/* Determine a canonical name for the current locale's character encoding.
Copyright (C) 2000-2006, 2008-2019 Free Software Foundation, Inc.
This program is free software; you can redistribute it and/or modify it
under the terms of the GNU Lesser General Public License as published
by the Free Software Foundation; either version 2, or (at your option)
any later version.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public License
along with this program; if not, see <https://www.gnu.org/licenses/>. */
/* Written by Bruno Haible <bruno@clisp.org>. */
#include <config.h>
/* Specification. */
#include "localcharset.h"
#include <stddef.h>
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#if defined __APPLE__ && defined __MACH__ && HAVE_LANGINFO_CODESET
# define DARWIN7 /* Darwin 7 or newer, i.e. Mac OS X 10.3 or newer */
#endif
#if defined _WIN32 && !defined __CYGWIN__
# define WINDOWS_NATIVE
# include <locale.h>
#endif
#if defined __EMX__
/* Assume EMX program runs on OS/2, even if compiled under DOS. */
# ifndef OS2
# define OS2
# endif
#endif
#if !defined WINDOWS_NATIVE
# if HAVE_LANGINFO_CODESET
# include <langinfo.h>
# else
# if 0 /* see comment regarding use of setlocale(), below */
# include <locale.h>
# endif
# endif
# ifdef __CYGWIN__
# define WIN32_LEAN_AND_MEAN
# include <windows.h>
# endif
#elif defined WINDOWS_NATIVE
# define WIN32_LEAN_AND_MEAN
# include <windows.h>
/* For the use of setlocale() below, the Gnulib override in setlocale.c is
not needed; see the platform lists in setlocale_null.m4. */
# undef setlocale
#endif
#if defined OS2
# define INCL_DOS
# include <os2.h>
#endif
/* For MB_CUR_MAX_L */
#if defined DARWIN7
# include <xlocale.h>
#endif
#if HAVE_LANGINFO_CODESET || defined WINDOWS_NATIVE || defined OS2
/* On these platforms, we use a mapping from non-canonical encoding name
to GNU canonical encoding name. */
/* With glibc-2.1 or newer, we don't need any canonicalization,
because glibc has iconv and both glibc and libiconv support all
GNU canonical names directly. */
# if !((defined __GNU_LIBRARY__ && __GLIBC__ >= 2) || defined __UCLIBC__)
struct table_entry
{
const char alias[11+1];
const char canonical[11+1];
};
/* Table of platform-dependent mappings, sorted in ascending order. */
static const struct table_entry alias_table[] =
{
# if defined __FreeBSD__ /* FreeBSD */
/*{ "ARMSCII-8", "ARMSCII-8" },*/
{ "Big5", "BIG5" },
{ "C", "ASCII" },
/*{ "CP1131", "CP1131" },*/
/*{ "CP1251", "CP1251" },*/
/*{ "CP866", "CP866" },*/
/*{ "GB18030", "GB18030" },*/
/*{ "GB2312", "GB2312" },*/
/*{ "GBK", "GBK" },*/
/*{ "ISCII-DEV", "?" },*/
{ "ISO8859-1", "ISO-8859-1" },
{ "ISO8859-13", "ISO-8859-13" },
{ "ISO8859-15", "ISO-8859-15" },
{ "ISO8859-2", "ISO-8859-2" },
{ "ISO8859-5", "ISO-8859-5" },
{ "ISO8859-7", "ISO-8859-7" },
{ "ISO8859-9", "ISO-8859-9" },
/*{ "KOI8-R", "KOI8-R" },*/
/*{ "KOI8-U", "KOI8-U" },*/
{ "SJIS", "SHIFT_JIS" },
