/*
Copyright (c) 2014, Matthias Schiffer <mschiffer@universe-factory.net>
All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions are met:
1. Redistributions of source code must retain the above copyright notice,
this list of conditions and the following disclaimer.
2. Redistributions in binary form must reproduce the above copyright notice,
this list of conditions and the following disclaimer in the documentation
and/or other materials provided with the distribution.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
/*
tplink-safeloader
Image generation tool for the TP-LINK SafeLoader as seen on
TP-LINK Pharos devices (CPE210/220/510/520)
*/
#include <assert.h>
#include <errno.h>
#include <stdbool.h>
#include <stdio.h>
#include <stdint.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#include <unistd.h>
#include <arpa/inet.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <limits.h>
#include "md5.h"
#define ALIGN(x,a) ({ typeof(a) __a = (a); (((x) + __a - 1) & ~(__a - 1)); })
#define MAX_PARTITIONS 32
/** An image partition table entry */
struct image_partition_entry {
const char *name;
size_t size;
uint8_t *data;
};
/** A flash partition table entry */
struct flash_partition_entry {
char *name;
uint32_t base;
uint32_t size;
};
/** Firmware layout description */
struct device_info {
const char *id;
const char *vendor;
const char *support_list;
char support_trail;
const char *soft_ver;
struct flash_partition_entry partitions[MAX_PARTITIONS+1];
const char *first_sysupgrade_partition;
const char *last_sysupgrade_partition;
};
/** The content of the soft-version structure */
struct __attribute__((__packed__)) soft_version {
uint32_t magic;
uint32_t zero;
uint8_t pad1;
