<div align="center">
<img height="170"
src="https://worldvectorlogo.com/logos/sass-1.svg">
<a href="https://github.com/webpack/webpack">
<img width="200" height="200"
src="https://webpack.js.org/assets/icon-square-big.svg">
</a>
</div>
[![npm][npm]][npm-url]
[![node][node]][node-url]
[![tests][tests]][tests-url]
[![coverage][cover]][cover-url]
[![chat][chat]][chat-url]
[![size][size]][size-url]
# sass-loader
Loads a Sass/SCSS file and compiles it to CSS.
## Getting Started
To begin, you'll need to install `sass-loader`:
```console
npm install sass-loader sass webpack --save-dev
```
or
```console
yarn add -D sass-loader sass webpack
```
or
```console
pnpm add -D sass-loader sass webpack
```
`sass-loader` requires you to install either [Dart Sass](https://github.com/sass/dart-sass), [Node Sass](https://github.com/sass/node-sass) on your own (more documentation can be found below) or [Sass Embedded](https://github.com/sass/embedded-host-node).
This allows you to control the versions of all your dependencies, and to choose which Sass implementation to use.
> **Note**
>
> We highly recommend using [Dart Sass](https://github.com/sass/dart-sass).
> **Warning**
>
> [Node Sass](https://github.com/sass/node-sass) does not work with [Yarn PnP](https://classic.yarnpkg.com/en/docs/pnp/) feature and doesn't support [@use rule](https://sass-lang.com/documentation/at-rules/use).
> **Warning**
>
> [Sass Embedded](https://github.com/sass/embedded-host-node) is experimental and in `beta`, therefore some features may not work
Chain the `sass-loader` with the [css-loader](https://github.com/webpack-contrib/css-loader) and the [style-loader](https://github.com/webpack-contrib/style-loader) to immediately apply all styles to the DOM or the [mini-css-extract-plugin](https://github.com/webpack-contrib/mini-css-extract-plugin) to extract it into a separate file.
Then add the loader to your Webpack configuration. For example:
**app.js**
```js
import "./style.scss";
```
**style.scss**
```scss
$body-color: red;
body {
color: $body-color;
}
```
**webpack.config.js**
```js
module.exports = {
module: {
rules: [
{
test: /\.s[ac]ss$/i,
use: [
// Creates `style` nodes from JS strings
"style-loader",
// Translates CSS into CommonJS
"css-loader",
// Compiles Sass to CSS
"sass-loader",
],
},
],
},
};
```
Finally run `webpack` via your preferred method.
### Resolving `import` at-rules
Webpack provides an [advanced mechanism to resolve files](https://webpack.js.org/concepts/module-resolution/).
The `sass-loader` uses Sass's custom importer feature to pass all queries to the Webpack resolving engine.
Thus you can import your Sass modules from `node_modules`.
```scss
@import "bootstrap";
```
Using `~` is deprecated and can be removed from your code (**we recommend it**), but we still support it for historical reasons.
Why can you remove it? The loader will first try to resolve `@import` as a relative path. If it cannot be resolved, then the loader will try to resolve `@import` inside [`node_modules`](https://webpack.js.org/configuration/resolve/#resolvemodules).
Prepending module paths with a `~` tells webpack to search through [`node_modules`](https://webpack.js.org/configuration/resolve/#resolvemodules).
```scss
@import "~bootstrap";
```
It's important to prepend it with only `~`, because `~/` resolves to the home directory.
Webpack needs to distinguish between `bootstrap` and `~bootstrap` because CSS and Sass files have no special syntax for importing relative files.
Writing `@import "style.scss"` is the same as `@import "./style.scss";`
### Problems with `url(...)`
Since Sass implementations don't provide [url rewriting](https://github.com/sass/libsass/issues/532), all linked assets must be relative to the output.
- If you pass the generated CSS on to the `css-loader`, all urls must be relative to the entry-file (e.g. `main.scss`).
