<!doctype html>
<title>CodeMirror: Scala mode</title>
<meta charset="utf-8"/>
<link rel=stylesheet href="../../doc/docs.css">
<link rel="stylesheet" href="../../lib/codemirror.css">
<link rel="stylesheet" href="../../theme/ambiance.css">
<script src="../../lib/codemirror.js"></script>
<script src="../../addon/edit/matchbrackets.js"></script>
<script src="clike.js"></script>
<div id=nav>
<a href="http://codemirror.net"><h1>CodeMirror</h1><img id=logo src="../../doc/logo.png"></a>
<ul>
<li><a href="../../index.html">Home</a>
<li><a href="../../doc/manual.html">Manual</a>
<li><a href="https://github.com/codemirror/codemirror">Code</a>
</ul>
<ul>
<li><a href="../index.html">Language modes</a>
<li><a class=active href="#">Scala</a>
</ul>
</div>
<article>
<h2>Scala mode</h2>
<form>
<textarea id="code" name="code">
/* __ *\
** ________ ___ / / ___ Scala API **
** / __/ __// _ | / / / _ | (c) 2003-2011, LAMP/EPFL **
** __\ \/ /__/ __ |/ /__/ __ | http://scala-lang.org/ **
** /____/\___/_/ |_/____/_/ | | **
** |/ **
\* */
package scala.collection
import generic._
import mutable.{ Builder, ListBuffer }
import annotation.{tailrec, migration, bridge}
import annotation.unchecked.{ uncheckedVariance => uV }
import parallel.ParIterable
/** A template trait for traversable collections of type `Traversable[A]`.
*
* $traversableInfo
* @define mutability
* @define traversableInfo
* This is a base trait of all kinds of $mutability Scala collections. It
* implements the behavior common to all collections, in terms of a method
* `foreach` with signature:
* {{{
* def foreach[U](f: Elem => U): Unit
* }}}
* Collection classes mixing in this trait provide a concrete
* `foreach` method which traverses all the
* elements contained in the collection, applying a given function to each.
* They also need to provide a method `newBuilder`
* which creates a builder for collections of the same kind.
*
* A traversable class might or might not have two properties: strictness
* and orderedness. Neither is represented as a type.
*
* The instances of a strict collection class have all their elements
* computed before they can be used as values. By contrast, instances of
* a non-strict collection class may defer computation of some of their
* elements until after the instance is available as a value.
* A typical example of a non-strict collection class is a
* <a href="../immutable/Stream.html" target="ContentFrame">
* `scala.collection.immutable.Stream`</a>.
* A more general class of examples are `TraversableViews`.
*
* If a collection is an instance of an ordered collection class, traversing
* its elements with `foreach` will always visit elements in the
* same order, even for different runs of the program. If the class is not
* ordered, `foreach` can visit elements in different orders for
* different runs (but it will keep the same order in the same run).'
*
* A typical example of a collection class which is not ordered is a
* `HashMap` of objects. The traversal order for hash maps will
* depend on the hash codes of its elements, and these hash codes might
* differ from one run to the next. By contrast, a `LinkedHashMap`
* is ordered because it's `foreach` method visits elements in the
* order they were inserted into the `HashMap`.
*
* @author Martin Odersky
* @version 2.8
* @since 2.8
* @tparam A the element type of the collection
* @tparam Repr the type of the actual collection containing the elements.
*
* @define Coll Traversable
* @define coll traversable collection
*/
trait TraversableLike[+A, +Repr] extends HasNewBuilder[A, Repr]
with FilterMonadic[A, Repr]
with TraversableOnce[A]
with GenTraversableLike[A, Repr]
with Parallelizable[A, ParIterable[A]]
{
self =>
import Traversable.breaks._
/** The type implementing this traversable */
protected type Self = Repr
/** The collection of type $coll underlying this `TraversableLike` object.
* By default this is implemented as the `TraversableLike` object itself,
* but this can be overridden.
*/
def repr: Repr = this.asInstanceOf[Repr]
/** The underlying collection seen as an instance of `$Coll`.
* By default this is implemented as the current collection object itself,
* but this can be overridden.
*/
protected[this] def thisCollection: Traversable[A] = this.asInstanceOf[Traversable[A]]
/** A conversion from collections of type `Repr` to `$Coll` objects.
