#include <bits/stdc++.h>
#include <windows.h>
using namespace std;
#define KEY_DOWN(VK_NONAME) ((GetAsyncKeyState(VK_NONAME) & 0x8000) ? 1:0)
#define M 6
char maps1[100][100] = {"************************",
"*** ***",
"*** ***",
"*** ***",
"***= f***",
"************************"};
char maps2[100][100] = {"************************",
"*** ***",
"*f ***",
"* * ***",
"*R*= ***",
"************************"};
char maps3[100][100] = {"************************",
"*** ***",
"*** ***",
"*** ***",
"***= f***",
"*******RR**RR**RR*******"};
char maps4[100][100] = {"************************",
"*** ***",
"*** ***",
"*** ***",
"***= f***",
"************RRR*********"};
char maps5[100][100] = {"************************",
"*** ***",
"*** ***",
"*** ***",
"***f =R**",
"************************"};
char maps6[100][100] = {"************************",
"**= ***",
"*** ***",
"*** ***",
"***R f***",
"************************"};
enum ConsoleColor {
BLACK = 0,
BLUE = 1,
GREEN = 2,
CYAN = 3,
RED = 4,
MAGENTA = 5,
BROWN = 6,
LIGHT_GRAY = 7,
DARK_GRAY = 8,
LIGHT_BLUE = 9,
LIGHT_GREEN = 10,
LIGHT_CYAN = 11,
LIGHT_RED = 12,
LIGHT_MAGENTA = 13,
YELLOW = 14,
WHITE = 15
};
void SetConsoleColor(ConsoleColor color) {
HANDLE hConsole = GetStdHandle(STD_OUTPUT_HANDLE);
SetConsoleTextAttribute(hConsole, color);
}
void animate_1(){
system("cls");
cout << "------------------\n";
Sleep(100);
cout << "------------------\n";
Sleep(100);
cout << "------------------\n";
Sleep(100);
cout << "------------------\n";
Sleep(100);
cout << "------------------\n";
Sleep(100);
cout << "------------------\n";
Sleep(100);
cout << "------------------\n";
Sleep(100);
system("cls");
}
void play_1(){
int lives = 5;
int startx = 0, starty = 0;
int x = 0, y = 0;
for(int i = 0; i < M; i++){
int n = strlen(maps1[i]);
for(int j = 0; j < n; j++){
if(maps1[i][j] == '='){
x = i, y = j;
startx = i, starty = j;
break;
}
}
}
{//first
bool numck = 0;
int cunx = 0;
int cuny = 0;
int up = 0;
int cntx1 = 0;
int cntx2 = 0;
int index = 0;
bool flag2 = 1;
while(1){
if(maps1[x + 1][y] != '*' && up == 0 && maps1[x + 1][y] != 'R'){
if(maps1[x + 1][y] == 'f'){
break;
}
maps1[x][y] = ' ';
x += 1;
maps1[x][y] = '=';
flag2 = 1;
}else if(maps1[x + 1][y] == 'R'){
if(lives != 0){
lives--;
system("cls");
cout <<"\n\n\n lives ¡Á" << lives;
Sleep(1000);
maps1[x][y] = ' ';
x = cunx;
y = cuny;
maps1[x][y] = '=';
flag2 = 1;
}else{
lives = 5;
