<p align="center">
<img alt="eagle.js" title="eagle.js" src="https://raw.githubusercontent.com/Zulko/eagle.js/master/img/logo.svg?sanitize=true" width="150">
</p>
<h1 align="center">Eagle.js - A slideshow framework for hackers</h1>
[](https://badge.fury.io/js/eagle.js)
[](https://travis-ci.org/Zulko/eagle.js)
- Slideshow system built on top of the Vue 2
- Supports animations, themes, interactive widgets (for web demos)
- Easy to reuse components, slides and styles across presentations
- Lightweight core and various helpful extensions
- All APIs public, maximum hackability
**This project is considered feature-completed and not actively maintained currently. We recommend you to use [slidev](https://sli.dev/) for better support of Vue 3.**
### For a quick tour, see [this slideshow](https://zulko.github.io/eaglejs-demo/#/introducing-eagle):
<p align="center"><a href="https://zulko.github.io/eaglejs-demo/#/introducing-eagle" target="_blank"><img alt="screenshot" src="https://raw.githubusercontent.com/Zulko/eagle.js/master/img/screenshot.jpg"></a></p>
Most of all, eagle.js aims at offering a simple and very hackable API so you
can get off the beaten tracks and craft the slideshows you really want.
Here is what the eagle.js syntax looks like (Example here are using [Pug](https://pugjs.org/api/getting-started.html), but you can still use plain HTML):
```pug
.eg-slideshow
slide
h1 My slideshow
h4 By Zulko
slide
h3 Title of this slide
p Paragraph 1.
p Paragraph 2.
slide(:steps=3)
h3 Slide with bullet points
p(v-if='step >= 2') This will appear first.
p(v-if='step >= 3') This will appear second.
```
If you are not familiar with Vue.js you will find eagle.js harder to use than, say, [Reveal.js](https://github.com/hakimel/reveal.js/), but on the long term eagle.js makes it easier to organize your slides and implement new ideas.
## Templates
- [**elpete/eaglejs-template**](https://github.com/elpete/eaglejs-template) Eagle.js Template for vue-cli.
- [**christian-nils/presentation_template**](https://github.com/christian-nils/presentation_template) Presentation template using VueJS and EagleJS, with some custom components. ([demo](https://christian-nils.github.io/phd_presentation/))
## Get started
You must have Node.js/npm installed to use eagle.js.
Then the best to get started is to clone [the example repo](https://github.com/Zulko/eaglejs-demo):
``` bash
$ git clone https://github.com/Zulko/eaglejs-demo.git
```
Install the dependencies (they will only be downloaded in a local folder):
```bash
$ cd eaglejs-demo
$ npm install
```
Then run ```npm run dev``` to start the server, and open your browser at [http://localhost:8080](http://localhost:8080) to see the slideshows.
To start editing, click on ``My first slideshow`` to display this slideshow, then open the file ``eagle/src/slideshows/first-slideshow/FirstSlideshow.vue`` and change the content of the first slide. Observe the changes happen automatically in your browser. The only times you need to refresh the page is when you add remove or add slides to the presentation.
