import tkinter as tk
from tkinter import ttk
import ttkbootstrap as ttkb
import custom_widget as tkpp
import pandas as pd
import joblib
import os
from PIL import Image,ImageTk
import ttkbootstrap.themes.user
import datetime
import lists
import numpy as np
from time import sleep
import tkinter.messagebox
model_path='model.pkl'
train_origin_path='train.csv'
test_origin_path='test.csv'
test_modified_path='test_modified.csv'
submission_path='submission.csv'
images_path='picture'
main_page_bg_path='images/main_page_bg.jpg'
sub_bg_path='images/sub_bg.jpg'
pre_single_path='images/pre_single_bg.jpg'
style=ttkb.Style(theme='litera')
root=style.master
sw=root.winfo_screenwidth()//2
sh=root.winfo_screenheight()//2
main_page_window_size=(900,650)
data_browser_window_size=(1400,800)
data_browser_search_window_size=(1400,300)
pre_data_page_window_size=(250,610)
predict_page_search_window_size=(250,100)
pre_single_page_window_size=data_browser_window_size
element_select_window_size=pre_single_page_window_size
analysis_page_window_size=(935,800)
message_box_window_size=(200,100)
image_size=(200,200)
def resize(image,k):
size=image.size
return image.resize((int(size[0]*k),int(size[1]*k)))
def load_npy(name):
return list(np.load('npy/%s.npy'%name,allow_pickle=True))
def set_window_position(root,pos):
ws=pos
root.geometry(str(ws[0])+'x'+str(ws[1]))
root.geometry('+{}+{}'.format(sw-ws[0]//2,sh-ws[1]//2))
root.resizable(0,0)
def multipleList(root,area,data=None,height=5,width=100,width_column=20,anchor='n',padding=(0,0,0,0),style='info',place_anchor='nw',relx=0,rely=0.1,command=None):#padding为表外留白
ac=[]
for i in range(len(area)):
ac.append(i)
ac=tuple(ac)
tv=ttkb.Treeview(
root,
columns=ac,
show='headings',
height=height,
padding=padding,
bootstyle=style)
for i in range(len(area)):
tv.column(
ac[i],
width=int(width/len(area)),
minwidth=width_column,
stretch=True,
anchor=anchor)
tv.heading(ac[i],text=area[i])
tv.bind('<Double-Button-1>',command)
tv.place(anchor=place_anchor,relx=relx,rely=rely)
if isinstance(data,pd.core.frame.DataFrame):
for index, row in data.iterrows():
tv.insert('','end',values=row.tolist())
elif data==None:
return tv
else:
for i in data:
tv.insert('','end',values=i)
return tv
class message_box:
def __init__(self,content):
self.root=ttkb.Toplevel(title=' ')
set_window_position(self.root,message_box_window_size)
ttkb.Label(
self.root,
text=content,
bootstyle='dark').place(anchor='n',relx=0.5,rely=0.2)
ttkb.Button(
self.root,
text='确定',
bootstyle='primary',
command=self.exit).place(anchor='n',relx=0.5,rely=0.55)
def exit(self):
self.root.destroy()
class data_browser:
def __init__(self,path):
if path==train_origin_path:
self.root=ttkb.Toplevel(title='训练数据集')
elif path==test_origin_path:
self.root=ttkb.Toplevel(title='测试数据集')
set_window_position(self.root,data_browser_window_size)
image=Image.open(sub_bg_path)
image=resize(image,1)
bg=ImageTk.PhotoImage(image)
cv =ttkb.Canvas(self.root,width=data_browser_window_size[0],height=data_browser_window_size[1])
