/*
* Copyright (C) 2009 Jeff Sharkey, http://jsharkey.org/
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.jsharkey.sky;
import android.appwidget.AppWidgetManager;
import android.content.ContentProvider;
import android.content.ContentResolver;
import android.content.ContentUris;
import android.content.ContentValues;
import android.content.Context;
import android.content.UriMatcher;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import android.database.sqlite.SQLiteQueryBuilder;
import android.net.Uri;
import android.provider.BaseColumns;
import android.util.Log;
/**
* Provider that holds widget configuration details, and any cached forecast
* data. Provides easy {@link ContentResolver} access to the data when building
* widget updates or showing detailed lists.
*/
public class ForecastProvider extends ContentProvider {
private static final String TAG = "ForecastProvider";
private static final boolean LOGD = true;
public static final String AUTHORITY = "org.jsharkey.sky";
public interface AppWidgetsColumns {
/**
* Title given by user to this widget, usually shown in medium widgets
* and details title bar.
*/
public static final String TITLE = "title";
public static final String LAT = "lat";
public static final String LON = "lon";
/**
* Temperature units to use when displaying forecasts for this widget,
* usually defaults to {@link #UNITS_FAHRENHEIT}.
*/
public static final String UNITS = "units";
public static final int UNITS_FAHRENHEIT = 1;
public static final int UNITS_CELSIUS = 2;
/**
* Last system time when forecasts for this widget were updated, usually
* as read from {@link System#currentTimeMillis()}.
*/
public static final String LAST_UPDATED = "lastUpdated";
/**
* Country code where this widget exists, such as US or FR. This code is
* used when updating forecasts to use the best-available data source.
*/
public static final String COUNTRY_CODE = "countryCode";
/**
* If known, the nearest METAR station to this location. The METAR data
* is used as a fall-back when no better forecast data is available.
*/
public static final String METAR_STATION = "metarStation";
/**
* Flag specifying if this widget has been configured yet, used to skip
* building widget updates.
*/
public static final String CONFIGURED = "configured";
public static final int CONFIGURED_TRUE = 1;
}
public static class AppWidgets implements BaseColumns, AppWidgetsColumns {
public static final Uri CONTENT_URI = Uri.parse("content://" + AUTHORITY + "/appwidgets");
/**
* Directory twig to request all forecasts for a specific widget.
*/
public static final String TWIG_FORECASTS = "forecasts";
/**
* Directory twig to request the forecast nearest the requested time.
*/
public static final String TWIG_FORECAST_AT = "forecast_at";
public static final String CONTENT_TYPE = "vnd.android.cursor.dir/appwidget";
public static final String CONTENT_ITEM_TYPE = "vnd.android.cursor.item/appwidget";
}
public interface ForecastsColumns {
/**
* The parent {@link AppWidgetManager#EXTRA_APPWIDGET_ID} of this
* forecast.
*/
public static final String APPWIDGET_ID = "widgetId";
/**
* Flag if this forecast is an alert.
*/
public static final String ALERT = "alert";
public static final int ALERT_TRUE = 1;
/**
* Timestamp when this forecast becomes valid, in base ready for
* comparison with {@link System#currentTimeMillis()}.
*/
public static final String VALID_START = "validStart";
/**
* High temperature during this forecast period, stored in Fahrenheit.
*/
public static final String TEMP_HIGH = "tempHigh";
/**
* Low temperature during this forecast period, stored in Fahrenheit.
*/
public static final String TEMP_LOW = "tempLow";
/**
* String describing the weather conditions.
*/
public static final String CONDITIONS = "conditions";
/**
* Web link where more details can be found about this forecast.
*/
public static final String URL = "url";
}
public static class Forecasts implements BaseColumns, ForecastsColumns {
public static final Uri CONTENT_URI = Uri.parse("content://" + AUTHORITY + "/forecasts");
public static final String CONTENT_TYPE = "vnd.android.cursor.dir/forecast";
public static final String CONTENT_ITEM_TYPE = "vnd.android.cursor.item/forecast";
}
private static final String TABLE_APPWIDGETS = "appwidgets";
private static final String TABLE_FORECASTS = "forecasts";
private DatabaseHelper mOpenHelper;
/**
* Helper to manage upgrading between versions of the forecast database.
