/*
* fcgiapp.h --
*
* Definitions for FastCGI application server programs
*
*
* Copyright (c) 1996 Open Market, Inc.
*
* See the file "LICENSE.TERMS" for information on usage and redistribution
* of this file, and for a DISCLAIMER OF ALL WARRANTIES.
*
* $Id: fcgiapp.h,v 1.14 2003/06/22 00:16:44 robs Exp $
*/
#ifndef _FCGIAPP_H
#define _FCGIAPP_H
/* Hack to see if we are building Tcl - Tcl needs varargs not stdarg */
#ifndef TCL_LIBRARY
#include <stdarg.h>
#else
#include <varargs.h>
#endif
#ifndef DLLAPI
#if defined (_WIN32) && defined (_MSC_VER)
#ifdef FCGI_API
#define DLLAPI __declspec(dllexport)
#else
#define DLLAPI __declspec(dllimport)
#endif
#else
#define DLLAPI
#endif
#endif
#if defined (c_plusplus) || defined (__cplusplus)
extern "C" {
#endif
/*
* Error codes. Assigned to avoid conflict with EOF and errno(2).
*/
#define FCGX_UNSUPPORTED_VERSION -2
#define FCGX_PROTOCOL_ERROR -3
#define FCGX_PARAMS_ERROR -4
#define FCGX_CALL_SEQ_ERROR -5
/*
* This structure defines the state of a FastCGI stream.
* Streams are modeled after the FILE type defined in stdio.h.
* (We wouldn't need our own if platform vendors provided a
* standard way to subclass theirs.)
* The state of a stream is private and should only be accessed
* by the procedures defined below.
*/
typedef struct FCGX_Stream {
unsigned char *rdNext; /* reader: first valid byte
* writer: equals stop */
unsigned char *wrNext; /* writer: first free byte
* reader: equals stop */
unsigned char *stop; /* reader: last valid byte + 1
* writer: last free byte + 1 */
unsigned char *stopUnget; /* reader: first byte of current buffer
* fragment, for ungetc
* writer: undefined */
int isReader;
int isClosed;
int wasFCloseCalled;
int FCGI_errno; /* error status */
void (*fillBuffProc) (struct FCGX_Stream *stream);
void (*emptyBuffProc) (struct FCGX_Stream *stream, int doClose);
void *data;
} FCGX_Stream;
/*
* An environment (as defined by environ(7)): A NULL-terminated array
* of strings, each string having the form name=value.
*/
typedef char **FCGX_ParamArray;
/*
* FCGX_Request Flags
*
* Setting FCGI_FAIL_ACCEPT_ON_INTR prevents FCGX_Accept() from
* restarting upon being interrupted.
*/
#define FCGI_FAIL_ACCEPT_ON_INTR 1
/*
* FCGX_Request -- State associated with a request.
*
* Its exposed for API simplicity, I expect parts of it to change!
*/
typedef struct FCGX_Request {
int requestId; /* valid if isBeginProcessed */
int role;
FCGX_Stream *in;
FCGX_Stream *out;
FCGX_Stream *err;
char **envp;
/* Don't use anything below here */
struct Params *paramsPtr;
int ipcFd; /* < 0 means no connection */
int isBeginProcessed; /* FCGI_BEGIN_REQUEST seen */
int keepConnection; /* don't close ipcFd at end of request */
int appStatus;
int nWriters; /* number of open writers (0..2) */
int flags;
int listen_sock;
int detached;
} FCGX_Request;
/*
*======================================================================
* Control
*======================================================================
*/
/*
*----------------------------------------------------------------------
*
* FCGX_IsCGI --
*
* Returns TRUE iff this process appears to be a CGI process
* rather than a FastCGI process.
*
*----------------------------------------------------------------------
*/
DLLAPI int FCGX_IsCGI(void);
/*
*----------------------------------------------------------------------
*
* FCGX_Init --
*
* Initialize the FCGX library. Call in multi-threaded apps
* before calling FCGX_Accept_r().
*
* Returns 0 upon success.
*
*----------------------------------------------------------------------
*/
DLLAPI int FCGX_Init(void);
/*
*----------------------------------------------------------------------
*
* FCGX_OpenSocket --
*
* Create a FastCGI listen socket.
*
* path is the Unix domain socket (named pipe for WinNT), or a colon
* followed by a port number. e.g. "/tmp/fastcgi/mysocket", ":5000"
*
* backlog is the listen queue depth used in the listen() call.
*
* Returns the socket's file descriptor or -1 on error.
*
*----------------------------------------------------------------------
*/
DLLAPI int FCGX_OpenSocket(const char *path, int backlog);
/*
*----------------------------------------------------------------------
*
* FCGX_InitRequest --
*
* Initialize a FCGX_Request for use with FCGX_Accept_r().
*
* sock is a file descriptor returned by FCGX_OpenSocket() or 0 (default).
* The only supported flag at this time is FCGI_FAIL_ON_INTR.
*
* Returns 0 upon success.
*----------------------------------------------------------------------
*/
DLLAPI int FCGX_InitRequest(FCGX_Request *request, int sock, int flags);
/*
*----------------------------------------------------------------------
*
* FCGX_Accept_r --
*
* Accept a new request (multi-thread safe). Be sure to call
* FCGX_Init() first.
*
* Results:
* 0 for successful call, -1 for error.
*
* Side effects:
*
* Finishes the request accepted by (and frees any
* storage allocated by) the previous call to FCGX_Accept.
* Creates input, output, and error streams and
* assigns them to *in, *out, and *err respectively.
* Creates a parameters data structure to be accessed
* via getenv(3) (if assigned to environ) or by FCGX_GetParam
* and assigns it to *envp.
*
* DO NOT retain pointers to the envp array or any strings
* contained in it (e.g. to the result of calling FCGX_GetParam),
* since these will be freed by the next call to FCGX_Finish
* or FCGX_Accept.
*
* DON'T use the FCGX_Request, its structure WILL change.
*
*----------------------------------------------------------------------
*/
DLLAPI int FCGX_Accept_r(FCGX_Request *request);
/*
*----------------------------------------------------------------------
*
* FCGX_Finish_r --
*
* Finish the request (multi-thread safe).
*
* Side effects:
*
* Finishes the request accepted by (and frees any
* storage allocated by) the previous call to FCGX_Accept.
*
* DO NOT retain pointers to the envp array or any strings
* contained in it (e.g. to the result of calling FCGX_GetParam),
* since these will be freed by the next call to FCGX_Finish
* or FCGX_Accept.
*
*----------------------------------------------------------------------
*/
DLLAPI void FCGX_Finish_r(FCGX_Request *request);
/*
*----------------------------------------------------------------------
*
* FCGX_Free --
*
* Free the memory and, if close is true,
* IPC FD associated with the request (multi-thread safe).
*
*----------------------------------------------------------------------
*/
DLLAPI void FCGX_Free(FCGX_Request * request, int close);
/*
*----------------------------------------------------------------------
*
* FCGX_Accept --
*
* Accept a new request (NOT multi-thread safe).
*
* Results:
* 0 for successful call, -1 for error.
*
* Side effects:
*
* Finishes the request accepted by (and frees any
* storage allocated by) the previous call to FCGX_Accept.
* Creates input, output, and error streams and
* assigns them to *in, *out, and *err respectively.
* Creates a parameters data structure to be accessed
* via getenv(3) (if assigned to environ) or by FCGX_GetParam
* and assigns it to *envp.
*
* DO NOT retain pointers to the envp array or any strings
* contained in it (e.g. to the result of calling FCGX_GetParam),
* since these will be freed by the next call to FCGX_Finish
* or FCGX_Accep
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
一、内容概况 QGIS是一个开源的、跨平台的地理信息系统(GIS)软件,用于浏览、编辑和分析地理空间数据,提供了一套丰富的功能,包括地图制作、空间分析、数据管理等。QGIS可以在Windows、Mac OS和Linux等操作系统上运行。 QGIS的跨平台编译需要一系列开源库的支持,本系列提供QGIS相关的编译成果。 本资源的内容为:基于Qt的FastCGI跨平台编译成果(MacOS版本)。 二、使用人群 QGIS编译、QGIS跨平台编译的人员或研究者。 三、使用场景及目标 在MacOS环境下使用。 既可以支撑QGIS在MacOS环境下的编译工作,也可以进行FastCGI的二次研发。 四、其他说明 在MacOS环境下,基于Qt Creator进行编译的FastCGI开源库。包含有头文件include、库文件dylib等,提供了Debug、Release版本。 当前采用的版本为FastCGI2-2.4.2,如果下载者,需要其他版本的FastCGI,请在评论区留言。
资源推荐
资源详情
资源评论
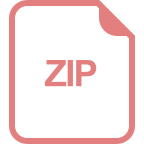
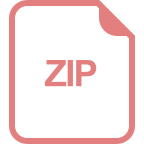
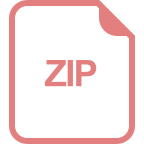
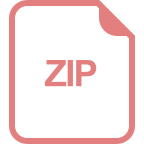
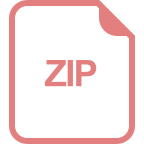
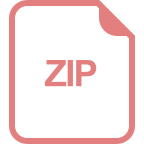
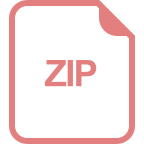
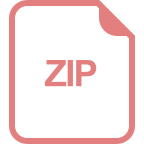
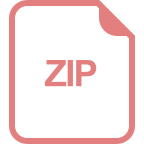
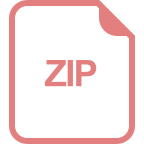
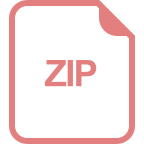
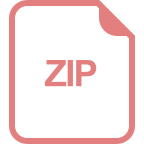
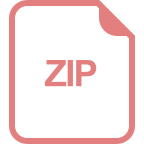
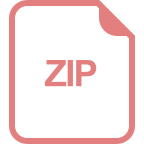
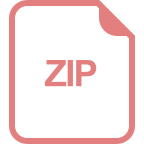
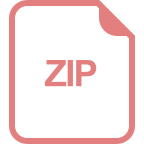
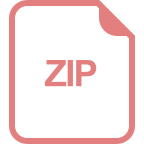
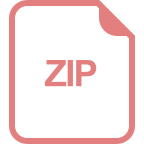
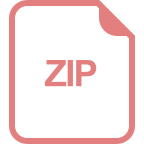
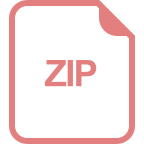
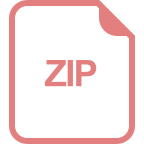
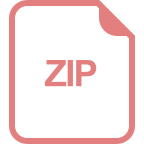
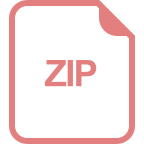
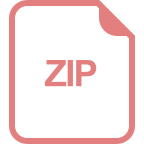
收起资源包目录



