# coding:utf-8
__author__ = "ila"
import os,sys
from django.http import JsonResponse, HttpResponse
from django.apps import apps
def index(request):
if request.method in ["GET", "POST"]:
msg = {"code": 200, "msg": "success", "data": []}
print("=================>index")
# allModels = apps.get_app_config('main').get_models()
# for m in allModels:
# print(m.__tablename__)
# print(dir(m))
# # for col in m._meta.fields:
# # print("col name============>",col.name)
# # print("col type============>",col.get_internal_type())
# print(allModels)
return JsonResponse(msg)
def test(request, p1):
if request.method in ["GET", "POST"]:
msg = {"code": 200, "msg": "success", "data": []}
print("=================>index ", p1)
return JsonResponse(msg)
def null(request,):
if request.method in ["GET", "POST"]:
msg = {"code": 200, "msg": "success", "data": []}
return JsonResponse(msg)
def check_suffix(filelName,path1):
try:
image_data = open(path1, "rb").read()
except:
image_data = "no file"
if '.js' in filelName:
return HttpResponse(image_data, content_type="application/javascript")
elif '.jpg' in filelName or '.jpeg' in filelName or '.png' in filelName or '.gif' in filelName:
return HttpResponse(image_data, content_type="image/png")
elif '.css' in filelName:
return HttpResponse(image_data, content_type="text/css")
elif '.ttf' in filelName or '.woff' in filelName:
return HttpResponse(image_data, content_type="application/octet-stream")
elif '.mp4' in filelName:
return HttpResponse(image_data, content_type="video/mp4")
elif '.mp3' in filelName:
return HttpResponse(image_data, content_type="audio/mp3")