*/
private static class DatabaseHelper extends SQLiteOpenHelper {
private static final String DATABASE_NAME = "forecasts.db";
private static final int VER_ORIGINAL = 2;
private static final int VER_ADD_METAR = 3;
private static final int DATABASE_VERSION = VER_ADD_METAR;
public DatabaseHelper(Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL("CREATE TABLE " + TABLE_APPWIDGETS + " ("
+ BaseColumns._ID + " INTEGER PRIMARY KEY,"
+ AppWidgetsColumns.TITLE + " TEXT,"
+ AppWidgetsColumns.LAT + " REAL,"
+ AppWidgetsColumns.LON + " REAL,"
+ AppWidgetsColumns.UNITS + " INTEGER,"
+ AppWidgetsColumns.LAST_UPDATED + " INTEGER,"
+ AppWidgetsColumns.COUNTRY_CODE + " TEXT,"
+ AppWidgetsColumns.CONFIGURED + " INTEGER);");
db.execSQL("CREATE TABLE " + TABLE_FORECASTS + " ("
+ BaseColumns._ID + " INTEGER PRIMARY KEY AUTOINCREMENT,"
+ ForecastsColumns.APPWIDGET_ID + " INTEGER,"
+ ForecastsColumns.ALERT + " INTEGER DEFAULT 0,"
+ ForecastsColumns.VALID_START + " INTEGER,"
+ ForecastsColumns.TEMP_HIGH + " INTEGER,"
+ ForecastsColumns.TEMP_LOW + " INTEGER,"
+ ForecastsColumns.CONDITIONS + " TEXT,"
