Native Abstractions for Node.js
===============================
**A header file filled with macro and utility goodness for making add-on development for Node.js easier across versions 0.8, 0.10 and 0.12 as well as io.js.**
***Current version: 2.0.9***
*(See [CHANGELOG.md](https://github.com/nodejs/nan/blob/master/CHANGELOG.md) for complete ChangeLog)*
[](https://nodei.co/npm/nan/) [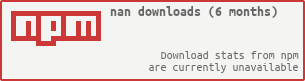](https://nodei.co/npm/nan/)
[](http://travis-ci.org/nodejs/nan)
[](https://ci.appveyor.com/project/RodVagg/nan)
Thanks to the crazy changes in V8 (and some in Node core), keeping native addons compiling happily across versions, particularly 0.10 to 0.12, is a minor nightmare. The goal of this project is to store all logic necessary to develop native Node.js addons without having to inspect `NODE_MODULE_VERSION` and get yourself into a macro-tangle.
This project also contains some helper utilities that make addon development a bit more pleasant.
* **[News & Updates](#news)**
* **[Usage](#usage)**
* **[Example](#example)**
* **[API](#api)**
* **[Tests](#tests)**
* **[Governance & Contributing](#governance)**
<a name="news"></a>
## News & Updates
<a name="usage"></a>
## Usage
Simply add **NAN** as a dependency in the *package.json* of your Node addon:
``` bash
$ npm install --save nan
```
Pull in the path to **NAN** in your *binding.gyp* so that you can use `#include <nan.h>` in your *.cpp* files:
``` python
"include_dirs" : [
"<!(node -e \"require('nan')\")"
]
```
This works like a `-I<path-to-NAN>` when compiling your addon.
<a name="example"></a>
## Example
Just getting started with Nan? Refer to a [quick-start **Nan** Boilerplate](https://github.com/fcanas/node-native-boilerplate) for a ready-to-go project that utilizes basic Nan functionality.
For a simpler example, see the **[async pi estimation example](https://github.com/nodejs/nan/tree/master/examples/async_pi_estimate)** in the examples directory for full code and an explanation of what this Monte Carlo Pi estimation example does. Below are just some parts of the full example that illustrate the use of **NAN**.
For another example, see **[nan-example-eol](https://github.com/CodeCharmLtd/nan-example-eol)**. It shows newline detection implemented as a native addon.
<a name="api"></a>
## API
Additional to the NAN documentation below, please consult:
* [The V8 Getting Started Guide](https://developers.google.com/v8/get_started)
* [The V8 Embedders Guide](https://developers.google.com/v8/embed)
* [V8 API Documentation](http://v8docs.nodesource.com/)
<!-- START API -->
### JavaScript-accessible methods
A _template_ is a blueprint for JavaScript functions and objects in a context. You can use a template to wrap C++ functions and data structures within JavaScript objects so that they can be manipulated from JavaScript. See the V8 Embedders Guide section on [Templates](https://developers.google.com/v8/embed#templates) for further information.
In order to expose functionality to JavaScript via a template, you must provide it to V8 in a form that it understands. Across the versions of V8 supported by NAN, JavaScript-accessible method signatures vary widely, NAN fully abstracts method declaration and provides you with an interface that is similar to the most recent V8 API but is backward-compatible with older versions that still use the now-deceased `v8::Argument` type.
