package com.mask.fileserver.controller;
import java.io.IOException;
import java.security.NoSuchAlgorithmException;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.servlet.mvc.support.RedirectAttributes;
import com.mask.fileserver.domain.File;
import com.mask.fileserver.service.FileService;
import com.mask.fileserver.util.MD5Util;
@CrossOrigin(origins = "*", maxAge = 3600) // 允许所有域名访问
@Controller
public class FileController {
@Autowired
private FileService fileService;
@RequestMapping(value = "/")
public String index(Model model) {
model.addAttribute("files", fileService.listFiles());
return "index";
}
/**
* 获取文件片信息
* @param id
* @return
*/
@GetMapping("/{id}")
@ResponseBody
public ResponseEntity serveFile(@PathVariable String id) {
File file = fileService.getFileById(id);
if (file != null) {
return ResponseEntity
.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; fileName=\"" + file.getName() + "\"")
.header(HttpHeaders.CONTENT_TYPE, "application/octet-stream" )
.header(HttpHeaders.CONTENT_LENGTH, file.getSize()+"")
.header("Connection", "close")
.body( file.getContent());
} else {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body("File was not fount");
}
}
/**
* 在线显示文件
* @param id
* @return
*/
@GetMapping("/view/{id}")
@ResponseBody
public ResponseEntity serveFileOnline(@PathVariable String id) {
File file = fileService.getFileById(id);
if (file != null) {
return ResponseEntity
.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "fileName=\"" + file.getName() + "\"")
.header(HttpHeaders.CONTENT_TYPE, file.getContentType() )
.header(HttpHeaders.CONTENT_LENGTH, file.getSize()+"")
.header("Connection", "close")
.body( file.getContent());
} else {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body("File was not fount");
}
}
/**
* 上传
* @param file
* @param redirectAttributes
* @return
*/
@PostMapping("/")
public String handleFileUpload(@RequestParam("file") MultipartFile file,
RedirectAttributes redirectAttributes) {
try {
File f = new File(file.getOriginalFilename(), file.getContentType(), file.getSize(), file.getBytes());
f.setMd5( MD5Util.getMD5(file.getInputStream()) );
fileService.saveFile(f);
} catch (IOException | NoSuchAlgorithmException ex) {
ex.printStackTrace();
redirectAttributes.addFlashAttribute("message",
"Your " + file.getOriginalFilename() + " is wrong!");
return "redirect:/";
}
redirectAttributes.addFlashAttribute("message",
"You successfully uploaded " + file.getOriginalFilename() + "!");
return "redirect:/";
}
@GetMapping("/del/{id}")
public String deleteFile(@PathVariable String id, RedirectAttributes redirectAttributes) {
try {
fileService.removeFile(id);
redirectAttributes.addFlashAttribute("message",
"You successfully delete " + id + "!");
} catch (Exception e) {
redirectAttributes.addFlashAttribute("message",
"fail to delete " + id + "!");
}
return "redirect:/";
}
@PostMapping("/upload")
@ResponseBody
public ResponseEntity<String> handleFileUpload(@RequestParam("file") MultipartFile file) {
File returnFile = null;
try {
File f = new File(file.getOriginalFilename(), file.getContentType(), file.getSize(),file.getBytes());
f.setMd5( MD5Util.getMD5(file.getInputStream()) );
returnFile = fileService.saveFile(f);
returnFile.setPath("http://localhost:8081/view/"+f.getId());
returnFile.setContent(null) ;
return ResponseEntity.status(HttpStatus.OK).body("http://localhost:8081/view/"+f.getId());
} catch (IOException | NoSuchAlgorithmException ex) {
ex.printStackTrace();
return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).body(ex.getMessage());
}
}
}


白话机器学习
- 粉丝: 1w+
- 资源: 7650
最新资源
- 四通道电子负载,电池容量测试仪器,全套资料,包含,原理图pcb 和bom程序源码非常全和宝贵资料
- 有需要学习基于分布式驱动电动汽车的搭建,附着系数估计,车辆状态参数估计(包括扩展卡尔曼,无迹卡尔曼,容积卡尔曼,高阶容积卡尔曼,平方根容积卡尔曼等方法)和电机无传感器控制等方向的内容
- 蒙特卡洛模拟研究,CFA模型,SEM模型,潜变量增长模型,统计功效,样本量,模拟研究 在matlab中用蒙特卡洛算法对电动汽车充电负荷进行模拟,可自己修改电动汽车数量,lunwen复现 参考lun
- 基于分布式驱动电动汽车的车辆状态估计,采用的是容积卡尔曼(ckf)观测器,可估计包括纵向速度,质心侧偏角,横摆角速度,侧倾角四个状态 模型中第一个模块是四轮驱动电机;第二个模块是carsim输出的真
- 七自由度整车模型 分别采用魔术公式和dugoff 两种轮胎模型建立的七自由度整车模型 包含模型所有文件和魔术公式轮胎模型和说明文档以及参考资料 本模型可进行角阶跃、制动、等速圆周等工况验证 可加入相应
- MATLAB Simulink仿真平台,蓄电池控制 包括蓄电池双向DC DC控制,采用电压外环电流内环控制,使输出电压稳定,也可采用功率外环电流内环控制,使输出功率稳定
- 自动驾驶,carsim,simulink联合仿真,基于lqr算法的路径跟踪控制, carsim2019,matlab2018,以上
- 基于深度强化学习的混合动力汽车能量管理策略 1.利用DQN算法控制电池和发动机发电机组的功率分配 2.状态量为需求功率和SOC,控制量为EGS功率 3.奖励函数设置为等效油耗和SOC维持
- FMCW激光雷达 正弦波 三角波 目标检测 双模调制
- 安-川7-内部资料,包含源码与详细说明,以及运行环境软件. 电流环扰动观测器、速度补偿、摩擦扰动观测器、标幺化计算、转矩补偿、位置环、速度环、电流环 三环分析、参数计算.....
- (Matlab)基于贝叶斯(bayes)优化卷积神经网络-门控循环单元(CNN-GRU)回归预测,BO-CNN-GRU Bayes-CNN-GRU多输入单输出模型 1.优化参数为:学习率,隐含层节点
- 运动控制卡 倒R角程序 G代码 halcon联合运动控制卡联合相机 运动控制卡内容: 回原点 单轴运动 速度控制 位置控制 直线插补 圆弧插补 直线圆弧插补 G代码计算 根据输入参数生产R角参数,并且
- C#联合halcon深度学习源码 继电器识别 在halcon等图像处理算法不稳定的情况下,需要用深度学习来解决 下面这个案例非常有参考价值,是基于深度学习来识别工厂的零件 因为这个零件种类比较多
- 永磁同步电机基于SVPWM改进的直接转矩控制 针对传统直接转矩控制存在的转矩脉动大、采样率高等问题,基于SVPWM改进的DTC可以解决上述存在的问题 模型仿真效果良好,可提供和对应的参考文献,适合入
- C#联合halcon条形码识别源代码 缺陷检测 飞拿 海康相机 海康相机,传感器检测到条形码后,触发相机拿照,识别二wei码,查找二wei码缺陷,发现缺陷后,通过串口发送指令停机并且报告
- 基于 Qt5.14+OpenCV4.6.0 的通用化视觉软件,qt编译器直接运行, qt编译器直接运行 支持多相机多线程,每个工具都是单独的DLL,主程序通过 公用的接口访问以及加载各个工具 算法工
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


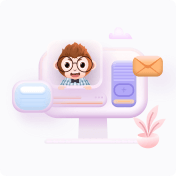