+ ForecastsColumns.URL + " TEXT);");
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
int version = oldVersion;
switch (version) {
case VER_ORIGINAL:
db.execSQL("ALTER TABLE " + TABLE_APPWIDGETS + " ADD COLUMN "
+ AppWidgetsColumns.COUNTRY_CODE + " TEXT");
db.execSQL("ALTER TABLE " + TABLE_APPWIDGETS + " ADD COLUMN "
+ AppWidgetsColumns.METAR_STATION + " TEXT"
没有合适的资源?快使用搜索试试~ 我知道了~
Android 天气预报加widget源码.zip
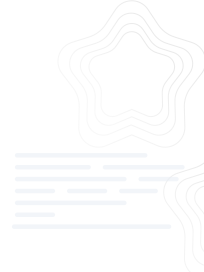
共212个文件
svn-base:81个
class:33个
all-wcprops:22个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 80 浏览量
2022-06-16
15:11:57
上传
评论
收藏 551KB ZIP 举报
温馨提示
Android 天气预报加widget源码.zip
资源推荐
资源详情
资源评论
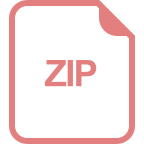
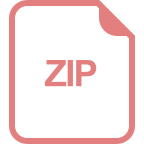
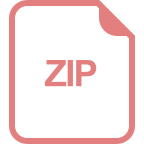
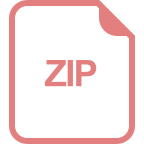
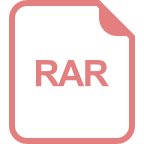
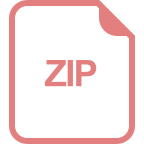
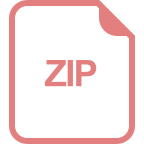
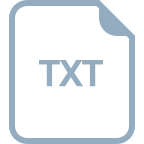
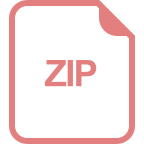
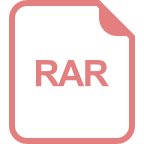
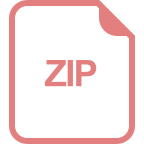
