package com.qiangyuyang.demo.widget;
import android.content.Context;
import android.util.AttributeSet;
import android.view.View;
import android.widget.FrameLayout;
import android.widget.LinearLayout;
import android.widget.TextView;
import com.qiangyuyang.demo.R;
/**
* yangqiangyu on 09/01/2017 22:32
* e-mail:168553877@qq.com
* blog:http://blog.csdn.net/yissan
*/
public class CommonLoadingView extends FrameLayout {
//加载时显示文字
protected TextView mLoadingTextTv;
public Context mContext;
//加载错误视图
protected LinearLayout mLoadErrorLl;
//加载错误点击事件处理
private LoadingHandler mLoadingHandler;
//加载view
private View loadingView;
//加载失败view
private View loadingErrorView;
//数据为空
private View emptyView;
public CommonLoadingView(Context context, AttributeSet attrs) {
this(context, attrs, 0);
}
public CommonLoadingView(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
mContext = context;
}
public void setLoadingHandler(LoadingHandler loadingHandler) {
mLoadingHandler = loadingHandler;
}
public void setLoadingErrorView(View loadingErrorView) {
this.removeViewAt(1);
this.loadingErrorView = loadingErrorView;
this.loadingErrorView.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
if (mLoadingHandler != null) {
mLoadingHandler.doRequestData();
CommonLoadingView.this.load();
}
}
});
this.addView(loadingErrorView,1);
}
public void setLoadingView(View loadingView) {
this.removeViewAt(0);
this.loadingView = loadingView;
this.addView(loadingView,0);
}
@Override
protected void onFinishInflate() {
super.onFinishInflate();
loadingView = inflate(mContext, R.layout.common_loading_view, null);
loadingErrorView = inflate(mContext, R.layout.network_layout, null);
emptyView = inflate(mContext, R.layout.empty_layout, null);
this.addView(loadingView);
this.addView(loadingErrorView);
this.addView(emptyView);
loadingErrorView.setVisibility(GONE);
emptyView.setVisibility(GONE);
initView(this);
}
public void setMessage(String message) {
mLoadingTextTv.setText(message);
}
private void initView(View rootView) {
mLoadingTextTv = (TextView) rootView.findViewById(R.id.loading_text_tv);
mLoadErrorLl = (LinearLayout) rootView.findViewById(R.id.load_error_ll);
mLoadErrorLl.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
if (mLoadingHandler != null) {
CommonLoadingView.this.load();
mLoadingHandler.doRequestData();
}
}
});
}
public void load(){
loadingView.setVisibility(VISIBLE);
loadingErrorView.setVisibility(GONE);
emptyView.setVisibility(GONE);
}
public void load(String message){
mLoadingTextTv.setText(message);
loadingView.setVisibility(VISIBLE);
loadingErrorView.setVisibility(GONE);
emptyView.setVisibility(GONE);
}
public void loadSuccess(){
this.loadSuccess(false);
}
public void loadSuccess(boolean isEmpty){
loadingView.setVisibility(GONE);
loadingErrorView.setVisibility(GONE);
if (isEmpty) {
emptyView.setVisibility(VISIBLE);
}else{
emptyView.setVisibility(GONE);
}
}
public void loadError(){
loadingView.setVisibility(GONE);
loadingErrorView.setVisibility(VISIBLE);
}
public interface LoadingHandler{
void doRequestData();
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
Android自定义通用加载view
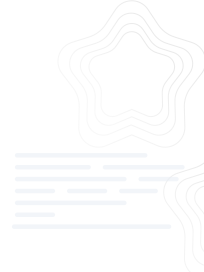
共89个文件
xml:44个
png:13个
bin:6个


温馨提示
1、显示加载视图,加载失败的时候显示加载失败视图,数据为空时显示数据为空视图,支持为失败视图设置点击事件重新加载数据。 2、支持个性化设置,自定义设置 加载、失败、空数据视图。 博客讲解:http://blog.csdn.net/yissan
资源推荐
资源详情
资源评论
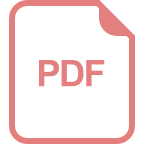
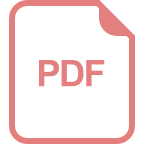
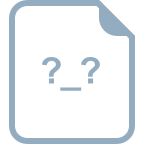
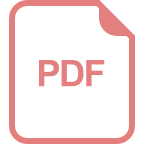
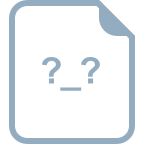
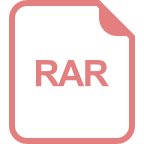
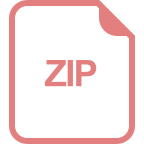
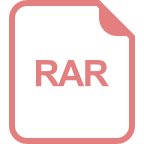
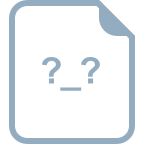
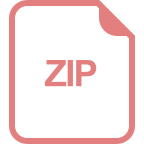
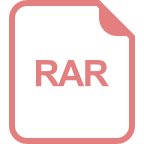
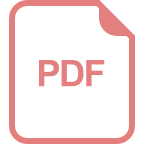
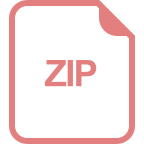
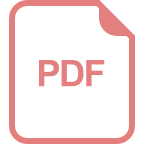
收起资源包目录