elif '.csv' in filelName:
return HttpResponse(image_data, content_type="application/CSV")
elif '.doc' in filelName:
return HttpResponse(image_data, content_type="application/msword")
elif '.docx' in filelName:
return HttpResponse(image_data, content_type="application/vnd.openxmlformats-officedocument.wordprocessingml.document")
elif '.xls' in filelName:
return HttpResponse(image_data, content_type="application/vnd.ms-excel")
elif '.xlsx' in filelName:
return HttpResponse(image_data, content_type="application/vnd.openxmlformats-officedocument.spreadsheetml.sheet")
elif '.ppt' in filelName:
return HttpResponse(image_data, content_type="application/vnd.ms-powerpoint")
elif '.pptx' in filelName:
return HttpResponse(image_data, content_type="application/vnd.openxmlformats-officedocument.presentationml.presentation")
elif '.zip' in filelName:
return HttpResponse(image_data, content_type="application/x-zip-compressed")
elif '.rar' in filelName:
return HttpResponse(image_data, content_type="application/octet-stream")
else:
return HttpResponse(image_data, content_type="text/html")
def admin_lib2(request, p1, p2):
if request.method in ["GET", "POST"]:
fullPath = request.get_full_path()
print("{}=============>".format(sys._getframe().f_code.co_name), fullPath)
path1 = os.path.join(os.getcwd(), "templates/front/admin/lib/", p1, p2)
return check_suffix(eval(eval(sys._getframe().f_code.co_name).__code__.co_varnames[-3]),path1)
