package com.sut.controller;
import com.sut.model.User;
import com.sut.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.servlet.ModelAndView;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
@Controller
@RequestMapping("/user")
public class UserController {
@Autowired
UserService userService;
@RequestMapping(path = "/employee", method = RequestMethod.GET)
@ResponseBody
public List<User> employeeList() {
List<User> result = userService.employeeList();
return result;
}
@RequestMapping(path = "/customer", method = RequestMethod.GET)
@ResponseBody
public List<User> customerList() {
List<User> result = userService.customerList();
return result;
}
@RequestMapping(path = "/customer/{id}", method = RequestMethod.GET)
@ResponseBody
public User getCustomer(@PathVariable Integer id) {
User result = userService.getCustomer(id);
return result;
}
@RequestMapping(path = "/employee/{id}", method = RequestMethod.GET)
@ResponseBody
public User getEmployee(@PathVariable Integer id) {
User result = userService.getEmployee(id);
return result;
}
@RequestMapping(path = "/employee/", method = RequestMethod.PUT)
@ResponseBody
public Map<String, Object> editEmployee(@RequestBody User user) {
Map<String, Object> map = new HashMap<>();
if (userService.editEmployee(user) > 0) {
map.put("result", "SUCCESS");
} else {
map.put("result", "没有更新");
}
return map;
}
@RequestMapping(path = "/employee/", method = RequestMethod.POST)
public ModelAndView addEmployee(User user) {
Map<String, Object> map = new HashMap<>();
if (userService.addEmployee(user) > 0) {
map.put("result", "SUCCESS");
} else {
map.put("result", "添加失败");
}
return new ModelAndView("admin/employee", map);
}
@RequestMapping(path = "/employee/", method = RequestMethod.DELETE)
@ResponseBody
public Map<String, Object> deleteEmployee(@RequestBody int id) {
Map<String, Object> map = new HashMap<>();
if (userService.deleteEmployee(id) > 0) {
map.put("result", "SUCCESS");
} else {
map.put("result", "删除失败");
}
return map;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。毕业设计、课程设计源码文件,已经过测试可以直接使用。
资源推荐
资源详情
资源评论
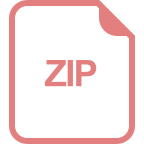
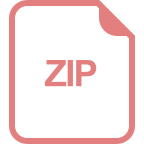
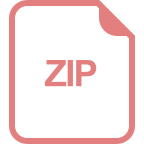
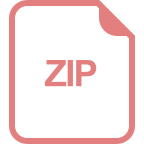
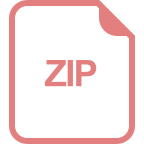
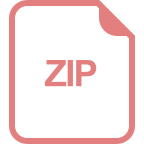
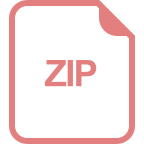
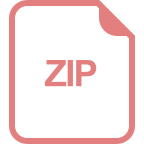
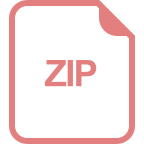
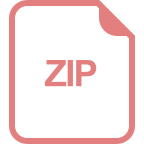
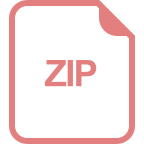
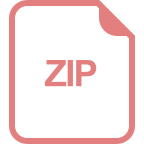
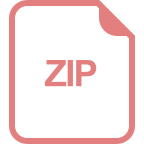
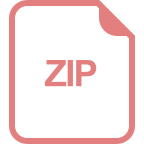
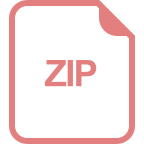
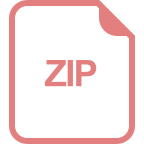
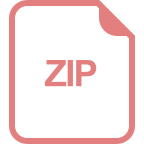
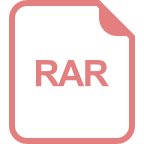
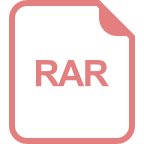
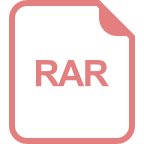
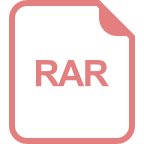
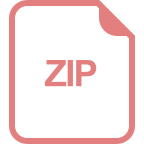
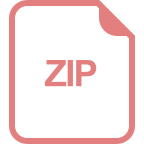
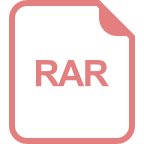
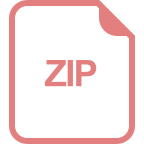
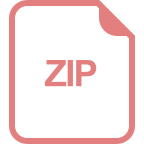
收起资源包目录


