/*
* Copyright (C) 2002-2022 Free Software Foundation, Inc.
*
* This file is part of LIBTASN1.
*
* LIBTASN1 is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2.1 of
* the License, or (at your option) any later version.
*
* LIBTASN1 is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with LIBTASN1; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA
* 02110-1301, USA
*
*/
/**
* SECTION:libtasn1
* @short_description: GNU ASN.1 library
*
* The Libtasn1 library provides Abstract Syntax Notation One (ASN.1, as
* specified by the X.680 ITU-T recommendation) parsing and structures
* management, and Distinguished Encoding Rules (DER, as per X.690)
* encoding and decoding functions.
*/
#ifndef LIBTASN1_H
# define LIBTASN1_H
# ifndef ASN1_API
# if defined ASN1_BUILDING && defined HAVE_VISIBILITY && HAVE_VISIBILITY
# define ASN1_API __attribute__((__visibility__("default")))
# elif defined ASN1_BUILDING && defined _MSC_VER && ! defined ASN1_STATIC
# define ASN1_API __declspec(dllexport)
# elif defined _MSC_VER && ! defined ASN1_STATIC
# define ASN1_API __declspec(dllimport)
# else
# define ASN1_API
# endif
# endif
# ifdef __GNUC__
# define __LIBTASN1_CONST__ __attribute__((const))
# define __LIBTASN1_PURE__ __attribute__((pure))
# else
# define __LIBTASN1_CONST__
# define __LIBTASN1_PURE__
# endif
# include <sys/types.h>
# include <time.h>
# include <stdio.h> /* for FILE* */
# ifdef __cplusplus
extern "C"
{
# endif
/**
* ASN1_VERSION:
*
* Version of the library as a string.
*/
# define ASN1_VERSION "4.19.0"
/**
* ASN1_VERSION_MAJOR:
*
* Major version number of the library.
*/
# define ASN1_VERSION_MAJOR 4
/**
* ASN1_VERSION_MINOR:
*
* Minor version number of the library.
*/
# define ASN1_VERSION_MINOR 19
/**
* ASN1_VERSION_PATCH:
*
* Patch version number of the library.
*/
# define ASN1_VERSION_PATCH 0
/**
* ASN1_VERSION_NUMBER:
*
* Version number of the library as a number.
*/
# define ASN1_VERSION_NUMBER 0x041300
# if defined __GNUC__ && !defined ASN1_INTERNAL_BUILD
# define _ASN1_GCC_VERSION (__GNUC__ * 10000 + __GNUC_MINOR__ * 100 + __GNUC_PATCHLEVEL__)
# if _ASN1_GCC_VERSION >= 30100
# define _ASN1_GCC_ATTR_DEPRECATED __attribute__ ((__deprecated__))
# endif
# endif
# ifndef _ASN1_GCC_ATTR_DEPRECATED
# define _ASN1_GCC_ATTR_DEPRECATED
# endif
/*****************************************/
/* Errors returned by libtasn1 functions */
/*****************************************/
# define ASN1_SUCCESS 0
# define ASN1_FILE_NOT_FOUND 1
# define ASN1_ELEMENT_NOT_FOUND 2
# define ASN1_IDENTIFIER_NOT_FOUND 3
# define ASN1_DER_ERROR 4
# define ASN1_VALUE_NOT_FOUND 5
# define ASN1_GENERIC_ERROR 6
# define ASN1_VALUE_NOT_VALID 7
# define ASN1_TAG_ERROR 8
# define ASN1_TAG_IMPLICIT 9
# define ASN1_ERROR_TYPE_ANY 10
# define ASN1_SYNTAX_ERROR 11
# define ASN1_MEM_ERROR 12
# define ASN1_MEM_ALLOC_ERROR 13
# define ASN1_DER_OVERFLOW 14
# define ASN1_NAME_TOO_LONG 15
# define ASN1_ARRAY_ERROR 16
# define ASN1_ELEMENT_NOT_EMPTY 17
# define ASN1_TIME_ENCODING_ERROR 18
# define ASN1_RECURSION 19
/*************************************/
/* Constants used in asn1_visit_tree */
/*************************************/
# define ASN1_PRINT_NAME 1
# define ASN1_PRINT_NAME_TYPE 2
# define ASN1_PRINT_NAME_TYPE_VALUE 3
# define ASN1_PRINT_ALL 4
/*****************************************/
/* Constants returned by asn1_read_tag */
/*****************************************/
# define ASN1_CLASS_UNIVERSAL 0x00 /* old: 1 */
# define ASN1_CLASS_APPLICATION 0x40 /* old: 2 */
# define ASN1_CLASS_CONTEXT_SPECIFIC 0x80 /* old: 3 */
# define ASN1_CLASS_PRIVATE 0xC0 /* old: 4 */
# define ASN1_CLASS_STRUCTURED 0x20
/*****************************************/
