c# FTP 上传 下载
需积分: 4 176 浏览量
2010-07-02
01:39:20
上传
评论 2
收藏 22KB TXT 举报

zyy216021
- 粉丝: 0
- 资源: 1
最新资源
- 基于stm32f103c8t6的简易VFD时钟
- 3D-MIMO从原理到技术.docx
- 5G SA被拒原因值汇总.xls
- Clearly-Reader
- 什么是yolov5网络结构图以及学习yolov5网络结构图的意义
- 什么是lstm以及学习lstm的意义是什么
- anaconda安装的内容是什么以及学习anaconda安装的意义
- Apifox Browser Extension
- 基于matlab实现证据理论中用于求取Jousselme证据距离的matlab 代码,自己编的哦!-.rar
- 这是我从网上下载到的(LLE)的基于matlab实现,希望对研究Manifold Leanring的同事有所帮助.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


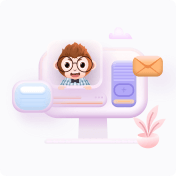