package com.wind;
import java.util.ArrayList;
import java.util.List;
import android.app.AlertDialog;
import android.app.Dialog;
import android.content.Context;
import android.content.DialogInterface;
import android.graphics.Color;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseExpandableListAdapter;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.CompoundButton;
import android.widget.CompoundButton.OnCheckedChangeListener;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.TextView;
import android.widget.Toast;
public class ExpandableListViewAdapter extends BaseExpandableListAdapter {
private Context context;
private List<ListRow> groupList = new ArrayList<ListRow>();
private List<List<ListRow>> childList = new ArrayList<List<ListRow>>();
public ExpandableListViewAdapter(Context context, List<ListRow> groupList,
List<List<ListRow>> childList) {
this.context = context;
this.groupList = groupList;
this.childList = childList;
}
@Override
public Object getChild(int groupPosition, int childPosition) {
return null;
}
@Override
public long getChildId(int groupPosition, int childPosition) {
return 0;
}
@Override
public View getChildView(int groupPosition, int childPosition,
boolean isLastChild, View convertView, ViewGroup parent) {
ListRow row = (ListRow) childList.get(groupPosition).get(childPosition);
ListRowView view = new ListRowView(context, row, groupPosition, childPosition);
return view;
}
@Override
public int getChildrenCount(int groupPosition) {
return childList.get(groupPosition).size();
}
@Override
public Object getGroup(int groupPosition) {
return null;
}
@Override
public int getGroupCount() {
return groupList.size();
}
@Override
public long getGroupId(int groupPosition) {
return 0;
}
@Override
public View getGroupView(int groupPosition, boolean isExpanded,
View convertView, ViewGroup parent) {
ListRow row = (ListRow) groupList.get(groupPosition);
List<RowContent> viewContents = row.getRowContents();
for(int i = 0; i < viewContents.size(); i++) {
RowContent content = viewContents.get(i);
if(content.getType() == ConstantUtils.EXPAND_IMAGE) {
if(isExpanded) {
content.setImage_id(R.drawable.btn_expand2);
}else {
content.setImage_id(R.drawable.btn_expand);
}
}
}
ListRowView view = new ListRowView(context, row, groupPosition, 0);
return view;
}
@Override
public boolean hasStableIds() {
return false;
}
@Override
public boolean isChildSelectable(int groupPosition, int childPosition) {
return false;
}
public static class ListRow {
private int rowType;
private boolean selectable;
private int layout_id;
private boolean isChecked;
private List<RowContent> rowContents = new ArrayList<RowContent>();
private int index;
public int getRowType() {
return rowType;
}
public void setRowType(int rowType) {
this.rowType = rowType;
}
public boolean isSelectable() {
return selectable;
}
public void setSelectable(boolean selectable) {
this.selectable = selectable;
}
public int getLayout_id() {
return layout_id;
}
public void setLayout_id(int layoutId) {
layout_id = layoutId;
}
public List<RowContent> getRowContents() {
return rowContents;
}
public void setRowContents(List<RowContent> rowContents) {
this.rowContents = rowContents;
}
public int getIndex() {
return index;
}
public void setIndex(int index) {
this.index = index;
}
public boolean isChecked() {
return isChecked;
}
public void setChecked(boolean isChecked) {
this.isChecked = isChecked;
}
}
public static class RowContent {
private int type;
private int layout_id;
private String text;
private int image_id = -1;
private int color = Color.WHITE;
public int getImage_id() {
return image_id;
}
public void setImage_id(int imageId) {
image_id = imageId;
}
public int getType() {
return type;
}
public void setType(int type) {
this.type = type;
}
public int getLayout_id() {
return layout_id;
}
public void setLayout_id(int layoutId) {
layout_id = layoutId;
}
public String getText() {
return text;
}
public void setText(String text) {
this.text = text;
}
public int getColor() {
return color;
}
public void setColor(int color) {
this.color = color;
}
}
public class ListRowView extends LinearLayout {
public ListRow row;
private int groupIndex;
private int childIndex;
private Context context;
public ListRowView(Context context, ListRow row, int groupIndex, int childIndex) {
super(context);
this.context = context;
this.row = row;
this.groupIndex = groupIndex;
this.childIndex = childIndex;
addView(inflate(context, row.getLayout_id(), null));
setData(row);
}
public ListRow getRow() {
return row;
}
public void setData(ListRow row) {
List<RowContent> viewContents = row.getRowContents();
if ((viewContents != null) && (viewContents.size() > 0)) {
for (RowContent viewContent : viewContents) {
int type = viewContent.getType();
int layout_id = viewContent.getLayout_id();
switch (type) {
case ConstantUtils.TEXT:
TextView textView = (TextView)this.findViewById(layout_id);
if(textView != null) {
textView.setText(viewContent.getText());
if(viewContent.getColor() != Color.WHITE)
textView.setTextColor(viewContent.getColor());
}
break;
case ConstantUtils.EXPAND_IMAGE:
case ConstantUtils.IMAGE:
int icon_id = viewContent.getImage_id();
if(icon_id != -1)
((ImageView)this.findViewById(layout_id)).setBackgroundResource(icon_id);
break;
case ConstantUtils.EDIT_TEXT:
EditText editText = (EditText)this.findViewById(layout_id);
if(editText != null) {
editText.setText(viewContent.getText());
}
// editText.setOnFocusChangeListener(focus_listener_noIM);
// editText.setOnTouchListener(touch_listener_noIM);
break;
case ConstantUtils.CHECK_BOX:
CheckBox cb = (CheckBox) this.findViewById(layout_id);
cb.setChecked(row.isChecked());
cb.setOnCheckedChangeListener(checkBoxListener);
break;
case ConstantUtils.BUTTON:
Button delete = (Button) this.findViewById(layout_id);
delete.setOnClickListener(deleteButtonOnClick);
break;
default:
break;
}
}
}
}
private OnClickListener deleteButtonOnClick = new OnClickListener() {
@Override
public void onClick(View v) {
Dialog dialog = null;
AlertDialog.Builder builder = new AlertDialog.Builder(context);
builder.setIcon(android.R.drawable.ic_dialog_alert).setTitle(
"您确定要刪除该项?").setPositiveButton("确定",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int whichButton) {
childList.get(groupIndex).remove(childIndex);
}
}).setNegativeButton("取消",
new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog,
int whichButton) {
}
});
dialog = builder.create();
dialog.show();
}
};
private OnCheckedChangeListener checkBoxListener = new OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView,
boolean isChecked) {
// TODO Auto-generated method stub
row.setChecked(isChecked)
没有合适的资源?快使用搜索试试~ 我知道了~
安卓源码 Android Demo 模仿QQ的扩展型很好的ExpandableListView
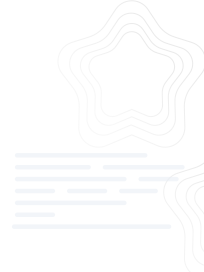
共39个文件
class:16个
png:7个
xml:5个

