/**
* Copyright (c) 2004-2011 QOS.ch
* All rights reserved.
*
* Permission is hereby granted, free of charge, to any person obtaining
* a copy of this software and associated documentation files (the
* "Software"), to deal in the Software without restriction, including
* without limitation the rights to use, copy, modify, merge, publish,
* distribute, sublicense, and/or sell copies of the Software, and to
* permit persons to whom the Software is furnished to do so, subject to
* the following conditions:
*
* The above copyright notice and this permission notice shall be
* included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
* MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
* LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
* OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
* WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
*
*/
package org.slf4j.ext;
import org.slf4j.Logger;
import org.slf4j.Marker;
import org.slf4j.helpers.FormattingTuple;
import org.slf4j.helpers.MessageFormatter;
import org.slf4j.spi.LocationAwareLogger;
/**
* A helper class wrapping an {@link org.slf4j.Logger} instance preserving
* location information if the wrapped instance supports it.
*
* @author Ralph Goers
* @author Ceki Gülcü
*/
public class LoggerWrapper implements Logger {
// To ensure consistency between two instances sharing the same name
// (homonyms)
// a LoggerWrapper should not contain any state beyond
// the Logger instance it wraps.
// Note that 'instanceofLAL' directly depends on Logger.
// fqcn depend on the caller, but its value would not be different
// between successive invocations of a factory class
protected final Logger logger;
final String fqcn;
// is this logger instance a LocationAwareLogger
protected final boolean instanceofLAL;
public LoggerWrapper(Logger logger, String fqcn) {
this.logger = logger;
this.fqcn = fqcn;
if (logger instanceof LocationAwareLogger) {
instanceofLAL = true;
} else {
instanceofLAL = false;
}
}
/**
* Delegate to the appropriate method of the underlying logger.
*/
public boolean isTraceEnabled() {
return logger.isTraceEnabled();
}
/**
* Delegate to the appropriate method of the underlying logger.
*/
public boolean isTraceEnabled(Marker marker) {
return logger.isTraceEnabled(marker);
}
/**
* Delegate to the appropriate method of the underlying logger.
*/
public void trace(String msg) {
if (!logger.isTraceEnabled())
return;
if (instanceofLAL) {
((LocationAwareLogger) logger).log(null, fqcn, LocationAwareLogger.TRACE_INT, msg, null, null);
} else {
logger.trace(msg);
}
}
/**
* Delegate to the appropriate method of the underlying logger.
*/
public void trace(String format, Object arg) {
if (!logger.isTraceEnabled())
return;
if (instanceofLAL) {
String formattedMessage = MessageFormatter.format(format, arg).getMessage();
((LocationAwareLogger) logger).log(null, fqcn, LocationAwareLogger.TRACE_INT, formattedMessage, new Object[] { arg }, null);
} else {
logger.trace(format, arg);
}
}
/**
* Delegate to the appropriate method of the underlying logger.
*/
public void trace(String format, Object arg1, Object arg2) {
if (!logger.isTraceEnabled())
return;
if (instanceofLAL) {
String formattedMessage = MessageFormatter.format(format, arg1, arg2).getMessage();
((LocationAwareLogger) logger).log(null, fqcn, LocationAwareLogger.TRACE_INT, formattedMessage, new Object[] { arg1, arg2 }, null);
} else {
logger.trace(format, arg1, arg2);
}
}
/**
* Delegate to the appropriate method of the underlying logger.
*/
public void trace(String format, Object... args) {
if (!logger.isTraceEnabled())
return;
if (instanceofLAL) {
String formattedMessage = MessageFormatter.arrayFormat(format, args).getMessage();
((LocationAwareLogger) logger).log(null, fqcn, LocationAwareLogger.TRACE_INT, formattedMessage, args, null);
} else {
logger.trace(format, args);
}
}
/**
* Delegate to the appropriate method of the underlying logger.
*/
public void trace(String msg, Throwable t) {
if (!logger.isTraceEnabled())
return;
if (instanceofLAL) {
((LocationAwareLogger) logger).log(null, fqcn, LocationAwareLogger.TRACE_INT, msg, null, t);
} else {
logger.trace(msg, t);
}
}
/**
* Delegate to the appropriate method of the underlying logger.