uint8_t version_major;
uint8_t version_minor;
uint8_t version_patch;
uint8_t year_hi;
uint8_t year_lo;
uint8_t month;
uint8_t day;
uint32_t rev;
uint8_t pad2;
};
static const uint8_t jffs2_eof_mark[4] = {0xde, 0xad, 0xc0, 0xde};
/**
Salt for the MD5 hash
Fortunately, TP-LINK seems to use the same salt for most devices which use
the new image format.
*/
static const uint8_t md5_salt[16] = {
0x7a, 0x2b, 0x15, 0xed,
0x9b, 0x98, 0x59, 0x6d,
0xe5, 0x04, 0xab, 0x44,
0xac, 0x2a, 0x9f, 0x4e,
};
/** Firmware layout table */
static struct device_info boards[] = {
/** Firmware layout for the CPE210/220 V1 */
{
.id = "CPE210",
.vendor = "CPE510(TP-LINK|UN|N300-5):1.0\r\n",
.support_list =
"SupportList:\r\n"
"CPE210(TP-LINK|UN|N300-2):1.0\r\n"
"CPE210(TP-LINK|UN|N300-2):1.1\r\n"
"CPE210(TP-LINK|US|N300-2):1.1\r\n"
"CPE210(TP-LINK|EU|N300-2):1.1\r\n"
"CPE220(TP-LINK|UN|N300-2):1.1\r\n"
"CPE220(TP-LINK|US|N300-2):1.1\r\n"
"CPE220(TP-LINK|EU|N300-2):1.1\r\n",
.support_trail = '\xff',
.soft_ver = NULL,
.partitions = {
{"fs-uboot", 0x00000, 0x20000},
{"partition-table", 0x20000, 0x02000},
{"default-mac", 0x30000, 0x00020},
{"product-info", 0x31100, 0x00100},
{"signature", 0x32000, 0x00400},
{"os-image", 0x40000, 0x200000},
{"file-system", 0x240000, 0x570000},
{"soft-version", 0x7b0000, 0x00100},
{"support-list", 0x7b1000, 0x00400},
{"user-config", 0x7c0000, 0x10000},
{"default-config", 0x7d0000, 0x10000},
{"log", 0x7e0000, 0x10000},
{"radio", 0x7f0000, 0x10000},
{NULL, 0, 0}
},
.first_sysupgrade_partition = "os-image",
.last_sysupgrade_partition = "support-list",
},
/** Firmware layout for the CPE210 V2 */
{
.id = "CPE210V2",
.vendor = "CPE210(TP-LINK|UN|N300-2|00000000):2.0\r\n",
.support_list =
