/*++
Copyright (c) 1999 - 2002 Microsoft Corporation
Module Name:
SwapBuffers.c
Abstract:
This is a sample filter which demonstrates proper access of data buffer
and a general guideline of how to swap buffers.
For now it only swaps buffers for:
IRP_MJ_READ
IRP_MJ_WRITE
IRP_MJ_DIRECTORY_CONTROL
By default this filter attaches to all volumes it is notified about. It
does support having multiple instances on a given volume.
Environment:
Kernel mode
--*/
#include <fltKernel.h>
#include <dontuse.h>
#include <suppress.h>
#pragma prefast(disable:__WARNING_ENCODE_MEMBER_FUNCTION_POINTER, "Not valid for kernel mode drivers")
PFLT_FILTER gFilterHandle;
/*************************************************************************
Pool Tags
*************************************************************************/
#define BUFFER_SWAP_TAG 'bdBS'
#define CONTEXT_TAG 'xcBS'
#define NAME_TAG 'mnBS'
#define PRE_2_POST_TAG 'ppBS'
/*************************************************************************
Local structures
*************************************************************************/
//
// This is a volume context, one of these are attached to each volume
// we monitor. This is used to get a "DOS" name for debug display.
//
typedef struct _VOLUME_CONTEXT {
//
// Holds the name to display
//
UNICODE_STRING Name;
//
// Holds the sector size for this volume.
//
ULONG SectorSize;
} VOLUME_CONTEXT, *PVOLUME_CONTEXT;
#define MIN_SECTOR_SIZE 0x200
//
// This is a context structure that is used to pass state from our
// pre-operation callback to our post-operation callback.
//
typedef struct _PRE_2_POST_CONTEXT {
//
// Pointer to our volume context structure. We always get the context
// in the preOperation path because you can not safely get it at DPC
// level. We then release it in the postOperation path. It is safe
// to release contexts at DPC level.
//
PVOLUME_CONTEXT VolCtx;
//
// Since the post-operation parameters always receive the "original"
// parameters passed to the operation, we need to pass our new destination
// buffer to our post operation routine so we can free it.
//
PVOID SwappedBuffer;
} PRE_2_POST_CONTEXT, *PPRE_2_POST_CONTEXT;
//
// This is a lookAside list used to allocate our pre-2-post structure.
//
NPAGED_LOOKASIDE_LIST Pre2PostContextList;
/*************************************************************************
Prototypes
*************************************************************************/
NTSTATUS
InstanceSetup (
__in PCFLT_RELATED_OBJECTS FltObjects,
__in FLT_INSTANCE_SETUP_FLAGS Flags,
__in DEVICE_TYPE VolumeDeviceType,
__in FLT_FILESYSTEM_TYPE VolumeFilesystemType
);
VOID
CleanupVolumeContext(
__in PFLT_CONTEXT Context,
__in FLT_CONTEXT_TYPE ContextType
);
NTSTATUS
InstanceQueryTeardown (
__in PCFLT_RELATED_OBJECTS FltObjects,
__in FLT_INSTANCE_QUERY_TEARDOWN_FLAGS Flags
);
NTSTATUS
DriverEntry (
__in PDRIVER_OBJECT DriverObject,
__in PUNICODE_STRING RegistryPath
);
NTSTATUS
FilterUnload (
__in FLT_FILTER_UNLOAD_FLAGS Flags
);
FLT_PREOP_CALLBACK_STATUS
SwapPreReadBuffers(
__inout PFLT_CALLBACK_DATA Data,
__in PCFLT_RELATED_OBJECTS FltObjects,
__deref_out_opt PVOID *CompletionContext
);
FLT_POSTOP_CALLBACK_STATUS
SwapPostReadBuffers(
__inout PFLT_CALLBACK_DATA Data,
__in PCFLT_RELATED_OBJECTS FltObjects,
__in PVOID CompletionContext,
__in FLT_POST_OPERATION_FLAGS Flags
);
FLT_POSTOP_CALLBACK_STATUS
SwapPostReadBuffersWhenSafe (