* **Method argument types**
- <a href="doc/methods.md#api_nan_function_callback_info"><b><code>Nan::FunctionCallbackInfo</code></b></a>
- <a href="doc/methods.md#api_nan_property_callback_info"><b><code>Nan::PropertyCallbackInfo</code></b></a>
- <a href="doc/methods.md#api_nan_return_value"><b><code>Nan::ReturnValue</code></b></a>
* **Method declarations**
- <a href="doc/methods.md#api_nan_method"><b>Method declaration</b></a>
- <a href="doc/methods.md#api_nan_getter"><b>Getter declaration</b></a>
- <a href="doc/methods.md#api_nan_setter"><b>Setter declaration</b></a>
- <a href="doc/methods.md#api_nan_property_getter"><b>Property getter declaration</b></a>
- <a href="doc/methods.md#api_nan_property_setter"><b>Property setter declaration</b></a>
- <a href="doc/methods.md#api_nan_property_enumerator"><b>Property enumerator declaration</b></a>
- <a href="doc/methods.md#api_nan_property_deleter"><b>Property deleter declaration</b></a>
- <a href="doc/methods.md#api_nan_property_query"><b>Property query declaration</b></a>
- <a href="doc/methods.md#api_nan_index_getter"><b>Index getter declaration</b></a>
- <a href="doc/methods.md#api_nan_index_setter"><b>Index setter declaration</b></a>
- <a href="doc/methods.md#api_nan_index_enumerator"><b>Index enumerator declaration</b></a>
- <a href="doc/methods.md#api_nan_index_deleter"><b>Index deleter declaration</b></a>
- <a href="doc/methods.md#api_nan_index_query"><b>Index query declaration</b></a>
* Method and template helpers
- <a href="doc/methods.md#api_nan_set_method"><b><code>Nan::SetMethod()</code></b></a>
- <a href="doc/methods.md#api_nan_set_named_property_handler"><b><code>Nan::SetNamedPropertyHandler()</code></b></a>
- <a href="doc/methods.md#api_nan_set_indexed_property_handler"><b><code>Nan::SetIndexedPropertyHandler()</code></b></a>
- <a href="doc/methods.md#api_nan_set_prototype_method"><b><code>Nan::SetPrototypeMethod()</code></b></a>
- <a href="doc/methods.md#api_nan_set_template"><b><code>Nan::SetTemplate()</code></b></a>
- <a href="doc/methods.md#api_nan_set_prototype_template"><b><code>Nan::SetPrototypeTemplate()</code></b></a>
- <a href="doc/methods.md#api_nan_set_instance_template"><b><code>Nan::SetInstanceTemplate()</code></b></a>
### Scopes
A _local handle_ is a pointer to an object. All V8 objects are accessed using handles, they are necessary because of the way the V8 garbage collector works.
A handle scope can be thought of as a container for any number of handles. When you've finished with your handles, instead of deleting each one individually you can simply delete their scope.
The creation of `HandleScope` objects is different across the supported versions of V8. Therefore, NAN provides its own implementations that can be used safely across these.
- <a href="doc/scopes.md#api_nan_handle_scope"><b><code>Nan::HandleScope</code></b></a>
- <a href="doc/scopes.md#api_nan_escapable_handle_scope"><b><code>Nan::EscapableHandleScope</code></b></a>
Also see the V8 Embedders Guide section on [Handles and Garbage Collection](https://developers.google.com/v8/embed#handles).
### Persistent references
An object reference that is independent of any `HandleScope` is a _persistent_ reference. Where a `Local` handle only lives as long as the `HandleScope` in which it was allocated, a `Persistent` handle remains valid until it is explicitly disposed.
Due to the evolution of the V8 API, it is necessary for NAN to provide a wrapper implementation of the `Persistent` classes to supply compatibility across the V8 versions supported.
- <a href="doc/persistent.md#api_nan_persistent_base"><b><code>Nan::PersistentBase & v8::PersistentBase</code></b></a>
- <a href="doc/persistent.md#api_nan_non_copyable_persistent_traits"><b><code>Nan::NonCopyablePersistentTraits & v8::NonCopyablePersistentTraits</code></b></a>
- <a href="doc/persistent.md#api_nan_copyable_persistent_traits"><b><code>Nan::CopyablePersistentTraits & v8::CopyablePersistentTraits</code></b></a>
- <a href="doc/persistent.md#api_nan_persistent"><b><code>Nan::Persistent</code></b></a>
- <a href="doc/persistent.md#api_nan_global"><b><code>Nan::Global</code></b></a>
- <a href="doc/persistent.md#api_nan_weak_callback_info"><b><code>Nan::WeakCallbackInfo</code></b></a>
- <a href="doc/persistent.md#api_nan_weak_callbac
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于node js、vue、mongodb等技术构建的web系统,界面美观,功能齐全,适合用作毕业设计、课程设计作业等,项目均经过测试,可快速部署运行! 基于node js、vue、mongodb等技术构建的web系统,界面美观,功能齐全,适合用作毕业设计、课程设计作业等,项目均经过测试,可快速部署运行! 基于node js、vue、mongodb等技术构建的web系统,界面美观,功能齐全,适合用作毕业设计、课程设计作业等,项目均经过测试,可快速部署运行!
