import os, re, bisect, gzip
import numpy as np
import pandas as pd
from src.util import save
import matplotlib
import matplotlib.pyplot as plt
import seaborn as sns
class Paraser:
def __init__(self, root_dir, arg, save_name):
self.root_dir = root_dir
self.save_name = save_name
self.save_path = arg.save_path
self.plot = arg.plot
self.final_test = arg.final_test
self.coordinate_x = []
self.coordinate_y = []
self.irdrop_map = None
self.total_power_map = None
# add new feature
# leakage_power switching_power internal_power
self.leakage_powe_map = None
self.switching_power_map = None
self.internal_power_map = None
#////
self.eff_res_VDD_map = None
self.eff_res_VSS_map = None
self.instance_count = None
self.instance_IR_drop = None
def get_IR_drop_features(self):
max_size = (1000, 1000)
self.irdrop_map = np.zeros(max_size)
self.instance_count = np.zeros(max_size)
self.instance_IR_drop = np.empty(max_size, dtype=object)
for i in np.ndindex(max_size):
self.instance_IR_drop[i] = []
self.instance_name = np.empty(max_size, dtype=object)
for i in np.ndindex(max_size):
self.instance_name[i] = []
self.total_power_map = np.zeros(max_size)
self.leakage_powe_map= np.zeros(max_size)
self.switching_power_map = np.zeros(max_size)
self.internal_power_map = np.zeros(max_size)
self.eff_res_VDD_map = np.zeros(max_size)
self.eff_res_VSS_map = np.zeros(max_size)
self.coordinate_x = np.arange(0,max_size[0],1.44)
self.coordinate_y = np.arange(0,max_size[1],1.152) # based on the row height from LEF
# try:
# if 'nvdla' in self.root_dir:
# data_power = pd.read_csv(os.path.join(self.root_dir, 'NV_nvdla.inst.power.rpt.gz'),sep='\s+',header=1, compression='gzip')
# elif 'Vortex' in self.root_dir:
# data_power = pd.read_csv(os.path.join(self.root_dir, 'Vortex.inst.power.rpt.gz'),sep='\s+',header=1, compression='gzip')
# else:
# data_power = pd.read_csv(os.path.join(self.root_dir, 'pulpino_top.inst.power.rpt.gz'),sep='\s+',header=1, compression='gzip')
# data_r = pd.read_csv(os.path.join(self.root_dir, 'eff_res.rpt.gz'),sep='\s+', low_memory=False, compression='gzip')
# if not self.final_test:
# data_ir = pd.read_csv(os.path.join(self.root_dir, 'static_ir.gz'),sep='\s+', compression='gzip')
# except Exception as e:
# print('one of the report not exists')
# return 0
try:
if 'nvdla' in self.root_dir:
data_power = pd.read_csv(os.path.join(self.root_dir, 'NV_nvdla.inst.power.rpt'),sep='\s+',header=1)
elif 'Vortex' in self.root_dir:
data_power = pd.read_csv(os.path.join(self.root_dir, 'Vortex.inst.power.rpt'),sep='\s+',header=1)
else:
data_power = pd.read_csv(os.path.join(self.root_dir, 'pulpino_top.inst.power.rpt'),sep='\s+',header=1)
data_r = pd.read_csv(os.path.join(self.root_dir, 'eff_res.rpt'),sep='\s+', low_memory=False)
if not self.final_test:
data_ir = pd.read_csv(os.path.join(self.root_dir, 'static_ir'),sep='\s+')
except Exception as e:
print('one of the report not exists')
return 0
# try:
# if 'nvdla' in self.root_dir:
# data_power = pd.read_csv(os.path.join(self.root_dir, 'NV_nvdla.inst.power.rpt'),sep='\s+',header=1)
# else:
# # '\s+' 表示使用空格或多个空格作为分隔符, header=1 指定了CSV文件的第一行(索引从0开始)作为列名
# data_power = pd.read_csv(os.path.join(self.root_dir, 'pulpino_top.inst.power.rpt'),sep='\s+',header=1)
# # low_memory=False会加快读取速度,占用更多内存; low_memory=True会降低读取速度,占用更少内存
# data_r = pd.read_csv(os.path.join(self.root_dir, 'eff_res.rpt'),sep='\s+', low_memory=False)
# data_ir = pd.read_csv(os.path.join(self.root_dir, 'static_ir'),sep='\s+')
max_x = 0
max_y = 0
# parse inst.power.rpt leakage_power switching_power internal_power
total_power = data_power['total_power']
leakage_power = data_power['leakage_power']
switching_power = data_power['switching_power']
internal_power = data_power['internal_power']
bbox = data_power['bbox']
name = data_power['*inst_name']
if self.final_test:
for i,j1,j2,j3,j4,k in zip(bbox, total_power,leakage_power,switching_power,internal_power, name):
x1, y1, x2, y2 = i[1:-1].split(',')
x = (float(x1)+float(x2))/2
