/*
* The copyright in this software is being made available under the 2-clauses
* BSD License, included below. This software may be subject to other third
* party and contributor rights, including patent rights, and no such rights
* are granted under this license.
*
* Copyright (c) 2002-2014, Universite catholique de Louvain (UCL), Belgium
* Copyright (c) 2002-2014, Professor Benoit Macq
* Copyright (c) 2001-2003, David Janssens
* Copyright (c) 2002-2003, Yannick Verschueren
* Copyright (c) 2003-2007, Francois-Olivier Devaux
* Copyright (c) 2003-2014, Antonin Descampe
* Copyright (c) 2005, Herve Drolon, FreeImage Team
* Copyright (c) 2006-2007, Parvatha Elangovan
* Copyright (c) 2008, Jerome Fimes, Communications & Systemes <jerome.fimes@c-s.fr>
* Copyright (c) 2010-2011, Kaori Hagihara
* Copyright (c) 2011-2012, Centre National d'Etudes Spatiales (CNES), France
* Copyright (c) 2012, CS Systemes d'Information, France
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS `AS IS'
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE
* LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR
* CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF
* SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS
* INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN
* CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
* ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE
* POSSIBILITY OF SUCH DAMAGE.
*/
#ifndef OPENJPEG_H
#define OPENJPEG_H
/*
==========================================================
Compiler directives
==========================================================
*/
/*
The inline keyword is supported by C99 but not by C90.
Most compilers implement their own version of this keyword ...
*/
#ifndef INLINE
#if defined(_MSC_VER)
#define INLINE __forceinline
#elif defined(__GNUC__)
#define INLINE __inline__
#elif defined(__MWERKS__)
#define INLINE inline
#else
/* add other compilers here ... */
#define INLINE
#endif /* defined(<Compiler>) */
#endif /* INLINE */
/* deprecated attribute */
#ifdef __GNUC__
#define OPJ_DEPRECATED(func) func __attribute__ ((deprecated))
#elif defined(_MSC_VER)
#define OPJ_DEPRECATED(func) __declspec(deprecated) func
#else
#pragma message("WARNING: You need to implement DEPRECATED for this compiler")
#define OPJ_DEPRECATED(func) func
#endif
#if defined(__GNUC__) && __GNUC__ >= 6
#define OPJ_DEPRECATED_STRUCT_MEMBER(memb, msg) __attribute__ ((deprecated(msg))) memb
#else
#define OPJ_DEPRECATED_STRUCT_MEMBER(memb, msg) memb
#endif
#if defined(OPJ_STATIC) || !defined(_WIN32)
/* http://gcc.gnu.org/wiki/Visibility */
# if !defined(_WIN32) && __GNUC__ >= 4
# if defined(OPJ_STATIC) /* static library uses "hidden" */
# define OPJ_API __attribute__ ((visibility ("hidden")))
# else
# define OPJ_API __attribute__ ((visibility ("default")))
# endif
# define OPJ_LOCAL __attribute__ ((visibility ("hidden")))
# else
# define OPJ_API
# define OPJ_LOCAL
# endif
# define OPJ_CALLCONV
#else
# define OPJ_CALLCONV __stdcall
/*
The following ifdef block is the standard way of creating macros which make exporting
from a DLL simpler. All files within this DLL are compiled with the OPJ_EXPORTS
symbol defined on the command line. this symbol should not be defined on any project
that uses this DLL. This way any other project whose source files include this file see
OPJ_API functions as being imported from a DLL, whereas this DLL sees symbols
defined with this macro as being exported.
*/
# if defined(OPJ_EXPORTS) || defined(DLL_EXPORT)
# define OPJ_API __declspec(dllexport)
# else
# define OPJ_API __declspec(dllimport)
# endif /* OPJ_EXPORTS */
#endif /* !OPJ_STATIC || !_WIN32 */
typedef int OPJ_BOOL;
#define OPJ_TRUE 1
#define OPJ_FALSE 0
typedef char OPJ_CHAR;
typedef float OPJ_FLOAT32;
typedef double OPJ_FLOAT64;
typedef unsigned char OPJ_BYTE;
#include "opj_stdint.h"
typedef int8_t OPJ_INT8;
typedef uint8_t OPJ_UINT8;
typedef int16_t OPJ_INT16;
typedef uint16_t OPJ_UINT16;
typedef int32_t OPJ_INT32;
typedef uint32_t OPJ_UINT32;
typedef int64_t OPJ_INT64;
typedef uint64_t OPJ_UINT64;
typedef int64_t OPJ_OFF_T; /* 64-bit file offset type */
#include <stdio.h>
typedef size_t OPJ_SIZE_T;
/* Avoid compile-time warning because parameter is not used */