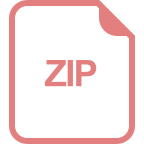
收起资源包目录

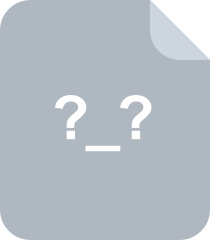
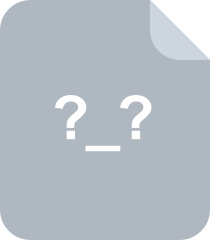
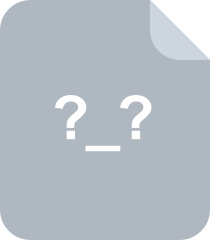
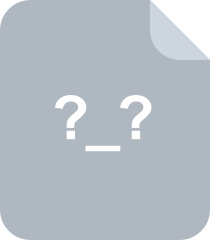
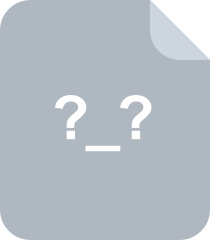
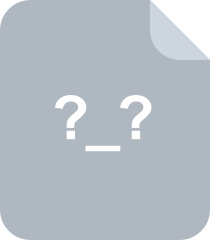
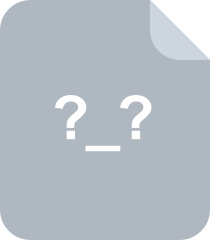
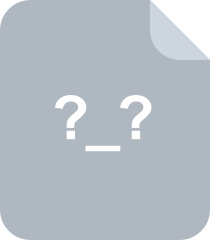
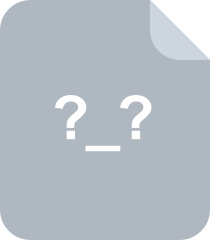
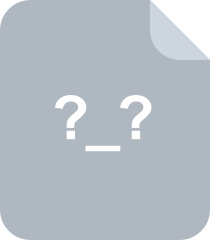
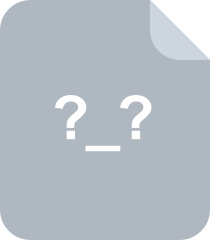
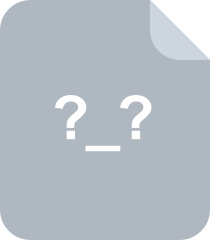
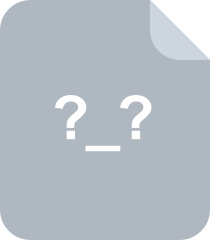
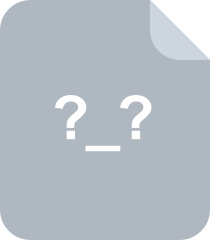
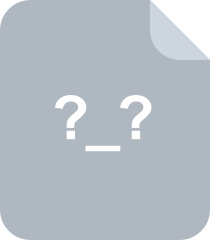
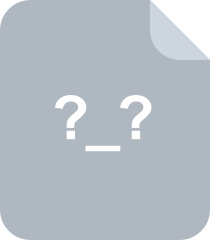
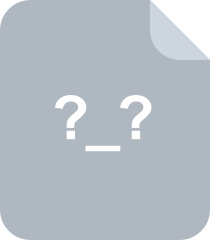
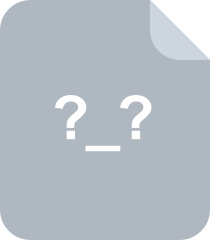
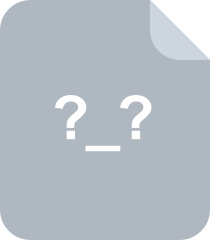
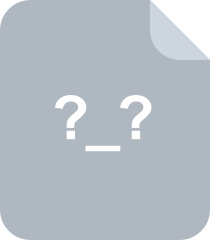
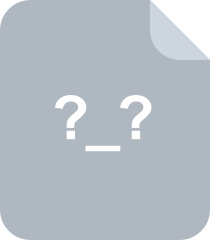
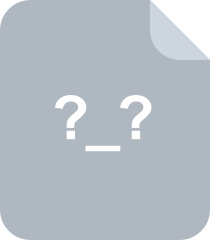
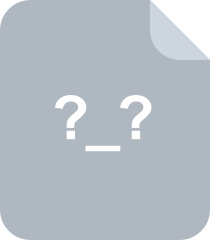
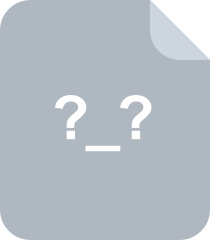
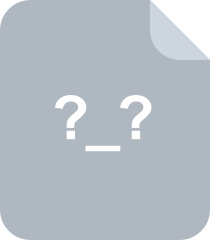