__inout PFLT_CALLBACK_DATA Data,
__in PCFLT_RELATED_OBJECTS FltObjects,
__in PVOID CompletionContext,
__in FLT_POST_OPERATION_FLAGS Flags
);
FLT_PREOP_CALLBACK_STATUS
SwapPreDirCtrlBuffers(
__inout PFLT_CALLBACK_DATA Data,
__in PCFLT_RELATED_OBJECTS FltObjects,
__deref_out_opt PVOID *CompletionContext
);
FLT_POSTOP_CALLBACK_STATUS
SwapPostDirCtrlBuffers(
__inout PFLT_CALLBACK_DATA Data,
__in PCFLT_RELATED_OBJECTS FltObjects,
__in PVOID CompletionContext,
__in FLT_POST_OPERATION_FLAGS Flags
);
FLT_POSTOP_CALLBACK_STATUS
SwapPostDirCtrlBuffersWhenSafe (
__inout PFLT_CALLBACK_DATA Data,
__in PCFLT_RELATED_OBJECTS FltObjects,
__in PVOID CompletionContext,
__in FLT_POST_OPERATION_FLAGS Flags
);
FLT_PREOP_CALLBACK_STATUS
SwapPreWriteBuffers(
__inout PFLT_CALLBACK_DATA Data,
__in PCFLT_RELATED_OBJECTS FltObjects,
__deref_out_opt PVOID *CompletionContext
);
FLT_POSTOP_CALLBACK_STATUS
SwapPostWriteBuffers(
__inout PFLT_CALLBACK_DATA Data,
__in PCFLT_RELATED_OBJECTS FltObjects,
__in PVOID CompletionContext,
__in FLT_POST_OPERATION_FLAGS Flags
);
VOID
ReadDriverParameters (
__in PUNICODE_STRING RegistryPath
);
//
// Assign text sections for each routine.
//
#ifdef ALLOC_PRAGMA
#pragma alloc_text(PAGE, InstanceSetup)
#pragma alloc_text(PAGE, CleanupVolumeContext)
#pragma alloc_text(PAGE, InstanceQueryTeardown)
#pragma alloc_text(INIT, DriverEntry)
#pragma alloc_text(INIT, ReadDriverParameters)
#pragma alloc_text(PAGE, FilterUnload)
#endif
//
// Operation we currently care about.
//
CONST FLT_OPERATION_REGISTRATION Callbacks[] = {
{ IRP_MJ_READ,
0,
SwapPreReadBuffers,
SwapPostReadBuffers },
{ IRP_MJ_WRITE,
0,
SwapPreWriteBuffers,
SwapPostWriteBuffers },
{ IRP_MJ_DIRECTORY_CONTROL,
0,
SwapPreDirCtrlBuffers,
SwapPostDirCtrlBuffers },
{ IRP_MJ_OPERATION_END }
};
//
// Context definitions we currently care about. Note that the system will
// create a lookAside list for the volume context because an explicit size
// of the context is specified.
//
CONST FLT_CONTEXT_REGISTRATION ContextNotifications[] = {
{ FLT_VOLUME_CONTEXT,
0,
CleanupVolumeContext,
sizeof(VOLUME_CONTEXT),
CONTEXT_TAG },
{ FLT_CONTEXT_END }
};
//
// This defines what we want to filter with FltMgr
//
CONST FLT_REGISTRATION FilterRegistration = {
sizeof( FLT_REGISTRATION ), // Size
FLT_REGISTRATION_VERSION, // Version
0, // Flags
ContextNotifications, // Context
Callbacks, // Operation callbacks
FilterUnload, // MiniFilterUnload
InstanceSetup, // InstanceSetup
InstanceQueryTeardown, // InstanceQueryTeardown
NULL, // InstanceTeardownStart
NULL, // InstanceTeardownComplete
NULL, // GenerateFileName
NULL, // GenerateDestinationFileName
NULL // NormalizeNameComponent
};
/*************************************************************************
Debug tracing information
*************************************************************************/
//
// Definitions to display log messages. The registry DWORD entry:
// "hklm\system\CurrentControlSet\Services\Swapbuffers\DebugFlags" defines
// the default state of these logging flags
//
#define LOGFL_ERRORS 0x00000001 // if set, display error messages
#define LOGFL_READ 0x00000002 // if set, display READ operation info
#define LOGFL_WRITE 0x00000004 // if set, display WRITE operation info
#define LOGFL_DIRCTRL 0x00000008 // if set, display DIRCTRL operation info
#de
没有合适的资源?快使用搜索试试~ 我知道了~
微软微过滤器MiniFilter的源码
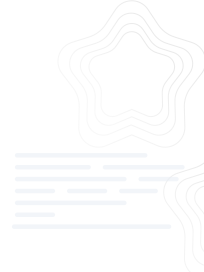
共81个文件
c:21个
h:15个
rc:11个


