Ajax登陆验证有助于更好的封装
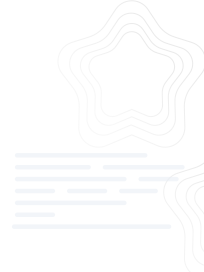

Ajax(Asynchronous JavaScript and XML)是一种在无需刷新整个网页的情况下,能够更新部分网页的技术。它通过在后台与服务器进行少量数据交换,使网页实现异步更新。这种技术的优点在于,用户可以一边浏览页面内容,一边进行其他操作,比如登录、提交表单,而无需等待整个页面重新加载。 在标题和描述中提到的"Ajax登录验证",是指利用Ajax技术来实现用户登录时的实时验证。传统的登录方式通常需要用户填写用户名和密码后点击提交按钮,然后整个页面会跳转或者刷新以显示验证结果。而使用Ajax进行登录验证,用户输入账号和密码后,这些信息会被发送到服务器进行验证,验证结果会通过Ajax回调返回,此时页面不会刷新,而是直接在当前界面显示验证状态,如成功、失败或错误提示,提供更好的用户体验。 以下是一些关于Ajax登录验证的关键知识点: 1. **异步请求**:Ajax的核心是XMLHttpRequest对象,它允许JavaScript在后台与服务器交换数据,无需用户交互。当用户填写完登录信息并触发验证时,这个对象会被用来发送HTTP请求。 2. **JSON格式**:虽然Ajax的名称中有XML,但现在更常见的数据交换格式是JSON(JavaScript Object Notation),因为它更简洁且易于处理。 3. **事件监听**:Ajax请求需要监听HTTP请求的状态,例如使用`onreadystatechange`事件来判断请求是否完成,以及请求是否成功。 4. **错误处理**:在发送Ajax请求时,需要处理可能出现的错误,例如网络问题、服务器响应错误等。这通常通过`try...catch`语句或特定的回调函数来实现。 5. **跨域问题**:由于同源策略的限制,Ajax请求通常只能向同一域名下的服务器发送。若需跨域,需要服务器端开启CORS(Cross-Origin Resource Sharing)支持。 6. **安全考虑**:在进行Ajax登录验证时,必须考虑到安全性。例如,防止CSRF(跨站请求伪造)攻击,使用HTTPS协议确保数据传输的安全,以及对敏感信息如密码进行加密处理。 7. **用户体验优化**:在等待服务器响应期间,可以使用加载动画或者提示信息让用户知道系统正在处理他们的请求,提高用户体验。 8. **前端验证与后端验证**:前端的Ajax验证只能作为初步检查,防止无效输入,但真正的安全验证应在服务器端进行,以防止恶意绕过前端验证。 9. **响应式设计**:考虑到不同设备和浏览器的兼容性,Ajax登录验证的代码应采用响应式设计,确保在各种环境下都能正常工作。 10. **框架与库的应用**:现代开发中,常使用jQuery、Vue.js、React.js等库或框架简化Ajax请求的编写,提高开发效率。 Ajax登录验证通过异步通信提高了用户交互体验,但同时也需要开发者关注安全性、兼容性和性能优化等多个方面。在实际开发中,合理运用Ajax技术和相关框架,能为用户提供更加流畅和安全的登录验证过程。

