/****************************************
* 重要:3.2.1.121,ws客户端已经改变调用方式,具体如下:
* get_chat_nick_p()
* get_contact_list()
* get_chatroom_memberlist()
* send_at_msg()
* send_attatch()
* send_txt_msg()
* send_pic_msg()
* get_personal_info()
* ***************************************/
const WebSocket = require('ws');
const ws = new WebSocket('ws://127.0.0.1:5555');
const chatBot = require("./chatgpt");
const HEART_BEAT = 5005;
const RECV_TXT_MSG = 1;
const RECV_PIC_MSG = 3;
const USER_LIST = 5000;
const GET_USER_LIST_SUCCSESS = 5001;
const GET_USER_LIST_FAIL = 5002;
const TXT_MSG = 555;
const PIC_MSG = 500;
const AT_MSG = 550;
const CHATROOM_MEMBER = 5010;
const CHATROOM_MEMBER_NICK = 5020;
const PERSONAL_INFO = 6500;
const DEBUG_SWITCH = 6000;
const PERSONAL_DETAIL =6550;
const DESTROY_ALL = 9999;
const NEW_FRIEND_REQUEST=37;//微信好友请求消息
const AGREE_TO_FRIEND_REQUEST=10000;//同意微信好友请求消息
const ATTATCH_FILE = 5003;
function getid()
{
const id = Date.now();
return id.toString();
}
function get_chat_nick_p(s_wxid,s_roomid)
{
const j={
id:getid(),
type:CHATROOM_MEMBER_NICK,
wxid:s_wxid,
roomid:s_roomid,
content:'null',
nickname:"null",
ext:'null'
};
const s= JSON.stringify(j);
return s;
}
function get_chat_nick()
{
/*const j={
id:getid(),
type:CHATROOM_MEMBER_NICK,
content:'23023281066@chatroom',//chatroom id 23023281066@chatroom 17339716569@chatroom
//5325308046@chatroom
//5629903523@chatroom
wxid:'ROOT'
};*/
const s= JSON.stringify(j);
return s;
}
function handle_nick(j){
const data = j.content;
let i = 0;
for(const item of data)
{
console.log(i++,item.nickname)
}
}
function handle_memberlist(j)
{
const data = j.content;
let i =0;
//get_chat_nick_p(j.roomid);
for(const item of data)
{
console.log("---------------",item.room_id,"--------------------");
//console.log("------"+item.roomid+"--------");
//ws.send(get_chat_nick_p(item.roomid));
const memberlist=item.member;
//console.log("hh",item.address,memberlist);
//const len = memberlist.length();
//console.log(memberlist);
for(const m of memberlist)
{
console.log(m);//获得每个成员的wxid
}
/*for(const n of nicklist)//目前不建议使用
{
console.log(n);//获得每个成员的昵称,注意,没有和wxi对应的关系
}*/
}
}
function get_chatroom_memberlist()
{
const j={
id:getid(),
type:CHATROOM_MEMBER,
roomid:'null',//null
wxid:'null',//not null
content:'null',//not null
nickname:'null',
ext:'null'
};
const s= JSON.stringify(j);
return s;
}
function send_attatch()
{
/*const j={
id:getid(),
type:ATTATCH_FILE,
content:'C:\\tmp\\log.txt',
wxid:'23023281066@chatroom'
};*/
const j={
id:getid(),
type:ATTATCH_FILE,
wxid:'23023281066@chatroom',//roomid或wxid,必填
roomid:'null',//此处为空
content:'C:\\tmp\\log.7z',
nickname:"null",//此处为空
ext:'null'//此处为空
//wxid:'22428457414@chatroom'
};
const s = JSON.stringify(j);
return s;
}
function send_at_msg()
{
const j={
id:getid(),
type:AT_MSG,
roomid:'23023281066@chatroom',//not null 23023281066@chatroom
wxid:'your wxid',//not null
content:'at msg test,hello world,真的有一套',//not null
nickname:'老张',
ext:'null'
};
const s = JSON.stringify(j);
return s;
}
function send_pic_msg()
{
const j={
id:getid(),
type:PIC_MSG,
wxid:'23023281066@chatroom',
roomid:'null',
content:'C:\\tmp\\2.jpg',
nickname:"null",
ext:'null'
//wxid:'22428457414@chatroom'
};
const s = JSON.stringify(j);
//console.log(s);
return s;
}
function get_personal_detail()
{
/*const j={
id:getid(),
type:PERSONAL_DETAIL,
content:'op:personal detail',
wxid:'zhanghua_cd'
};*/
const s = JSON.stringify(j);
return s;
}
/**
* send_txt_msg : 发送消息给好友
*
*/
function get_personal_info()
{
const j={
id:getid(),
type:PERSONAL_INFO,
wxid:'null',
roomid:'null',
content:'null',
nickname:"null",
ext:'null'
//wxid:'22428457414@chatroom'
};
