
A simple, intuitive audio framework for iOS and OSX.
## Welcome to 1.0.0!
Thank you guys for being so patient over the last year - I've been working like crazy the last few weeks rewriting and extending the EZAudio core and interface components and squashing bugs. Finally, EZAudio is now at its 1.0.0 release with all new updated components, examples, and documentation. Happy coding!
## Apps Using EZAudio
I'd really like to start creating a list of projects made using EZAudio. If you've used EZAudio to make something cool, whether it's an app or open source visualization or whatever, please email me at syedhali07[at]gmail.com and I'll add it to our wall of fame!
To start it off:
- [Detour](https://www.detour.com/) - Gorgeous location-aware audio walks
- [Jumpshare](https://jumpshare.com/) - Incredibly fast, real-time file sharing
##Features
**Awesome Components**
I've designed six audio components and two interface components to allow you to immediately get your hands dirty recording, playing, and visualizing audio data. These components simply plug into each other and build on top of the high-performance, low-latency AudioUnits API and give you an easy to use API written in Objective-C instead of pure C.
[EZAudioDevice](#EZAudioDevice)
A useful class for getting all the current and available inputs/output on any Apple device. The `EZMicrophone` and `EZOutput` use this to direct sound in/out from different hardware components.
[EZMicrophone](#EZMicrophone)
A microphone class that provides its delegate audio data from the default device microphone with one line of code.
[EZOutput](#EZOutput)
An output class that will playback any audio it is provided by its datasource.
[EZAudioFile](#EZAudioFile)
An audio file class that reads/seeks through audio files and provides useful delegate callbacks.
[EZAudioPlayer](#EZAudioPlayer)
A replacement for `AVAudioPlayer` that combines an `EZAudioFile` and a `EZOutput` to perform robust playback of any file on any piece of hardware.
[EZRecorder](#EZRecorder)
A recorder class that provides a quick and easy way to write audio files from any datasource.
[EZAudioPlot](#EZAudioPlot)
A Core Graphics-based audio waveform plot capable of visualizing any float array as a buffer or rolling plot.
[EZAudioPlotGL](#EZAudioPlotGL)
An OpenGL-based, GPU-accelerated audio waveform plot capable of visualizing any float array as a buffer or rolling plot.
**Cross Platform**
`EZAudio` was designed to work transparently across all iOS and OSX devices. This means one universal API whether you're building for Mac or iOS. For instance, under the hood an `EZAudioPlot` knows that it will subclass a UIView for iOS or an NSView for OSX and the `EZMicrophone` knows to build on top of the RemoteIO AudioUnit for iOS, but defaults to the system defaults for input and output for OSX.
##<a name="Examples">Examples & Docs
Within this repo you'll find the examples for iOS and OSX to get you up to speed using each component and plugging them into each other. With just a few lines of code you'll be recording from the microphone, generating audio waveforms, and playing audio files like a boss. See the full Getting Started guide for an interactive look into each of components.
### Example Projects
**_EZAudioCoreGraphicsWaveformExample_**

Shows how to use the `EZMicrophone` and `EZAudioPlot` to visualize the audio data from the microphone in real-time. The waveform can be displayed as a buffer or a rolling waveform plot (traditional waveform look).
**_EZAudioOpenGLWaveformExample_**

Shows how to use the `EZMicrophone` and `EZAudioPlotGL` to visualize the audio data from the microphone in real-time. The drawing is using OpenGL so the performance much better for plots needing a lot of points.
**_EZAudioPlayFileExample_**

Shows how to use the `EZAudioPlayer` and `EZAudioPlotGL` to playback, pause, and seek through an audio file while displaying its waveform as a buffer or a rolling waveform plot.
**_EZAudioRecordWaveformExample_**

Shows how to use the `EZMicrophone`, `EZRecorder`, and `EZAudioPlotGL` to record the audio from the microphone input to a file while displaying the audio waveform of the incoming data. You can then playback the newly recorded audio file using AVFoundation and keep adding more audio data to the tail of the file.
**_EZAudioWaveformFromFileExample_**

Shows how to use the `EZAudioFile` and `EZAudioPlot` to animate in an audio waveform for an entire audio file.