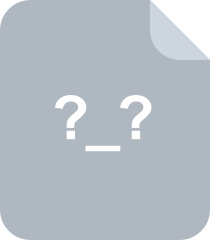
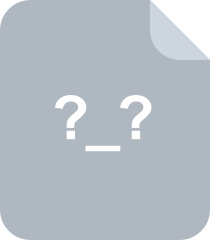
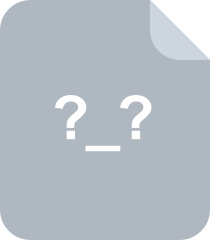
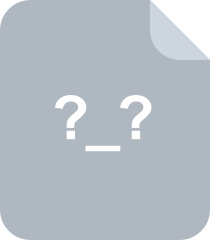
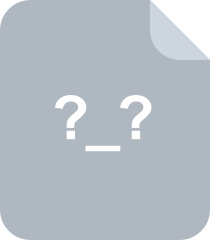
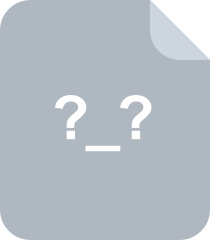
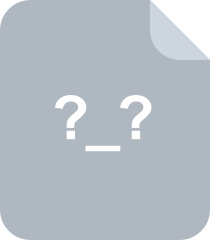
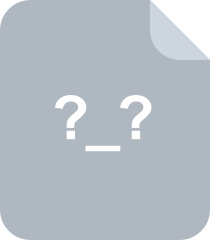
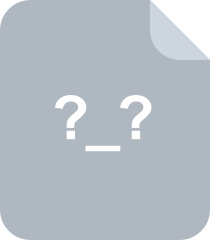
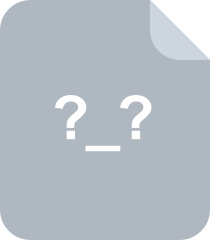
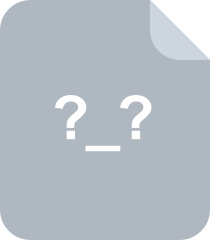
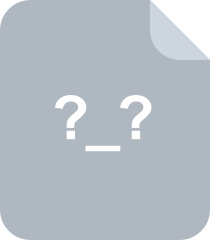
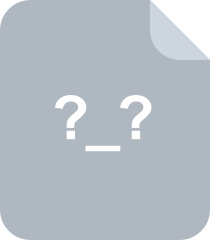
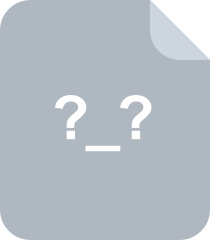
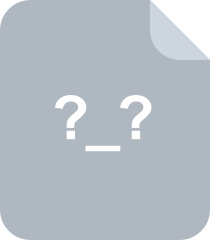
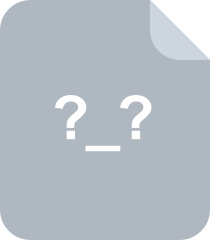
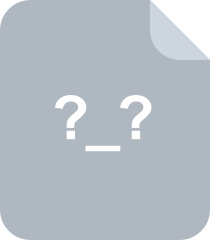
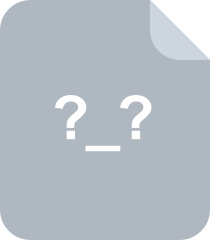
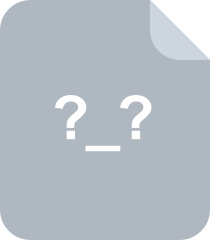
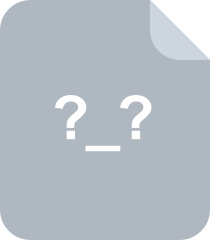
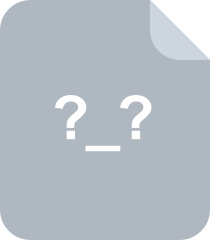
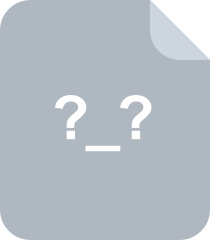
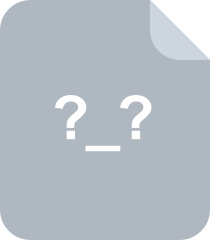
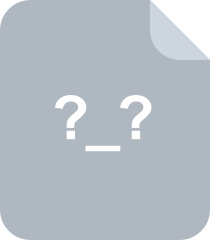
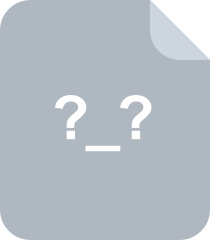
