package org.csu.mypetstore.controller;
import org.csu.mypetstore.domain.Account;
import org.csu.mypetstore.service.AccountService;
import org.csu.mypetstore.service.AdminService;
import org.csu.mypetstore.service.InventoryService;
import org.csu.mypetstore.service.VerificationService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.bind.support.SessionStatus;
import javax.servlet.http.HttpSession;
@Controller
@RequestMapping("/account")
@SessionAttributes({"username","account", "admin"})
public class AccountController {
@Autowired
AccountService accountService;
@Autowired
VerificationService verificationService;
@Autowired
AdminService adminService;
@Autowired
InventoryService inventoryService;
//登录或修改用户信息的验证码
@GetMapping("/getVerification")
@ResponseBody
public String getVerification(Account account, Model model){
if(accountService.getAccount(account.getUsername())==null){
String message_ver = "用户名不存在";
model.addAttribute("message_ver",message_ver);
return message_ver;
}else{
verificationService.creatVerificationCodeByUsername(account.getUsername());
String email = accountService.getAccount(account.getUsername()).getEmail();
email = email.replace(email.substring(2,email.indexOf('@')-2),"*******");
String message_ver = "已向 "+email+" 发送验证码";
model.addAttribute("message_ver",message_ver);
return message_ver;
}
}
//注册时的验证码
@PostMapping("/getVerificationSignOn")
@ResponseBody
public String getVerificationSignOn(Account account,Model model){
if(account.getUsername().equals("")||account.getUsername()==null||account.getEmail().equals("")||account.getEmail()==null){
String message_ver_sign = "缺少必要信息,请将注册信息填写完整";
model.addAttribute("message_ver_sign",message_ver_sign);
return message_ver_sign;
}else{
verificationService.creatVerificationCode(account);
String email = account.getEmail();
email = email.replace(email.substring(2,email.indexOf('@')-1),"*******");
String message_ver_sign = "已向 "+email+" 发送验证码";
model.addAttribute("message_ver_sign",message_ver_sign);
return message_ver_sign;
}
}
@GetMapping("/signon")
@ResponseBody
public String signon(Account account,String verification,String Fruit, Model model) {
if(Fruit.equals("User")){
if(verificationService.getVerificationCodeByUsername(account.getUsername())==null){//判断是否已经获得验证码
String message_login = "请先获得验证码";
model.addAttribute("message_login",message_login);
return "account/login";
}else if(!verificationService.getVerificationCodeByUsername(account.getUsername()).getCode().equals(verification)){//判断验证码是否正确
String message_login = "验证码错误";
model.addAttribute("message_login",message_login);
return "account/login";
}else{//判断用户名密码是否正确
verificationService.delVerificationCodeByUsername(account.getUsername());
if(accountService.getAccount(account.getUsername(), account.getPassword()) == null) {
String message_login = "用户名或密码错误";
model.addAttribute("message_login",message_login);
return "account/login";
}
else {
model.addAttribute("account",accountService.getAccount(account.getUsername(), account.getPassword()));
model.addAttribute("username",account.getUsername());
return "catalog/Main";
}
}
}else{
if(adminService.getAdmin(account.getUsername(),account.getPassword())!=null){