"SupportList:\r\n"
"CPE210(TP-LINK|EU|N300-2|00000000):2.0\r\n"
"CPE210(TP-LINK|EU|N300-2|45550000):2.0\r\n"
"CPE210(TP-LINK|EU|N300-2|55530000):2.0\r\n"
"CPE210(TP-LINK|UN|N300-2|00000000):2.0\r\n"
"CPE210(TP-LINK|UN|N300-2|45550000):2.0\r\n"
"CPE210(TP-LINK|UN|N300-2|55530000):2.0\r\n"
"CPE210(TP-LINK|US|N300-2|55530000):2.0\r\n"
"CPE210(TP-LINK|UN|N300-2):2.0\r\n"
"CPE210(TP-LINK|EU|N300-2):2.0\r\n"
"CPE210(TP-LINK|US|N300-2):2.0\r\n",
.support_trail = '\xff',
.soft_ver = NULL,
.partitions = {
{"fs-uboot", 0x00000, 0x20000},
{"partition-table", 0x20000, 0x02000},
{"default-mac", 0x30000, 0x00020},
{"product-info", 0x31100, 0x00100},
{"device-info", 0x31400, 0x00400},
{"signature", 0x32000, 0x00400},
{"device-id", 0x33000, 0x00100},
{"firmware", 0x40000, 0x770000},
{"soft-version", 0x7b0000, 0x00100},
{"support-list", 0x7b1000, 0x01000},
{"user-config", 0x7c0000, 0x10000},
{"default-config", 0x7d0000, 0x10000},
{"log", 0x7e0000, 0x10000},
{"radio", 0x7f0000, 0x10000},
{NULL, 0, 0}
},
.first_sysupgrade_partition = "os-image",
.last_sysupgrade_partition = "support-list",
},
/** Firmware layout for the CPE210 V3 */
{
.id = "CPE210V3",
.vendor = "CPE210(TP-LINK|UN|N300-2|00000000):3.0\r\n",
.support_list =
"SupportList:\r\n"
"CPE210(TP-LINK|EU|N300-2|45550000):3.0\r\n"
"CPE210(TP-LINK|UN|N300-2|00000000):3.0\r\n"
"CPE210(TP-LINK|UN|N300-2):3.0\r\n"
"CPE210(TP-LINK|EU|N300-2):3.0\r\n",
.support_trail = '\xff',
.soft_ver = NULL,
.partitions = {
{"fs-uboot", 0x00000, 0x20000},
{"partition-table", 0x20000, 0x01000},
{"default-mac", 0x30000, 0x00020},
{"product-info", 0x31100, 0x00100},
{"device-info", 0x31400, 0x00400},
{"signature", 0x32000, 0x00400},
{"device-id", 0x33000, 0x00100},