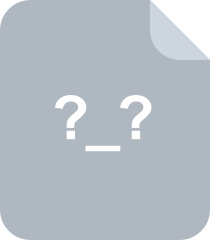
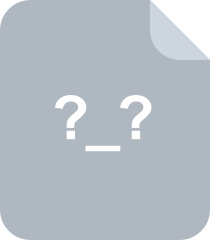
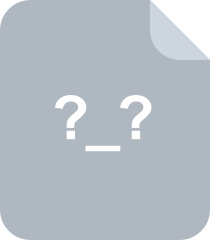
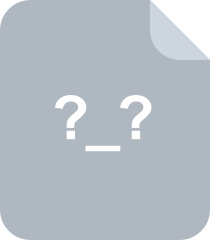


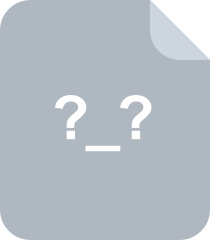
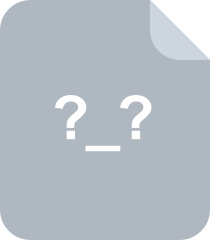
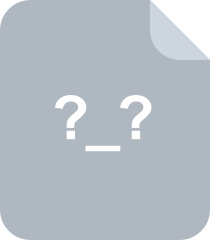
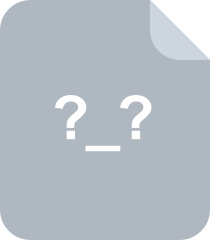
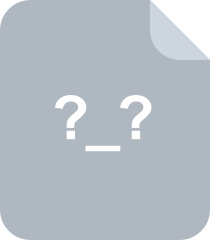
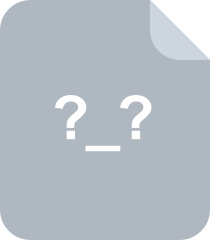
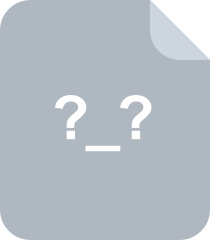
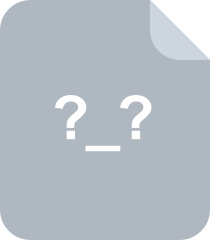
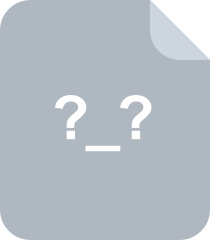
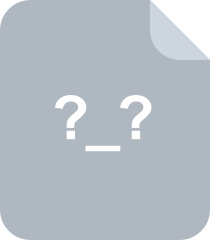
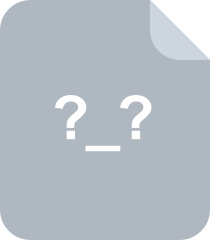
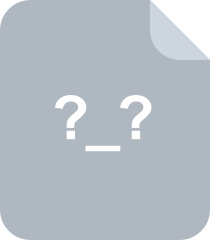
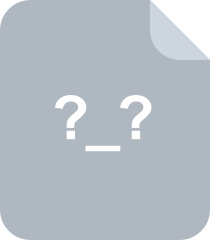
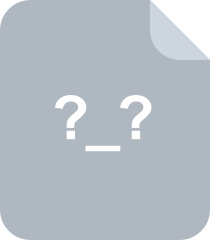
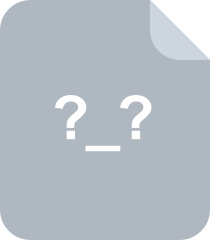
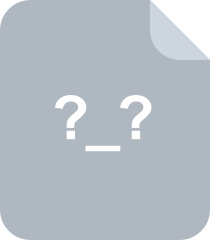
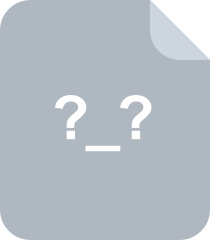
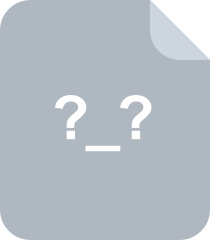
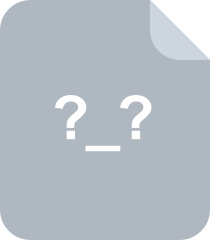
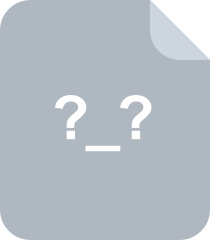
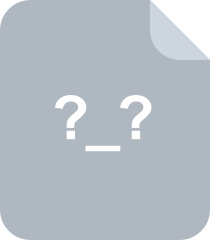
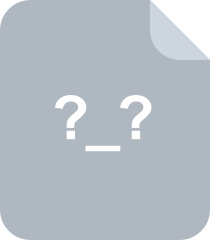
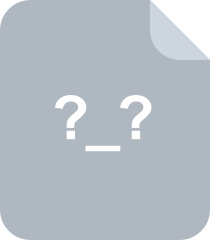
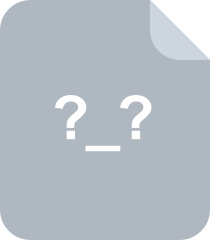
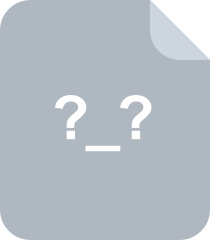
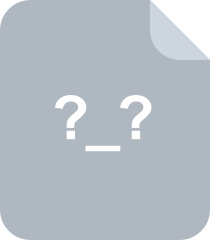
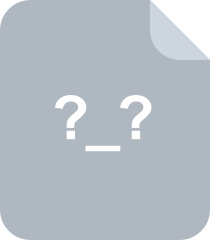
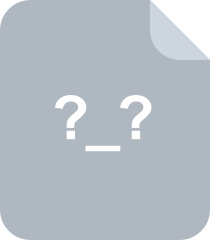
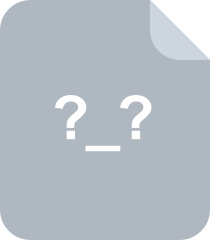
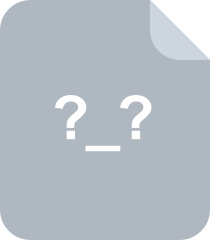