温馨提示
微软微过滤器MiniFilter的源码 Windows Server 2008 IFSDDK里面的东东 有兴趣的研究下
资源推荐
资源详情
资源评论
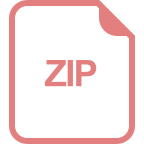
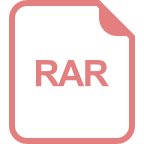
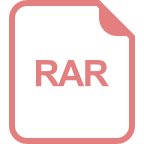
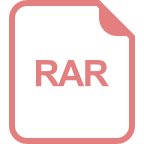
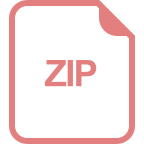
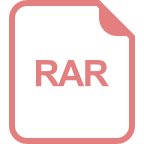
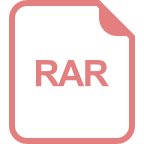
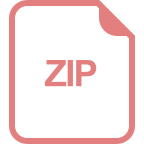
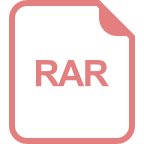
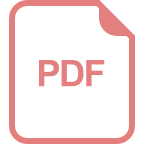
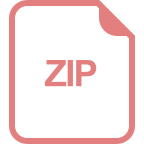
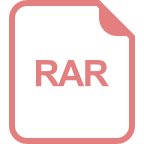
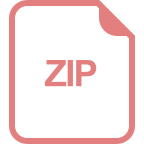
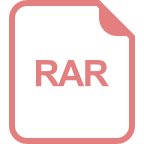
收起资源包目录



