/**
* @file Mcu_PBcfg.c
* @implements Mcu_PBcfg.c_Artifact
* @version 1.0.1
*
* @brief AUTOSAR Mcu - Data structures for the Mcu driver.
* @details Postbuild structure configurations for the driver initalization.
*
* @addtogroup MCU
* @{
*/
/*==================================================================================================
* Project : AUTOSAR 4.3 MCAL
* Platform : ARM
* Peripheral : MC
* Dependencies : none
*
* Autosar Version : 4.3.1
* Autosar Revision : ASR_REL_4_3_REV_0001
* Autosar Conf.Variant :
* SW Version : 1.0.1
* Build Version : S32K14x_MCAL_1_0_1_RTM_ASR_REL_4_3_REV_0001_20190621
*
* (c) Copyright 2006-2016 Freescale Semiconductor, Inc.
* Copyright 2017-2019 NXP
* All Rights Reserved.
==================================================================================================*/
/*==================================================================================================
==================================================================================================*/
#ifdef __cplusplus
extern "C"
{
#endif
/**
* @page misra_violations MISRA-C:2004 violations
*
* @section Mcu_PBcfg_c_REF_1
* Violates MISRA 2004 Required Rule 19.15, Repeated include file MemMap.h, Precautions shall be
* taken in order to prevent the contents of a header file being included twice This is not a violation
* since all header files are protected against multiple inclusions
*
* @section Mcu_PBcfg_c_REF_2
* Violates MISRA 2004 Advisory Rule 19.1, only preprocessor statements and comments
* before '#include' MemMap.h included after each section define in order to set the current memory section
*
* @section Mcu_PBcfg_c_REF_3
* Violates MISRA 2004 Required Rule 1.4, The compiler/linker shall be checked to ensure
* that 31 character significance and case sensitivity are supported for external identifiers.
* The defines are validated.
*
* @section Mcu_PBcfg_c_REF_4
* Violates MISRA 2004 Required Rule 11.1, Conversions shall not be performed between
* a pointer to a function and any type other than an integral type.
* Appears when accessing memory-mapped registers.
*
* @section Mcu_PBcfg_c_REF_5
* Violates MISRA 2004 Advisory Rule 11.4, A cast should not be performed
* between a pointer to object type and a different pointer to object type.
*
* @section Mcu_PBcfg_c_REF_6
* Violates MISRA 2004 Required Rule 20.2, The names of standard macros, objects and
* functions shall not be reused
*
* @section Mcu_PBcfg_c_REF_7
* Violates MISRA 2004 Required Rule 8.10, All declarations and definitions of objects or functions
* at file scope shall have internal linkage unless external linkage is requiered.
*
* @section Mcu_PBcfg_c_REF_8
* Violates MISRA 2004 Required Rule 8.12, when an array is declared with external linkage, it's size shall be stated explicitly
* or defined implicitly by initialisation
*
* @section Mcu_PBcfg_c_REF_9
* Violates MISRA 2004 Required Rule 8.8, external object should be declared in one and only one file
*
* @section Mcu_PBcfg_c_REF_10
* Violates MISRA 2004 Advisory Rule 11.3, A cast should not be performed between
* a pointer type and an integral type
* The cast is used to access memory mapped registers.
*
* @section [global]
* Violates MISRA 2004 Required Rule 5.1, Identifiers (internal and external) shall not rely
* on the significance of more than 31 characters. The used compilers use more than 31 chars for
* identifiers.
*
*/
/*==================================================================================================
INCLUDE FILES
1) system and project includes
2) needed interfaces from external units
3) internal and external interfaces from this unit
==================================================================================================*/
#include "Mcu.h"
#include "Reg_eSys_PCC.h"
#include "Reg_eSys_PMC.h"
#include "Reg_eSys_RCM.h"
#include "Reg_eSys_SCG.h"
#include "Reg_eSys_SIM.h"
#include "Reg_eSys_SMC.h"
#include "Reg_eSys_CMU.h"
#if (MCU_DISABLE_DEM_REPORT_ERROR_STATUS == STD_OFF)
#include "Dem.h"
#endif /* (MCU_DISABLE_DEM_REPORT_ERROR_STATUS == STD_OFF) */
/*==================================================================================================
* SOURCE FILE VERSION INFORMATION
==================================================================================================*/
