package com.zw.spring;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import javax.sql.DataSource;
import com.zw.spring.dao.BookAddInterface;
public class BookAddImpl implements BookAddInterface {
private DataSource dataSource;
public Connection conn;
private Book book;
public Book getBook() {
return book;
}
public void setBook(Book book) {
this.book = book;
}
public DataSource getDataSource() {
return dataSource;
}
public void setDataSource(DataSource dataSource) {
this.dataSource = dataSource;
}
public boolean addBook(Book book) {
// TODO Auto-generated method stub
System.out.println(book.getAuthor()+","+book.getBookname());
try {
conn=getDataSource().getConnection();
String sql="insert into dbo.book (bookname,price,author,press,pressdate) values (?,?,?,?,?)";
PreparedStatement ptmt=conn.prepareStatement(sql);
ptmt.setString(1,book.getBookname());
ptmt.setString(2, book.getPrice());
ptmt.setString(3, book.getAuthor());
ptmt.setString(4, book.getPress());
ptmt.setString(5,book.getPressdate());
boolean f=ptmt.execute();
if(f==false)
{
return true;
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
System.out.println("失败了");
}
return false;
}
public boolean delete(String ids) {
// TODO Auto-generated method stub
try {
conn=getDataSource().getConnection();
System.out.println(ids);
String[] id=ids.split(",");
for(int i=1;i<id.length;i++)
{
String sql="delete from dbo.book where id="+id[i];
System.out.println(sql);
Statement stmt=conn.createStatement();
stmt.executeUpdate(sql);
}
return true;
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
return false;
}
}
public ArrayList<Book> resultList(String bookname) {
// TODO Auto-generated method stub
ArrayList<Book> array=new ArrayList<Book>();
try {
conn=dataSource.getConnection();
String sql="select * from dbo.book where bookname like '%"+bookname+"%'";
java.sql.Statement stmt=conn.createStatement();
ResultSet result=stmt.executeQuery(sql);
while(result.next())
{
book=new Book();
book.setBookname(result.getString(1));
book.setPrice(result.getString(2));
book.setAuthor(result.getString(3));
book.setPress(result.getString(4));
book.setPressdate(result.getString(5));
book.setId(result.getInt(6));
array.add(book);
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return array;
}
public ArrayList<Book> getAllBooks() {
// TODO Auto-generated method stub
ArrayList<Book> array=new ArrayList<Book>();
try {
conn=dataSource.getConnection();
String sql="select * from book";
java.sql.Statement stmt=conn.createStatement();
ResultSet result=stmt.executeQuery(sql);
while(result.next())
{
book=new Book();
book.setBookname(result.getString(1));
book.setPrice(result.getString(2));
book.setAuthor(result.getString(3));
book.setPress(result.getString(4));
book.setPressdate(result.getString(5));
book.setId(result.getInt(6));
array.add(book);
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return array;
}
public boolean updateBook(Book book) {
// TODO Auto-generated method stub
try {
System.out.println(book.getId());
conn=dataSource.getConnection();
String sql="update book set bookname=?,price=?,author=?,press=?,pressdate=? where id=?";
PreparedStatement ptmt=conn.prepareStatement(sql);
ptmt.setString(1, book.getBookname());
ptmt.setString(2, book.getPrice());
ptmt.setString(3, book.getAuthor());
ptmt.setString(4, book.getPress());
ptmt.setString(5, book.getPressdate());
ptmt.setInt(6, book.getId());
int f=ptmt.executeUpdate();
System.out.println(f);
if(f==1)
{
return true;
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
return false;
}
return false;
}
public Book getBook(int id) {
// TODO Auto-generated method stub
try {
conn=dataSource.getConnection();
Statement stmt=conn.createStatement();
String sql="select * from book where id="+id;
ResultSet result=stmt.executeQuery(sql);
while(result.next())
{
book.setBookname(result.getString(1));
book.setPrice(result.getString(2));
book.setAuthor(result.getString(3));
book.setPress(result.getString(4));
book.setPressdate(result.getString(5));
book.setId(result.getInt(6));
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return book;
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
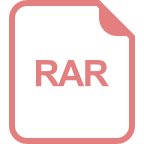
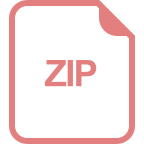
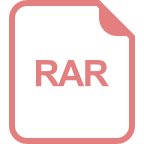
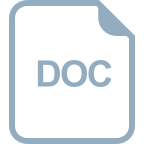
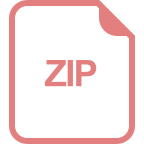
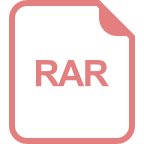
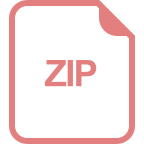
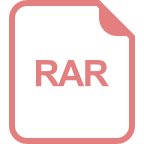
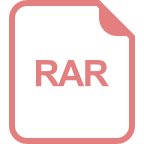
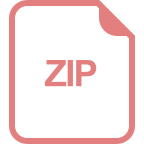
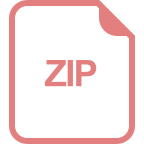
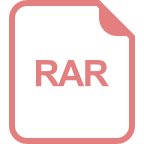
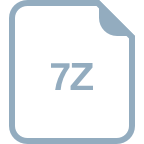
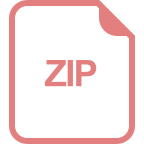
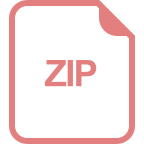
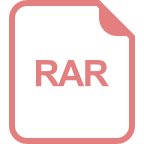
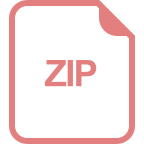
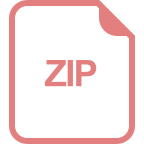
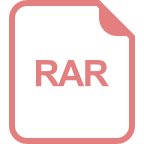
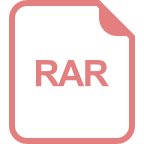
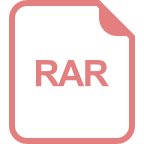
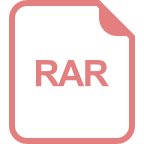
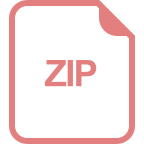
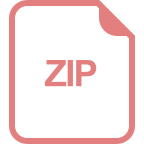
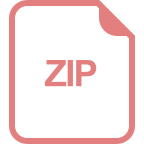
收起资源包目录