* By default this is implemented as just a cast, but this can be overridden.
*/
protected[this] def toCollection(repr: Repr): Traversable[A] = repr.asInstanceOf[Traversable[A]]
/** Creates a new builder for this collection type.
*/
protected[this] def newBuilder: Builder[A, Repr]
protected[this] def parCombiner = ParIterable.newCombiner[A]
/** Applies a function `f` to all elements of this $coll.
*
* Note: this method underlies the implementation of most other bulk operations.
* It's important to implement this method in an efficient way.
*
*
* @param f the function that is applied for its side-effect to every element.
* The result of function `f` is discarded.
*
* @tparam U the type parameter describing the result of function `f`.
* This result will always be ignored. Typically `U` is `Unit`,
* but this is not necessary.
*
* @usecase def foreach(f: A => Unit): Unit
*/
def foreach[U](f: A => U): Unit
/** Tests whether this $coll is empty.
*
* @return `true` if the $coll contain no elements, `false` otherwise.
*/
def isEmpty: Boolean = {
var result = true
breakable {
for (x <- this) {
result = false
break
}
}
result
}
/** Tests whether this $coll is known to have a finite size.
* All strict collections are known to have finite size. For a non-strict collection
* such as `Stream`, the predicate returns `true` if all elements have been computed.
* It returns `false` if the stream is not yet evaluated to the end.
*
* Note: many collection methods will not work on collections of infinite sizes.
*
* @return `true` if this collection is known to have finite size, `false` otherwise.
*/
def hasDefiniteSize = true
def ++[B >: A, That](that: GenTraversableOnce[B])(implicit bf: CanBuildFrom[Repr, B, That]): That = {
val b = bf(repr)
if (that.isInstanceOf[IndexedSeqLike[_, _]]) b.sizeHint(this, that.seq.size)
b ++= thisCollection
b ++= that.seq
b.result
}
@bridge
def ++[B >: A, That](that: TraversableOnce[B])(implicit bf: CanBuildFrom[Repr, B, That]): That =
++(that: GenTraversableOnce[B])(bf)
/** Concatenates this $coll with the elements of a traversable collection.
* It differs from ++ in that the right operand determines the type of the
* resulting collection rather than the left one.
*
* @param that the traversable to append.
* @tparam B the element type of the returned collection.
* @tparam That $thatinfo
* @param bf $bfinfo
* @return a new co
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于node js、vue、mongodb等技术构建的web系统,界面美观,功能齐全,适合用作毕业设计、课程设计作业等,项目均经过测试,可快速部署运行! 基于node js、vue、mongodb等技术构建的web系统,界面美观,功能齐全,适合用作毕业设计、课程设计作业等,项目均经过测试,可快速部署运行! 基于node js、vue、mongodb等技术构建的web系统,界面美观,功能齐全,适合用作毕业设计、课程设计作业等,项目均经过测试,可快速部署运行!
资源推荐
资源详情
资源评论
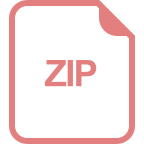
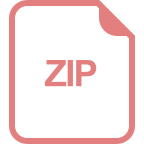
