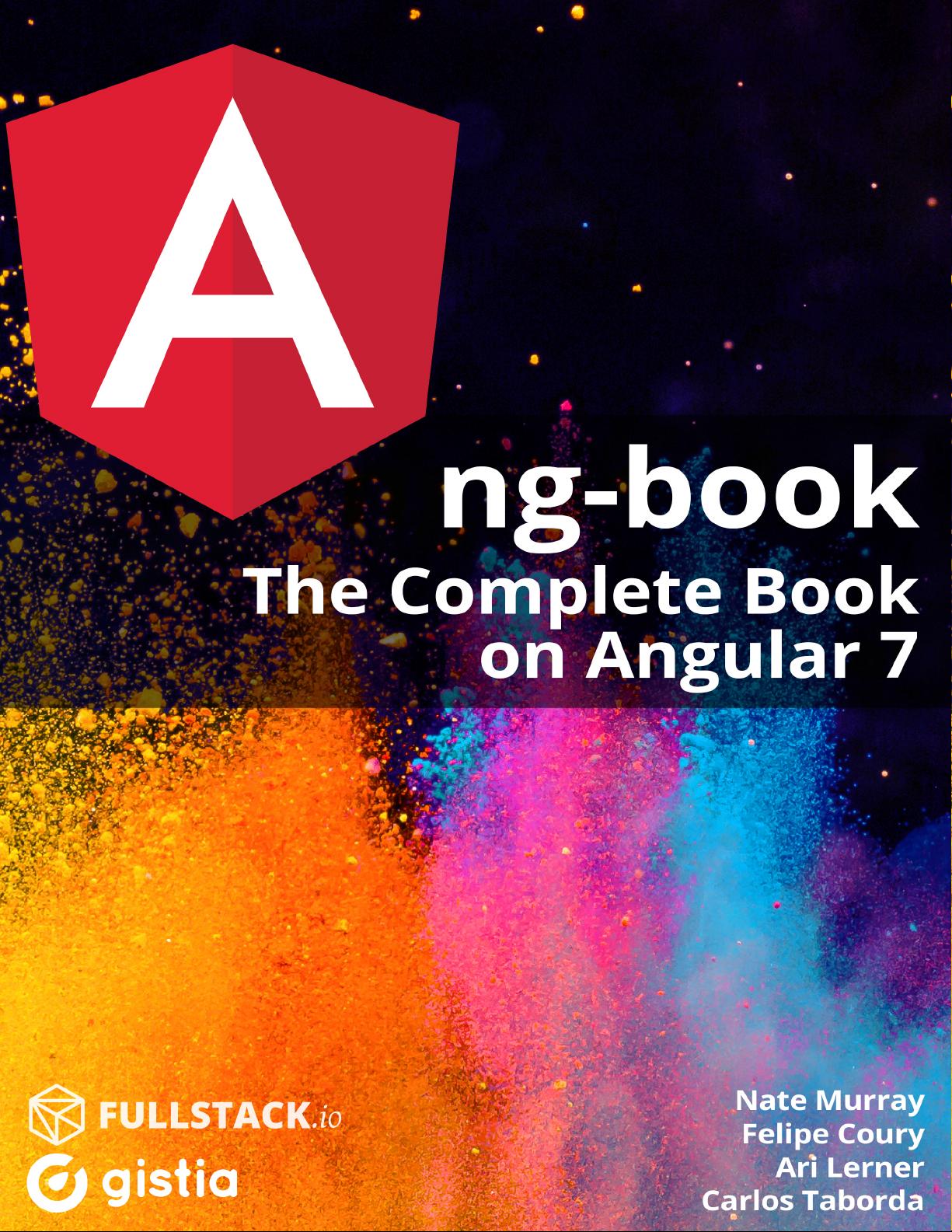
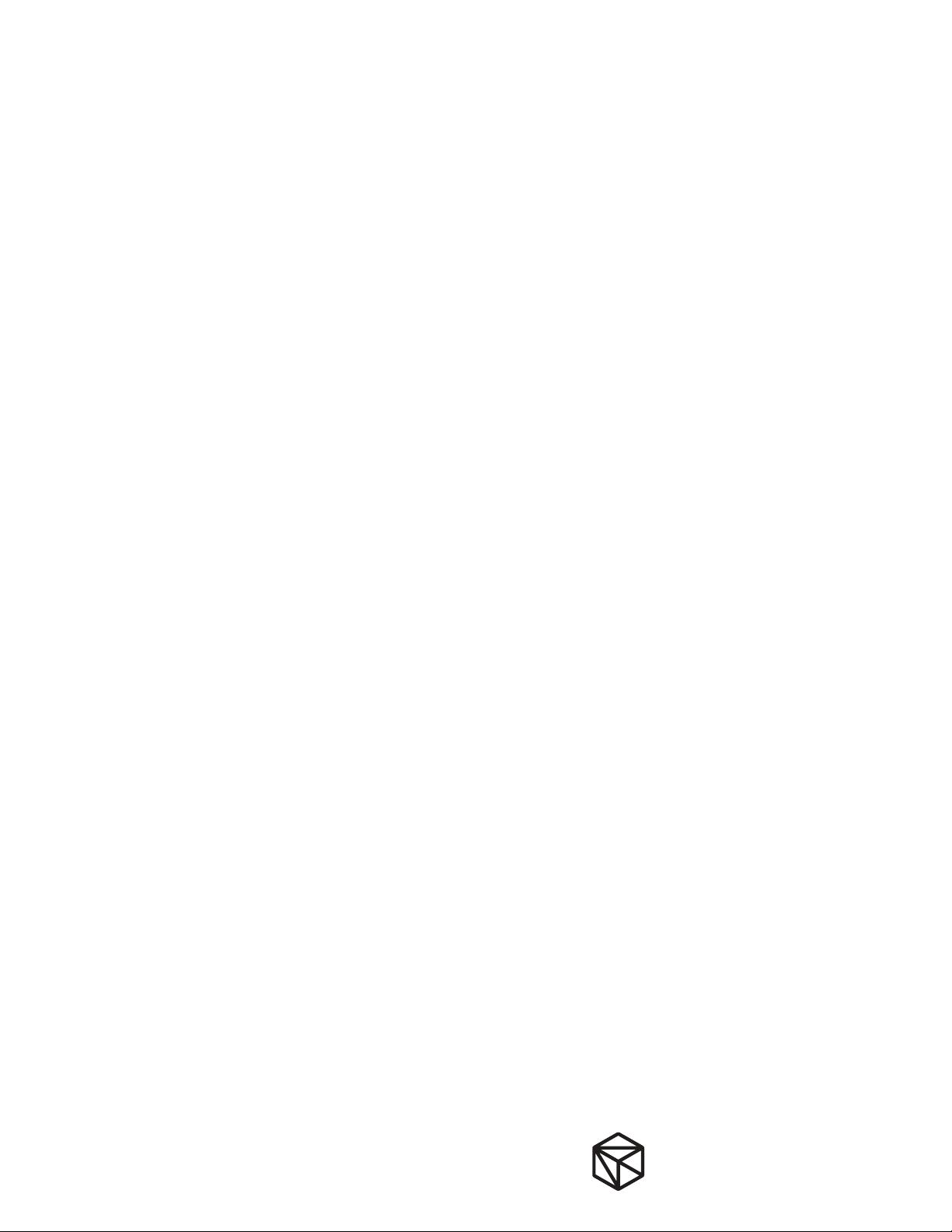
ng-book
The Complete Guide to Angular
Wri!en by Nate Murray, Felipe Coury, Ari Lerner, and Carlos Taborda
© 2018 Fullstack.io
All rights reserved. No portion of the book manuscript may be reproduced, stored in a retrieval
system, or transmi!ed in any form or by any means beyond the number of purchased copies,
except for a single backup or archival copy. "e code may be used freely in your projects,
commercial or otherwise.
"e authors and publisher have taken care in preparation of this book, but make no expressed
or implied warranty of any kind and assume no responsibility for errors or omissions. No
liability is assumed for incidental or consequential damagers in connection with or arising out
of the use of the information or programs container herein.
Published in San Francisco, California by Fullstack.io.
!
