<h1 align="center">
<b>
<a href="https://axios-http.com"><img src="https://axios-http.com/assets/logo.svg" /></a><br>
</b>
</h1>
<p align="center">Promise based HTTP client for the browser and node.js</p>
<p align="center">
<a href="https://axios-http.com/"><b>Website</b></a> â¢
<a href="https://axios-http.com/docs/intro"><b>Documentation</b></a>
</p>
<div align="center">
[](https://www.npmjs.org/package/axios)
[](https://cdnjs.com/libraries/axios)
[](https://github.com/axios/axios/actions/workflows/ci.yml)
[](https://gitpod.io/#https://github.com/axios/axios)
[](https://coveralls.io/r/mzabriskie/axios)
[](https://packagephobia.now.sh/result?p=axios)
[](https://bundlephobia.com/package/axios@latest)
[](https://npm-stat.com/charts.html?package=axios)
[](https://gitter.im/mzabriskie/axios)
[](https://www.codetriage.com/axios/axios)
[](https://snyk.io/test/npm/axios)
</div>
## Table of Contents
- [Features](#features)
- [Browser Support](#browser-support)
- [Installing](#installing)
- [Package manager](#package-manager)
- [CDN](#cdn)
- [Example](#example)
- [Axios API](#axios-api)
- [Request method aliases](#request-method-aliases)
- [Concurrency ð](#concurrency-deprecated)
- [Creating an instance](#creating-an-instance)
- [Instance methods](#instance-methods)
- [Request Config](#request-config)
- [Response Schema](#response-schema)
- [Config Defaults](#config-defaults)
- [Global axios defaults](#global-axios-defaults)
- [Custom instance defaults](#custom-instance-defaults)
- [Config order of precedence](#config-order-of-precedence)
- [Interceptors](#interceptors)
- [Multiple Interceptors](#multiple-interceptors)
- [Handling Errors](#handling-errors)
- [Cancellation](#cancellation)