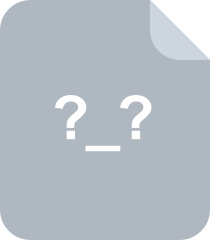


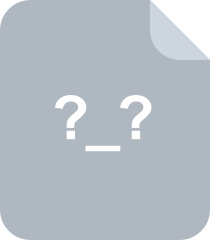
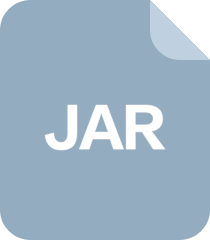





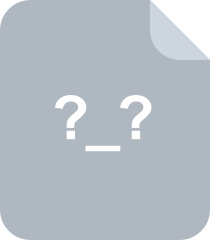
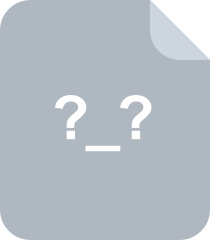

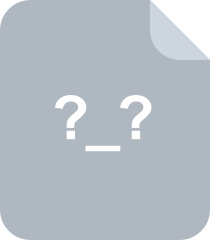
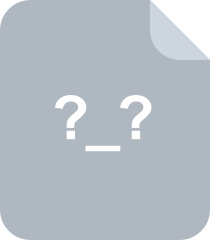