资源推荐
资源详情
资源评论
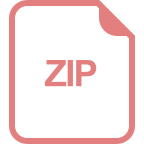
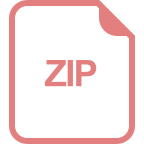
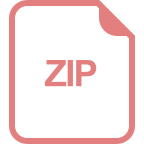
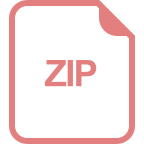
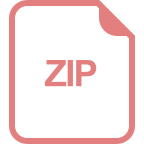
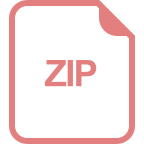
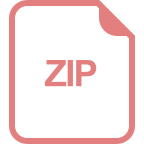
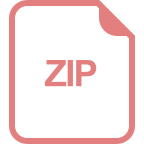
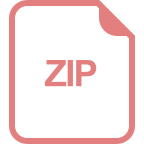
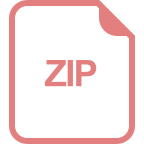
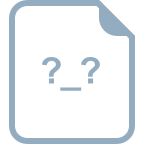
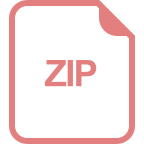
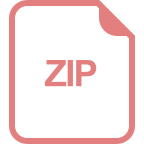
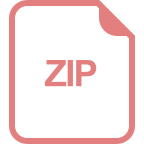
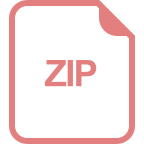
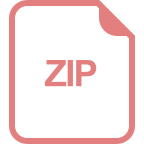
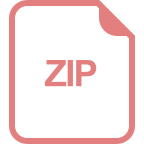
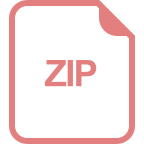
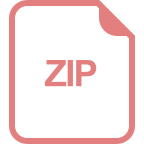
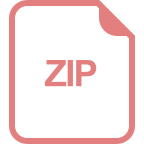
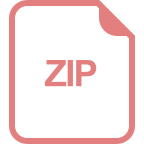
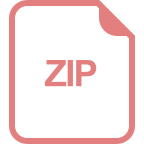
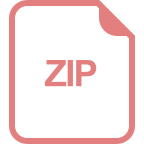
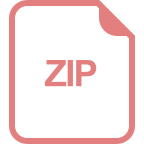
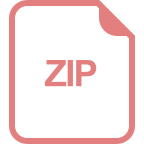
收起资源包目录

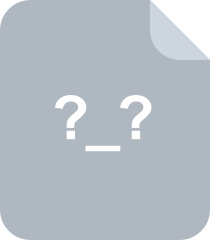