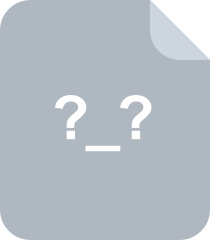

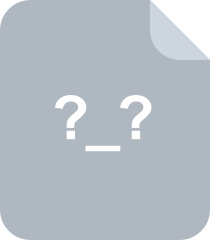





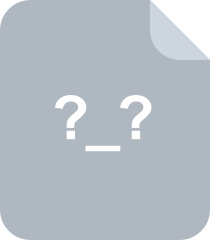
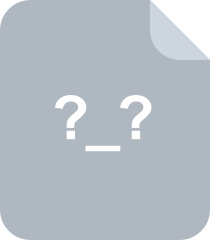
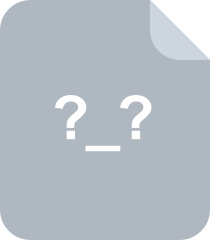


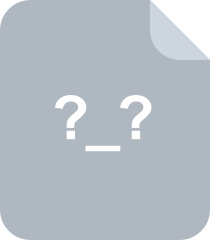
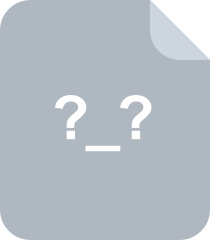
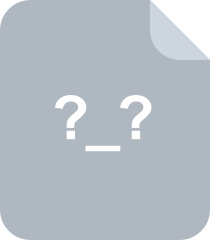
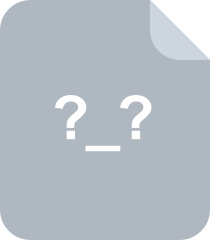
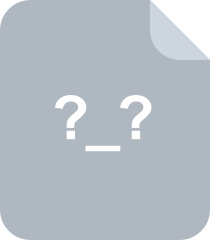
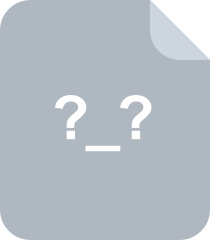
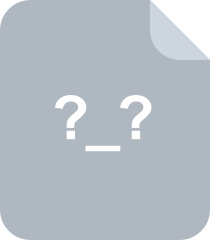
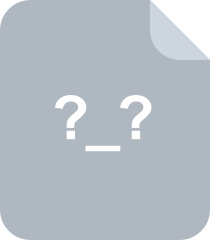
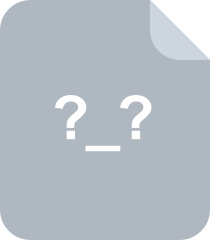
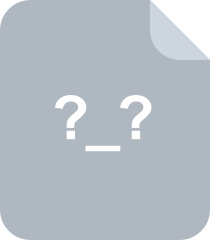