- [AbortController](#abortcontroller)
- [CancelToken ð](#canceltoken-deprecated)
- [Using application/x-www-form-urlencoded format](#using-applicationx-www-form-urlencoded-format)
- [URLSearchParams](#urlsearchparams)
- [Query string](#query-string-older-browsers)
- [ð Automatic serialization](#-automatic-serialization-to-urlsearchparams)
- [Using multipart/form-data format](#using-multipartform-data-format)
- [FormData](#formdata)
- [ð Automatic serialization](#-automatic-serialization-to-formdata)
- [Files Posting](#files-posting)
- [HTML Form Posting](#-html-form-posting-browser)
- [ð Progress capturing](#-progress-capturing)
- [ð Rate limiting](#-progress-capturing)
- [Semver](#semver)
- [Promises](#promises)
- [TypeScript](#typescript)
- [Resources](#resources)
- [Credits](#credits)
- [License](#license)
## Features
- Make [XMLHttpRequests](https://developer.mozilla.org/en-US/docs/Web/API/XMLHttpRequest) from the browser
- Make [http](https://nodejs.org/api/http.html) requests from node.js
- Supports the [Promise](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Promise) API
- Intercept request and response
- Transform request and response data
- Cancel requests
- Automatic transforms for [JSON](https://www.json.org/json-en.html) data
- ð Automatic data object serialization to `multipart/form-data` and `x-www-form-urlencoded` body encodings
- Client side support for protecting against [XSRF](https://en.wikipedia.org/wiki/Cross-site_request_forgery)
## Browser Support
 |  |  |  |  |  |
--- | --- | --- | --- | --- | --- |
Latest â | Latest â | Latest â | Latest â | Latest â | 11 â |
[](https://saucelabs.com/u/axios)
## Installing
### Package manager
Using npm:
```bash
$ npm install axios