cv.pack()
cv.create_image(data_browser_window_size[0]//2,data_browser_window_size[1]//2,image=bg)
self.data=pd.read_csv(path)
self.data2=self.data
self.data=self.data.astype(str)
self.features=list(self.data)
size=len(self.data)
self.balloon=None
ttkb.Button(
self.root,
text='退出',
bootstyle='primary',
command=self.exit).place(anchor='n',relx=0.04,rely=0.05)
self.search_layer=ttkb.Combobox(
self.root,
values=self.features,
width=8,
bootstyle="primary")
self.search_layer.place(anchor='n',relx=0.75,rely=0.05)
# self.search_layer.bind('<<ComboboxSelected>>',self.seperate)
self.search_entry = ttkb.Entry(
self.root,
width=9,
bootstyle='dark')
self.search_entry.place(anchor='n', relx=0.83, rely=0.05)
self.search_entry.bind('<Enter>',lambda event=True,x=0.80,y=0.05-0.03:self.balloon_show(event,x,y))
self.search_entry.bind('<Leave>',self.balloon_hide)
# self.left_search_entry=None
# self.label_to=None
# self.right_search_entry=None
search_button=ttkb.Button(
self.root,
text='搜索',
bootstyle='primary',
command=self.search)
search_button.place(anchor='n',relx=0.9,rely=0.05)
multipleList(
self.root,
self.features,
self.data,
width=data_browser_window_size[0],
width_column=100,
height=38)
show_size=ttkb.Label(
self.root,
bootstyle='info',
text='共 '+str(size)+' 个样本')
show_size.place(anchor='n',relx=0.05,rely=0.91)
self.root.mainloop()
# def seperate(self,event):
# _type=self.data2.dtypes[self.search_layer.get()]
# if _type==np.dtype('float64') or _type==np.dtype('int64'):
# self.search_entry.destroy()
# self.left_search_entry=ttkb.Entry(
# self.root,
# width=4,
# bootstyle='dart')
# self.left_search_entry.place(anchor='nw', relx=0.79, rely=0.05)
# self.label_to=ttkb.Label(
# self.root,
# text='~',
# bootstyle='dart')
# self.label_to.place(anchor='nw', relx=0.83, rely=0.055)
# self.right_search_entry=ttkb.Entry(
# self.root,
# width=4,
# bootstyle='dart')
# self.right_search_entry.place(anchor='nw', relx=0.844, rely=0.05)
# else:
# self.left_search_entry.destroy()
# self.label_to.destroy()
# self.right_search_entry.destroy()
# self.search_entry = ttkb.Entry(
# self.root,
# width=9,
# bootstyle='dark')
# self.search_entry.place(anchor='n', relx=0.83, rely=0.05)
def balloon_show(self,event,x,y):
_type=self.data2.dtypes[self.search_layer.get()]
if _type==np.dtype('float64') or _type==np.dtype('int64'):
self.balloon=ttkb.Label(
self.root,
text="输入单个数或用'-'分隔")
else:
self.balloon=ttkb.Label(
self.root,
text='输入单个值')
self.balloon.place(relx=x,rely=y)
def balloon_hide(self,event):
self.balloon.destroy()
def search(self):
feature=self.search_layer.get()
if self.data2.dtypes[self.search_layer.get()] == object:
result=self.data.loc[self.data[feature]==self.search_entry.get()]
else:
try:
sp=self.search_entry.get().split('-')
if len(sp)==2:
bottom,top=sp
result=self.data.loc[(self.data2[feature]>=float(bottom))&(self.data2[feature]<=float(top))]
else:
result=self.data.loc[self.data2[feature]==float(sp[0])]
# print(bottom,top)
except:
message_box('请规范输入!')