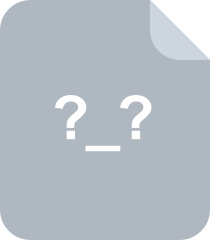
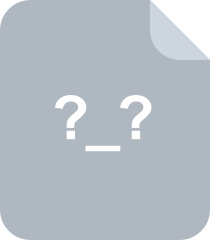
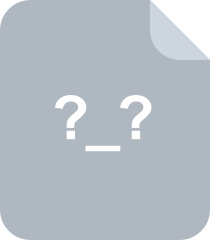
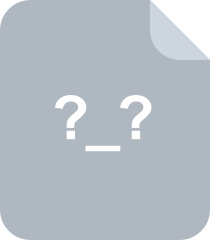
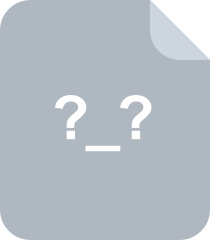
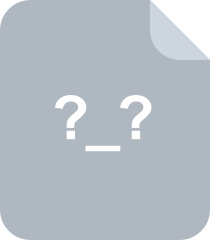
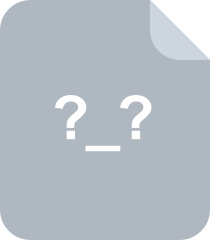
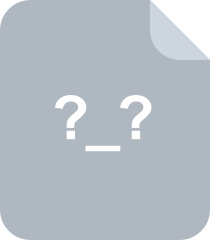
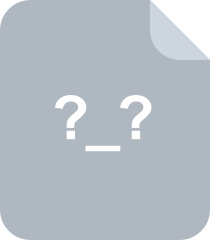
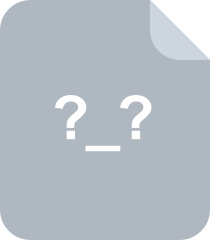
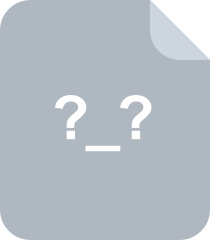
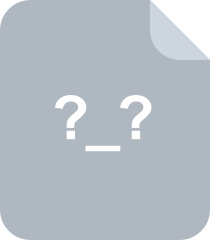
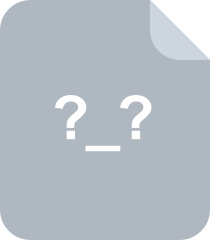
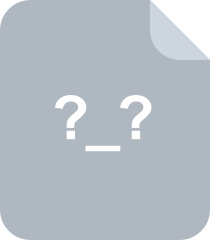
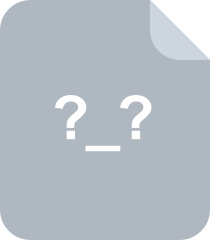
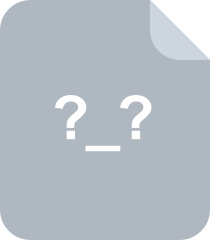
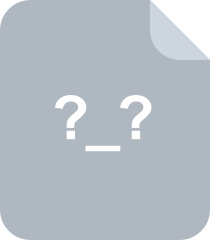
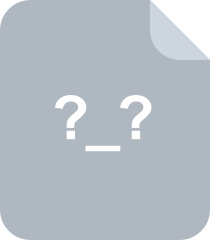
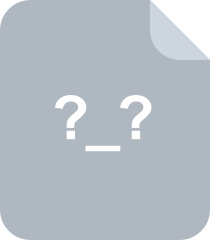
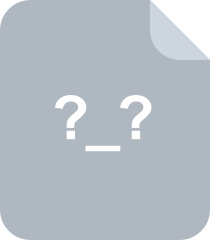
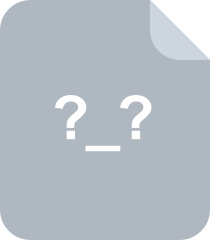
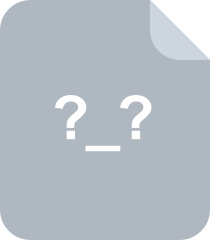
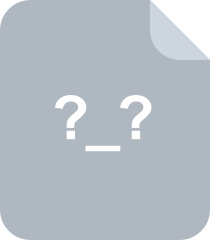
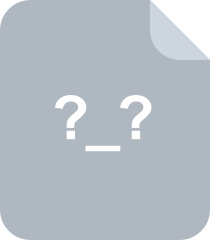
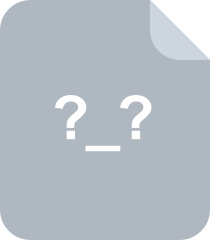
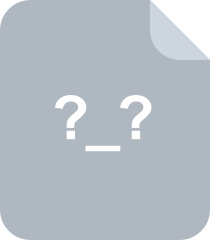