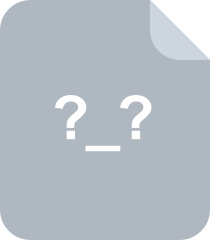
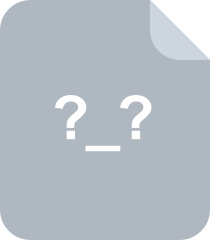
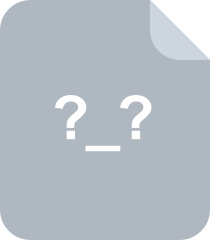
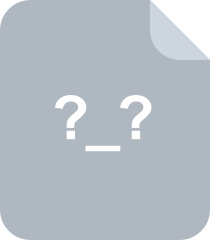
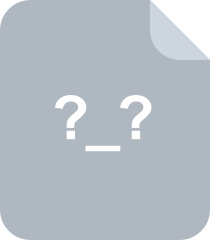
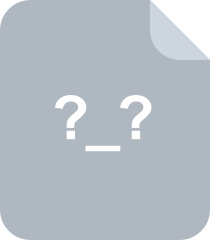
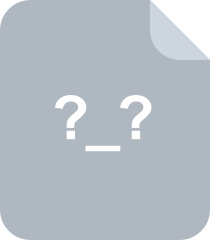
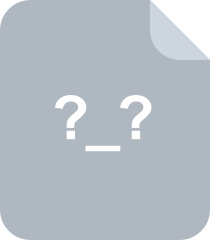
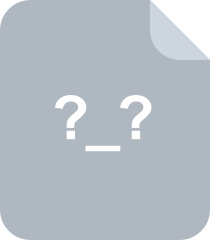
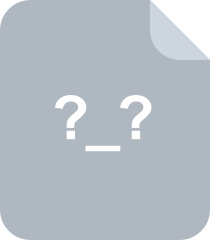
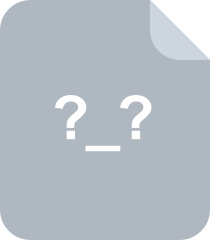
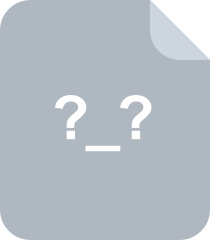
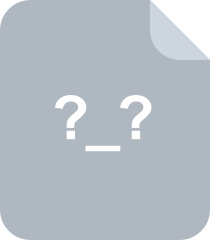
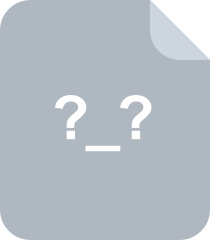
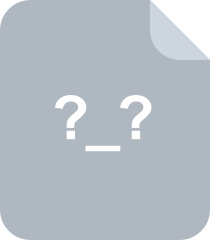
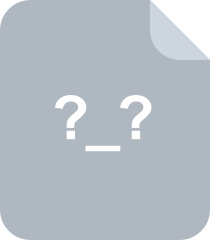
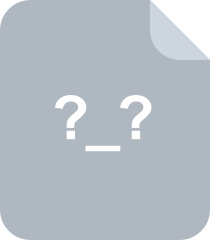
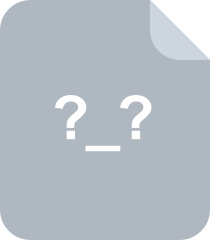
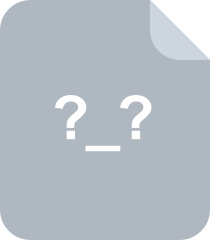
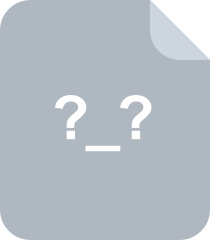
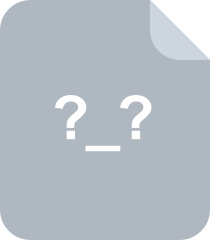
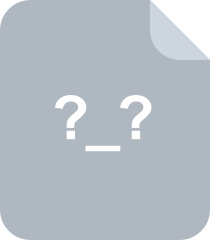
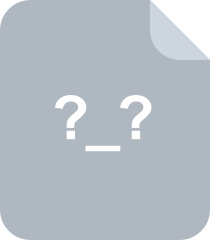
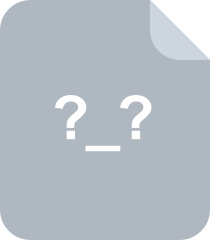
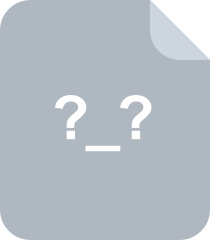
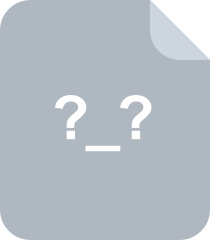
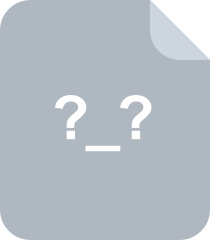
