/* Include files */
#include <stddef.h>
#include "blas.h"
#include "PID2_sfun.h"
#include "c3_PID2.h"
#include "mwmathutil.h"
#define CHARTINSTANCE_CHARTNUMBER (chartInstance->chartNumber)
#define CHARTINSTANCE_INSTANCENUMBER (chartInstance->instanceNumber)
#include "PID2_sfun_debug_macros.h"
#define _SF_MEX_LISTEN_FOR_CTRL_C(S) sf_mex_listen_for_ctrl_c(sfGlobalDebugInstanceStruct,S);
/* Type Definitions */
/* Named Constants */
#define CALL_EVENT (-1)
/* Variable Declarations */
/* Variable Definitions */
static real_T _sfTime_;
static const char * c3_debug_family_names[33] = { "l", "m", "g", "Ix", "Iy",
"Iz", "Jr", "d", "b", "w2", "w4", "w1", "w3", "uu", "nargin", "nargout", "u1",
"u2", "u3", "u4", "p", "q", "r", "p1", "q1", "r1", "u5", "dx2", "dy2", "dz2",
"dp2", "dq2", "dr2" };
/* Function Declarations */
static void initialize_c3_PID2(SFc3_PID2InstanceStruct *chartInstance);
static void initialize_params_c3_PID2(SFc3_PID2InstanceStruct *chartInstance);
static void enable_c3_PID2(SFc3_PID2InstanceStruct *chartInstance);
static void disable_c3_PID2(SFc3_PID2InstanceStruct *chartInstance);
static void c3_update_debugger_state_c3_PID2(SFc3_PID2InstanceStruct
*chartInstance);
static const mxArray *get_sim_state_c3_PID2(SFc3_PID2InstanceStruct
*chartInstance);
static void set_sim_state_c3_PID2(SFc3_PID2InstanceStruct *chartInstance, const
mxArray *c3_st);
static void finalize_c3_PID2(SFc3_PID2InstanceStruct *chartInstance);
static void sf_gateway_c3_PID2(SFc3_PID2InstanceStruct *chartInstance);
static void c3_chartstep_c3_PID2(SFc3_PID2InstanceStruct *chartInstance);
static void initSimStructsc3_PID2(SFc3_PID2InstanceStruct *chartInstance);
static void init_script_number_translation(uint32_T c3_machineNumber, uint32_T
c3_chartNumber, uint32_T c3_instanceNumber);
static const mxArray *c3_sf_marshallOut(void *chartInstanceVoid, void *c3_inData);
static real_T c3_emlrt_marshallIn(SFc3_PID2InstanceStruct *chartInstance, const
mxArray *c3_dr2, const char_T *c3_identifier);
static real_T c3_b_emlrt_marshallIn(SFc3_PID2InstanceStruct *chartInstance,
const mxArray *c3_u, const emlrtMsgIdentifier *c3_parentId);
static void c3_sf_marshallIn(void *chartInstanceVoid, const mxArray
*c3_mxArrayInData, const char_T *c3_varName, void *c3_outData);
