package com.zxc.struts.updownload.services;
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStreamReader;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import org.apache.struts.upload.FormFile;
import bean.DBAccess;
import bean.FileInfo;
public class FileService {
DBAccess db = new DBAccess();
public boolean isExsitSameFileName(String filename,
HttpServletRequest request) {
boolean flag = false;
PreparedStatement ps = null;
ResultSet rs = null;
Connection connection = db.getConnection();
String sql = "select * from file where filename=?";
try {
ps = connection.prepareStatement(sql);
ps.setString(1, filename);
rs = ps.executeQuery();
if (rs.next()) {
FileInfo fileInfo = new FileInfo();
fileInfo.setFileId(rs.getString("fileid"));
fileInfo.setFileInfo(rs.getString("fileinfo"));
fileInfo.setFileIp(rs.getString("fileip"));
fileInfo.setFileName(rs.getString("filename"));
fileInfo.setFileSavename(rs.getString("filesavename"));
fileInfo.setFileSize((rs
.getString("filesize")));
fileInfo.setFileTag(rs.getString("filetag"));
fileInfo.setFileTimes(Integer.parseInt(rs
.getString("filetimes")));
fileInfo.setFileType(rs.getString("filetype"));
fileInfo.setFileUptime(rs.getString("fileuptime"));
java.io.File f = new java.io.File(request.getSession()
.getServletContext().getRealPath("/")
+ fileInfo.getFileSavename());
if (f.isFile())
flag = true;
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
if (rs != null)
rs.close();
if (ps != null)
ps.close();
connection.close();
} catch (Exception e) {
e.printStackTrace();
}
}
return flag;
}
public boolean saveFile(FileInfo fileInfo) {
boolean flag = false;
PreparedStatement ps = null;
Connection connection = db.getConnection();
String sql = "insert into file(fileId,fileSavename,fileName,fileType,fileSize,fileInfo,fileUptime,fileIp,fileTimes,fileTag) "
+ "values(?,?,?,?,?,?,?,?,?,?)";
try {
ps = connection.prepareStatement(sql);
ps.setString(1, fileInfo.getFileId());
ps.setString(2, fileInfo.getFileSavename());
ps.setString(3, fileInfo.getFileName());
ps.setString(4, fileInfo.getFileType());
ps.setString(5, String.valueOf(fileInfo.getFileSize()));
String fileString = fileInfo.getFileInfo();
ps.setString(6, fileString);
ps.setString(7, fileInfo.getFileUptime());
ps.setString(8, fileInfo.getFileIp());
ps.setString(9, String.valueOf(fileInfo.getFileTimes()));
ps.setString(10, fileInfo.getFileTag());
int i = ps.executeUpdate();
if (i == 1)
flag = true;
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
ps.close();
connection.close();
} catch (Exception e) {
e.printStackTrace();
}
}
return flag;
}
public List queryFile(HttpServletRequest request) {
boolean flag = false;
PreparedStatement ps = null;
PreparedStatement ps1 = null;
ResultSet rs = null;
List list = new ArrayList<FileInfo>();
Connection connection = db.getConnection();
String sql1 = "select * from file order by fileuptime desc";
String sql2 = "delete from file where filesavename=?";
try {
ps = connection.prepareStatement(sql1);
rs = ps.executeQuery();
while (rs.next()) {
FileInfo fileInfo = new FileInfo();
fileInfo.setFileId(rs.getString("fileid"));
fileInfo
.setFileInfo(changehtml(formatString(rs.getString("fileinfo"), 15)));
fileInfo.setFileInfoReal(rs.getString("fileinfo"));
fileInfo.setFileIp(rs.getString("fileip"));
String filename = rs.getString("filename");
fileInfo.setFileName(filename);
fileInfo.setFileSavename(rs.getString("filesavename"));
Double filesize=Double.parseDouble(rs.getString("filesize"));
fileInfo.setFileSize(formatFileSize(filesize));
fileInfo.setFileTag(rs.getString("filetag"));
fileInfo.setFileTimes(Integer.parseInt(rs
.getString("filetimes")));
fileInfo.setFileType(rs.getString("filetype"));
fileInfo.setFileUptime(rs.getString("fileuptime"));
java.io.File f = new java.io.File(request.getSession()
.getServletContext().getRealPath("/")
+ fileInfo.getFileSavename());
if (f.isFile())
list.add(fileInfo);
else {
ps1 = connection.prepareStatement(sql2);
ps1.setString(1, fileInfo.getFileSavename());
ps1.executeUpdate();
}
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
rs.close();
ps.close();
connection.close();
} catch (Exception e) {
e.printStackTrace();
}
}
System.out.println("查询列表成功!");
return list;
}
public FileInfo findFileByName(String filesavename) {
PreparedStatement ps = null;
ResultSet rs = null;
Connection connection = db.getConnection();
FileInfo fileInfo = null;
String sql = "select * from file where filesavename=?";
try {
ps = connection.prepareStatement(sql);
ps.setString(1, filesavename);
rs = ps.executeQuery();
if (rs.next()) {
fileInfo = new FileInfo();
fileInfo.setFileId(rs.getString("fileid"));
fileInfo
.setFileInfo(formatString(rs.getString("fileinfo"), 15));
fileInfo.setFileInfoReal(rs.getString("fileinfo"));
fileInfo.setFileIp(rs.getString("fileip"));
fileInfo.setFileName(rs.getString("filename"));
fileInfo.setFileSavename(rs.getString("filesavename"));
fileInfo.setFileSize((rs
.getString("filesize")));
fileInfo.setFileTag(rs.getString("filetag"));
fileInfo.setFileTimes(Integer.parseInt(rs
.getString("filetimes")));
fileInfo.setFileType(rs.getString("filetype"));
fileInfo.setFileUptime(rs.getString("fileuptime"));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
rs.close();
ps.close();
connection.close();
} catch (Exception e) {
e.printStackTrace();
}
}
return fileInfo;
}
public FileInfo findFileById(String fileid) {
PreparedStatement ps = null;
ResultSet rs = null;
Connection connection = db.getConnection();
FileInfo fileInfo = null;
String sql = "select * from file where fileid=?";
try {
ps = connection.prepareStatement(sql);
ps.setString(1, fileid);
rs = ps.executeQuery();
if (rs.next()) {
fileInfo = new FileInfo();
fileInfo.setFileId(rs.getString("fileid"));
fileInfo
.setFileInfo(formatString(rs.getString("fileinfo"), 15));
fileInfo.setFileInfoReal(rs.getString("fileinfo"));
fileInfo.setFileIp(rs.getString("fileip"));
fileInfo.setFileName(rs.getString("filename"));
fileInfo.setFileSavename(rs.getString("filesavename"));
fileInfo.setFileSize((rs
.getString("filesize")));
fileInfo.setFileTag(rs.getString("filetag"));
fileInfo.setFileTimes(Integer.parseInt(rs
.getString("filetimes")));
fileInfo.setFileType(rs.getString("filetype"));
fileInfo.setFileUptime(rs.getString("fileuptime"));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} finally {
try {
rs.close();
ps.close();
connection.close();
} catch (Exception e) {
e.printStackTrace();
}
}
return fileInfo;
}
public boolean deleteFile(String filesavename) {
boolean flag = false;
PreparedStatement ps = null;
Connection connection = db.getConnection();
String sql = "delete from file where fil
没有合适的资源?快使用搜索试试~ 我知道了~
struts+SmartUpload实现的一个简易上传下载系统
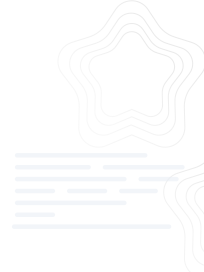
共63个文件
java:16个
class:15个
properties:4个