y = (float(y1)+float(y2))/2
gcell_x = bisect.bisect_left(self.coordinate_x, float(x)-10)
gcell_y = bisect.bisect_left(self.coordinate_y, float(y)-10)
if gcell_x > max_x:
max_x = gcell_x
if gcell_y > max_y:
max_y = gcell_y
self.total_power_map[gcell_x, gcell_y] += j1
self.leakage_powe_map[gcell_x, gcell_y] +=j2
self.switching_power_map[gcell_x, gcell_y] +=j3
self.internal_power_map[gcell_x, gcell_y] +=j4
self.instance_name[gcell_x, gcell_y].append(k)
self.instance_count[gcell_x, gcell_y] += 1
self.total_power_map = self.total_power_map[0:max_x+1,0:max_y+1]
self.leakage_powe_map = self.leakage_powe_map[0:max_x+1,0:max_y+1]
self.switching_power_map = self.switching_power_map[0:max_x+1,0:max_y+1]
self.internal_power_map = self.internal_power_map[0:max_x+1,0:max_y+1]
save(self.save_path, 'features/instance_count_from_power_rpt', self.save_name, self.instance_count)
self.instance_name = np.concatenate(self.instance_name.ravel())
# 以npz形式保存以节省空间
instance_name_save_path = os.path.join(self.save_path, 'features/instance_name_from_power_rpt', self.save_name)
if not os.path.exists(os.path.dirname(instance_name_save_path)):
os.makedirs(os.path.dirname(instance_name_save_path))
np.savez_compressed(instance_name_save_path, instance_name=self.instance_name)
else:
for i,j1,j2,j3,j4 in zip(bbox, total_power,leakage_power,switching_power,internal_power):
x1, y1, x2, y2 = i[1:-1].split(',')
x = (float(x1)+float(x2))/2
y = (float(y1)+float(y2))/2
gcell_x = bisect.bisect_left(self.coordinate_x, float(x)-10)
gcell_y = bisect.bisect_left(self.coordinate_y, float(y)-10)
if gcell_x > max_x:
max_x = gcell_x
if gcell_y > max_y:
max_y = gcell_y
self.total_power_map[gcell_x, gcell_y] += j1
self.leakage_powe_map[gcell_x, gcell_y] +=j2
self.switching_power_map[gcell_x, gcell_y] +=j3
self.internal_power_map[gcell_x, gcell_y] +=j4
self.total_power_map = self.total_power_map[0:max_x+1,0:max_y+1]
self.leakage_powe_map = self.leakage_powe_map[0:max_x+1,0:max_y+1]
self.switching_power_map = self.switching_power_map[0:max_x+1,0:max_y+1]
self.internal_power_map = self.internal_power_map[0:max_x+1,0:max_y+1]
save(self.save_path, 'features/total_power', self.save_name, self.total_power_map)
save(self.save_path, 'features/leakage_power', self.save_name, self.leakage_powe_map)
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于python机器学习的Soc静态压降预测源码+项目说明.zip基于python机器学习的Soc静态压降预测源码+项目说明.zip基于python机器学习的Soc静态压降预测源码+项目说明.zip基于python机器学习的Soc静态压降预测源码+项目说明.zip 【优质项目推荐】 1.项目代码完整且功能都经验证ok,确保稳定可靠运行后才上传。欢迎下载使用!在使用过程中,如有问题或建议,请及时私信沟通,帮助解答。 2.项目主要针对各个计算机相关专业,包括计科、信息安全、数据科学与大数据技术、人工智能、通信、物联网等领域的在校学生、专业教师或企业员工使用。 3.项目具有丰富的拓展空间,不仅可作为入门进阶,也可直接作为毕设、课程设计、大作业、项目初期立项演示等用途。 4.如果基础还行,或热爱钻研,可基于此项目进行二次开发,DIY其他不同功能。 【特别强调】 项目下载解压后,项目名字和项目路径不要用中文,否则可能会出现解析不了的错误,建议解压重命名为因为名字后再运行!有问题私信沟通,祝顺利! 基于python机器学习的Soc静态压降预测源码+项目说明.zip基于python机器学习的Soc静态压降预测源码+项目说明.zip基于python机器学习的Soc静态压降预测源码+项目说明.zip基于python机器学习的Soc静态压降预测源码+项目说明.zip基于python机器学习的Soc静态压降预测源码+项目说明.zip
资源推荐
资源详情
资源评论
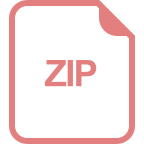
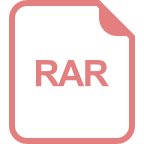
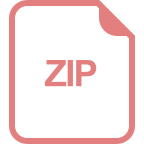
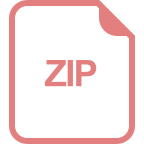
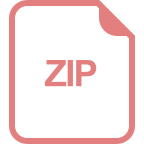
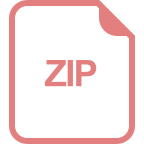
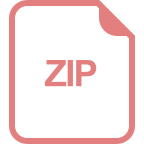
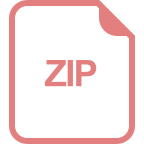
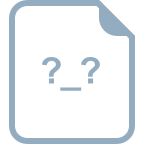
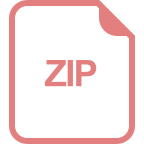
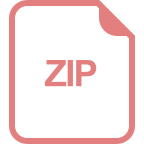
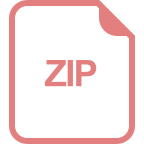
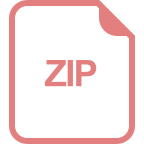
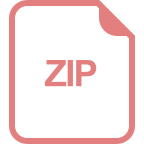
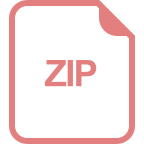
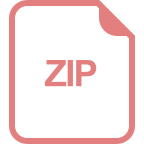
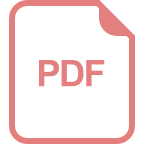
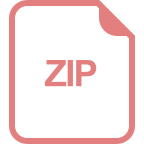
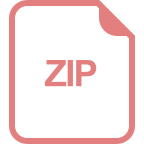
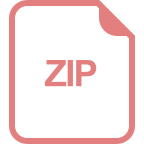
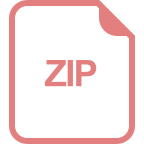
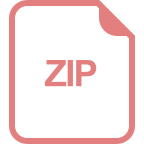
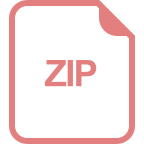
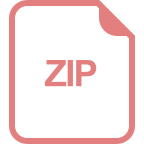
收起资源包目录