**_EZAudioPassThroughExample_**

Shows how to use the `EZMicrophone`, `EZOutput`, and the `EZAudioPlotGL` to pass the microphone input to the output for playback while displaying the audio waveform (as a buffer or rolling plot) in real-time.
**_EZAudioFFTExample_**

Shows how to calculate the real-time FFT of the audio data coming from the `EZMicrophone` and the Accelerate framework. The audio data is plotted using two `EZAudioPlots` for the time and frequency displays.
### Documentation
The official documentation for EZAudio can be found here: http://cocoadocs.org/docsets/EZAudio/1.1.4/
<br>You can also generate the docset yourself using appledocs by running the appledocs on the EZAudio source folder.
##<a name="GettingStarted">Getting Started
To begin using `EZAudio` you must first make sure you have the proper build requirements and frameworks. Below you'll find explanations of each component and code snippets to show how to use each to perform common tasks like getting microphone data, updating audio waveform plots, reading/seeking through audio files, and performing playback.
###Build Requirements
**iOS**
- 6.0+
**OSX**
- 10.8+
###Frameworks
**iOS**
- Accelerate
- AudioToolbox
- AVFoundation
- GLKit
**OSX**
- Accelerate
- AudioToolbox
- AudioUnit
- CoreAudio
- QuartzCore
- OpenGL
- GLKit
###<a name="AddingToProject">Adding To Project
You can add EZAudio to your project in a few ways: <br><br>1.) The easiest way to use EZAudio is via <a href="http://cocoapods.org/", target="_blank">Cocoapods</a>. Simply add EZAudio to your <a href="http://guides.cocoapods.org/using/the-podfile.html", target="_blank">Podfile</a> like so:
`
pod 'EZAudio', '~> 1.1.4'
`
####<a name="AmazingAudioEngineCocoapod">Using EZAudio & The Amazing Audio Engine
If you're also using the Amazing Audio Engine then use the `EZAudio/Core` subspec like so:
`
pod 'EZAudio/Core', '~> 1.1.4'
`
2.) EZAudio now supports Carthage (thanks Andrew and Tommaso!). You can refer to Carthage's installation for a how-to guide:
https://github.com/Carthage/Carthage
3.) Alternatively, you can check out the iOS/Mac examples for how to setup a project using the EZAudio project as an embedded project and utilizing the frameworks. Be sure to set your header search path to the folder containing the EZAudio source.
##<a name="CoreComponents"></a>Core Components
`EZAudio` currently offers six audio components that encompass a wide range of functionality. In addition to the functional aspects of these components such as pulling audio data, reading/writing from files, and p
没有合适的资源?快使用搜索试试~ 我知道了~
毕设:基于stm32+树莓派+springboot的小程序智能家居控制系统设计与实现.zip
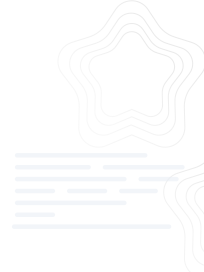
共1472个文件
jpg:211个
h:190个
d:156个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 138 浏览量
2023-06-27
09:40:47
上传
评论
收藏 62.71MB ZIP 举报
温馨提示
本资源中的源码都是经过本地编译过可运行的,下载后按照文档配置好环境就可以运行。资源项目的难度比较适中,内容都是经过助教老师审定过的,应该能够满足学习、使用需求,如果有需要的话可以放心下载使用。有任何问题也可以随时私信博主,博主会第一时间给您解答!!! 本资源中的源码都是经过本地编译过可运行的,下载后按照文档配置好环境就可以运行。资源项目的难度比较适中,内容都是经过助教老师审定过的,应该能够满足学习、使用需求,如果有需要的话可以放心下载使用。有任何问题也可以随时私信博主,博主会第一时间给您解答!!! 本资源中的源码都是经过本地编译过可运行的,下载后按照文档配置好环境就可以运行。资源项目的难度比较适中,内容都是经过助教老师审定过的,应该能够满足学习、使用需求,如果有需要的话可以放心下载使用。有任何问题也可以随时私信博主,博主会第一时间给您解答!!!
