<%@page language="java" import="java.io.*,java.sql.*,java.util.*" %>
<%
/**
* Caption: Zaval Database Front-end
* $Revision: 1.51 $
* $Date: 2002/03/13 14:30:17 $
*
* @author: Alexander Ivanyukovich
* @version: 1.2
*
* The Zaval Database Front-end provides end users with a light database
* query tool for databases that have JDBC-compliant drivers using Web
* interface. This tool is dedicated for software developers and
* database administrators.
*
* For more info on this product read Zaval Database Front-End User's Guide
* (It comes within this package).
* The latest product version is always available from the product's homepage:
* http://www.zaval.org/products/db-front-end/
* and from the SourceForge:
* http://sourceforge.net/projects/zaval0000/
*
* Contacts:
* Support : support@zaval.org
* Change Requests : change-request@zaval.org
* Feedback : feedback@zaval.org
* Other : info@zaval.org
*
* Copyright (C) 2001-2002 Zaval Creative Engineering Group (http://www.zaval.org)
*
* This program is free software; you can redistribute it and/or
* modify it under the terms of the GNU General Public License
* (version 2) as published by the Free Software Foundation.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
*
*/
/**
* File name 'index.jsp' I chosen for this tool is not always appropriate,
* so you can change it. After file renaming change the variable value.
*/
String file = new String("index.jsp");
/**
* In most databases you can execute several queries as one.
* But there are databases that do not allow this functionality.
* To make life easier we just parse input query and take it to pieces.
* Each subquery is executed separately.
* queryDelimiter constant is a delimiter symbol between subqueries.
*/
char queryDelimiter = ';';
/**
* Usually, transaction is roll backed on any failure during queries execution.
* If you want to change this behavior just set it to false.
*/
boolean rollbackOnFailure = true;
/**
* To set browser encoding (via <meta>) we use pageEncoding array.
*/
String [] pageEncodings = new String[]{"ISO-8859-1", "Windows-1251"};
/**
* While experimenting with various jdbc drivers we faced with a problem of national characters suport.
* The most flexible way is to allow user choose the appropriate encodings - both database internal and actual.
* The first encoding is a default one.
*/
String [] javaEncodings = new String[]{"ISO-8859-1", "US-ASCII", "UTF-8", "UTF-16", "Cp1251"};
/**
* Transaction status (if supported).
*/
String [] transactionStatus = new String[]{"commit", "accumulation", "rollback"};
String query = new String("");
int totalParams = 12; //number of fields to expect.
HashMap hash = new HashMap(totalParams, (float)1.0);
byte [] tempArr = null;
if(session.getValue("connection") != null){
int length = request.getContentLength();
int nameStart = "Content-Disposition: form-data; name=\"".length();
int i = 0, j = 0, k = 0, tmp = 0;
InputStream is = new BufferedInputStream(request.getInputStream(), length);
byte [] arr = new byte[length];
while(i < length){
tmp = is.read(arr, i, length);
i += tmp; //i store current position(total bytes read)
length -= tmp;
}
String tempStr = new String(arr);
j = tempStr.indexOf('\n') - 1;
String separator = tempStr.substring(0, j);
i = tempStr.indexOf(separator, j);//search end position
while(i != -1 && k < totalParams){
tmp = tempStr.indexOf('\n', j+2); //skiping '\n\n' after Content-Disposition string
if(tempStr.charAt(tempStr.indexOf("\"", j+nameStart+2)+1) == ';'){ //is it a file ?
tmp = tempStr.indexOf('\n', tmp+1) + 2; //skiping Content-Type string + '\n\n'
tempArr = new byte[i-tmp-3];
System.arraycopy(arr, tmp+1, tempArr, 0, i-tmp-3); //skiping trailing '\n\n'
}else{
hash.put(tempStr.substring(j+nameStart+2, tempStr.indexOf("\"", j+nameStart+2)), tempStr.substring(tmp+1, i-2).trim()); //property's name/value
}
j = i + separator.length();
i = tempStr.indexOf(separator, i+1);
k++;
}
}
/**
* Variables initialization.