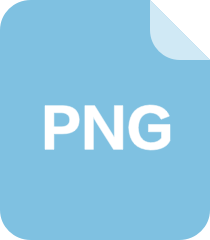
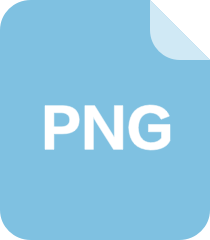
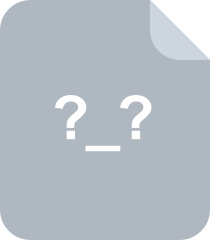

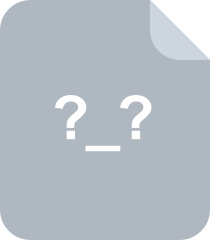
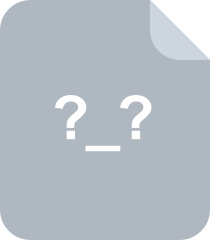

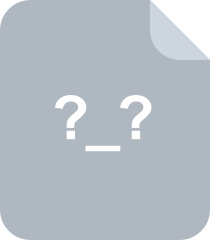
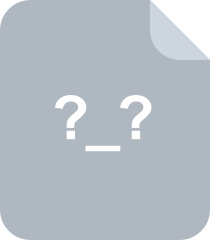
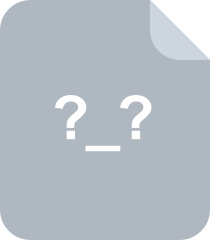

