/**
******************************************************************************
* @file mcb_1sf_driver.c
* @author iC-Haus GmbH
* @version 1.1.0
* @note Designed according to iC-MCB datasheet release B1 for chip revision Z.
******************************************************************************
* @attention
*
* Software and its documentation is provided by iC-Haus GmbH or contributors "AS IS" and is
* subject to the ZVEI General Conditions for the Supply of Products and Services with iC-Haus
* amendments and the ZVEI Software clause with iC-Haus amendments (http://www.ichaus.de/EULA).
*
******************************************************************************
*/
#include "mcb_1sf_driver.h"
#include "main.h"
#include "spi.h"
#include "bsp_spi.h"
extern DMA_HandleTypeDef hdma_spi2_rx;
extern DMA_HandleTypeDef hdma_spi2_tx;
/* iC-MCB opcodes */
enum MCB_OPCODES {
MCB_OPCODE_TRANSMIT_SDAD = 0xA6,
MCB_OPCODE_READ_REGISTER = 0x81,
MCB_OPCODE_WRITE_REGISTER = 0xCF,
MCB_OPCODE_SENSOR_FEEDTHROUGH = 0xE3,
};
/* iC-MCB parameters */
const struct mcb_param MCB_DLEN1 = { .addr = 0x40, .pos = 5, .len = 6 };
const struct mcb_param MCB_ENDC1 = { .addr = 0x40, .pos = 6, .len = 1 };
const struct mcb_param MCB_GRAY1 = { .addr = 0x40, .pos = 7, .len = 1 };
const struct mcb_param MCB_CPOLY1 = { .addr = 0x41, .pos = 1, .len = 2 };
const struct mcb_param MCB_CSTART1 = { .addr = 0x41, .pos = 7, .len = 6 };
const struct mcb_param MCB_DLEN2 = { .addr = 0x42, .pos = 5, .len = 6 };
const struct mcb_param MCB_ENDC2 = { .addr = 0x42, .pos = 6, .len = 1 };
const struct mcb_param MCB_CPOLY2 = { .addr = 0x43, .pos = 1, .len = 2 };
const struct mcb_param MCB_CSTART2 = { .addr = 0x43, .pos = 7, .len = 6 };
const struct mcb_param MCB_BUSY = { .addr = 0x44, .pos = 7, .len = 8 };
const struct mcb_param MCB_REGPROT = { .addr = 0x45, .pos = 0, .len = 1 };
const struct mcb_param MCB_NTOA = { .addr = 0x45, .pos = 1, .len = 1 };
const struct mcb_param MCB_TOS = { .addr = 0x45, .pos = 2, .len = 1 };
const struct mcb_param MCB_ASID = { .addr = 0x45, .pos = 3, .len = 1 };
const struct mcb_param MCB_CMD01DI = { .addr = 0x45, .pos = 4, .len = 1 };
const struct mcb_param MCB_CMD2EN = { .addr = 0x45, .pos = 5, .len = 1 };
const struct mcb_param MCB_ENSSI = { .addr = 0x45, .pos = 6, .len = 1 };
const struct mcb_param MCB_RSSI = { .addr = 0x45, .pos = 7, .len = 1 };
const struct mcb_param MCB_ACQMODE = { .addr = 0x46, .pos = 0, .len = 1 };
const struct mcb_param MCB_BANKSW = { .addr = 0x46, .pos = 1, .len = 1 };
const struct mcb_param MCB_USDST = { .addr = 0x46, .pos = 2, .len = 1 };
const struct mcb_param MCB_DLFSI = { .addr = 0x47, .pos = 5, .len = 6 };
const struct mcb_param MCB_ENFSI = { .addr = 0x47, .pos = 6, .len = 1 };
const struct mcb_param MCB_HEADL = { .addr = 0x48, .pos = 3, .len = 4 };
const struct mcb_param MCB_STAFSI = { .addr = 0x48, .pos = 5, .len = 2 };
const struct mcb_param MCB_IDLE = { .addr = 0x48, .pos = 6, .len = 1 };
const struct mcb_param MCB_CPOL = { .addr = 0x49, .pos = 0, .len = 1 };