温馨提示
struts+SmartUpload实现的一个简易上传下载系统,此系统的主要代码已经放到俺博客上了,欢迎访问 “http://blog.csdn.net/zxingchao2009/archive/2010/09/08/5872106.aspx”
资源推荐
资源详情
资源评论
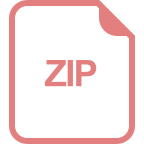
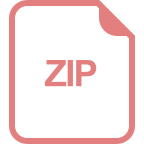
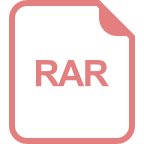
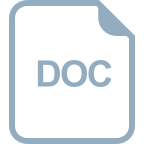
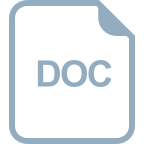
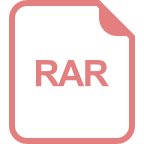
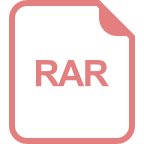
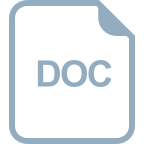
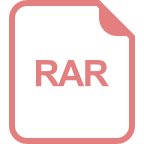
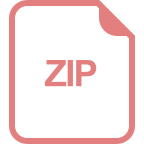
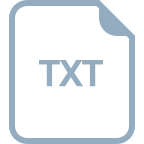
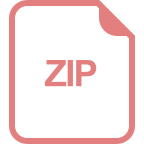
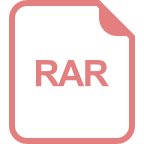
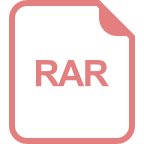
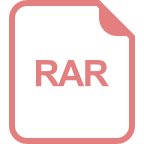
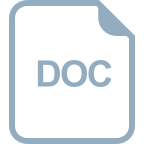
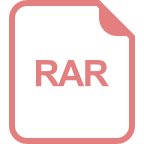
收起资源包目录


