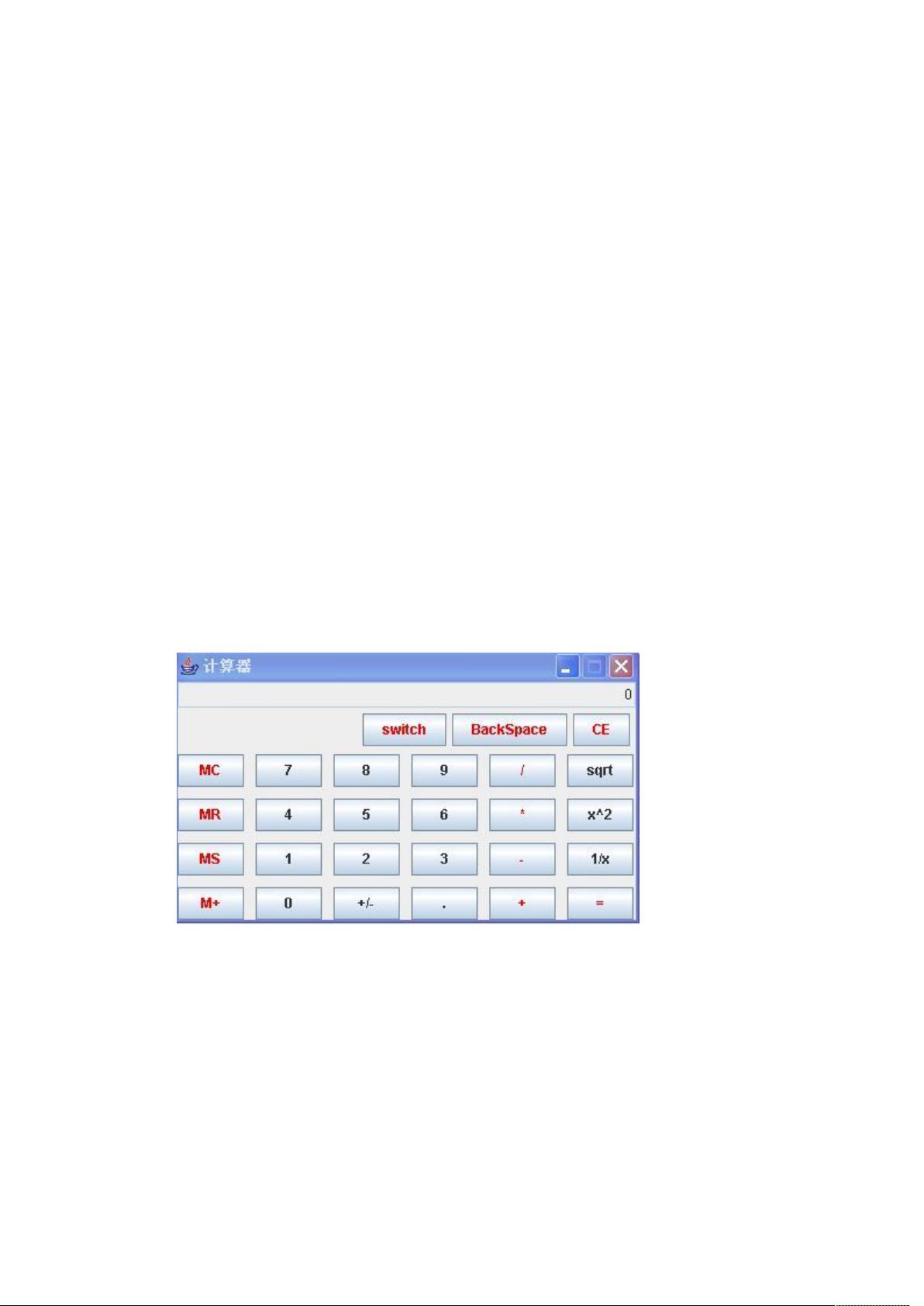
计算器课程设计
——章小龙 软件三班 20062377
一、 原理及设计方法
原理很简单,就是仿 Windows 计算器实现一些简单的运算。同时增加
了
四则运算的输入判错处理。这里采用 JAVA 语言实现,界面各按钮的功能很简
单,差不多就是 Windows 自带计算器的仿造。输入四则运算判错程序通过一个
switch 按钮进行切换,采用了算符优先文法的相关知识,按运算规则中各运算
的优先关系设置一个运算符优先关系矩阵,同时用两个数组栈保存数字和运算
符(这里用#作为表达式结束符),每次压入运算符都按优先关系矩阵进行比对,
以决定是入栈还是取数作运算。
二、 编程运行环境:
1、 硬件环境:PC 兼容机,AMD Sempron™ Processor 3600+ 2.00GHz
处理器,512M 内存。
2、 软件环境:
Microsoft Windows XP 操作系统(可以移植到大部分机器上)
MyEclipse 6.0.1
3、程序设计语言:java 1.5.0_03
三、 设计实现
1. 界面的设计
如上图:各个板块及按钮的功能很明显一看就懂。(关于 MC,MR,MS,M+几个按钮也
是观察 Windows 计算器知道的,MS 保存显示框中的数字,MC 清楚保存的
数字,MR 将保存的数字显示到显示框,M+将保存的数字作*2 后进行保
存)
2. 程序源代码
程序源代码如下(附有详细的注释信息):
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.FlowLayout;
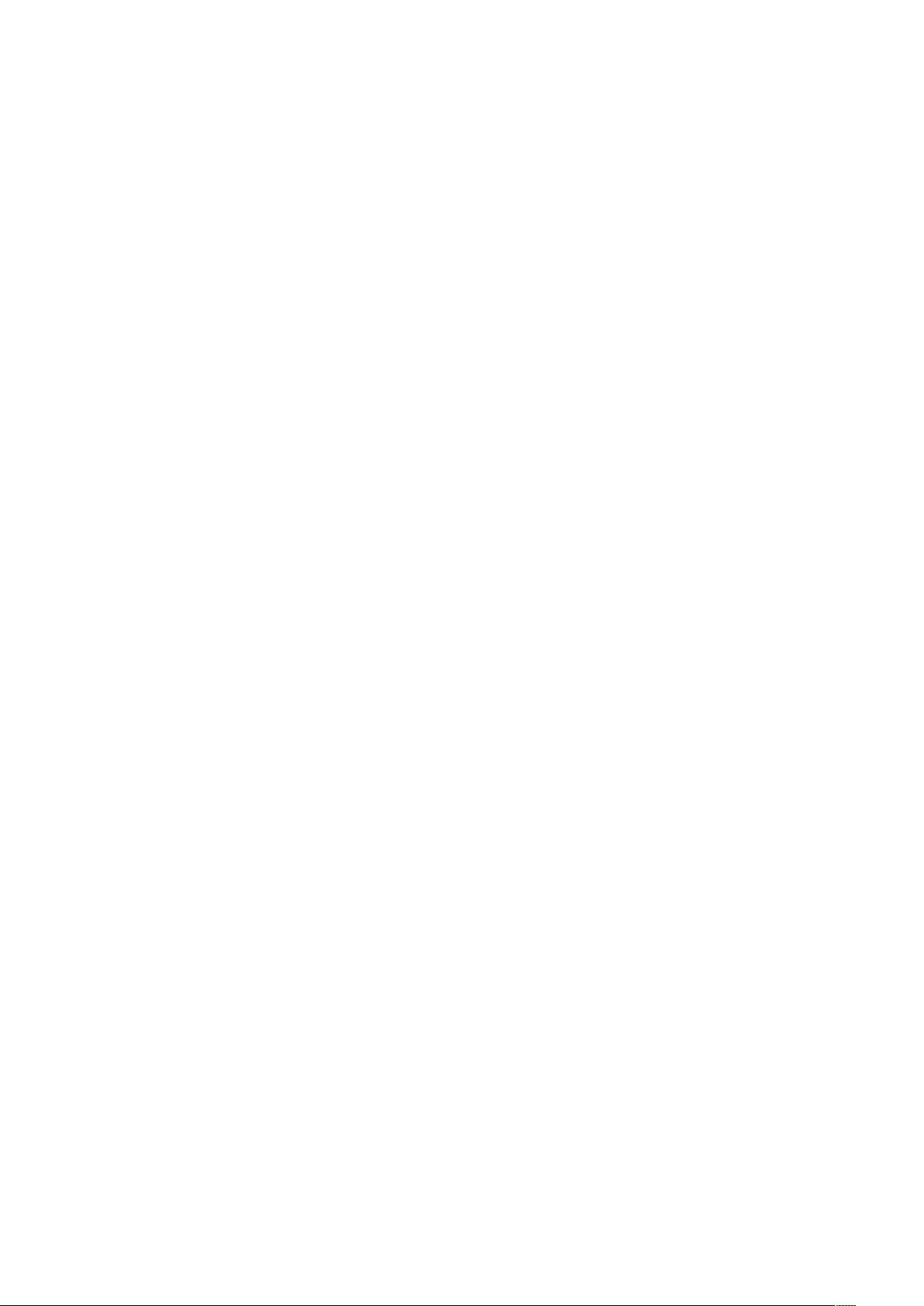
import java.awt.GridLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JTextField;