- If you're just generating CSS without passing it to the `css-loader`, it must be relative to your web root.
You will be disrupted by this first issue. It is natural to expect relative references to be resolved against the `.sass`/`.scss` file in which they are specified (like in regular `.css` files).
Thankfully there are a two solutions to this problem:
- Add the missing url rewriting using the [resolve-url-loader](https://github.com/bholloway/resolve-url-loader). Place it before `sass-loader` in the loader chain.
- Library authors usually provide a variable to modify the asset path. [bootstrap-sass](https://github.com/twbs/bootstrap-sass) for example has an `$icon-font-path`.
## Options
- **[`implementation`](#implementation)**
- **[`sassOptions`](#sassoptions)**
- **[`sourceMap`](#sourcemap)**
- **[`additionalData`](#additionaldata)**
- **[`webpackImporter`](#webpackimporter)**
- **[`warnRuleAsWarning`](#warnruleaswarning)**
### `implementation`
Type:
```ts
type implementation = object | string;
```
Default: `sass`
The special `implementation` option determines which implementation of Sass to use.
By default the loader resolve the implementation based on your dependencies.
Just add required implementation to `package.json` (`sass` or `node-sass` package) and install dependencies.
Example where the `sass-loader` loader uses the `sass` (`dart-sass`) implementation:
**package.json**
```json
{
"devDependencies": {
"sass-loader": "^7.2.0",
"sass": "^1.22.10"
}
}
```
Example where the `sass-loader` loader uses the `node-sass` implementation:
**package.json**
```json
{
"devDependencies": {
"sass-loader": "^7.2.0",
"node-sass": "^5.0.0"
}
}
```
Beware the situation when `node-sass` and `sass` were installed! By default the `sass-loader` prefers `sass`.
In order to avoid this situation you can use the `implementation` option.
The `implementation` options either accepts `sass` (`Dart Sass`) or `node-sass` as a module.
#### `object`
For example, to use Dart Sass, you'd pass:
```js
module.exports = {
module: {
rules: [
{
test: /\.s[ac]ss$/i,
use: [
"style-loader",
"css-loader",
{
loader: "sass-loader",
options: {
// Prefer `dart-sass`
implementation: require("sass"),
},
},
],
},
],
},
};
```
#### `string`
For example, to use Dart Sass, you'd pass:
```js
module.exports = {
module: {
rules: [
{
test: /\.s[ac]ss$/i,
use: [
"style-loader",
"css-loader",
{
loader: "sass-loader",
options: {
// Prefer `dart-sass`
implementation: require.resolve("sass"),
},
},
],
},
],
},
};
```
Note that when using `sass` (`Dart Sass`), **synchronous compilation is twice as fast as asynchronous compilation** by default, due to the overhead of asynchronous callbacks.
To avoid this overhead, you can use the [fibers](https://www.npmjs.com/package/fibers) package to call asynchronous importers from the synchronous code path.
We automatically inject the [`fibers`](https://github.com/laverdet/node-fibers) package (setup `sassOptions.fiber`) for `Node.js` less v16.0.0 if is possible (i.e. you need install the [`fibers`](https://github.com/laverdet/node-fibers) package).
> **Warning**
>
> Fibers is not compatible with `Node.js` v16.0.0 or later. Unfortunately, v8 commit [dacc2fee0f](https://github.com/v8/v8/commit/dacc2fee0f815823782a7e432c79c2a7767a4765) is a breaking change and workarounds are non-trivial. ([see introduction to readme](https://github.com/laverdet/node-fibers)).
**package.json**
```json
{
"devDependencies": {
"sass-loader": "^7.2.0",
"sass": "^1.22.10",
"fibers": "^4.0.1"
}
}
```
You can disable automatically injecting the [`fibers`](https://github.