const struct mcb_param MCB_CPHA = { .addr = 0x49, .pos = 1, .len = 1 };
const struct mcb_param MCB_REQ_FT = { .addr = 0x49, .pos = 2, .len = 1 };
const struct mcb_param MCB_G2B = { .addr = 0x49, .pos = 3, .len = 1 };
const struct mcb_param MCB_CLKDIV = { .addr = 0x49, .pos = 7, .len = 4 };
const struct mcb_param MCB_HEADER = { .addr = 0x4A, .pos = 7, .len = 8 };
const struct mcb_param MCB_CB_FSI = { .addr = 0x4C, .pos = 2, .len = 3 };
const struct mcb_param MCB_CB_CLK = { .addr = 0x4C, .pos = 3, .len = 1 };
const struct mcb_param MCB_CB_IRQ = { .addr = 0x4C, .pos = 4, .len = 1 };
const struct mcb_param MCB_CB_MAO = { .addr = 0x4C, .pos = 5, .len = 1 };
const struct mcb_param MCB_CB_SLI = { .addr = 0x4C, .pos = 6, .len = 1 };
const struct mcb_param MCB_CB_SLO = { .addr = 0x4C, .pos = 7, .len = 1 };
const struct mcb_param MCB_CHPREL = { .addr = 0x4D, .pos = 3, .len = 4 };
const struct mcb_param MCB_ESE = { .addr = 0x4D, .pos = 5, .len = 1 };
const struct mcb_param MCB_CFGOK = { .addr = 0x4D, .pos = 7, .len = 1 };
const struct mcb_param MCB_RDATA = { .addr = 0x4E, .pos = 7, .len = 8 };
const struct mcb_param MCB_CVALID = { .addr = 0x4F, .pos = 2, .len = 3 };
const struct mcb_param MCB_IVALID = { .addr = 0x4F, .pos = 3, .len = 1 };
const struct mcb_param MCB_CONFIRM = { .addr = 0x4F, .pos = 4, .len = 1 };
/* globals */
/**
* @brief This function transmits sensor data in the configured format.
*
* @param data_tx is a pointer to a buffer the transmitted actuator data is stored in.
* @param data_rx is a pointer to a buffer the received sensor data is written to.
* @param datasize is the number of bytes required to transmit the configured format.
* @param status is a pointer to a byte the received STATUS byte is written to.
* @retval None
*/
void mcb_transmit_SDAD(const uint8_t *data_tx, uint8_t *data_rx, uint8_t datasize, uint8_t *status) {
static uint8_t buf_tx[0xFF + 3]; // Dimensioning according to max. datasize + 3 byte header
static uint8_t buf_rx[0xFF + 3];
static uint16_t bufsize;
bufsize = datasize + 1;
buf_tx[0] = MCB_OPCODE_TRANSMIT_SDAD;
for (uint8_t i = 0; i < datasize; i++) {
buf_tx[i + 1] = data_tx[i];
}
// SPI2_CS_LOW();
// mcb_spi_transfer(buf_tx, buf_rx, bufsize);
// HAL_SPI_TransmitReceive(&hspi2,buf_tx, buf_rx, bufsize,0xff);
HAL_SPI_MY_TransmitReceive_DMA0(&hspi2,buf_tx, buf_rx, bufsize);
/* HAL_SPI_TransmitReceive_DMA(&hspi2,buf_tx, buf_rx, bufsize);
while(__HAL_DMA_GET_COUNTER(&hdma_spi2_rx)!=0);
HAL_GPIO_WritePin(GPIOB,GPIO_PIN_12, GPIO_PIN_SET);*/
// SPI2_CS_HIGH();
status[0] = buf_rx[0];
for (uint8_t i = 0; i < datasize; i++) {
data_rx[i] = buf_rx[i + 1];
}
}
/**
* @brief This function is used to read data from any number of consecutive registers.
*
* @param addr is the address of the first register to start reading from.
* @param data_rx is a pointer to a buffer the received data is stored in.
* @param datasize is the number of consecutive registers to be read.
* @param status is a pointer to a byte the received STATUS byte is written to.
* @param ctrl_1 is a pointer to a byte the received CTRL1 byte is written to.