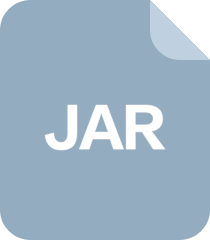
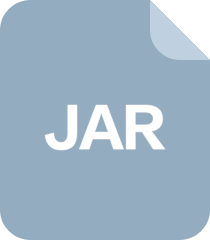
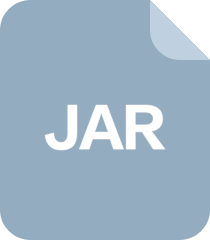
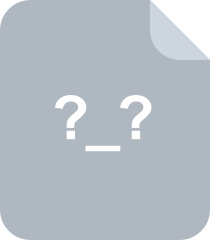
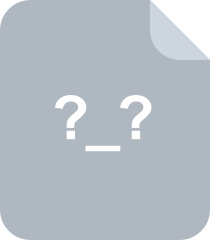
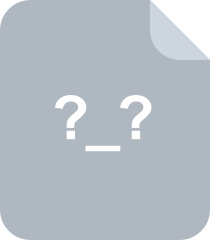
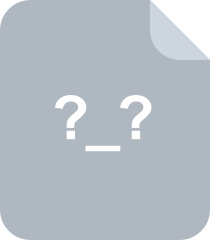
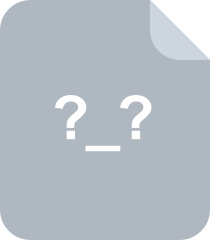
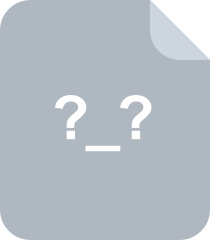
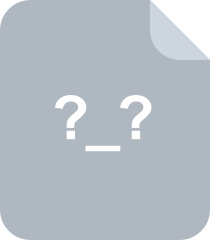
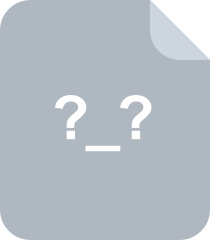
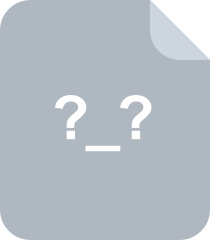
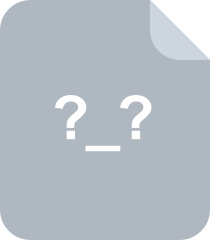
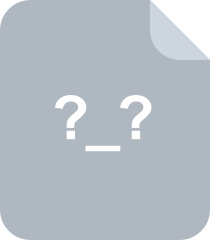
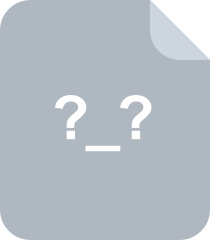
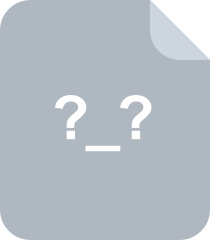
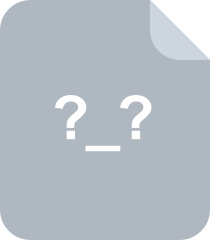
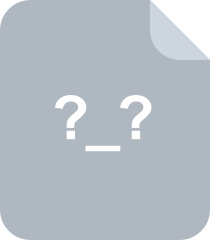
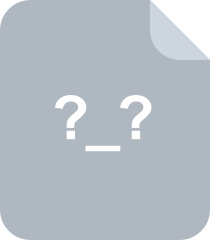
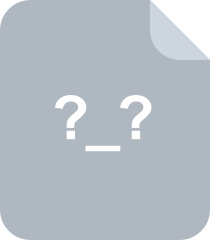
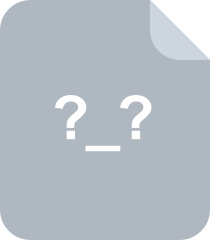
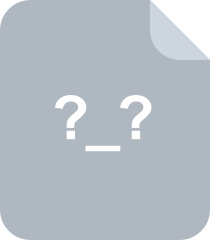
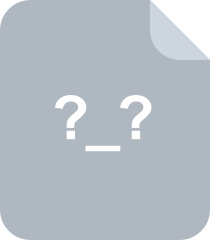
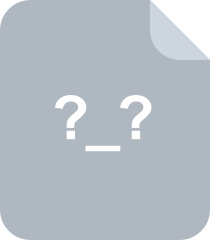
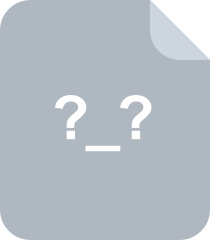
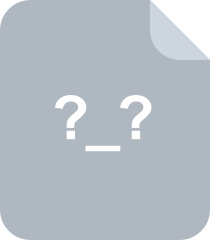
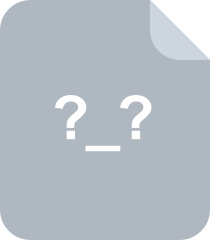
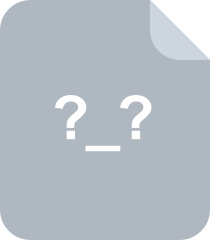
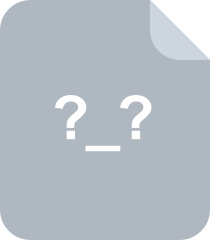