model.addAttribute("admin",adminService.getAdmin(account.getUsername(),account.getPassword()));
int[] BIRDS = {inventoryService.getSoldOutNumByByCategoryName("BIRDS"), inventoryService.getUnsoldNumByCategoryName("BIRDS"), inventoryService.getOrderedNumByCategoryName("BIRDS")};
int[] CATS = {inventoryService.getSoldOutNumByByCategoryName("CATS"), inventoryService.getUnsoldNumByCategoryName("CATS"), inventoryService.getOrderedNumByCategoryName("CATS")};
int[] DOGS = {inventoryService.getSoldOutNumByByCategoryName("DOGS"), inventoryService.getUnsoldNumByCategoryName("DOGS"), inventoryService.getOrderedNumByCategoryName("DOGS")};
int[] FISH = {inventoryService.getSoldOutNumByByCategoryName("FISH"), inventoryService.getUnsoldNumByCategoryName("FISH"), inventoryService.getOrderedNumByCategoryName("FISH")};
int[] REPTILES = {inventoryService.getSoldOutNumByByCategoryName("REPTILES"), inventoryService.getUnsoldNumByCategoryName("REPTILES"), inventoryService.getOrderedNumByCategoryName("REPTILES")};
model.addAttribute("BirdList", BIRDS);
model.addAttribute("CatList", CATS);
model.addAttribute("DogList", DOGS);
model.addAttribute("FishList", FISH);
model.addAttribute("ReptileList", REPTILES);
return "usual/index";
}else{
String message_login = "管理员用户名或密码错误";
model.addAttribute("message_login",message_login);
return "login";
}
}
}
@PostMapping("/newAccount")
public String newAccount(Account account,String verification,Model model){
if(account.getPassword()!=""||account.getPassword()!=null||account.getUsername()!=""||account.getUsername()!=null||account.getEmail()!=""||account.getEmail()!=null){
if(!verificationService.getVerificationCodeByUsername(account.getUsername()).getCode().equals(verification)){
model.addAttribute("message_new","验证码错误");
return "验证码错误";
}else{
account.setLanguagePreference("english");
account.setListOption(false);
account.setBannerOption(false);
account.setFavouriteCategoryId("CATS");
accountService.insertAccount(account);
model.addAttribute("message_new","注册用户成功");
verificationService.delVerificationCodeByUsername(account.getUsername());
return "注册用户成功";
}
}else{
model.addAttribute("message_new","注册信息不完整");
return "注册用户成功";
}
}
@PostMapping("/checkAccount")
@ResponseBody
public String checkAccount(Account account){
if(accountService.getAccount(account.getUsername())!=null){
return "用户名已存在";
}else{
return "用户名可以使用";
}
}
//获得用户信息
@GetMapping("/getAccount")
public String getAccount(Model model, HttpSession session){
// if(session.getAttribute("account")!=null){
model.addAttribute("account",session.getAttribute("account"));
return "account/EditAccountForm";
// }else{
// return "account/login";
// }
}
//获得用户信息
@GetMapping("/signout")
public String signout(HttpSession session,SessionStatus sessionStatus){
session.invalidate();
sessionStatus.setComplete();
return "catalog/Main";
}
//�
没有合适的资源?快使用搜索试试~ 我知道了~
基于Springboot+Mybatis实现的宠物商店项目源码+数据库(课程设计).zip
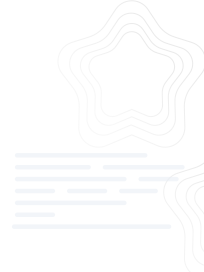
共223个文件
java:49个
gif:38个
mtn:31个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉

温馨提示
基于Springboot+Mybatis实现的宠物商店项目源码+数据库(课程设计).zip Springboot+Mybatis 宠物商店项目源码 +带有数据库 【备注】 主要针对计算机相关专业的正在做毕设的学生和需要项目实战的Java学习者。 也可作为课程设计、期末大作业。包含:项目源码、数据库脚本、项目说明等,该项目可以直接作为毕设使用。 也可以用来学习参考借鉴!