*/
public void trace(Marker marker, String msg) {
if (!logger.isTraceEnabled(marker))
return;
if (instanceofLAL) {
((LocationAwareLogger) logger).log(marker, fqcn, LocationAwareLogger.TRACE_INT, msg, null, null);
} else {
logger.trace(marker, msg);
}
}
/**
* Delegate to the appropriate method of the underlying logger.
*/
public void trace(Marker marker, String format, Object arg) {
if (!logger.isTraceEnabled(marker))
return;
if (instanceofLAL) {
String formattedMessage = MessageFormatter.format(format, arg).getMessage();
((LocationAwareLogger) logger).log(marker, fqcn, LocationAwareLogger.TRACE_INT, formattedMessage, new Object[] { arg }, null);
} else {
logger.trace(marker, format, arg);
}
}
/**
* Delegate to the appropriate method of the underlying logger.
*/
public void trace(Marker marker, String format, Object arg1, Object arg2) {
if (!logger.isTraceEnabled(marker))
return;
if (instanceofLAL) {
String formattedMessage = MessageFormatter.format(format, arg1, arg2).getMessage();
((LocationAwareLogger) logger).log(marker, fqcn, LocationAwareLogger.TRACE_INT, formattedMessage, new Object[] { arg1, arg2 }, null);
} else {
logger.trace(marker, format, arg1, arg2);
}
}
/**
* Delegate to the appropriate method of the underlying logger.
*/
public void trace(Marker marker, String format, Object... args) {
