# mquery
`mquery` is a fluent mongodb query builder designed to run in multiple environments.
[](https://travis-ci.org/aheckmann/mquery)
[](http://badge.fury.io/js/mquery)
[](https://www.npmjs.com/package/mquery)
## Features
- fluent query builder api
- custom base query support
- MongoDB 2.4 geoJSON support
- method + option combinations validation
- node.js driver compatibility
- environment detection
- [debug](https://github.com/visionmedia/debug) support
- separated collection implementations for maximum flexibility
## Use
```js
require('mongodb').connect(uri, function (err, db) {
if (err) return handleError(err);
// get a collection
var collection = db.collection('artists');
// pass it to the constructor
mquery(collection).find({..}, callback);
// or pass it to the collection method
mquery().find({..}).collection(collection).exec(callback)
// or better yet, create a custom query constructor that has it always set
var Artist = mquery(collection).toConstructor();
Artist().find(..).where(..).exec(callback)
})
```
`mquery` requires a collection object to work with. In the example above we just pass the collection object created using the official [MongoDB driver](https://github.com/mongodb/node-mongodb-native).
## Fluent API
- [find](#find)
- [findOne](#findOne)
- [count](#count)
- [remove](#remove)
- [update](#update)
- [findOneAndUpdate](#findoneandupdate)
- [findOneAndDelete, findOneAndRemove](#findoneandremove)
- [distinct](#distinct)
- [exec](#exec)
- [stream](#stream)
- [all](#all)
- [and](#and)
- [box](#box)
- [circle](#circle)
- [elemMatch](#elemmatch)
- [equals](#equals)
- [exists](#exists)
- [geometry](#geometry)
- [gt](#gt)
- [gte](#gte)
- [in](#in)
- [intersects](#intersects)
- [lt](#lt)
- [lte](#lte)
- [maxDistance](#maxdistance)
- [mod](#mod)
- [ne](#ne)
- [nin](#nin)
- [nor](#nor)
- [near](#near)
- [or](#or)
- [polygon](#polygon)
- [regex](#regex)
- [select](#select)
- [selected](#selected)
- [selectedInclusively](#selectedinclusively)
- [selectedExclusively](#selectedexclusively)
- [size](#size)
- [slice](#slice)
- [within](#within)
- [where](#where)
- [$where](#where-1)
- [batchSize](#batchsize)
- [collation](#collation)
- [comment](#comment)
- [hint](#hint)
- [j](#j)
- [limit](#limit)
- [maxScan](#maxscan)
- [maxTime, maxTimeMS](#maxtime)
- [skip](#skip)
- [sort](#sort)
- [read, setReadPreference](#read)
- [readConcern, r](#readconcern)
- [slaveOk](#slaveok)
- [snapshot](#snapshot)
- [tailable](#tailable)
- [writeConcern, w](#writeconcern)
- [wtimeout, wTimeout](#wtimeout)
## Helpers
- [collection](#collection)
- [then](#then)
- [thunk](#thunk)
- [merge](#mergeobject)
- [setOptions](#setoptionsoptions)
- [setTraceFunction](#settracefunctionfunc)
- [mquery.setGlobalTraceFunction](#mquerysetglobaltracefunctionfunc)
- [mquery.canMerge](#mquerycanmerge)
- [mquery.use$geoWithin](#mqueryusegeowithin)
### find()
Declares this query a _find_ query. Optionally pass a match clause and / or callback. If a callback is passed the query is executed.
```js
mquery().find()
mquery().find(match)
mquery().find(callback)
mquery().find(match, function (err, docs) {
assert(Array.isArray(docs));
})
```
### findOne()
Declares this query a _findOne_ query. Optionally pass a match clause and / or callback. If a callback is passed the query is executed.
```js
mquery().findOne()
mquery().findOne(match)
mquery().findOne(callback)
mquery().findOne(match, function (err, doc) {
if (doc) {
// the document may not be found
console.log(doc);
}
})
```
### count()
Declares this query a _count_ query. Optionally pass a match clause and / or callback. If a callback is passed the query is executed.
```js
mquery().count()
mquery().count(match)
mquery().count(callback)
mquery().count(match, function (err, number){
console.log('we found %d matching documents', number);
})
```
### remove()
Declares this query a _remove_ query. Optionally pass a match clause and / or callback. If a callback is passed the query is executed.