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
chatgpt免费使用 ChatGPT 镜像站:免注册就能使用,只不过背后的 GPT 模型大多为 3.0,不如原版的 3.5 那般「聪明」,体验感不如想象的智能,但是毕竟直接。 Notion:Notion作为当下最流行的笔记工具,在接入 ChatGPT 的基础上推出了 Notion AI 的功能,可以在整理笔记的时候,通过ChatGPT般的AI能力提供人工智能的思路,让写作更流畅。 boardmix博思白板:国内白板工具中的佼佼者,日常很多学生,培训讲师,产研人员用它做富媒体思维整理, 贴图,展示文档,画画思维导图,看板图等等,其上线的 AI助手功能,可回答问题、翻译英文、头脑风暴、创意协作、制作旅游攻略等,几乎无所不能,其AI助手回答问题的能力也非常智能。 下面就以 boardmix博思白板为例,简要介绍使用内置的 AI助手的方法: 直接打开 boardmix博思白板,创建一个白板文件,点击左侧菜单栏的「AI助手」按钮,启用博思白板接入的 AI助手,在打开的输入框中输入你的问题,AI 助手就会像 ChatGPT 那样,给出相应的回答。
资源推荐
资源详情
资源评论
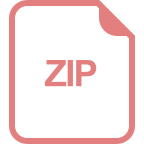
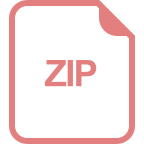
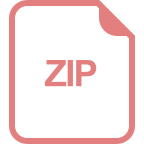
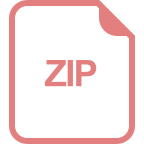
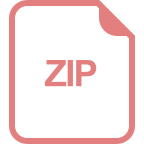
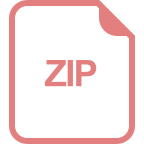
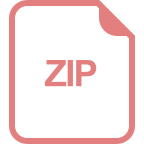
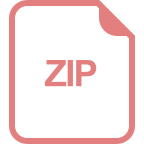
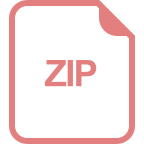
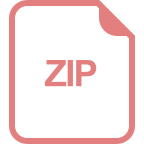
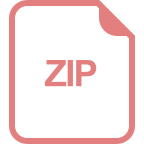