if (!logger.isTraceEnabled(marker))
return;
if (instanceofLAL) {
String formattedMessage = MessageFormatter.arrayFormat(format, args).getMessage();
((LocationAwareLogger) logger).log(marker, fqcn, LocationAwareLogger.TRACE_INT, formattedMessage, args, null);
} else {
logger.trace(marker, format, args);
}
}
/**
* Delegate to the appropriate method of the underlying logger.
*/
public void trace(Marker marker, String msg, Throwable t) {
if (!logger.isTraceEnabled(marker))
return;
if (instanceofLAL) {
((LocationAwareLogger) logger).log(marker, fqcn, LocationAwareLogger.TRACE_INT, msg, null, t);
} else {
logger.trace(marker, msg, t);
}
}
/**
* Delegate to the appropriate method of the underlying logger.
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
slf4j、log4j等jar包 Log4j是Apache的一个开源项目,通过使用Log4j,我们可以控制日志信息输送的目的地是控制台、文件、GUI组件,甚至是套接口服务器、NT的事件记录器、UNIX Syslog守护进程等;我们也可以控制每一条日志的输出格式;通过定义每一条日志信息的级别,我们能够更加细致地控制日志的生成过程。最令人感兴趣的就是,这些可以通过一个配置文件来灵活地进行配置,而不需要修改应用的代码。
资源推荐
资源详情
资源评论
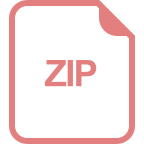
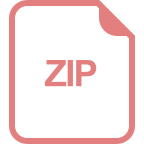
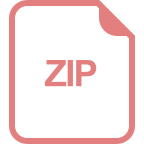
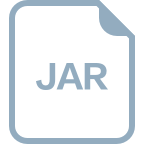
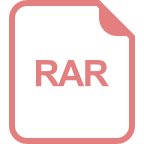
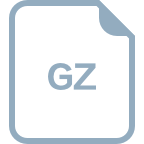
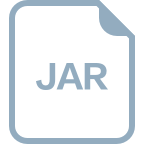