```
Using bower:
```bash
$ bower install axios
```
Using yarn:
```bash
$ yarn add axios
```
Using pnpm:
```bash
$ pnpm add axios
```
Once the package is installed, you can import the library using `import` or `require` approach:
```js
import axios, {isCancel, AxiosError} from 'axios';
```
You can also use the default export, since the named export is just a re-export from the Axios factory:
```js
import axios from 'axios';
console.log(axios.isCancel('something'));
````
If you use `require` for importing, **only default export is available**:
```js
const axios = require('axios');
console.log(axios.isCancel('something'));
```
For cases where something went wrong when trying to import a module into a custom or legacy environment,
you can try importing the module package directly:
```js
const axios = require('axios/dist/browser/axios.cjs'); // browser commonJS bundle (ES2017)
// const axios = require('axios/dist/node/axios.cjs'); // node commonJS bundle (ES2017)
```
### CDN
Using jsDelivr CDN (ES5 UMD browser module):
```html
<script src="https://cdn.jsdelivr.net/npm/axios@1.1.2/dist/axios.min.js"></script>
```
Using unpkg CDN:
```html
<script src="https://unpkg.com/axios@1.1.2/dist/axios.min.js"></script>
```
## Example
> **Note** CommonJS usage
> In order to gain the TypeScript typings (for intellisense / autocomplete) while using CommonJS imports with `require()`, use the following approach:
```js
import axios from 'axios';
//const axios = require('axios'); // legacy way
// Make a request for a user with a given ID
axios.get('/user?ID=12345')
.then(function (response) {
// handle success
console.log(response);
})
.catch(function (error) {
// handle error
console.log(error);
})