资源推荐
资源详情
资源评论
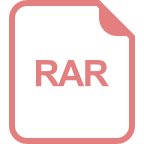
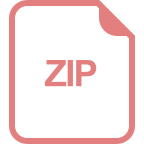
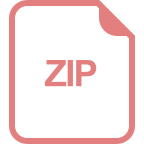
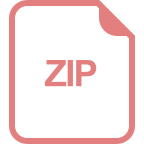
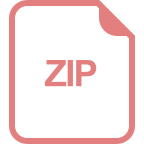
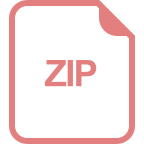
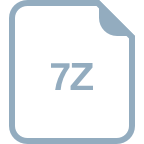
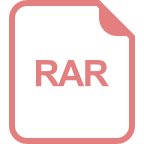
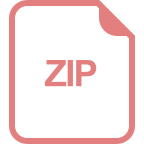
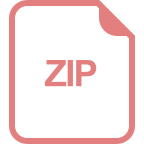
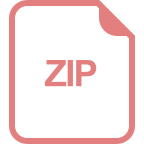
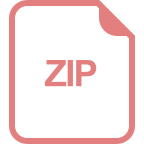
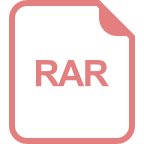
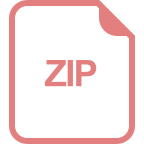
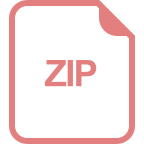
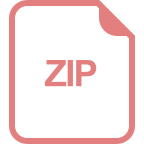
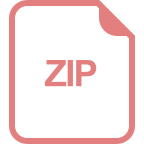
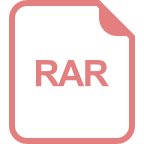
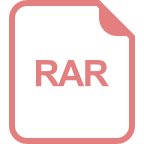
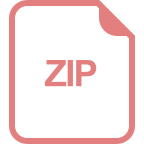
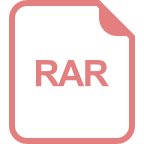
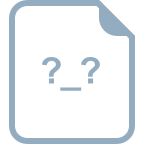
收起资源包目录

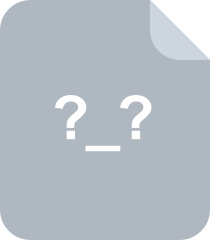
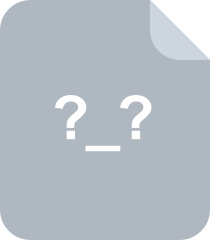
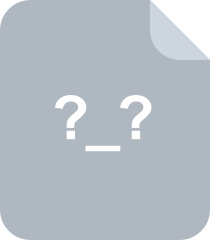
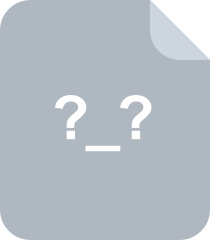
