package com.zhf.common.util;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Locale;
import org.apache.commons.lang.StringUtils;
import org.apache.commons.lang.math.NumberUtils;
import java.text.DateFormat;
import java.text.ParsePosition;
import java.util.Calendar;
import java.util.GregorianCalendar;
import static org.apache.commons.lang3.time.DateUtils.isSameDay;
public class DateUtil {
private DateUtil(){
}
private static String getDateFormat(Date date, String dateFormatType) {
SimpleDateFormat simformat = new SimpleDateFormat(dateFormatType);
return simformat.format(date);
}
public static String formatCSTTime(String date, String format) throws ParseException {
SimpleDateFormat sdf = new SimpleDateFormat("EEE MMM dd HH:mm:ss zzz yyyy", Locale.US);
Date d = sdf.parse(date);
return DateUtil.getDateFormat(d, format);
}
public final static long ONE_DAY_SECONDS = 86400;
/*
* private static DateFormat dateFormat = null; private static DateFormat
* longDateFormat = null; private static DateFormat dateWebFormat = null;
*/
public final static String shortFormat = "yyyyMMdd";
public final static String longFormat = "yyyyMMddHHmmss";
public final static String webFormat = "yyyy-MM-dd";
public final static String timeFormat = "HHmmss";
public final static String monthFormat = "yyyyMM";
public final static String chineseDtFormat = "yyyy年MM月dd日";
public final static String newFormat = "yyyy-MM-dd HH:mm:ss";
public final static String newFormat2 = "yyyy-MM-dd HH:mm";
public final static String newFormat3 = "yyyy-MM-dd HH";
public final static String FULL_DATE_FORMAT = "yyyy-MM-dd HH:mm:ss.SSS";
static {
/*
* dateFormat = new SimpleDateFormat(shortFormat);
* dateFormat.setLenient(false); longDateFormat = new
