# -*- coding: utf-8 -*-
"""
This module implements community detection.
"""
from __future__ import print_function
import array
import random
import networkx as nx
from community_status import Status
__author__ = """Thomas Aynaud (thomas.aynaud@lip6.fr)"""
# Copyright (C) 2009 by
# Thomas Aynaud <thomas.aynaud@lip6.fr>
# All rights reserved.
# BSD license.
__PASS_MAX = -1
__MIN = 0.0000001
def partition_at_level(dendrogram, level):
"""Return the partition of the nodes at the given level
A dendrogram is a tree and each level is a partition of the graph nodes.
Level 0 is the first partition, which contains the smallest communities,
and the best is len(dendrogram) - 1.
The higher the level is, the bigger are the communities
Parameters
----------
dendrogram : list of dict
a list of partitions, ie dictionnaries where keys of the i+1 are the
values of the i.
level : int
the level which belongs to [0..len(dendrogram)-1]
Returns
-------
partition : dictionnary
A dictionary where keys are the nodes and the values are the set it
belongs to
Raises
------
KeyError
If the dendrogram is not well formed or the level is too high
See Also
--------
best_partition which directly combines partition_at_level and
generate_dendrogram to obtain the partition of highest modularity
Examples
--------
>>> G=nx.erdos_renyi_graph(100, 0.01)
>>> dendrogram = generate_dendrogram(G)
>>> for level in range(len(dendrogram) - 1) :
>>> print("partition at level", level, "is", partition_at_level(dendrogram, level)) # NOQA
"""
partition = dendrogram[0].copy()
for index in range(1, level + 1):
for node, community in partition.items():
partition[node] = dendrogram[index][community]
return partition
def modularity(partition, graph, weight='weight'):
"""Compute the modularity of a partition of a graph
Parameters
----------
partition : dict
the partition of the nodes, i.e a dictionary where keys are their nodes
and values the communities
graph : networkx.Graph
the networkx graph which is decomposed
weight : str, optional
the key in graph to use as weight. Default to 'weight'
Returns
-------
modularity : float
The modularity
Raises
------
KeyError
If the partition is not a partition of all graph nodes
ValueError
If the graph has no link
TypeError
If graph is not a networkx.Graph
References
----------
.. 1. Newman, M.E.J. & Girvan, M. Finding and evaluating community
structure in networks. Physical Review E 69, 26113(2004).
Examples
--------
>>> G=nx.erdos_renyi_graph(100, 0.01)
>>> part = best_partition(G)
>>> modularity(part, G)
"""
if graph.is_directed():
raise TypeError("Bad graph type, use only non directed graph")
inc = dict([])
deg = dict([])
links = graph.size(weight=weight)
if links == 0:
raise ValueError("A graph without link has an undefined modularity")
for node in graph:
com = partition[node]
deg[com] = deg.get(com, 0.) + graph.degree(node, weight=weight)
for neighbor, datas in graph[node].items():
edge_weight = datas.get(weight, 1)
if partition[neighbor] == com:
if neighbor == node:
inc[com] = inc.get(com, 0.) + float(edge_weight)
else:
inc[com] = inc.get(com, 0.) + float(edge_weight) / 2.
res = 0.
for com in set(partition.values()):
res += (inc.get(com, 0.) / links) - \
(deg.get(com, 0.) / (2. * links)) ** 2
return res
def best_partition(graph, partition=None,
weight='weight', resolution=1., randomize=False):
"""Compute the partition of the graph nodes which maximises the modularity
(or try..) using the Louvain heuristices
This is the partition of highest modularity, i.e. the highest partition
of the dendrogram generated by the Louvain algorithm.
Parameters
----------
graph : networkx.Graph
the networkx graph which is decomposed
partition : dict, optional
the algorithm will start using this partition of the nodes.
It's a dictionary where keys are their nodes and values the communities
weight : str, optional
the key in graph to use as weight. Default to 'weight'
resolution : double, optional
Will change the size of the communities, default to 1.
represents the time described in
"Laplacian Dynamics and Multiscale Modular Structure in Networks",
R. Lambiotte, J.-C. Delvenne, M. Barahona
randomize : boolean, optional
Will randomize the node evaluation order and the community evaluation
order to get different partitions at each call
Returns
-------
partition : dictionnary
The partition, with communities numbered from 0 to number of communities
Raises
------
NetworkXError
If the graph is not Eulerian.