{ "US-ASCII", "ASCII" },
{ "eucCN", "GB2312" },
{ "eucJP", "EUC-JP" },
{ "eucKR", "EUC-KR" }
# define alias_table_defined
# endif
# if defined __NetBSD__ /* NetBSD */
{ "646", "ASCII" },
/*{ "ARMSCII-8", "ARMSCII-8" },*/
/*{ "BIG5", "BIG5" },*/
{ "Big5-HKSCS", "BIG5-HKSCS" },
/*{ "CP1251", "CP1251" },*/
/*{ "CP866", "CP866" },*/
/*{ "GB18030", "GB18030" },*/
/*{ "GB2312", "GB2312" },*/
{ "ISO8859-1", "ISO-8859-1" },
{ "ISO8859-13", "ISO-8859-13" },
{ "ISO8859-15", "ISO-8859-15" },
{ "ISO8859-2", "ISO-8859-2" },
{ "ISO8859-4", "ISO-8859-4" },
{ "ISO8859-5", "ISO-8859-5" },
{ "ISO8859-7", "ISO-8859-7" },
/*{ "KOI8-R", "KOI8-R" },*/
/*{ "KOI8-U", "KOI8-U" },*/
/*{ "PT154", "PT154" },*/
{ "SJIS", "SHIFT_JIS" },
{ "eucCN", "GB2312" },
{ "eucJP", "EUC-JP" },
{ "eucKR", "EUC-KR" },
{ "eucTW", "EUC-TW" }
# define alias_table_defined
# endif
# if defined __OpenBSD__ /* OpenBSD */
{ "646", "ASCII" },
{ "ISO8859-1", "ISO-8859-1" },
{ "ISO8859-13", "ISO-8859-13" },
{ "ISO8859-15", "ISO-8859-15" },
{ "ISO8859-2", "ISO-8859-2" },
{ "ISO8859-4", "ISO-8859-4" },
{ "ISO8859-5", "ISO-8859-5" },
{ "ISO8859-7", "ISO-8859-7" },
{ "US-ASCII", "ASCII" }
# define alias_table_defined
# endif
# if defined __APPLE__ && defined __MACH__ /* Mac OS X */
/* Darwin 7.5 has nl_langinfo(CODESET), but sometimes its value is
useless:
- It returns the empty string when LANG is set to a locale of the
form ll_CC, although ll_CC/LC_CTYPE is a symlink to an UTF-8
LC_CTYPE file.
- The environment variables LANG, LC_CTYPE, LC_ALL are not set by
the system; nl_langinfo(CODESET) returns "US-ASCII" in this case.
- The documentation says:
"... all code that calls BSD system routines should ensure
that the const *char parameters of these routines are in UTF-8
encoding. All BSD system functions expect their string
parameters to be in UTF-8 encoding and nothing else."
It also says
"An additional caveat is that string parameters for files,
paths, and other file-system entities must be in canonical
UTF-8. In a canonical UTF-8 Unicode string, all decomposable
characters are decomposed ..."
but this is not true: You can pass non-decomposed UTF-8 strings
to file system functions, and it is the OS which will convert
them to decomposed UTF-8 before accessing the file system.
- The Apple Terminal application displays UTF-8 by default.
- However, other applications are free to use different encodings:
- xterm uses ISO-8859-1 by default.
- TextEdit uses MacRoman by default.
We prefer UTF-8 over decomposed UTF-8-MAC because one should
minimize the use of decomposed Unicode. Unfortunately, through the
Darwin file system, decomposed UTF-8 strings are leaked into user
space nevertheless.
Then there are also the locales with encodings other than US-ASCII
and UTF-8. These locales can be occasionally useful to users (e.g.
when grepping through ISO-8859-1 encoded text files), when all their
file names are in US-ASCII.