* SimpleDateFormat(longFormat); longDateFormat.setLenient(false);
* dateWebFormat = new SimpleDateFormat(webFormat);
* dateWebFormat.setLenient(false);
*/
}
public static long ONE_DAY_MILL_SECONDS = 86400000;
public static Date getNowDate() {
return new Date();
}
public static Date getDate(long millsecord) {
return new Date(millsecord);
}
public static DateFormat getNewDateFormat(String pattern) {
DateFormat df = new SimpleDateFormat(pattern);
df.setLenient(false);
return df;
}
public static void main(String[] args) {
Date n = new Date();
Date mm = formatDate(n, "yyyy-MM-dd");
System.out.println(mm.toString());
}
//日期格式转换,返回日期类型
public static Date formatDate(Date date, String format) {
SimpleDateFormat formatter = new SimpleDateFormat(format);
String dateString = formatter.format(date);
ParsePosition pos = new ParsePosition(0);
Date newDate = formatter.parse(dateString, pos);
return newDate;
}
//日期格式转换,返回String
public static String format(Date date, String format) {
if (date == null) {
return null;
}
return new SimpleDateFormat(format).format(date);
}
public static String formatByLong(long date, String format) {
return new SimpleDateFormat(format).format(new Date(date));
}
public static String formatByString(String date, String format) {
if (StringUtils.isNotBlank(date)) {
return new SimpleDateFormat(format).format(new Date(NumberUtils
.toLong(date)));
}
return StringUtils.EMPTY;
}
public static String formatShortFormat(Date date) {
if (date == null) {
return null;
}
return new SimpleDateFormat(shortFormat).format(date);
}
public static Date parseDateNoTime(String sDate) throws ParseException {
DateFormat dateFormat = new SimpleDateFormat(shortFormat);