资源推荐
资源详情
资源评论
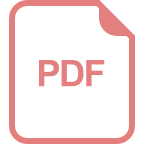
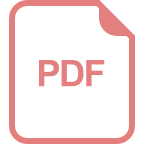
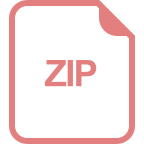
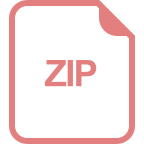
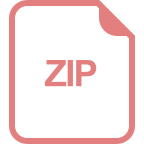
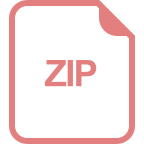
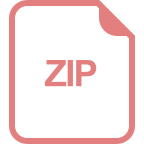
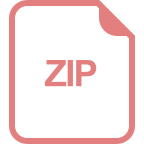
收起资源包目录

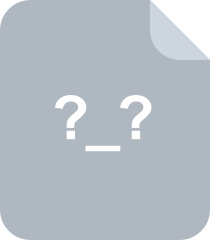
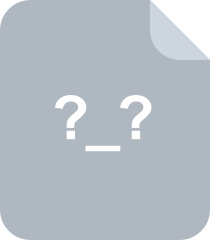
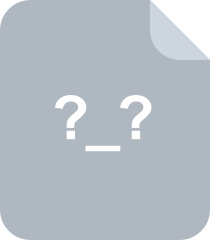
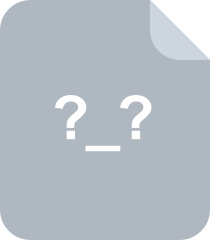
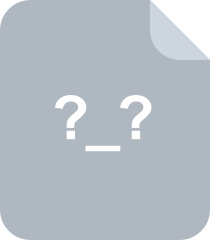
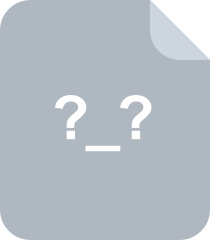
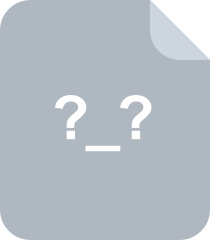
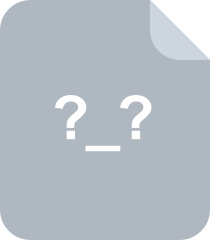
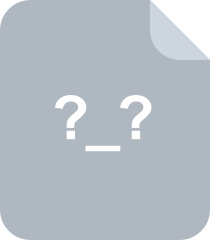
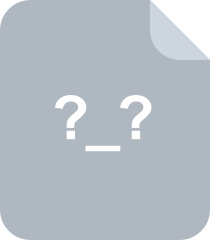
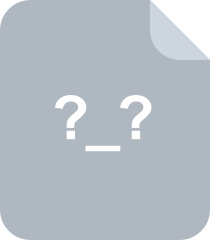
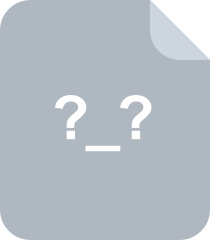
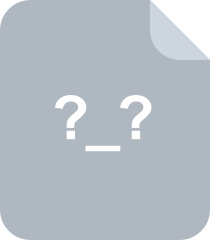
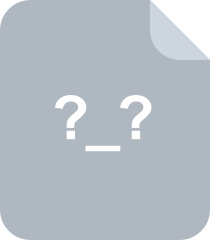
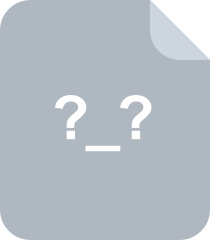
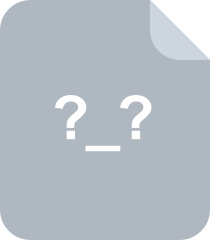