* @param ctrl_2 is a pointer to a byte the received CTRL2 byte is written to.
* @retval None
*/
void mcb_read_register(uint8_t addr, uint8_t *data_rx, uint8_t datasize, uint8_t *status, uint8_t *ctrl_1, uint8_t *ctrl_2) {
static uint8_t buf_tx[0xFF + 3]; // Dimensioning according to max. datasize + 3 byte header
static uint8_t buf_rx[0xFF + 3];
static uint16_t bufsize;
bufsize = datasize + 3;
buf_tx[0] = MCB_OPCODE_READ_REGISTER;
buf_tx[1] = addr;
for (uint8_t i = 0; i < datasize; i++) {
buf_tx[i + 2] = 0;
}
// SPI2_CS_LOW();
//mcb_spi_transfer(buf_tx, buf_rx, bufsize);
// HAL_SPI_TransmitReceive(&hspi2,buf_tx, buf_rx, bufsize,0xff);
HAL_SPI_MY_TransmitReceive_DMA0(&hspi2,buf_tx, buf_rx, bufsize);
/* HAL_SPI_TransmitReceive_DMA(&hspi2,buf_tx, buf_rx, bufsize);
while(__HAL_DMA_GET_COUNTER(&hdma_spi2_rx)!=0);
HAL_GPIO_WritePin(GPIOB,GPIO_PIN_12, GPIO_PIN_SET);*/
// SPI2_CS_HIGH();
*status = buf_rx[0];
*ctrl_1 = buf_rx[1];
*ctrl_2 = buf_rx[2];
for (uint8_t i = 0; i < datasize; i++) {
data_rx[i] = buf_rx[i + 3];
}
}
/**
* @brief This function is used to write data to any number of consecutive registers.
*
* @param addr is the address of the first register to start writing to.
* @param data_tx is a pointer to a buffer the transmitted data is stored in.
* @param datasize is the number of consecutive registers to be written.
* @param status is a pointer to a byte the received STATUS byte is written to.
* @param ctrl_1 is a pointer to a byte the received CTRL1 byte is written to.
* @param ctrl_2 is a pointer to a byte the received CTRL2 byte is writ
没有合适的资源?快使用搜索试试~ 我知道了~
IC-MCB驱动demo
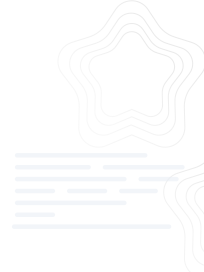
共2个文件
h:1个
c:1个

需积分: 0 3 下载量 44 浏览量
更新于2024-10-12
1
收藏 4KB RAR 举报
STM32使用SPI+DMA模式和IC-MCB的基础通讯
收起资源包目录


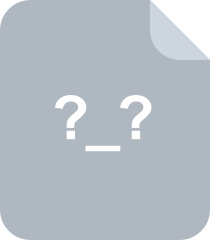
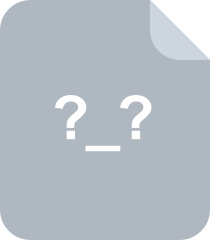
共 2 条
- 1
资源推荐
资源预览
资源评论
126 浏览量
159 浏览量
130 浏览量
2022-09-23 上传
2009-11-28 上传
144 浏览量
120 浏览量
185 浏览量
173 浏览量
2023-10-24 上传
134 浏览量
103 浏览量
167 浏览量
2020-08-31 上传
2011-12-28 上传
2022-09-21 上传
178 浏览量
2022-09-23 上传
2009-11-09 上传
106 浏览量
2009-08-25 上传
103 浏览量
2014-02-22 上传
2015-09-13 上传
资源评论
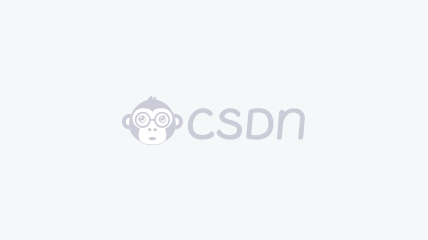

ChanTongJing
- 粉丝: 20
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

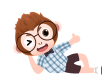
安全验证
文档复制为VIP权益,开通VIP直接复制