#define MCU_PBCFG_VENDOR_ID_C 43
#define MCU_PBCFG_AR_RELEASE_MAJOR_VERSION_C 4
#define MCU_PBCFG_AR_RELEASE_MINOR_VERSION_C 3
#define MCU_PBCFG_AR_RELEASE_REVISION_VERSION_C 1
#define MCU_PBCFG_SW_MAJOR_VERSION_C 1
#define MCU_PBCFG_SW_MINOR_VERSION_C 0
#define MCU_PBCFG_SW_PATCH_VERSION_C 1
/*==================================================================================================
FILE VERSION CHECKS
==================================================================================================*/
/**
* @brief The MCU module's implementer shall avoid the integration of incompatible files.
*/
/* Check if current file and MCU header file are of the same vendor */
#if (MCU_PBCFG_VENDOR_ID_C != MCU_VENDOR_ID)
#error "Mcu_PBcfg.c and Mcu.h have different vendor ids"
#endif
/* Check if current file and MCU header file are of the same Autosar version */
#if ((MCU_PBCFG_AR_RELEASE_MAJOR_VERSION_C != MCU_AR_RELEASE_MAJOR_VERSION) || \
(MCU_PBCFG_AR_RELEASE_MINOR_VERSION_C != MCU_AR_RELEASE_MINOR_VERSION) || \
(MCU_PBCFG_AR_RELEASE_REVISION_VERSION_C != MCU_AR_RELEASE_REVISION_VERSION) \
)
#error "AutoSar Version Numbers of Mcu_PBcfg.c and Mcu.h are different"
#endif
/* Check if current file and MCU header file are of the same Software version */
#if ((MCU_PBCFG_SW_MAJOR_VERSION_C != MCU_SW_MAJOR_VERSION) || \
(MCU_PBCFG_SW_MINOR_VERSION_C != MCU_SW_MINOR_VERSION) || \
(MCU_PBCFG_SW_PATCH_VERSION_C != MCU_SW_PATCH_VERSION) \
)
#error "Software Version Numbers of Mcu_PBcfg.c and Mcu.h are different"
#endif
/* Check if source file and Reg_eSys_PCC.h are from the same vendor */
#if (MCU_PBCFG_VENDOR_ID_C != REG_ESYS_PCC_VENDOR_ID)
#error "Mcu_PBcfg.c and Reg_eSys_PCC.h have different vendor ids"
#endif
/* Check if source file and Reg_eSys_PCC.h are of the same Autosar version */
#if ((MCU_PBCFG_AR_RELEASE_MAJOR_VERSION_C != REG_ESYS_PCC_AR_RELEASE_MAJOR_VERSION) || \
(MCU_PBCFG_AR_RELEASE_MINOR_VERSION_C != REG_ESYS_PCC_AR_RELEASE_MINOR_VERSION) || \
(MCU_PBCFG_AR_RELEASE_REVISION_VERSION_C != REG_ESYS_PCC_AR_RELEASE_REVISION_VERSION) \
)
#error "AutoSar Version Numbers of Mcu_PBcfg.c and Reg_eSys_PCC.h are different"
#endif
/*Check if source file and Reg_eSys_PCC.h are of the same Software version */
#if ((MCU_PBCFG_SW_MAJOR_VERSION_C != REG_ESYS_PCC_SW_MAJOR_VERSION) || \
(MCU_PBCFG_SW_MINOR_VERSION_C != REG_ESYS_PCC_SW_MINOR_VERSION) || \
(MCU_PBCFG_SW_PATCH_VERSION_C != REG_ESYS_PCC_SW_PATCH_VERSION) \
)
#error "Software Version Numbers of Mcu_PBcfg.c and Reg_eSys_PCC.h are different"
#endif
/* Check if source file and Reg_eSys_SMC.h are from the same vendor */
#if (MCU_PBCFG_VENDOR_ID_C != REG_ESYS_SMC_VENDOR_ID)
#error "Mcu_PBcfg.c and Reg_eSys_SMC.h have different vendor ids"
#endif
/* Check if source file and Reg_eSys_SMC.h are of the same Autosar version */
#if ((MCU_PBCFG_AR_RELEASE_MAJOR_VERSION_C != REG_ESYS_SMC_AR_RELEASE_MAJOR_VERSION) || \
(MCU_PBCFG_AR_RELEASE_MINOR_VERSION_C != REG_ESYS_SMC_AR_RELEASE_MINOR_VERSION) || \
(MCU_PBCFG_AR_RELEASE_REVISION_VERSION_C != REG_ESYS_SMC_AR_RELEASE_REVISION_VERSION) \
)
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
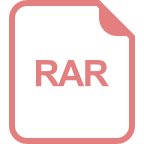
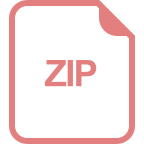
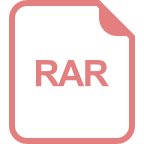
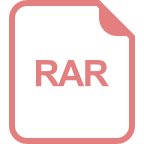
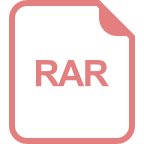
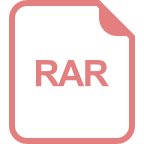
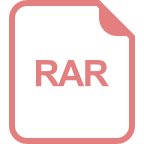
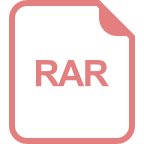
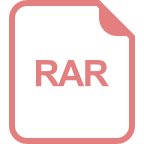
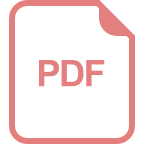
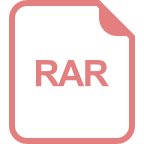
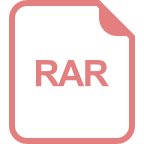
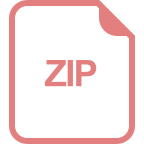
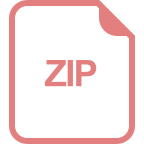
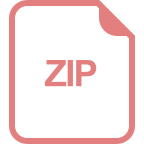
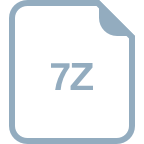
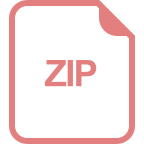
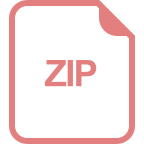
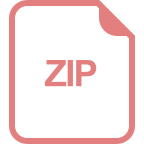
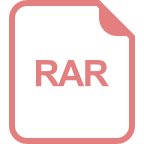
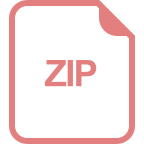
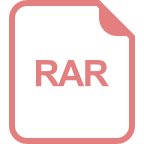
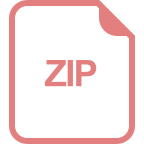
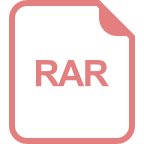
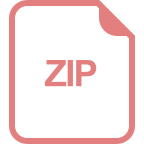
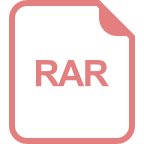
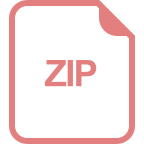
收起资源包目录




