function [trainedNet, info] = trainNetwork(varargin)
% trainNetwork Train a neural network
%
% trainedNet = trainNetwork(ds, layers, options) trains and returns a
% network trainedNet for a classification problem. ds is an
% imageDatastore with categorical labels or a MiniBatchable Datastore
% with responses, layers is an array of network layers or a LayerGraph
% and options is a set of training options.
%
% trainedNet = trainNetwork(X, Y, layers, options) trains and returns a
% network, trainedNet. The format for X depends on the input layer. For
% an image input layer, X is a numeric array of images arranged so that
% the first three dimensions are the width, height and channels, and the
% last dimension indexes the individual images. In a classification
% problem, Y specifies the labels for the images as a categorical vector.
% In a regression problem, Y contains the responses arranged as a matrix
% of size number of observations by number of responses, or a four
% dimensional numeric array, where the last dimension corresponds to the
% number of observations.
%
% trainedNet = trainNetwork(C, Y, layers, options) trains an LSTM network
% for classifcation and regression problems for sequence or time-series
% data. layers must define an LSTM network. It must begin with a sequence
% input layer. C is a cell array containing sequence or time-series
% predictors. The entries of C are D-by-S matrices where D is the number
% of values per timestep, and S is the length of the sequence. For
% sequence-to-label classification problems, Y is a categorical vector of
% labels. For sequence-to-sequence classification problems, Y is a cell
% array of categorical sequences. For sequence-to-one regression
% problems, Y is a matrix of targets. For sequence-to-sequence regression
% problems, Y is a cell array of numeric sequences. For
% sequence-to-sequence problems, the number of time steps of the
% sequences in Y must be identical to the corresponding predictor
% sequences in C. For sequence-to-sequence problems with one observation,
% C can be a matrix, and Y must be a categorical sequence of labels or a
% matrix of responses.
%
% trainedNet = trainNetwork(tbl, layers, options) trains and returns a
% network, trainedNet. For networks with an image input layer, tbl is a
% table containing predictors in the first column as either absolute or
% relative image paths or images. Responses must be in the second column
% as categorical labels for the images. In a regression problem,
% responses must be in the second column as either vectors or cell arrays
% containing 3-D arrays or in multiple columns as scalars. For networks
% with a sequence input layer, tbl is a table containing absolute or
% relative MAT file paths of predictors in the first column. For a
% sequence-to-label classification problem, the second column must be a
% categorical vector of labels. For a sequence-to-one regression problem,
% the second column must be a numeric array of responses or in multiple
% columns as scalars. For a sequence-to-sequence classification problem,
% the second column must be an absolute or relative file path to a MAT
% file with a categorical sequence. For a sequence-to-sequence regression
% problem, the second column must be an absolute or relative file path to
% a MAT file with a numeric response sequence.
%
% trainedNet = trainNetwork(tbl, responseNames, ...) trains and returns a
% network, trainedNet. responseNames is a character vector, a string
% array, or a cell array of character vectors specifying the names of the
% variables in tbl that contain the responses.
%
% [trainedNet, info] = trainNetwork(...) trains and returns a network,
% trainedNet. info contains information on training progress.
%
% Example 1:
% Train a convolutional neural network on some synthetic images
% of handwritten digits. Then run the trained network on a test
% set, and calculate the accuracy.
%
% [XTrain, YTrain] = digitTrain4DArrayData;
%
% layers = [ ...
% imageInputLayer([28 28 1])
% convolution2dLayer(5,20)
% reluLayer
% maxPooling2dLayer(2,'Stride',2)
% fullyConnectedLayer(10)
% softmaxLayer
% classificationLayer];
% options = trainingOptions('sgdm', 'Plots', 'training-progress');
% net = trainNetwork(XTrain, YTrain, layers, options);
%
% [XTest, YTest] = digitTest4DArrayData;
%
% YPred = classify(net, XTest);
% accuracy = sum(YTest == YPred)/numel(YTest)
%
% Example 2:
% Train a long short-term memory network to classify speakers of a
% spoken vowel sounds on preprocessed speech data. Then make
% predictions using a test set, and calculate the accuracy.