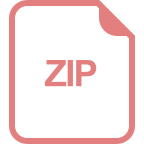
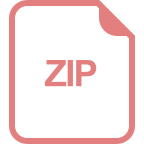
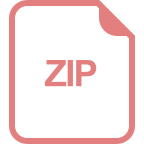
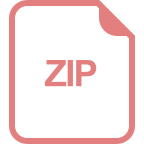
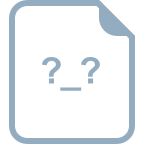
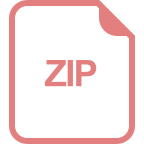
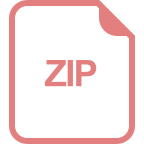
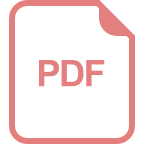
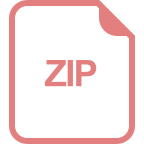
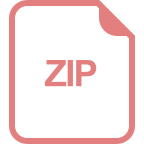
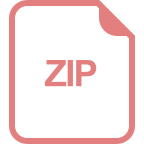
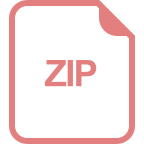
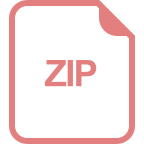
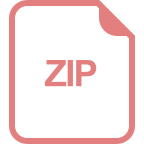
收起资源包目录

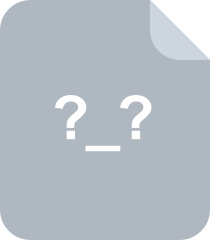
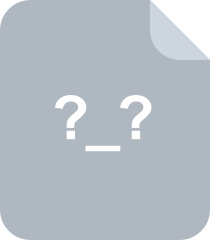
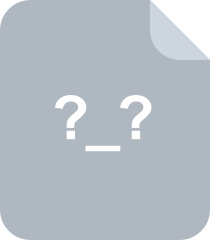
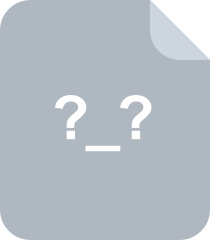
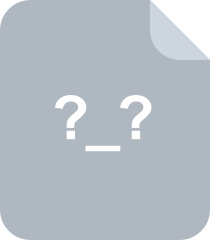
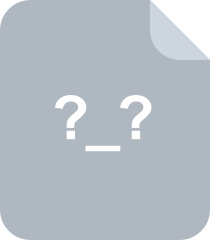
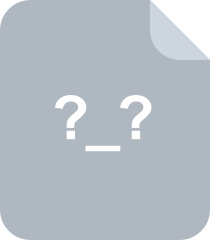
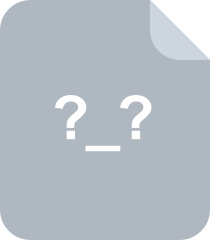
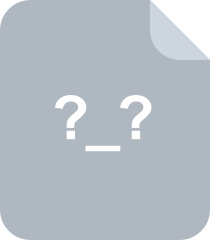
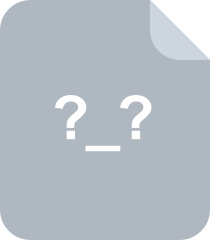
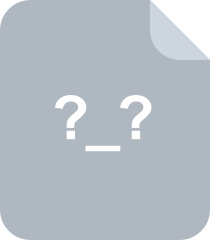