# try:
# image_data = open(path1, "rb").read()
# except:
# image_data="no file"
# if '.js' in p2:
# return HttpResponse(image_data, content_type="application/javascript")
# elif '.jpg' in p2 or '.jpeg' in p2 or '.png' in p2 or '.gif' in p2:
# return HttpResponse(image_data, content_type="image/png")
# elif '.css' in p2:
# return HttpResponse(image_data, content_type="text/css")
# elif '.ttf' in p2 or '.woff' in p2:
# return HttpResponse(image_data, content_type="application/octet-stream")
# elif '.mp4' in p2:
# return HttpResponse(image_data, content_type="video/mp4")
# elif '.mp3' in p2:
# return HttpResponse(image_data, content_type="audio/mp3")
# else:
# return HttpResponse(image_data, content_type="text/html")
def admin_lib3(request, p1, p2, p3):
if request.method in ["GET", "POST"]:
fullPath = request.get_full_path()
print("{}=============>".format(sys._getframe().f_code.co_name), fullPath)
path1 = os.path.join(os.getcwd(), "templates/front/admin/lib/", p1, p2, p3)
return check_suffix(eval(eval(sys._getframe().f_code.co_name).__code__.co_varnames[-3]),path1)
# try:
# image_data = open(path1, "rb").read()
# except:
# image_data="no file"
# if '.js' in p3:
# return HttpResponse(image_data, content_type="application/javascript")
# elif '.jpg' in p3 or '.jpeg' in p3 or '.png' in p3 or '.gif' in p3:
# return HttpResponse(image_data, content_type="image/png")
# elif '.css' in p3:
# return HttpResponse(image_data, content_type="text/css")
# elif '.ttf' in p3 or '.woff' in p3:
# return HttpResponse(image_data, content_type="application/octet-stream")
# elif '.mp4' in p3:
# return HttpResponse(image_data, content_type="video/mp4")
# elif '.mp3' in p3:
# return HttpResponse(image_data, content_type="audio/mp3")
# else:
# return HttpResponse(image_data, content_type="text/html")
def admin_lib4(request, p1, p2, p3, p4):