## Install
Install by npm
```bash
npm install --save eagle.js
```
Or install by yarn
```bash
yarn add eagle.js
```
## Usage
Eagle.js is a vue plugin. You need to `use` eagle.js in your vue app's main file.
<br>**New in 0.3**: `animate.css` is now a peer dependency. User need install their own version.
<br>**New in 0.5**: By default eagle.js doesn't export all plugins but only core components. You have to explicitly use your widgets or plugins from now on. See more on [extensions section](https://github.com/Zulko/eagle.js#extensions).
<br>**New in 0.6**: You do not need to explicitly import the default style anymore.
```javascript
import Eagle from 'eagle.js'
// import animate.css for slide transition
import 'animate.css'
Vue.use(Eagle)
```
### Basic idea
Eagle.js's basic components are `slideshow` and `slide`. You use `slideshow` as mixin to write `slideshow` component, which could include multiple `slide`s. A very basic Single File Component for `slideshow` would look like this:
```vue
<template lang="pug">
slide(:steps="4")
p(v-if="step >= 1")
| {{step}}
p(v-if="step >= 2")
| {{step}}
p(v-if="step >= 3")
| {{step}}
p(v-if="step >= 4")
| {{step}}
</template>
<script>
import { Slideshow } from 'eagle.js'
export default {
mixins: [Slideshow]
}
</script>
```
We use `slideshow`'s data `step` to control the conditional rendering in `slide`, thus `slideshow` is used as a mixin. Also by this way eagle.js exposes the maximum hackability to users.
### slideshow
`slideshow` can only be used as mixin.
*Note*: For vue mixins, template cannot be extended. `slideshow` needs one HTML element to wrap around your following `slide`s because there are events registered to `slideshow` after component mounted. **We recommend you to wrap your template in a `eg-slideshow` div for default styling.** Also, do not add conditional rendering on `slideshow` (for example, add `v-if="active"` on your `slideshow` template) as it would break `slideshow`'s events registration as well.
You can configure your authored `slideshow` component with these properties:
| Property | Default | Description |
| -------------------- | --------------- | --------------------------------------------------------- |
| `firstSlide` | `1` | |
| `lastSlide` | `null` | |
| `startStep` | `1` | |
| `mouseNavigation` | `true` | Navigate with mouse click or scroll event |
| `keyboardNavigation` | `true` | Navigate with keyboard |
| `embedded` | `false` | |
| `inserted` | `false` | |
| `onStartExit` | `null` | event callback for exiting slideshow through first slide |
| `onEndExit` | `null` | event callback for exiting slideshow through last slide |
| `backBySlide` | `false` | slideshow navigates back by step by default |
| `repeat` | `false` | go to first slide automatically when reaching the last one|