.finally(function () {
// always executed
});
// Optionally the request above could also be done as
axios.get('/user', {
params: {
ID: 12345
}
})
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
})
.finally(function () {
// always executed
});
// Want to use async/await? Add the `async` keyword to your outer function/method.
async function getUser() {
try {
const response = await axios.get('/user?ID=12345');
console.log(response);
} catch (error) {
console.error(error);
}
}
```
> **Note**: `async/await` is part of ECMAScript 2017 and is not supported in Internet
> Explorer and older browsers, so use with caution.
Performing a `POST` request
```js
axios.post('/user', {
firstName: 'Fred',
lastName: 'Flintstone'
})
.then(function (respons
没有合适的资源?快使用搜索试试~ 我知道了~
node-modules.rar
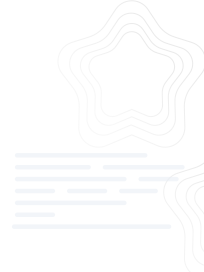
共2000个文件
js:1271个
vue:160个
ts:149个

需积分: 1 0 下载量 124 浏览量
2023-09-25
14:37:02
上传
评论
收藏 17.09MB RAR 举报
温馨提示
AI大模型
资源推荐
资源详情
资源评论
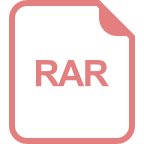
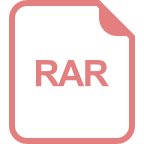
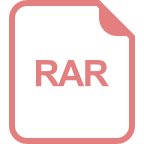
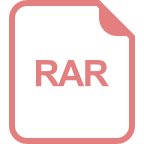
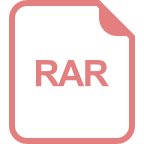
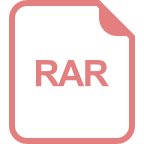
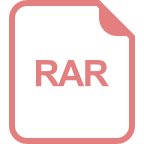
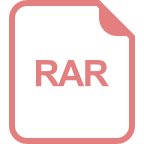
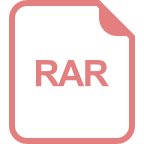
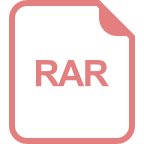
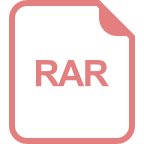
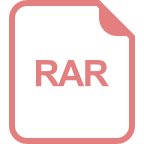
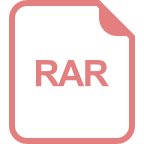
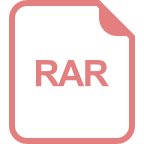
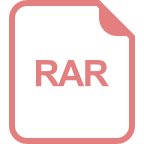
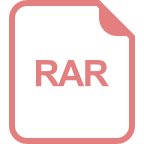
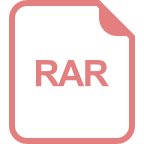
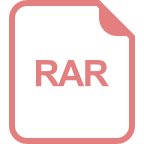