cout << "\n\n\n No lives! \n\n\n";
Sleep(1000);
maps1[x][y] = ' ';
maps1[startx][starty] = '=';
x = startx, y = starty;
flag2 = 1;
}
}
if(up == 1 && index <= 1 && maps1[x - 1][y] != '*' && maps1[x - 1][y] != 'f'){
maps1[x][y] = ' ';
maps1[x - 1][y] = '=';
x--;
index++;
flag2 = 1;
}else if(maps1[x - 1][y] == 'f'){
break;
}else{
up = 0, index = 0;
}
if(y == 6 && !numck){
maps1[5][7] = 'R';
maps1[5][8] = 'R';
flag2 = 1;
numck = 1;
}
if(flag2){
system("cls");
cout << "level 1:\n";
for(int i = 0; i < M; i++){
int n = strlen(maps1[i]);
for(int j = 0; j < n; j++){
if(j < n){
if(maps1[i][j] == 'R'){
SetConsoleColor(RED);
cout << "¨";
}else if(maps1[i][j] == 'f'){
SetConsoleColor(GREEN);
cout << "f";
}
else{
SetConsoleColor(WHITE);
cout << maps1[i][j];
}
}
}
cout << '\n';
}
flag2 = 0;
}
Sleep(25);
if(KEY_DOWN(87) && maps1[x + 1][y] == '*'){
up = 1;
}
if(KEY_DOWN(65) && y > 0 && maps1[x][y - 1] != '*'){
if(maps1[x][y - 1] == 'f'){
break;
}
maps1[x][y] = ' ';
y--;
cntx2++;
maps1[x][y] = '=';
flag2 = 1;
}
if(KEY_DOWN(68) && maps1[x][y + 1] != '*'){
if(maps1[x][y + 1] == 'f'){
return;
}
maps1[x][y] = ' ';
y++;
cntx1++;
maps1[x][y] = '=';
flag2 = 1;
}
if(KEY_DOWN(67)){
cunx = x;
cuny = y;
}
}
}
}
void play_2(){
int lives = 5;
int startx = 0, starty = 0;
int x = 0, y = 0;
for(int i = 0; i < M; i++){
int n = strlen(maps2[i]);
for(int j = 0; j < n; j++){
if(maps2[i][j] == '='){
x = i, y = j;
startx = i, starty = j;
break;
}
}
}
{//second
int cunx = 0;
int cuny = 0;
int up = 0;
int cntx1 = 0;
int cntx2 = 0;
int index = 0;
bool flag2 = 1;
while(1){
if(maps2[x + 1][y] != '*' && up == 0 && maps2[x + 1][y] != 'R'){
if(maps2[x + 1][y] == 'f'){
break;
}
maps2[x][y] = ' ';
x += 1;
maps2[x][y] = '=';
flag2 = 1;
}else if(maps2[x + 1][y] == 'R'){
if(lives != 0){
lives--;
system("cls");
cout <<"\n\n\n lives ¡Á" << lives;
Sleep(1000);
maps2[x][y] = ' ';
x = cunx;
y = cuny;
maps2[x][y] = '=';
flag2 = 1;
}else{
lives = 5;
cout << "\n\n\n No lives! \n\n\n";
Sleep(1000);
maps2[x][y] = ' ';
maps2[startx][starty] = '=';
x = startx, y = starty;
flag2 = 1;
}
}
if(up == 1 && index <= 1 && maps2[x - 1][y] != '*' && maps2[x - 1][y] != 'f'){
maps2[x][y] = ' ';
maps2[x - 1][y] = '=';
x--;
index++;
flag2 = 1;
}else if(maps2[x - 1][y] == 'f'){
break;
}else{
up = 0, index = 0;
}
if(flag2){
system("cls");
cout << "level 2:\n";
for(int i = 0; i < M; i++){
int n = strlen(maps2[i]);
for(int j = 0; j < n; j++){