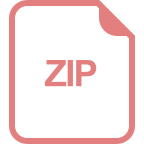
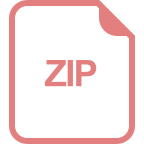
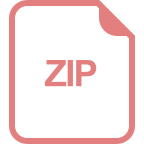
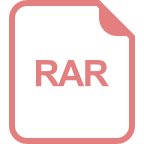
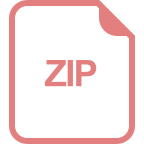
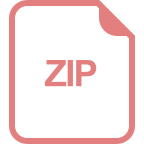
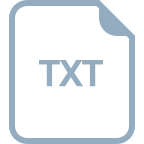
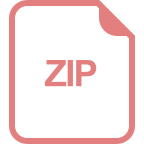
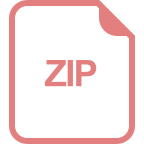
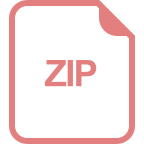
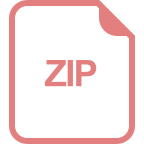
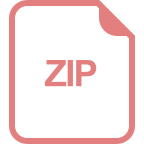
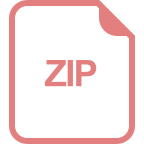
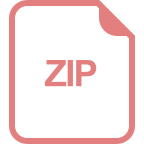
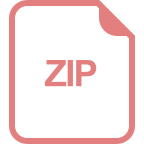
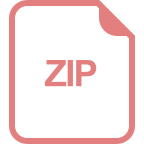
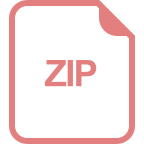
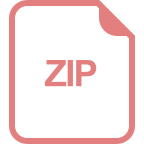
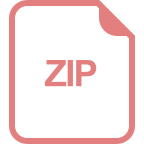
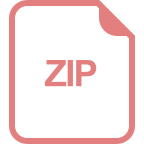
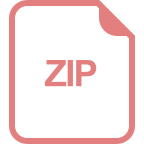
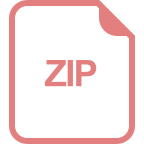
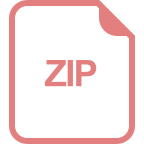
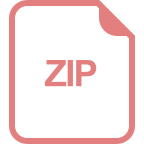
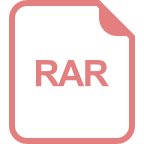
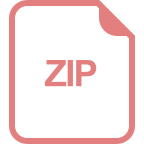
收起资源包目录

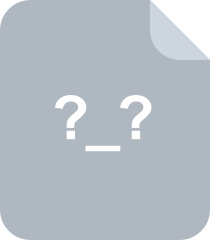
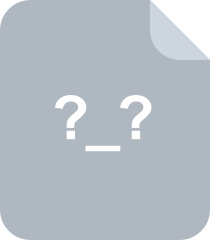
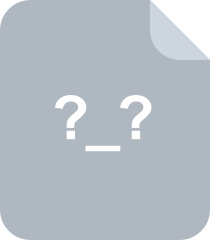
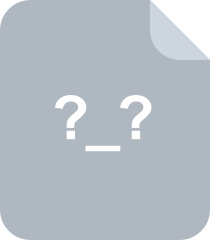
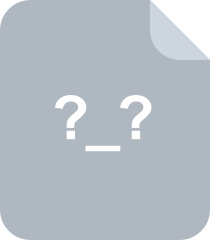
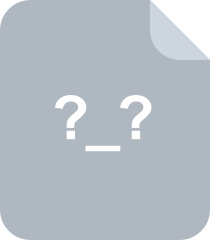
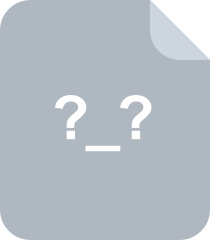
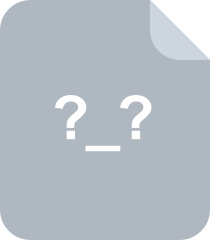
