#include "SWFaceMatchApi.h"
#include "facerecon.h"
#include "dataprocess.h"
#include <fstream>
#include <time.h>
#include <math.h>
#include <stddef.h>
#include <stdlib.h>
#include <string.h>
#include <iostream>
#include <list>
using namespace cv;
using namespace std;
double g_meanface[15] = { -31.1297 , 34.0010 , 122.4674,
30.9267 , 33.7814 , 122.1970 ,
0.0132 , 4.4303 , 161.5600 ,
-29.3909 , -33.8801 , 128.1061 ,
29.1029 , -33.5093 , 127.8227};
double g_meanfacelm2[10] = { 30.2946, 51.6963 - 25,
65.5318, 51.5014 - 25,
48.0252, 71.7366,
33.5493, 92.3615,
62.7299, 92.2041 };
int g_img_size = 450;
Size m_cropimg_size = Size(96,112);
extern int g_bnormalize ;
extern int g_bnormalizeX ;
extern int g_bnormalizeY ;
//vector<Point2f> g_meanface2D = {Point2f(30.2946, 51.6963),
// Point2f(65.5318, 51.5014),
// Point2f(48.0252, 71.7366),
// Point2f(33.5493, 92.3655),
// Point2f(62.7299, 92.2041)};
Mat tformfwd(const Mat& trans, const Mat& uv)
{
Mat uv_h = Mat::ones(uv.rows, 3, CV_64FC1);
uv.copyTo(uv_h(Rect(0, 0, 2, uv.rows)));
Mat xv_h = uv_h*trans;
return xv_h(Rect(0, 0, 2, uv.rows));
}
Mat find_none_flectives_similarity(const Mat& uv, const Mat& xy)
{
Mat A = Mat::zeros(2 * xy.rows, 4, CV_64FC1);
Mat b = Mat::zeros(2 * xy.rows, 1, CV_64FC1);
Mat x = Mat::zeros(4, 1, CV_64FC1);
xy(Rect(0, 0, 1, xy.rows)).copyTo(A(Rect(0, 0, 1, xy.rows)));//x
xy(Rect(1, 0, 1, xy.rows)).copyTo(A(Rect(1, 0, 1, xy.rows)));//y
A(Rect(2, 0, 1, xy.rows)).setTo(1.);
xy(Rect(1, 0, 1, xy.rows)).copyTo(A(Rect(0, xy.rows, 1, xy.rows)));//y
(xy(Rect(0, 0, 1, xy.rows))).copyTo(A(Rect(1, xy.rows, 1, xy.rows)));//-x
A(Rect(1, xy.rows, 1, xy.rows)) *= -1;
A(Rect(3, xy.rows, 1, xy.rows)).setTo(1.);
uv(Rect(0, 0, 1, uv.rows)).copyTo(b(Rect(0, 0, 1, uv.rows)));
uv(Rect(1, 0, 1, uv.rows)).copyTo(b(Rect(0, uv.rows, 1, uv.rows)));
cv::solve(A, b, x, cv::DECOMP_SVD);
Mat trans_inv = (Mat_<double>(3, 3) << x.at<double>(0), -x.at<double>(1), 0,
x.at<double>(1), x.at<double>(0), 0,
x.at<double>(2), x.at<double>(3), 1);
Mat trans = trans_inv.inv(cv::DECOMP_SVD);
trans.at<double>(0, 2) = 0;
trans.at<double>(1, 2) = 0;
trans.at<double>(2, 2) = 1;
return trans;
}
Mat find_similarity(const Mat& uv, const Mat& xy)
{
Mat trans1 = find_none_flectives_similarity(uv, xy);
Mat xy_reflect = xy;
xy_reflect(Rect(0, 0, 1, xy.rows)) *= -1;
Mat trans2r = find_none_flectives_similarity(uv, xy_reflect);
Mat reflect = (Mat_<double>(3, 3) << -1, 0, 0, 0, 1, 0, 0, 0, 1);
Mat trans2 = trans2r*reflect;
Mat xy1 = tformfwd(trans1, uv);
double norm1 = cv::norm(xy1 - xy);
Mat xy2 = tformfwd(trans2, uv);
double norm2 = cv::norm(xy2 - xy);
Mat trans;
if (norm1 < norm2){