需积分: 50 0 下载量 53 浏览量
2024-04-04
15:40:19
上传
评论
收藏 883KB ZIP 举报
温馨提示
安卓源码 Android Demo 模仿QQ的扩展型很好的ExpandableListView 安卓源码 Android Demo 模仿QQ的扩展型很好的ExpandableListView安卓源码 Android Demo 模仿QQ的扩展型很好的ExpandableListView安卓源码 Android Demo 模仿QQ的扩展型很好的ExpandableListView安卓源码 Android Demo 模仿QQ的扩展型很好的ExpandableListView安卓源码 Android Demo 模仿QQ的扩展型很好的ExpandableListView安卓源码 Android Demo 模仿QQ的扩展型很好的ExpandableListView安卓源码 Android Demo 模仿QQ的扩展型很好的ExpandableListView安卓源码 Android Demo 模仿QQ的扩展型很好的ExpandableListView安卓源码 Android Demo 模仿QQ的扩展型很好的ExpandableListView安卓源码 Android Demo 模仿QQ的扩展型很好的
资源推荐
资源详情
资源评论



















收起资源包目录




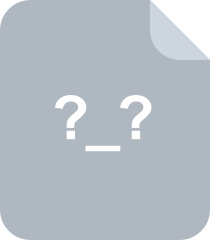




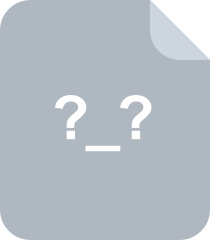
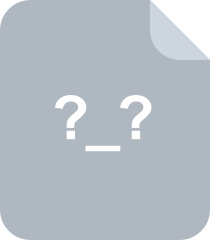
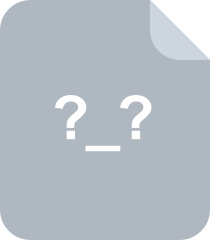