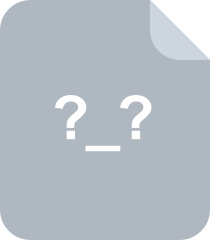

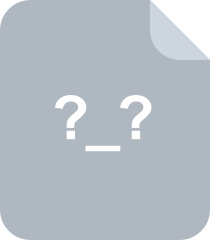





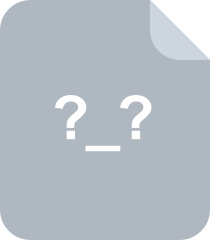
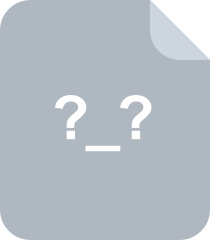
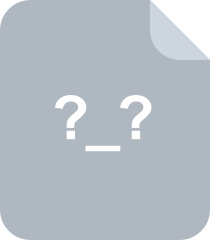


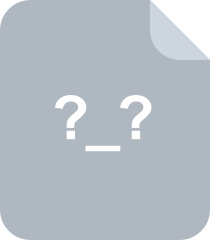
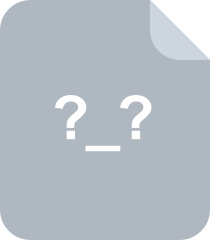
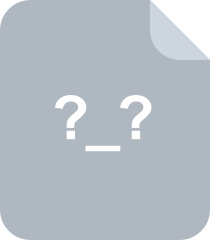
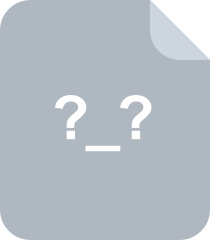
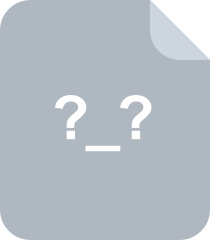
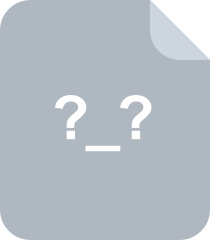
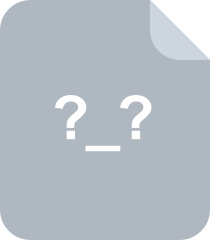
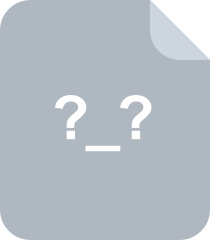
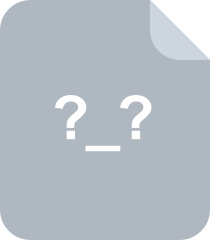