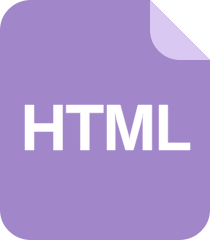
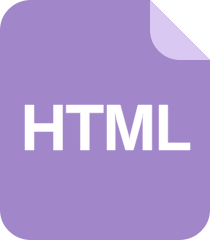
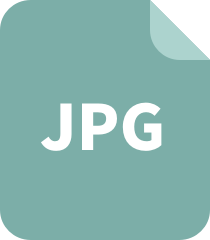
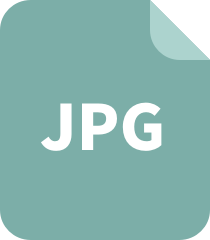
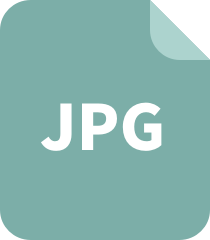
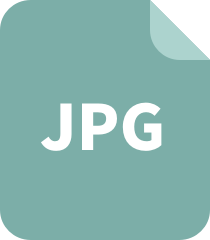
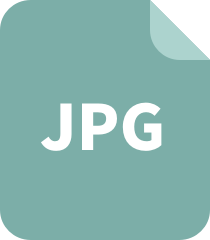
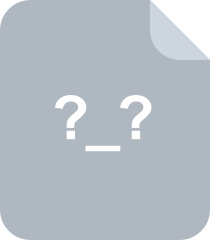
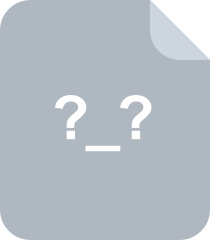
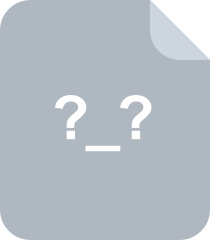
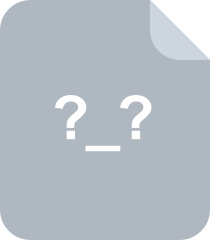
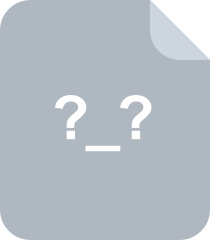
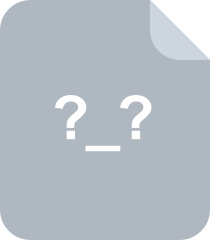
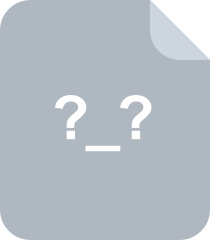
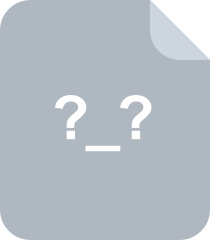
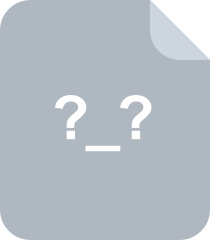
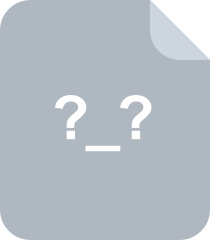
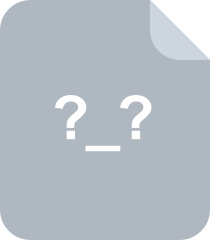
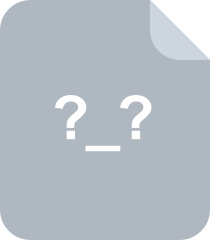
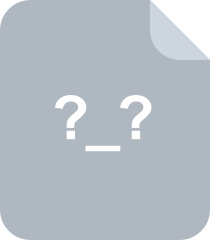
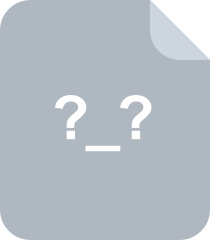
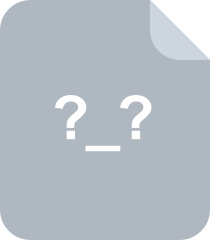
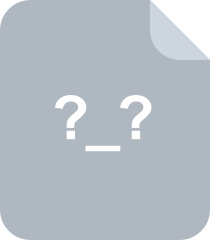
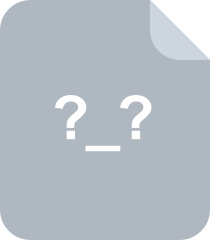