%
% [XTrain, YTrain] = japaneseVowelsTrainData;
%
% layers = [ ...
% sequenceInputLayer(12)
% lstmLayer(100, 'OutputMode', 'last')
% fullyConnectedLayer(9)
% softmaxLayer
% classificationLayer];
% options = trainingOptions('adam', 'Plots', 'training-progress');
% net = trainNetwork(XTrain, YTrain, layers, options);
%
% [XTest, YTest] = japaneseVowelsTestData;
%
% YPred = classify(net, XTest);
% accuracy = sum(YTest == YPred)/numel(YTest)
%
% Example 3:
% Train a network on synthetic digit data, and measure its
% accuracy:
%
% [XTrain, YTrain] = digitTrain4DArrayData;
%
% layers = [
% imageInputLayer([28 28 1], 'Name', 'input')
% convolution2dLayer(5, 20, 'Name', 'conv_1')
% reluLayer('Name', 'relu_1')
% convolution2dLayer(3, 20, 'Padding', 1, 'Name', 'conv_2')
% reluLayer('Name', 'relu_2')
% convolution2dLayer(3, 20, 'Padding', 1, 'Name', 'conv_3')
% reluLayer('Name', 'relu_3')
% additionLayer(2,'Name', 'add')
% fullyConnectedLayer(10, 'Name', 'fc')
% softmaxLayer('Name', 'softmax')
% classificationLayer('Name', 'classoutput')];
%
% lgraph = layerGraph(layers);
%
% lgraph = connectLayers(lgraph, 'relu_1', 'add/in2');
%
% plot(lgraph);
%
% options = trainingOptions('sgdm', 'Plots', 'training-progress');
% [net,info] = trainNetwork(XTrain, YTrain, lgraph, options);
%
% [XTest, YTest] = digitTest4DArrayData;
% YPred = classify(net, XTest);
% accuracy = sum(YTest == YPred)/numel(YTest)
%
% See also nnet.cnn.layer, trainingOptions, SeriesNetwork, DAGNetwork, LayerGraph.
% Copyright 2015-2018 The MathWorks, Inc.
narginchk(3,4);
try
[layersOrGraph, opts, X, Y] = iParseInputArguments(varargin{:});
[trainedNet, info] = doTrainNetwork(layersOrGraph, opts, X, Y);
catch e
iThrowCNNException( e );
end
end
function [trainedNet, info] = doTrainNetwork(layersOrGraph, opts, X, Y)
haveDAGNetwork = iHaveDAGNetwork(layersOrGraph);
analyzedLayers = iInferParameters(layersOrGraph);
layers = analyzedLayers.ExternalLayers;
internalLayers = analyzedLayers.InternalLayers;
% Validate training data
iValidateTrainingDataForProblem( X, Y, layers );
% Set desired precision
precision = nnet.internal.cnn.util.Precision('single');
% Set up and validate parallel training
isRNN = nnet.internal.cnn.util.isRNN( internalLayers );
executionSettings = nnet.internal.cnn.assembler.setupExecutionEnvironment(...
opts, isRNN, X, precision );
% Create a training dispatcher
trainingDispatcher = iCreateTrainingDataDispatcher(X, Y, opts, ...
executionSettings, layers);
% Create a validation dispatcher if validation data was passed in
validationDispatcher = iValidationDispatcher( opts, executionSettings, ...
