### 知识点一:Spring与Hibernate整合及配置 #### Spring XML配置文件示例 在进行Spring与Hibernate的整合时,通常需要配置Spring来管理Hibernate的SessionFactory实例,并配置数据源、事务管理器等。以下是一个基本的Spring配置文件示例: ```xml <?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd"> <!-- 配置数据源 --> <bean id="dataSource" class="org.apache.commons.dbcp.BasicDataSource" destroy-method="close"> <property name="driverClassName" value="com.mysql.jdbc.Driver"/> <property name="url" value="jdbc:mysql://127.0.0.1:3306/db1"/> <property name="username" value="username"/> <property name="password" value="password"/> </bean> <!-- Hibernate SessionFactory 配置 --> <bean id="sessionFactory" class="org.springframework.orm.hibernate5.LocalSessionFactoryBean"> <property name="dataSource" ref="dataSource"/> <property name="hibernateProperties"> <props> <prop key="hibernate.dialect">org.hibernate.dialect.MySQLDialect</prop> <prop key="hibernate.show_sql">true</prop> <prop key="hibernate.hbm2ddl.auto">update</prop> </props> </property> <property name="mappingResources"> <list> <value>table1.hbm.xml</value> </list> </property> </bean> <!-- 事务管理器配置 --> <bean id="transactionManager" class="org.springframework.orm.hibernate5.HibernateTransactionManager"> <property name="sessionFactory" ref="sessionFactory"/> </bean> <!-- 启用基于注解的事务管理 --> <tx:annotation-driven transaction-manager="transactionManager"/> </beans> ``` #### Hibernate 映射文件 (table1.hbm.xml) Hibernate映射文件用于描述Java对象与数据库表之间的映射关系。以下是`table1`的映射文件示例: ```xml <?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE hibernate-mapping PUBLIC "-//Hibernate/Hibernate Mapping DTD 3.0//EN" "http://www.hibernate.org/dtd/hibernate-mapping-3.0.dtd"> <hibernate-mapping> <class name="com.example.Table1" table="table1"> <id name="id" column="id"> <generator class="increment"/> </id> <property name="name" column="name"/> </class> </hibernate-mapping> ``` ### 知识点二:根据XML Schema生成XML文档 #### 使用XMLBeans生成JAR包 使用XMLBeans可以从XML Schema生成对应的Java类和工具类,并打包成JAR文件。生成命令如下: ```bash xjc -Xjb -d target/generated-sources -p com.example.model http://mydomain.com/myApp schema.xsd mvn compile ``` 其中`schema.xsd`是你的XML Schema文件路径。 #### Java程序示例 接下来是一个简单的Java程序示例,用于生成符合给定Schema的XML文档: ```java import org.apache.xmlbeans.XmlException; import org.apache.xmlbeans.XmlObject; import com.example.model.E1Document; public class GenerateXML { public static void main(String[] args) { try { E1Document e1 = E1Document.Factory.newInstance(); com.example.model.E1 e1Type = e1.addNewE1(); com.example.model.E1.E11 e11 = e1Type.addNewE11(); e11.setIntValue(11); com.example.model.E1.E12 e12 = e1Type.addNewE12(); e12.setE121("e121"); e12.setE122(123456789L); e1.save("output.xml", true); } catch (XmlException e) { e.printStackTrace(); } } } ``` ### 知识点三:模拟登录并获取邮件列表 #### 模拟登录并获取邮件列表 为了实现通过Web界面登录特定网站并获取邮件列表的功能,可以使用Selenium WebDriver进行页面自动化操作。