if ((sDate == null) || (sDate.length() < shortFormat.length())) {
throw new ParseException("length too little", 0);
}
if (!StringUtils.isNumeric(sDate)) {
throw new ParseException("not all digit", 0);
}
return dateFormat.parse(sDate);
}
public static Date parseDateNoTime(String sDate, String format)
throws ParseException {
if (StringUtils.isBlank(format)) {
throw new ParseException("Null format. ", 0);
}
DateFormat dateFormat = new SimpleDateFormat(format);
if ((sDate == null) || (sDate.length() < format.length())) {
throw new ParseException("length too little", 0);
}
return dateFormat.parse(sDate);
}
public static Date parseDateNoTimeWithDelimit(String sDate, String delimit)
throws ParseException {
sDate = sDate.replaceAll(delimit, "");
DateFormat dateFormat = new SimpleDateFormat(shortFormat);
if ((sDate == null) || (sDate.length() != shortFormat.length())) {
throw new ParseException("length not match", 0);
}
return dateFormat.parse(sDate);
}
public static Date parseDateLongFormat(String sDate) {
DateFormat dateFormat = new SimpleDateFormat(longFormat);
Date d = null;
if ((sDate != null) && (sDate.length() == longFormat.length())) {
try {
d = dateFormat.parse(sDate);
} catch (ParseException ex) {
return null;
}
}
return d;
}
public static Date parseDateNewFormat(String sDate) {
Date d = parseDateHelp(sDate, newFormat);
if (null != d) {
return d;
}
d = parseDateHelp(sDate, newFormat2);
if (null != d) {
return d;
}
d = parseDateHelp(sDate, newFormat3);
if (null != d) {
return d;
}
d = parseDateHelp(sDate, webFormat);
if (null != d) {
return d;
}
try {
DateFormat dateFormat = new SimpleDateFormat(newFormat);
return dateFormat.parse(sDate);
} catch (ParseException ex) {