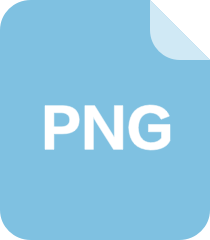

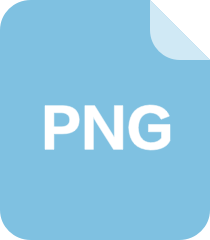

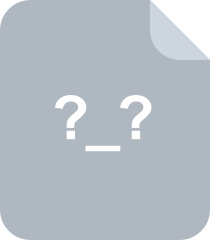

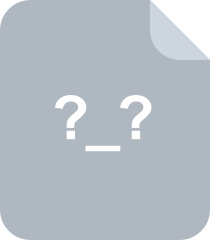
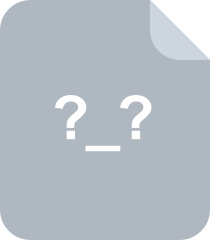
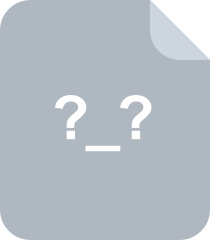

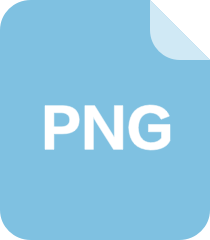
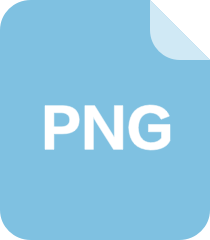
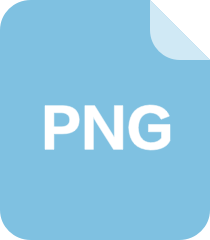
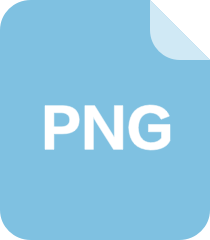
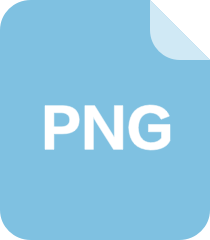

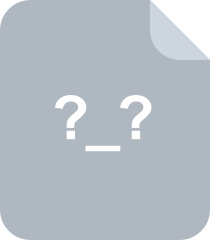
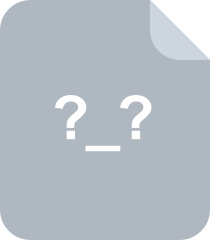
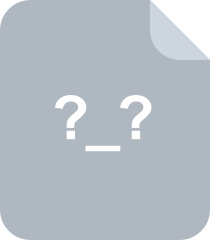