See Also
--------
generate_dendrogram to obtain all the decompositions levels
Notes
-----
Uses Louvain algorithm
References
----------
.. 1. Blondel, V.D. et al. Fast unfolding of communities in
large networks. J. Stat. Mech 10008, 1-12(2008).
Examples
--------
>>> #Basic usage
>>> G=nx.erdos_renyi_graph(100, 0.01)
>>> part = best_partition(G)
>>> #other example to display a graph with its community :
>>> #better with karate_graph() as defined in networkx examples
>>> #erdos renyi don't have true community structure
>>> G = nx.erdos_renyi_graph(30, 0.05)
>>> #first compute the best partition
>>> partition = community.best_partition(G)
>>> #drawing
>>> size = float(len(set(partition.values())))
>>> pos = nx.spring_layout(G)
>>> count = 0.
>>> for com in set(partition.values()) :
>>> count += 1.
>>> list_nodes = [nodes for nodes in partition.keys()
>>> if partition[nodes] == com]
>>> nx.draw_networkx_nodes(G, pos, list_nodes, node_size = 20,
node_color = str(count / size))
>>> nx.draw_networkx_edges(G, pos, alpha=0.5)
>>> plt.show()
"""
dendo = generate_dendrogram(graph,
partition,
weight,
resolution,
randomize)
return partition_at_level(dendo, len(dendo) - 1)
def generate_dendrogram(graph,
part_init=None,
weight='weight',
resolution=1.,
randomize=False):
"""Find communities in the graph and return the associated dendrogram
A dendrogram is a tree and each level is a partition of the graph nodes.
Level 0 is the first partition, which contains the smallest communities,
and the best is len(dendrogram) - 1. The higher the level is, the bigger
are the communities
Parameters
----------
graph : networkx.Graph
the networkx graph which will be decomposed
part_init : dict, optional
the algorithm will start using this partition of the nodes. It's a
dictionary where keys are their nodes and values the communities
weight : str, optional
the key in graph to use as weight. Default to 'weight'
resolution : double, optional
Will change the size of the communities, default to 1.
represents the time described in
"Laplacian Dynamics and Multiscale Modular Structure in Networks",
R. Lambiotte, J.-C. Delvenne, M. Barahona
Returns
-------
dendrogram : list of dictionaries
a list of partitions, ie di
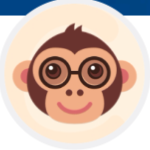
onnx
- 粉丝: 9971
- 资源: 5626
最新资源
- YOLO算法-废物分类数据集-410张图像带标签-瓶子.zip
- YOLO算法-车辆数据集-230张图像带标签-奔驰.zip
- YOLO算法-刀数据集-400张图像带标签-刀.zip
- YOLO算法-列车检测数据集-191张图像带标签-火车.zip
- YOLO算法-易拉罐识别数据集-512张图像带标签-可口可乐.zip
- YOLO算法-水泥路面裂纹检测数据集-213张图像带标签-裂纹.zip
- YOLO算法-道路裂纹数据集-139张图像带标签-裂纹.zip
- YOLO算法-下水道缺陷数据集-2364张图像带标签-关节偏移-障碍物-裂纹-带扣-洞-公用设施入侵-碎片.zip
- YOLO算法-刀具数据数据集-168张图像带标签-刀.zip
- YOLO算法-刀数据集-198张图像带标签-刀-枪.zip
- YOLO算法-检测驾驶员侧车窗是否关闭数据集-85张图像带标签-汽车车窗-汽车.zip
- YOLO算法-树数据集-75张图像带标签-树.zip
- YOLO算法-刀具检测数据集-61张图像带标签-.zip
- YOLO算法-汽车数据集-120张图像带标签-汽车.zip
- YOLO算法-工作场所安全隐患数据集-60张图像带标签-倒下的工人-配备个人防护装备的工人-无个人防护装备的工人-火.zip
- YOLO算法-水泥路面裂纹数据集-42张图像带标签-裂纹.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


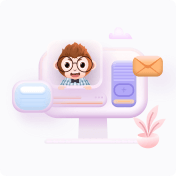
- 1
- 2
前往页