if len(result)>0:
show=ttkb.Toplevel(title='搜索结果 共 '+str(len(result))+'
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【资源说明】 基于python+tkinter的房价预测可视化系统源码(含数据集+模型).zip基于python+tkinter的房价预测可视化系统源码(含数据集+模型).zip 基于python+tkinter的房价预测可视化系统源码(含数据集+模型).zip 基于python+tkinter的房价预测可视化系统源码(含数据集+模型).zip 基于python+tkinter的房价预测可视化系统源码(含数据集+模型).zip 主文件:house_price.py 模型训练:house-price-prediction.ipynb 生成分析图:analysis.ipynb 【备注】 1、该资源内项目代码都经过测试运行成功,功能ok的情况下才上传的,请放心下载使用! 2、本项目适合计算机相关专业(如计科、人工智能、通信工程、自动化、电子信息等)的在校学生、老师或者企业员工下载使用,也适合小白学习进阶,当然也可作为毕设项目、课程设计、作业、项目初期立项演示等。 3、如果基础还行,也可在此代码基础上进行修改,以实现其他功能,也可直接用于毕设、课设、作业等。 欢迎下载,沟通交流,互相学习,共同进步!
资源推荐
资源详情
资源评论
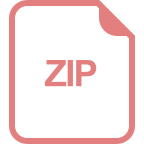
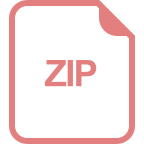
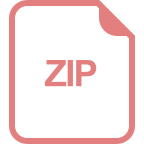
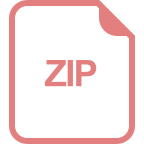
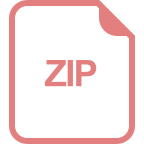
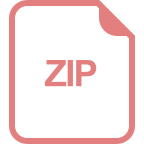
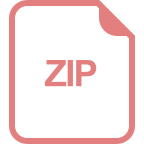
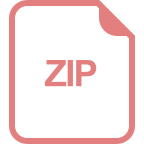
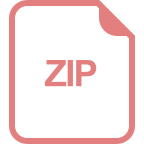
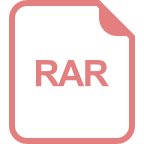
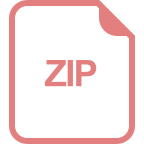
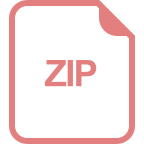
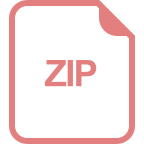
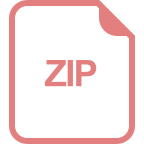
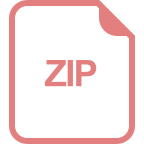