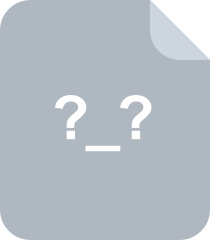
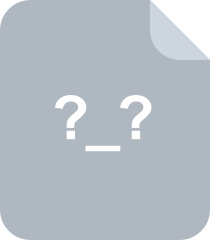
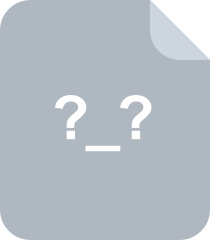
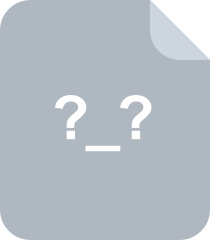
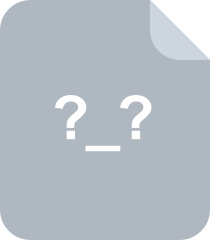
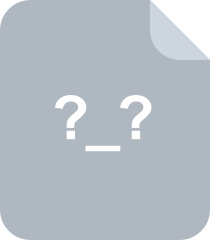
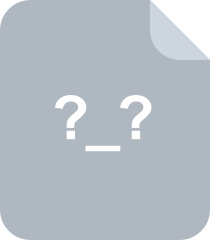
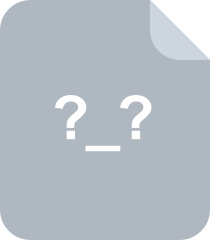
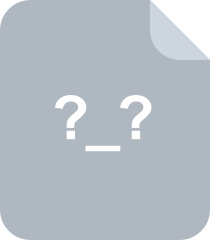
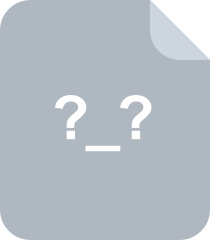
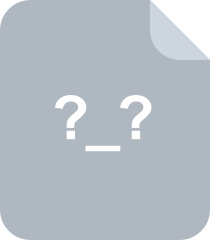
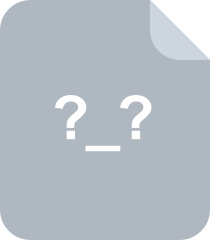
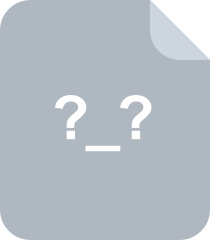
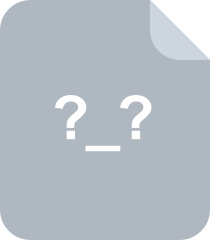
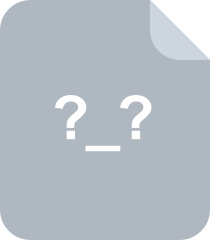
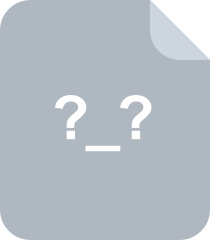
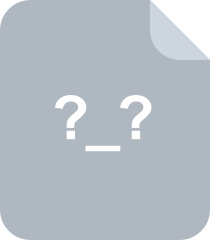
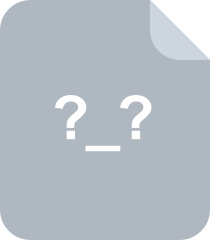
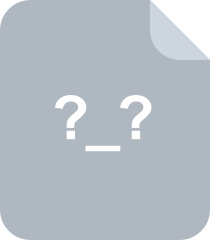
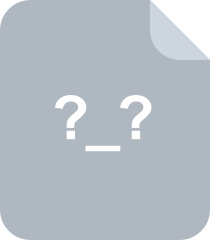
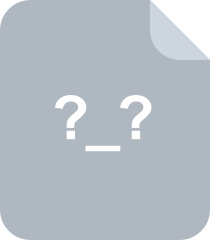
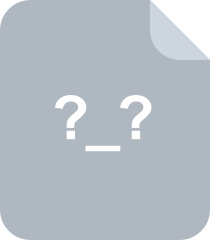
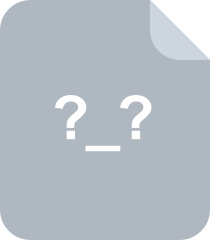
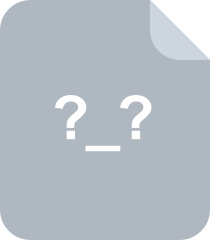
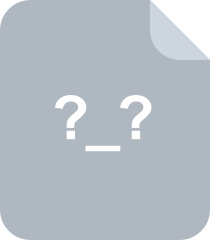
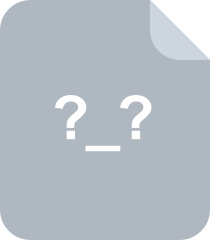
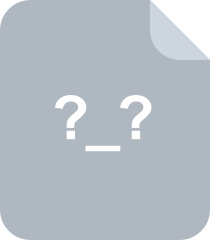
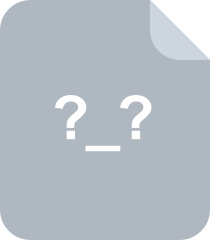
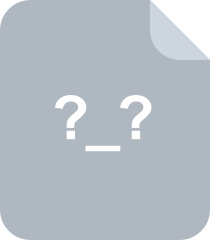
