在计算机科学中,中缀表达式是我们日常生活中最常见的数学表达式形式,比如 \(2 + 3 \times 4\)。然而,在计算机处理时,后缀表达式(也称为逆波兰表示法)通常更为方便,因为它不需要括号来明确运算顺序,如 \(2 3 4 * +\)。这个转换过程涉及到了栈这一数据结构。 栈是一种具有“后进先出”(LIFO, Last In First Out)特性的数据结构。在中缀表达式转后缀表达式的过程中,我们主要使用两个栈:一个运算符栈和一个中间结果栈。 我们需要定义一个模板类`List`,它代表了一个抽象的列表,包含了基本的栈操作如`push`(压入元素)、`top`(获取栈顶元素但不删除)、`pop`(弹出栈顶元素)以及检查栈是否已满或为空的方法。在这个例子中,`List`是作为`stack`类的基类,提供了抽象接口。 `stack`类继承自`List`,实现了具体的栈操作。它维护了栈的最大容量`MaxSize`、当前栈顶位置`stackTop`和存储元素的动态数组`list`。`push`方法用于向栈中添加元素,当栈满时,通过`doubleSize`方法动态扩大栈的容量。`Isfull`和`Isempty`方法分别用于检查栈是否已满和是否为空。`pop`和`top`方法则处理元素的弹出和查看。 接下来,我们引入了几个辅助函数,如`suanshifu`,用于检查字符或字符串是否为运算符,以及`Zkuohao`和`Ykuohao`,用来判断是否为左括号或右括号。 在主函数`main`中,程序从用户那里接收一个中缀表达式,然后逐字符处理。对于数字,直接将其压入中间结果栈`str2`;对于运算符,根据优先级规则决定是立即输出到后缀表达式中还是压入运算符栈`str`。遇到左括号时,直接压入运算符栈;遇到右括号,会连续弹出运算符栈的元素直到遇到左括号,并将这些运算符输出到后缀表达式中。当整个中缀表达式处理完毕,运算符栈中剩余的元素也依次输出。 在后缀表达式生成完成后,可以使用另一个栈来计算表达式的值。从后缀表达式的末尾开始,遇到数字就压入结果栈,遇到运算符就弹出栈顶的两个数字进行计算,结果再压回栈中。