* dbEnc - is an internal database encoding.
* outEnc - is an internal java encoding we used to represent String object.
* pageEnc - is a browser encoding (we set it via <meta> tag).
* showResults - indicates query results status.
* saveInput - indicates query string status after request.
* parseQuery - by default query devided into subqueries and they executes one-by-one.
* If they are not specified set them to default values.
*/
if(hash.get("dbEnc") == null)
hash.put("dbEnc", javaEncodings[0]);
if(hash.get("outEnc") == null)
hash.put("outEnc", javaEncodings[0]);
if(hash.get("pageEnc") == null)
hash.put("pageEnc", pageEncodings[0]);
if(hash.get("source") == null)
hash.put("source", "0");
if(hash.get("transactionStatus") == null)
hash.put("transactionStatus", transactionStatus[0]);
%>
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.0 Transitional//EN">
<html>
<head>
<title>Zaval Database Front-end</title>
<meta name="description" content="Zaval Database Front-end (http://www.zaval.org)" />
<meta http-equiv="Content-Type" content="text/html; charset=<%= (String)hash.get("pageEnc") %>" />
<style>
.login { font-family: Verdana, Arial, Helvetica, sans-serif; font-size: 8pt; color: #666666; width:320px; }
.header { font-family:Verdana, Arial, Helvetica, sans-serif; font-size: 10pt; color: #666666; font-weight: bold; }
.tableHeader { background-color: #c0c0c0; color: #666666;}
.tableHeaderLight { background-color: #cccccc; color: #666666;}
.main { font-family:Verdana, Arial, Helvetica, sans-serif; font-size: 8pt; color: #666666;}
.copy { font-family:Verdana, Arial, Helvetica, sans-serif; font-size: 8pt; color: #999999;}
.copy:Hover { color: #666666; text-decoration : underline; }
td { font-family:Verdana, Arial, Helvetica, sans-serif; font-size: 8pt; color: #666666;}
A { text-decoration: none; }
A:Hover { color : Red; text-decoration : underline; }
BODY { OVERFLOW: auto; font-family:Verdana, Arial, Helvetica, sans-serif; font-size: 8pt; color: #666666; }
</style>
<script language="JavaScript">
function completeFields(val, id){
document.forms[0].elements[id].value = val;
}
</script>
</head>
<body bgcolor="#FFFFFF">
<center>
<h2>Zaval Database Front-end</h2>
</center>
<%
// we store connection in the session, so we cut connection expences down.
if(session.getValue("connection") == null){
//lets check whether user passed to script url for the connection otherwise show login page.
if(request.getParameter("url") == null || request.getParameter("url").toString().trim().length() == 0 || request.getParameter("url").toString().trim().equalsIgnoreCase("jdbc:odbc:")){
%>
<center>
<table border="0" cellpadding="0" cellspacing="0">
<form action="<%= file %>" method="post">
<tr>
<td align="right">Driver :</td>
<td align="right"> </td>
<td align="left" colspan="2"><input class="login" type="Text" name="dri

zrh1179767
- 粉丝: 1
- 资源: 2
最新资源
- base(1).apk.1
- K618977005_2012-12-6_beforeP_000.txt.PRM
- 秋招信息获取与处理基础教程
- 程序员面试笔试面经技巧基础教程
- Python实例-21个自动办公源码-数据处理技术+Excel+自动化脚本+资源管理
- 全球前8GDP数据图(python动态柱状图)
- 汽车检测7-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- 检测高压线电线-YOLO(v5至v9)、COCO、Darknet、VOC数据集合集.rar
- 检测行路中的人脸-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、VOC数据集合集.rar
- Image_17083039753012.jpg
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


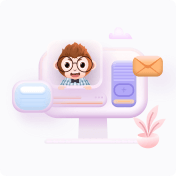