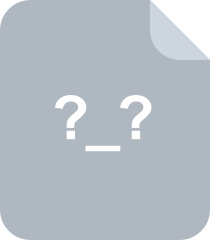
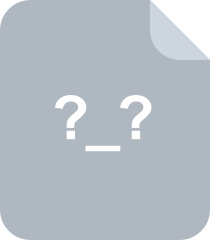
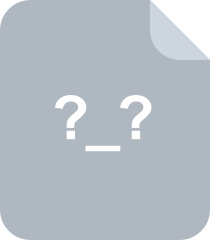
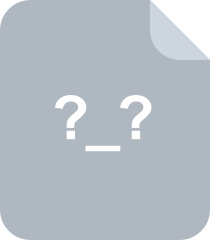
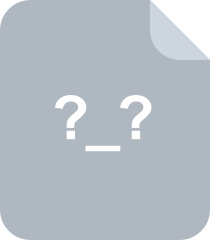
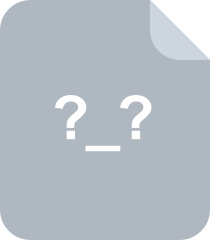
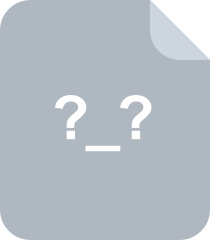
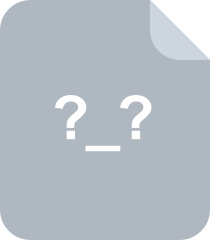
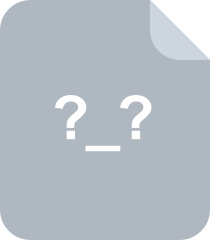
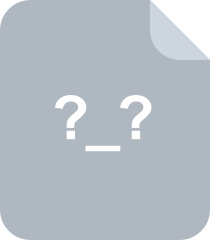
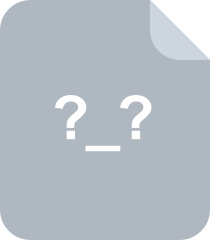
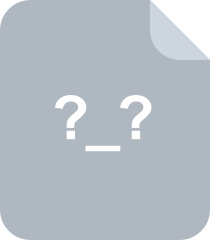
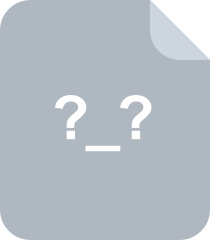
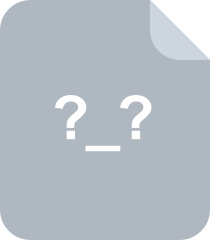
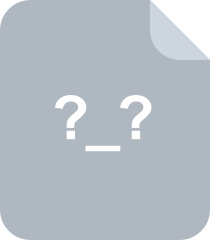
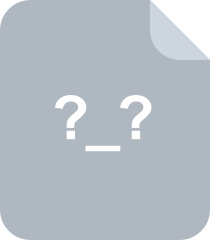
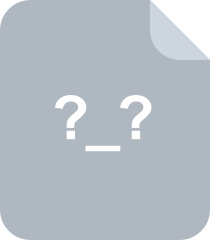
- 1
- 2
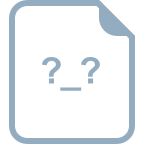
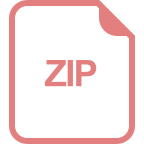
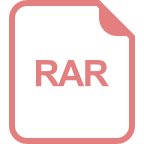
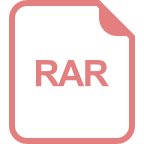
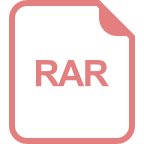
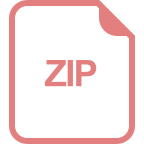
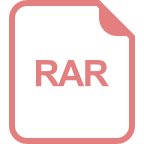
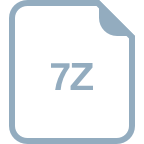
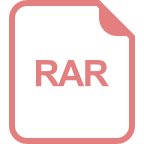
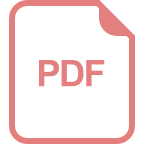
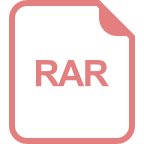