FULLSTACK
.io
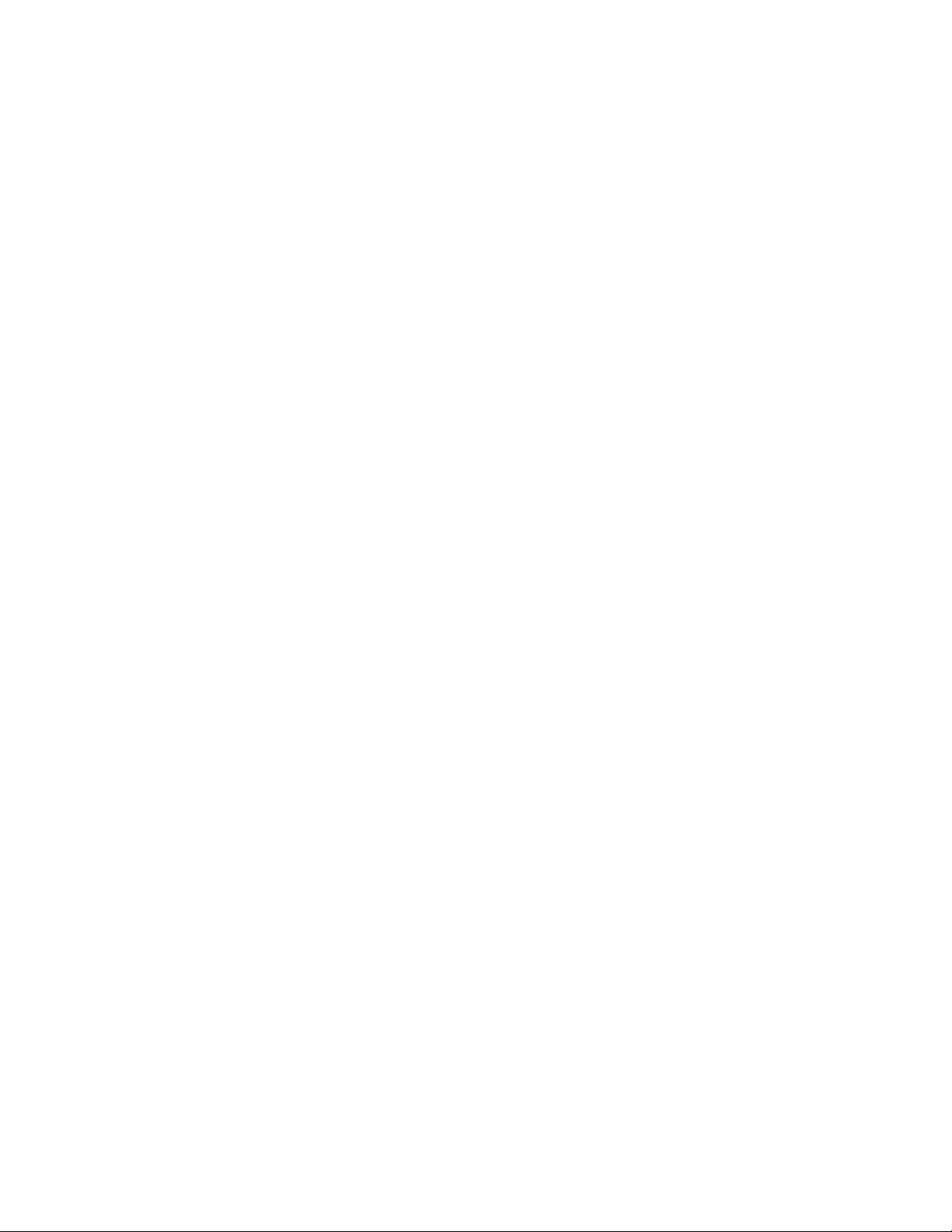
Contents
Book Revision . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1
Bug Reports . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1
Chat With The Community! . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1
Vote for New Content (new!) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1
Be notified of updates via Twitter . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1
We’d love to hear from you! . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1
How to Read This Book . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2
Running Code Examples . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2
Angular CLI . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
Code Blocks and Context . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
Code Block Numbering . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
A Word on Versioning . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
Getting Help . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
Emailing Us . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
Chapter Overview . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
Writing Your First Angular Web Application . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1
Simple Reddit Clone . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1
Getting started . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
Node.js and npm . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
TypeScript . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
Browser . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
Special instruction for Windows users . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
Angular CLI . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
Example Project . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 6
Writing Application Code . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10
Running the application . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10
Making a Component . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 12
Importing Dependencies . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 13
Component Decorators . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14
Adding a template with templateUrl . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14
Adding a template . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15
Adding CSS Styles with styleUrls . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 15
Loading Our Component . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16
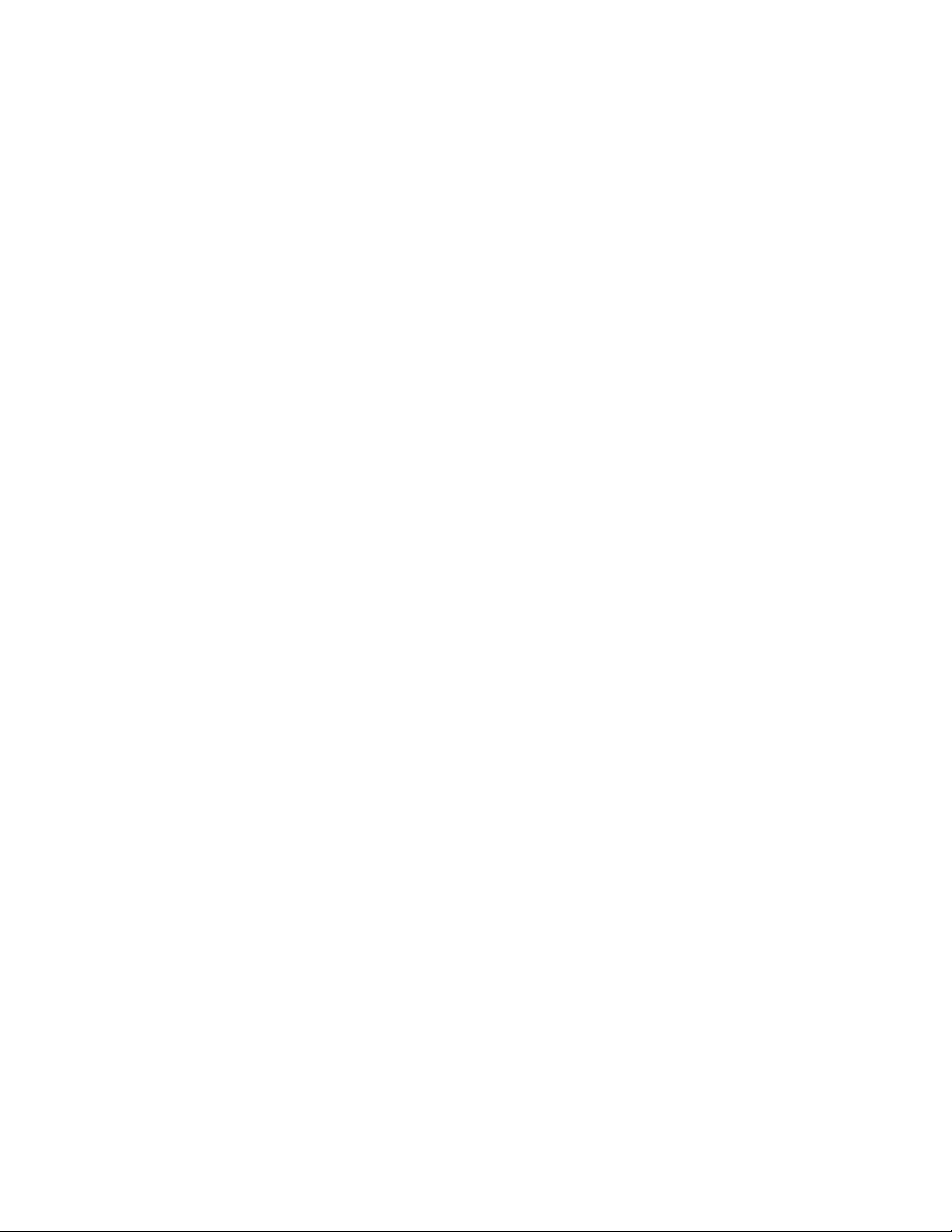
CONTENTS
Adding Data to the Component . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 17
Working With Arrays . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 20
Using the User Item Component . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 23
Rendering the UserItemComponent . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 23
Accepting Inputs . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 25
Passing an Input value . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 25
Bootstrapping Crash Course . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 27
declarations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 28
imports . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 29
providers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 29
bootstrap . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 29
Expanding our Application . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 30
Adding CSS . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 31
The Application Component . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 32
Adding Interaction . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 33
Adding the Article Component . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 37
Rendering Multiple Rows . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 45
Creating an Article class . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 45
Storing Multiple Articles . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 50
Configuring the ArticleComponent with inputs . . . . . . . . . . . . . . . . . . . . . . . 51
Rendering a List of Articles . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 54
Adding New Articles . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 56
Finishing Touches . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 57
Displaying the Article Domain . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 57
Re-sorting Based on Score . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 58
Deployment . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 59
Building Our App for Production . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 60
Uploading to a Server . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 60
Installing now . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 61
Full Code Listing . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 61
Wrapping Up . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 61
Getting Help . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 62
TypeScript . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 63
Angular is built in TypeScript . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 63
What do we get with TypeScript? . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 64
Types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 65
Trying it out with a REPL . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 66
Built-in types . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 67
Classes . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 69
Properties . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 69
Methods . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 69
Constructors . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 71