*/
{ "ARMSCII-8", "ARMSCII-8" },
{ "Big5", "BIG5" },
{ "Big5HKSCS", "BIG5-HKSCS" },
{ "CP1131", "CP1131" },
{ "CP1251", "CP1251" },
{ "CP866", "CP866" },
{ "CP949", "CP949" },
{ "GB18030", "GB18030" },
{ "GB2312", "GB2312" },
{ "GBK", "GBK" },
/*{ "ISCII-DEV", "?" },*/
{ "ISO8859-1", "ISO-8859-1" },
{ "ISO8859-13", "ISO-8859-13" },
{ "ISO8859-15", "ISO-8859-15" },
{ "ISO8859-2", "ISO-8859-2" },
{ "ISO8859-4", "ISO-8859-4" },
{ "ISO8859-5", "ISO-8859-5" },
{ "ISO8859-7", "ISO-8859-7" },
{ "ISO8859-9", "ISO-8859-9" },
{ "KOI8-R", "KOI8-R" },
{ "KOI8-U", "KOI8-U" },
{ "PT154", "PT154" },
{ "SJIS", "SHIFT_JIS" },
{ "eucCN", "GB2312" },
{ "eucJP", "EUC-JP" },
{ "eucKR", "EUC-KR" }
# define alias_table_defined
# endif
# if defined _AIX /* AIX */
/*{ "GBK", "GBK" },*/
{ "IBM-1046", "CP1046" },
{ "IBM-1124", "CP1124" },
{
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
一、内容概况 QGIS是一个开源的、跨平台的地理信息系统(GIS)软件,用于浏览、编辑和分析地理空间数据,提供了一套丰富的功能,包括地图制作、空间分析、数据管理等。QGIS可以在Windows、Mac OS和Linux等操作系统上运行。 QGIS的跨平台编译需要一系列开源库的支持,本系列提供QGIS相关的编译成果。 本资源的内容为:基于Qt的iconv跨平台编译源码(含qt pro文件)。 二、使用人群 QGIS编译、QGIS跨平台编译的人员或研究者。 三、使用场景及目标 在Windows、Linux、MacOS环境下编译使用。 既可以支撑QGIS的跨平台编译工作,也可以进行iconv的二次研发。 四、其他说明 基于Qt Creator进行跨平台编译的iconv工程源码。包含有各类源码,以及配置好的Qt工程文件。 只需用Qt Creator程序打开pro文件,即可完成在Windows、Linux、MacOS等多环境下的跨平台编译。编译后会自动生成头文件、库文件、动态库等。 当前采用的版本为iconv-1.17,如果下载者,需要其他版本的iconv,请在评论区留言。