{"firmware", 0x40000, 0x770000},
{"soft-version", 0x7b0000, 0x00100},
{"support-list", 0x7b1000, 0x01000},
{"user-config", 0x7c0000, 0x10000},
{"default-config", 0x7d0000, 0x10000},
{"log", 0x7e0000, 0x10000},
{"radio", 0x7f0000, 0x10000},
{NULL, 0, 0}
},
.first_sysupgrade_partition = "os-image",
.last_sysupgrade_partition = "support-list",
},
/** Firmware layout for the CPE220 V2 */
{
.id = "CPE220V2",
.vendor = "CPE510(TP-LINK|UN|N300-5):1.0\r\n",
.support_list =
"SupportList:\r\n"
"CPE220(TP-LINK|EU|N300-2|00000000):2.0\r\n"
"CPE220(TP-LINK|EU|N300-2|45550000):2.0\r\n"
"CPE220(TP-LINK|EU|N300-2|55530000):2.0\r\n"
"CPE220(TP-LINK|UN|N300-2|00000000):2.0\r\n"
"CPE220(TP-LINK|UN|N300-2|45550000):2.0\r\n"
"CPE220(TP-LINK|UN|N300-2|55530000):2.0\r\n"
"CPE220(TP-LINK|US|N300-2|55530000):2.0\r\n"
"CPE220(TP-LINK|UN|N300-2):2.0\r\n"
"CPE220(TP-LINK|EU|N300-2):2.0\r\n"
"CPE220(TP-LINK|US|N300-2):2.0\r\n",
.support_trail = '\xff',
.soft_ver = NULL,
.partitions = {
{"fs-uboot", 0x00000, 0x20000},
{"partition-table", 0x20000, 0x02000},
{"default-mac", 0x30000, 0x00020},
{"product-info", 0x31100, 0x00100},
{"signature", 0x32000, 0x00400},
{"os-image", 0x40000, 0x200000},
{"file-system", 0x240000, 0x570000},
{"soft-version", 0x7b0000, 0x00100},
{"support-list", 0x7b1000, 0x00400},
{"user-config", 0x7c0000, 0x10000},
{"default-config", 0x7d0000, 0x10000},
{"log", 0x7e0000, 0x10000},
{"radio", 0x7f0000, 0x10000},
{NULL, 0, 0}
},
.first_sysupgrade_partition = "os-image",
.last_sysupgrade_partition = "support-list",
},
/** Firmwar
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
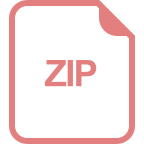
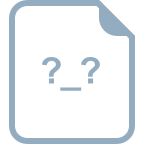