```js
mquery().remove()
mquery().remove(match)
mquery().remove(callback)
mquery().remove(match, function (err){})
```
### update()
Declares this query an _update_ query. Optionally pass an update document, match clause, options or callback. If a callback is passed, the query is executed. To force execution without passing a callback, run `update(true)`.
```js
mquery().update()
mquery().update(match, updateDocument)
mquery().update(match, updateDocument, options)
// the following all execute the command
mquery().update(callback)
mquery().update({$set: updateDocument, callback)
mquery().update(match, updateDocument, callback)
mquery().update(match, updateDocument, options, function (err, result){})
mquery().update(true) // executes (unsafe write)
```
##### the update document
All paths passed that are not `$atomic` operations will become `$set` ops. For example:
```js
mquery(collection).where({ _id: id }).update({ title: 'words' }, callback)
```
becomes
```js
collection.update({ _id: id }, { $set: { title: 'words' }}, callback)
```
This behavior can be overridden using the `overwrite` option (see below).
##### options
Options are passed to the `setOptions()` method.
- overwrite
Passing an empty object `{ }` as the update document will result in a no-op unless the `overwrite` option is passed. Without the `overwrite` option, the update operation will be ignored and the callback executed without sending the command to MongoDB to prevent accidently overwritting documents in the collection.
```js