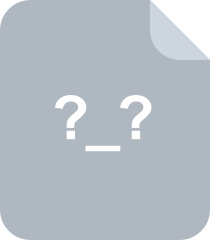
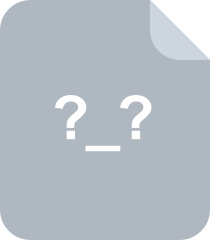
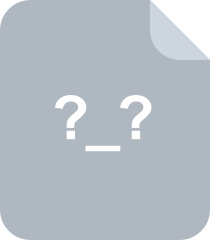
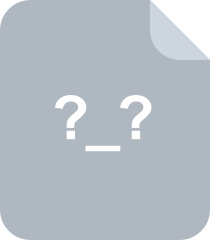
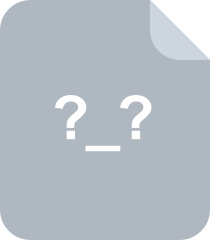
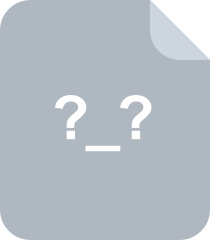
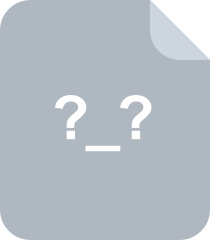
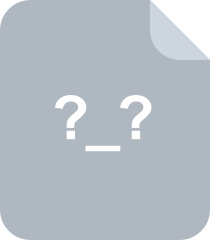
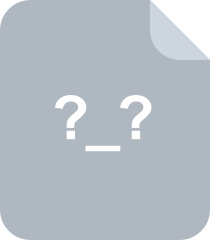
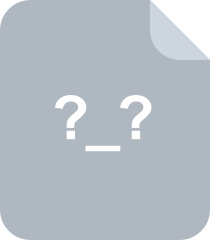
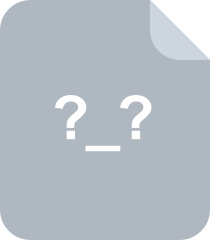
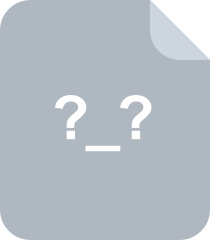
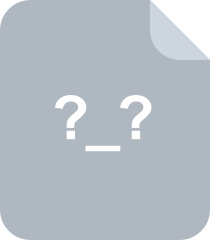
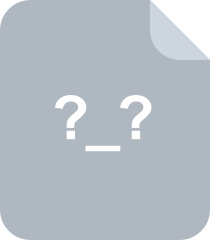
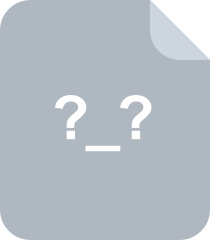
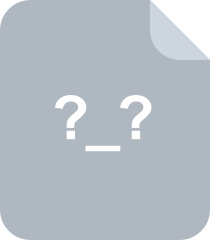
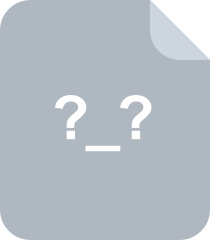
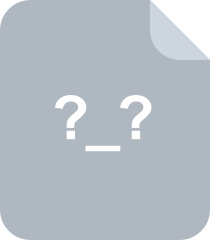
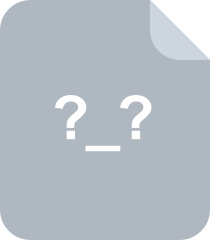
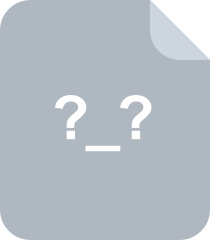
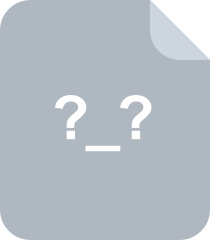
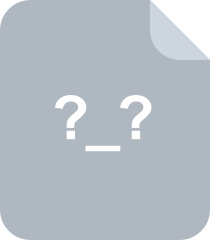
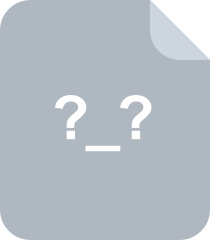
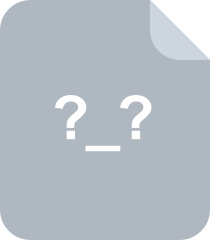
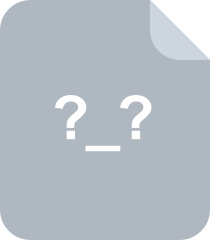
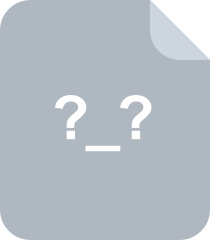
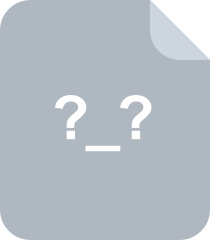
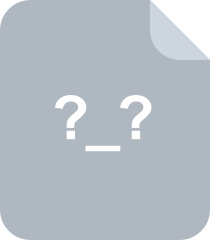
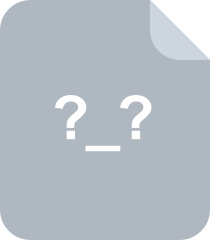
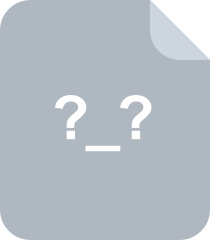
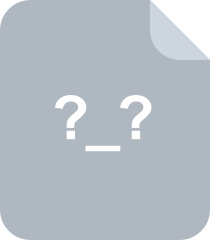
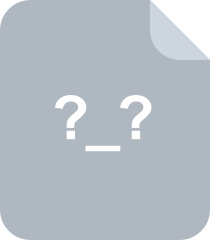
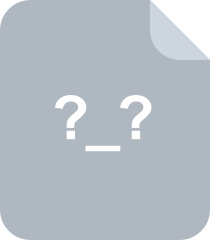