/* Constants returned by asn1_read_tag */
/*****************************************/
# define ASN1_TAG_BOOLEAN 0x01
# define ASN1_TAG_INTEGER 0x02
# define ASN1_TAG_SEQUENCE 0x10
# define ASN1_TAG_SET 0x11
# define ASN1_TAG_OCTET_STRING 0x04
# define ASN1_TAG_BIT_STRING 0x03
# define ASN1_TAG_UTCTime 0x17
# define ASN1_TAG_GENERALIZEDTime 0x18
# define ASN1_TAG_OBJECT_ID 0x06
# define ASN1_TAG_ENUMERATED 0x0A
# define ASN1_TAG_NULL 0x05
# define ASN1_TAG_GENERALSTRING 0x1B
# define ASN1_TAG_NUMERIC_STRING 0x12
# define ASN1_TAG_IA5_STRING 0x16
# define ASN1_TAG_TELETEX_STRING 0x14
# define ASN1_TAG_PRINTABLE_STRING 0x13
# define ASN1_TAG_UNIVERSAL_STRING 0x1C
# define ASN1_TAG_BMP_STRING 0x1E
# define ASN1_TAG_UTF8_STRING 0x0C
# define ASN1_TAG_VISIBLE_STRING 0x1A
/**
* asn1_node:
*
* Structure definition used for the node of the tree
* that represents an ASN.1 DEFINITION.
*/
typedef struct asn1_node_st asn1_node_st;
typedef asn1_node_st *asn1_node;
typedef const asn1_node_st *asn1_node_const;
/**
* ASN1_MAX_NAME_SIZE:
*
* Maximum number of characters of a name
* inside a file with ASN1 definitions.
*/
# define ASN1_MAX_NAME_SIZE 64
/**
* asn1_static_node:
* @name: Node name
* @type: Node typ
* @value: Node value
*
* For the on-disk format of ASN.1 trees, created by asn1_parser2array().
*/
typedef struct asn1_static_node_st
{
const char *name; /* Node name */
unsigned int type; /* Node type */
const void *value; /* Node value */
} asn1_static_node;
/* List of constants for field type of asn1_static_node */
# define ASN1_ETYPE_INVALID 0
# define ASN1_ETYPE_CONSTANT 1
# define ASN1_ETYPE_IDENTIFIER 2
# define ASN1_ETYPE_INTEGER 3
# define ASN1_ETYPE_BOOLEAN 4
# define ASN1_ETYPE_SEQUENCE 5
# define ASN1_ETYPE_BIT_STRING 6
# define ASN1_ETYPE_OCTET_STRING 7
# define ASN1_ETYPE_TAG 8
# define ASN1_ETYPE_DEFAULT 9
# define ASN1_ETYPE_SIZE 10
# define ASN1_ETYPE_SEQUENCE_OF 11
# define ASN1_ETYPE_OBJECT_ID 12
# define ASN1_ETYPE_ANY 13
# define ASN1_ETYPE_SET 14
# define ASN1_ETYPE_SET_OF 15
# define ASN1_ETYPE_DEFINITIONS 16
# define ASN1_ETYPE_CHOICE 18
# define ASN1_ETYPE_IMPORTS 19
# define ASN1_ETYPE_NULL 20
# define ASN1_ETYPE_ENUMERATED 21
# define ASN1_ETYPE_GENERALSTRING 27
# define ASN1_ETYPE_NUMERIC_STRING 28
# define ASN1_ETYPE_IA5_STRING 29
# define ASN1_ETYPE_TELETEX_STRING 30
# define ASN1_ETYPE_PRINTABLE_STRING 31
# define ASN1_ETYPE_UNIVERSAL_STRING 32
# define ASN1_ETYPE_BMP_STRING 33
# define ASN1_ETYPE_UTF8_STRING 34
# define ASN1_ETYPE_VISIBLE_STRING 35
# define ASN1_ETYPE_UTC_TIME 36
# define ASN1_ETYPE_GENERALIZED_TIME 37
/**
* ASN1_DELETE_FLAG_ZEROIZE:
*
* Used by: asn1_delete_structure2()
*
* Zeroize values prior to deinitialization.
*/
# define ASN1_DELETE_FLAG_ZEROIZE 1
/**
* ASN1_DECODE_FLAG_ALLOW_PADDING:
*
* Used by: asn1_der_decoding2()
*
* This flag would allow arbitrary data past the DER data.
*/
# define ASN1_DECODE_FLAG_ALLOW_PADDING 1
/**
* ASN1_DECODE_FLAG_STRICT_DER:
*
* Used by: asn1_der_decoding2()
*
* This flag would ensure that no BER decoding takes place.
*/
# define ASN1_DECODE_FLAG_STRICT_DER (1<<1)
/**
* ASN1_DECODE_FLAG_ALLOW_INCORRECT_TIME:
*
* Used by: asn1_der_decoding2()
*
* This flag will tolerate Time encoding errors when in strict DER.
*/
# define ASN1_DECODE_FLAG_ALLOW_INCORRECT_TIME (1<<2)
/* *INDENT-OFF* */
/**
* asn1_data_node_st:
* @name: Node name
* @value: Node value
* @value_len: Node value size
* @type: Node value type (ASN1_ETYPE_*)
*
* Data node inside a #asn1_node structure.
*
没有合适的资源?快使用搜索试试~ 我知道了~
【QGIS跨平台编译】之【libtasn1跨平台编译】:MacOS环境下编译成果(支撑QGIS跨平台编译,以及二次研发)
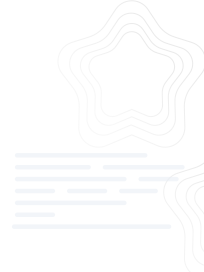
共3个文件
dylib:2个
h:1个