var q = mquery(collection).where({ _id: id }).setOptions({ overwrite: true });
q.update({ }, callback); // overwrite with an empty doc
```
The `overwrite` option isn't just for empty objects, it also provides a means to override the default `$set` conversion and send the update document as is.
```js
// create a base query
var base = mquery({ _id: 108 }).collection(collection).toConstructor();
base().findOne(function (err, doc) {
console.log(doc); // { _id: 108, name: 'cajon' })
base().setOptions({ overwrite: true }).update({ changed: true }, function (err) {
base.findOne(function (err, doc) {
console.log(doc); // { _id: 108, changed: true }) - the doc was overwritten
});
});
})
```
- multi
Updates only modify a single document by default. To update multiple documents, set the `multi` option to `true`.
```js
mquery()
.collection(coll)
.update({ name: /^match/ }, { $addToSet: { arr: 4 }}, { multi: true }, callback)
// another way of doing it
mquery({ name: /^match/ })
.collection(coll)
.setOptions({ multi: true })
.update({ $addToSet: { arr: 4 }}, callback)
// update multiple documents with an empty doc
var q = mquery(collection).where({ name: /^match/ });
q.setOptions({ multi: true, overwrite: true })
q.update({ });
q.update(function (err, result) {
console.log(arguments);
});
```
### findOneAndUpdate()
Declares this query a _findAndModify_ with update query. Optionally pass a match clause, update document, options, or callback. If a callback is passed, the query is executed.