if request.method in ["GET", "POST"]:
fullPath = request.get_full_path()
print("{}=============>".format(sys._getframe().f_code.co_name), fullPath)
path1 = os.path.join(os.getcwd(), "templates/front/admin/lib/", p1, p2, p3, p4)
return check_suffix(eval(eval(sys._getframe().f_code.co_name).__code__.co_varnames[-3]),path1)
# try:
# image_data = open(path1, "rb").read()
# except:
# image_data="no file"
# if '.js' in p4:
# return HttpResponse(image_data, content_type="application/javascript")
# elif '.jpg' in p4 or '.jpeg' in p4 or '.png' in p4 or '.gif' in p4:
# return HttpResponse(image_data, content_type="image/png")
# elif '.css' in p4:
# return HttpResponse(image_data, content_type="text/css")
# elif '.ttf' in p4 or '.woff' in p4:
# return HttpResponse(image_data, content_type="application/octet-stream")
# elif '.mp4' in p4:
# return HttpResponse(image_data, content_type="video/mp4")
# elif '.mp3' in p4:
# return HttpResponse(image_data, content_type="audio/mp3")
# else:
# return HttpResponse(image_data, content_type="text/html")
def admin_page(request, p1):
if request.method in ["GET", "POST"]:
fullPath = request.get_full_path()
print("{}=============>".format(sys._getframe().f_code.co_name), fullPath)
path1 = os.path.join(os.getcwd(), "templates/front/admin/page/", p1)
return check_suffix(eval(eval(sys._getframe().f_code.co_name).__code__.co_varnames[-3]),path1)