资源推荐
资源详情
资源评论
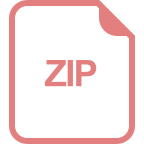
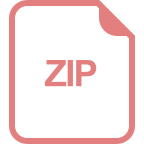
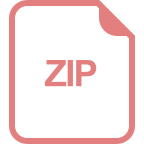
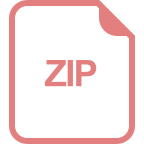
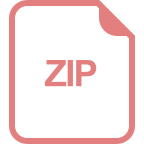
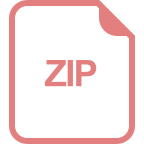
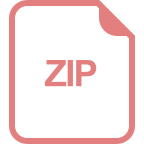
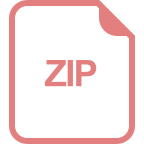
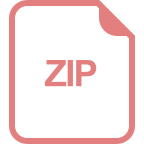
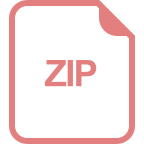
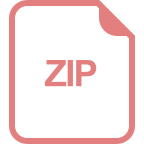
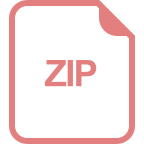
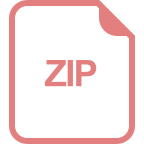
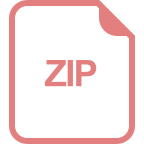
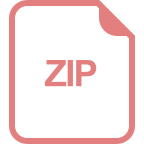
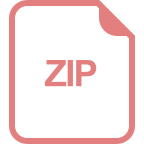
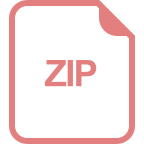
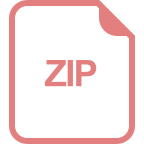
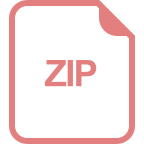
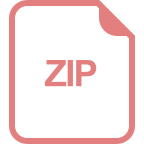
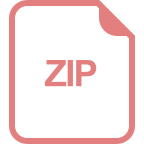
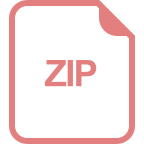
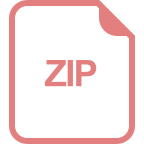
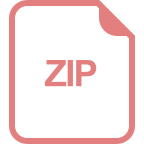
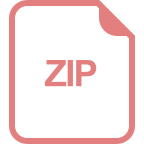
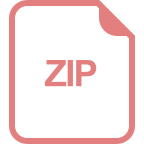
收起资源包目录