When executed, the first matching document (if found) is modified according to the update document and passed back to the callback.
##### options
Options are passed to the `setOptions()` method.
- `new`: boolean - true to return the modified document rather than the original. defaults to true
- `upsert`: boolean - creates the object if it doesn't exist. defaults to false
- `sort`: if multiple docs are found by the match condition, set
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
毕业设计基于node.js的学生信息管理系统源码, 安装教程 进入admin文件夹。使用npm install 安装依赖,使用npm run serve运行前端页面 进入server文件夹,使用npm install 安装依赖,使用npm run serve运行后端服务 mongodb的数据没有导出。如果想用的话,在server文件夹下面自己找schema,然后设计表吧。一定要有一个管理员的数据,其他数据可以慢慢添加。 test文件夹下面的表,可以导入
资源推荐
资源详情
资源评论
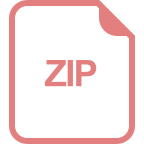
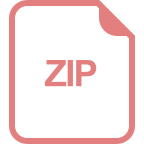
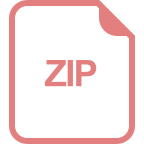
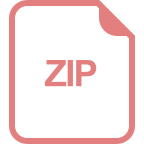
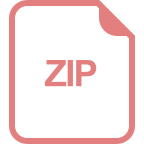
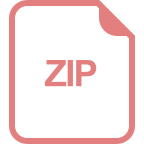
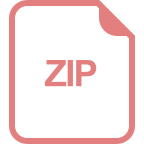
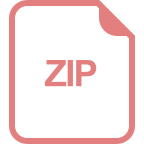
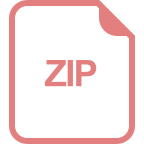
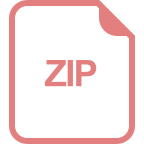
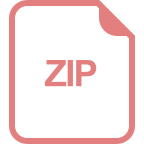
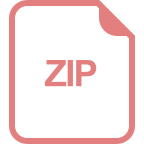
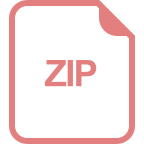
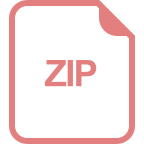
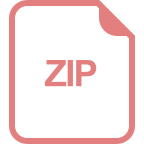
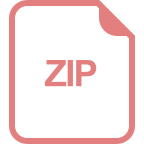
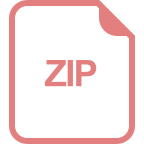
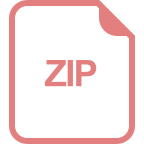
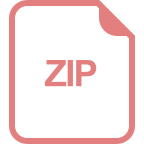
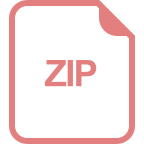
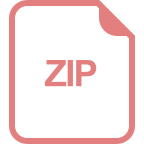