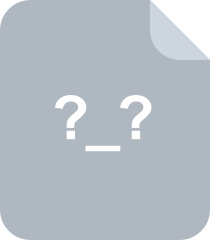



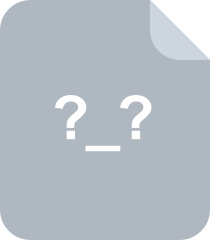

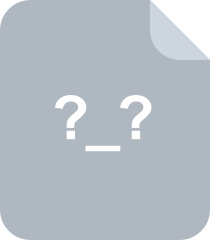
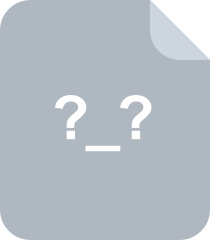
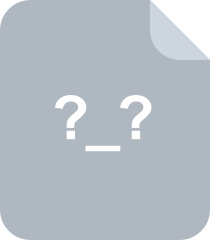
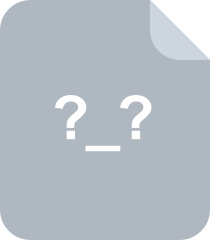
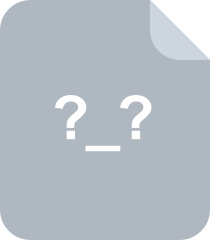
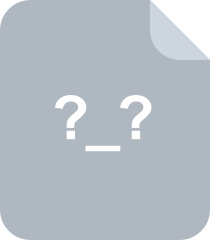




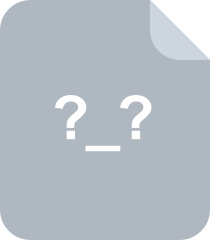

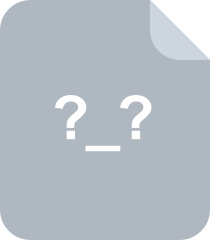
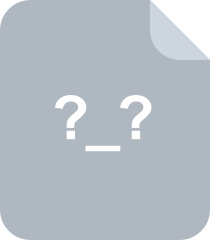

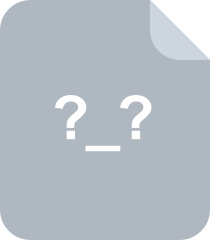

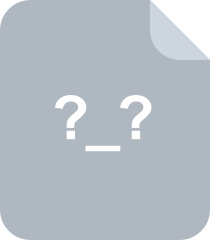

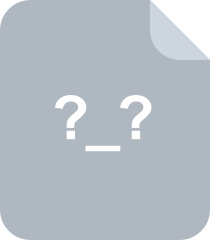


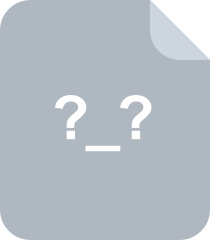
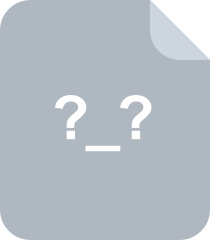