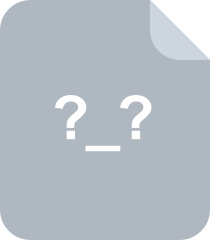
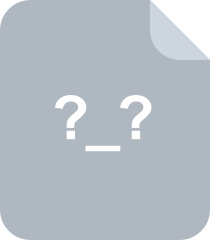
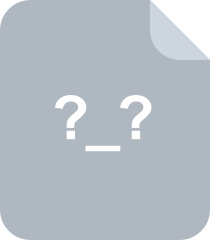
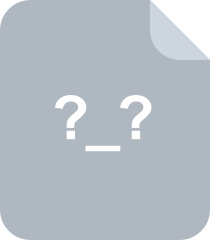
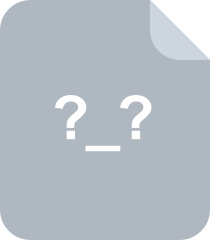
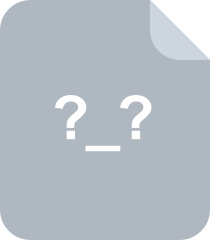
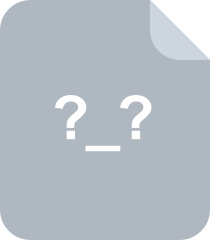
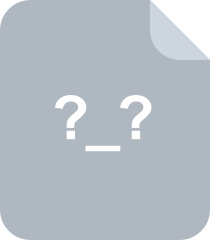
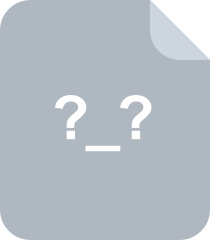
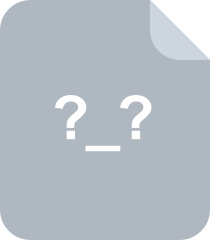
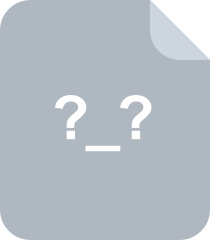
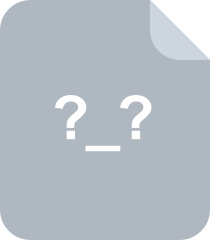
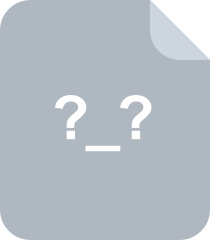
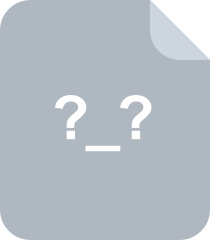
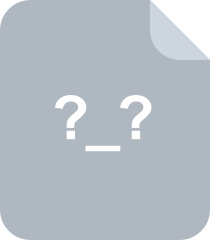
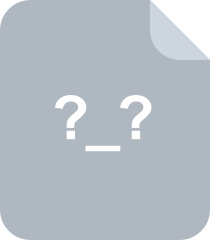
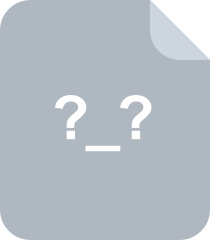
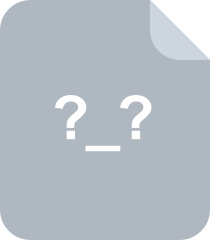
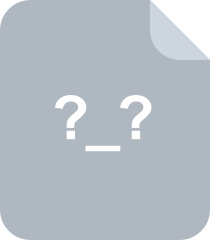
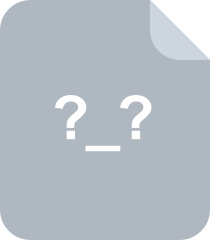
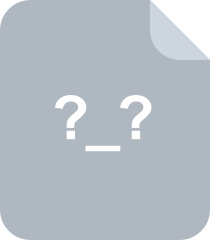
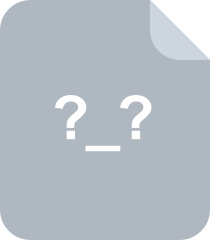
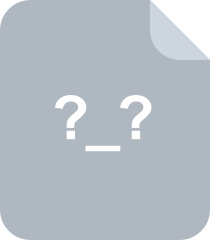
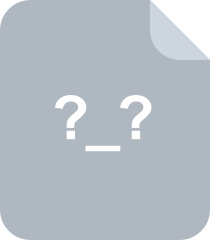
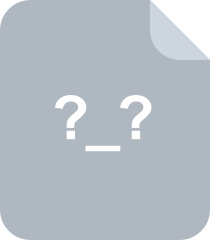
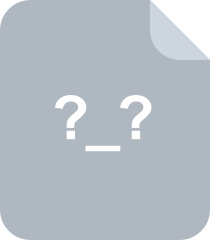
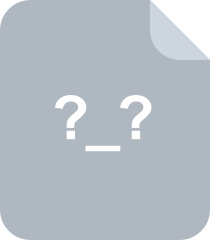
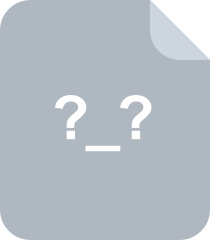
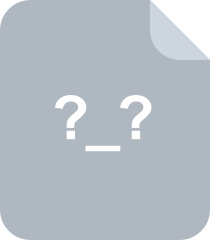
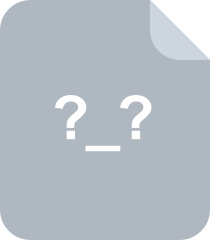