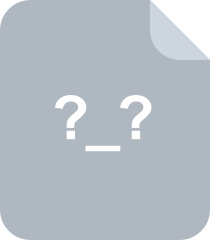

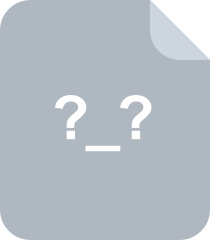

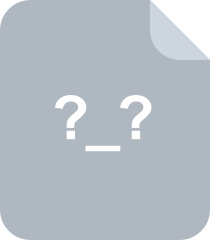
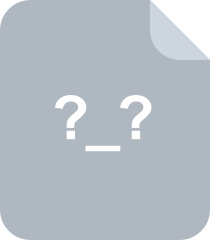
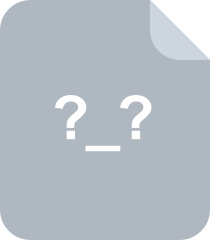
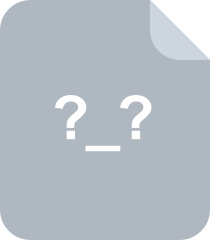
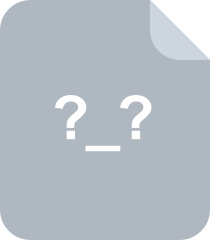
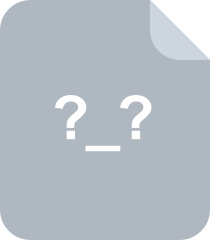

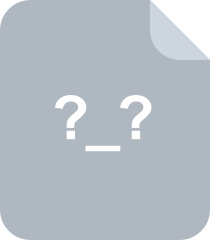


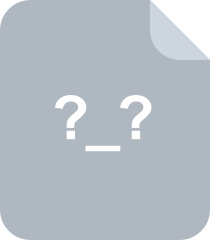
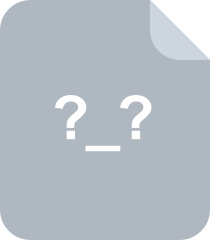
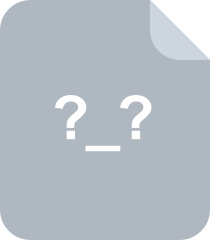

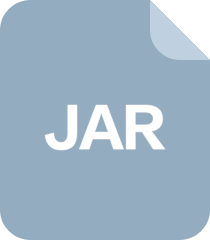
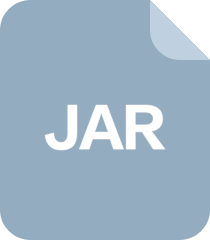
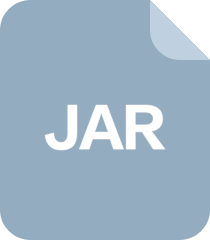
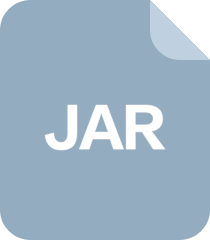


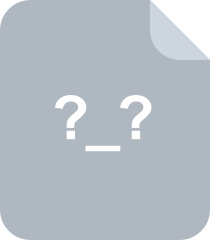
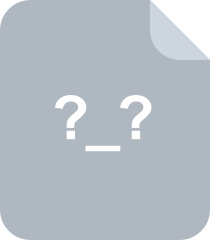
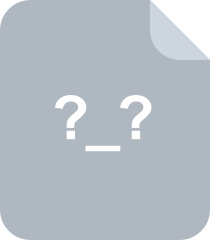
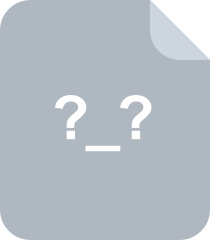
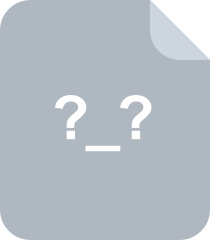
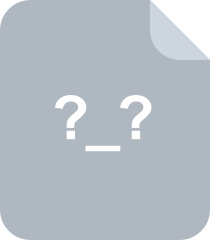