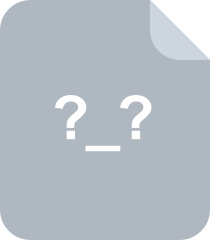
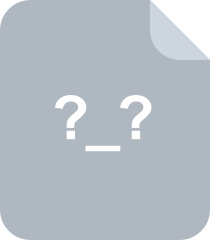
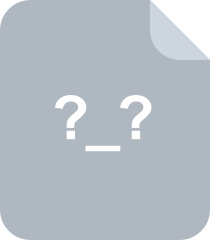
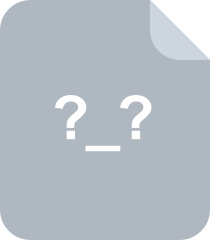
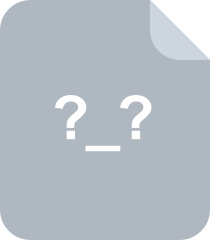
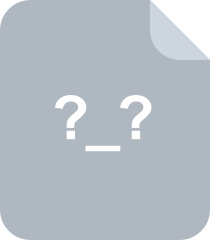
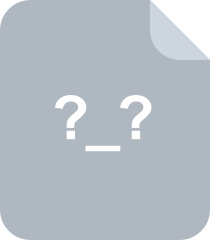
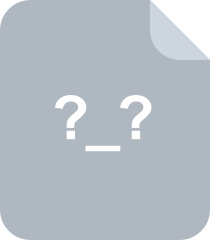
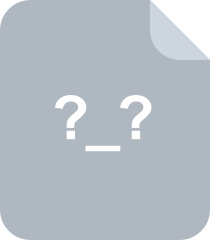
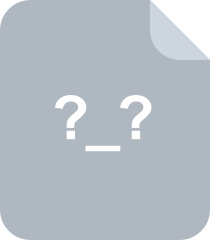
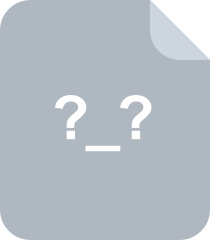
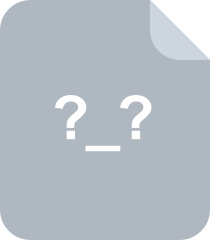
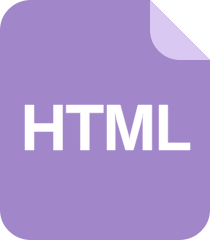
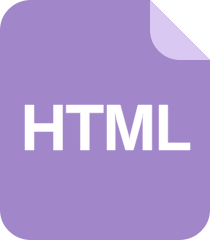
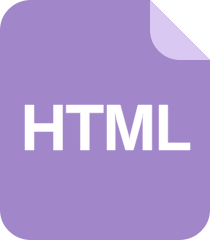
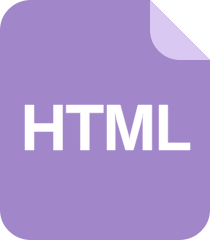
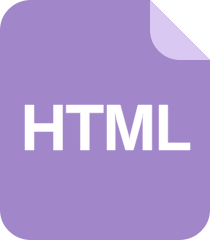
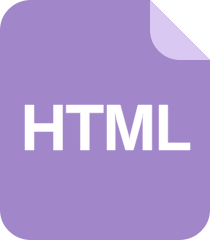
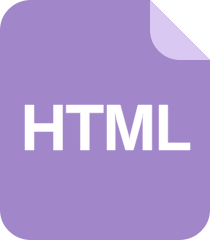
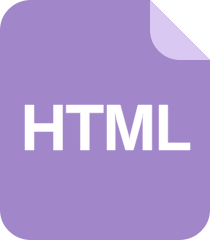
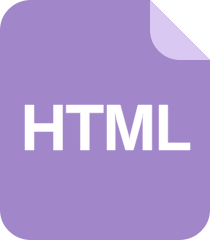
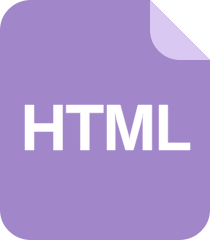
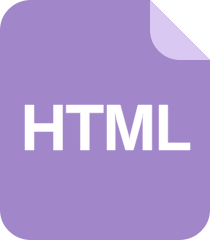
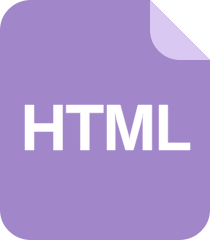
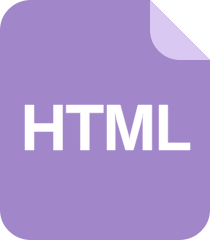
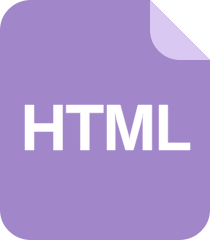