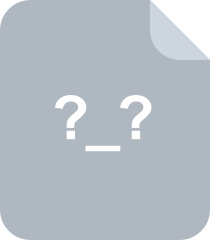
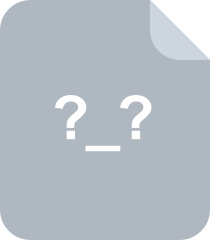
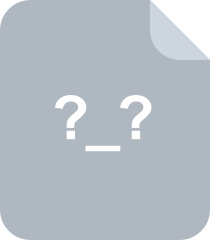
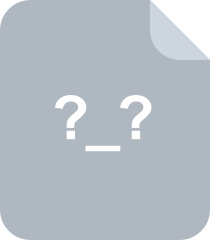
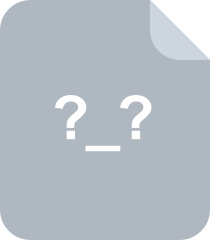
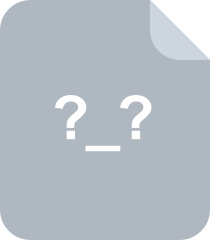
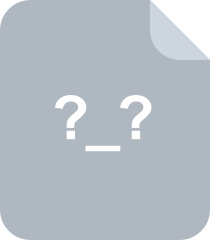
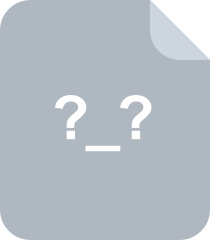
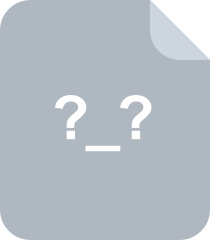
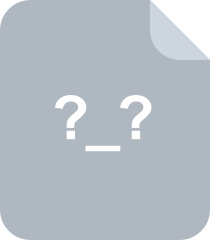
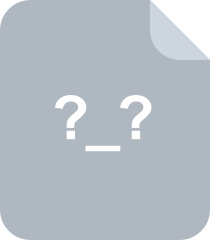
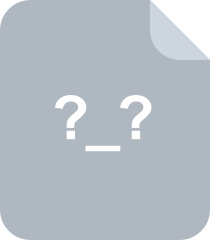
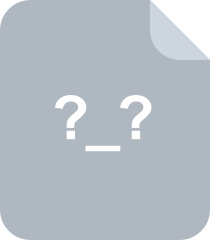
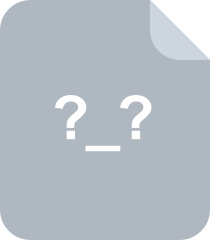
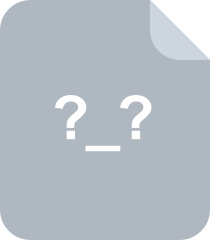
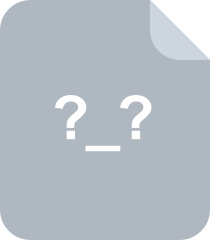
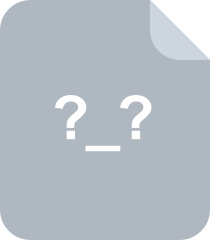
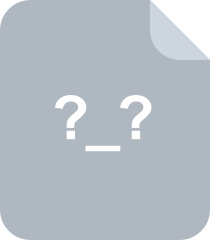
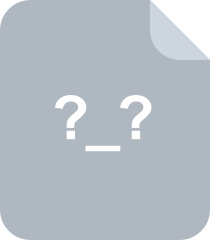
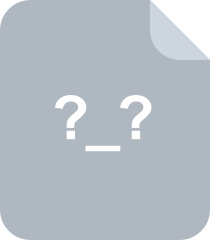
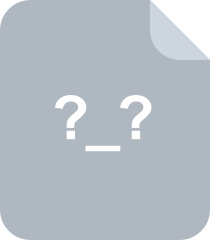
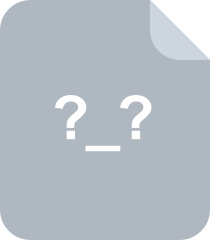
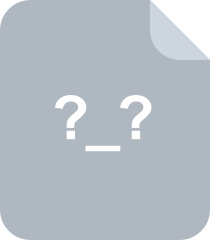
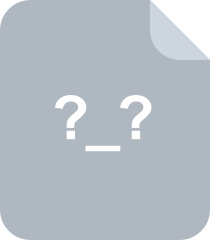
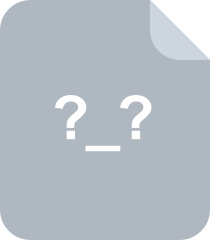