# try:
# image_data = open(path1, "rb").read()
# except:
# image_data="no file"
# if '.js' in p1:
# return HttpResponse(image_data, content_type="application/javascript")
# elif '.jpg' in p1 or '.jpeg' in p1 or '.png' in p1 or '.gif' in p1:
# return HttpResponse(image_data, content_type="image/png")
# elif '.css' in p1:
# return HttpResponse(image_data, content_type="text/css")
# elif '.ttf' in p1 or '.woff' in p1:
# return HttpResponse(image_data, content_type="application/octet-stream")
# elif '.mp4' in p1
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
小程序开发说明 开发语言:Java 框架:ssm JDK版本:JDK1.8 服务器:tomcat7 数据库:mysql 5.7(一定要5.7版本) 数据库工具:Navicat11 开发软件:eclipse/myeclipse/idea Maven包:Maven3.3.9 浏览器:谷歌浏览器 小程序框架:uniapp 小程序开发软件:HBuilder X 小程序运行软件:微信开发者 微信小程序配置环境链接:https://pan.baidu.com/s/1TAzjNmxQ0RfJpVIjlxh_gQ 后台路径地址:localhost:8080/项目名称/admin/dist/index.html 管理员账号:abo 管理员密码:abo
资源推荐
资源详情
资源评论
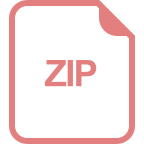
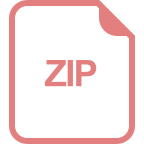
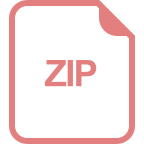
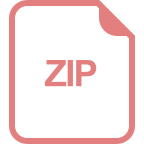
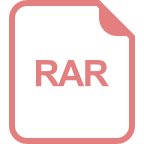
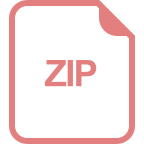
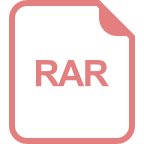
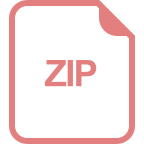
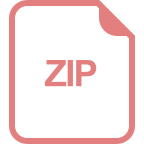
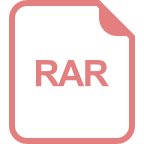
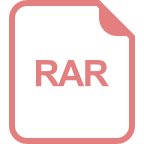
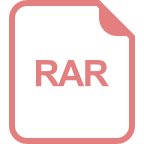
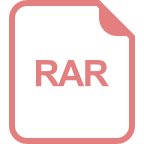
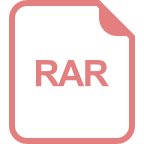
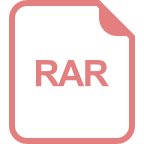
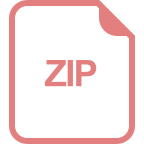
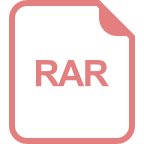
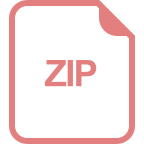
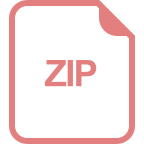
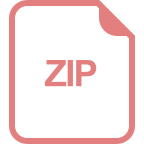
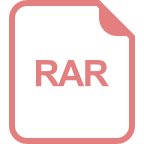
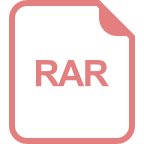