#define OPJ_ARG_NOT_USED(x) (void)(x)
/*
==========================================================
Useful constant definitions
==========================================================
*/
#define OPJ_PATH_LEN 4096 /**< Maximum allowed size for filenames */
#define OPJ_J2K_MAXRLVLS 33 /**< Number of maximum resolution level authorized */
#define OPJ_J2K_MAXBANDS (3*OPJ_J2K_MAXRLVLS-2) /**< Number of maximum sub-band linked to number of resolution level */
#define OPJ_J2K_DEFAULT_NB_SEGS 10
#define OPJ_J2K_STREAM_CHUNK_SIZE 0x100000 /** 1 mega by default */
#define OPJ_J2K_DEFAULT_HEADER_SIZE 1000
#define OPJ_J2K_MCC_DEFAULT_NB_RECORDS 10
#define OPJ_J2K_MCT_DEFAULT_NB_RECORDS 10
/* UniPG>> */ /* NOT YET USED IN THE V2 VERSION OF OPENJPEG */
#define JPWL_MAX_NO_TILESPECS 16 /**< Maximum number of tile parts expected by JPWL: increase at your will */
#define JPWL_MAX_NO_PACKSPECS 16 /**< Maximum number of packet parts expected by JPWL: increase at your will */
#define JPWL_MAX_NO_MARKERS 512 /**< Maximum number of JPWL markers: increase at your will */
#define JPWL_PRIVATEINDEX_NAME "jpwl_index_privatefilename" /**< index file name used when JPWL is on */
#define JPWL_EXPECTED_COMPONENTS 3 /**< Expect this number of components, so you'll find better the first EPB */
#define JPWL_MAXIMUM_TILES 8192 /**< Expect this maximum number of tiles, to avoid some crashes */
#define JPWL_MAXIMUM_HAMMING 2 /**< Expect this maximum number of bit errors in marker id's */
#define JPWL_MAXIMUM_EPB_ROOM 65450 /**< Expect this maximum number of bytes for composition of EPBs */
/* <<UniPG */
/**
* EXPERIMENTAL FOR THE MOMENT
* Supported options about file information used only in j2k_dump
*/
#define OPJ_IMG_INFO 1 /**< Basic image information provided to the user */
#define OPJ_J2K_MH_INFO 2 /**< Codestream information based only on the main header */
#define OPJ_J2K_TH_INFO 4 /**< Tile information based on the current tile header */
#define OPJ_J2K_TCH_INFO 8 /**< Tile/Component information of all tiles */
#define OPJ_J2K_MH_IND 16 /**< Codestream index based only on the main header */
#define OPJ_J2K_TH_IND 32 /**< Tile index based on the current tile */
/*FIXME #define OPJ_J2K_CSTR_IND 48*/ /**< */
#define OPJ_JP2_INFO 128 /**< JP2 file information */
#define OPJ_JP2_IND 256 /**< JP2 file index */
/**
* JPEG 2000 Profiles, see Table A.10 from 15444-1 (updated in various AMD)
* These values help choosing the RSIZ value for the J2K codestream.
* The RSIZ value triggers various encoding options, as detailed in Table A.10.
* If OPJ_PROFILE_PART2 is chosen, it has to be combined with one or more extensions
* described hereunder.
* Example: rsiz
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
一、内容概况 QGIS是一个开源的、跨平台的地理信息系统(GIS)软件,用于浏览、编辑和分析地理空间数据,提供了一套丰富的功能,包括地图制作、空间分析、数据管理等。QGIS可以在Windows、Mac OS和Linux等操作系统上运行。 QGIS的跨平台编译需要一系列开源库的支持,本系列提供QGIS相关的编译成果。 本资源的内容为:基于Qt的OpenJpeg跨平台编译成果(Linux版本)。 二、使用人群 QGIS编译、QGIS跨平台编译的人员或研究者。 三、使用场景及目标 在Linux环境下使用。 既可以支撑QGIS在Linux环境下的编译工作,也可以进行OpenJpeg的二次研发。 四、其他说明 在Linux环境下,基于Qt Creator进行编译的OpenJpeg开源库。包含有头文件include、库文件so等,提供了Debug、Release版本。 当前采用的版本为OpenJpeg-2.5.0,如果下载者,需要其他版本的OpenJpeg,请在评论区留言。
资源推荐
资源详情
资源评论
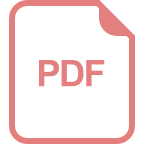
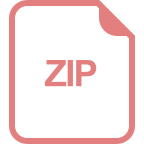
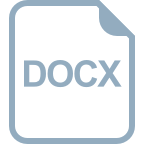
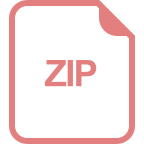
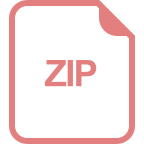
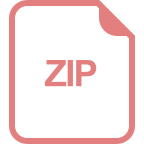
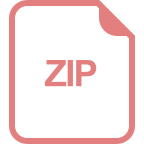
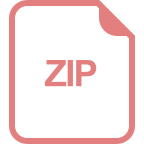
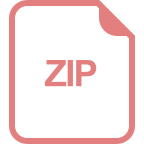
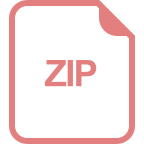
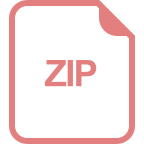
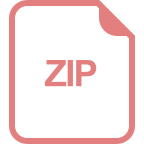
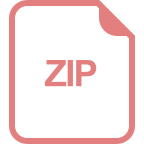
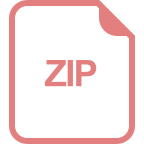
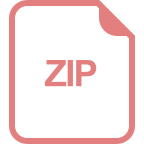
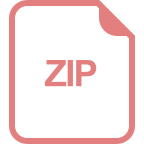
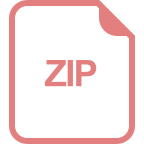
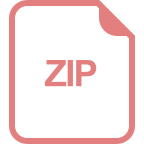
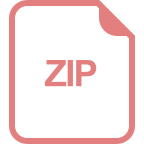
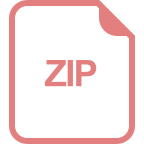
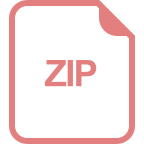
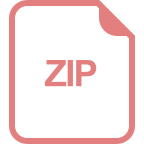
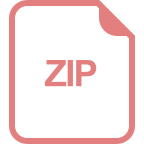
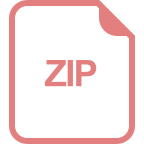
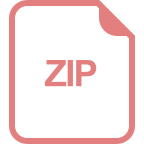
收起资源包目录