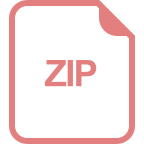
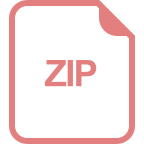
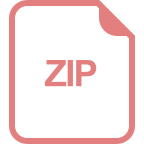
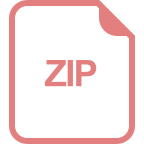
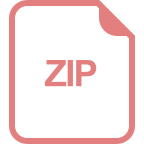
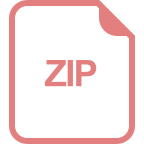
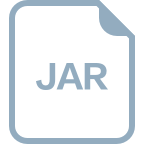
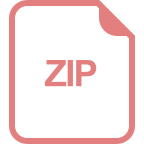
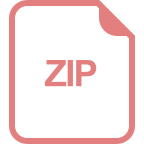
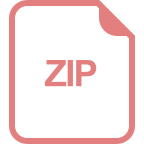
收起资源包目录

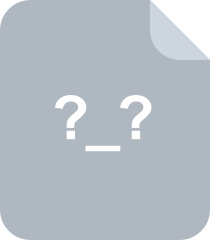
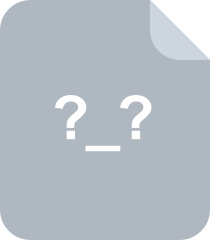
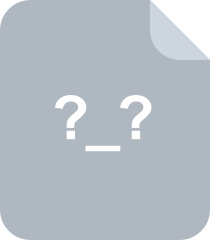
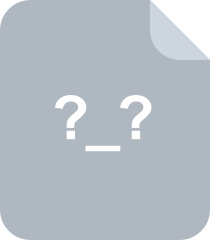
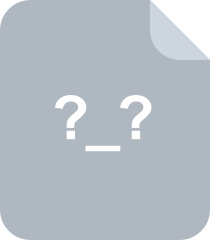
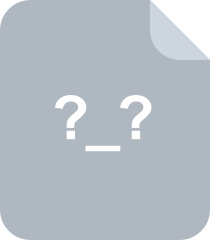
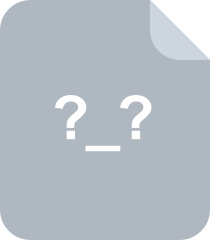
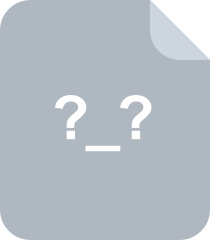
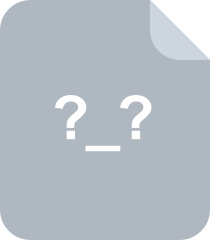
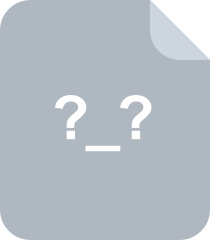
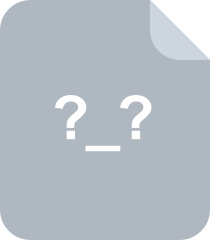
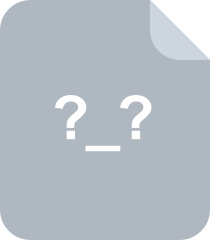
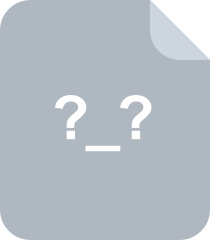
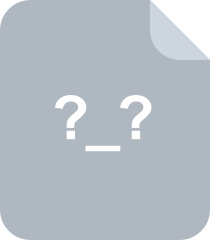
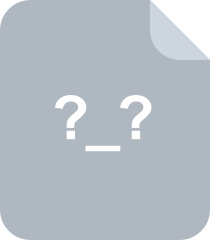
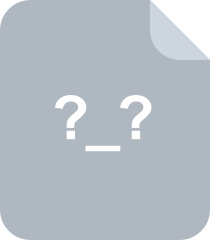
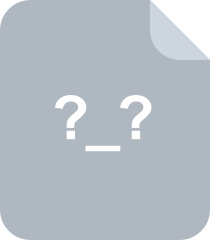
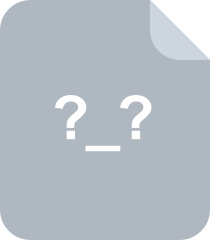
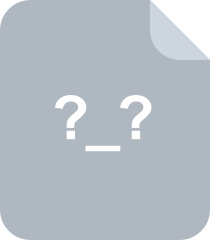
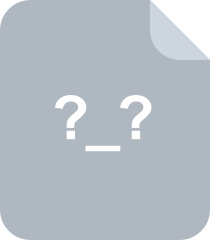
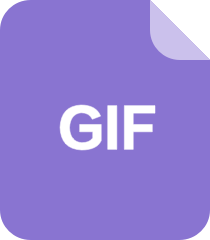