static void c3_info_helper(const mxArray **c3_info);
static const mxArray *c3_emlrt_marshallOut(const char * c3_u);
static const mxArray *c3_b_emlrt_marshallOut(const uint32_T c3_u);
static const mxArray *c3_b_sf_marshallOut(void *chartInstanceVoid, void
*c3_inData);
static int32_T c3_c_emlrt_marshallIn(SFc3_PID2InstanceStruct *chartInstance,
const mxArray *c3_u, const emlrtMsgIdentifier *c3_parentId);
static void c3_b_sf_marshallIn(void *chartInstanceVoid, const mxArray
*c3_mxArrayInData, const char_T *c3_varName, void *c3_outData);
static uint8_T c3_d_emlrt_marshallIn(SFc3_PID2InstanceStruct *chartInstance,
const mxArray *c3_b_is_active_c3_PID2, const char_T *c3_identifier);
static uint8_T c3_e_emlrt_marshallIn(SFc3_PID2InstanceStruct *chartInstance,
const mxArray *c3_u, const emlrtMsgIdentifier *c3_parentId);
static void init_dsm_address_info(SFc3_PID2InstanceStruct *chartInstance);
/* Function Definitions */
static void initialize_c3_PID2(SFc3_PID2InstanceStruct *chartInstance)
{
chartInstance->c3_sfEvent = CALL_EVENT;
_sfTime_ = sf_get_time(chartInstance->S);
chartInstance->c3_is_active_c3_PID2 = 0U;
}
static void initialize_params_c3_PID2(SFc3_PID2InstanceStruct *chartInstance)
{
(void)chartInstance;
}
static void enable_c3_PID2(SFc3_PID2InstanceStruct *chartInstance)
{
_sfTime_ = sf_get_time(chartInstance->S);
}
static void disable_c3_PID2(SFc3_PID2InstanceStruct *chartInstance)
{
_sfTime_ = sf_get_time(chartInstance->S);
}
static void c3_update_debugger_state_c3_PID2(SFc3_PID2InstanceStruct
*chartInstance)
{
(void)chartInstance;
}
static const mxArray *get_sim_state_c3_PID2(SFc3_PID2InstanceStruct
*chartInstance)
{
const mxArray *c3_st;
const mxArray *c3_y = NULL;
real_T c3_hoistedGlobal;
real_T c3_u;
const mxArray *c3_b_y = NULL;
real_T c3_b_hoistedGlobal;
real_T c3_b_u;
const mxArray *c3_c_y = NULL;
real_T c3_c_hoistedGlobal;
real_T c3_c_u;
const mxArray *c3_d_y = NULL;
real_T c3_d_hoistedGlobal;
real_T c3_d_u;
const mxArray *c3_e_y = NULL;
real_T c3_e_hoistedGlobal;
real_T c3_e_u;
const mxArray *c3_f_y = NULL;
real_T c3_f_hoistedGlobal;
real_T c3_f_u;
const mxArray *c3_g_y = NULL;
real_T c3_g_hoistedGlobal;
real_T c3_g_u;
const mxArray *c3_h_y = NULL;
uint8_T c3_h_hoistedGlobal;
uint8_T c3_h_u;
const mxArray *c3_i_y = NULL;