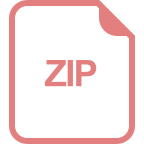
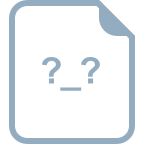
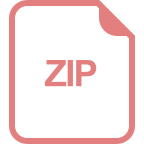
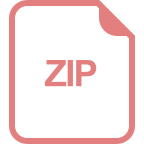
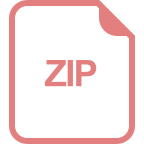
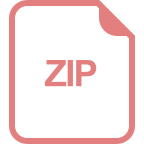
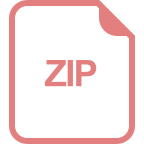
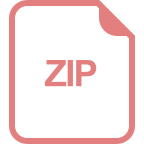
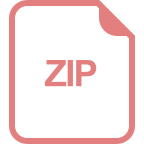
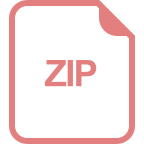
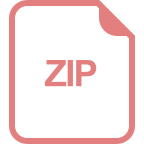
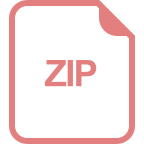
收起资源包目录


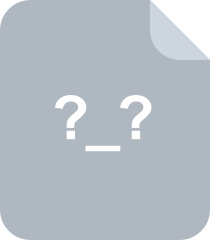
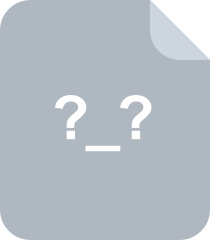
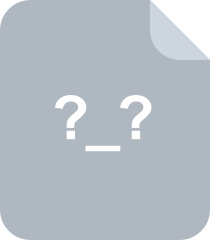
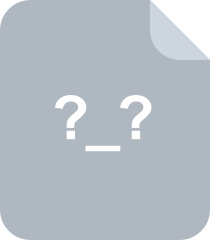
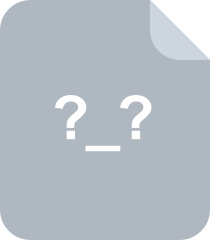
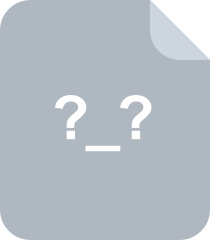
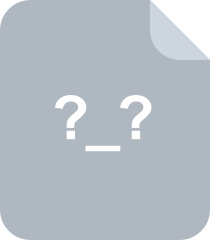
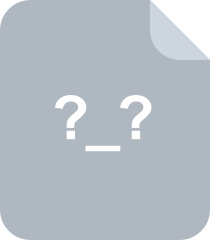
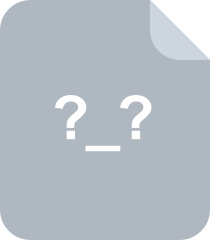
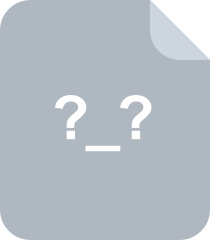
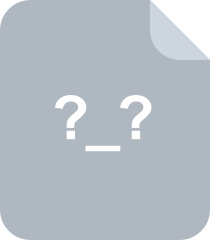
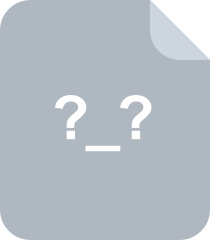
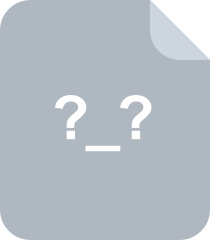

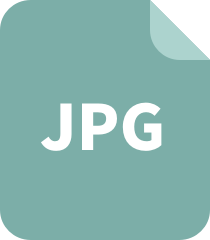
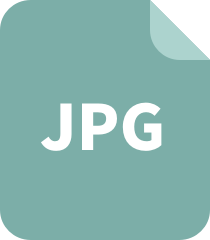
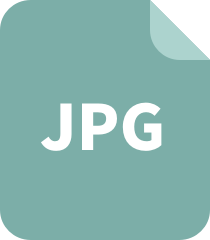
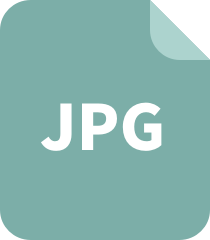
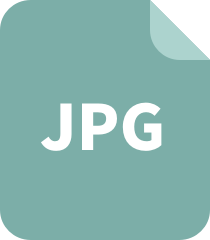