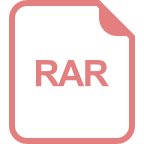
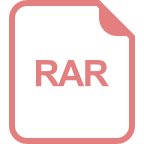
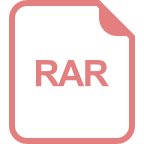
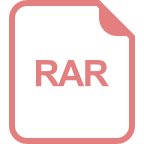
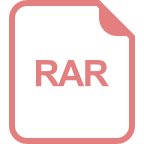
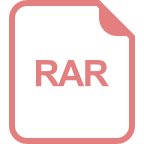
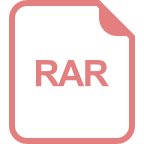
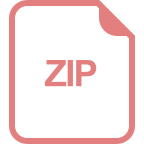
收起资源包目录

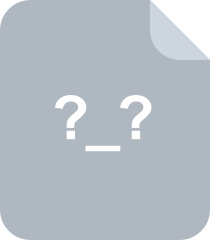
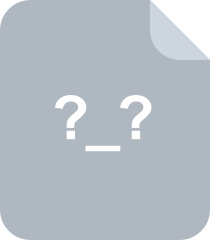
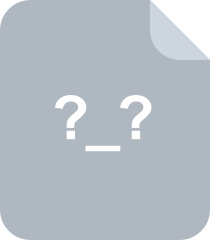
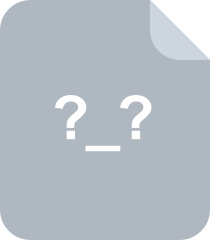
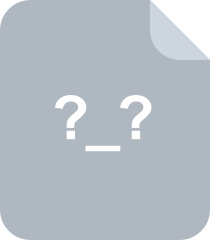
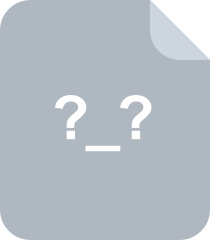
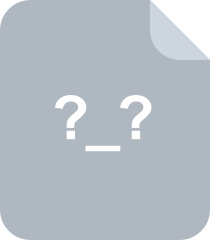
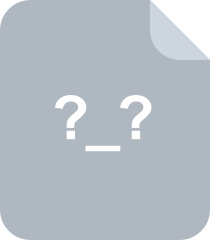
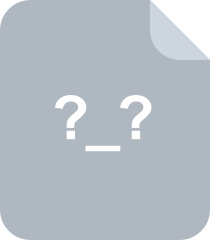
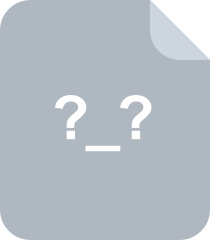
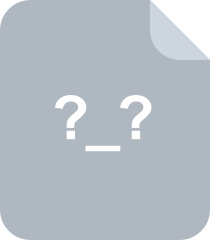
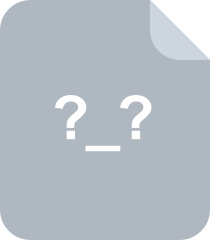
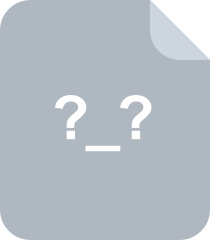
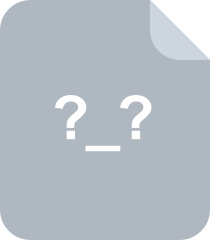
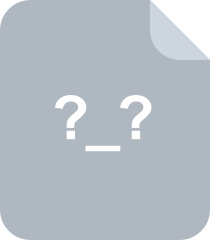
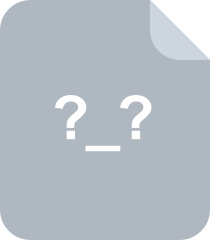
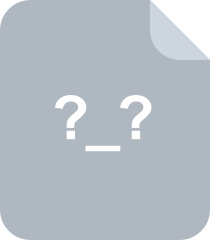
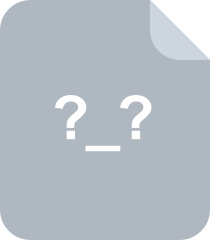
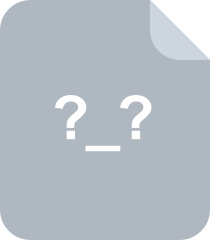
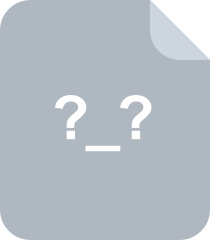
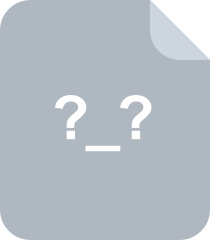
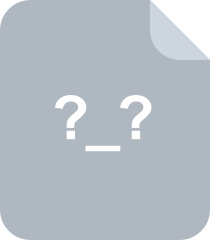
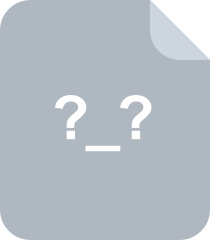
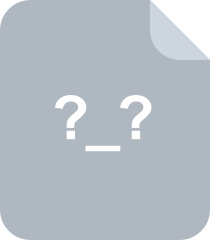
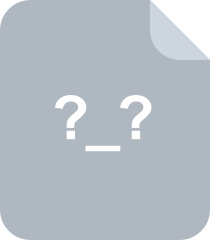
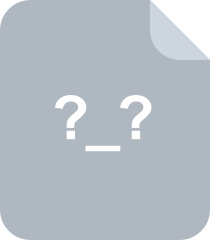
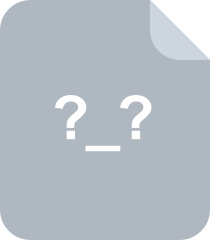
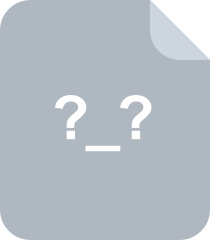
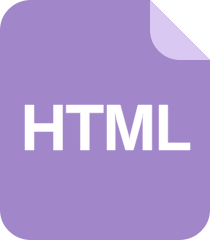
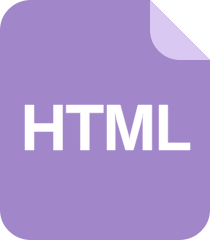
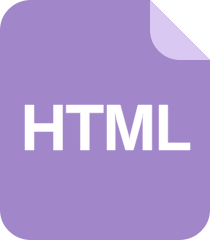
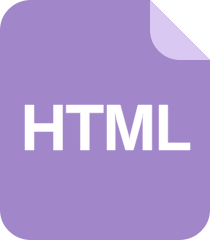
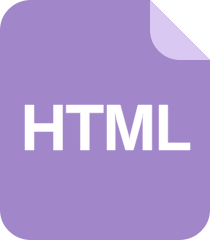
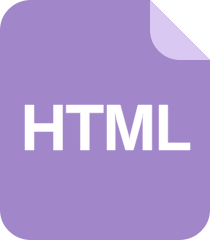