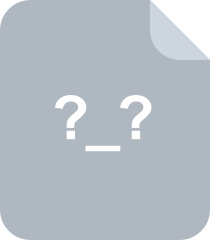
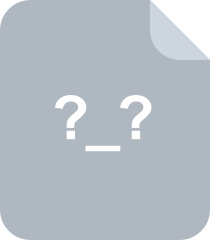
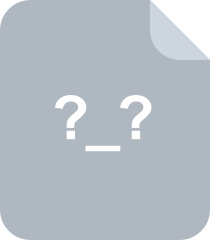
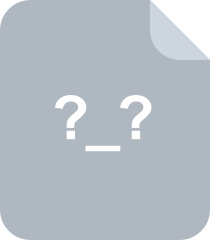
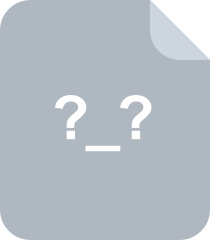

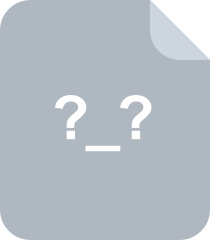
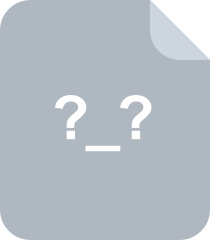
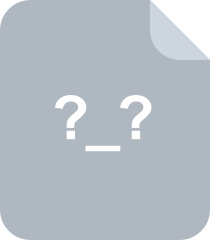
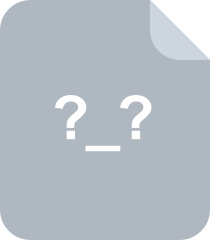
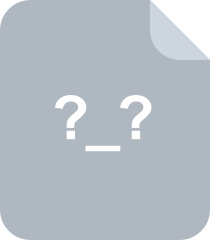

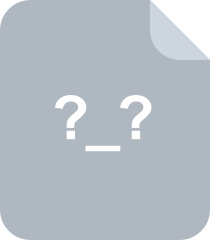
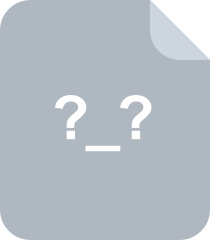
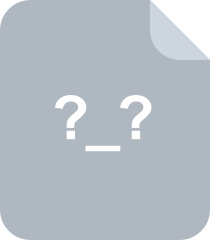
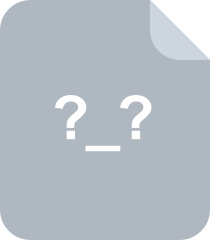
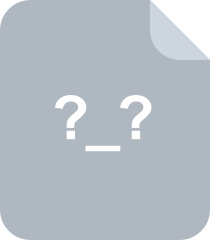

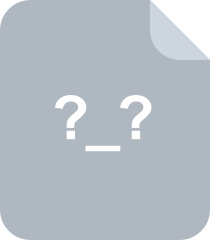
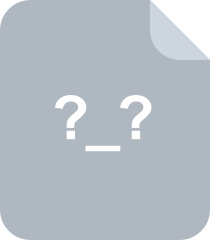
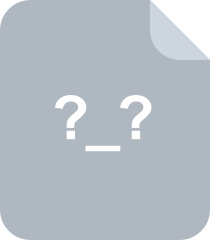
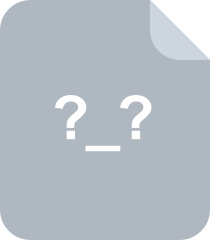
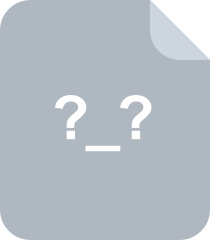
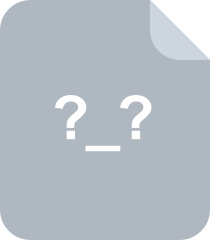
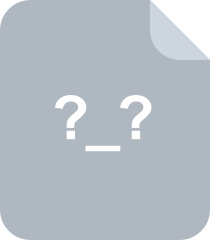
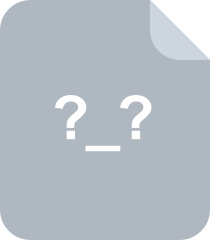
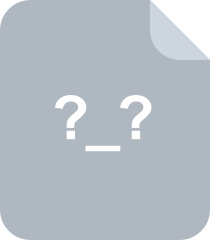
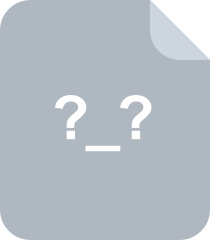
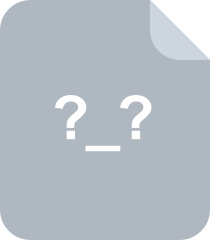