import javax.swing.SwingConstants;
class Topanel extends JPanel{
JTextField text=new JTextField("0");//text先是输入和结果
JButton backspace=new JButton("BackSpace");
JButton ce=new JButton("CE");//置0键
JButton mswitch=new JButton("switch");//输入模式转换
JPanel toolpanel=new JPanel();
JLabel mshow=new JLabel();
Topanel(){
text.setHorizontalAlignment(SwingConstants.RIGHT);//将输入的数字
或得到的结果在text的右边显示
text.setEditable(false);//文本框不能编辑
mshow.setForeground(Color.red);
ce.setForeground(Color.red);
mswitch.setForeground(Color.red);
backspace.setForeground(Color.red);
toolpanel.setLayout(new FlowLayout(FlowLayout.RIGHT));
toolpanel.add(mswitch);
toolpanel.add(backspace);
toolpanel.add(ce);
setLayout(new BorderLayout());//设定布局管理器边框布局
add(text,BorderLayout.NORTH);//text放置在窗体的中间
add(mshow,BorderLayout.WEST);
add(toolpanel,BorderLayout.EAST);
}
}
class Number_Key extends JPanel{
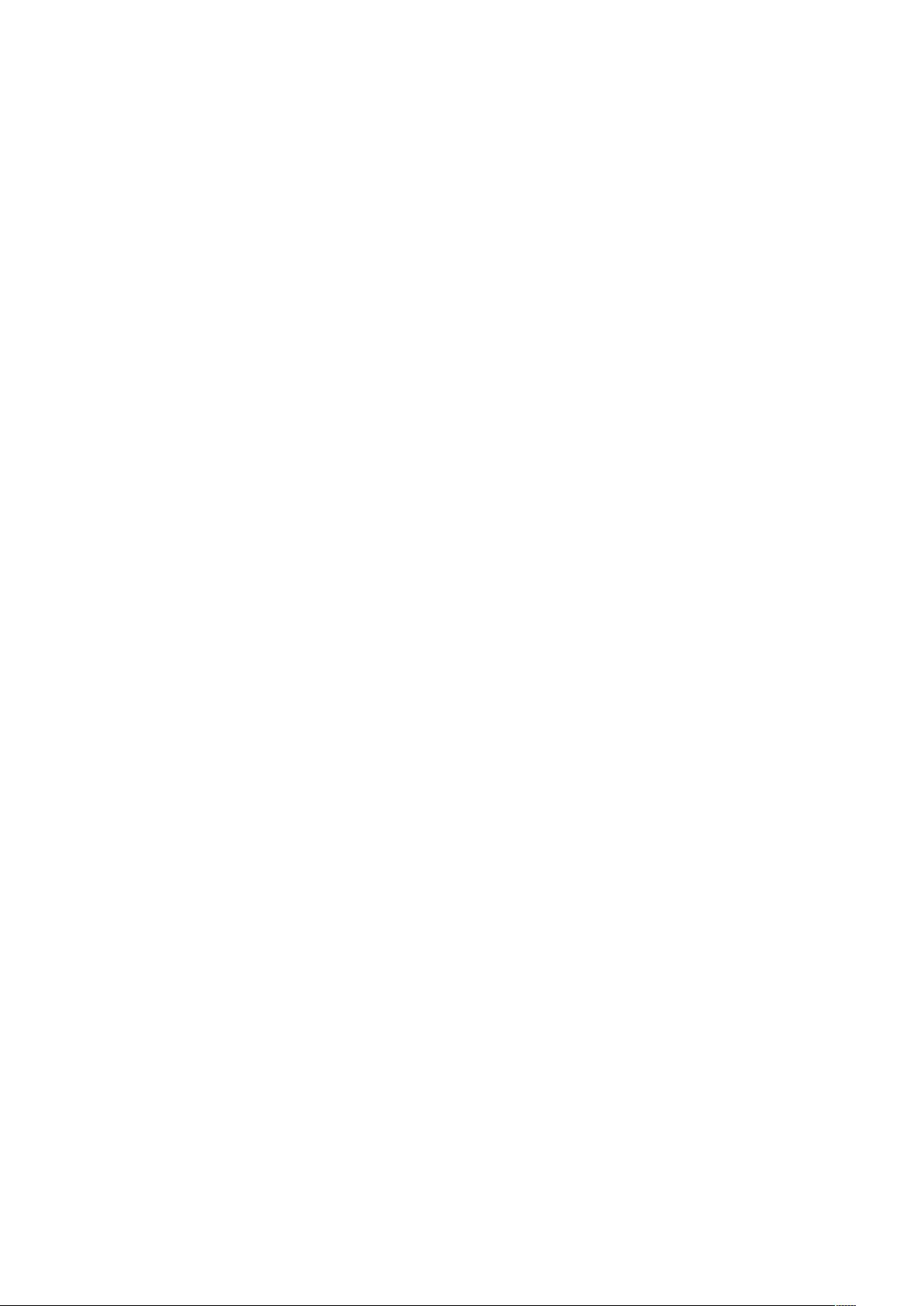
JButton zero=new JButton("0");
JButton one=new JButton("1");
JButton two=new JButton("2");
JButton three=new JButton("3");
JButton four=new JButton("4");
JButton five=new JButton("5");
JButton six=new JButton("6");
JButton seven=new JButton("7");
JButton eight=new JButton("8");
JButton nine=new JButton("9");
JButton plus=new JButton("+");
JButton sub=new JButton("-");
JButton mul=new JButton("*");
JButton div=new JButton("/");
JButton equal=new JButton("=");
JButton porn=new JButton("+/-");
JButton point=new JButton(".");
JButton MC=new JButton("MC");
JButton MR=new JButton("MR");
JButton MS=new JButton("MS");
JButton M=new JButton("M+");
JButton sqrt=new JButton("sqrt");
JButton coutdown=new JButton("1/x");
JButton square=new JButton("x^2");
Number_Key(){