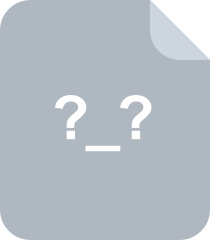
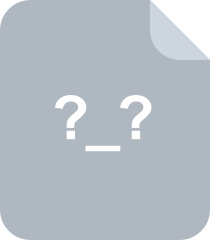
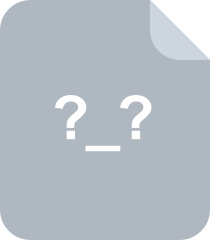
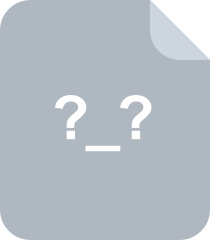
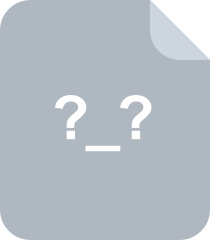
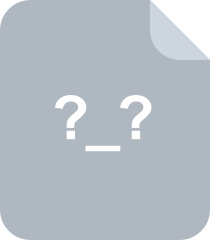
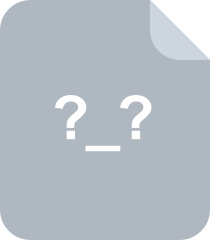
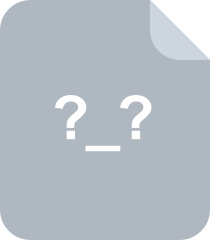