const s = JSON.stringify(j);
return s;
}
function send_txt_msg(wxid,text)
{
//必须按照该json格式,否则服务端会出异常
const j={
id:getid(),
type:TXT_MSG,
wxid:wxid,//roomid或wxid,必填
roomid:'null',//此处为空
content:text,
nickname:"null",//此处为空
ext:'null'//此处为空
};
const s = JSON.stringify(j);
return s;
}
/**
* send_wxuser_list : 获取微信通讯录用户名字和wxid
*/
function get_contact_list()
{
/*const j={
id:getid(),
type:USER_LIST,
content:'user list',
wxid:'null'
};*/
const j={
id:getid(),
type:USER_LIST,
roomid:'null',//null
wxid:'null',//not null
content:'null',//not null
nickname:'null',
ext:'null'
};
const s = JSON.stringify(j);
//console.log(s);
return s;
}
/**
* handle_wxuser_list : websocket返回的用户信息
* @param {*} j json数组
* 数组里面的wxid,要保存下来,供发送微信的时候用
*
*/
function handle_wxuser_list(j)
{
const j_ary = j.content;
var i = 0;
for(const item of j_ary)
{
i=i+1;
const id = item.wxid;
const m = id.match(/@/);
if(m!=null){
//console.log(id);
console.log(item.wxid,item.name);
}
//console.log(m);
//
}
}
/**
*
* @param {*} j json对象
*/
async function handle_recv_msg(j)
{
/*const content = j.content;
const wxid =j.wxid;
const sender = j.sender;
consoconsole.log(j);le.log('接收人:'+wxid);
console.log('内容:'+content);
console.log('发送人:'+sender);*///如果为空,那就是你自己发的
console.log("接收到消息:" , j);
let res = await chatBot.sendMsg(j.content);
console.log("Chatgpt回复:" + res);
ws.send(send_txt_msg(j.wxid,res));
}
function heartbeat(j)
{
//console.log(j);
//console.log(utf16ToUtf8(wxid),utf16ToUtf8(name));
}
ws.on('open', function open()
{
console.info("连接微信成功");
//ws.send(get_personal_info());
//ws.send(get_contact_list());
//ws.send(send_attatch());
//ws.send("hello world");
//ws.send(destroy_all());
//ws.send(get_chat_nick_p("zhanghua_cd","23023281066@chatroom"));
//for(const item of roomid_list)
//{
//console.log(item);
//ws.send(get_chat_nick_p(item));
//}
//ws.send(get_chat_nick());
//ws.send(get_personal_info());
//ws.send(send_pic_msg());
//ws.send(send_txt_msg());
//ws.send(get_chatroom_memberlist());
//ws.send(send_at_msg());
//ws.send(get_personal_detail());
//ws.send(debug_switch()
//ws.send(send_wxuser_list());
//ws.send(send_txt_msg());
/** 获取chatroom 成员昵称
* ws.send(get_chat_nick());
*/
/** 通过wxid获取好友详细细腻
* ws.send(get_personal_detail());
*/
/** debugview调试信息开关,默认为关
* ws.send(debug_switch());
*/
/** 获取微信个人信息
* ws.send(get_personal_info());
*/
/** 获取群好友列表
* ws.send(get_chatroom_memberlist());
*/
/** 发送群AT消息
* ws.send(send_at_msg());
*/
/**获取微信通讯录好友列�
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
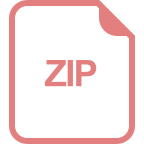
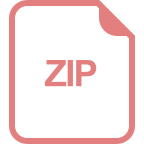
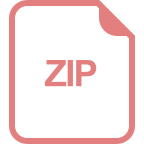
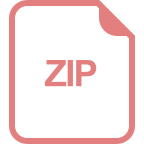
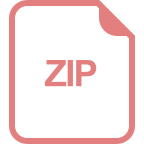
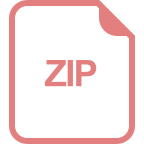
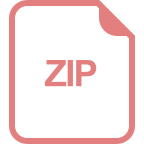
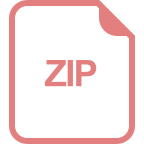
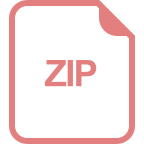
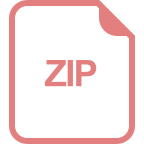
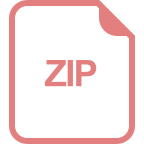
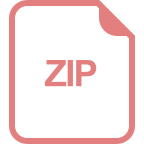
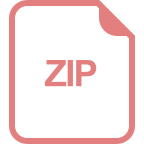
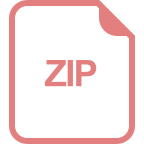
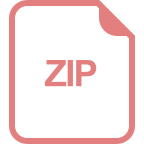
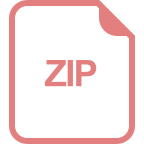
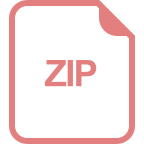
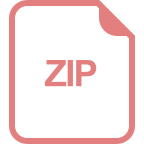
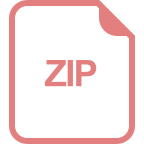
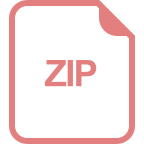
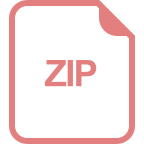
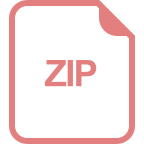
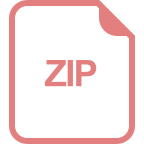
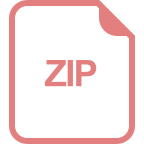
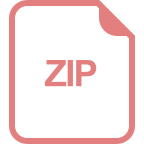
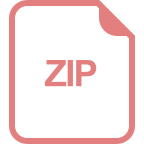
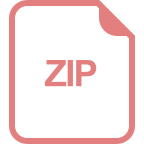
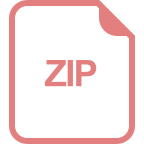
收起资源包目录