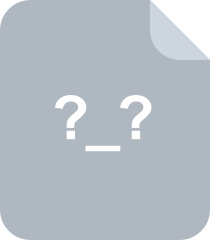
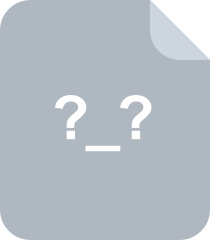
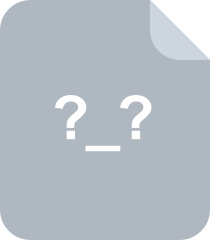
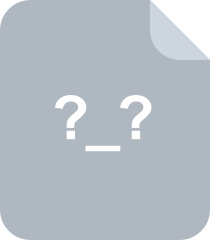
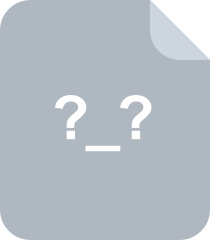
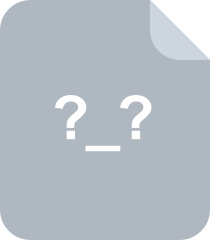
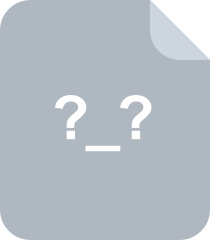
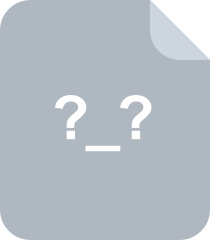
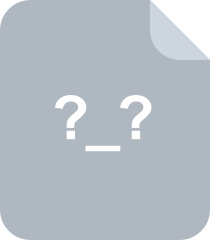
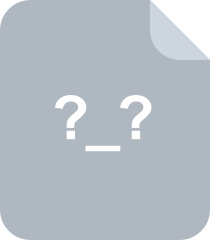
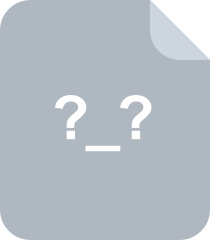
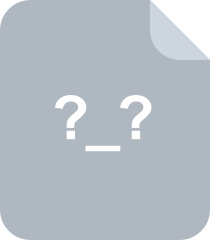
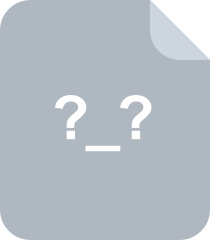
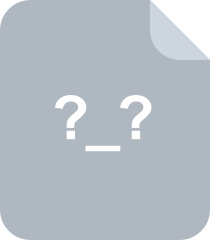
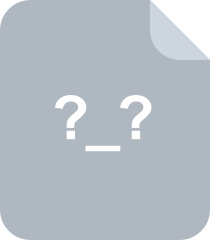
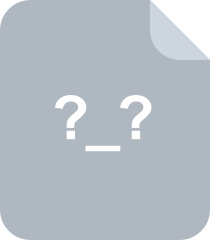
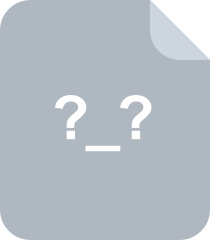
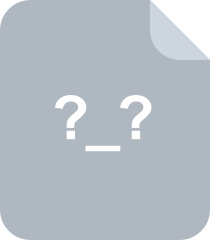
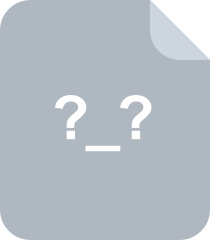
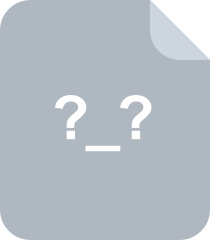
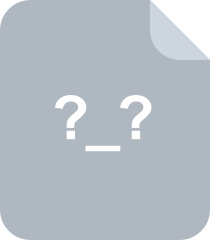
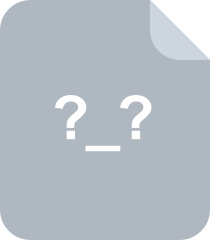
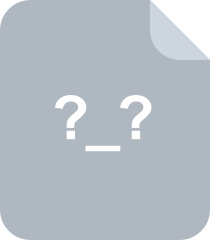
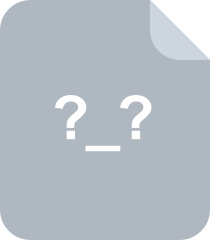
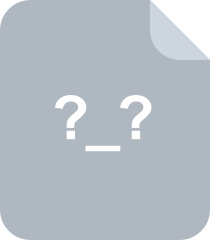
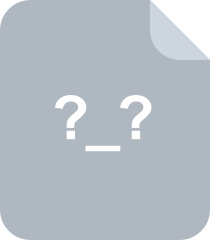
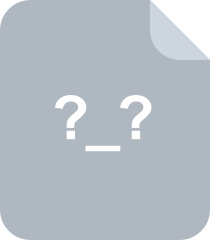
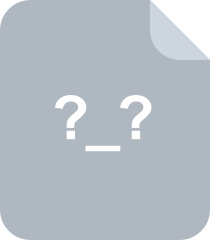
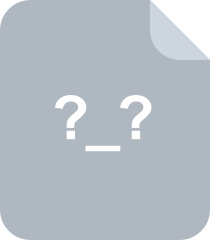
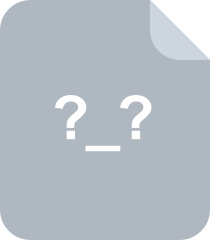