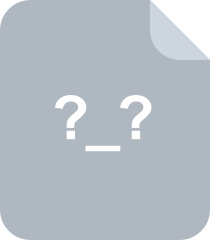
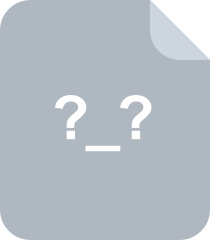




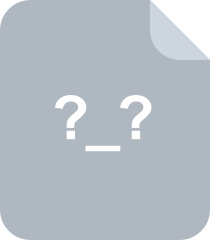
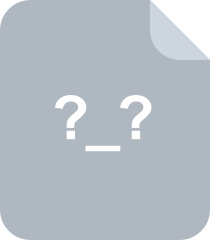
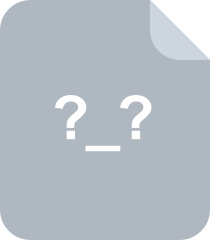
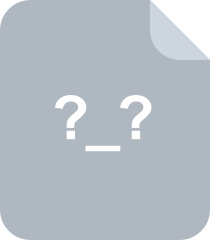

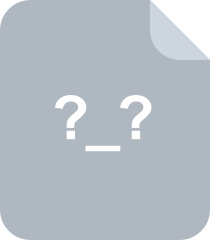
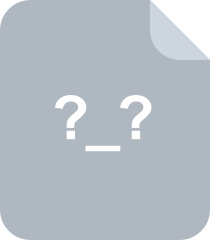

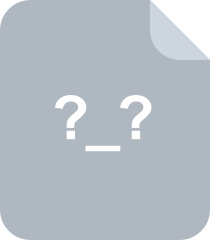
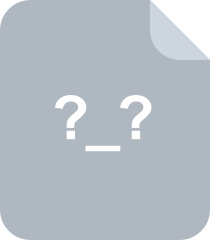
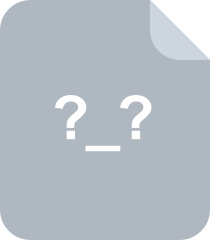
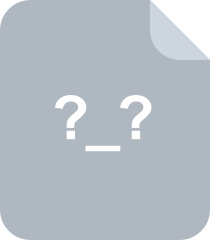

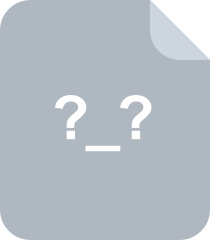
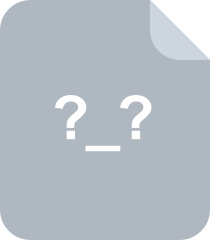
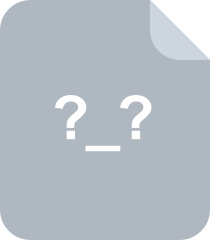

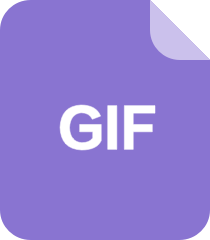
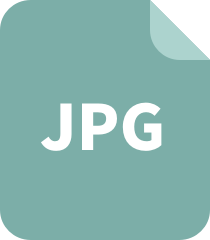
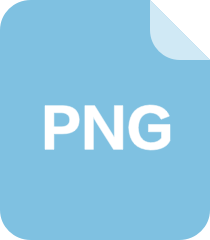
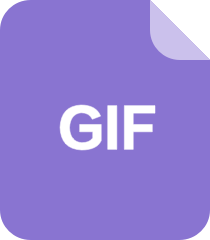
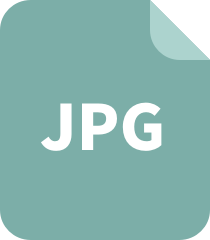