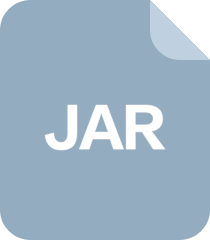
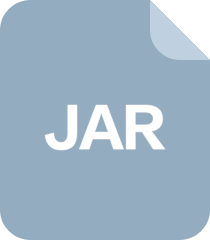
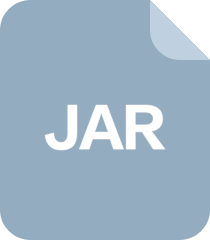
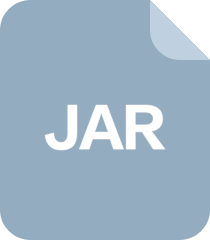
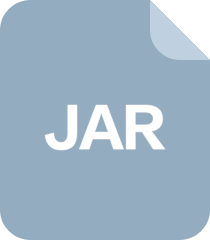
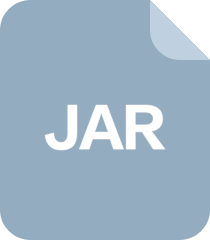
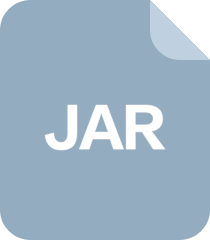
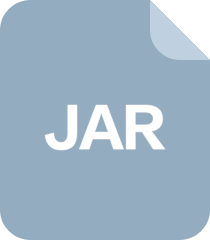
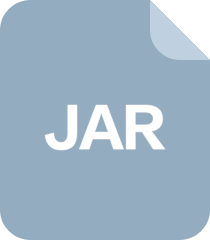
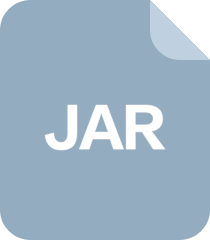
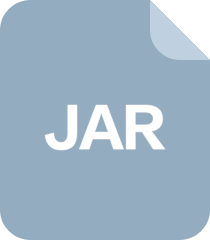
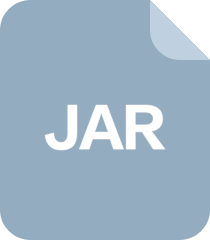
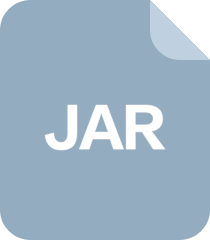
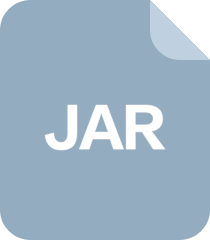
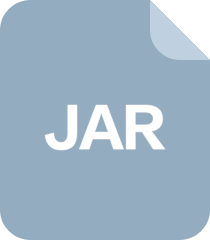
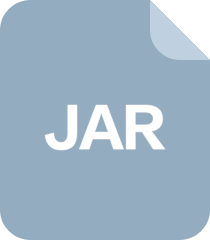
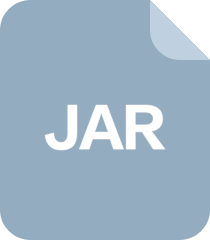
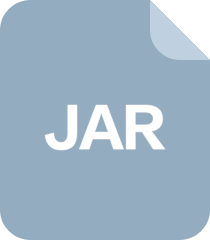
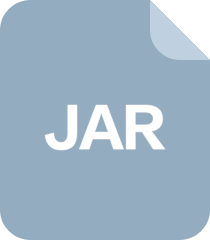
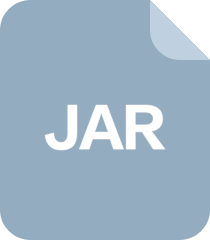
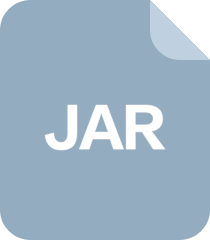
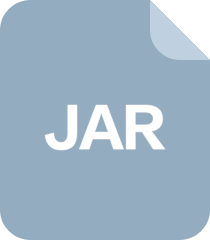
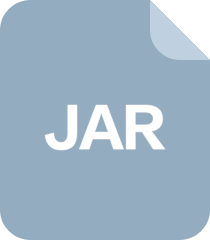
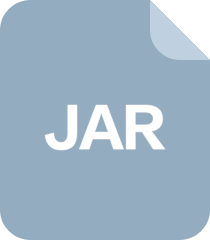
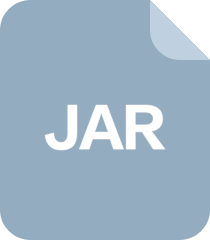
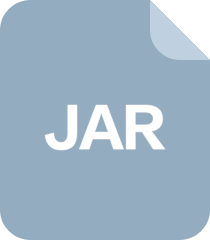