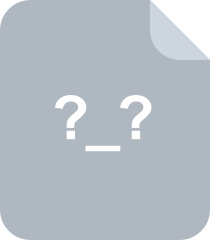
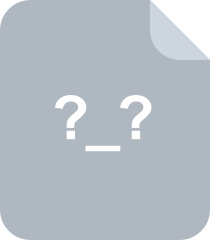
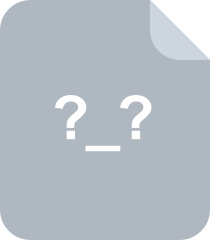
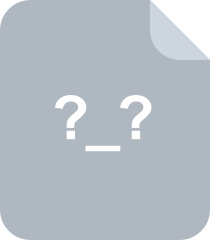
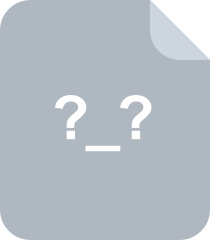
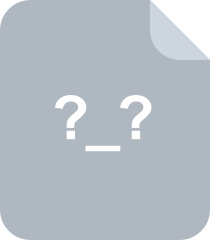
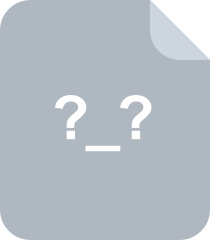
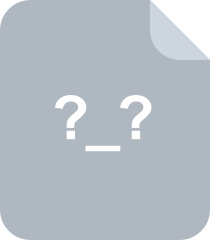
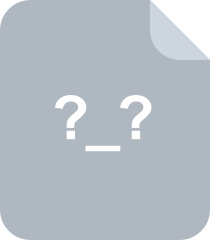
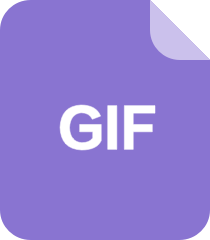
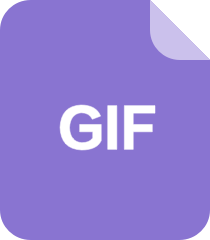
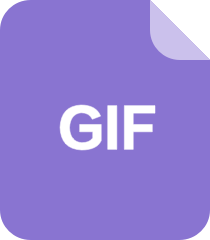
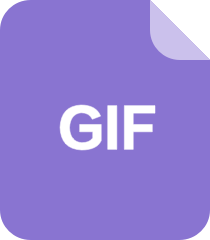
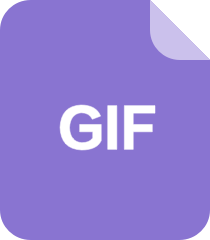
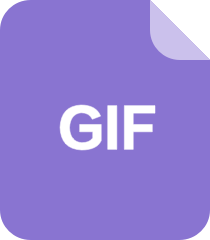
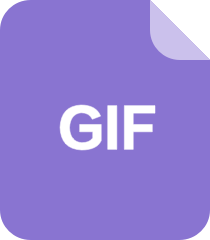
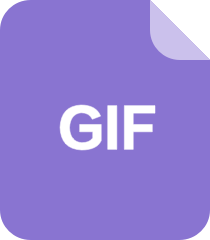
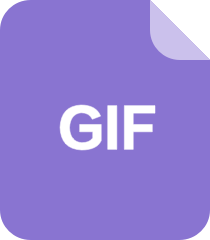
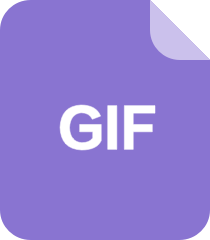
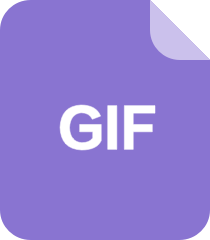
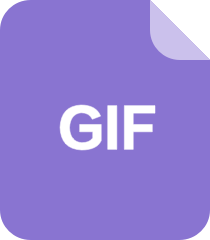
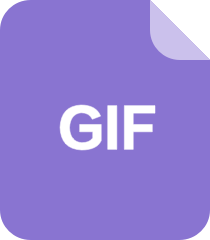
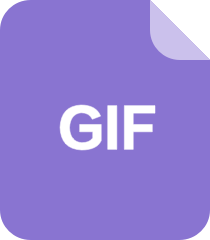
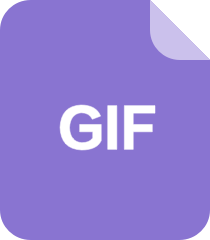
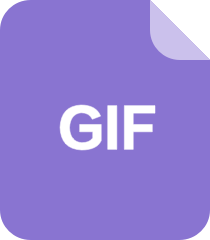
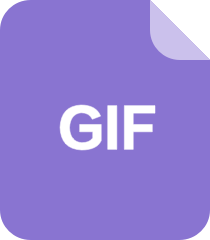
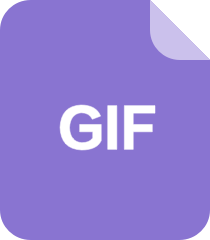