return null;
}
}
private static Date parseDateHelp(String sDate, String format) {
if ((sDate != null) && (sDate.length() == format.length())) {
try {
DateFormat dateFormat = new SimpleDateFormat(format);
return dateFormat.parse(sDate);
} catch (ParseException ex) {
return null;
}
}
return null;
}
/**
* 计算当前时间几小时之后的时间
*
* @param date
* @param hours
* @return
*/
public static Date addHours(Date date, long hours) {
return addMinutes(date, hours * 60);
}
/**
* 计算当前时间几分钟之后的时间
*
* @param date
* @param minutes
* @return
*/
public static Date addMinutes(Date date, long minutes) {
return addSeconds(date, minutes * 60);
}
/**
* @param date1
* @param secs
* @return
*/
public static Date addSeconds(Date date1, long secs) {
return new Date(date1.getTime() + (secs * 1000));
}
/**
* 判断输入的字符串是否为合法的小时
*
* @param hourStr
* @return true/false
*/
public static boolean isValidHour(String hourStr) {
if (!StringUtils.isEmpty(hourStr) && StringUtils.isNumeric(hourStr)) {
int hour = new Integ
没有合适的资源?快使用搜索试试~ 我知道了~
毕设-基于Python的股票自动交易系统的设计与实现源码.zip
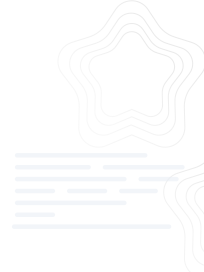
共687个文件
jpg:169个
png:132个
java:131个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 175 浏览量
2024-05-16
12:34:35
上传
评论
收藏 14.71MB ZIP 举报
温馨提示
毕设-基于Python的股票自动交易系统的设计与实现源码.zip毕设-基于Python的股票自动交易系统的设计与实现源码.zip毕设-基于Python的股票自动交易系统的设计与实现源码.zip毕设-基于Python的股票自动交易系统的设计与实现源码.zip毕设-基于Python的股票自动交易系统的设计与实现源码.zip毕设-基于Python的股票自动交易系统的设计与实现源码.zip毕设-基于Python的股票自动交易系统的设计与实现源码.zip毕设-基于Python的股票自动交易系统的设计与实现源码.zip毕设-基于Python的股票自动交易系统的设计与实现源码.zip
资源推荐
资源详情
资源评论
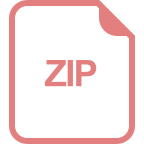
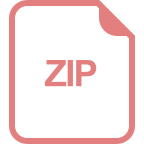
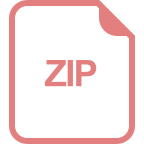
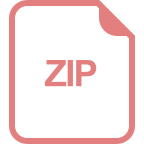