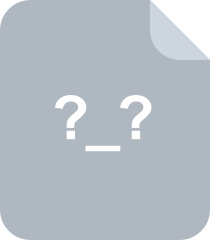
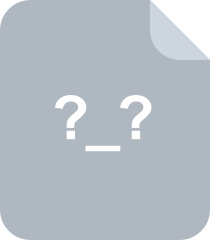
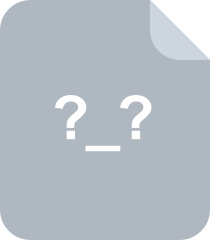
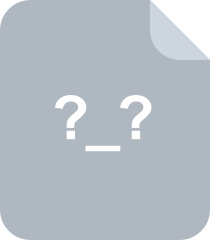
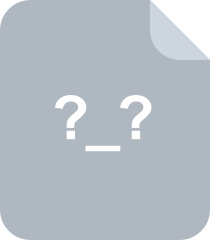
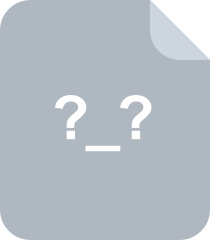
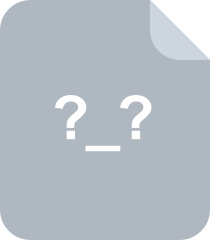
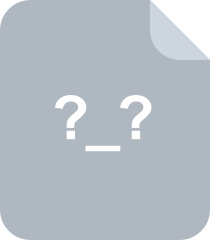
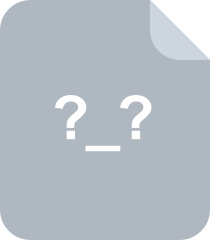
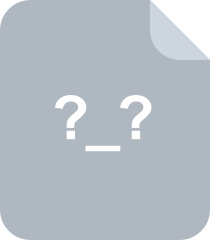
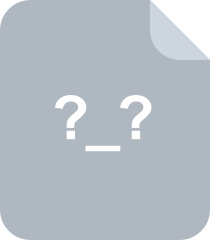
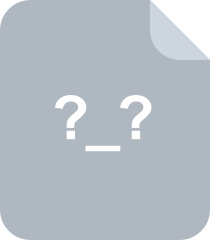
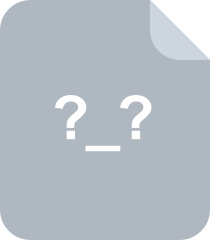
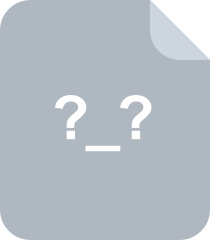
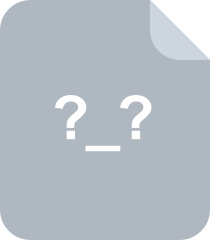
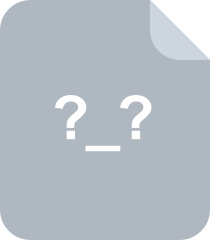
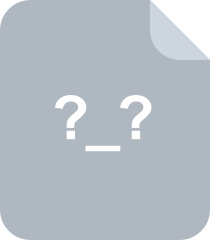
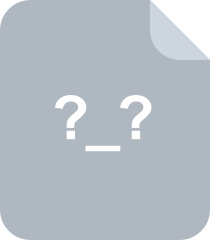
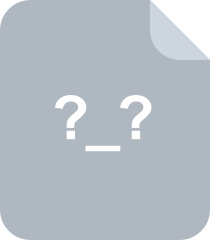
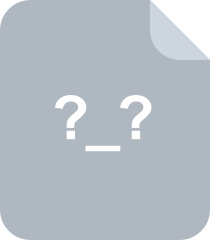
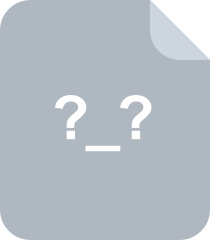
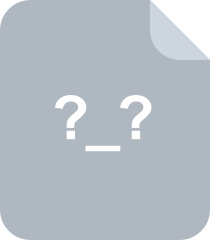
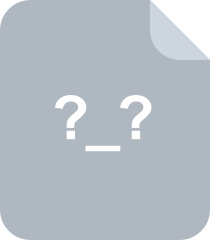
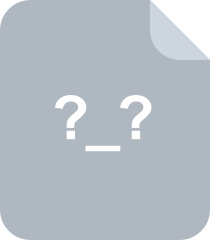
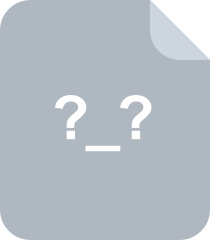