trans = trans1;
}
else {
trans = trans2;
}
return trans;
}
Mat get_similarity_transform(const vector<Point2f>& src_points, const vector<Point2f>& dst_points, bool reflective = true)
{
Mat trans;
Mat src((int)src_points.size(), 2, CV_32FC1, (void*)(&src_points[0].x));
src.convertTo(src, CV_64FC1);
Mat dst((int)dst_points.size(), 2, CV_32FC1, (void*)(&dst_points[0].x));
dst.convertTo(dst, CV_64FC1);
if (reflective){
trans = find_similarity(src, dst);
}
else {
trans = find_none_flectives_similarity(src, dst);
}
Mat trans_cv2 = trans(Rect(0, 0, 2, trans.rows)).t();
return trans_cv2;
}
Mat align_face(const cv::Mat& src, const double* landmark)
{
vector<Point2f> detect_points;
for (int i = 0; i < 5; i++)
{
detect_points.push_back(Point2f(landmark[i], landmark[i + 5]));
}
vector<Point2f> reference_points;
reference_points.push_back(cv::Point2f(30.2946, 51.6963 - 25));
reference_points.push_back(cv::Point2f(65.5318, 51.5014 - 25));
reference_points.push_back(cv::Point2f(48.0252, 71.7366));
reference_points.push_back(cv::Point2f(33.5493, 92.3615));
reference_points.push_back(cv::Point2f(62.7299, 92.2041));
Mat tfm = get_similarity_transform(detect_points, reference_points);
Mat aligned_face;
warpAffine(src, aligned_face, tfm, m_cropimg_size);
return aligned_face;
}
Mat align_face(const cv::Mat& src, const double* landmark, int ptCount, int lossindex)
{
std::vector<Point2f> detect_points;
for (int i = 0; i < ptCount; i++)
{
detect_points.push_back(Point2f(landmark[i], landmark[i + ptCount]));
}
std::vector<Point2f> reference_points;
for (int i = 0, n = 0; i < ptCount; n++, i++)
{
if (n == lossindex)
n++;
reference_points.push_back(cv::Point2f(g_meanfacelm2[2 * n], g_meanfacelm2[2 * n + 1]));
}
Mat tfm = get_similarity_transform(detect_points, reference_points);
Mat aligned_face;
warpAffine(src, aligned_face, tfm, m_cropimg_size);
return aligned_face;
}
Mat align_face2(const cv::Mat& src, const double* landmark, int ptCount, int lossindex)
{
std::vector<Point2f> detect_points;
for (int i = 0; i < ptCount; i++)
{
detect_points.push_back(Point2f(landmark[i * 2], landmark[i * 2 + 1]));
}
std::vector<Point2f> reference_points;
for (int i = 0, n = 0; i < ptCount; n++, i++)
{
if (n == lossindex)
n++;
reference_points.push_back(cv::Point2f(g_meanfacelm2[2 * n], g_meanfacelm2[2 * n + 1]));
}
Mat tfm = get_similarity_transform(detect_points, reference_points);
Mat aligned_face;
warpAffine(src, aligned_face, tfm, m_cropimg_size);
return aligned_face;
}
Mat align_face224(const cv::Mat& src, const double* landmark, int ptCount, int lossindex)
{
std::vector<Point2f> detect_points;