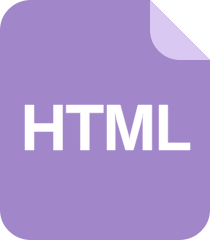
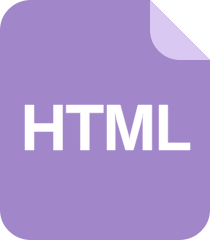
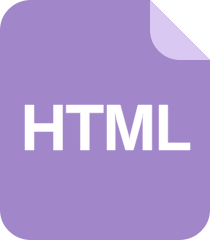
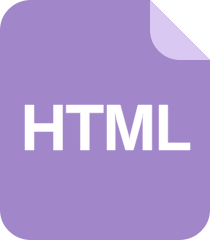
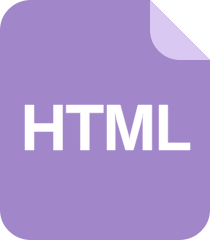
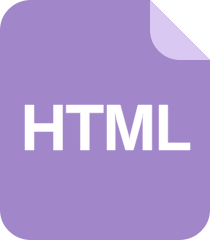
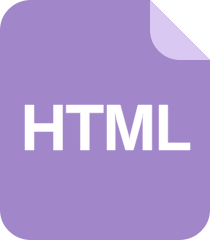
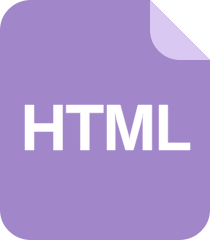
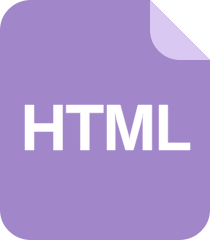
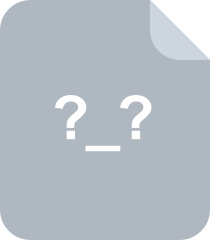
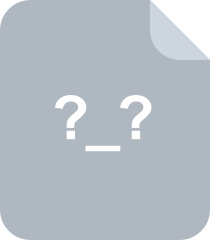
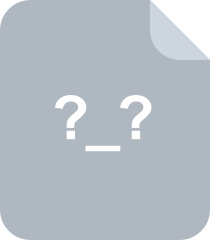
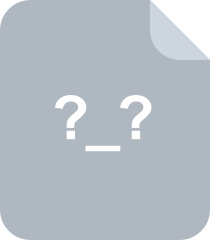
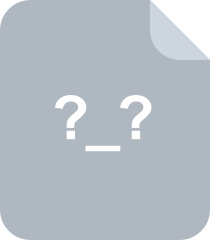
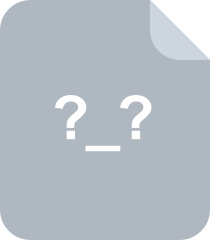
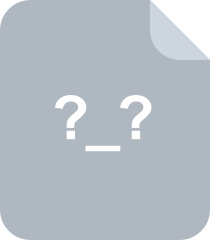
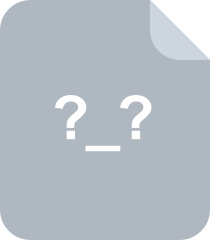
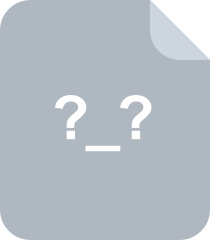
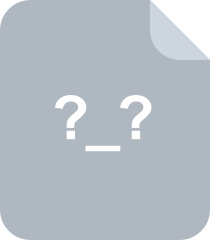
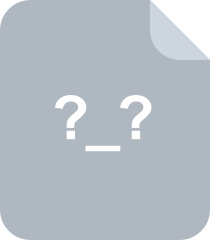
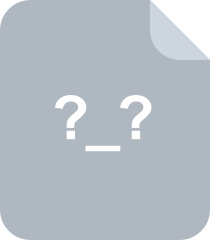
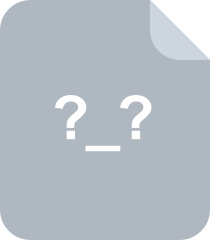
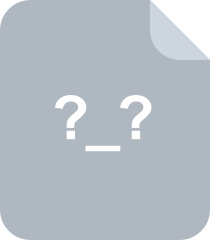
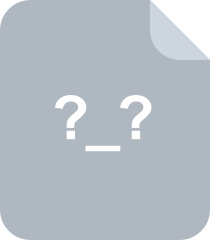
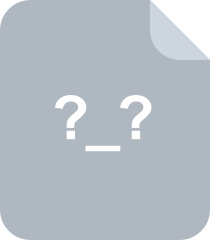
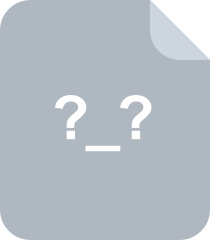
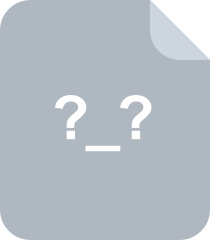
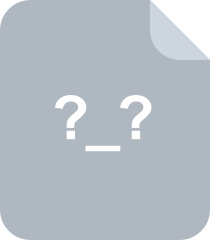
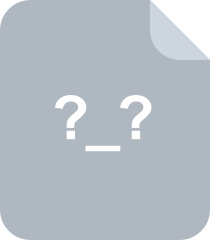
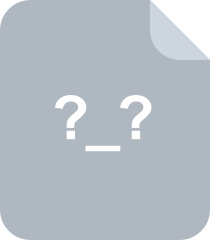
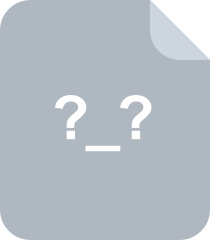
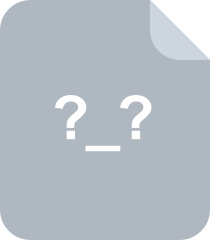
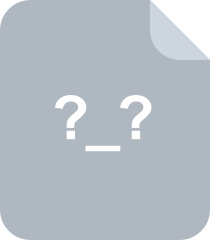
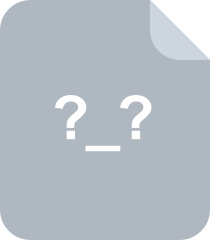
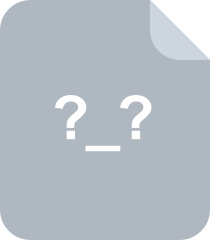
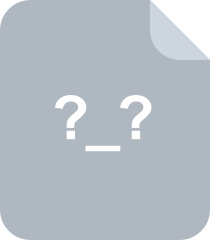
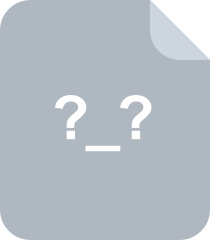
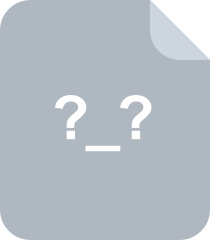
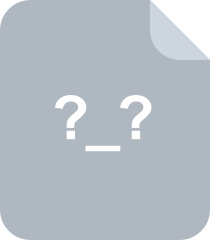
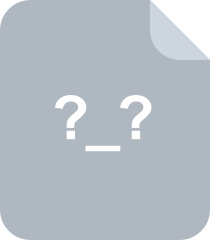
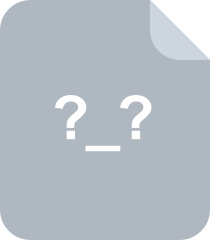
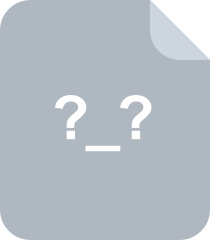