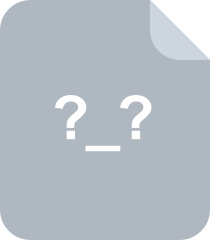
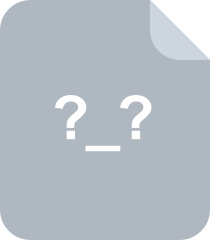
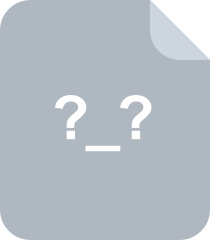
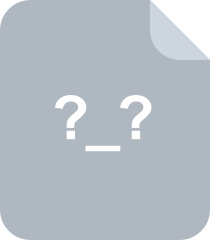
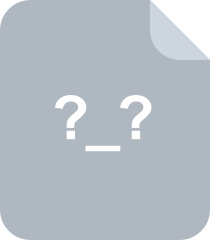
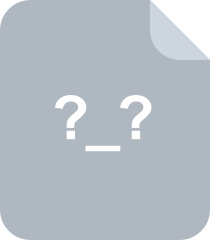
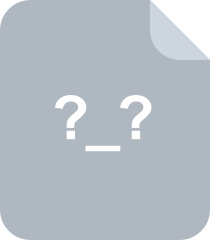
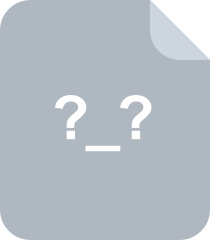
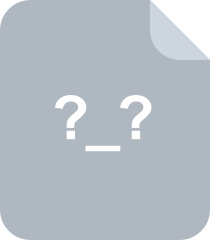
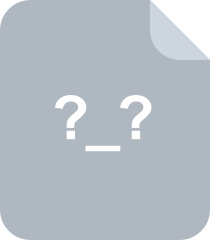
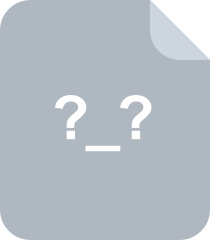
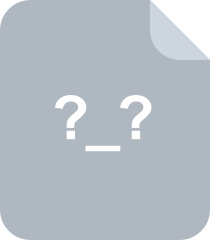
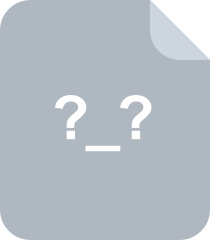
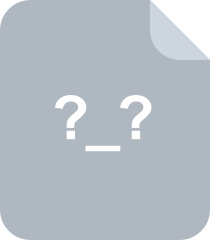
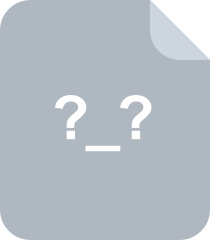
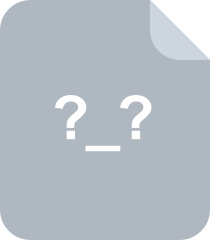
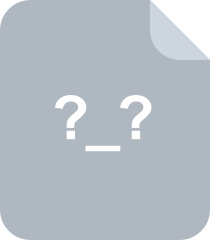
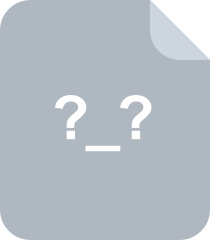
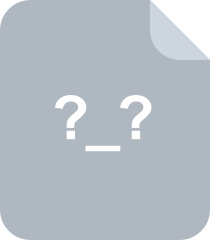
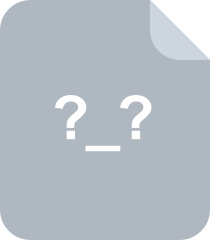
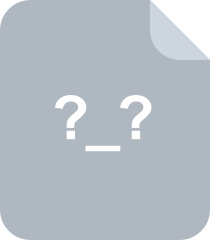
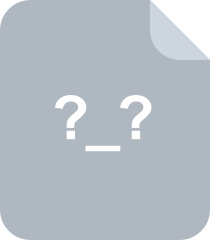
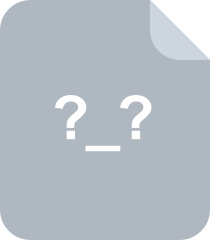
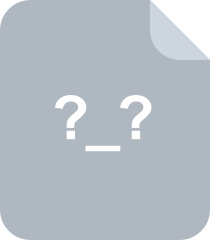
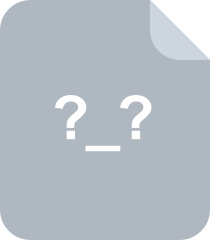