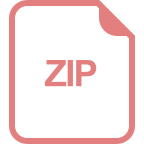
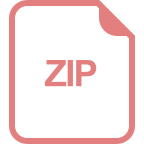
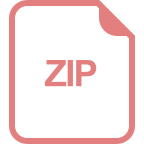
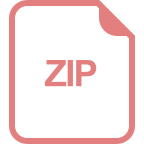
收起资源包目录

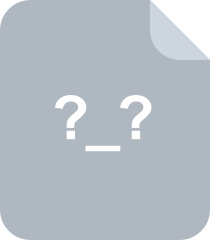
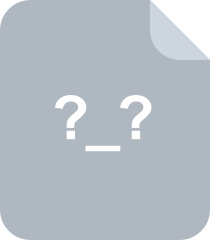
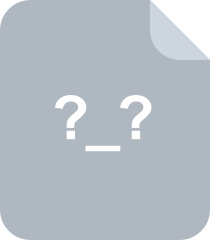
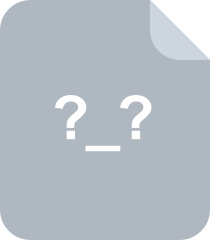
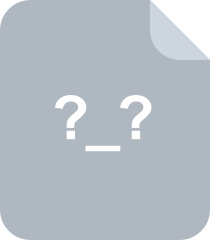
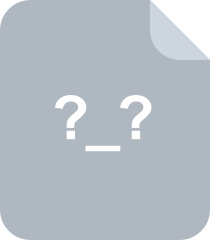
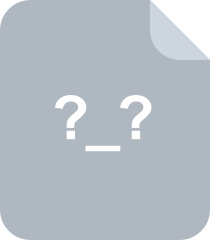
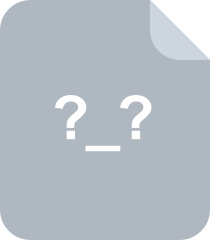
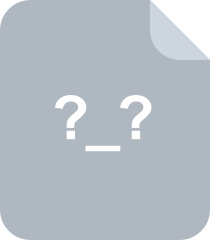
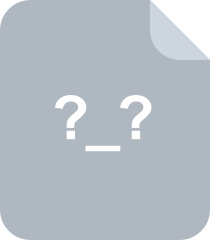
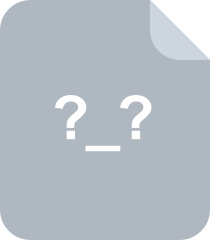
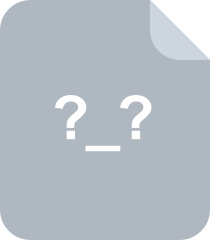
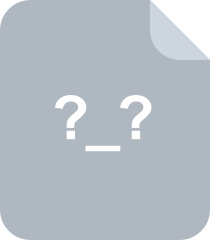
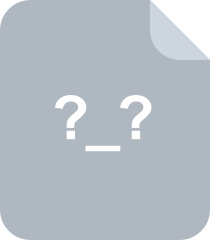
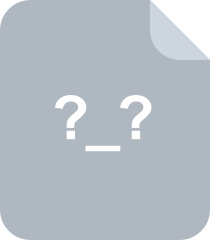
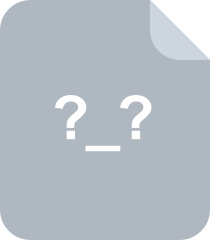
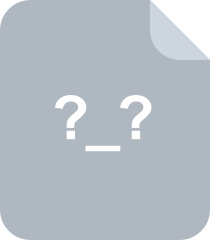
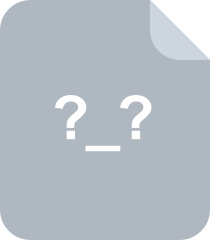
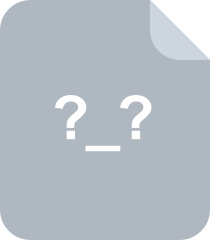
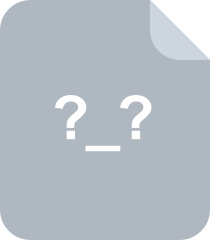
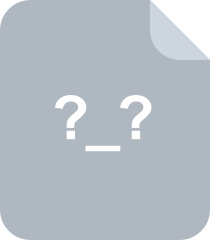
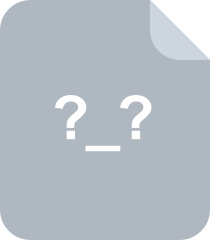
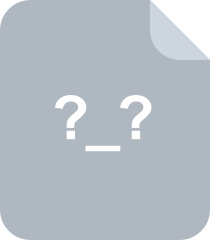
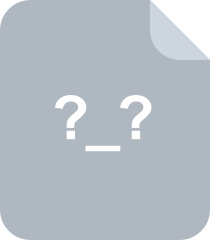
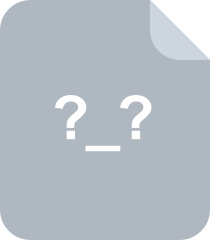
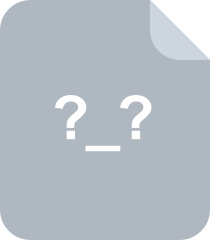
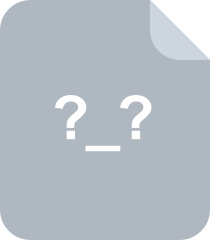
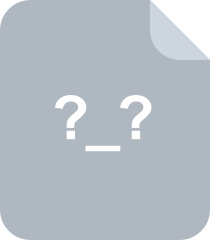
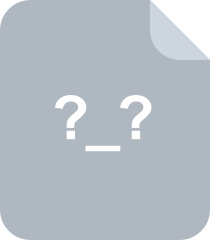
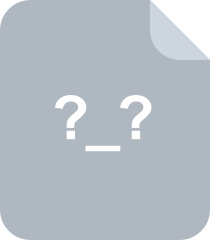
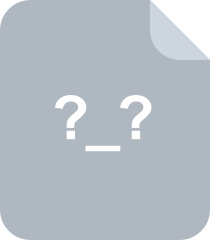
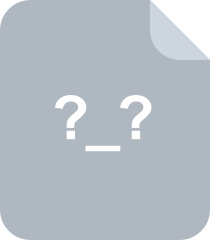
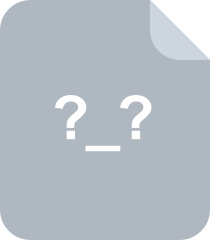