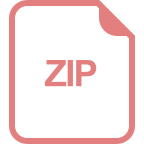
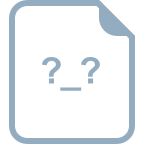
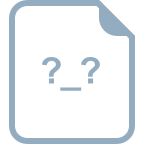
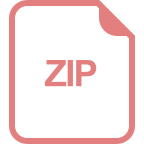
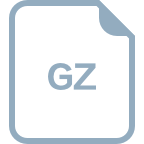
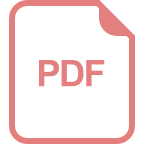
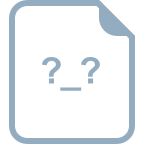
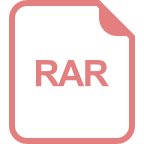
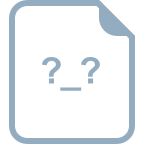
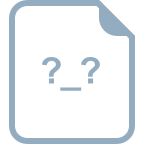
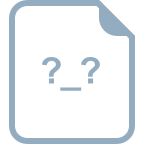
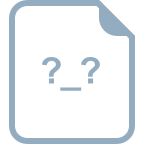
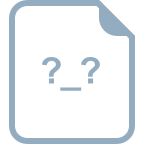
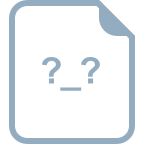
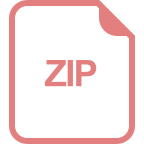
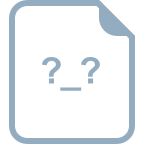
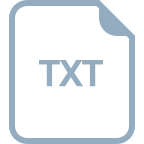
收起资源包目录

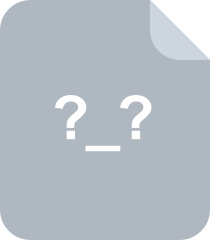
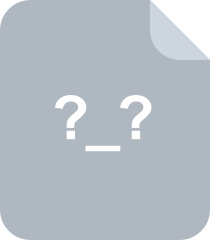
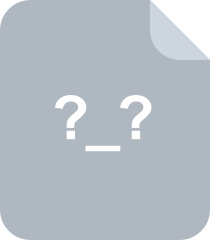
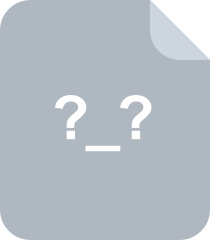
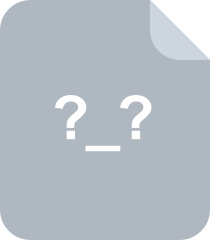
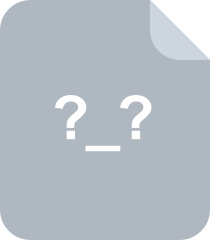
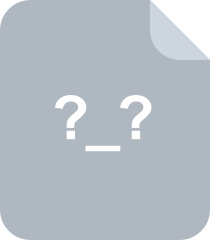
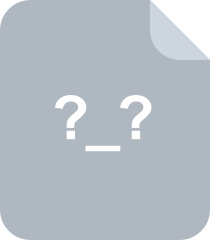
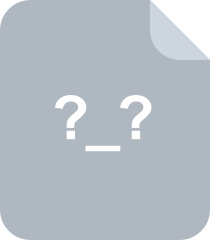
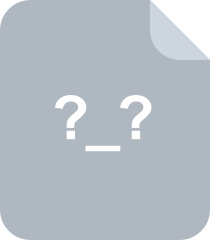
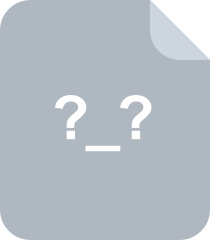
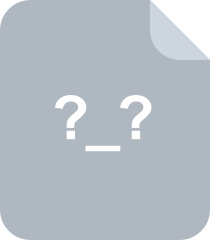
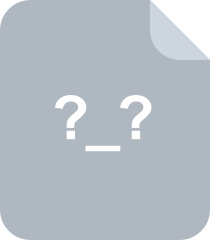
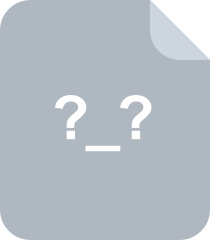
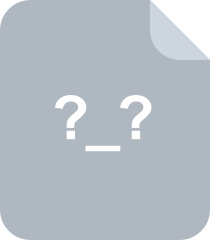
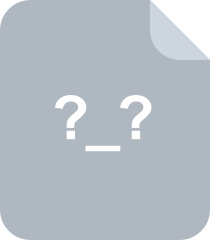
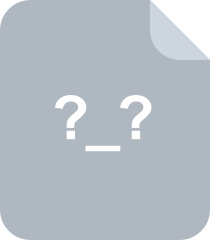
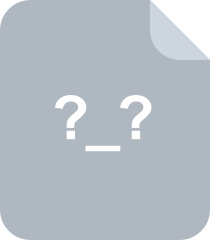
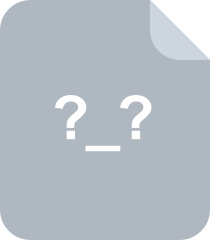
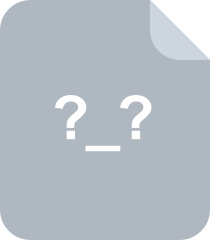
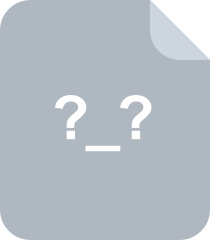
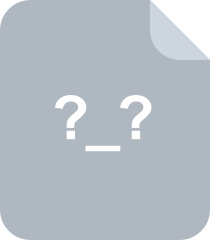
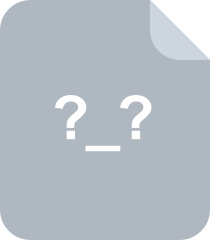
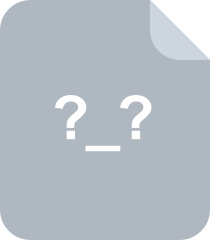
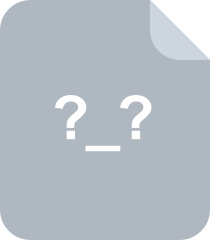