0 下载量 177 浏览量
2024-04-06
11:41:12
上传
评论
收藏 79KB ZIP 举报
温馨提示
一、内容概况 QGIS是一个开源的、跨平台的地理信息系统(GIS)软件,用于浏览、编辑和分析地理空间数据,提供了一套丰富的功能,包括地图制作、空间分析、数据管理等。QGIS可以在Windows、Mac OS和Linux等操作系统上运行。 QGIS的跨平台编译需要一系列开源库的支持,本系列提供QGIS相关的编译成果。 本资源的内容为:基于Qt的libtasn1跨平台编译成果(MacOS版本)。 二、使用人群 QGIS编译、QGIS跨平台编译的人员或研究者。 三、使用场景及目标 在MacOS环境下使用。 既可以支撑QGIS在MacOS环境下的编译工作,也可以进行libtasn1的二次研发。 四、其他说明 在MacOS环境下,基于Qt Creator进行编译的libtasn1开源库。包含有头文件include、库文件dylib等,提供了Debug、Release版本。 当前采用的版本为libtasn1-4.19.0,如果下载者,需要其他版本的libtasn1,请在评论区留言。
资源推荐
资源详情
资源评论
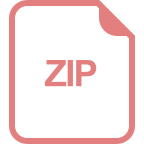
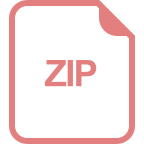
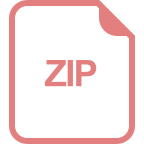
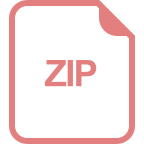
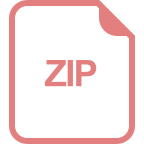
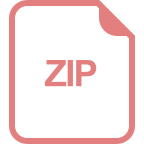
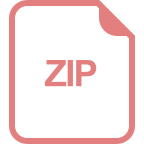
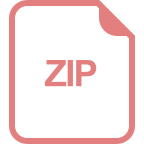
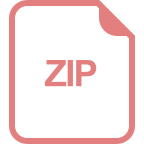
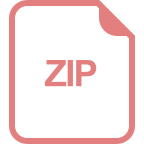
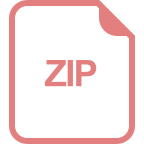
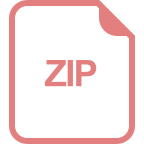
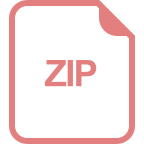
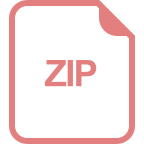
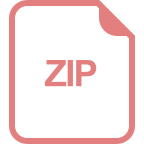
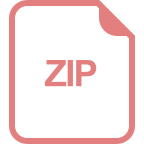
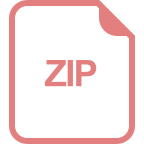
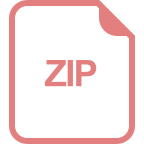
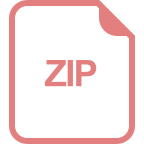
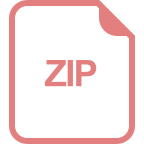
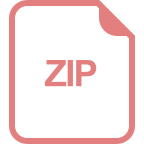
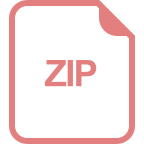
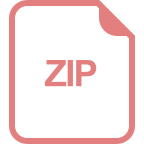
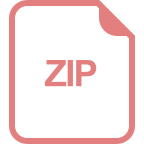
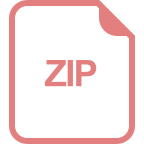
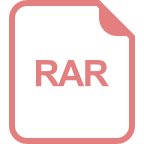
收起资源包目录