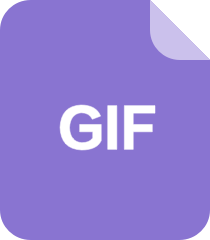
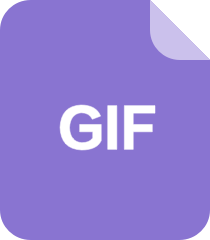
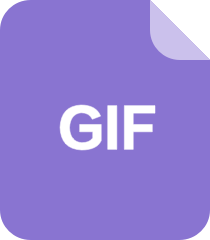
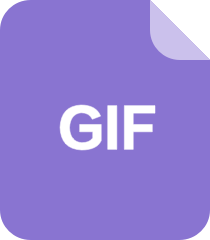
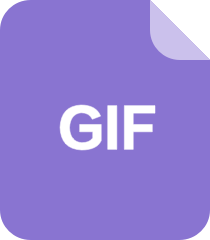
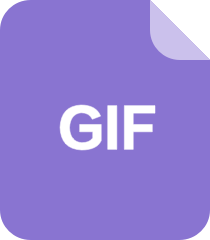
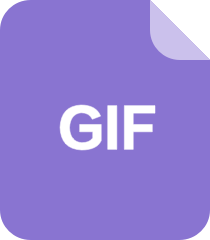
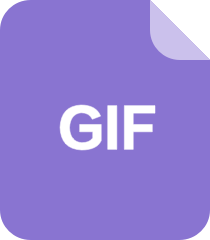
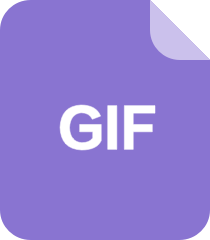
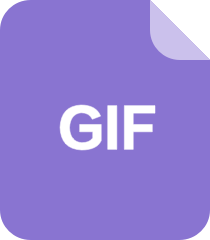
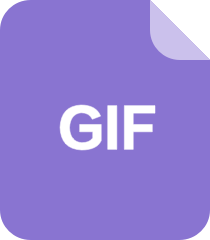
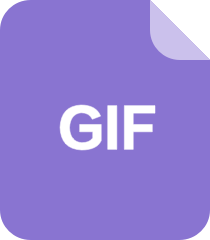
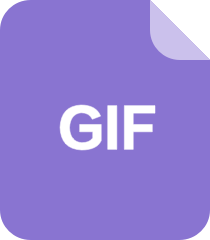
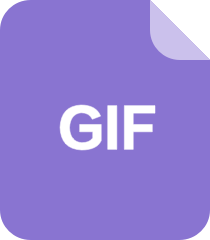
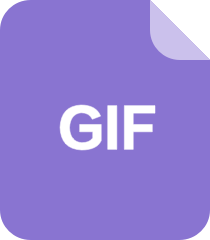
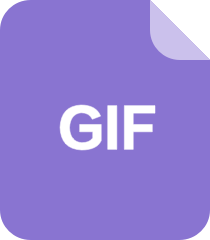
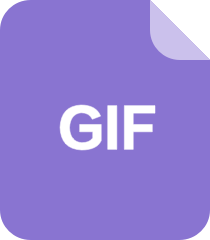
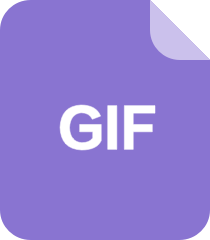
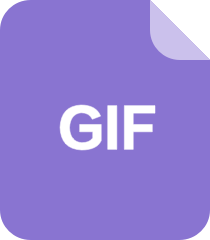
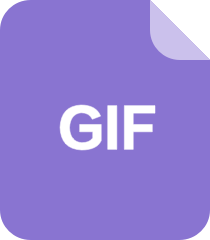
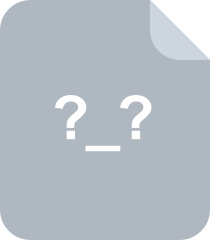
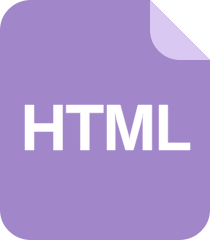
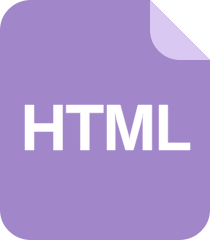
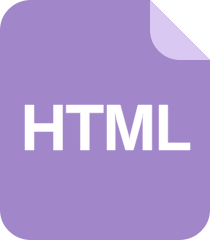
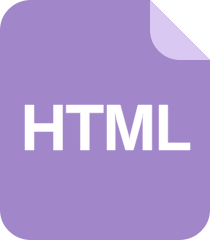
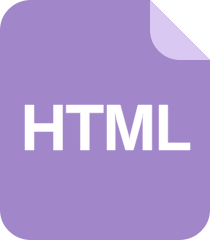
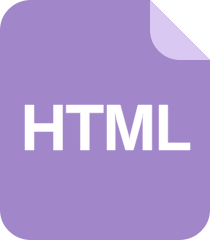