real_T *c3_dp2;
real_T *c3_dq2;
real_T *c3_dr2;
real_T *c3_dx2;
real_T *c3_dy2;
real_T *c3_dz2;
real_T *c3_u5;
c3_dr2 = (real_T *)ssGetOutputPortSignal(chartInstance->S, 7);
c3_dq2 = (real_T *)ssGetOutputPortSignal(chartInstance->S, 6);
c3_dp2 = (real_T *)ssGetOutputPortSignal(chartInstance->S, 5);
c3_dz2 = (real_T *)ssGetOutputPortSignal(chartInstance->S, 4);
c3_dy2 = (real_T *)ssGetOutputPortSignal(chartInstance->S, 3);
c3_dx2 = (real_T *)ssGetOutputPortSignal(chartInstance->S, 2);
c3_u5 = (real_T *)ssGetOutputPortSignal(chartInstance->S, 1);
c3_st = NULL;
c3_st = NULL;
c3_y = NULL;
sf_mex_assign(&c3_y, sf_mex_createcellmatrix(8, 1), false);
c3_hoistedGlobal = *c3_dp2;
c3_u = c3_hoistedGlobal;
c3_b_y = NULL;
sf_mex_assign(&c3_b_y, sf_mex_create("y", &c3_u, 0, 0U, 0U, 0U, 0), false);
sf_mex_setcell(c3_y, 0, c3_b_y);
c3_b_hoistedGlobal = *c3_dq2;
c3_b_u = c3_b_hoistedGlobal;
c3_c_y = NULL;
sf_mex_assign(&c3_c_y, sf_mex_create("y", &c3_b_u, 0, 0U, 0U, 0U, 0), false);
sf_mex_setcell(c3_y, 1, c3_c_y);
c3_c_hoistedGlobal = *c3_dr2;
c3_c_u = c3_c_hoistedGlobal;
c3_d_y = NULL;
sf_mex_assign(&c3_d_y, sf_mex_create("y", &c3_c_u, 0, 0U, 0U, 0U, 0), false);
sf_mex_setcell(c3_y, 2, c3_d_y);
c3_d_hoistedGlobal = *c3_dx2;
c3_d_u = c3_d_hoistedGlobal;
c3_e_y = NULL;
sf_mex_assign(&c3_e_y, sf_mex_create("y", &c3_d_u, 0, 0U, 0U, 0U, 0), false);
sf_mex_setcell(c3_y, 3, c3_e_y);
c3_e_hoistedGlobal = *c3_dy2;
c3_e_u = c3_e_hoistedGlobal;
c3_f_y = NULL;
sf_mex_assign(&c3_f_y, sf_mex_create("y", &c3_e_u, 0, 0U, 0U, 0U, 0), false);
sf_mex_setcell(c3_y, 4, c3_f_y);
c3_f_hoistedGlobal = *c3_dz2;
c3_f_u = c3_f_hoistedGlobal;
c3_g_y = NULL;
sf_mex_assign(&c3_g_y, sf_mex_create("y", &c3_f_u, 0, 0U, 0U, 0U, 0), false);
sf_mex_setcell(c3_y, 5, c3_g_y);
c3_g_hoistedGlobal = *c3_u5;
c3_g_u = c3_g_hoistedGlobal;
c3_h_y = NULL;
sf_mex_assign(&c3_h_y, sf_mex_create("y", &c3_g_u, 0, 0U, 0U, 0U, 0), false);
sf_mex_setcell(c3_y, 6, c3_h_y);
c3_h_hoistedGlobal = chartInstance->c3_is_active_c3_PID2;
c3_h_u = c3_h_hoistedGlobal;
c3_i_y = NULL;
sf_mex_assign(&c3_i_y, sf_mex_create("y", &c3_h_u, 3, 0U, 0U, 0U, 0), false);
sf_mex_setcell(c3_y, 7, c3_i_y);
sf_mex_assign(&c3_st, c3_y, false);
return c3_st;
}
static void set_sim_state_c3_PID2(SFc3_PID2InstanceStruct *chartInstance, const