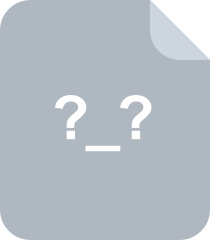
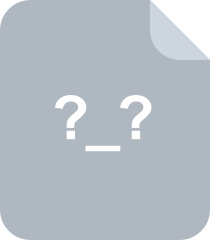
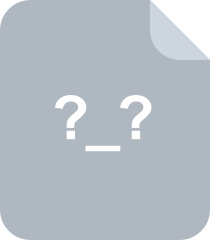
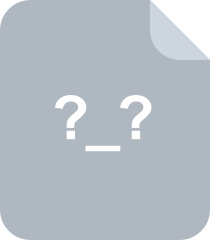
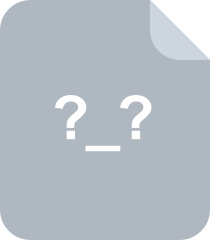
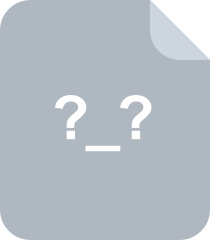
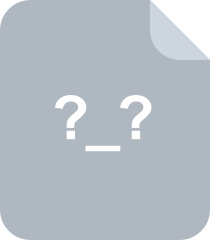
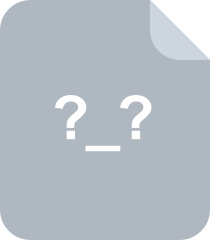
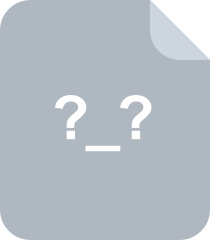
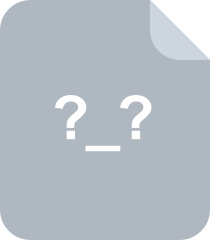
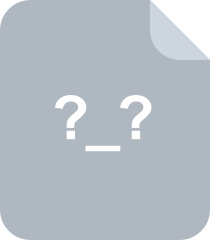
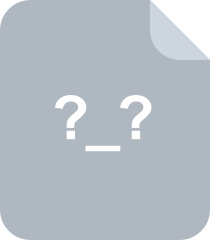
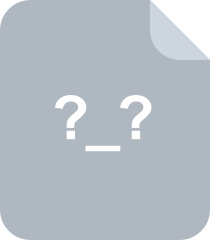
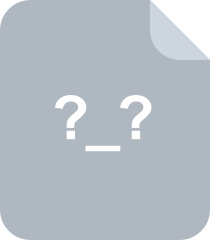
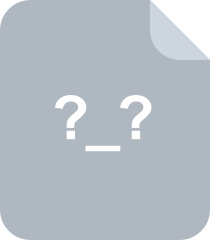
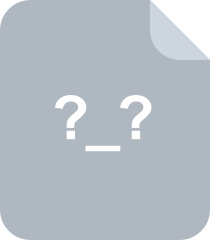
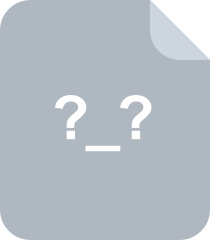
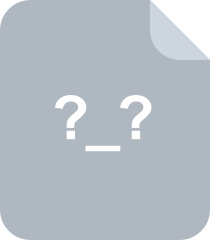
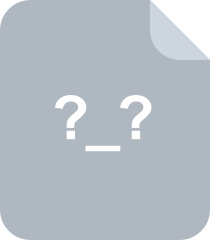
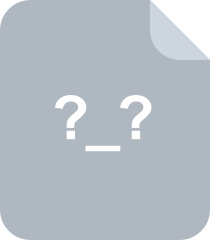
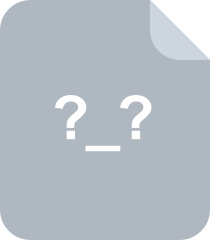
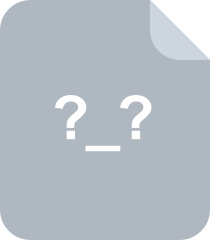
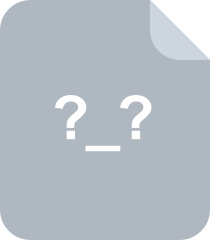
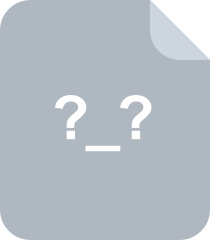
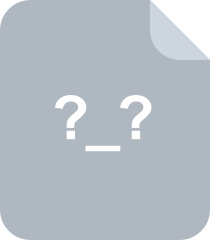
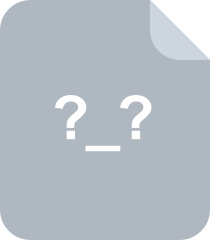
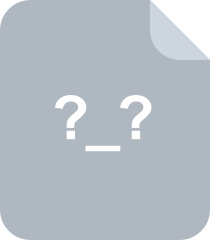
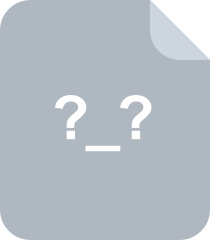
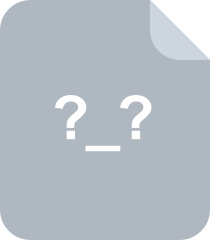
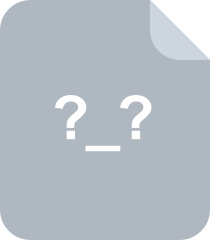
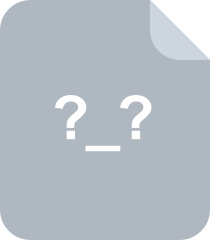
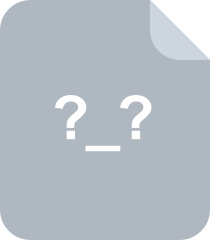
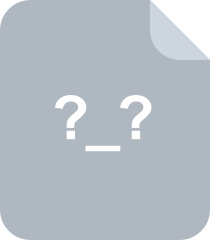
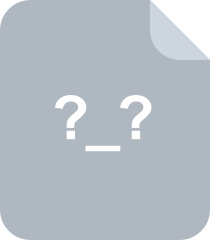
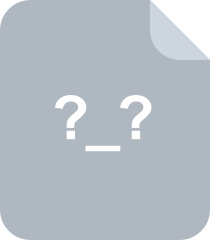
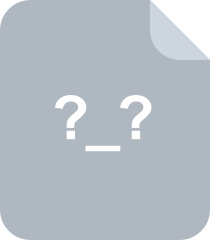
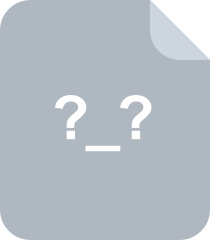
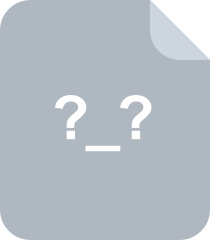
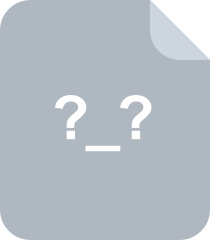
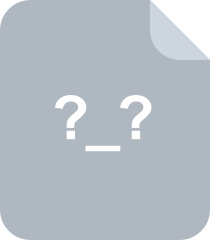
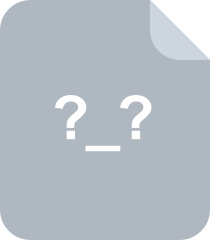