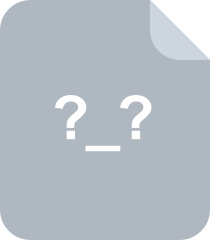
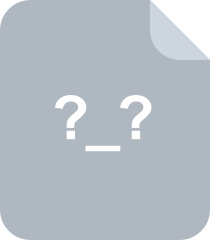
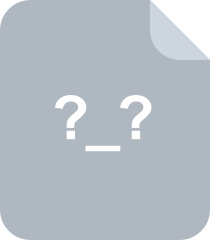
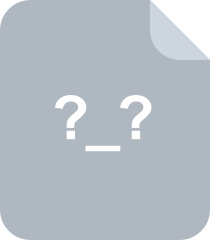
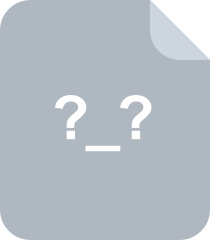
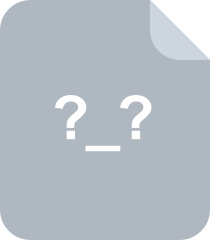
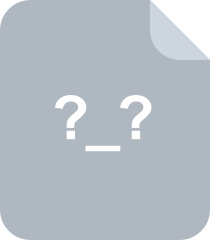
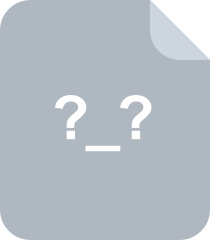
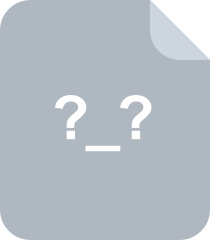
共 1472 条
- 1
- 2
- 3
- 4
- 5
- 6
- 15
资源评论
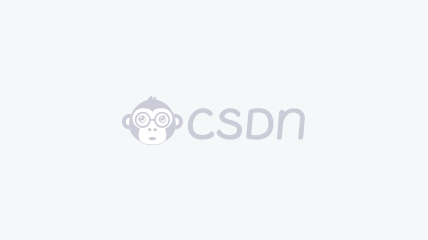


白话机器学习
- 粉丝: 1w+
- 资源: 7673
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

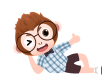
最新资源
- C2000DSP-BootLoader-GUI C#
- 图像分割,训练数据集,train-15【train-11~train-20免积分】
- cc数据库安装包操作系统dll问题解决
- 二手房数据-数据分析练习资源(csv表格)
- 图像分割,训练数据集,train-13【train-11~train-85免积分】
- Unity 山水树木的资源文件
- templatespider-机器人开发资源源代码
- 基于springboot的健身房管理系统(可做毕设参考)+源码+文档+sql.rar
- Spring Cloud电商项目精讲:架构设计与开发技巧课程
- 图像分割,训练数据集,train-11【train-11~train-95免积分】
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


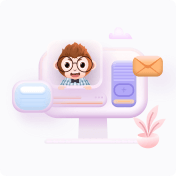
安全验证
文档复制为VIP权益,开通VIP直接复制