plus.setForeground(Color.red);
sub.setForeground(Color.red);
mul.setForeground(Color.red);
div.setForeground(Color.red);
equal.setForeground(Color.red);
MC.setForeground(Color.red);
MR.setForeground(Color.red);
MS.setForeground(Color.red);
M.setForeground(Color.red);
setLayout(new GridLayout(4,6,10,10));
add(MC);
add(seven);
add(eight);
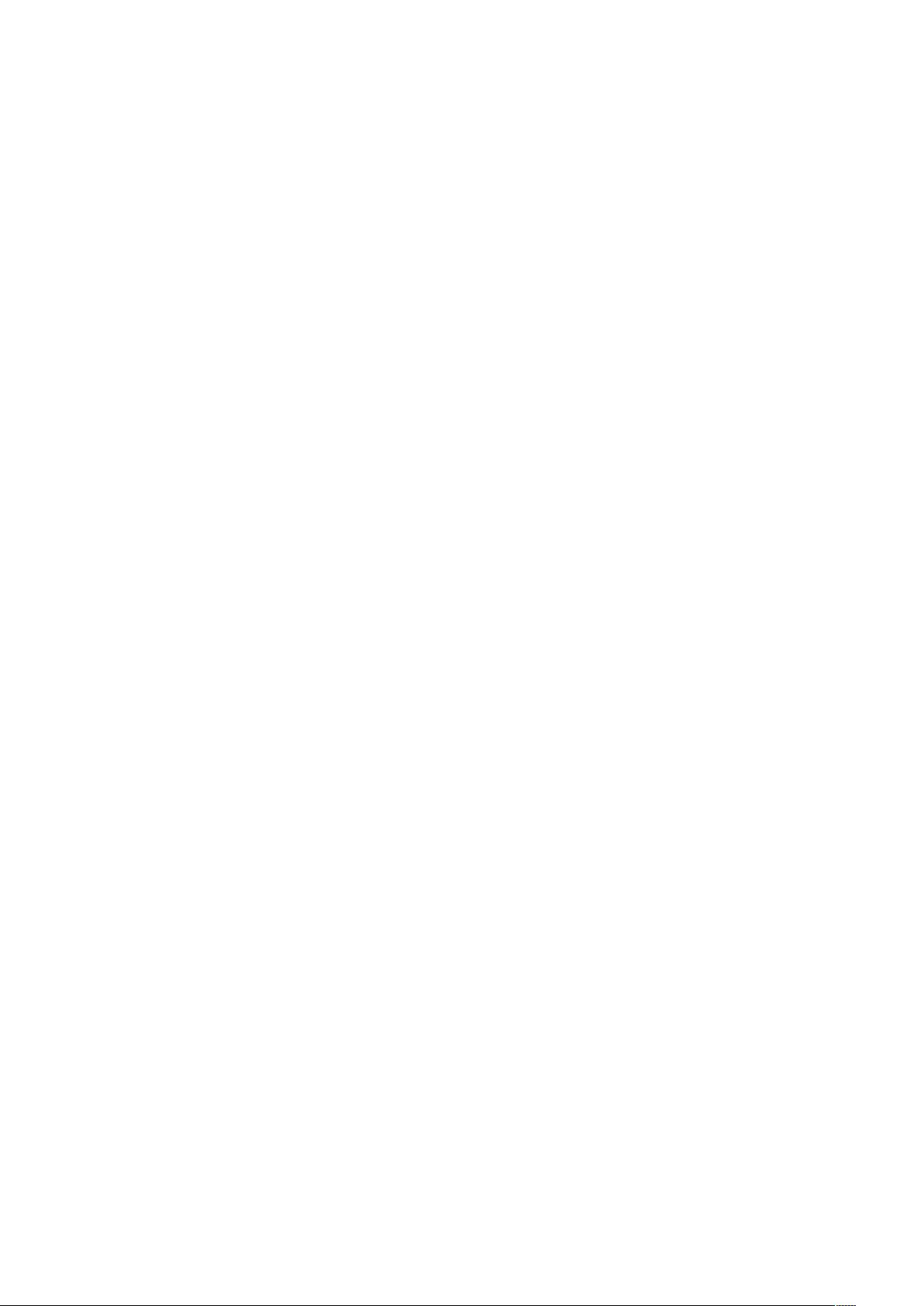
add(nine);
add(div);
add(sqrt);
add(MR);
add(four);
add(five);
add(six);
add(mul);
add(square);
add(MS);
add(one);
add(two);
add(three);
add(sub);
add(coutdown);
add(M);
add(zero);
add(porn);
add(point);
add(plus);
add(equal);
}
}
public class SmartCalculator extends JFrame implements
ActionListener{
Topanel top=new Topanel();
Number_Key number_key=new Number_Key();
int i=0,j=0; //当i=0时说明是我们第一次输入,字符串sum不会累加.当j=0时说
明我第一次输入运算符,不会立即输出结果
String sum=" "; //存放text的内容