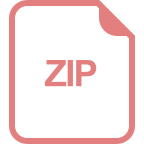
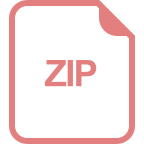
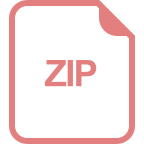
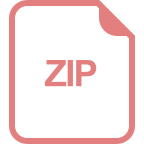
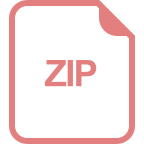
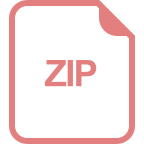
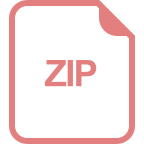
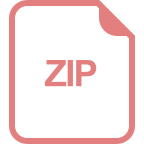
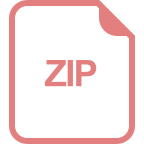
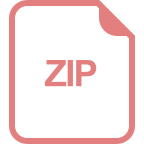
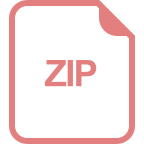
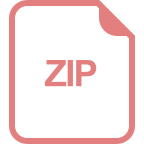
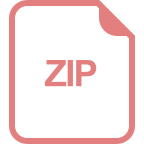
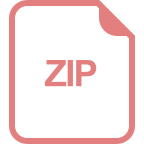
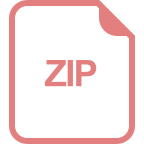
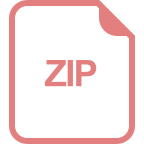
收起资源包目录

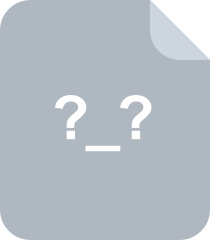
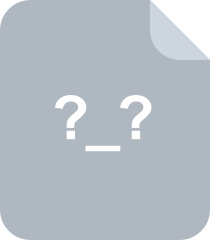
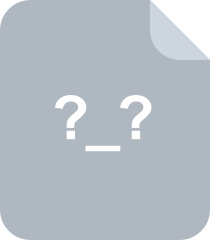
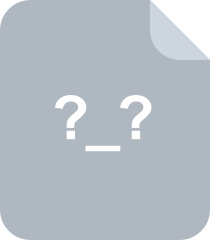
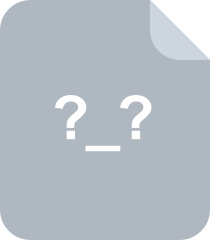
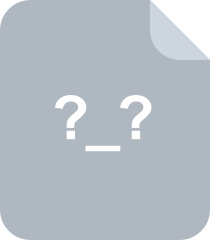
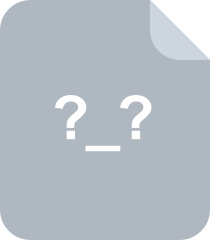
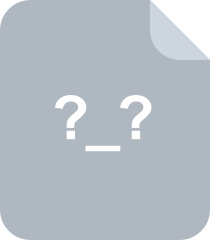
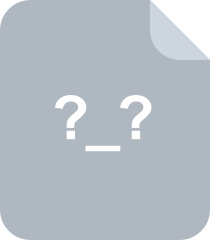
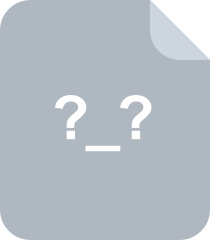
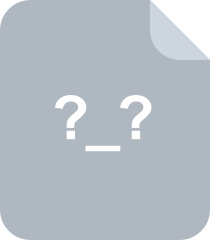
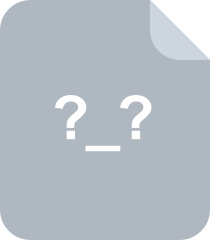
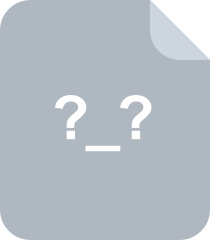
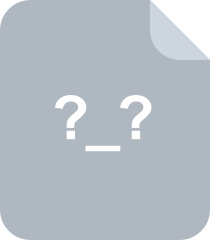
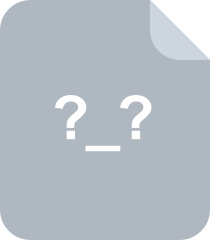
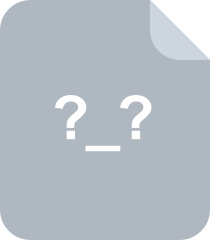
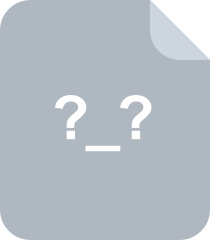
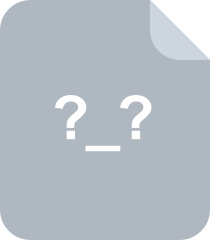
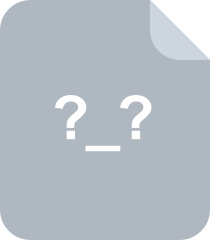
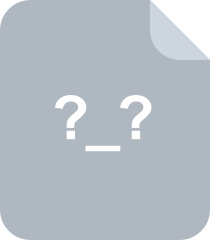
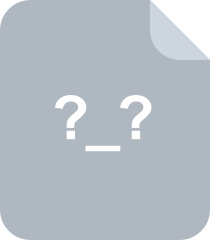
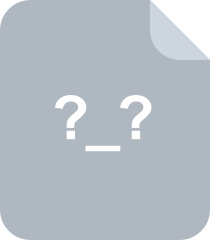
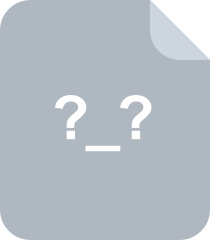
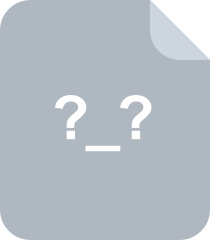
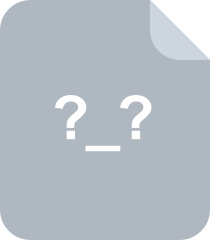
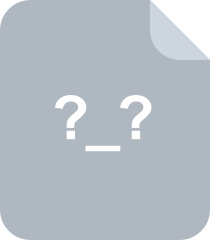