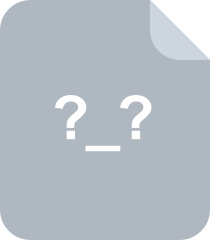
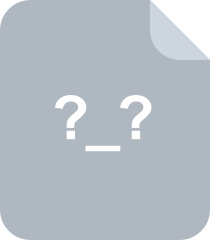
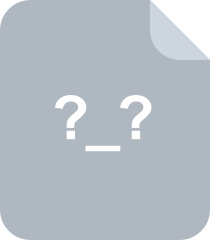
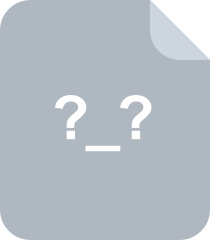
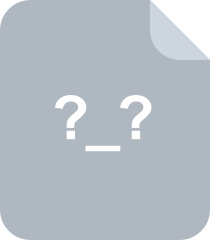
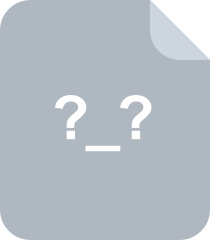
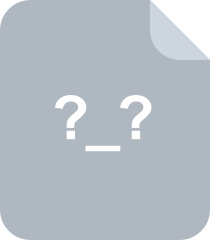
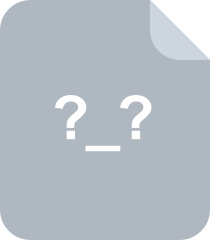
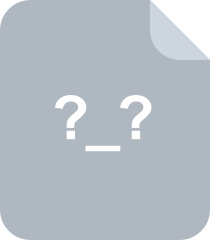
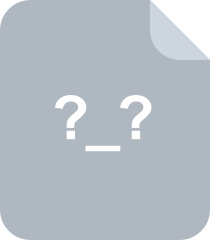
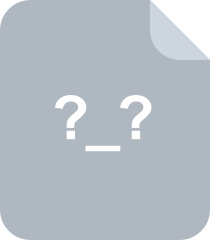
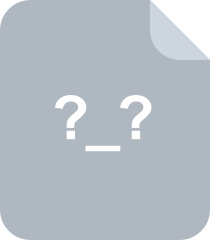
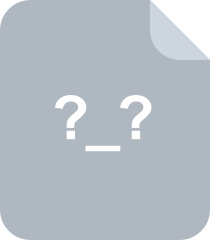
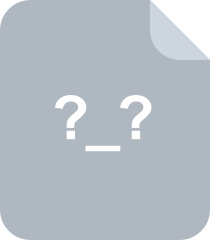
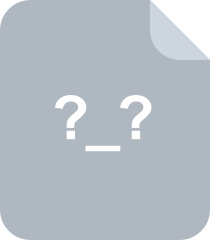
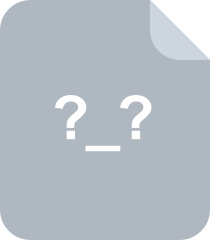
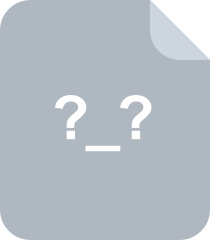
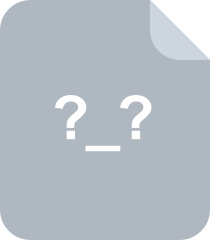
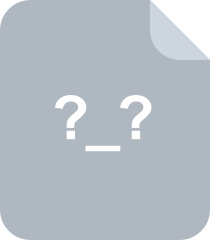



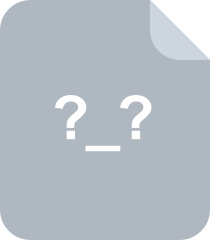
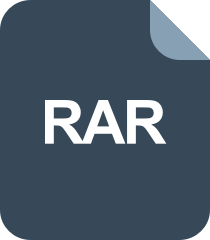
共 39 条
- 1
资源评论
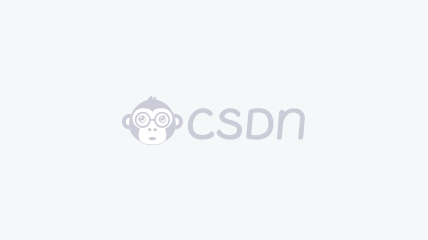
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整

金克斯在coding
- 粉丝: 1865
- 资源: 151
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

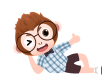
最新资源
- 跨境电商B2C数据运营(中级)PPT
- 工业自动化中欧姆龙PLC CP1E与柯力XK3101电子称重仪表的Modbus RTU通信及应用
- _24442000000463330175.pdf
- 软件设计师考试通关秘籍:全面解析考试要点与备考技巧
- 跨境电商B2C数据运营(中级)教材配套理论PPT
- 麒麟桌面操作系统应用-教学PPT
- C#与VB.NET操作SQL及Access数据库及Excel报表导出打印(VS2015 & Office 2013)
- 人工智能技术应用导论(第2版)配套PPT
- 人工智能技术应用导论(第2版)配套代码
- Modbus Poll、Modbus Slave及VSPD安装包
- 人工智能应用基础(第2版)实例教程
- Cruise与Matlab联合仿真:增程式混动四驱的最佳经济性扭矩分配、内燃机功率跟随及能量回收策略
- 图说图解机器学习(第2版)-电子教案-教学指南
- 电子硬件学习指南:课后习题解析及其在实际应用中的重要性
- 网络设备配置与调试项目实训(第4版) ppt
- 信号处理领域中EMD及其改进方法的Matlab实现与端点效应克服
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


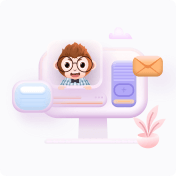
安全验证
文档复制为VIP权益,开通VIP直接复制