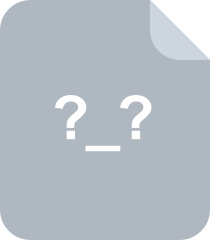
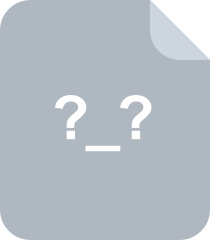
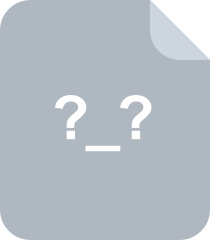
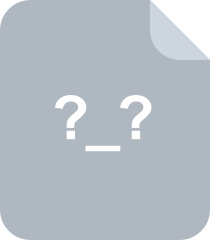
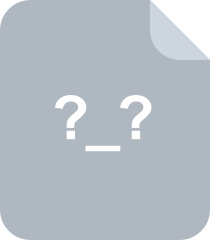
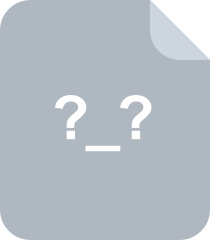
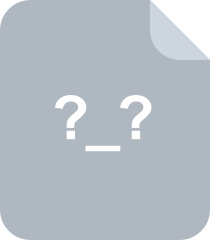
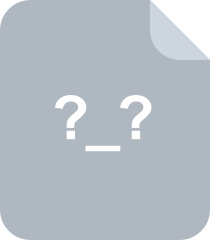
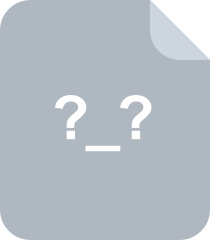
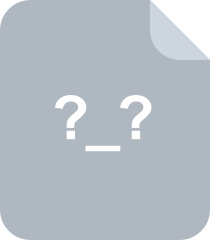
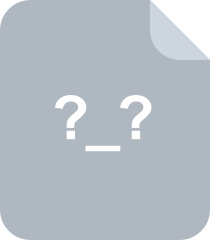
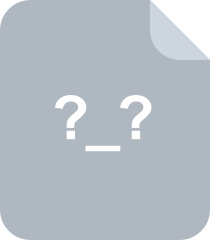
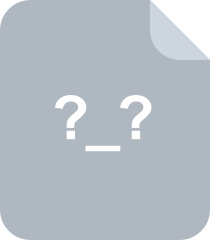
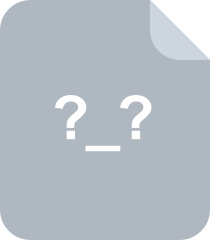
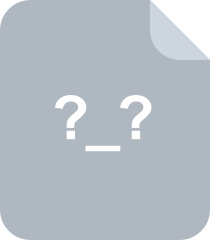
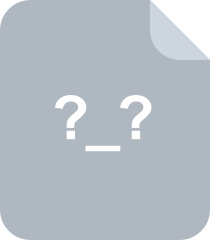
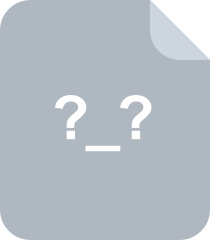
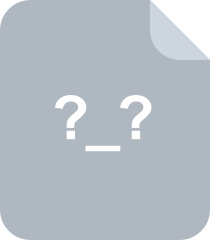
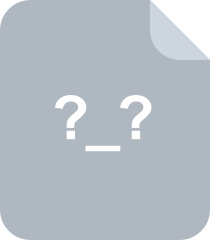
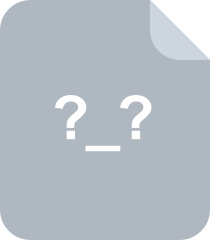
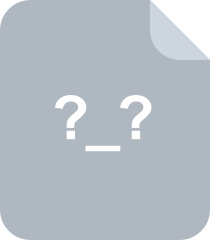
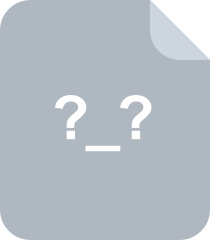
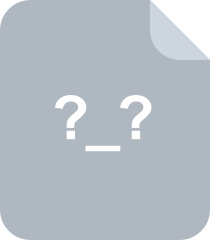
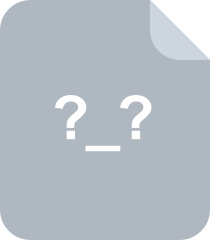
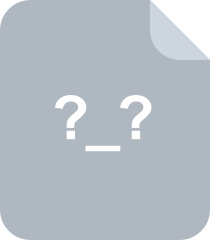
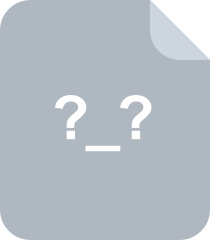
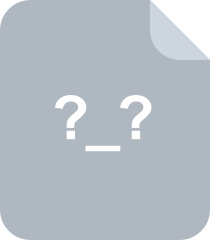
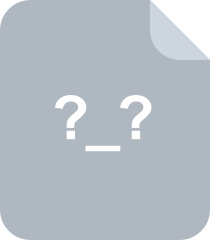
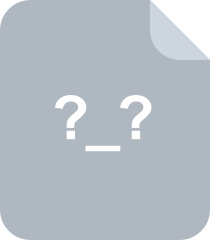
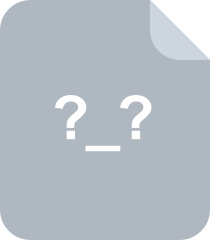