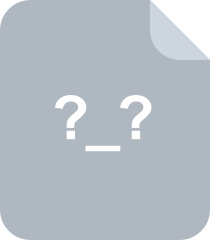
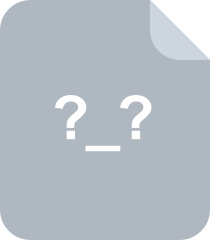
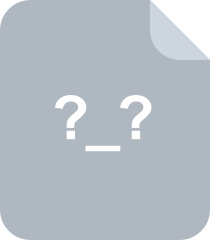
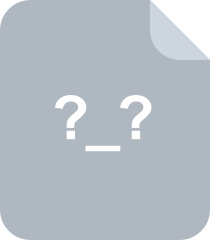
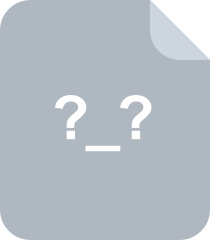
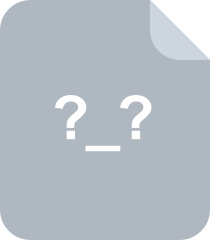
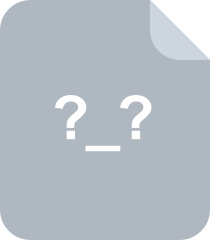
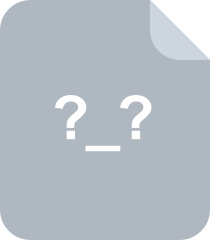
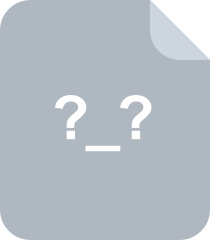
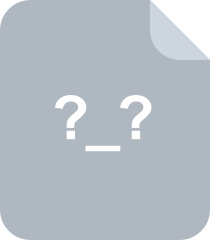
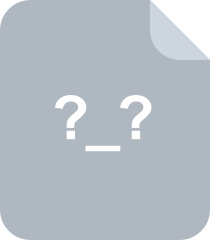
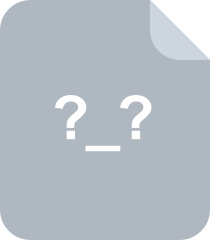
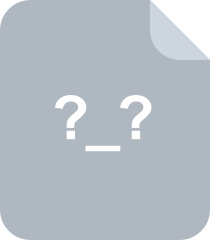
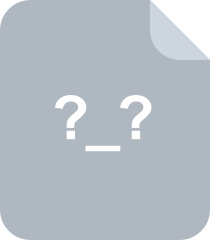
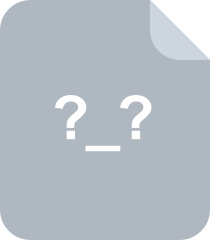
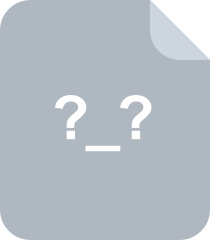
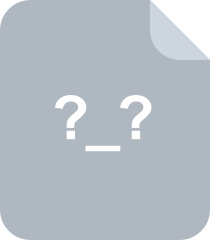
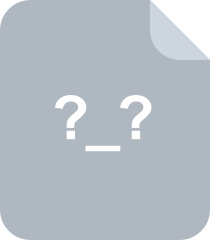
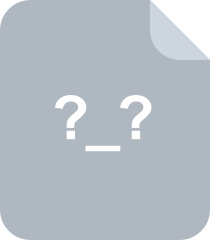
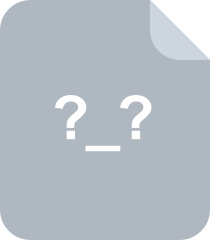
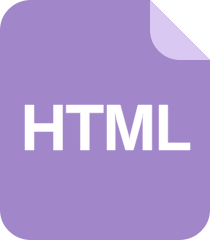
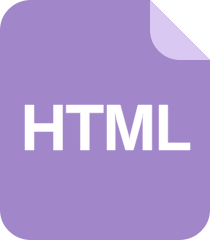
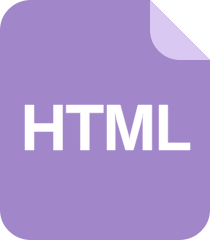
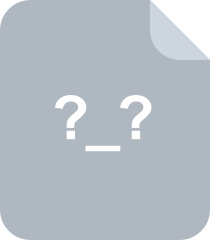
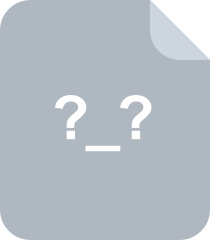
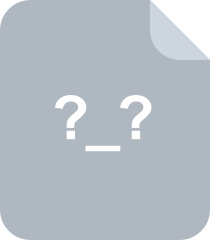
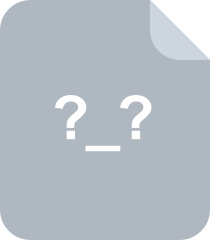
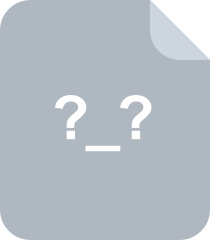
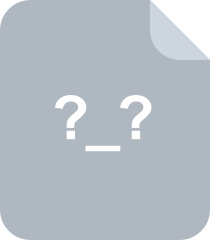
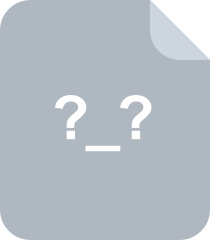
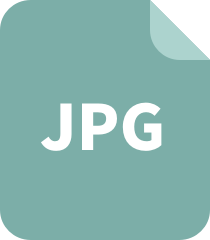
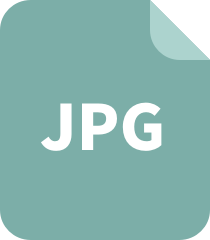
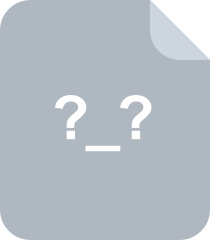
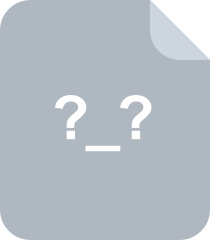
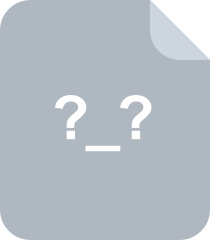
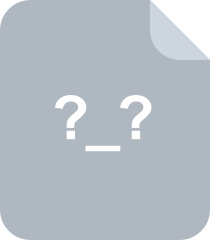
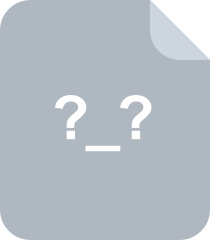
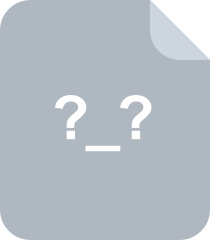