以下是一个使用Selenium的Java程序示例: ```java import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class FetchEmailList { public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "path/to/chromedriver"); WebDriver driver = new ChromeDriver(); String url = "http://mail.people.com.cn/"; String username = "your_username"; String password = "your_password"; driver.get(url); WebElement loginLink = driver.findElement(By.linkText("登录")); loginLink.click(); WebElement usernameField = driver.findElement(By.name("username")); WebElement passwordField = driver.findElement(By.name("password")); usernameField.sendKeys(username); passwordField.sendKeys(password); WebElement loginButton = driver.findElement(By.id("login_button")); loginButton.click(); // 等待页面加载完成 Thread.sleep(5000); WebElement inboxLink = driver.findElement(By.linkText("收件箱")); inboxLink.click(); // 获取邮件标题列表 List<WebElement> emailTitles = driver.findElements(By.className("email_title")); for (WebElement title : emailTitles) { System.out.println(title.getText()); } driver.quit(); } } ``` ### 知识点四:使用POP3协议收取Gmail邮件 #### 使用POP3协议收取Gmail邮件 为了定期从Gmail账户收取邮件,可以使用JavaMail API结合POP3协议实现这一功能。以下是一个简单的Java程序示例: ```java import javax.mail.*; import javax.mail.internet.InternetAddress; import javax.mail.internet.MimeMessage; import java.util.Properties; public class FetchGmail { public static void main(String[] args) { String username = "your_email@gmail.com"; String password = "your_password"; Properties props = new Properties(); props.put("mail.store.protocol", "pop3s"); props.put("mail.pop3s.host", "pop.gmail.com"); props.put("mail.pop3s.port", "995"); props.put("mail.pop3s.starttls.enable", "true"); Session session = Session.getDefaultInstance(props, new Authenticator() { protected PasswordAuthentication getPasswordAuthentication() { return new PasswordAuthentication(username, password); } }); Store store = null; try { store = session.getStore(); store.connect(); Folder inbox = store.getFolder("INBOX"); inbox.open(Folder.READ_ONLY); Message[] messages = inbox.getMessages(); for (int i = 0; i < messages.length; i++) { MimeMessage message = (MimeMessage) messages[i]; System.out.println("Subject: " + message.getSubject()); } inbox.close(false); store.close(); } catch (MessagingException e) { e.printStackTrace(); } finally { if (store != null) { try { store.close(); } catch (MessagingException e) { e.printStackTrace(); } } } } } ``` ### 总结 本文介绍了四个与IT相关的知识点:Spring与Hibernate的整合配置、根据XML Schema生成XML文档、模拟登录并获取邮件列表以及使用POP3协议收取Gmail邮件。这些知识点涵盖了企业级应用开发中的常见场景和技术,对于深入理解和掌握Java开发具有重要意义。
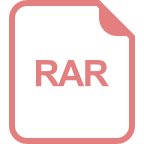
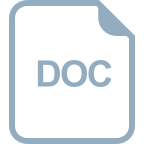
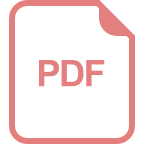
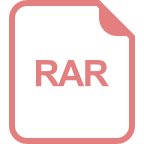
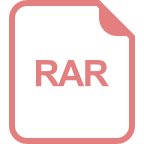
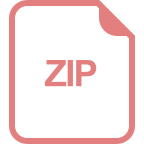
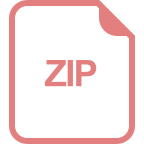
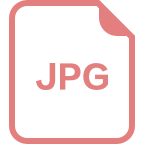
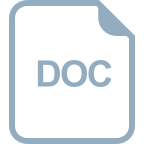
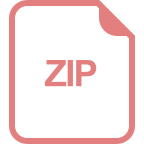
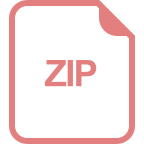
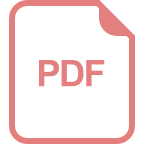
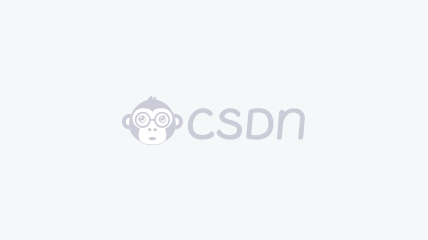

- 粉丝: 16
- 资源: 8
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

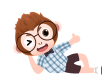
最新资源

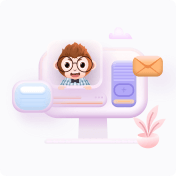