for (int i = 0; i < ptCount; i++)
{
detect_points.push_back(Point2f(landmark[i * 2], landmark[i * 2 + 1]));
}
std::vector<Point2f> reference_points;
for (int i = 0, n = 0; i < ptCount; n++, i++)
{
if (n == lossindex)
n++;
reference_points.push_back(cv::Point2f(g_meanfacelm2[2 * n] * 2, g_meanfacelm2[2 * n + 1] * 2));
}
Mat tfm = get_similarity_transform(detect_points, reference_points);
Mat aligned_face;
warpAffine(src, aligned_face, tfm, m_cropimg_size * 2);
return aligned_face;
}
template<typename Ty>
void savedata(const char* filename, Ty *data,int num,int dim)
{
ofstream ofile; //定义输出文件
ofile.open(filename, ios::out); //作为输出文件打开
for(int i =0;i<num;i++)
{ for(int j =0;j<dim;j++)
ofile << data[i+j*num] << " ";
ofile<<endl;
}
ofile.close();
}
Mat procrustes(double *meanfacelm3, double *facelm3, int keycount, double *data, int nPtCount, int dim = 3)
{
Mat meanface(keycount, 1, CV_64FC3, meanfacelm3);
meanface = meanface.t();
Mat face(keycount, 1, CV_64FC3, facelm3);
face = face.t();
Scalar mu_x = cv::mean(meanface);
Mat tempmeanface = meanface - Mat(meanface.size(), meanface.type(), mu_x);
double normX = cv::norm(tempmeanface);
tempmeanface = tempmeanface / normX;
tempmeanface = tempmeanface.reshape(1, keycount);
Scalar mu_y = cv::mean(face);
Mat tempface = face - Mat(face.size(), face.type(), mu_y);
double normY = cv::norm(tempface);
tempface = tempface / normY;
tempface = tempface.reshape(1, keycount);
Mat A = tempmeanface.t()*tempface;
Mat w, u, vt;
SVDecomp(A, w, u, vt);
Mat T = vt.t()*u.t();
double traceTA = cv::sum(w)[0];
double b = traceTA * normX / normY;
double d = 1 - traceTA *traceTA;
Mat muY = Mat(Size(3, 1), CV_64FC3, mu_y);
muY = muY.reshape(1, 3);
Mat muX = Mat(Size(3, 1), CV_64FC3, mu_x);
muX = muX.reshape(1, 3);
Mat c = muX - b*muY*T;
Mat trans = -1 / b *c*T.t();
Mat transM = Mat::zeros(4, 4, CV_64FC1);
for (int i = 0; i < 3; i++)
{
for (int j = 0; j < 3; j++)
{
transM.at<double>(i, j) = 1.0 / b * T.at<double>(i, j);
}
}
transM.at<double>(0, 3) = trans.at<double>(0, 0);
transM.at<double>(1, 3) = trans.at<double>(0, 1);
transM.at<doubl
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【资源说明】 基于C++实现的三维点云的深度投影和纹理投影源码.zip基于C++实现的三维点云的深度投影和纹理投影源码.zip基于C++实现的三维点云的深度投影和纹理投影源码.zip基于C++实现的三维点云的深度投影和纹理投影源码.zip基于C++实现的三维点云的深度投影和纹理投影源码.zip基于C++实现的三维点云的深度投影和纹理投影源码.zip基于C++实现的三维点云的深度投影和纹理投影源码.zip基于C++实现的三维点云的深度投影和纹理投影源码.zip基于C++实现的三维点云的深度投影和纹理投影源码.zip基于C++实现的三维点云的深度投影和纹理投影源码.zip 【备注】 1、该资源内项目代码都经过测试运行成功,功能ok的情况下才上传的,请放心下载使用! 2、本项目适合计算机相关专业(如计科、人工智能、通信工程、自动化、电子信息等)的在校学生、老师或者企业员工下载使用,也适合小白学习进阶,当然也可作为毕设项目、课程设计、作业、项目初期立项演示等。 3、如果基础还行,也可在此代码基础上进行修改,以实现其他功能,也可直接用于毕设、课设、作业等。 欢迎下载,沟通交流,互相学习,共同进步!