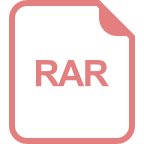
收起资源包目录

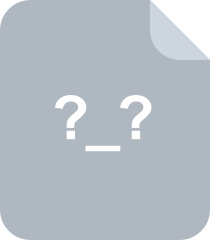
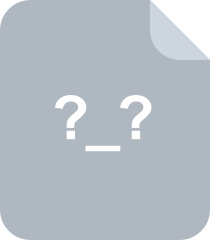
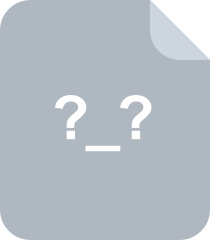
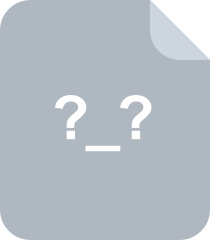
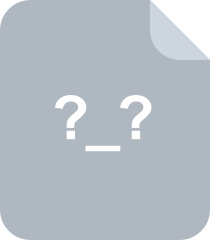
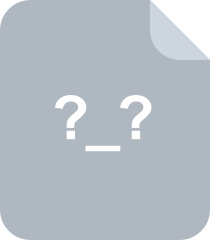
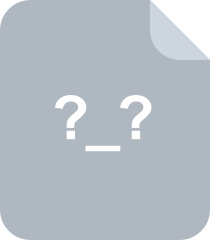
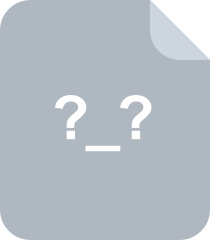
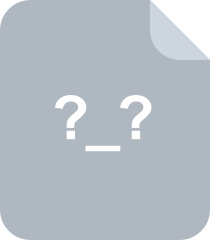
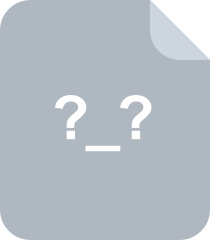
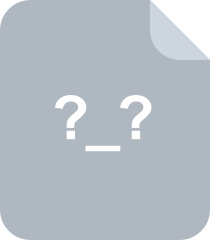
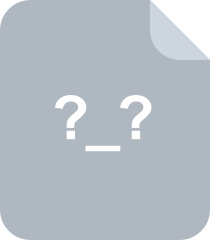
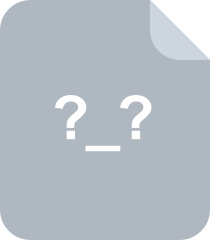
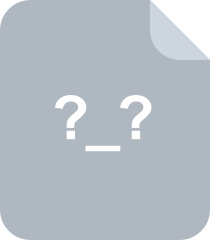
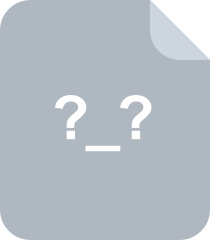
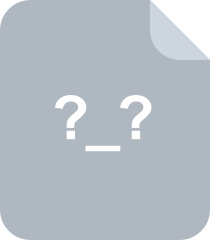
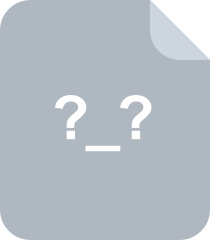
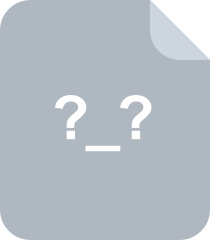
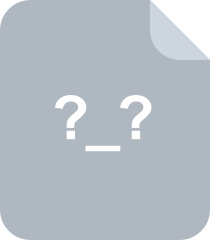
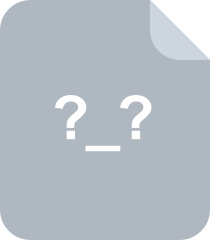
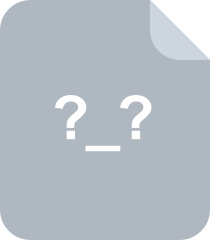
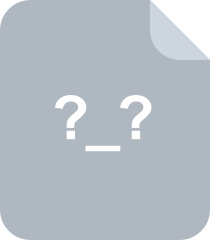
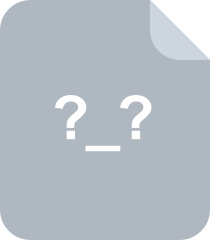
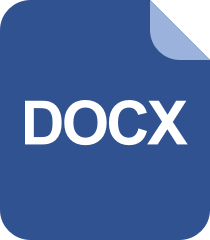
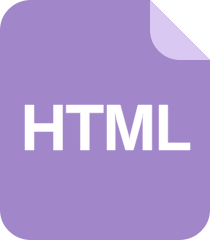
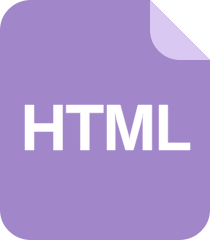
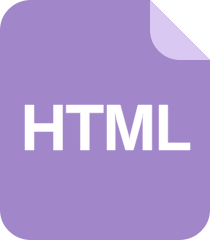
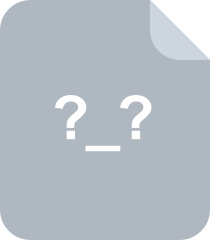
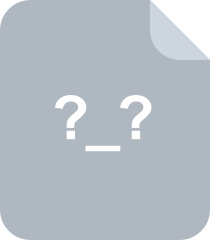
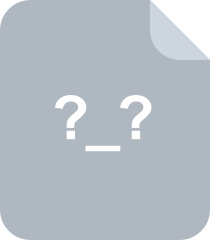
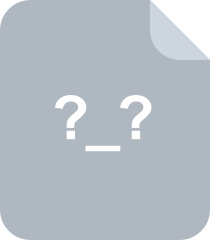
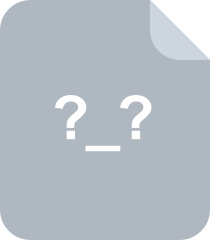
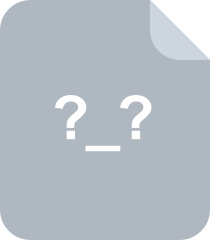
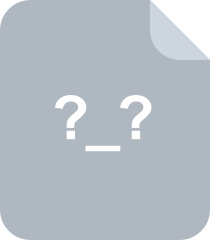
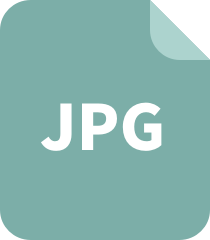
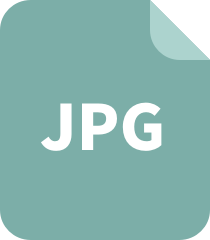
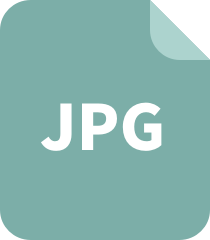
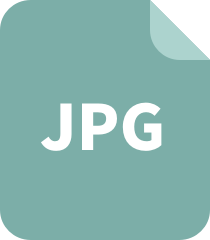