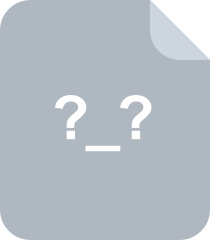
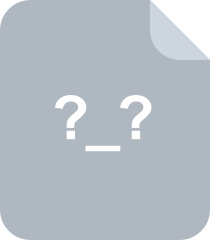
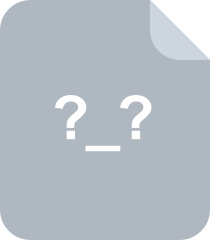
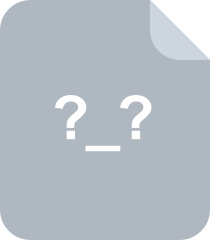
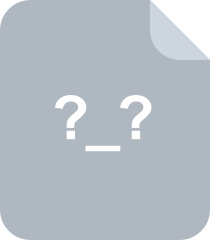
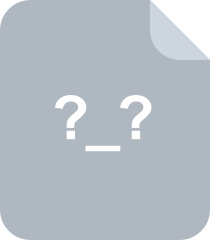
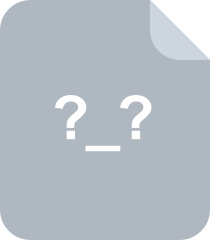
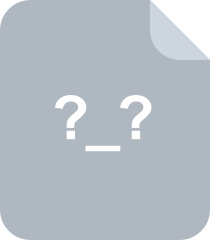
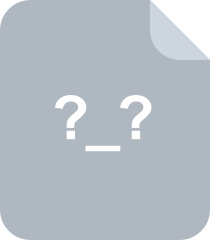

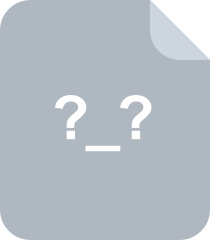
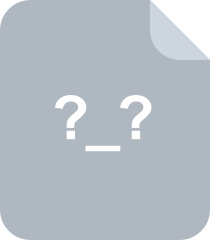
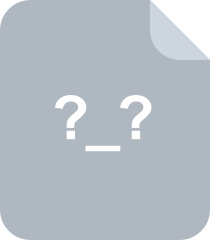
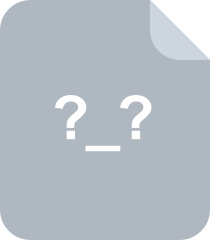
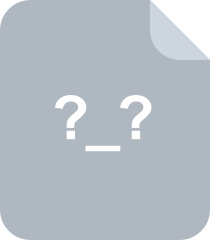
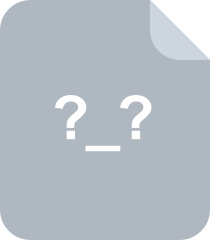


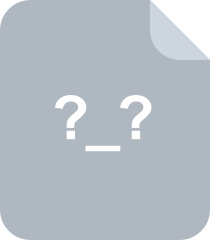
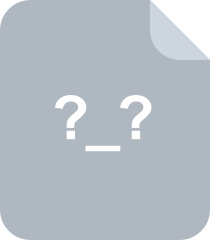
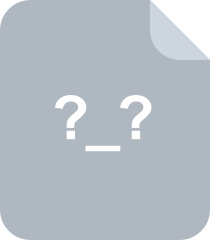
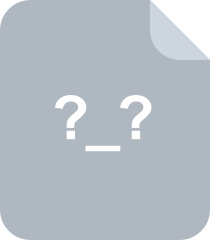
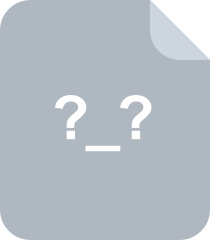