| `zoom` | `true` | alt + click can zoom on slide |
More explaination on `backBySlide`:
By default, slideshow navigates back by step, but you can change the behavior to be slide based: so if you go back to the previous slide, it lands on the first step instead of last step. See a comparison:
|Back by Step: |Back by slide:|
|--------------|--------------|
|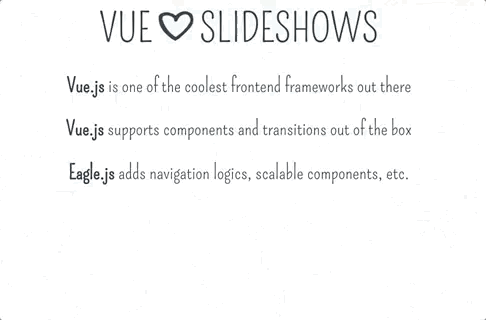|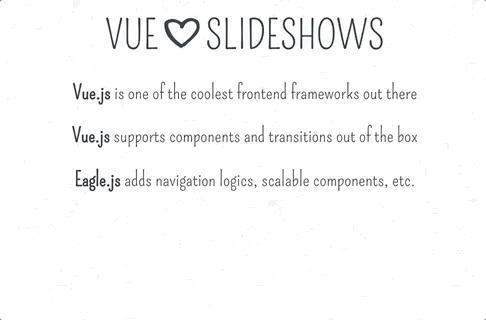|
Please note, if you have any embedded slideshows, you have to use default back mode, because for now parent slideshow cannot know how many steps child slideshow backs. This is a feature to be implemented in the future.
#### Nested slideshow
A nested slideshow can be an `inserted` one or an `embedded` one. If the nested slideshow's parent is a slideshow, then it's an `inserted` slideshow; if the parent is a slide, then it's an `embedded` slideshow.
An `embedded` slideshow would have its own events and embedded styles, while an `inserted` slideshow does not. *Do not mix them up:* a `embedded` slideshow in a slideshow will replace its
没有合适的资源?快使用搜索试试~ 我知道了~
使用 Vue.js 构建的可破解幻灯片框架.zip
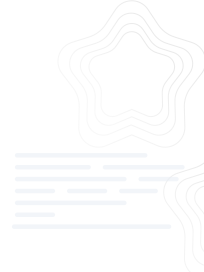
共49个文件
vue:14个
js:12个
scss:7个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 156 浏览量
2024-12-02
02:04:29
上传
评论
收藏 3.92MB ZIP 举报
温馨提示
Eagle.js - 黑客的幻灯片框架 基于 Vue 2 构建的幻灯片系统支持动画、主题、交互式小部件(用于网络演示)轻松在演示文稿中重复使用组件、幻灯片和样式轻量级核心和各种有用的扩展所有 API 均公开,最大程度提高黑客攻击风险该项目被认为功能已完成,目前没有积极维护。我们建议您使用slidev以更好地支持 Vue 3。如需快速浏览,请参阅此幻灯片最重要的是,eagle.js 旨在提供一个简单且易于破解的 API,以便您可以摆脱常规,制作您真正想要的幻灯片。eagle.js 语法如下(此处的示例使用Pug,但您仍然可以使用纯 HTML).eg-slideshow slide h1 My slideshow h4 By Zulko slide h3 Title of this slide p Paragraph 1. p Paragraph 2. slide(:steps=3) h3 Slide with bullet points
资源推荐
资源详情
资源评论
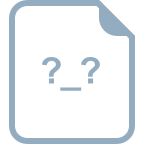
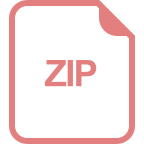
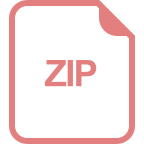
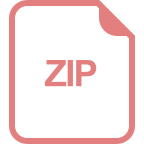
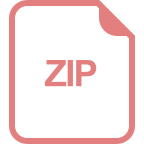
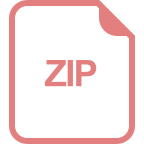
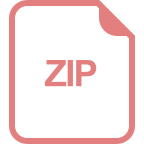
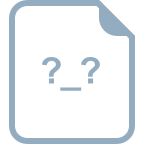
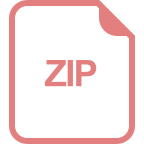
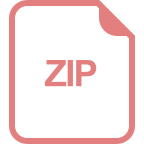
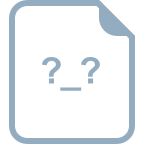
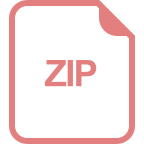
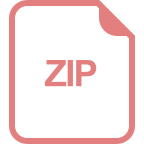
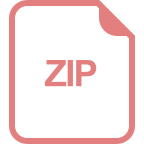
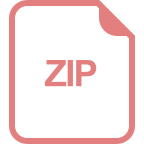
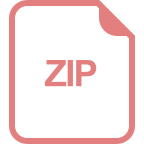
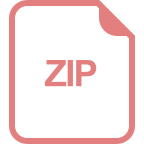
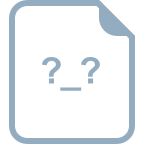
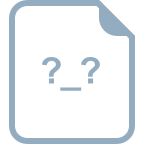
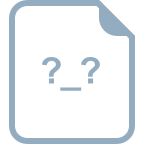
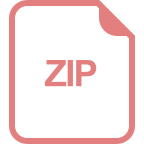
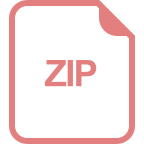
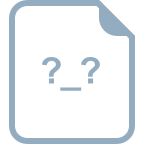
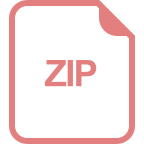
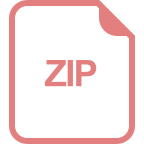
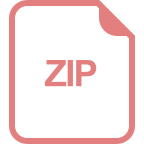
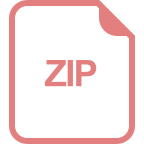
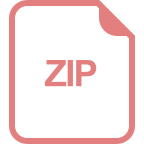
收起资源包目录