资源推荐
资源详情
资源评论
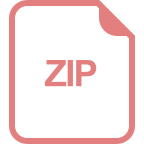
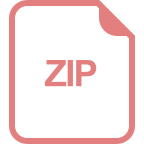
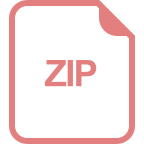
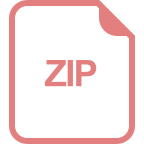
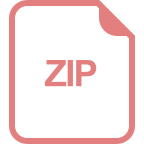
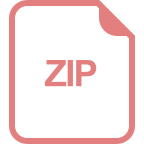
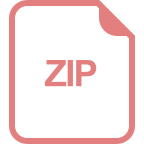
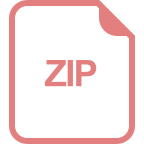
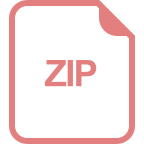
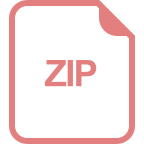
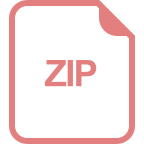
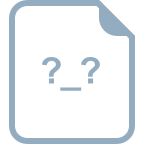
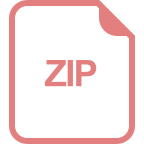
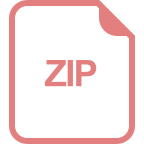
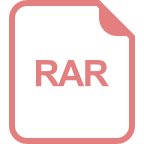
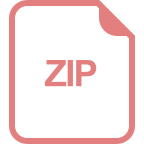
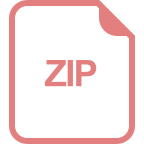
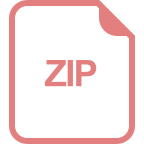
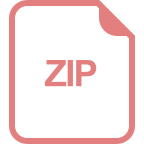
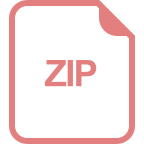
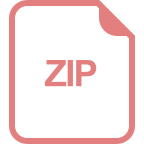
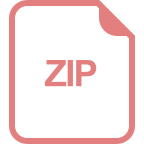
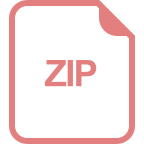
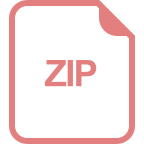
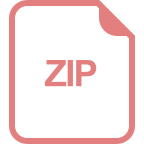
收起资源包目录

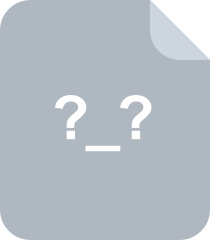
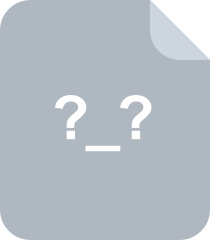
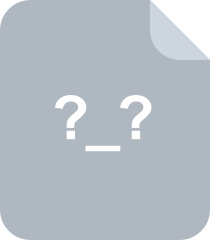
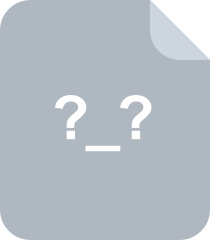
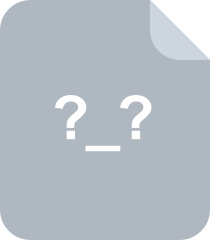
共 5 条
- 1
资源评论
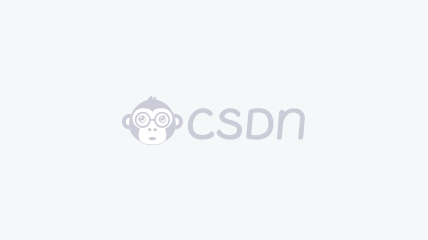
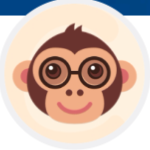
onnx
- 粉丝: 9544
- 资源: 5595
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

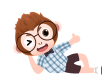
最新资源
- CLShanYanSDKDataList.sqlite
- C#ASP.NET销售管理系统源码数据库 SQL2008源码类型 WebForm
- 1111232132132132
- 基于MAPPO算法与DL优化预编码的多用户MISO通信系统双时间尺度传输方案设计源码
- 基于微信拍照功能的ohos开源CameraView控件设计源码
- 基于JavaCV的RTSP转HTTP-FLV流媒体服务设计源码
- 基于Python的西北工业大学MobilePhone软件开发项目设计源码
- 基于Java语言实现的LeetCode-hot100题库精选设计源码
- 基于ThinkPHP5.0的壹凯巴cms设计源码,适用于小型企业建站灵活组装开发
- C#ASP.NET酒店管理系统源码(WPF)数据库 Access源码类型 WinForm
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


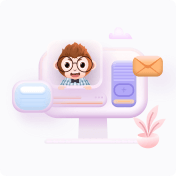
安全验证
文档复制为VIP权益,开通VIP直接复制