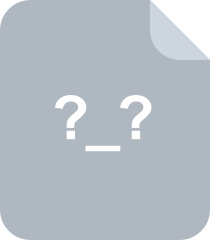

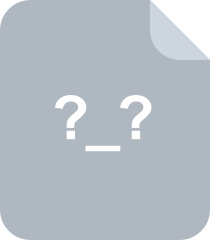
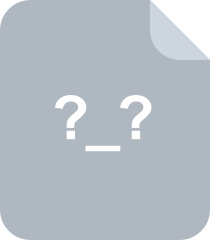
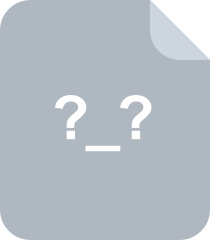
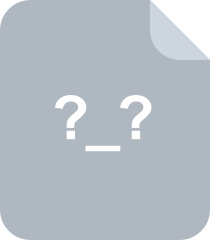
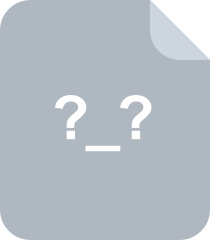
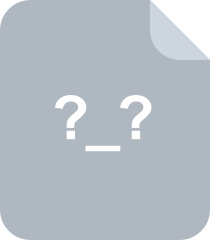
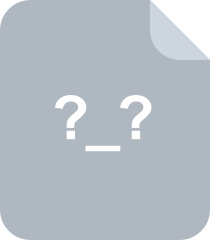


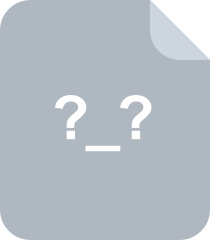
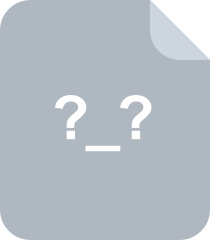
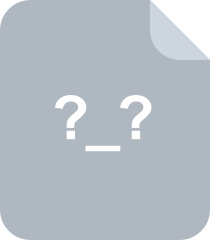
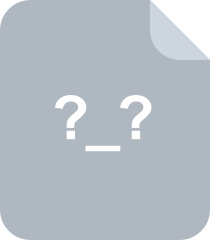
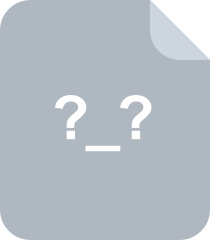
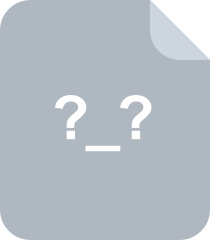
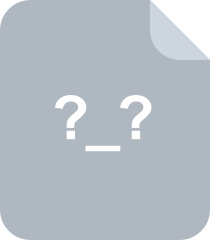

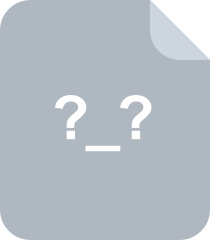

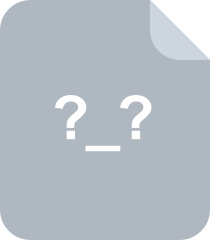
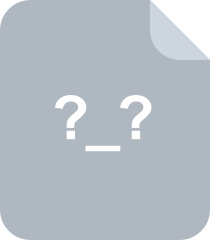
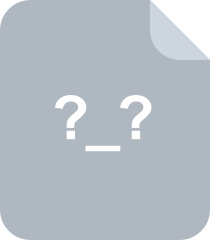
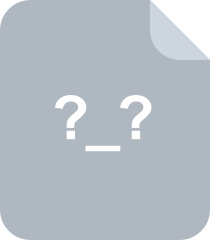
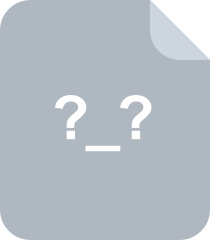
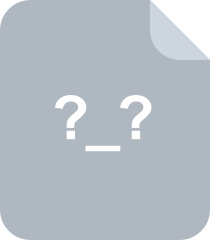
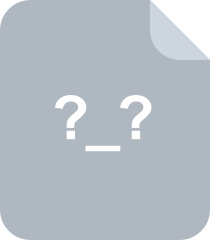
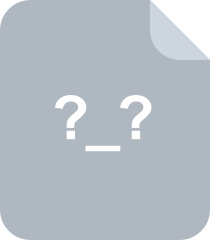