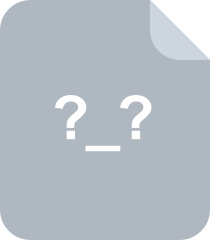
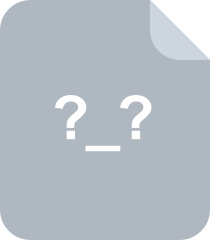
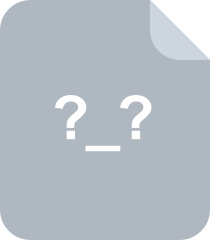
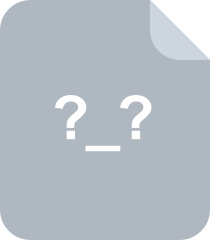
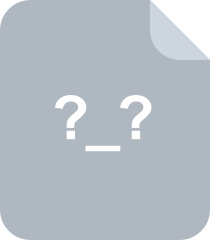
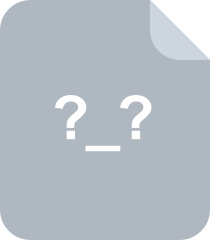
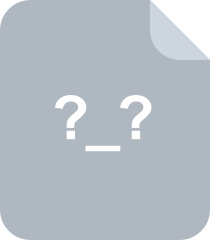
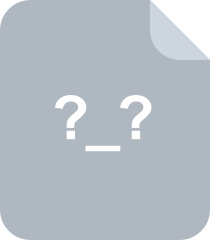
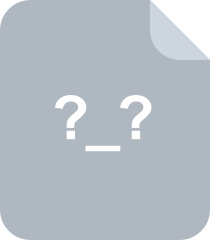
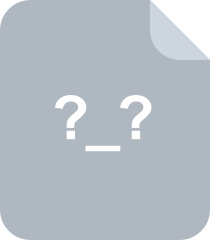
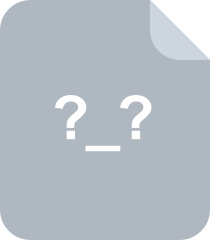
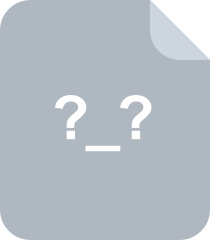
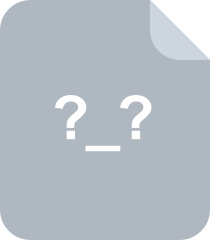
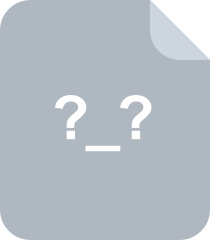
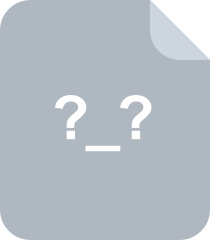
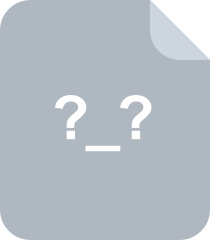
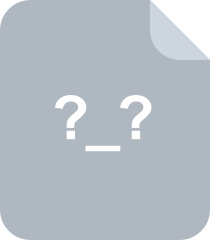
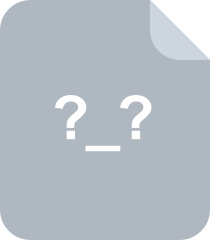
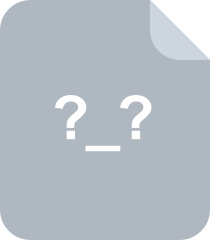
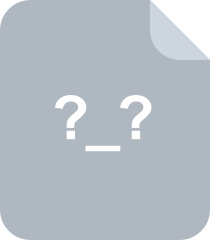
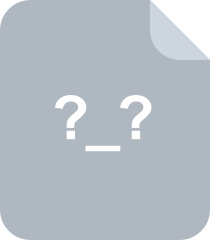
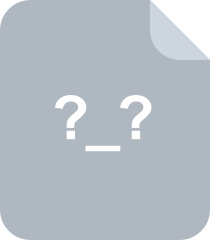
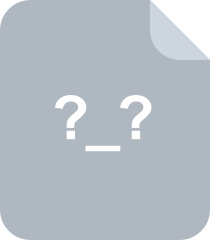
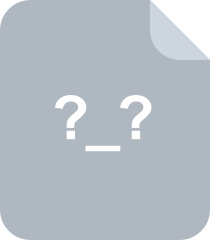
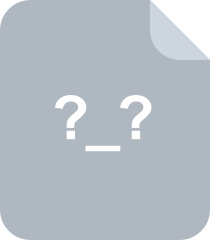
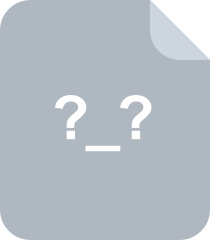
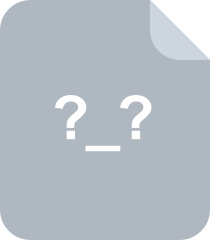
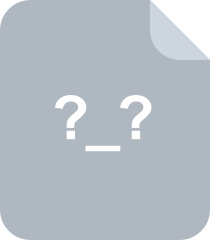
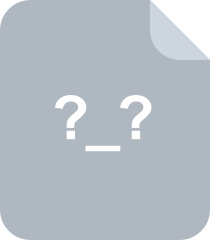
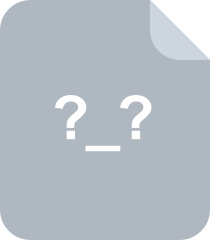
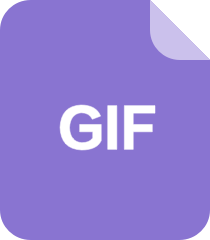
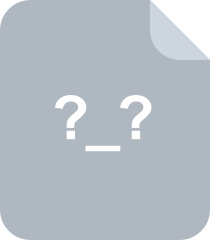
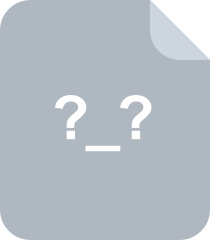
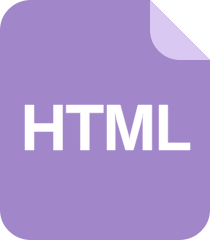
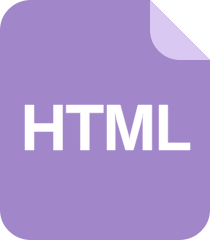
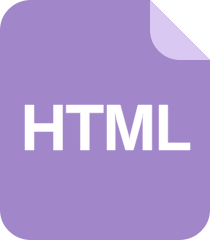
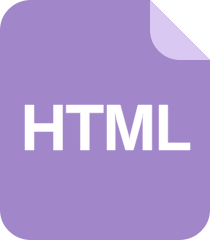
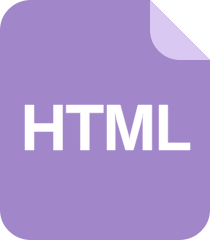
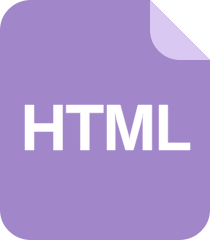
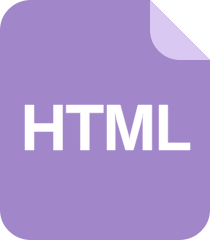
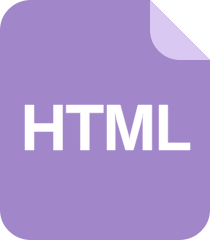