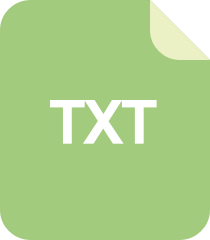

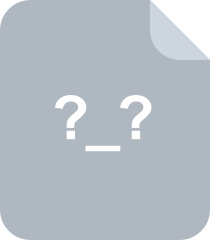
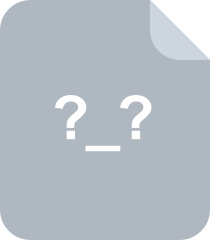
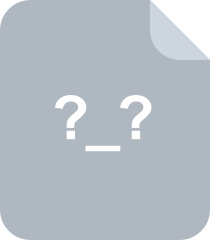
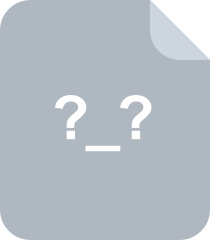
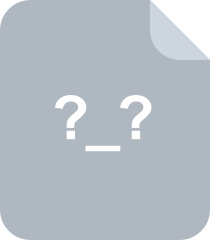

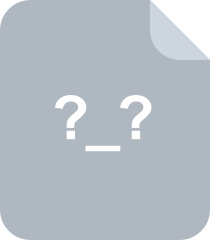
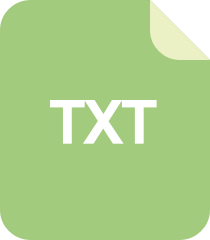
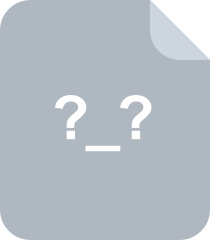

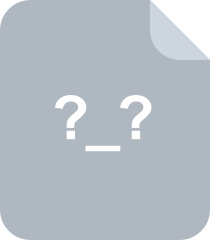


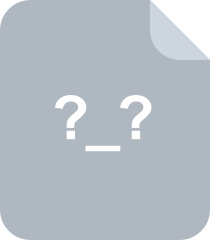
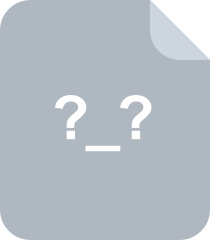
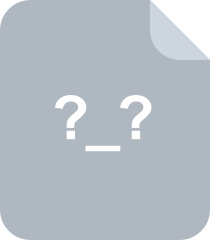
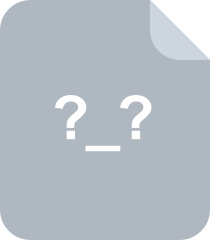

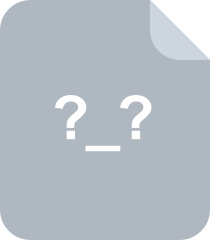
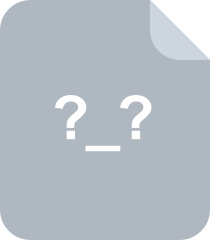
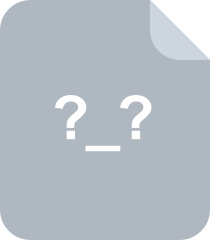
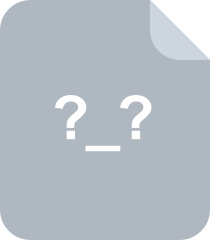
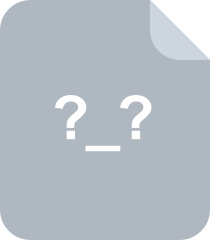
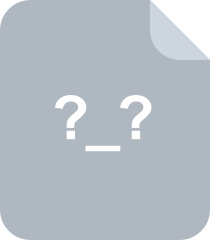
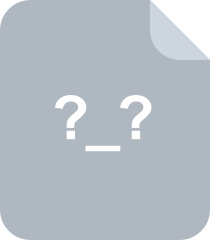
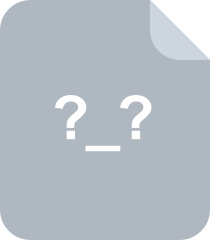
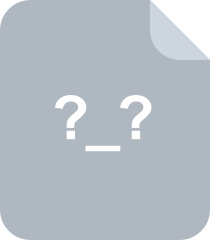


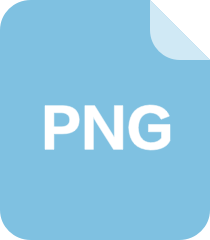
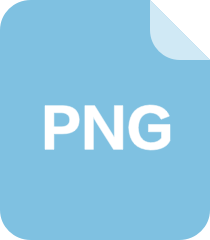
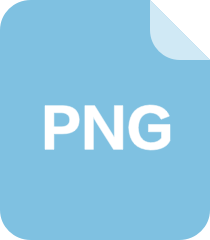
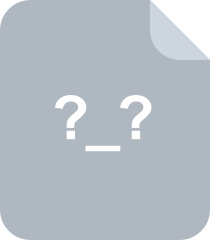

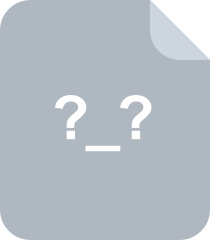
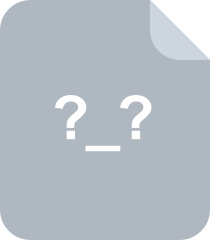