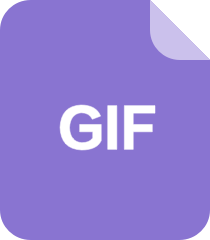
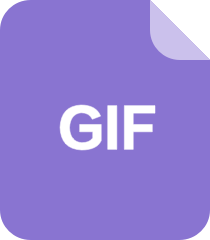
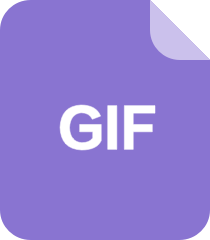
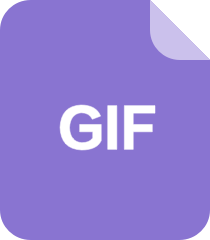
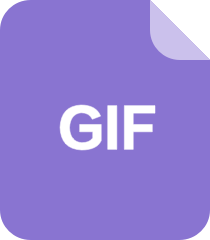
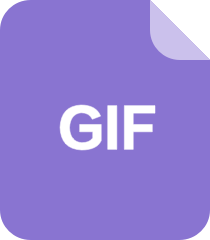
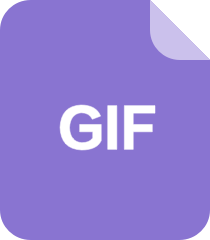
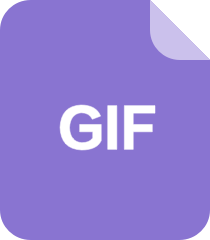
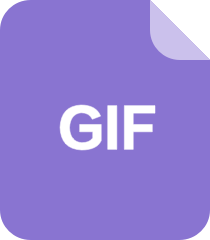
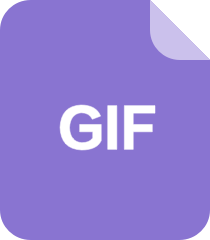
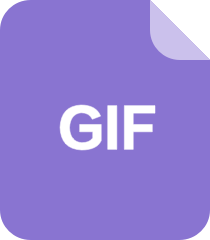
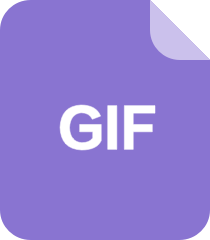
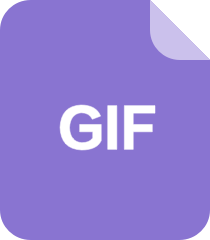
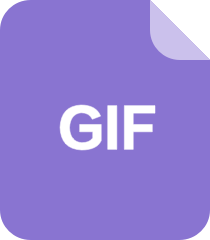
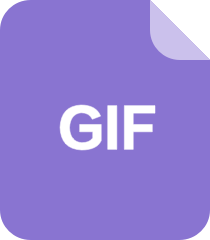
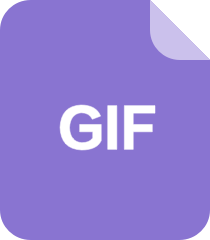
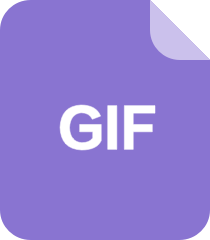
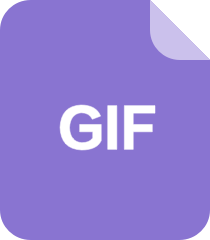
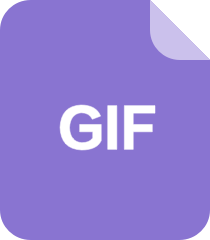
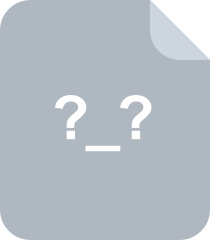
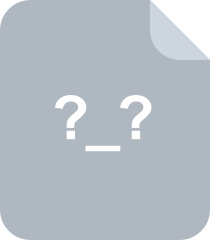
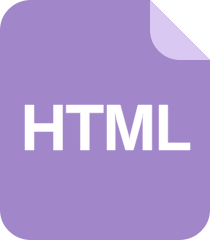
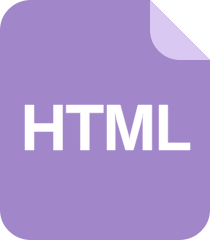
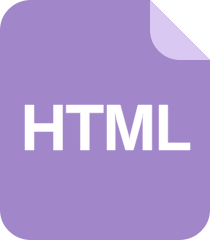
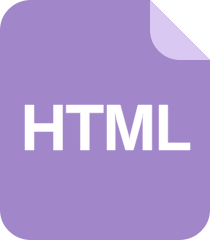
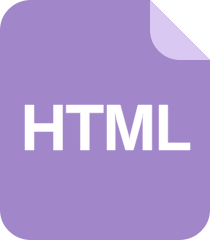
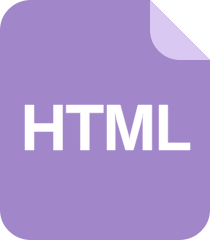
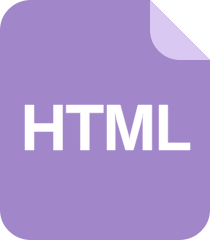
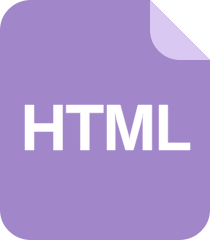
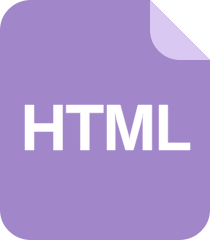
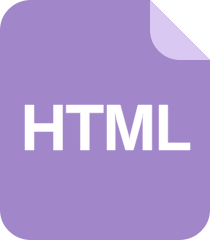