mxArray *c3_st)
{
const mxArray *c3_u;
real_T *c3_dp2;
real_T *c3_dq2;
real_T *c3_dr2;
real_T *c3_dx2;
real_T *c3_dy2;
real_T *c3_dz2;
real_T *c3_u5;
c3_dr2 = (real_T *)ssGetOutputPortSignal(chartInstance->S, 7);
c3_dq2 = (real_T *)ssGetOutputPortSignal(chartInstance->S, 6);
c3_dp2 = (real_T *)ssGetOutputPortSignal(chartInstance->S, 5);
c3_dz2 = (real_T *)ssGetOutputPortSignal(chartInstance->S, 4);
c3_dy2 = (real_T *)ssGetOutputPortSignal(chartInstance->S, 3);
c3_dx2 = (real_T *)ssGetOutputPortSignal(chartInstance->S, 2);
c3_u5 = (real_T *)ssGetOutputPortSignal(chartInstance->S, 1);
chartInstance->c3_doneDoubleBufferReInit = true;
c3_u = sf_mex_dup(c3_st);
*c
没有合适的资源?快使用搜索试试~ 我知道了~
电路仿真基于matlab Simulink四旋翼PID控制【含Matlab源码 454期】.zip
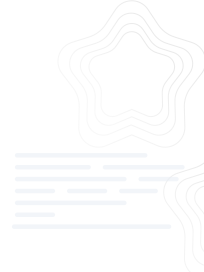
共29个文件
h:6个
obj:5个
c:4个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
CSDN海神之光上传的全部代码均可运行,亲测可用,尽我所能,为你服务; 1、代码压缩包内容 slx文件; 调用函数文件;无需运行 运行结果效果图; 2、代码运行版本 Matlab 2019b;若运行有误,根据提示修改;若不会,可私信博主; 3、运行操作步骤 步骤一:将所有文件放到Matlab的当前文件夹中; 步骤二:双击打开slx文件; 步骤三:点击运行,等程序运行完得到结果; 4、物理应用 仿真:导航、地震、电磁、电路、电能、机械、工业控制、水位控制、直流电机、平面电磁波、管道瞬变流 光学:光栅、杨氏双缝、单缝、多缝、圆孔、矩孔衍射、夫琅禾费、干涉、拉盖尔高斯、光束、光波、涡旋 定位问题:chan、taylor、RSSI、music、卡尔曼滤波UWB 气动学:弹道、气体扩散、龙格库弹道 运动学:倒立摆、泊车 天体学:卫星轨道、姿态
资源推荐
资源详情
资源评论
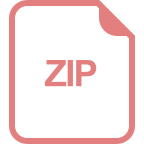
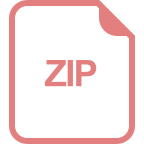
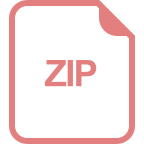
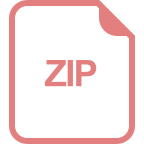
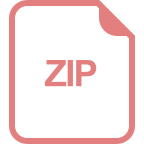
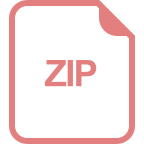
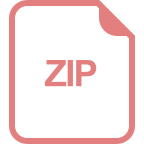
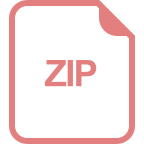
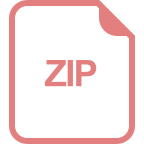
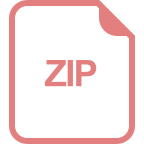
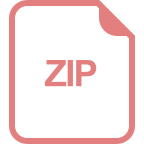
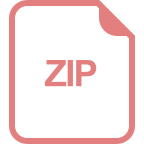
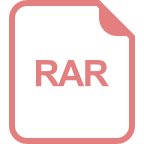
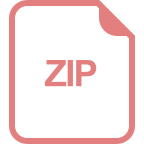
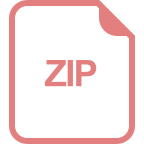
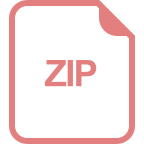
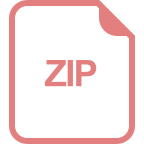
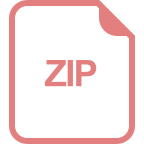
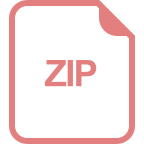
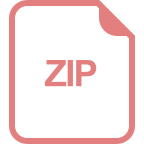
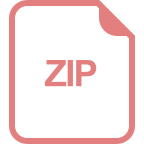
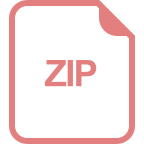
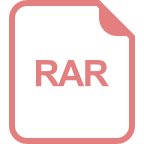
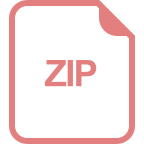
收起资源包目录