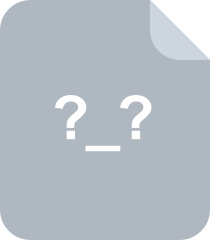

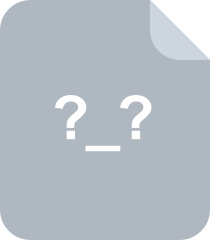
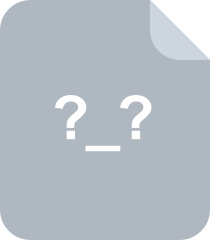
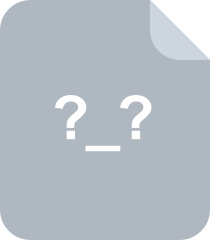

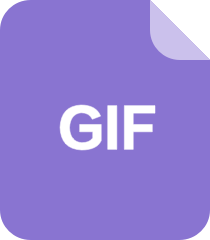
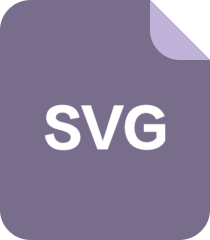
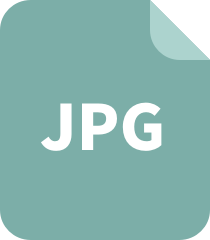
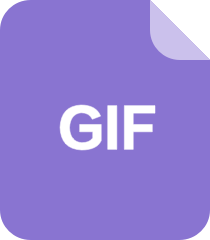
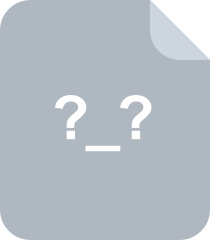

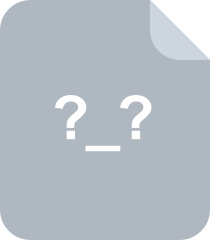
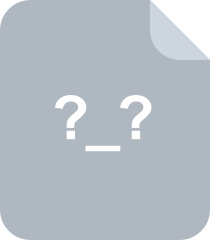
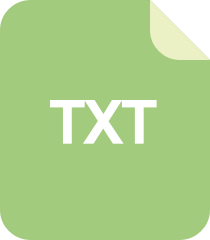

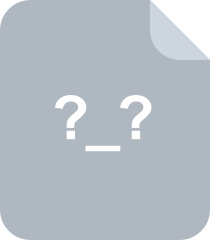


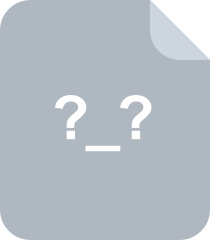

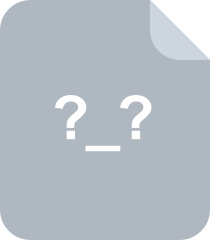
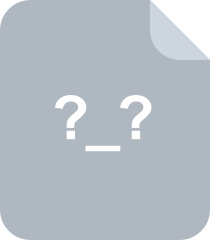
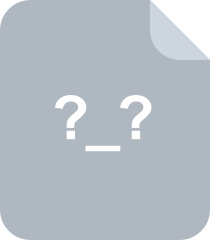
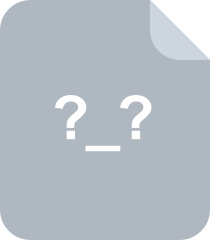
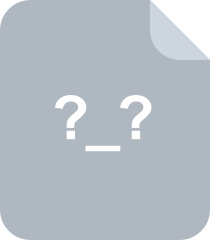
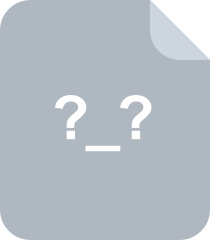
共 49 条
- 1
资源评论
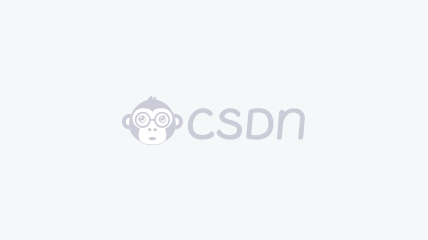

徐浪老师
- 粉丝: 8254
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

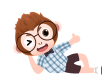
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


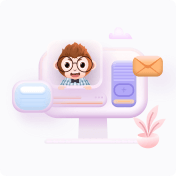
安全验证
文档复制为VIP权益,开通VIP直接复制