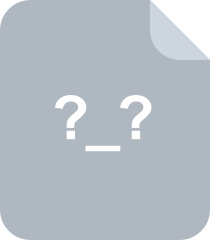




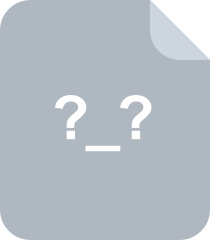
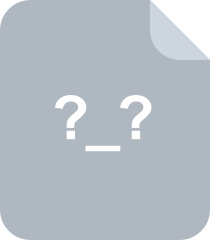
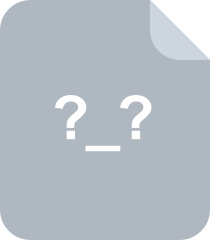
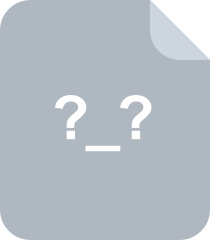
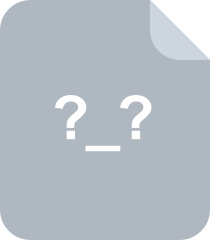
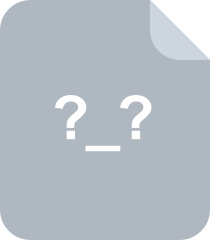

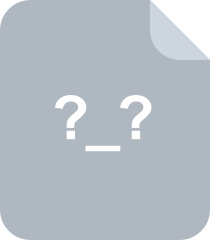
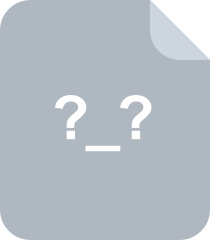




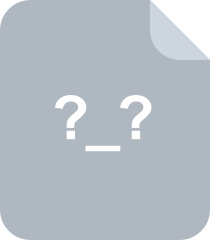
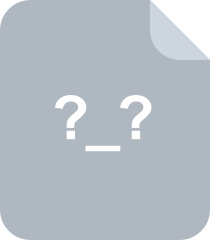
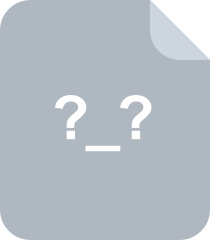
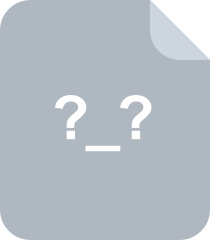

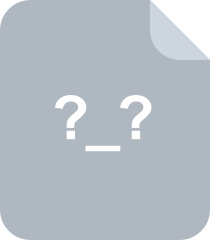
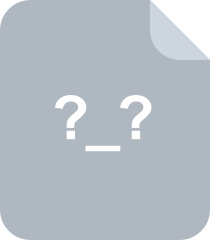

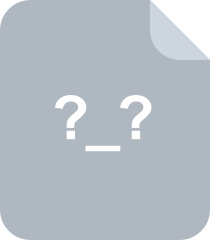
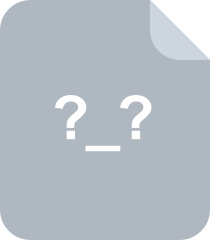
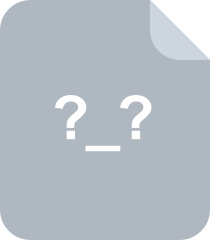
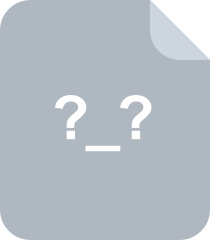
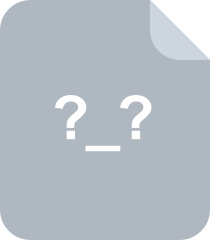
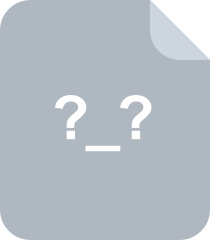
共 63 条
- 1

UPC_思念
- 粉丝: 290
- 资源: 40
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

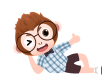
最新资源
- 学校课程软件工程常见10道题目以及答案demo
- javaweb新手开发中常见的目录结构讲解
- 新手小白的git使用的手册入门学习demo
- 基于Java观察者模式的info-express多对多广播通信框架设计源码
- 利用python爬取豆瓣电影评分简单案例demo
- 机器人开发中常见的几道问题以及答案demo
- 基于SpringBoot和layuimini的简洁美观后台权限管理系统设计源码
- 实验报告五六代码.zip
- hdw-dubbo-ui基于vue、element-ui构建开发,实现后台管理前端功能.zip
- (Grafana + Zabbix + ASP.NET Core 2.1 + ECharts + Dapper + Swagger + layuiAdmin)基于角色授权的权限体系.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


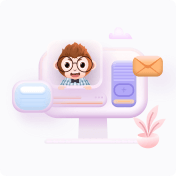
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页