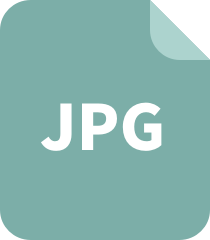
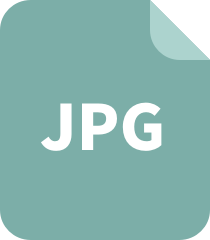
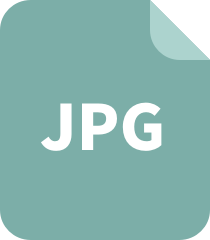
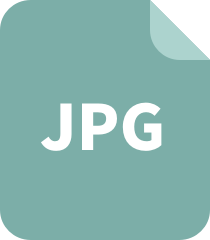
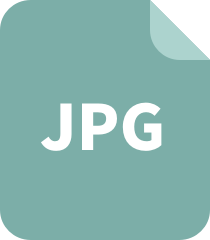
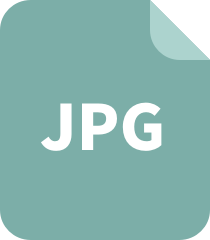
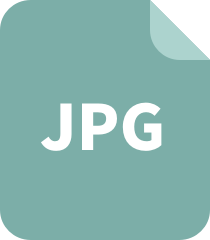
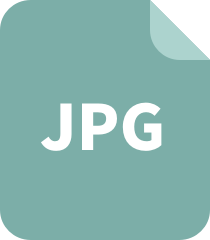
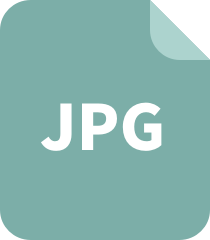
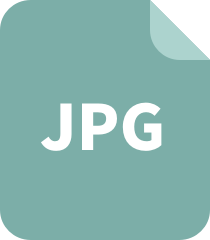
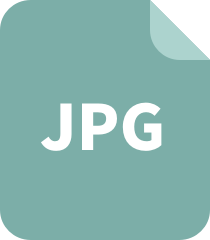
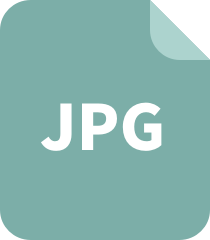
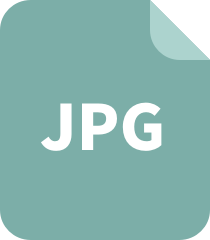
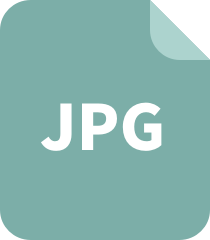
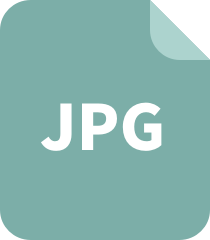
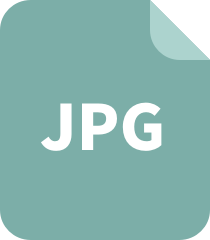
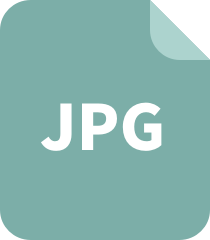
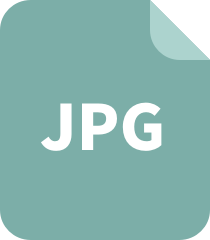
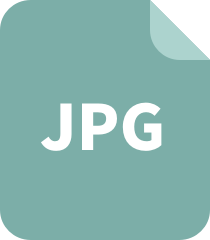
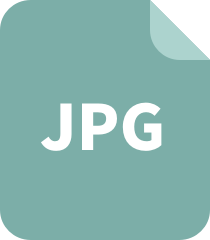
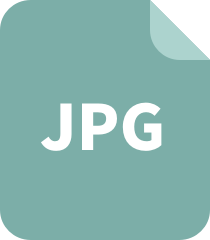
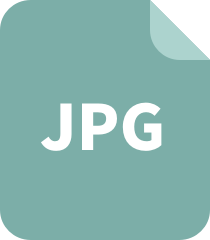
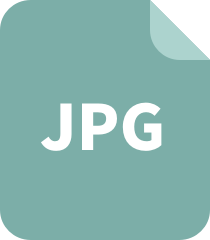
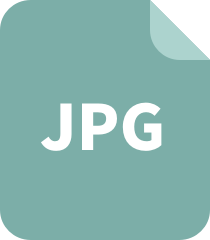
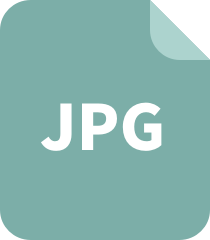
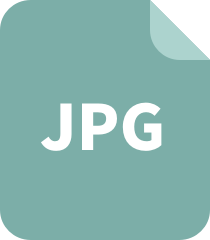
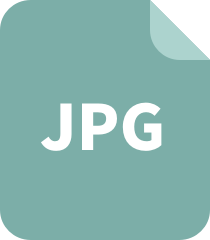
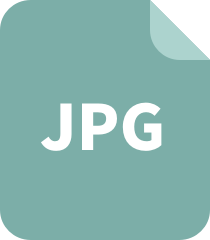
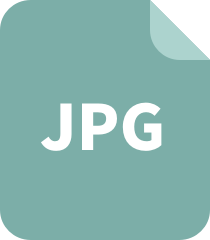
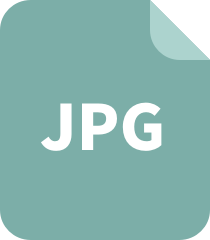
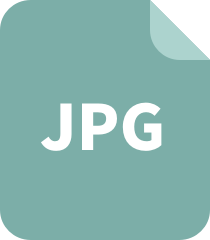
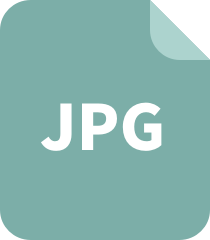
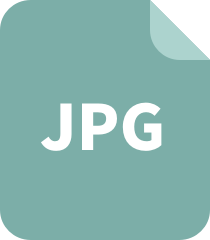
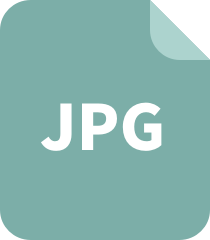
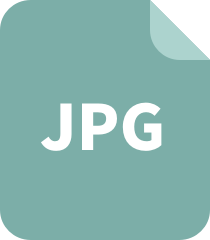
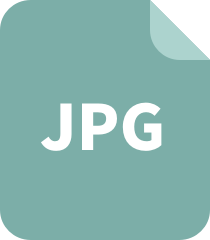
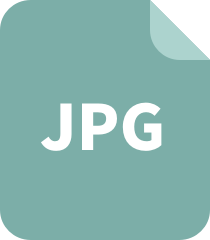
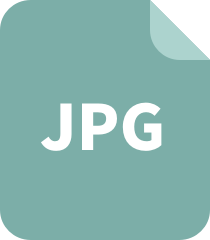
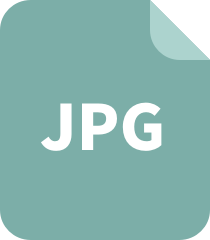
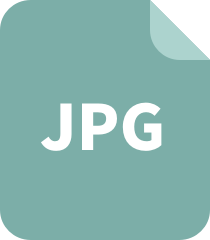