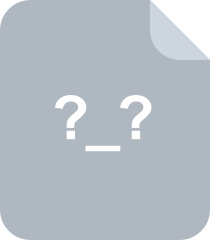
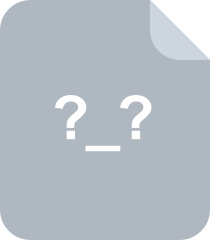
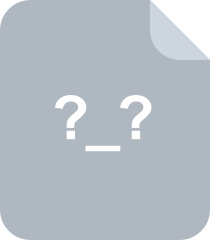
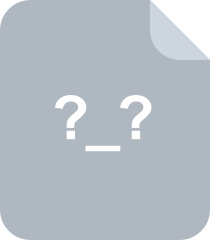
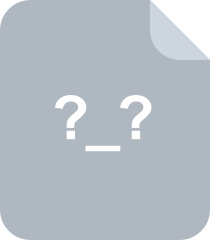
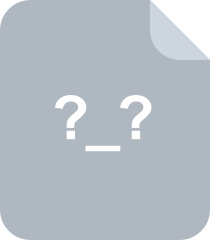
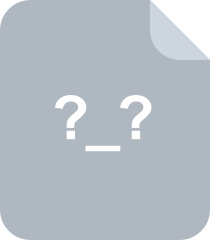
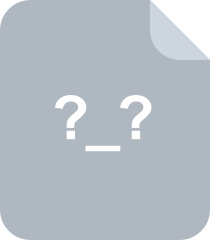
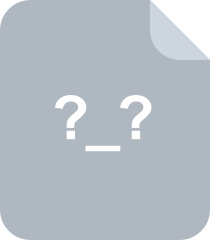
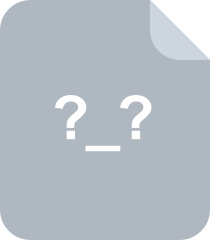
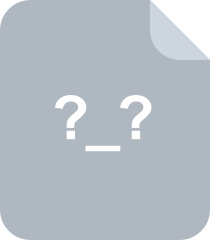
共 81 条
- 1
资源评论
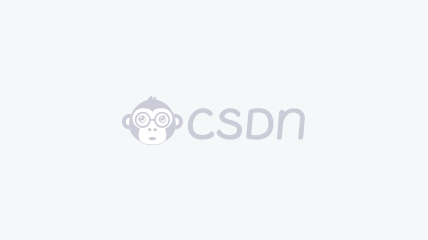
- wrgg02013-04-01之前在学习sfilter,后来发现minifilter好像更简单点,这应该是不错的东西。感谢分享。
- u0106863902013-06-14下载后才发现已经安装过了,不过还是给分。
- luohegongmin2013-03-22要是代码详细些就好了
- keary0932011-10-14就是WinDDK自带的东西,不过还是不错了~

888atao
- 粉丝: 5
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

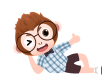
最新资源
- yolov5,SSD 可能使用到的一些代码
- 介绍离散性制造行业的MES系统流程
- 基于IDEA-CCNL/Randeng-Pegasus-238M-Summary-Chines微调的中文文本摘要任务源码+数据集
- 微信小程序源码 车源宝 二手车交易平台 源码下载
- 微信小程序源码 实现 城市切换 demo 根据城市首字母排序城市 选择城市 源码下载
- 2024新版计算机编译原理期末速成全套视频教程(视频+配套资料)
- VMware7.0虚拟机硬盘无法编辑,无法连接到Profile-Driven Storage Service
- arm64内核的mongo镜像
- 基于stm32f103c单片机+MPU6050+0.96英寸OLED显示屏双柄遥控器硬件(原理图+PCB)工程文件.zip
- 整理的关于少儿编程的学习路径,以及如何在小升初,初升高和大学充分的利用起来编程经验的优势
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


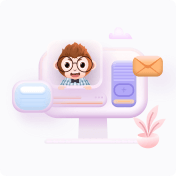
安全验证
文档复制为VIP权益,开通VIP直接复制