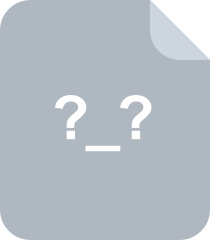
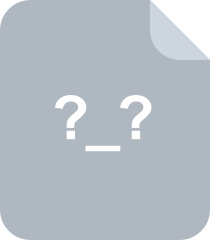
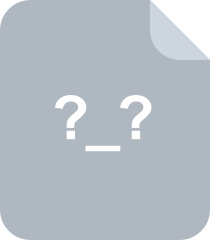
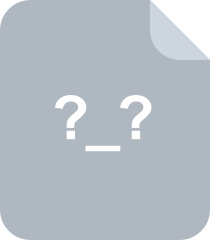
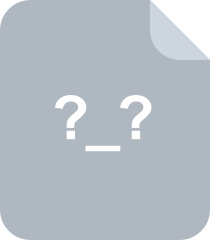
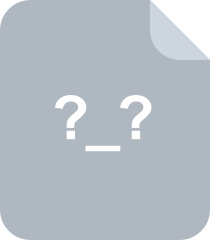
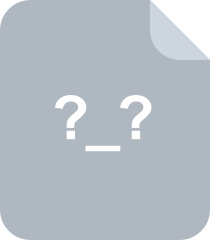
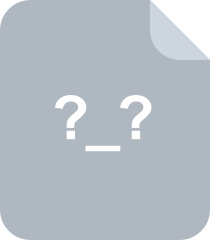
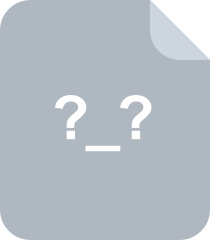
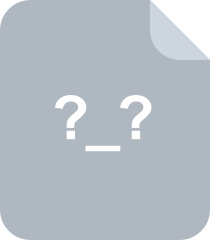
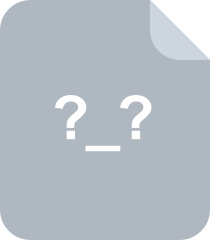
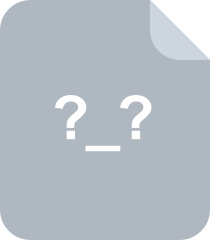
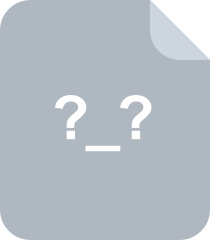
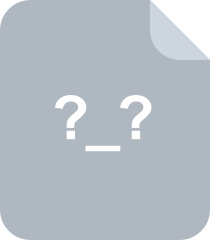
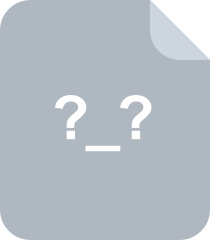
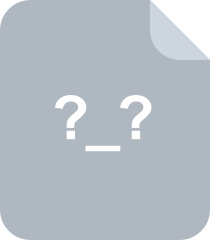
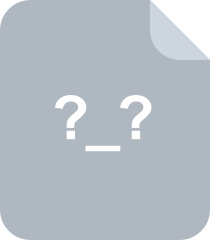
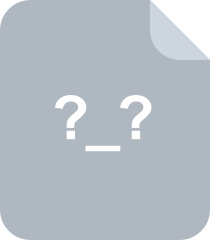
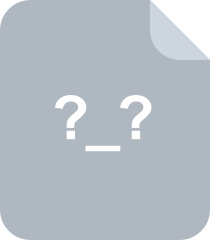
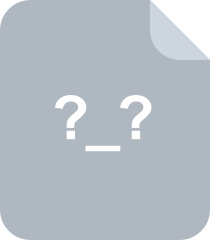
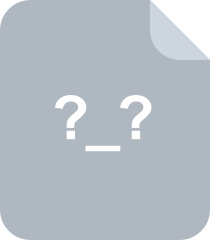
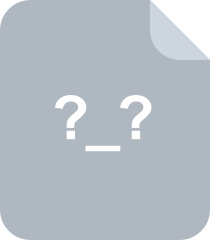
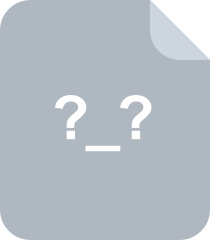
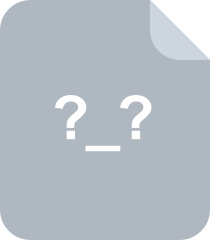
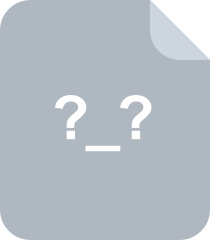