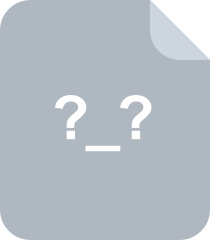
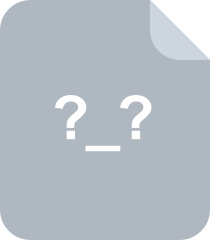
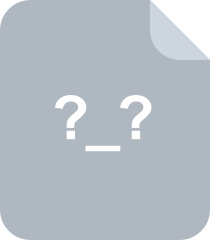
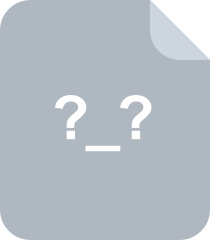
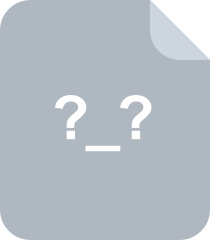
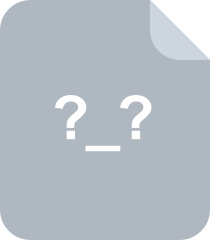


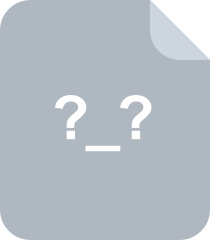
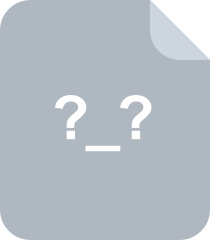
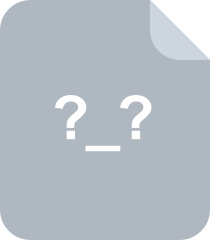
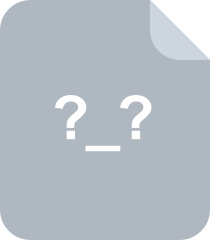

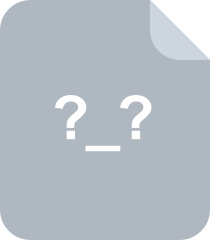
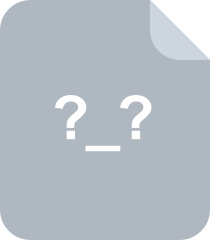
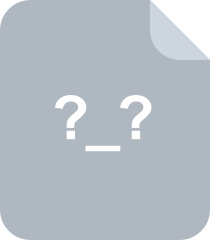
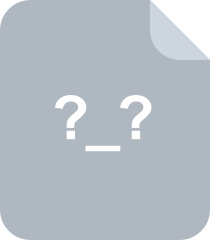

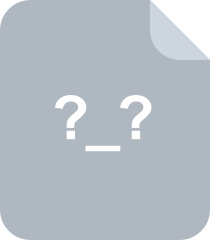
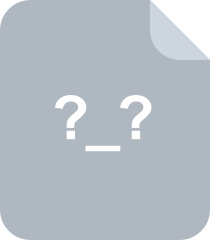
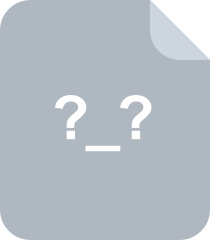
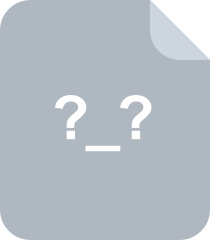
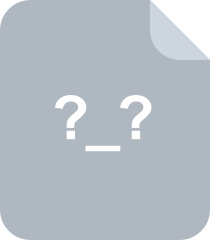
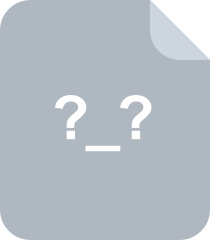

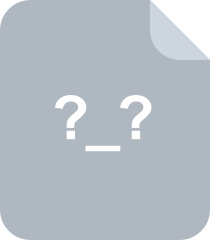
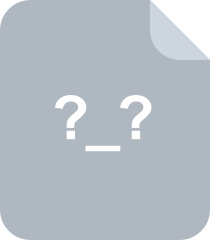
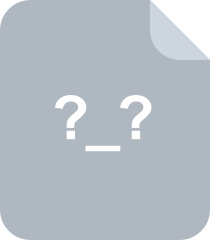
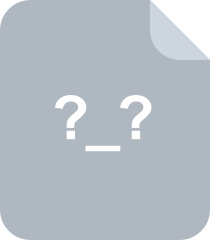
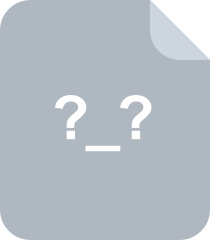
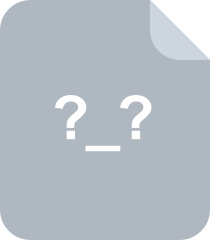