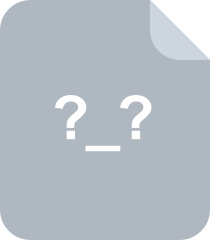
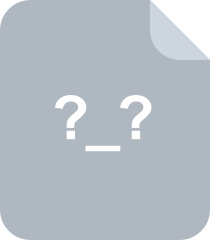
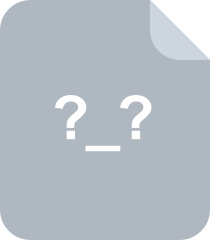
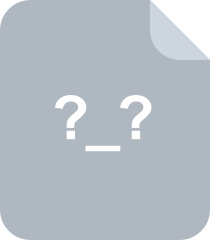
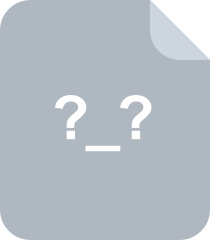
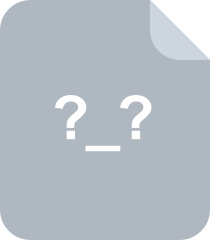
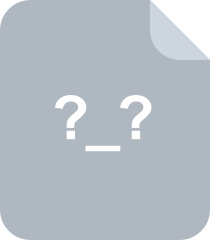
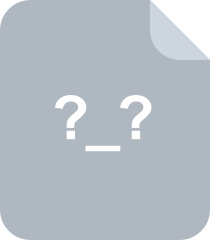
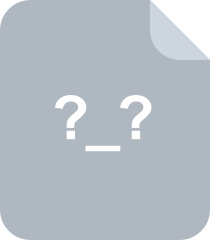
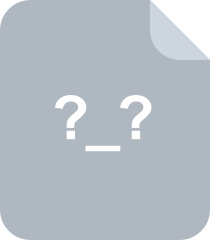
共 299 条
- 1
- 2
- 3
资源评论
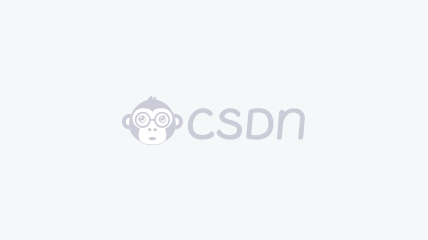

翰墨之道
- 粉丝: 3608
- 资源: 182
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

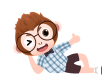
最新资源
- 東耳篮球馆会员信息管理系统(编号:98721117).zip
- 房屋系统(编号:45266146).zip
- 大学生志愿者信息管理系统(编号:96654262).zip
- 房屋租赁系统(编号:49930163).zip
- 付费自习室管理系统(编号:46724236)(1).zip
- 学术论文撰写技巧:施一公提高英文论文写作能力的六点建议
- 科研真问题从何而来-中科院院士分享
- 通过matlab语言读取csv文件.zip
- 通过Django实现用户注册和登录的简单认证系统.zip
- 通过汇编语言计算两个整数和,将结果存储在另一个变量中.zip
- Aruba%20Instant%20On_2.3.0_apk-dl.com.apk.1.1
- Ruby参考手册中文CHM版最新版本
- RubyonRails字符串处理中文最新版本
- 基于 selenium 模拟微博登录爬虫资料齐全+详细文档+源码.zip
- 基于chromeDriver+selenium蓝桥杯题库爬虫资料齐全+详细文档+源码.zip
- 基于java+selenium爬虫资料齐全+详细文档+源码.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


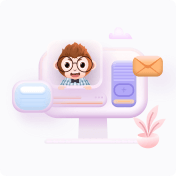
安全验证
文档复制为VIP权益,开通VIP直接复制