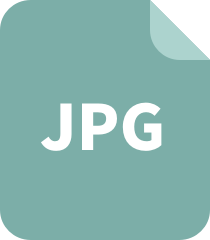
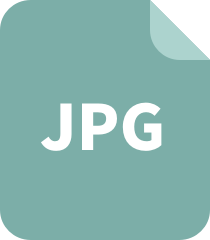
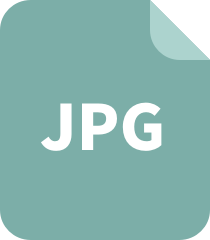
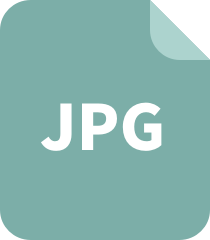
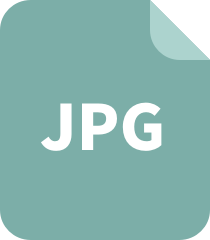
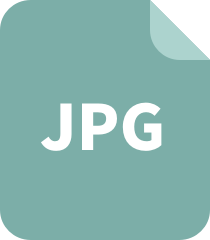
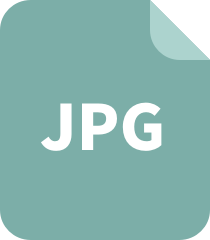
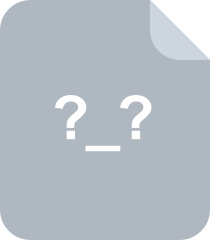
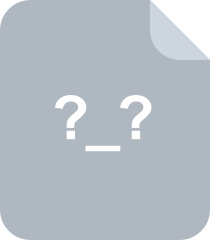
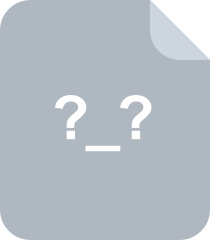
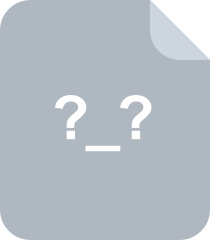
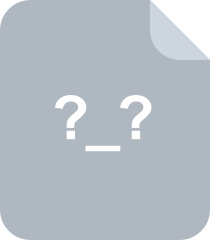
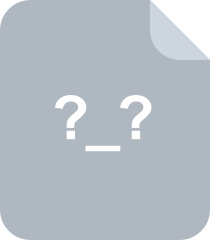
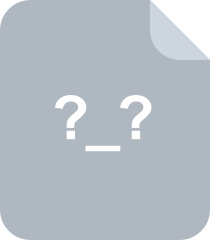
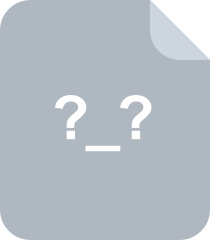
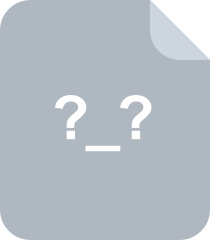
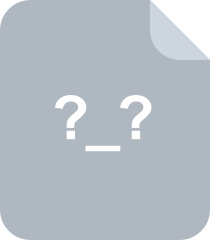
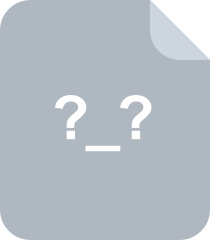

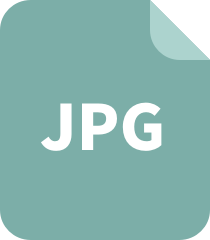
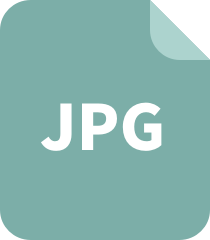
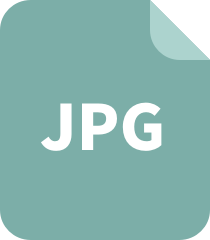
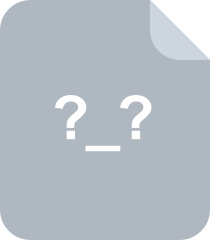
共 40 条
- 1
资源评论
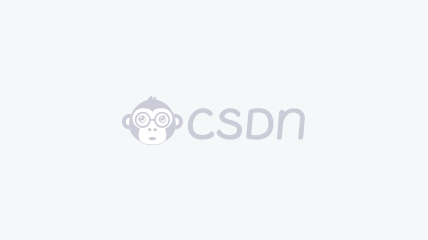
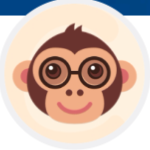
onnx
- 粉丝: 9637
- 资源: 5598
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

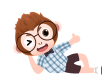
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


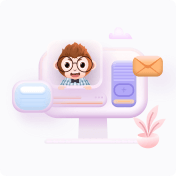
安全验证
文档复制为VIP权益,开通VIP直接复制