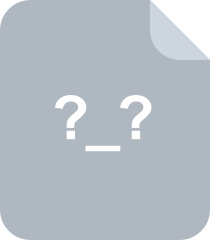
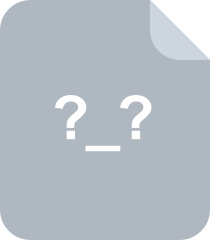
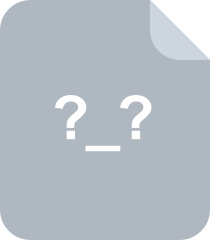
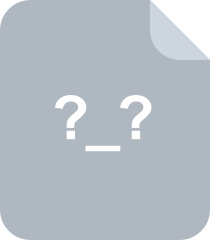
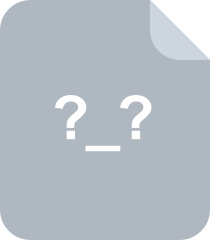
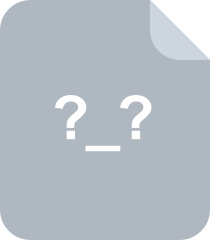
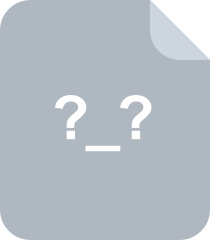
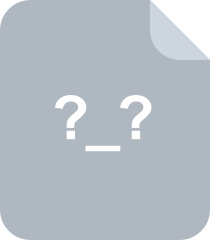
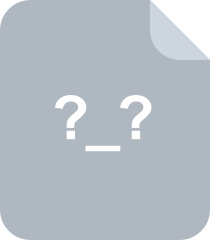
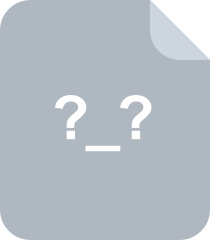
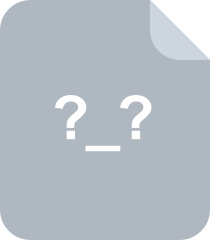
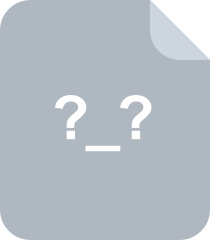
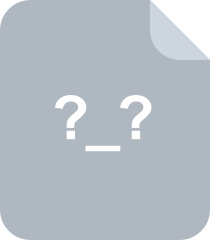
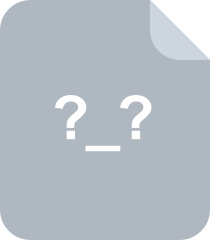
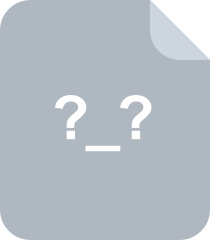
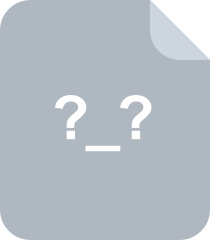
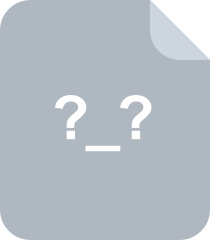
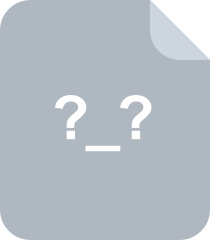
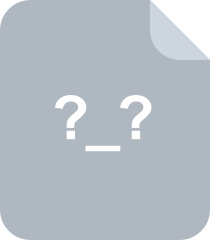
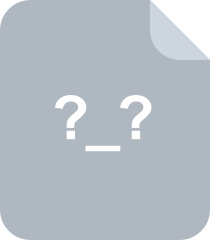
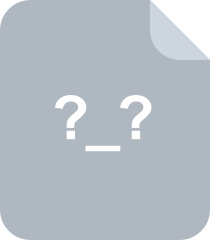
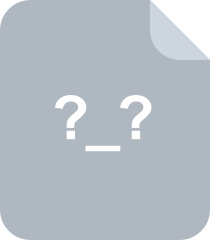
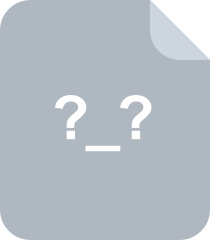
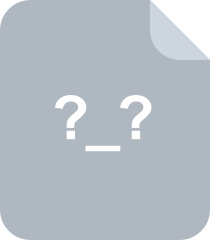
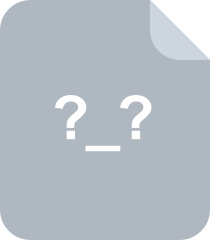
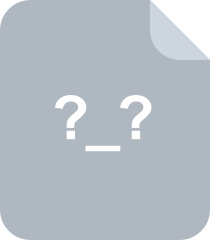
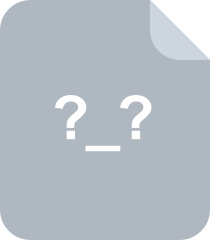