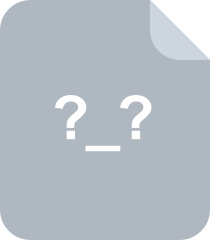
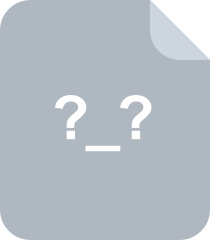
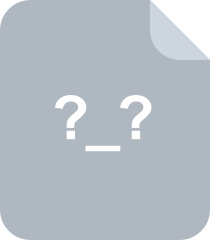
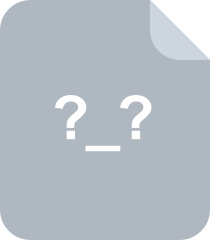
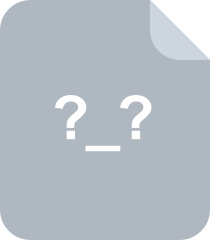
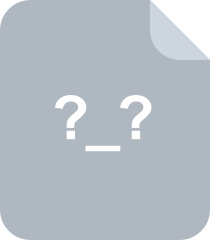
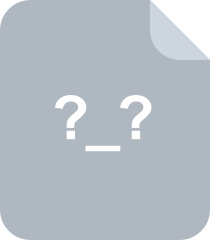
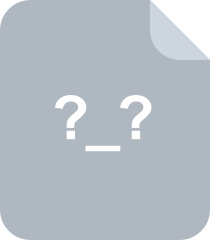
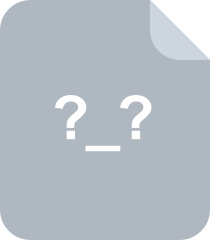
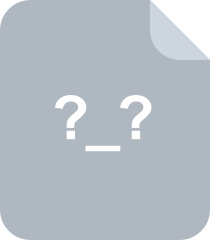
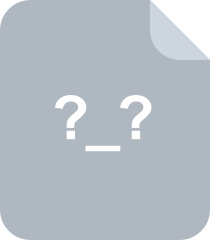
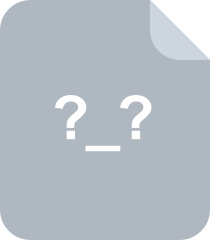
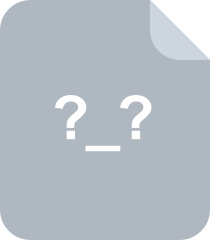
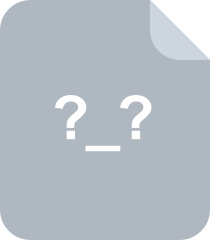
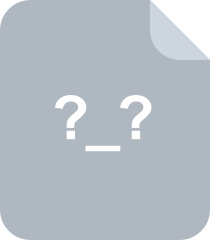
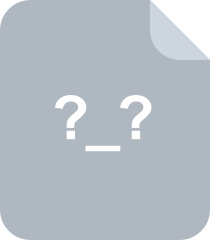
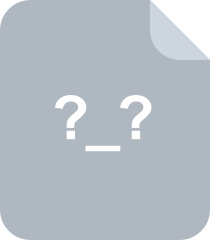
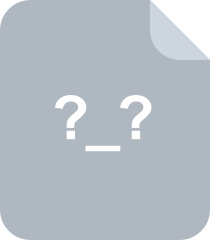
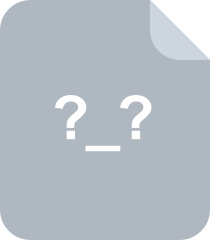
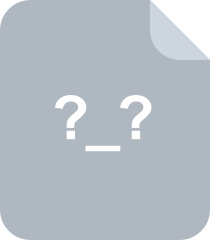
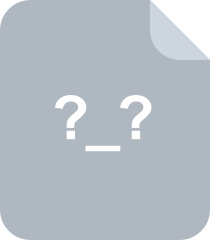
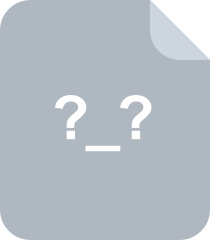
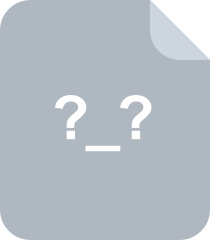
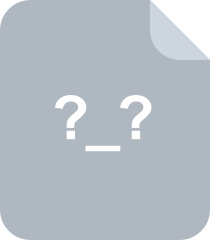
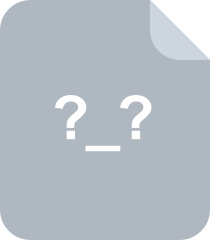
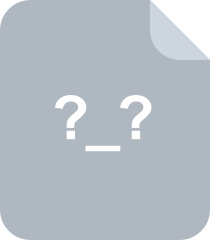
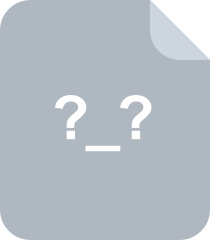
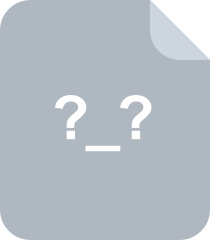
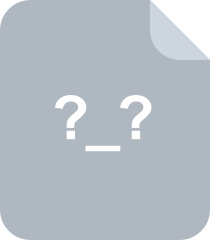
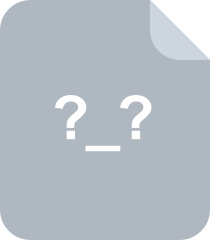
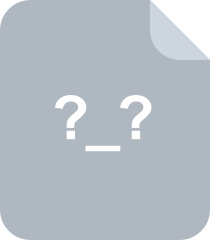
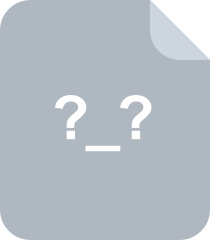
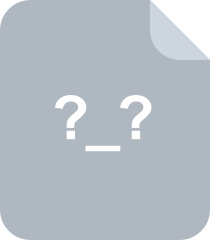
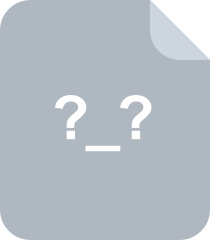
共 8947 条
- 1
- 2
- 3
- 4
- 5
- 6
- 90
资源评论
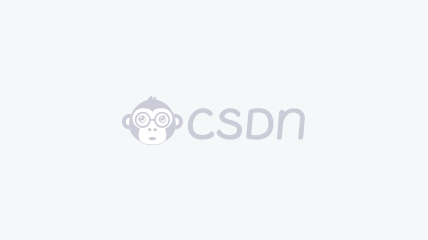

zwave
- 粉丝: 2
- 资源: 40
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

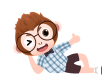
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


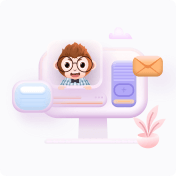
安全验证
文档复制为VIP权益,开通VIP直接复制