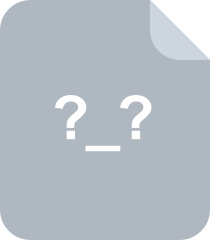
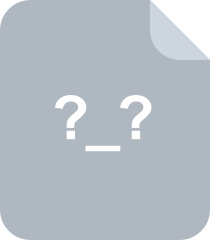
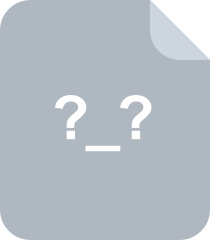
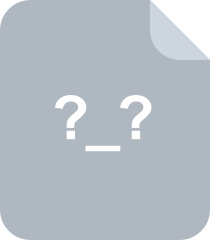
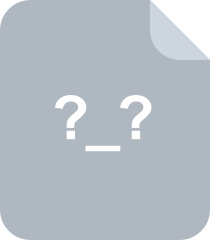

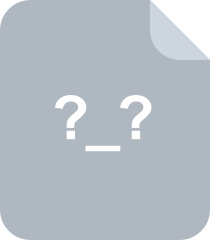
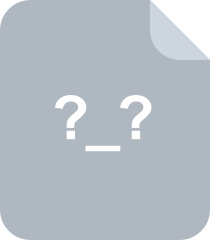
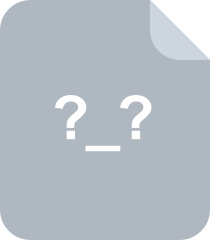
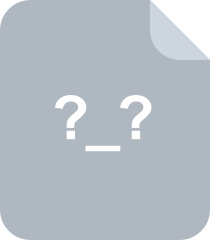

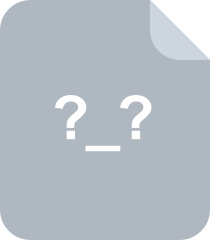
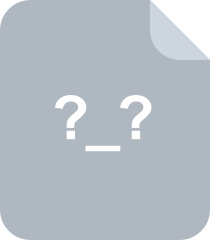
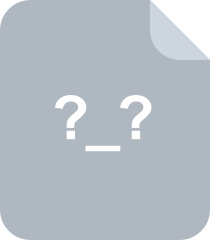
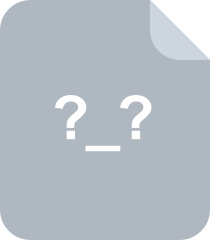
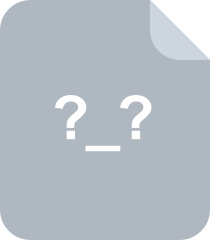
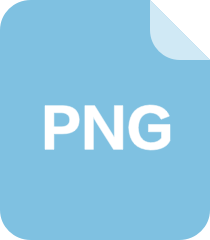
共 49 条
- 1
资源评论
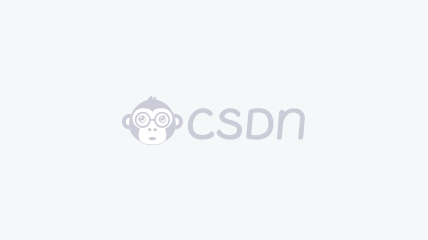

.whl
- 粉丝: 3908
- 资源: 4858
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

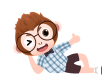
最新资源
- 2011-2024年各省数字普惠金融指数数据.zip
- 数据结构排序算法:插入排序、希尔排序、冒泡排序及快速排序算法
- Nosql期末复习资料
- Python新年庆典倒计时与节日活动智能管理助手
- 塑料、玻璃、金属、纸张、木材检测36-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 系统DLL文件修复工具
- 数据结构之哈希查找方法
- Python圣诞节倒计时与节日活动管理系统
- 塑料检测23-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- PPT模板WHUT-博学之光-PPT模板
- C#上位机开发与工控通讯实战课程
- HCIA-Datacom教师笔记-数据通信基础知识及网络模型详解
- MobileNet V2 网络实现的计算机视觉大项目:8种常见茶叶病害种类识别
- 文件格式是一种撒很快的哈的东西
- Python之正则表达式基础知识
- JLINK-OB下载器的原理图和HEX文件
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


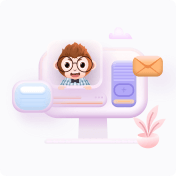
安全验证
文档复制为VIP权益,开通VIP直接复制