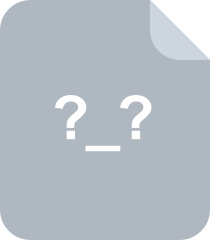
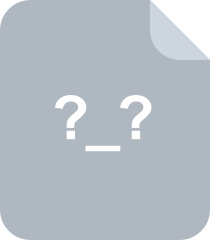
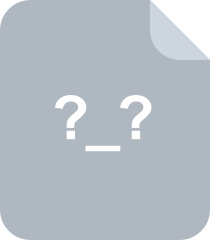
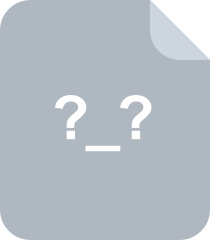
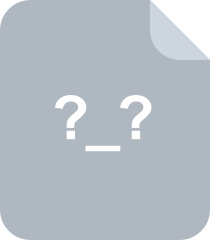
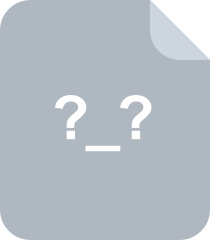
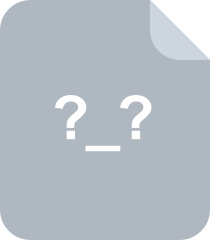
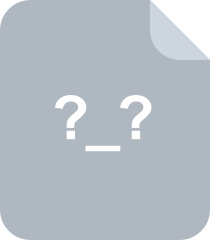
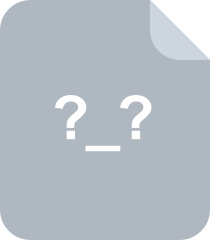
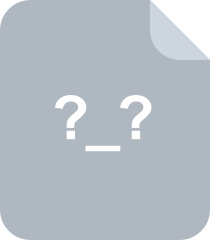

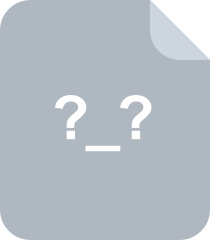






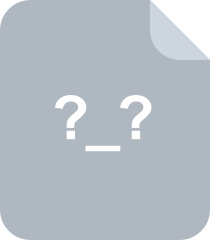





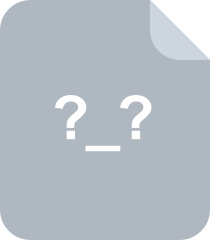

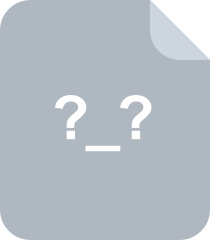


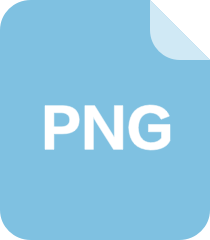

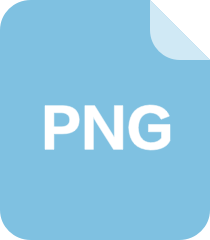

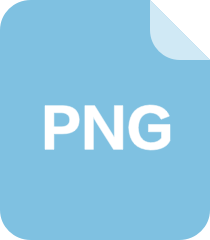

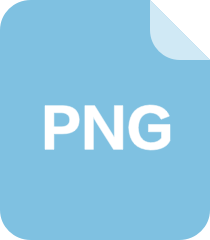
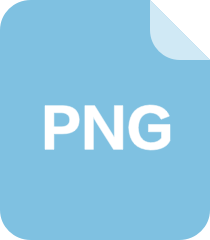

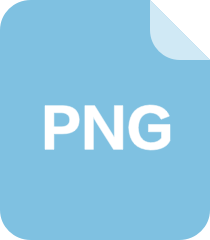

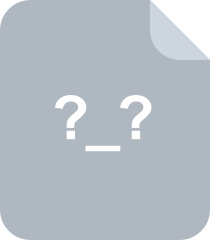

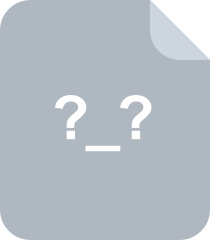
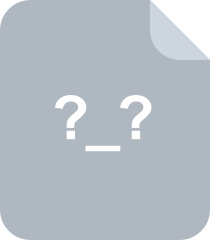
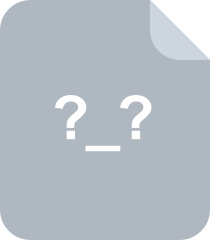
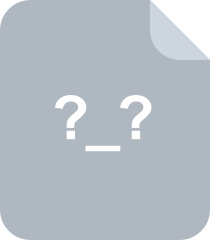
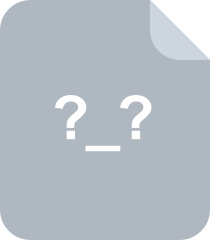
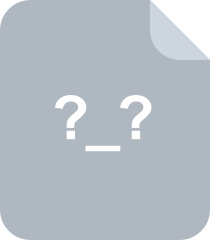