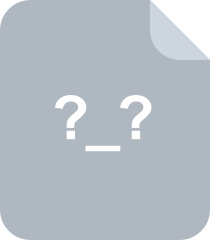
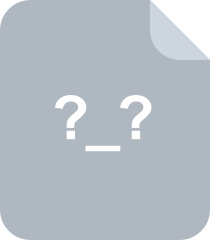
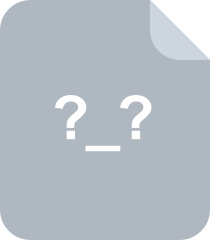
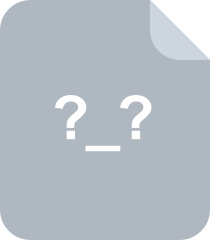
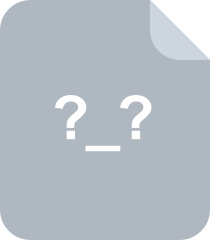
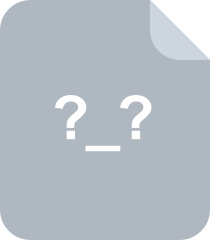
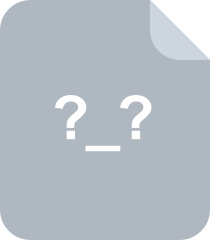
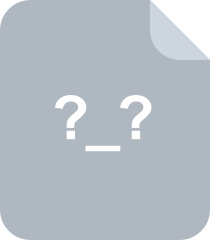
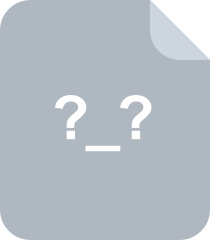
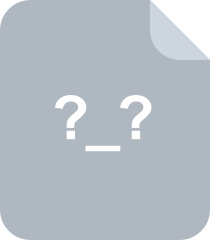
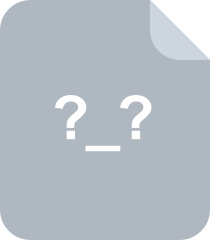
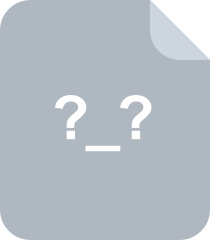
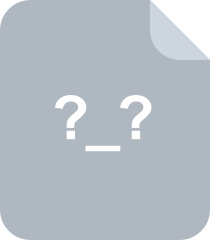
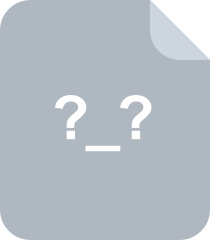
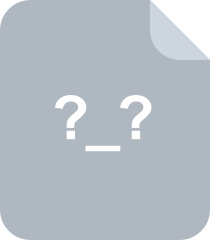
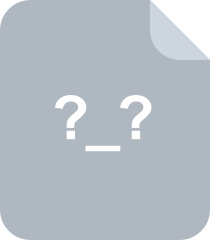
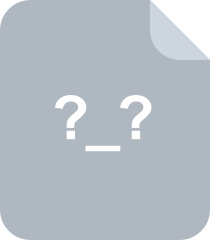
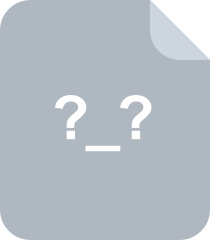
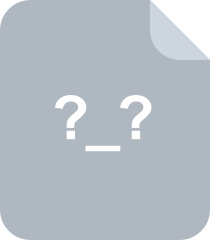
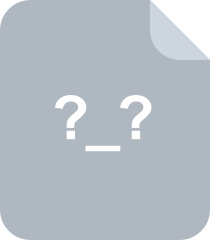
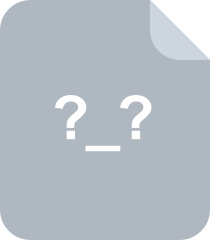
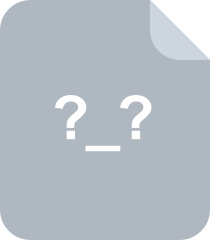
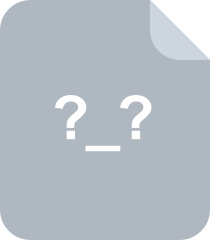
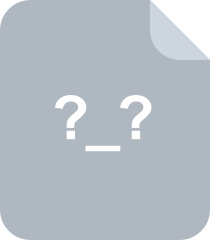
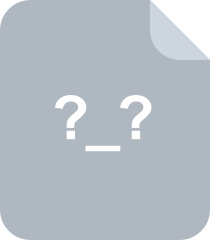
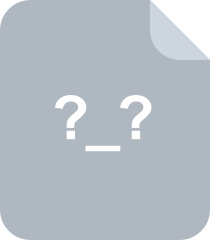
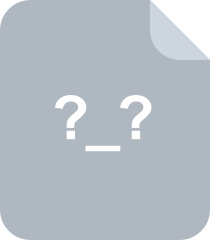
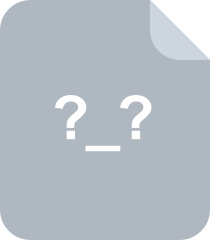
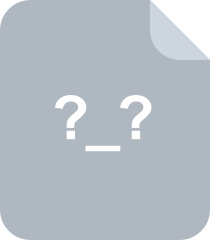
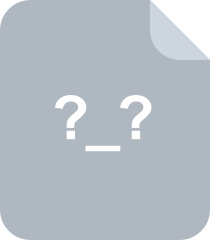
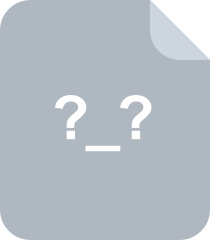
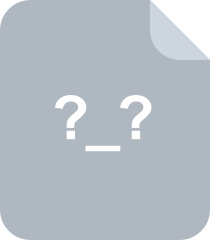
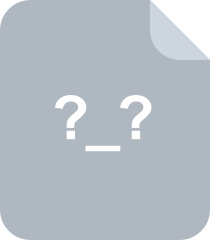
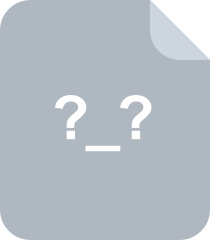