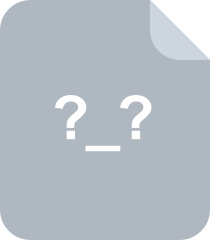
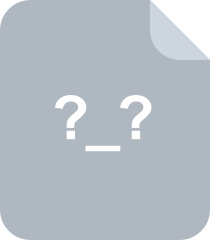
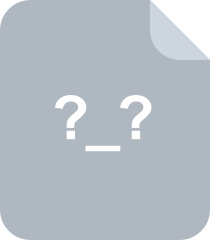
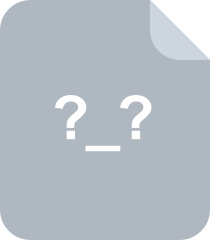
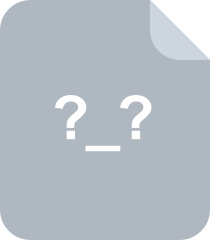
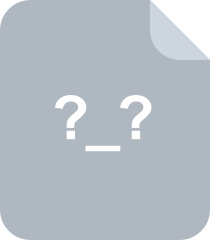
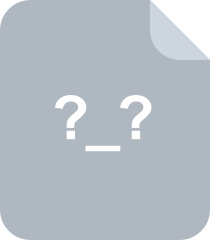
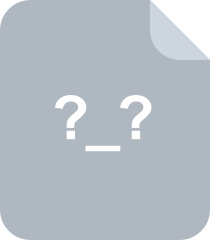
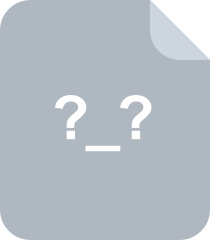
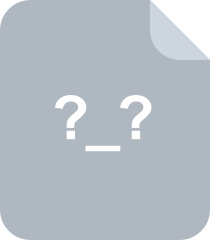
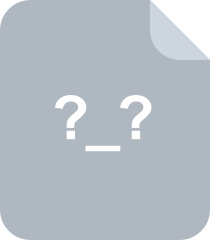
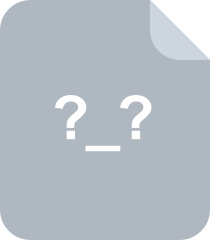
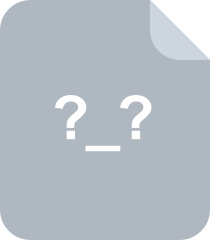
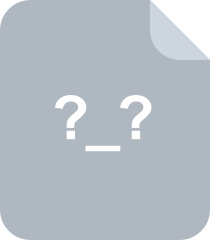
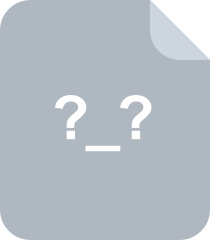
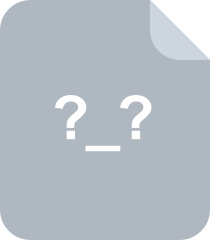
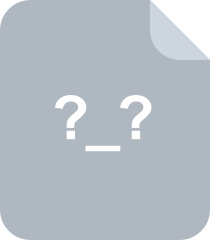
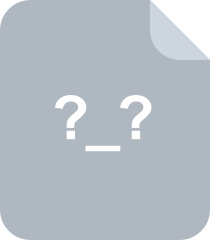
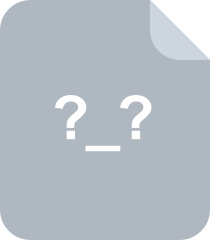
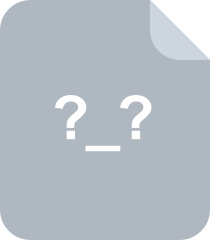
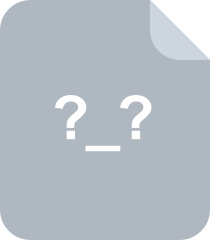
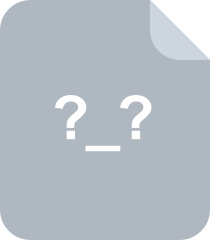
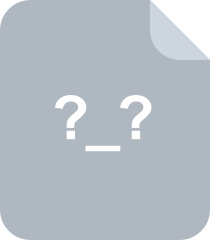
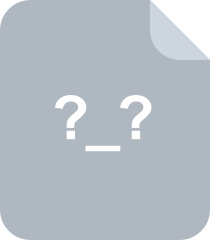
