFua29nYWk=');// u8s above
Base64.decode( '5bCP6aO85by+');// 小飼弾
// note .decodeURI() is unnecessary since it accepts both flavors
Base64.decode( '5bCP6aO85by-');// 小飼弾
```
### Built-in Extensions
By default `Base64` leaves built-in prototypes untouched. But you can extend them as below.
```javascript
// you have to explicitly extend String.prototype
Base64.extendString();
// once extended, you can do the following
'dankogai'.toBase64(); // ZGFua29nYWk=
'小飼弾'.toBase64(); // 5bCP6aO85by+
'小飼弾'.toBase64(true); // 5bCP6aO85by-
'小飼弾'.toBase64URI(); // 5bCP6aO85by- ab alias of .toBase64(true)
'小飼弾'.toBase64URL(); // 5bCP6aO85by- an alias of .toBase64URI()
'ZGFua29nYWk='.fromBase64(); // dankogai
'5bCP6aO85by+'.fromBase64(); // 小飼弾
'5bCP6aO85by-'.fromBase64(); // 小飼弾
'5bCP6aO85by-'.toUint8Array();// u8s above
```
```javascript
// you have to explicitly extend String.prototype
Base64.extendString();
// once extended, you can do the following
u8s.toBase64(); // 'ZGFua29nYWk='
u8s.toBase64URI(); // 'ZGFua29nYWk'
u8s.toBase64URL(); // 'ZGFua29nYWk' an alias of .toBase64URI()
```
```javascript
// extend all at once
Base64.extendBuiltins()
```
## `.decode()` vs `.atob` (and `.encode()` vs `btoa()`)
Suppose you have:
```
var pngBase64 =
"iVBORw0KGgoAAAANSUhEUgAAAAEAAAABCAQAAAC1HAwCAAAAC0lEQVR42mNkYAAAAAYAAjCB0C8AAAAASUVORK5CYII=";
```
Which is a Base64-encoded 1x1 transparent PNG, **DO NOT USE** `Base64.decode(pngBase64)`. Use `Base64.atob(pngBase64)` instead. `Base64.decode()` decodes to UTF-8 string while `Base64.atob()` decodes to bytes, which is compatible to browser built-in `atob()` (Which is absent in node.js). The same rule applies to the opposite direction.
Or even better, `Base64.toUint8Array(pngBase64)`.
### If you really, really need an ES5 version
You can transpiles to an ES5 that runs on IE11. Do the following in your shell.
```shell
$ make base64.es5.js
```

爬山虎的博客
- 粉丝: 4
- 资源: 8
最新资源
- Python中的数据集离群点检测技术与实践
- BAPI-特征&类&BOM&MRP3视图维护&可配置物料相关事务
- 这份PPT详细介绍了图形系统的各个方面,从硬件设备到软件系统,再到常见的图形API和库
- 芋道微服务包含工作流的sql
- CPLD,FPGA入门知识介绍
- 这份PPT介绍了图形系统的概念、组成和应用,涵盖了图形硬件、软件、API以及常见图形库等内容,帮助理解图形显示的原理和流程
- 基于小波的图像压缩技术,里面有源码和报告文档,使用python编写,可做期末大作业,下载即可运行
- 图形学是计算机图形学是利用计算机研究图形的表示、生成、处理和显示的一门重要的计算机学科分支
- Java服务端开发指南:框架选型与最佳实践
- 基于STM32的卫星GPS路径记录仪(源代码+原理图)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


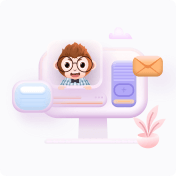