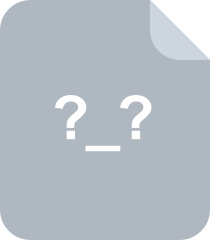
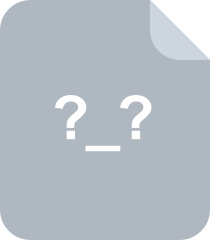
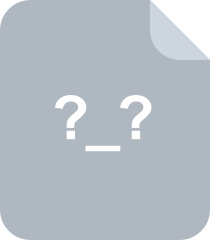
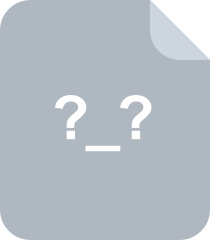
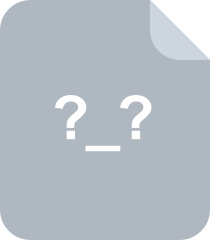
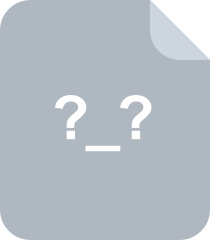

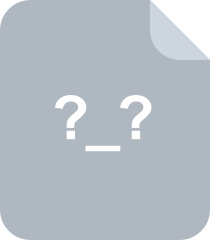


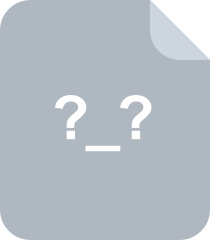
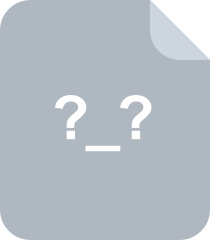
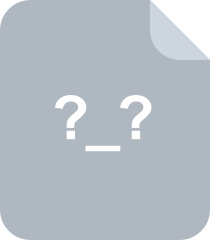
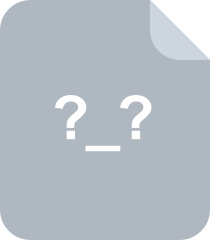
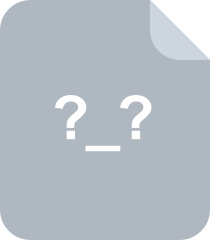
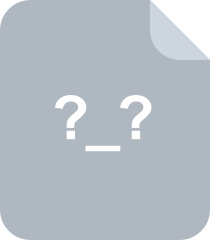
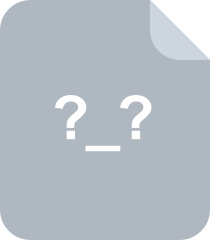
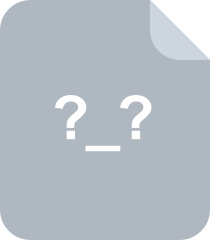

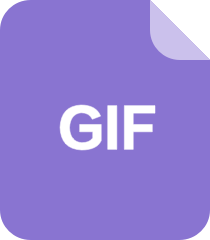
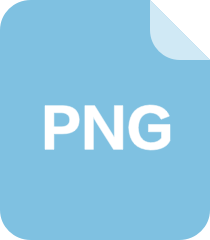

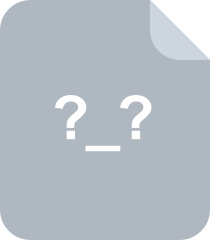
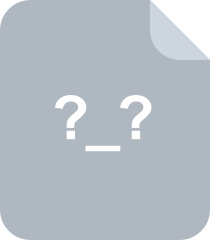
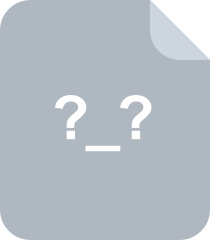
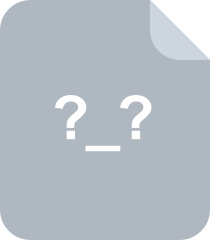
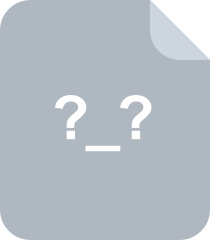
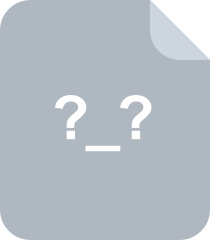
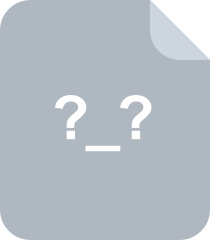
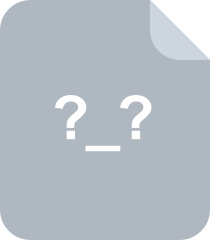
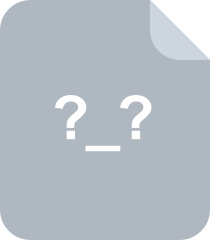
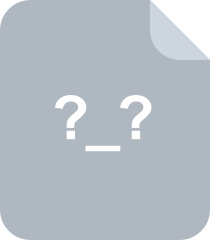