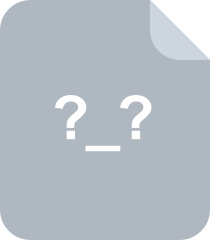
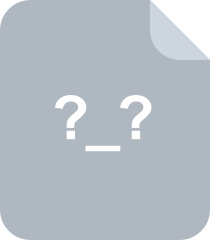
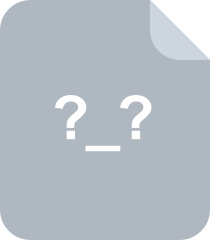
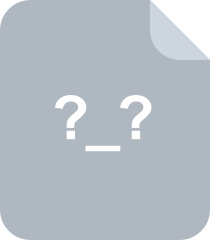
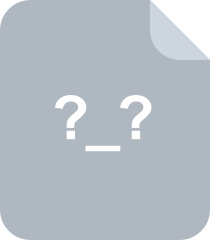
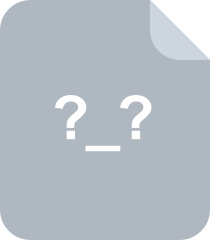
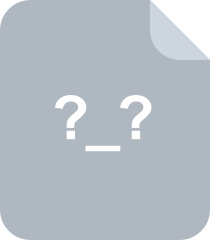
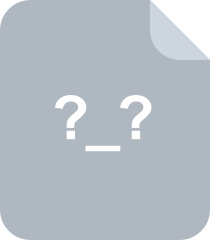
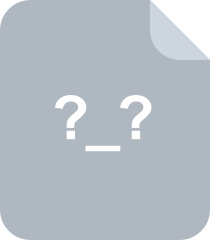
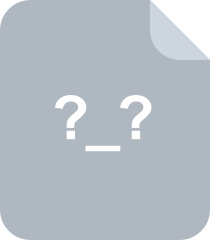
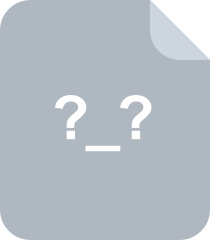
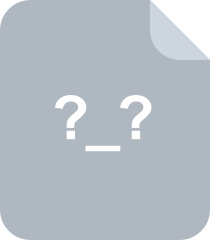
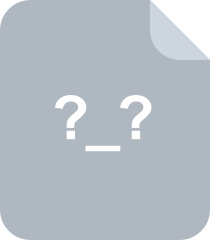
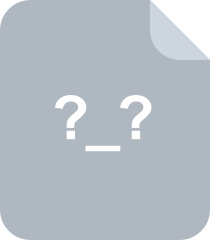
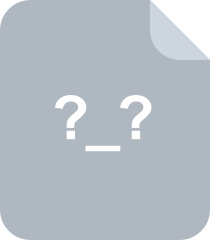
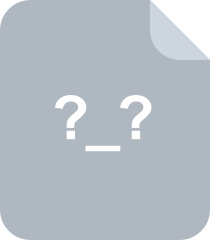
共 1644 条
- 1
- 2
- 3
- 4
- 5
- 6
- 17
资源评论
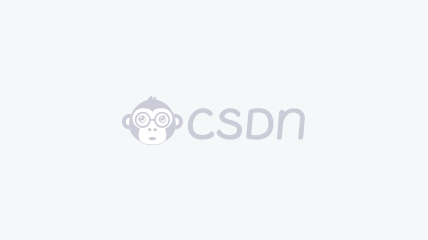
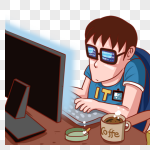
野生的狒狒
- 粉丝: 3393
- 资源: 2436

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

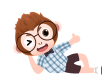
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


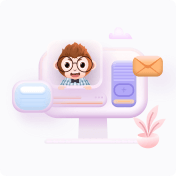
安全验证
文档复制为VIP权益,开通VIP直接复制