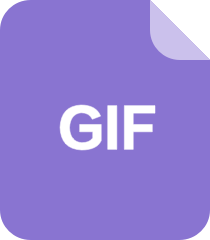
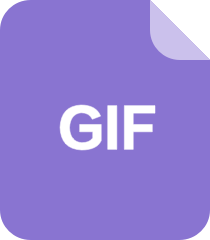
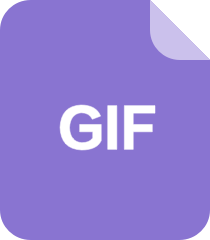
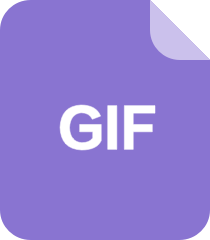
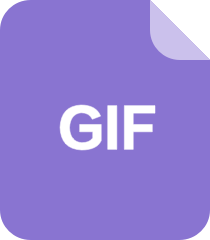
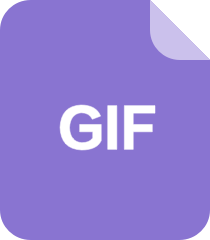
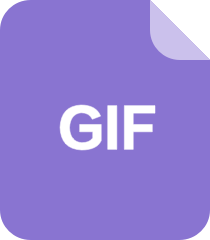
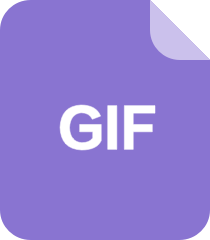
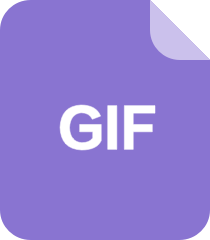
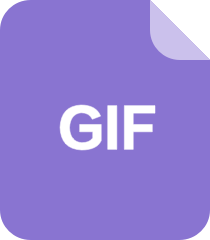
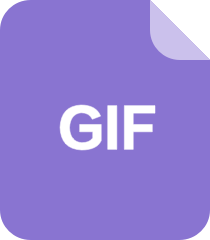
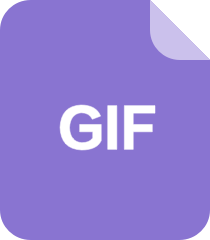
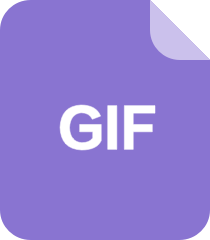
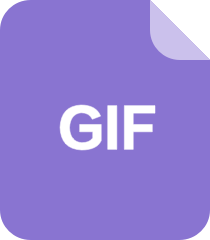
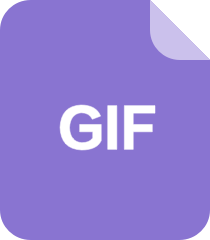
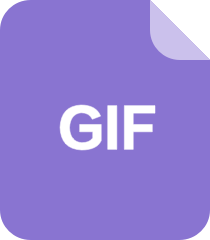
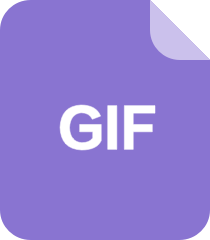
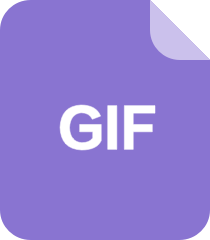
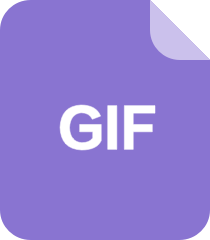
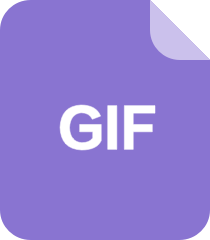
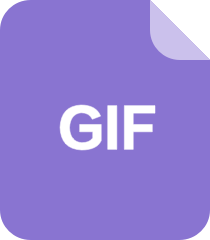
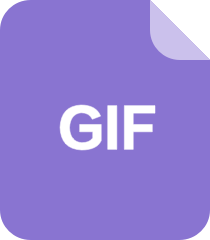
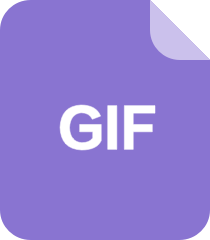
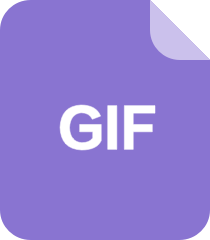
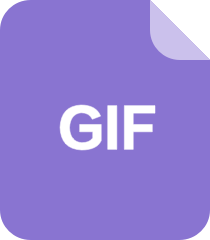
共 563 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
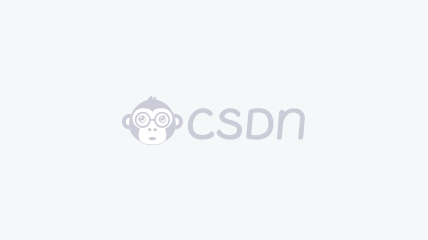


白话机器学习
- 粉丝: 1w+
- 资源: 7671
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

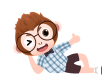
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


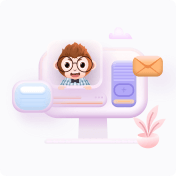
安全验证
文档复制为VIP权益,开通VIP直接复制