layers );
% Assert that trai
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于MATLAB编程,用长短期神经网络LSTM进行电子密度预测,,代码完整,包含数据,有注释,方便扩展应用 1,如有疑问,不会运行,可以私信, 2,需要创新,或者修改可以扫描二维码联系博主, 3,本科及本科以上可以下载应用或者扩展, 4,内容不完全匹配要求或需求,可以联系博主扩展。
资源推荐
资源详情
资源评论
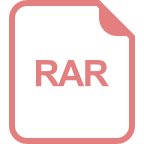
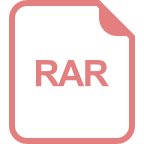
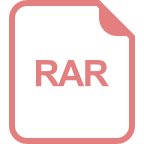
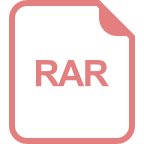
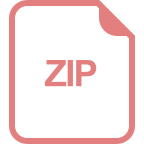
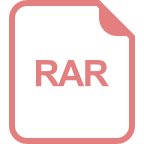
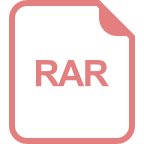
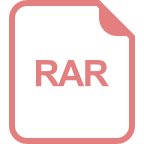
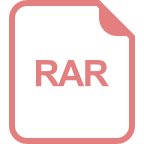
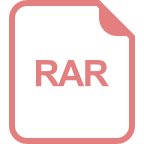
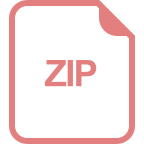
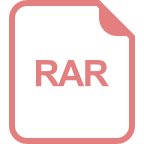
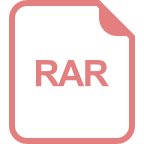
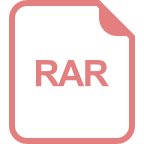
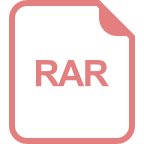
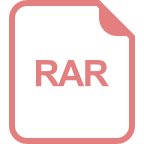
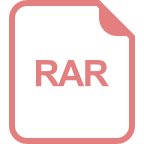
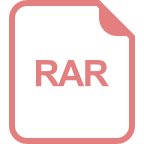
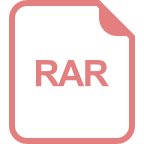
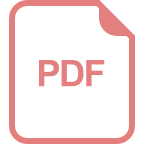
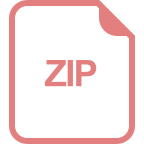
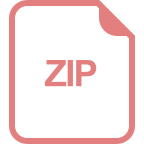
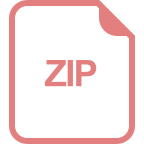
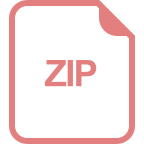
收起资源包目录

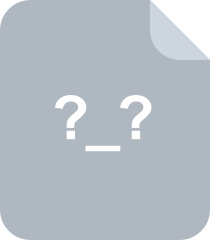
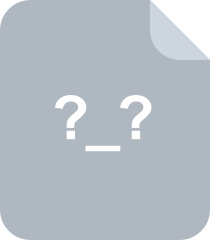