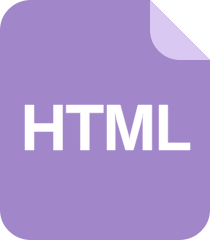
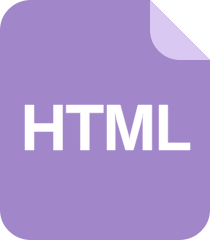
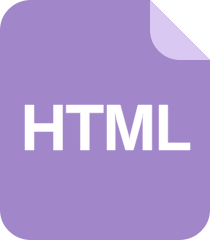
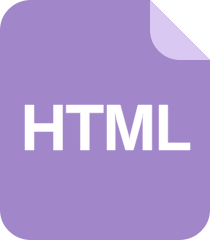
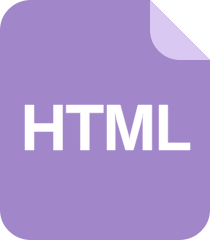
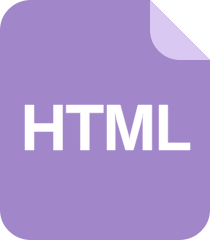
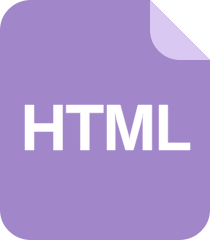
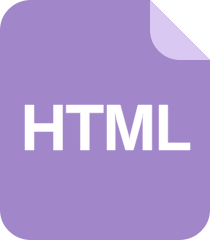
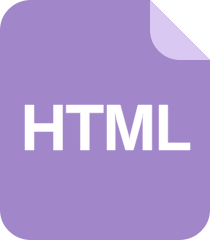
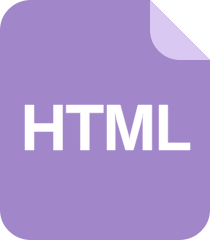
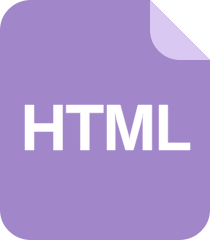
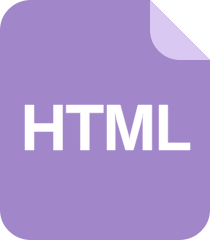
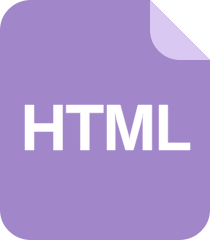
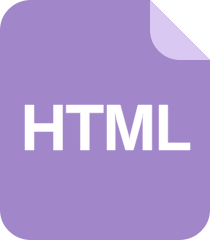
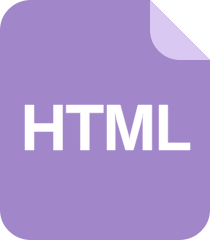
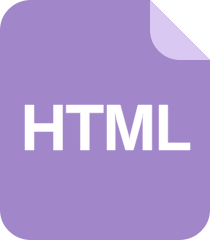
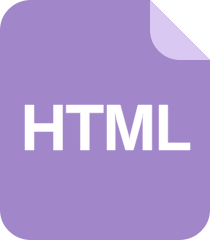
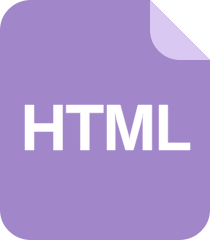
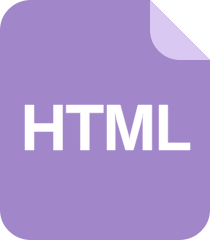
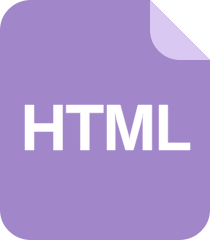
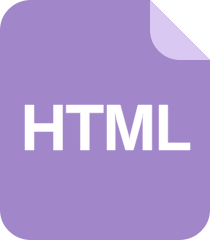
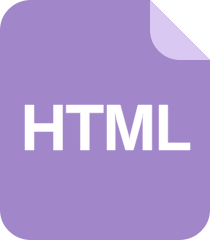
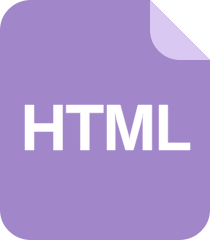
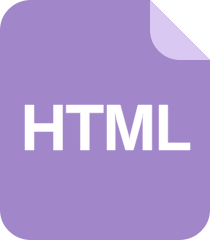
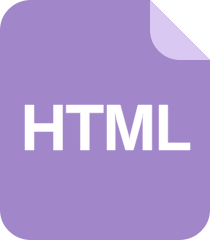
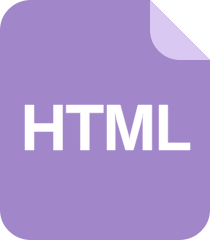
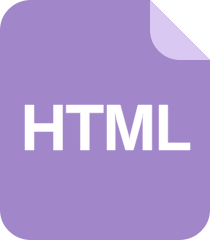
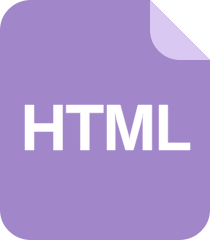
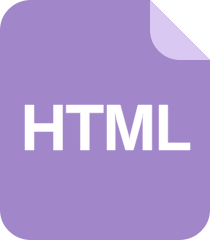
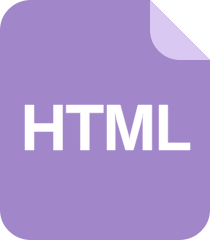
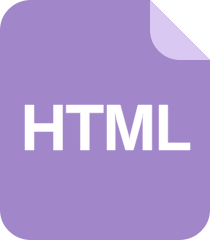
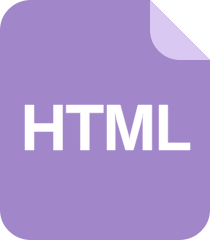