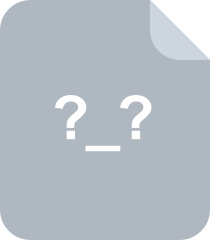
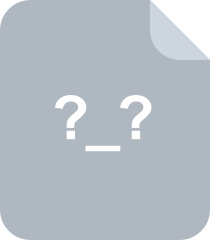
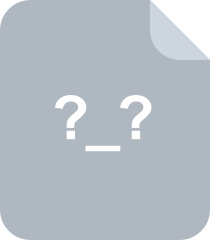

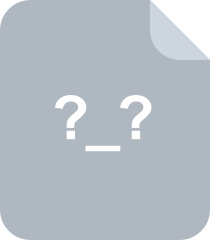
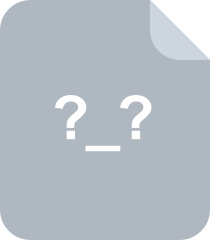

共 5 条
- 1
资源评论
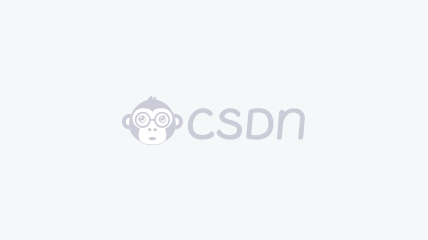

翰墨之道
- 粉丝: 3584
- 资源: 182
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

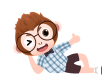
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


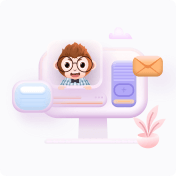
安全验证
文档复制为VIP权益,开通VIP直接复制