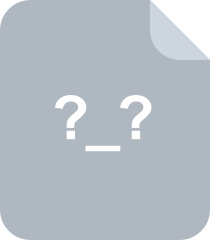
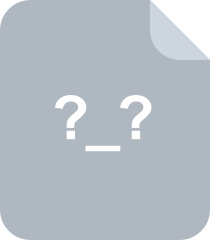
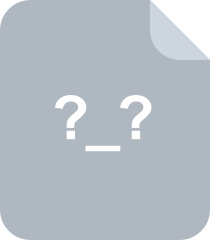
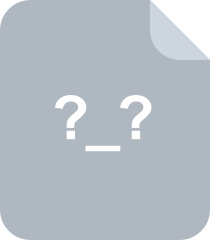
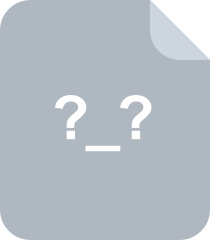

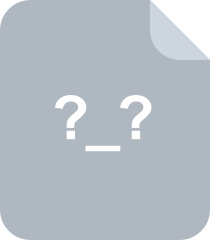
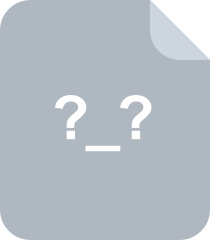
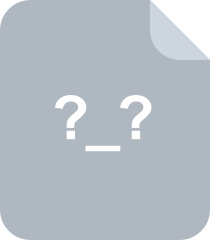
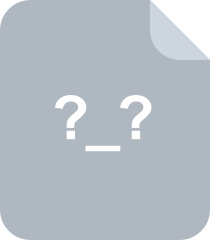
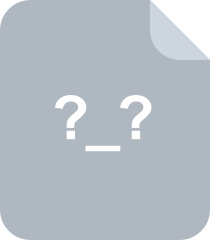