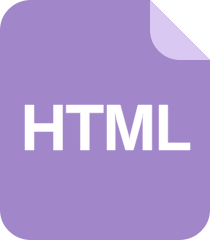
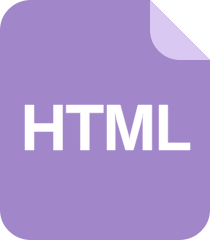
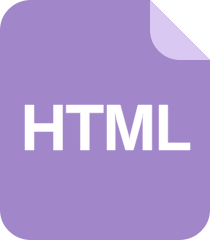
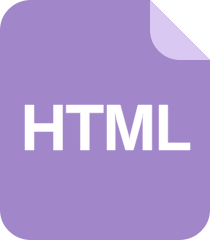
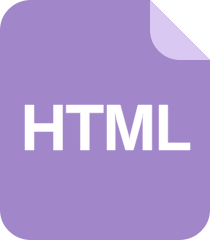
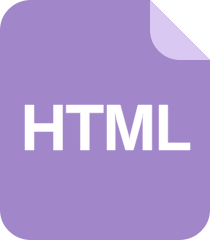
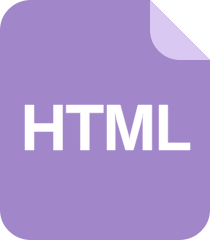
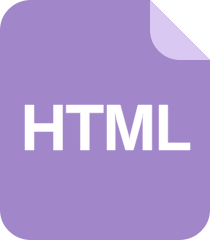
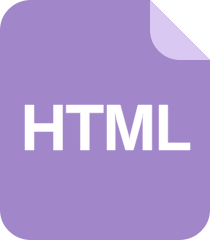
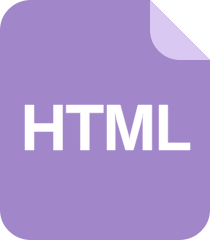
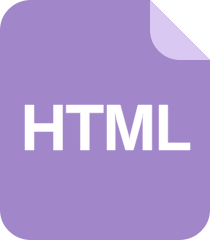
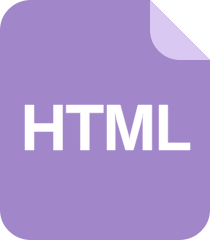
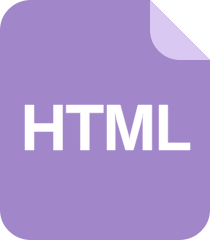
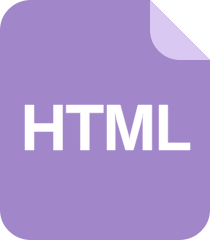
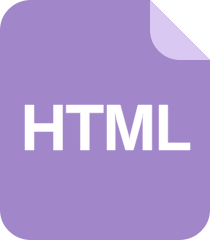
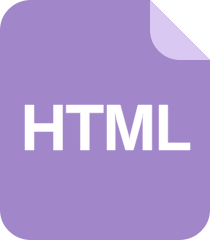
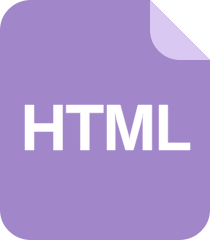
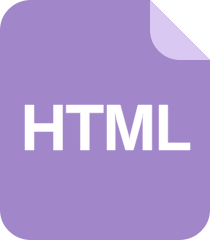
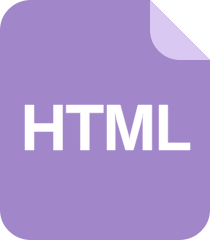
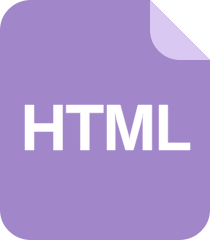
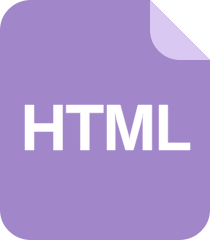
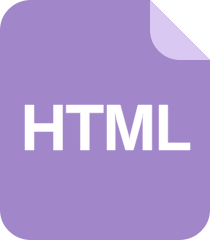
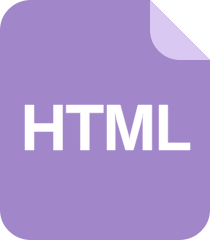
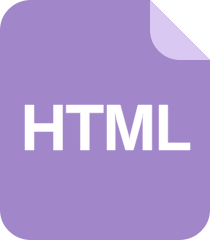
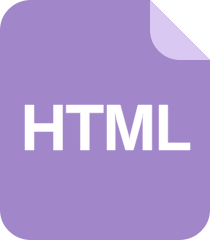
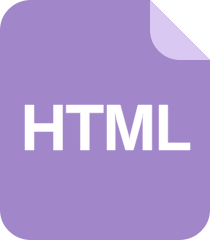
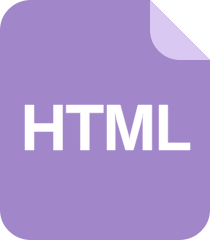
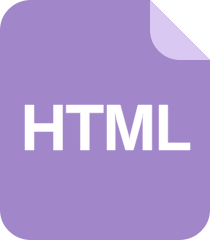
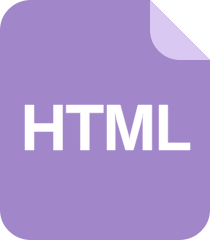
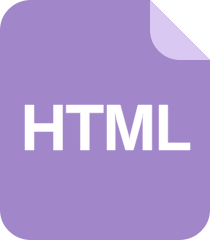
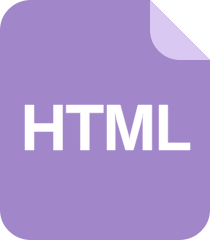
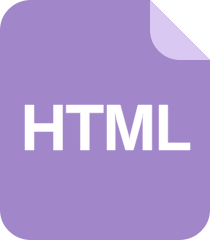
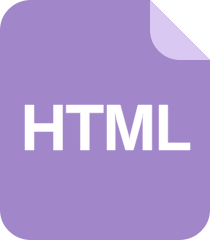
共 687 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源评论
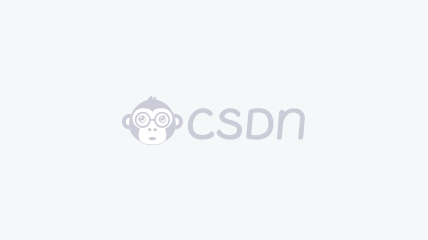

生活家小毛.
- 粉丝: 6036
- 资源: 7290
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

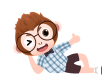
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


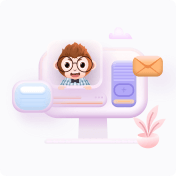
安全验证
文档复制为VIP权益,开通VIP直接复制