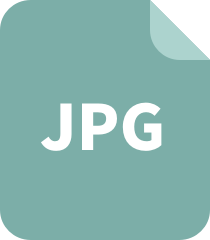
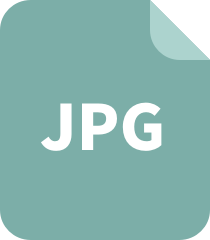
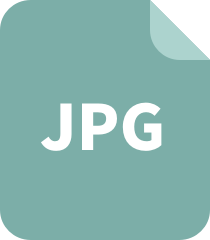
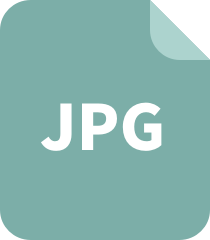
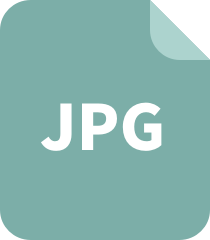
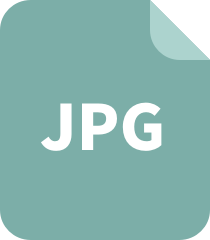
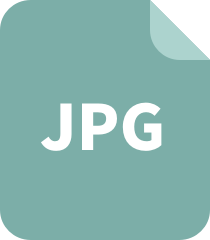
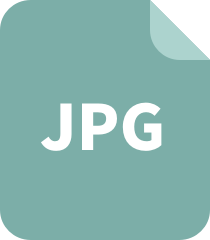
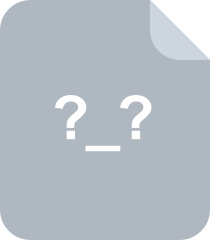
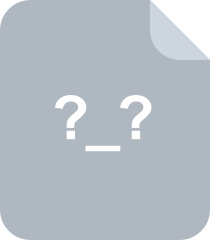
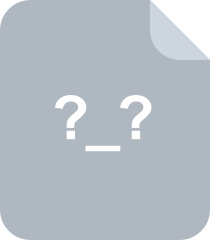
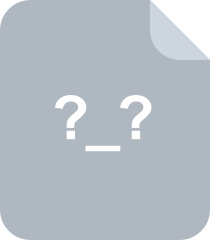
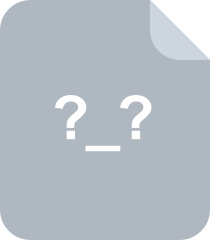
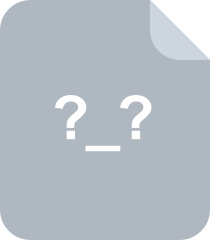
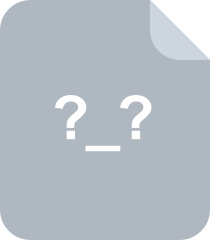
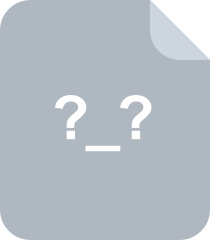
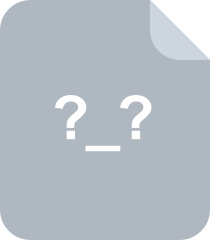
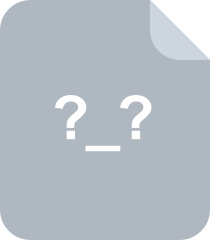
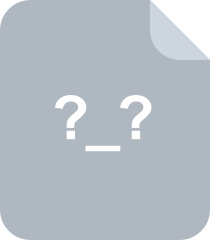
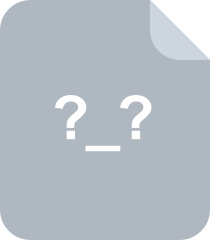
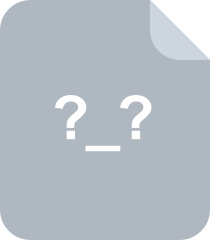
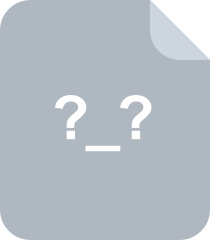
共 1558 条
- 1
- 2
- 3
- 4
- 5
- 6
- 16
资源评论
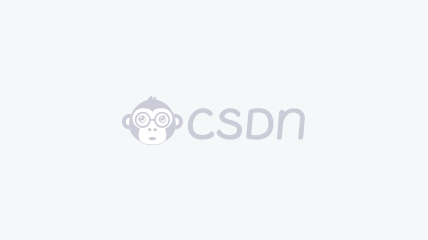

毕设王同学
- 粉丝: 127
- 资源: 1193
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

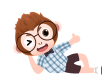
最新资源
- 光纤到户及通信基础设施报装申请表.docx
- 踝关节功能丧失程度评定表.docx
- 环保设施投资估算表.docx
- 既有建筑物通信报装申请表.docx
- 既有建筑物通信报装现场查勘报告.docx
- 监督机构检查记录表.docx
- 肩关节功能丧失程度评定表.docx
- 大学生创新创业训练计划大创项目的全流程指南
- 简易低风险工业厂房通信报装申请表.docx
- 建设工程消防验收各阶段意见回复表.docx
- 建设工程消防验收模拟验收意见表.docx
- 建设工程消防验收图纸核查意见表.docx
- 建设工程消防验收现场指导意见表.docx
- 建筑工程竣工验收消防设计质量检查报告(表格填写模板).docx
- 建筑工程消防查验意见和结论.docx
- 建筑工程消防施工竣工报告(表格填写模板).docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


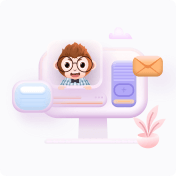
安全验证
文档复制为VIP权益,开通VIP直接复制