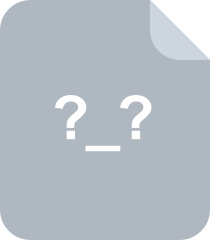
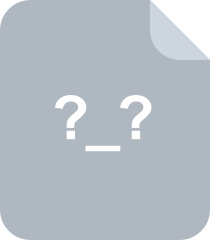

共 10 条
- 1
资源评论
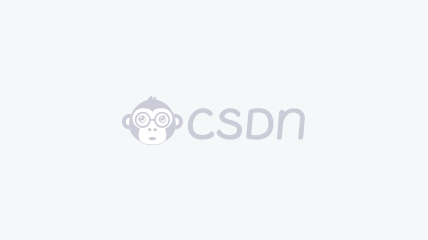

翰墨之道
- 粉丝: 3586
- 资源: 182
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

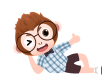
最新资源
- Hybrid开发,基于h5+ API和mui前端框架,以及seajs模块化开发的一套跨平台APP开发框架.zip
- 计算机组成原理(COD)综合实验,带三级浮点流水的五级RISCV流水线.zip
- sm2解密出Invalid point encoding问题的解决办法
- 乐跑刷数据代码 (1).exe
- 计算机科学与工程学院15级大三短学期JAVA课设-虚拟校园系统.zip
- 备战2025电赛03-驱动1.8寸TFT-LCD屏幕
- 一个基于Java SE的跳跃忍者游戏.zip
- 大数据产业园多类型楼宇群电能共享优化运行策略
- 一个采用MVC架构设计、Java实现的泡泡堂游戏.zip
- 一个基于java socket的可以网络对战的俄罗斯方块游戏.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


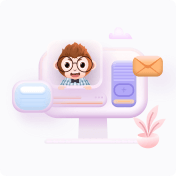
安全验证
文档复制为VIP权益,开通VIP直接复制