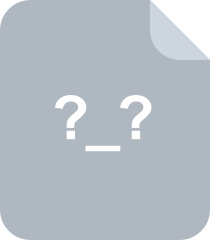
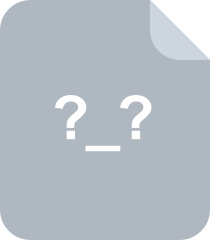
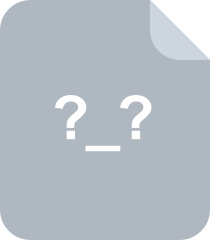
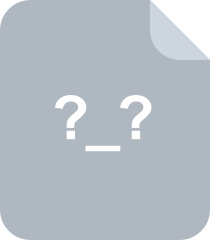
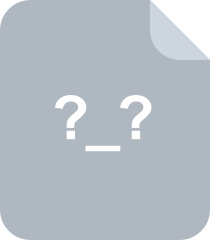
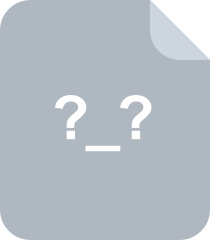
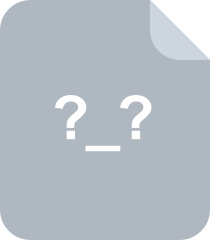
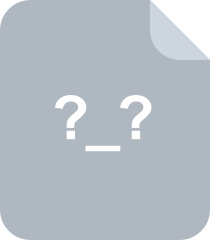
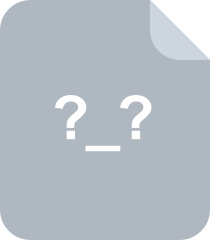
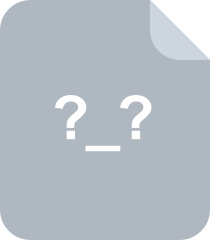
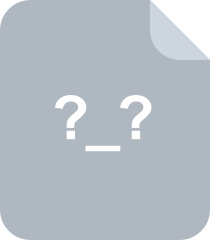
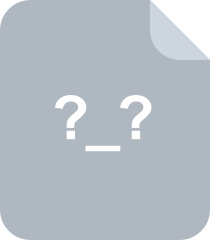
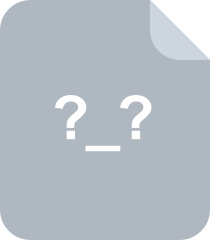
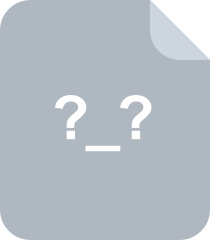
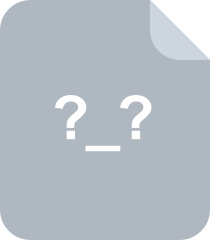
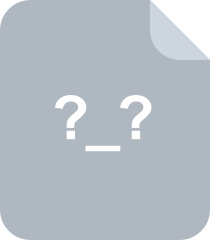
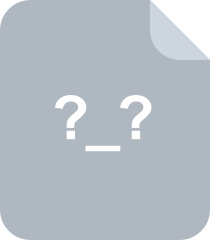
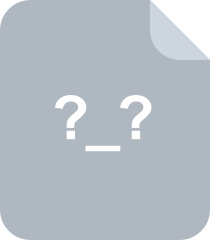
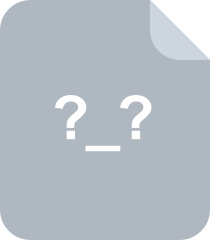
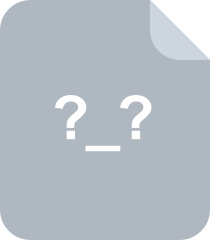
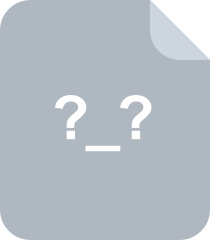
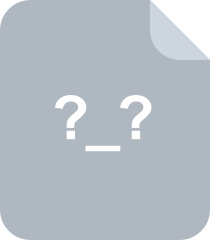
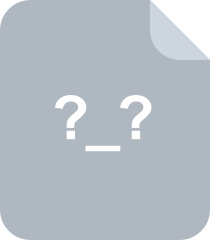
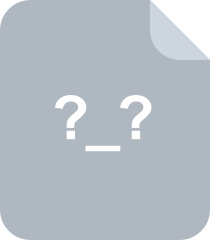
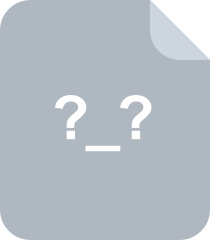
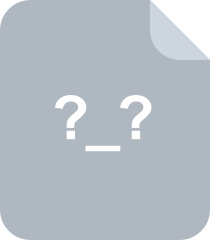
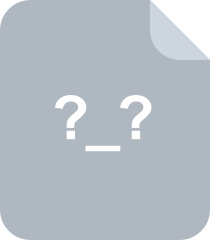
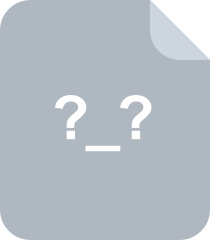
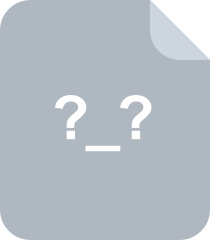
共 1368 条
- 1
- 2
- 3
- 4
- 5
- 6
- 14
资源评论
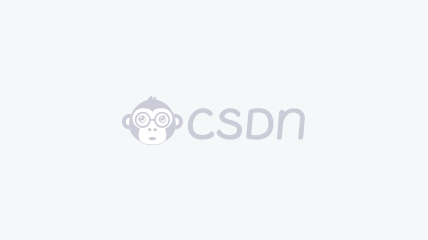

生活家小毛.
- 粉丝: 6050
- 资源: 9295
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

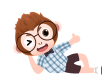
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


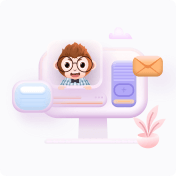
安全验证
文档复制为VIP权益,开通VIP直接复制