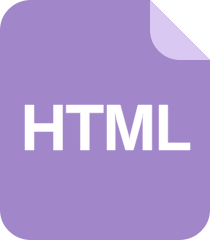
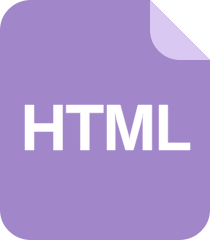
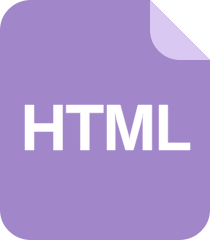
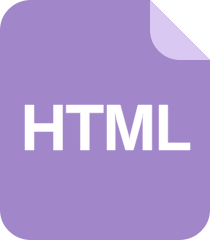
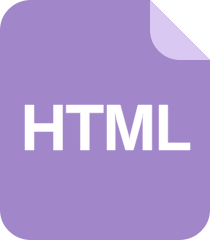
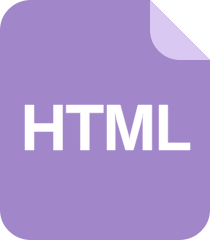
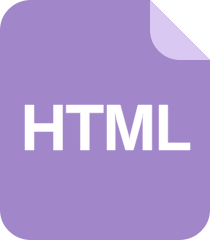
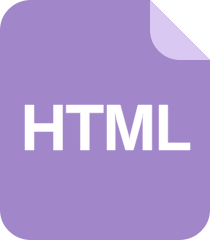
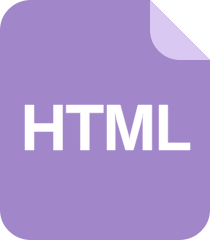
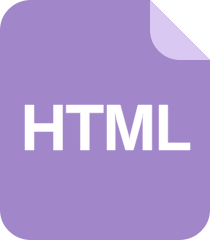
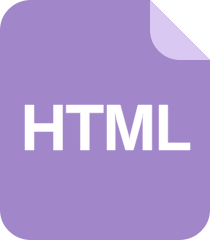
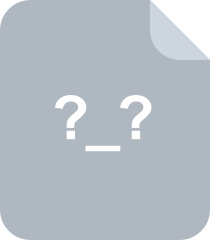
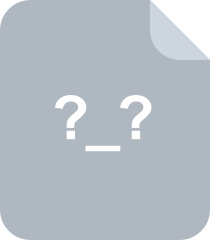
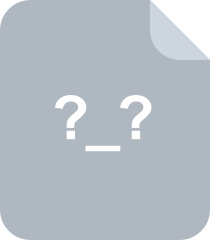
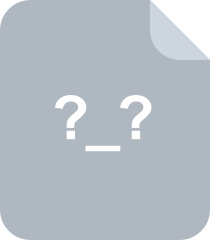
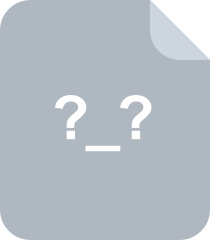
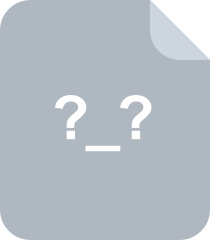
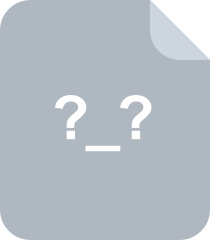
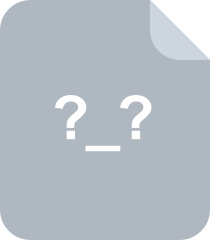
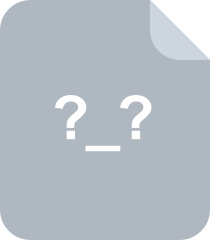
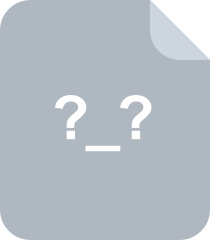
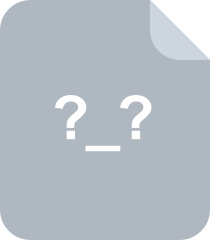
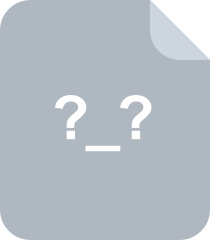
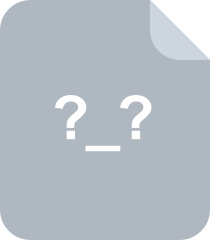
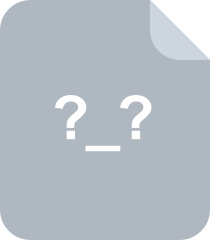
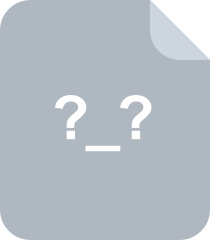
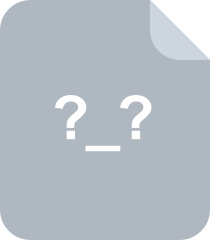