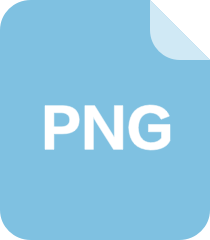
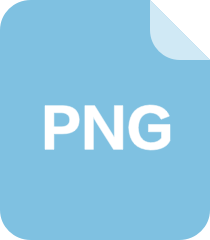

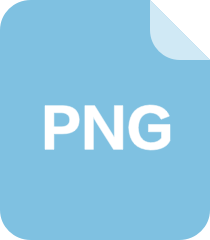
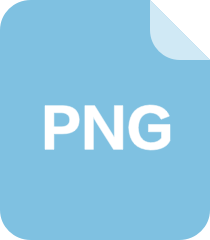

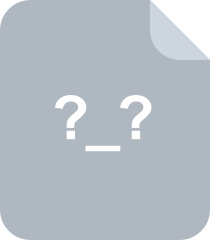

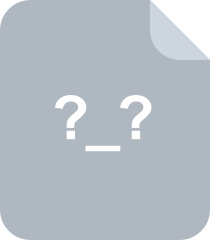
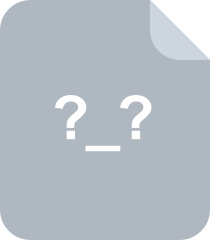
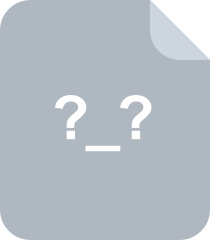
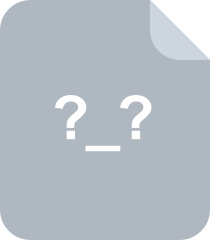

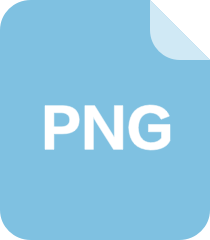
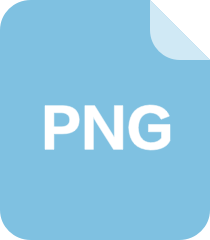

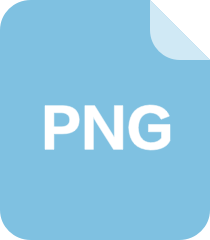





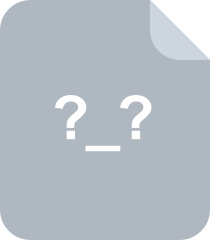
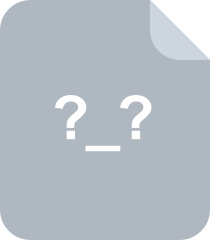
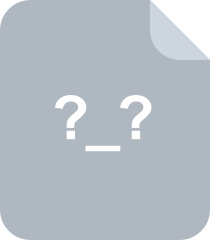
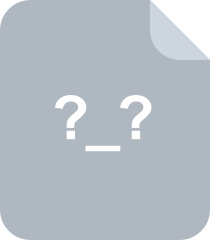

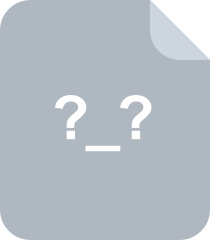
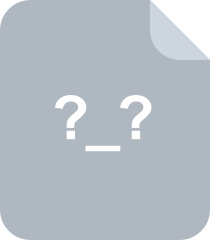
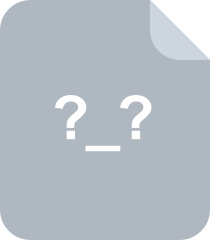
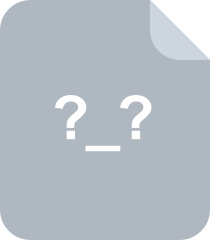
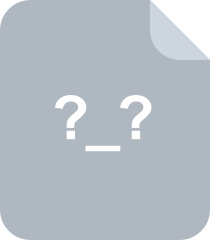
共 89 条
- 1
资源评论
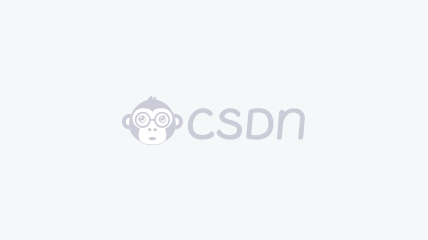
- lwjbzgy2018-07-16不怎么样不怎么样

程序员yqy
- 粉丝: 1335
- 资源: 25
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

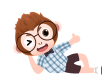
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


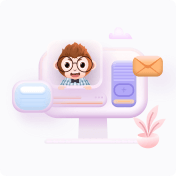
安全验证
文档复制为VIP权益,开通VIP直接复制