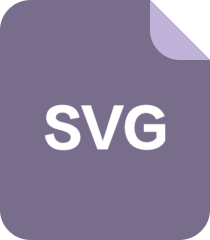
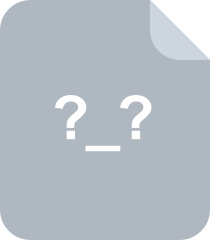
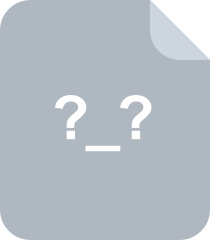
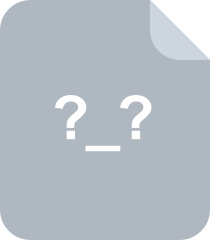
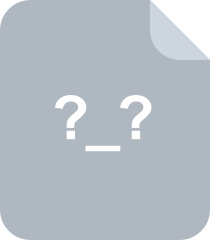

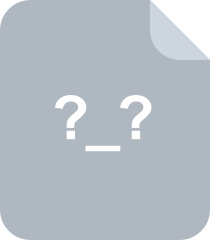
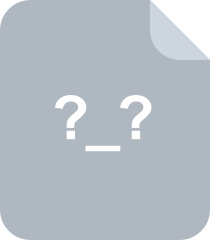
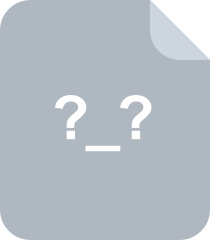
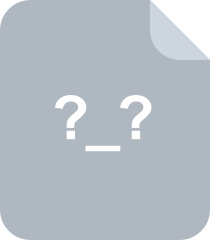
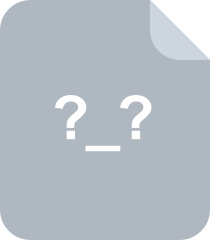
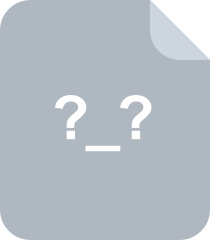
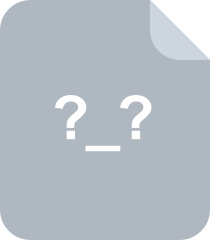
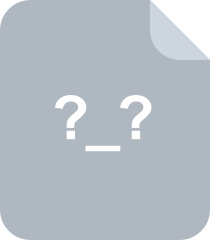
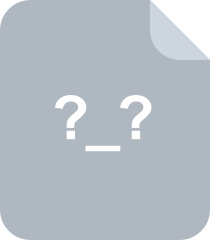
共 57 条
- 1
资源评论
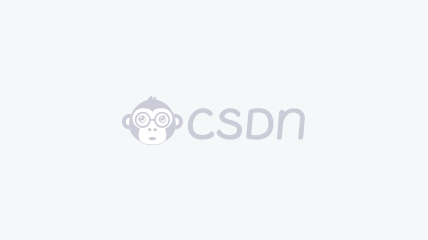

zy_zeros
- 粉丝: 952
- 资源: 320
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

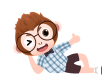
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


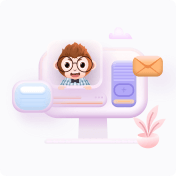
安全验证
文档复制为VIP权益,开通VIP直接复制