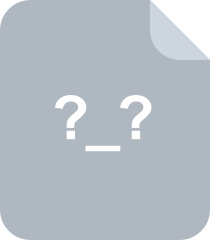
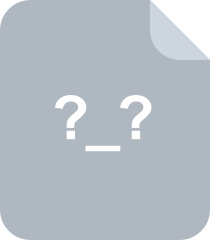
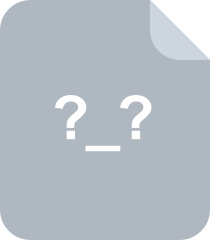
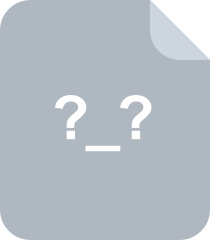
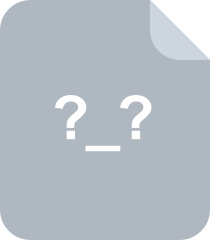
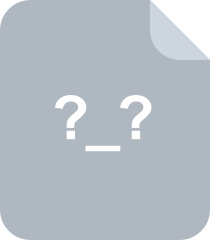
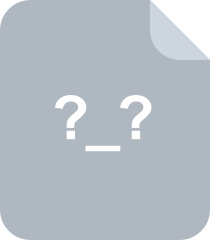
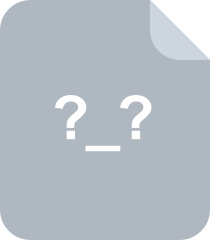
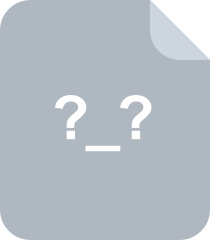
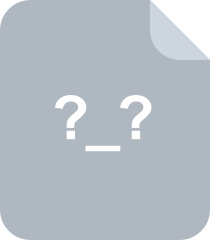
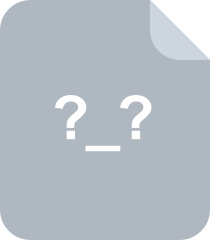
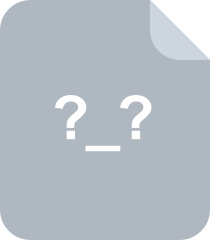
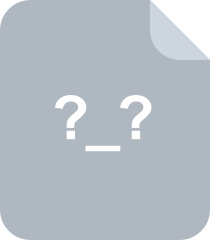
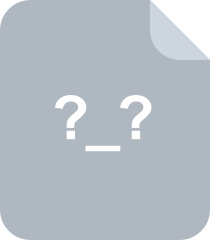
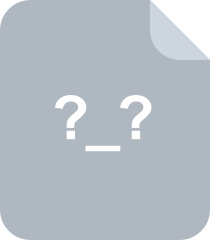
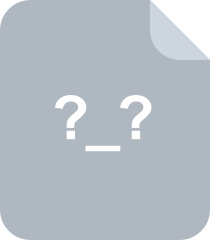
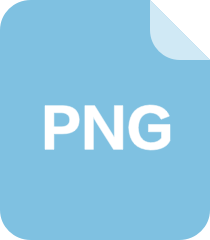
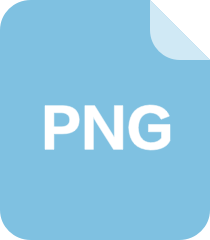
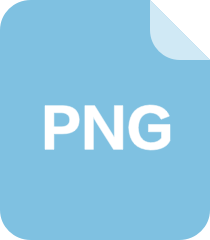
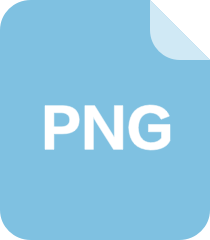
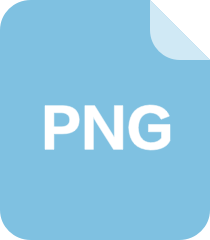
共 212 条
- 1
- 2
- 3
资源评论
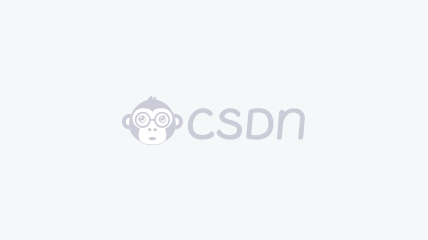

BryanDing
- 粉丝: 299
- 资源: 5583
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

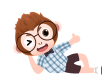
安全验证
文档复制为VIP权益,开通VIP直接复制