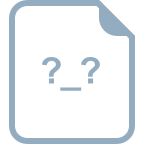
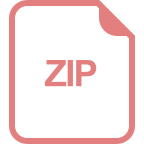
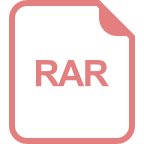
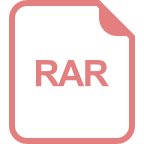
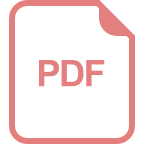
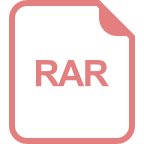
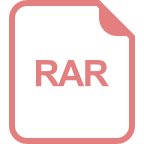
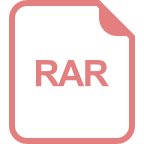
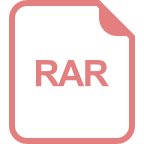
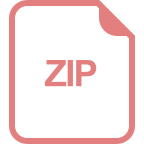
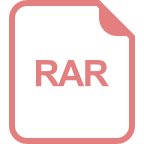
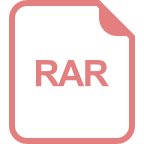
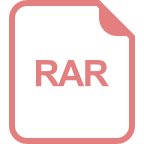
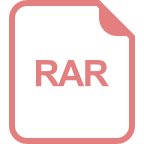
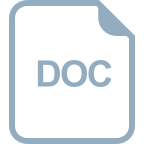
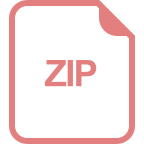
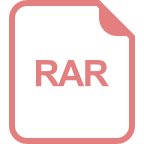

- 粉丝: 0
- 资源: 2
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

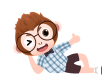
最新资源
- 毕业设计《基于Springboot+Vue+Python深度神经网络学习算法水质管理预测》+项目源码+文档说明
- PLC项目 5号卸垛机.mwp
- 基于 nodejs+SQL server 实现的学生-教师评价系统课程设计
- PLC项目程序 2号卸笼.gxw
- BZ-00-03 C008053 SAP2000 刚性连接转换
- java图书管理微信小程序源码数据库 MySQL源码类型 WebForm
- Qt QChart绘制跟随鼠标的十字线
- Baidunetdisk_AndroidPhone_1023843j-1.apk
- PLC 程序 2号卸垛AD778899.gxw
- C#ASP.NET大学在线考试系统源码数据库 SQL2008源码类型 WebForm

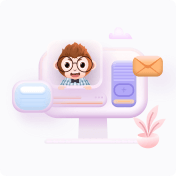

评论0