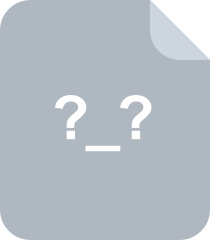
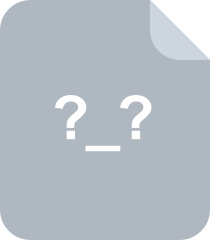
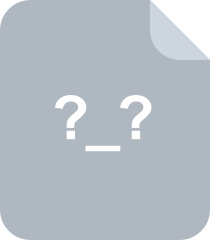
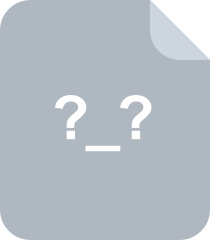
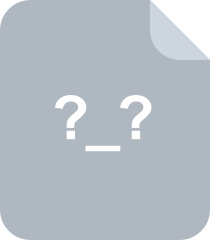
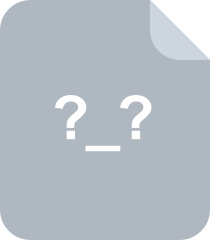
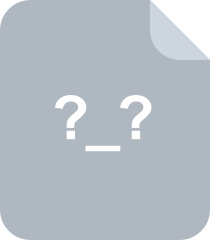
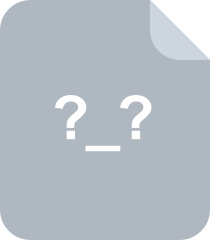
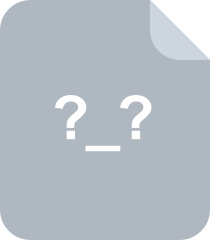
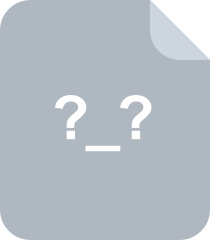
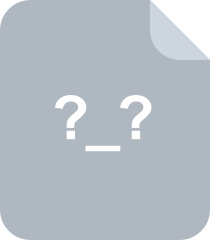
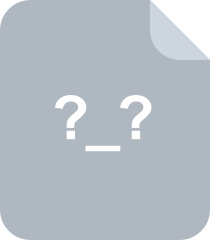
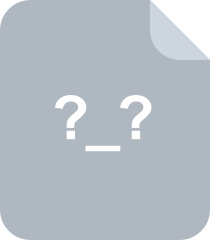
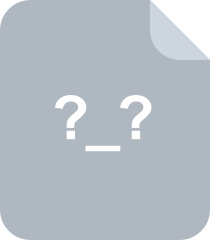
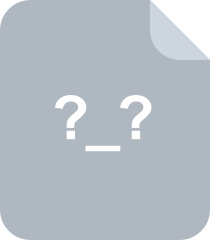
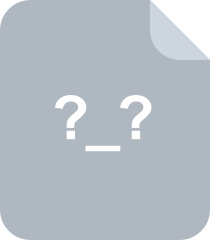
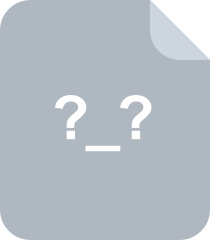
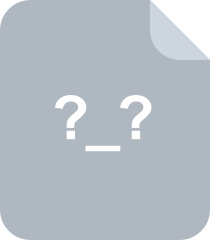
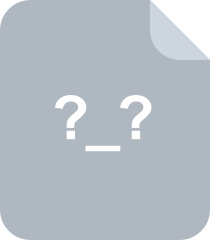
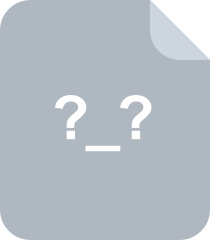
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
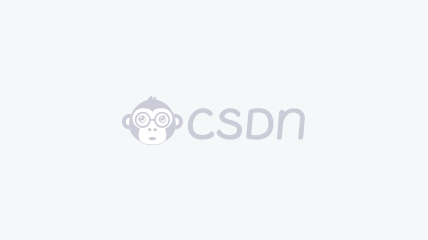


白话机器学习
- 粉丝: 1w+
- 资源: 7672
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

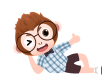
安全验证
文档复制为VIP权益,开通VIP直接复制