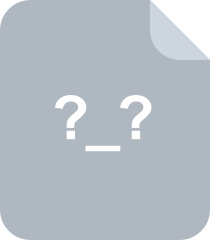
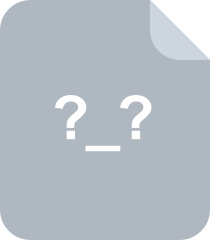
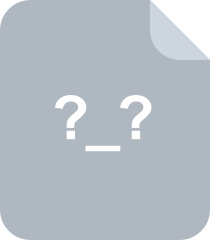
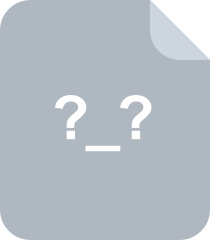
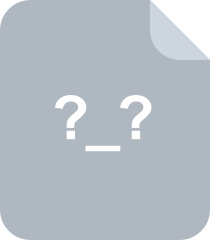
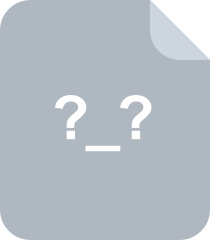
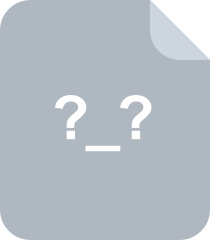
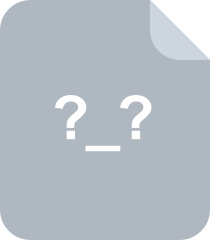
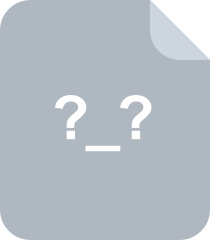
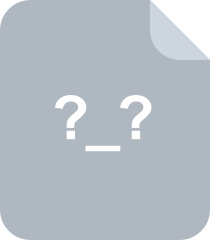
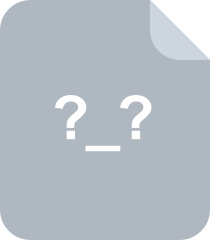
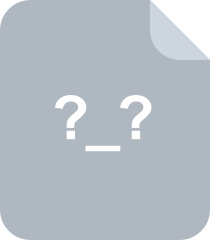
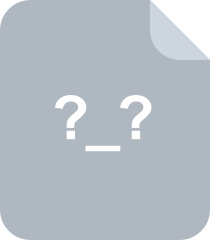
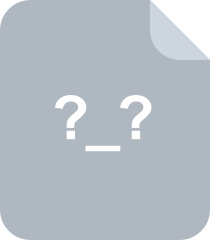
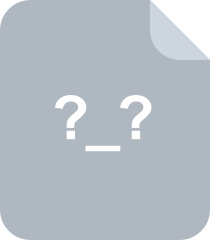
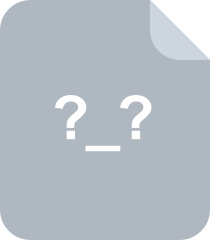
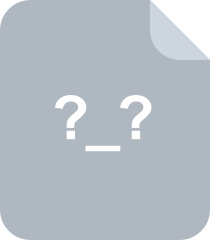
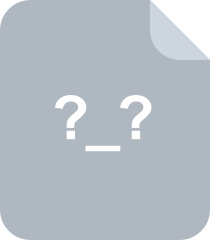
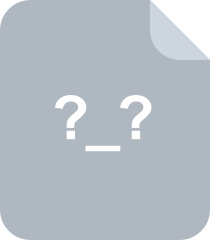
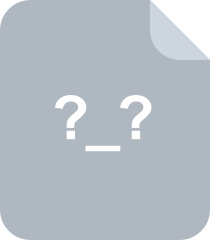
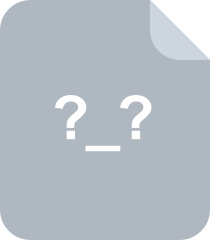
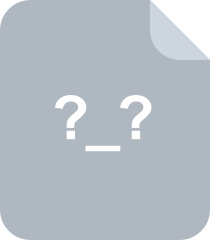
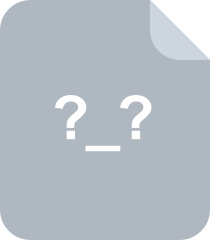
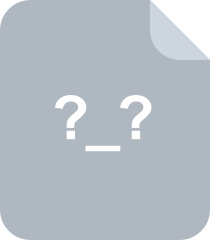
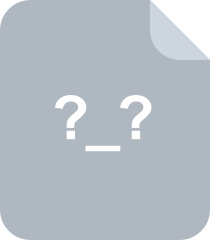
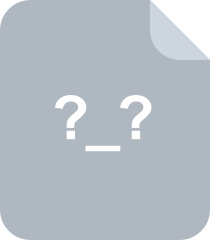
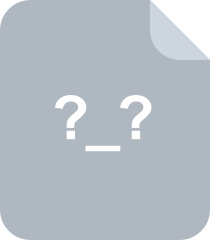
共 29 条
- 1
资源评论
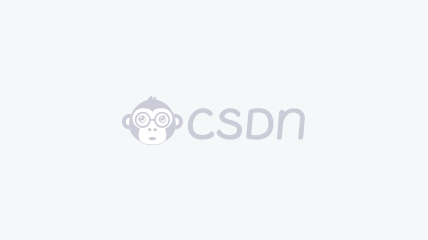


神经网络机器学习智能算法画图绘图
- 粉丝: 2436
- 资源: 598
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

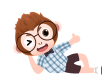
安全验证
文档复制为VIP权益,开通VIP直接复制