这样,当处理完后缀表达式,结果栈中仅剩的数字就是原中缀表达式的值。 这种转换和计算方法是基于算法的,通常称为Shunting-yard算法,由Edsger Dijkstra提出。这个算法在编译器设计、计算器程序和某些解析任务中有着广泛的应用。通过使用栈,我们可以有效地处理复杂的运算顺序和括号嵌套,确保计算的正确性。
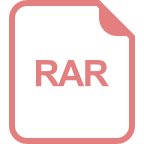
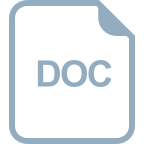
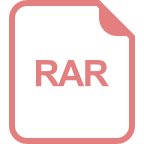
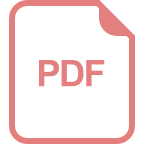
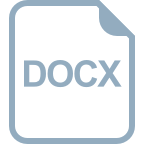
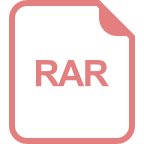
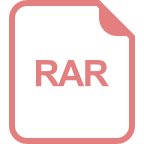
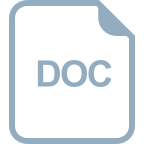
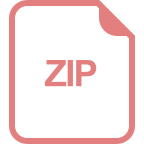
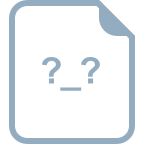
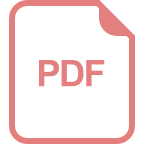
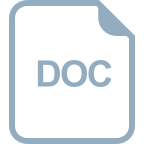
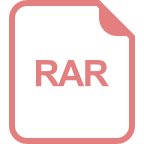
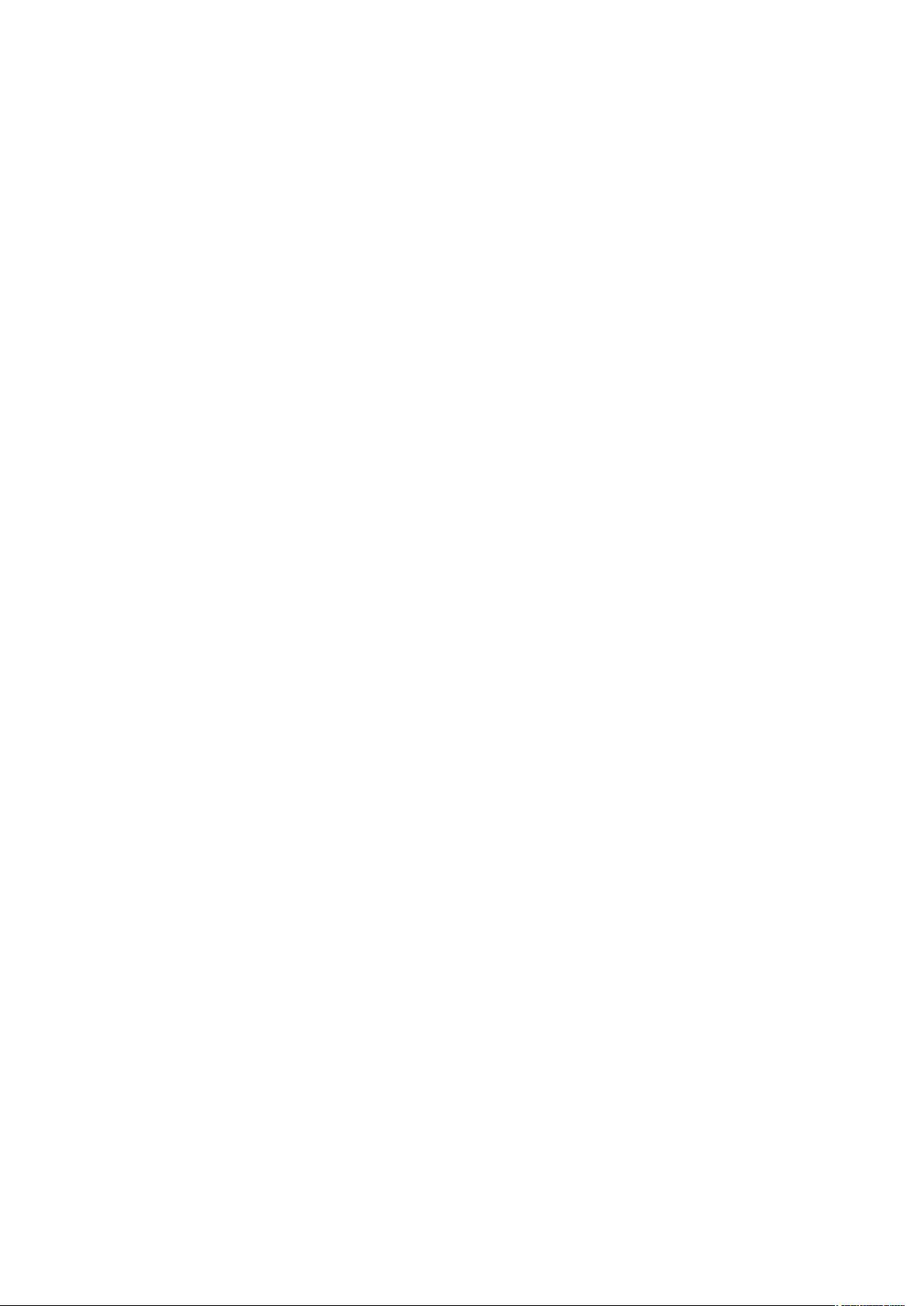
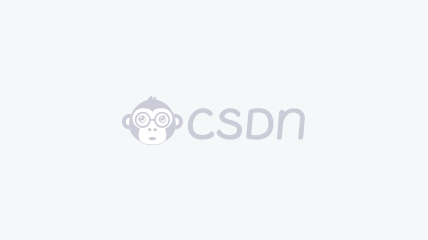

- 粉丝: 0
- 资源: 4
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

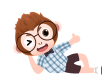