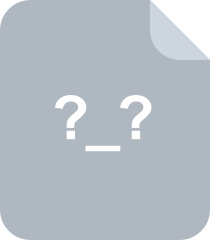
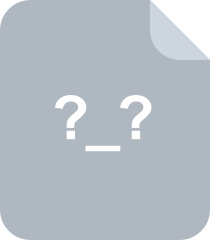

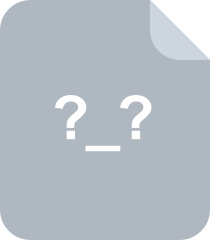


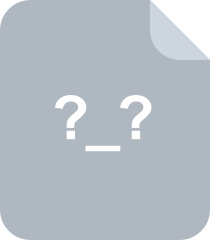

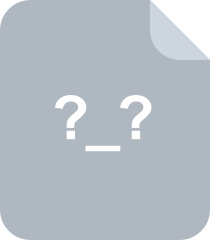
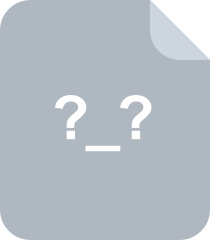

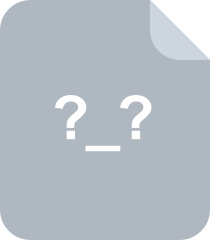
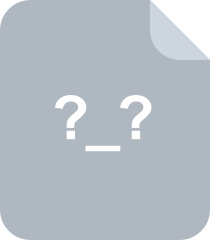
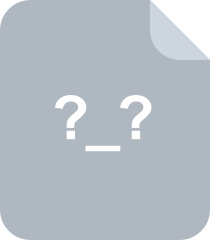
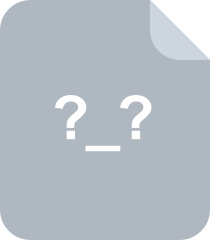

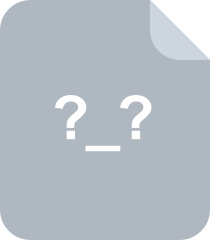
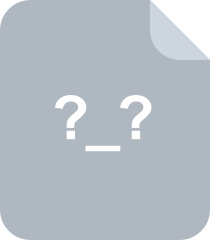
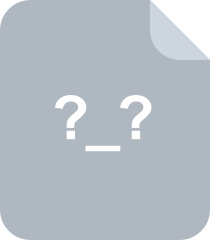
共 13 条
- 1
资源评论
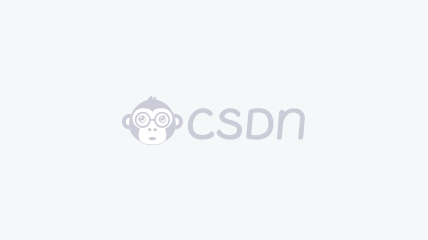

2401_87496566
- 粉丝: 976
- 资源: 5118
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

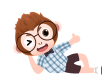
最新资源
- 基于 Javascript 实现的图像裁剪,图像缩放(最邻近插值,双线性差值,三次卷积插值),图像滤镜(灰度,模糊,锐化,卡通)
- S14英雄联盟全球总决赛B站直播弹幕
- 基于javafx+swing实现桌面应用记事本项目(完整的项目,包含源码和素材)
- 新年年会抽奖券批量打印工具ver2.07 -2024.xlsm
- 代码公示-CSP-J2-S2-2024-已解密.zip
- 基于javaFx+swing开发桌球小游戏项目(完整的项目,包含源码和素材)
- 友价免签约支付接口插件最新版
- 个人回归分析学习笔记-1
- 17年国赛,基于 python 实现的图像识别(彩图找黑点和绿点,单纯找黑点,优先绿点后黑点)
- 毕业设计项目:基于Java的网站内容管理系统
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


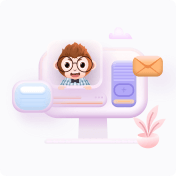
安全验证
文档复制为VIP权益,开通VIP直接复制