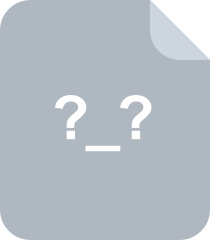

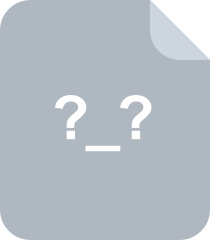
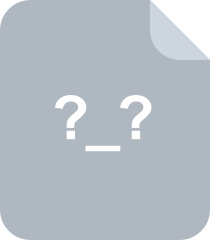

共 3 条
- 1
资源评论
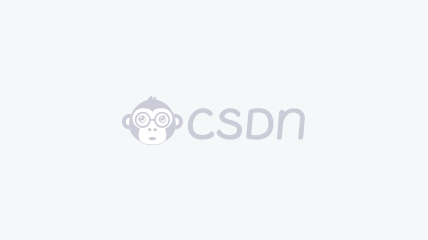

翰墨之道
- 粉丝: 3618
- 资源: 182
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

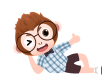
最新资源
- 基于SpringBoot的学生心理咨询评估平台
- 开题报告springboot005学生心理咨询评估系统开题.doc
- Java-springboot大学生心理咨询管理系统计算机毕业设计程序.zip
- 第二届阿里巴巴大数据智能云上编程大赛冠军解决方案.zip
- 开题报告springboot019高校心理教育辅导设计与实现开题报告
- STM32 定时器的使用
- 掌上客网页小程序前端+后端 开源版本.zip
- 线上迁移大表数据.zip
- EPSON-L3110 清零软件
- 2、Python量化交易-三剑客之pandas ==== 对应的jupyter笔记
- linux-lite-7.0下载种子文件
- 2023.1-2024.4城市空气质量指数数据(月度)(含PM2.5、PM10、SO2、CO、NO2、O3)
- java 小游戏,个人学习整理,仅供参考
- java实现2048小游戏的代码
- 佳能打印机通用清零软件
- 小功率调幅发射机(仿真+报告)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


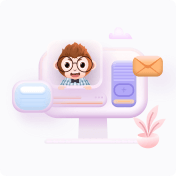
安全验证
文档复制为VIP权益,开通VIP直接复制