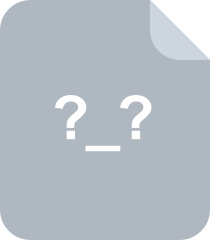
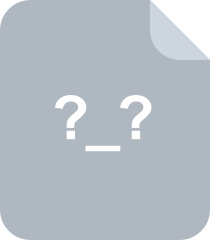
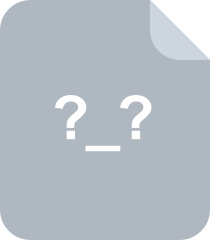
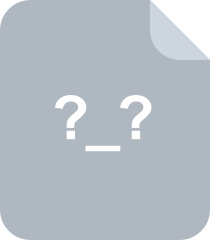
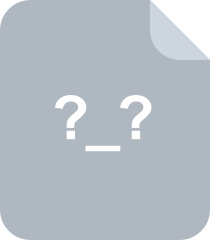
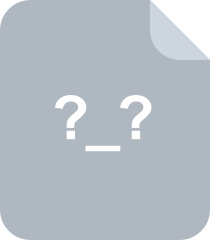
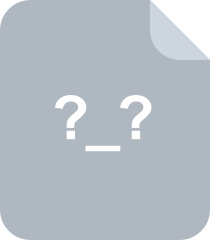
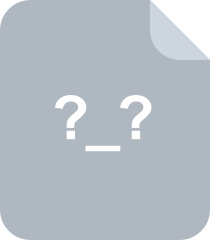
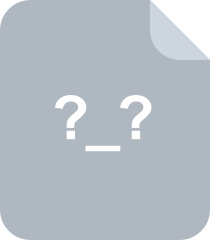
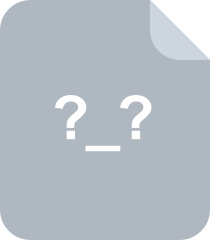
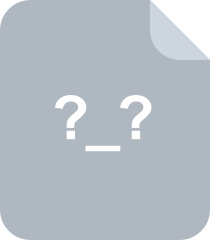
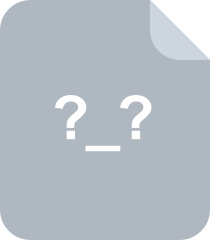
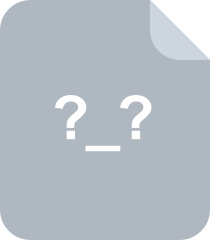
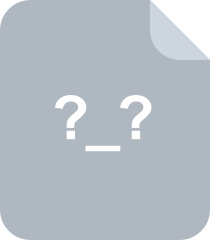
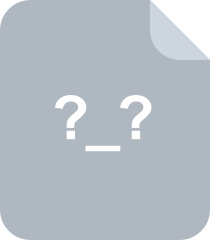
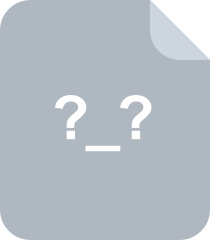
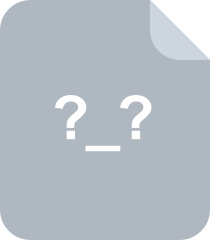
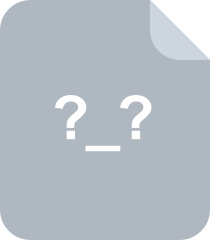
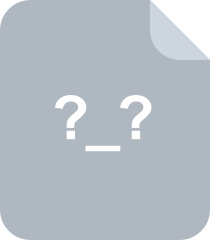
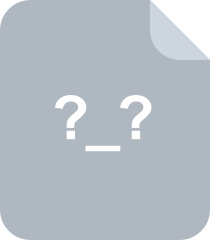
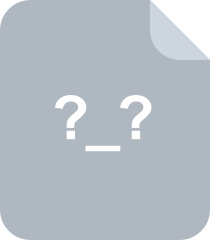
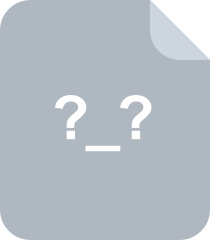
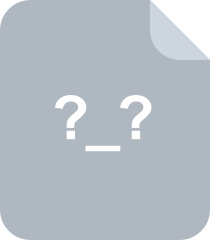
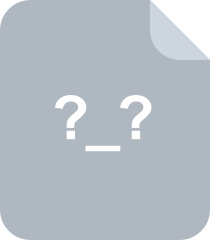
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
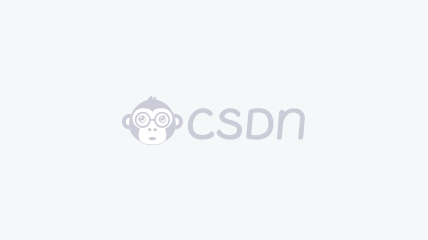

大宝贱
- 粉丝: 453
- 资源: 498
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

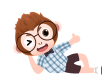
最新资源
- 用于操作 ESC,POS 打印机的 Python 库.zip
- 用于控制“Universal Robots”机器人的 Python 库.zip
- 用于控制 Broadlink RM2,3 (Pro) 遥控器、A1 传感器平台和 SP2,3 智能插头的 Python 模块.zip
- 用于接收和交互来自 Slack 的 RTM API 的事件的框架.zip
- 用于将日志发送到 LogDNA 的 Python 包.zip
- 用于将 Python 计算转换为渲染的乳胶的 Python 库 .zip
- 用于实现推荐系统的 Python 库.zip
- 用于实施无服务器最佳实践并提高开发人员速度的开发人员工具包 .zip
- 用于地理数据的 Python 工具.zip
- 全国大学生FPGA创新设计竞赛作品 泡罩包装药品质量在线检测平台.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


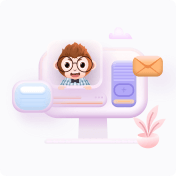
安全验证
文档复制为VIP权益,开通VIP直接复制