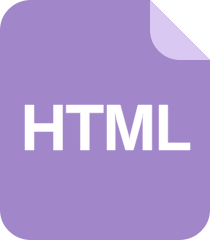
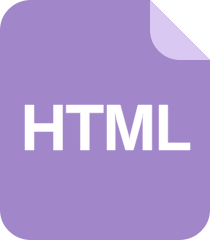
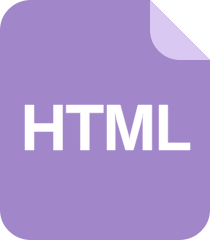
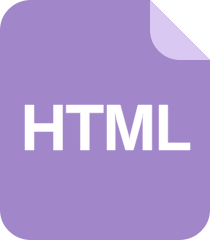
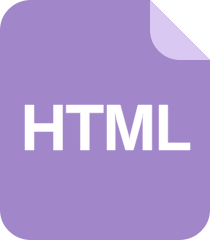
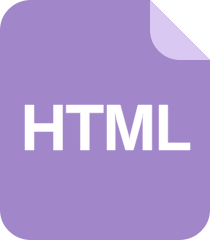
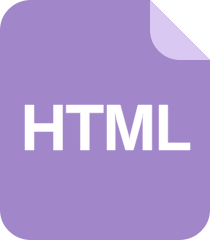
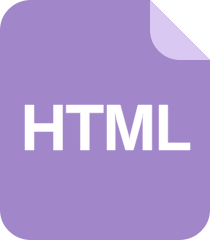
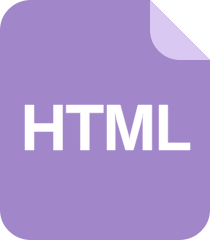
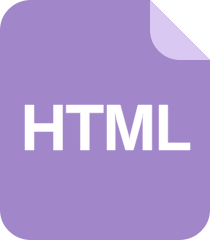
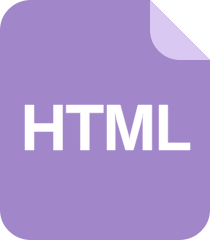
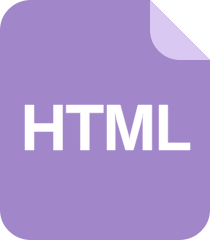
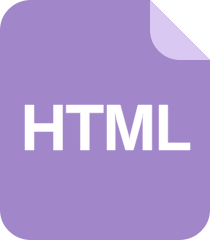
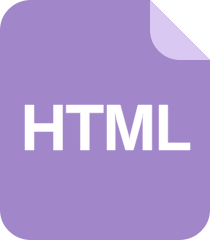
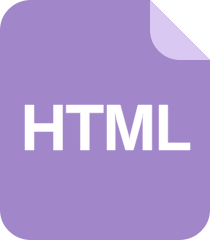
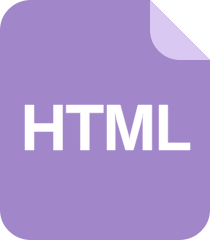
共 1501 条
- 1
- 2
- 3
- 4
- 5
- 6
- 16
资源评论
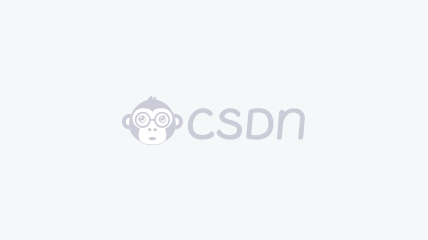

故事つ
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

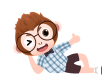
最新资源
- RxJava 2 和 Retrofit 结合使用的几个最常见的使用方式举例.zip
- RxJava 2 Android 示例 - 如何在 Android 中使用 RxJava 2.zip
- 上传OpenCV开发资源OpenCv开发资源
- Spring Boot与Vue 3前后端分离技术详解及应用
- C#开发的一款批量查快递批量分析物流状态的winform应用软件
- PubNub JavaScript SDK 文档.zip
- paho.mqtt.javascript.zip
- Packt 发布的《Java 编码问题》.zip
- OpenTelemetry Java SDK.zip
- OBD-II Java API.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


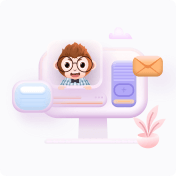
安全验证
文档复制为VIP权益,开通VIP直接复制