String sum1=""; //将text内容存入内存中
double total=0; //存放击按钮+,-,*,/之前的数值
double k=0; //用k来暂存输入的加数,以便连续按“=”总用k来计算
int symbol=0; //+,-,*,/的代号分别为1,2,3,4
boolean isSwitched=false;//是否有按下输入状态转换键
SmartCalculator(){
super("计算器");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);//设定关闭窗体时
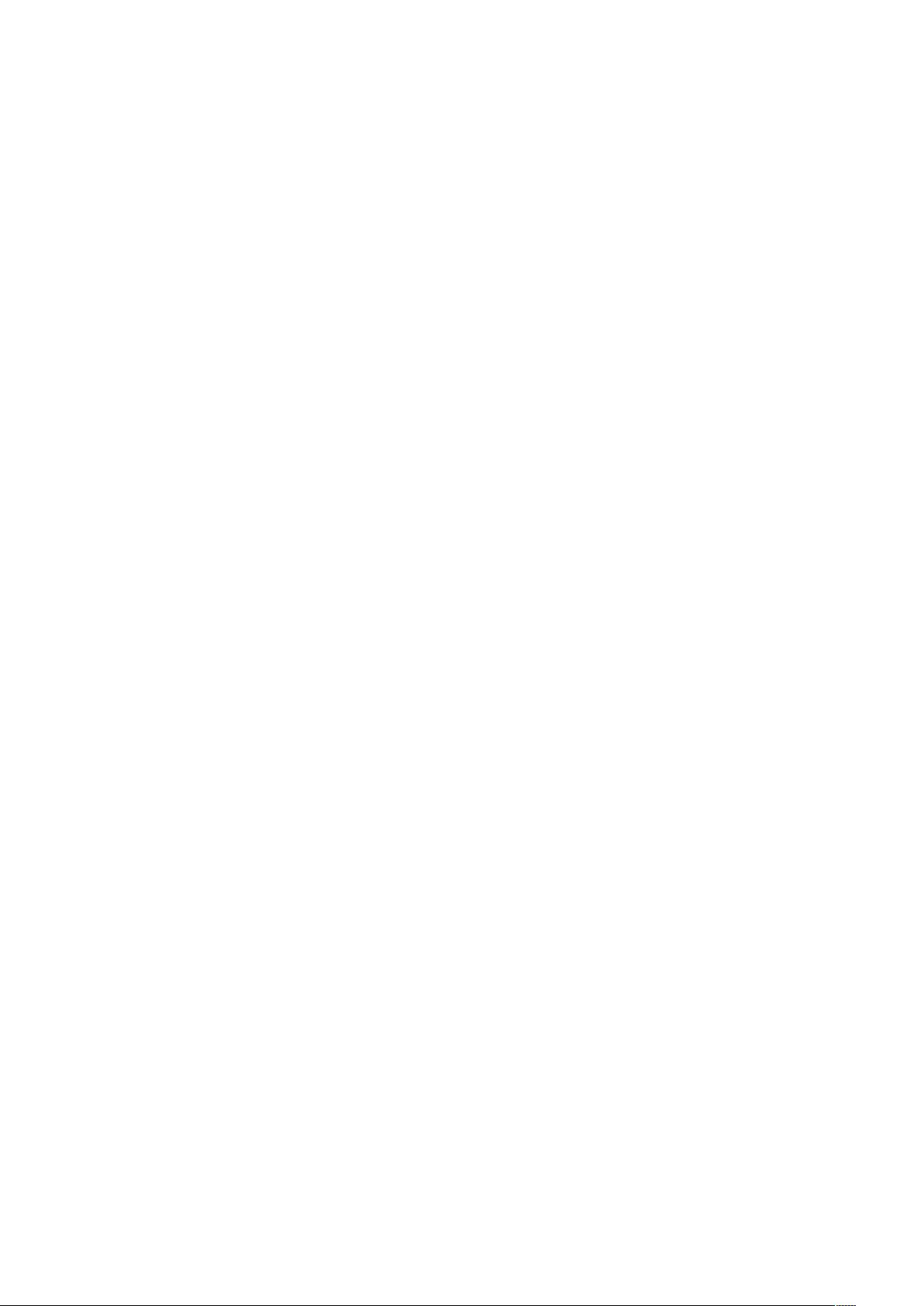
退出程序
JPanel pane=new JPanel();
pane.setLayout(new BorderLayout());
pane.add(top,BorderLayout.NORTH);
pane.add(number_key,BorderLayout.SOUTH);
top.backspace.addActionListener(this);
top.mswitch.addActionListener(this);
top.ce.addActionListener(this);
number_key.one.addActionListener(this);
number_key.two.addActionListener(this);
number_key.three.addActionListener(this);
number_key.four.addActionListener(this);
number_key.five.addActionListener(this);
number_key.six.addActionListener(this);
number_key.seven.addActionListener(this);
number_key.eight.addActionListener(this);
number_key.nine.addActionListener(this);
number_key.zero.addActionListener(this);
number_key.plus.addActionListener(this);
number_key.sub.addActionListener(this);
number_key.mul.addActionListener(this);
number_key.div.addActionListener(this);
number_key.point.addActionListener(this);
number_key.porn.addActionListener(this);
number_key.sqrt.addActionListener(this);
number_key.square.addActionListener(this);
number_key.coutdown.addActionListener(this);
number_key.equal.addActionListener(this);
number_key.MS.addActionListener(this);
number_key.MC.addActionListener(this);
number_key.MR.addActionListener(this);
number_key.M.addActionListener(this);
setContentPane(pane);
pack(); setResizable(false);
}
public void actionPerformed(ActionEvent e){
//击数字按钮前先要判断是否在此之前击了数字按钮及小数点,如果是,那么i=1,