if(j < n){
if(i == 2 && j == 2){
SetConsoleColor(WHITE);
cout << '*';
}
else if(maps2[i][j] == 'R'){
SetConsoleColor(RED);
cout << "¨";
}
else if(maps2[i][j] == 'f'){
SetConsoleColor(GREEN);
cout << "f";
}
else{
SetConsoleColor(WHITE);
cout << maps2[i][j];
}
}
}
cout << '\n';
}
flag2 = 0;
}
Sleep(25);
if(KEY_DOWN(87) && maps2[x + 1][y] == '*'){
up = 1;
}
if(KEY_DOWN(65) && y > 0 && maps2[x][y - 1] != '*'){
if(maps2[x][y - 1] == 'f'){
break;
}
maps2[x][y] = ' ';
y--;
cntx2++;
maps2[x][y] = '=';
flag2 = 1;
}
if(KEY_DOWN(68) && maps2[x][y + 1] != '*'){
if(maps2[x][y + 1] == 'f'){
return;
}
maps2[x][y] = ' ';
y++;
cntx1++;
maps2[x][y] = '=';
flag2 = 1;
}
if(KEY_DOWN(67)){
cunx = x;
cuny = y;
}
}
}
}
void play_3(){
int lives = 5;
int startx = 0, starty = 0;
int x = 0, y = 0;
for(int i = 0; i < M; i++){
int n = strlen(maps3[i]);
for(int j = 0; j < n; j++){
if(maps3[i][j] == '='){
x = i, y = j;
startx = i, starty = j;
break;
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
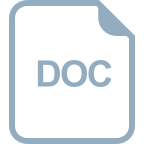
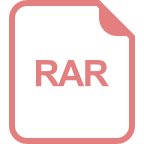
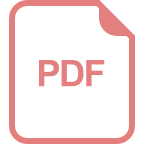
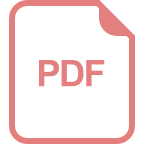
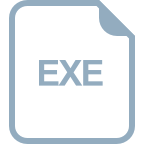
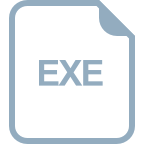
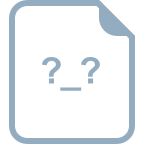
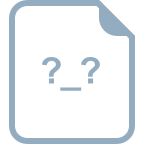
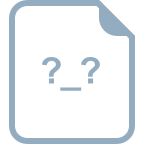
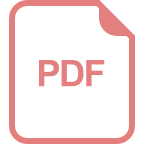
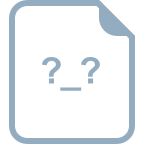
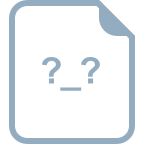
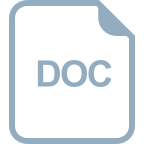
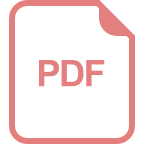
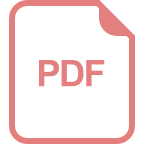
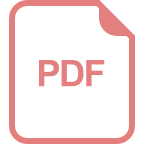
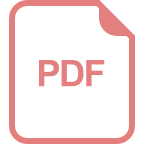
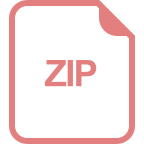
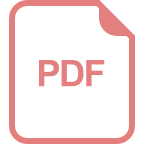
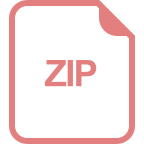
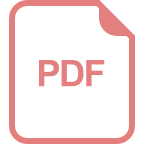
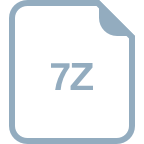
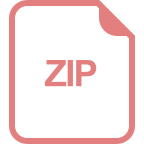
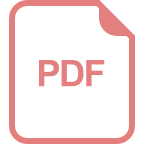
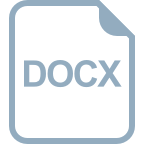
收起资源包目录


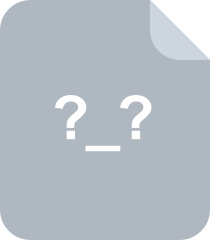
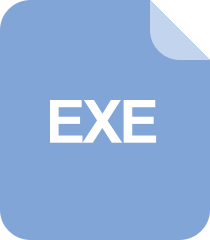
共 2 条
- 1
资源评论
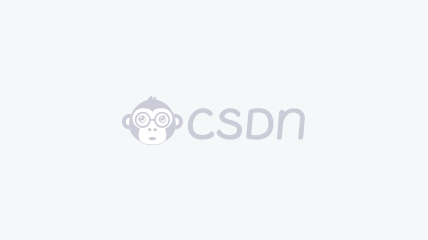

本人沙都不是
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

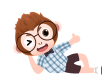
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


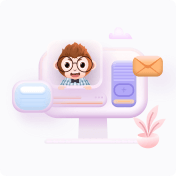
安全验证
文档复制为VIP权益,开通VIP直接复制