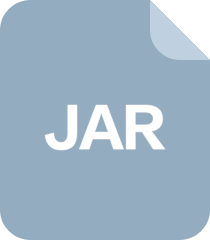
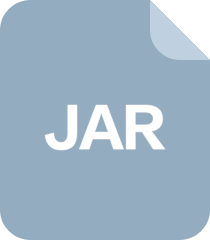
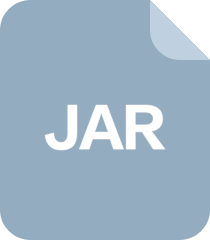
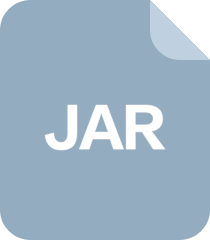
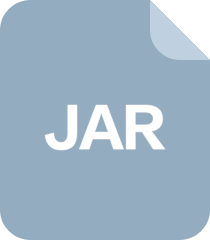
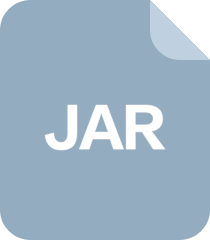
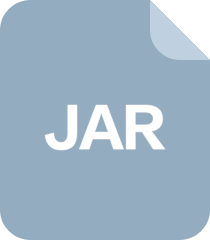
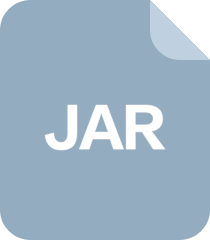
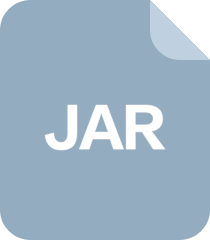
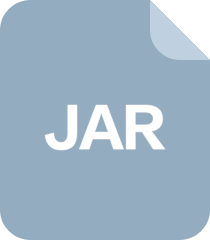
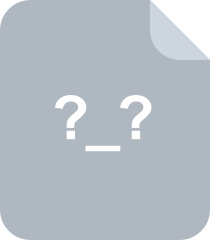
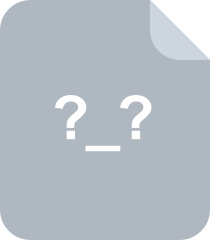
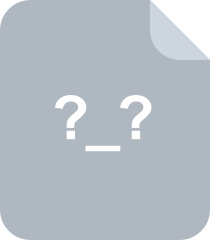
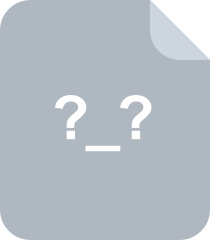
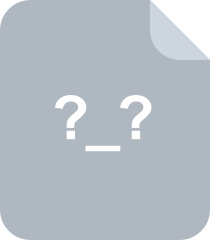
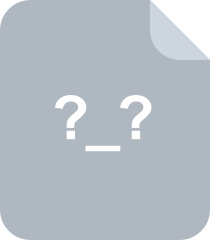
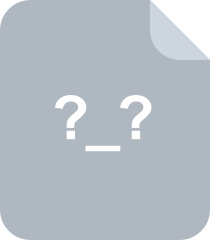
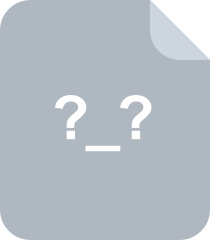
共 73 条
- 1
资源评论
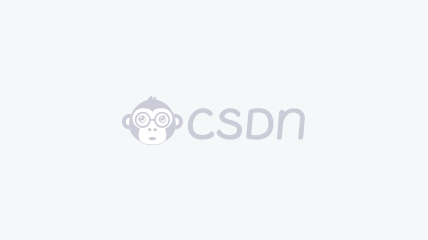
- Evi2013-09-27还行吧,初学
- xuezhimeng20102013-03-01不错的 初学者资料
- x5027139942013-11-26开始配置还好,问题是,配置好了后面还是有一堆问题
- 别怪我-太神经2017-10-30还没用不清楚
- GANLAN12342013-01-04挺好的 初学资料

zwxiaole
- 粉丝: 20
- 资源: 8
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

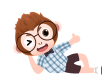
安全验证
文档复制为VIP权益,开通VIP直接复制