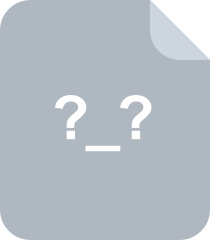
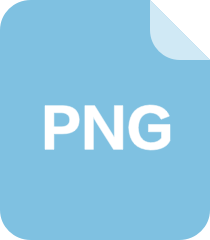






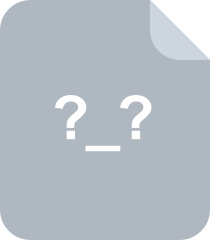
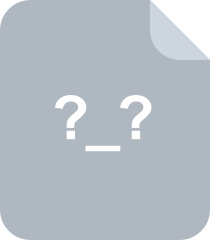
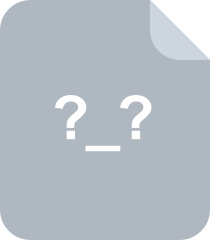

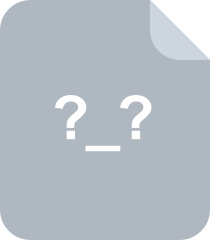
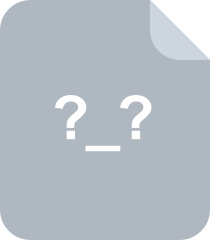
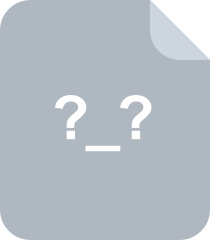
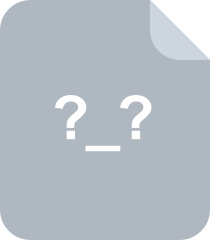
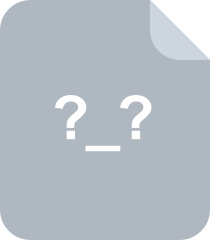
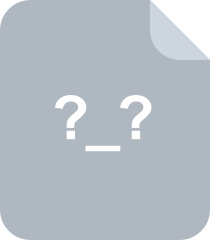
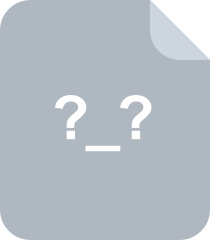
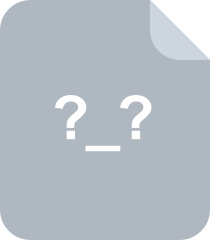
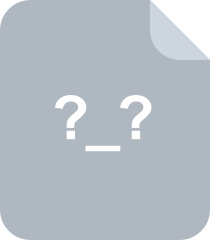
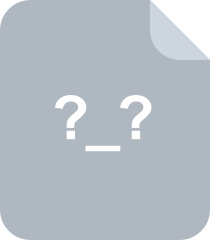
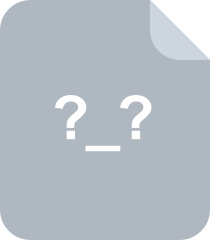
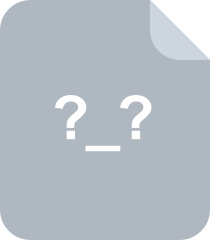
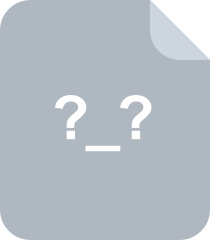
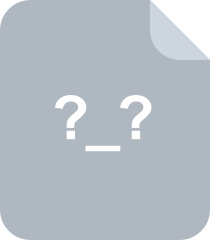
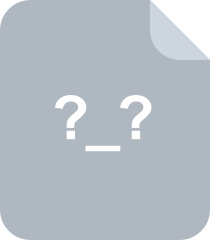
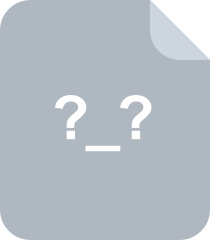
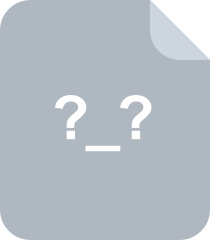
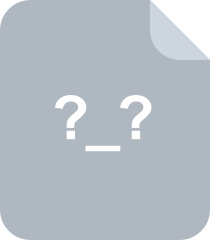
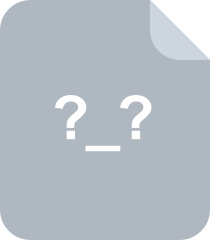
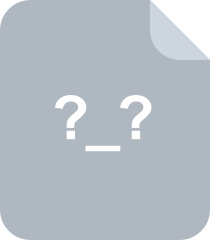
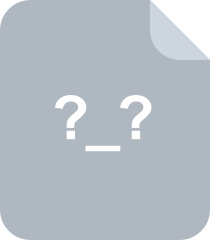
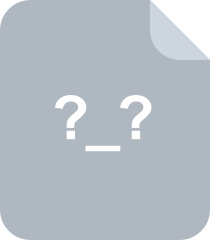
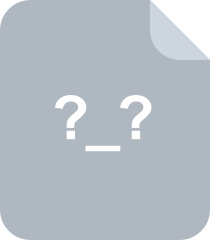



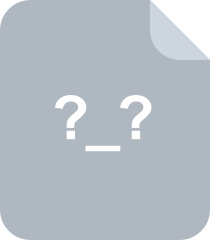
共 29 条
- 1
资源评论
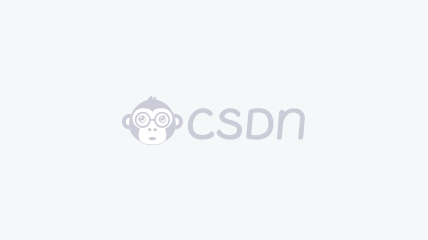
- 大老师Holmes2024-04-09什么垃圾代码,根本看不懂,骗钱是吧 #毫无价值
- 晓5042022-09-02资源中能够借鉴的内容很多,值得学习的地方也很多,大家一起进步!
- 薯条不蘸酱2024-04-24资源值得借鉴的内容很多,那就浅学一下吧,值得下载!


海神之光
- 粉丝: 5w+
- 资源: 6477
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

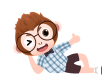
最新资源
- Oracle10gDBA学习手册中文PDF清晰版最新版本
- 扒网站数据软件项目全套技术资料100%好用.zip
- AI爬虫项目全套技术资料100%好用.zip
- 倪海厦讲义及笔记,易学数据测算
- 智能图书管理系统项目全套技术资料.zip
- 基于java写的爬虫项目全套技术资料.zip
- 218) Leverage - 创意机构与作品集 WordPress 主题 2.2.7.zip
- 220) Vinkmag - 多概念创意报纸新闻杂志 WordPress v5.0.zip
- 219) Axtra - 数字机构创意作品集主题 v2.0.zip
- 217) Voice - 清洁新闻 - 杂志 WordPress 主题 v3.0.3.zip
- 215) Classiera – 分类广告 WordPress 主题 v4.0.28.zip
- 216) Creote - 企业与咨询业务 WordPress 主题 v2.7.8.zip
- 212) Outgrid - 多用途 Elementor WordPress 主题 v2.0.0.zip
- 213) Blacksilver - 摄影 WordPress 主题 v9.4.zip
- 214) Nokri - 招聘板 WordPress 主题 v1.5.9.zip
- 211) TopDeal - 多供应商市场 WordPress 主题(移动布局就绪) v2.3.15.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


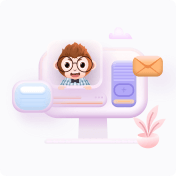
安全验证
文档复制为VIP权益,开通VIP直接复制