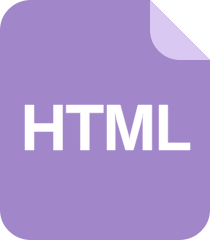
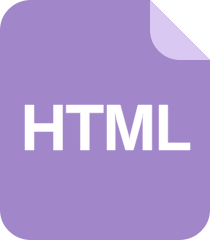
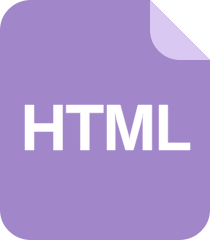
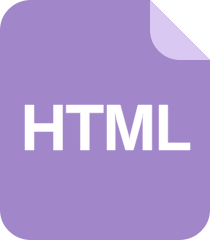
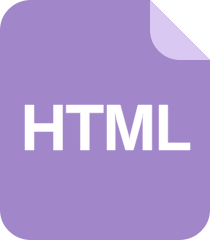
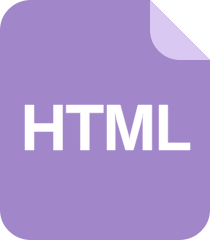
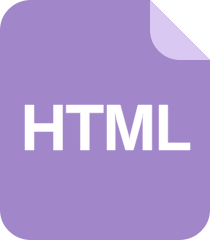
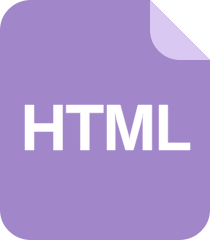
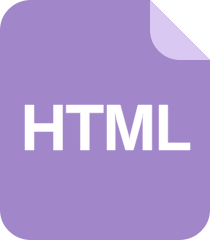
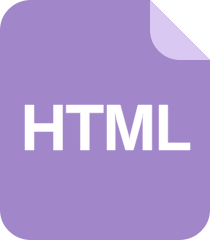
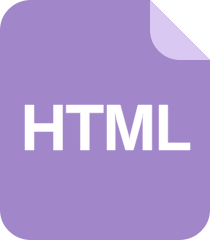
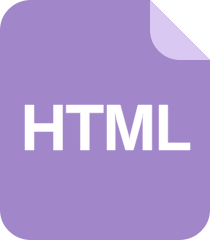
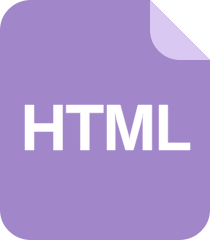
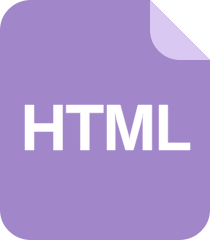
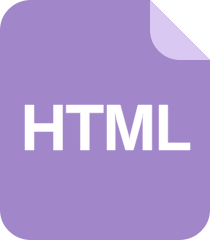
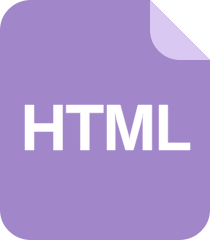
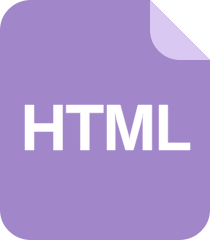
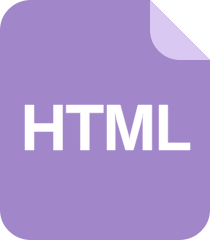
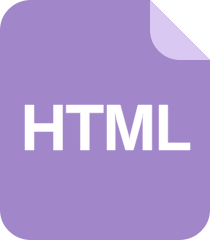
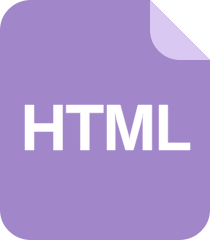
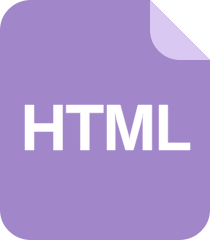
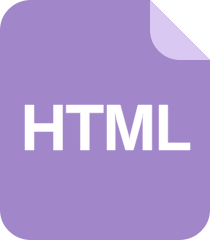
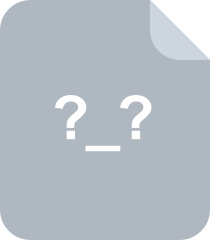
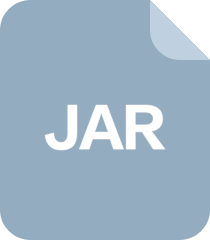
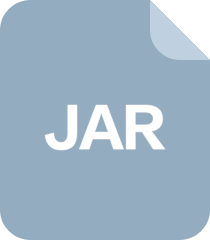
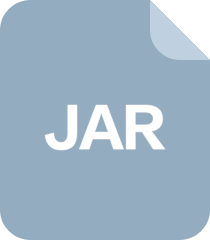
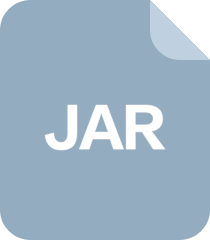