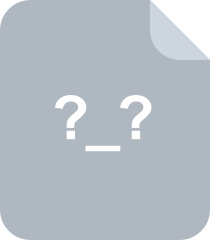
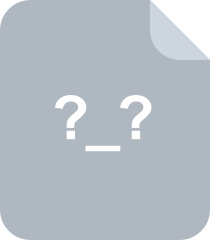
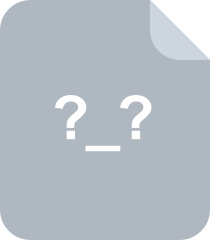
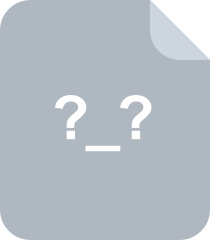
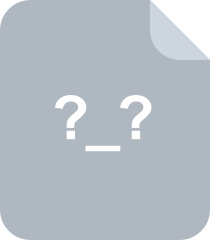


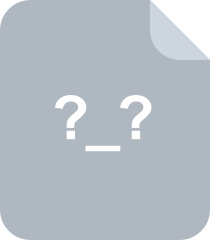
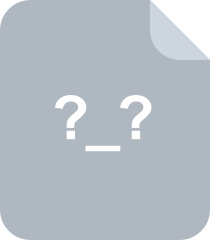
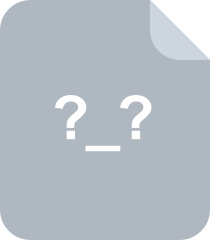
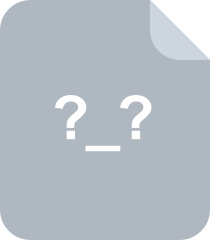
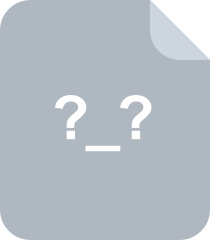

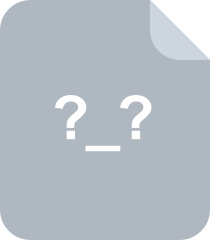
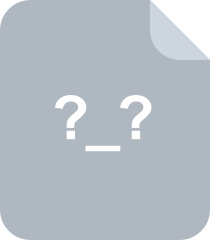
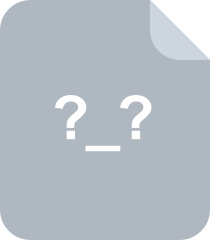
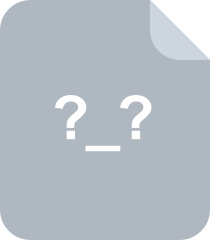
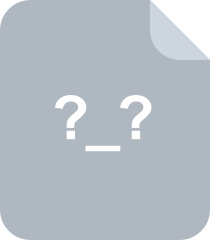

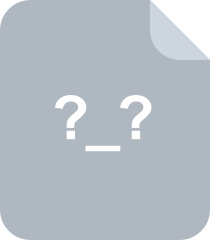
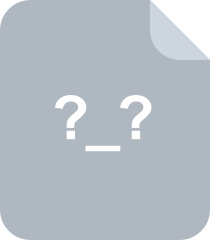
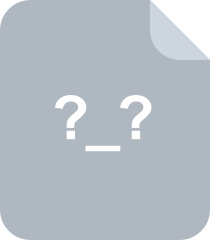
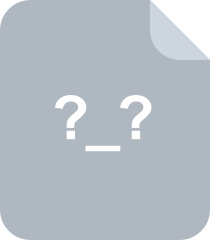
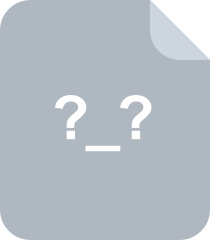
共 30 条
- 1
资源评论
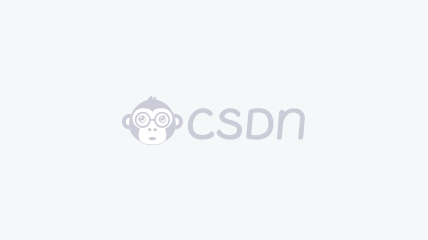

OnlyMars
- 粉丝: 209
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

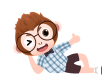
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


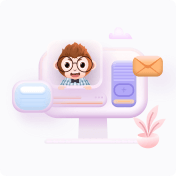
安全验证
文档复制为VIP权益,开通VIP直接复制