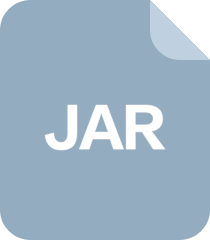
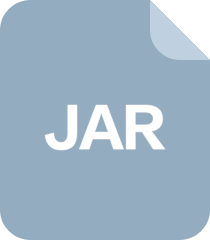
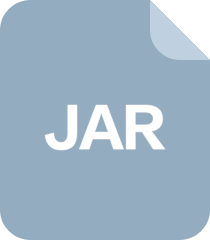
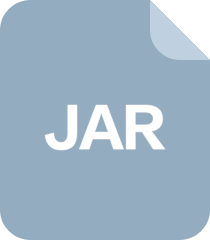
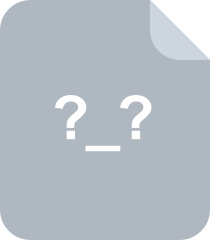
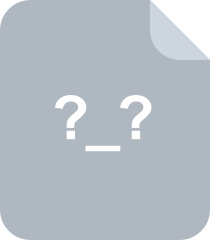
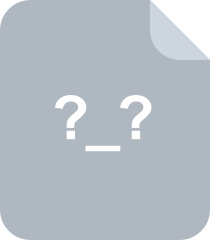
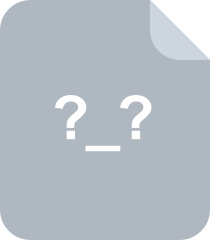
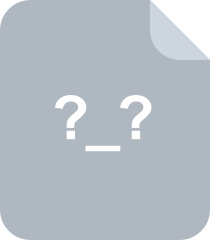
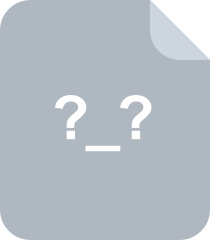
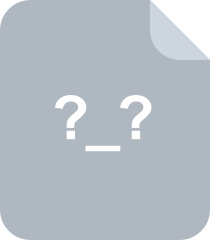
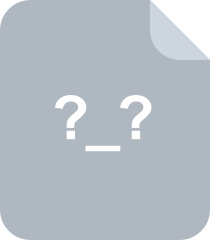
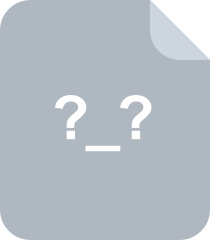
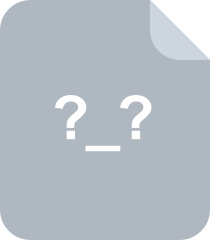
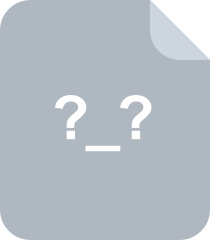
共 223 条
- 1
- 2
- 3
资源评论
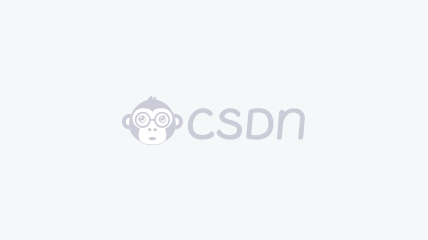
- xuzhi1242024-01-30资源内容详尽,对我有使用价值,谢谢资源主的分享。
- ddserbupt2023-01-03资源内容详细,总结地很全面,与描述的内容一致,对我启发很大,学习了。onnx2024-01-23感谢认可和支持,加油
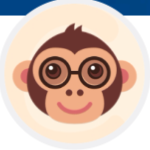
onnx
- 粉丝: 9679
- 资源: 5598
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

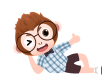
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


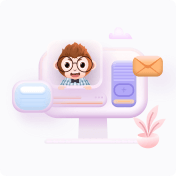
安全验证
文档复制为VIP权益,开通VIP直接复制
